Зміст курсу
Building a Classic Snake Game
The Snake Creation
Now that we've laid the groundwork for our fruit-eating digital serpent, it's time to bring our slithering friend to life. Here what we will work on:
Body Segments | The snake will consist of body segments, forming its length. |
Movement | It will be able to move across the game area in four directions: up, down, left and right. |
Growth | When consuming a piece of fruit, the snake will increase in length by adding a new body segment. |
Collision Detection | We need to detect collisions between the snake's head and other game elements, such as walls or itself. |
As you can see there is a lot things to do but firstly we need to think what properties our snake should have based on these characteristics the basic things that comes to mind are:
- field: The game field where the snake will move.
- sprite: The sprite image representing the snake.
- positions: An array that stores all positions of body segments.
- length: The initial length of the snake (default is 2).
- direction: Current direction of the snake.
Attributes
Passed attributes
The field
and sprite
and positions
are the same concept as in a Fruit
class, except 'positions' now represents an array containing the individual positions of each body segment of the snake.
Directions
Before delving into our snake class, you'll spot some constantsˀ representing tuples. These tuples represent the directions the snake can move in. Inside the constructor, make sure to give our snake a directions attribute, set to the default direction of RIGHT
.
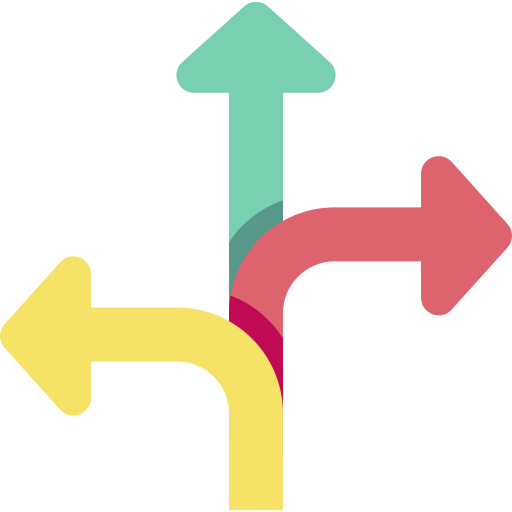
Our game field is represented as a tuple with 'x' and 'y' attributes. For instance, (0, 0) denotes the upper left corner. To move one block to the right, we need to increase the 'x' coordinate, resulting in (1, 0). We explore it more in future chapter.
Body segments
To represent the body segments, we'll keep track of all positions in a list as tuples. This way, it's easy to update each position and add new segments as required, making the process more manageable and flexible.
Note
The positions variable should be an array of tuples, with each tuple representing a position occupied by the snake. Its length should correspond to the length of the snake. You can initialize it like this:
self.positions = [(0, 0)] * length
.
With this initialization, our Snake object is equipped with the necessary attributes.
Swipe to start coding
- Create a Snake class.
- Add a constructor that will take a game
field
,sprite
, and initiallength
with a default value as parameters. - Add
positions
anddirection
attribute inside__init__
.
Рішення
Дякуємо за ваш відгук!