Try-catch structure
How to Handle Exceptions
From the previous chapter, you may have understood that exceptions completely halt the program's execution. Such an outcome is not desirable for us because we need the program to continue running without crashing.
Simply removing exceptions is not an option, as in that case, the program would work incorrectly. Therefore, we need to learn how to handle errors using the try-catch
structure.
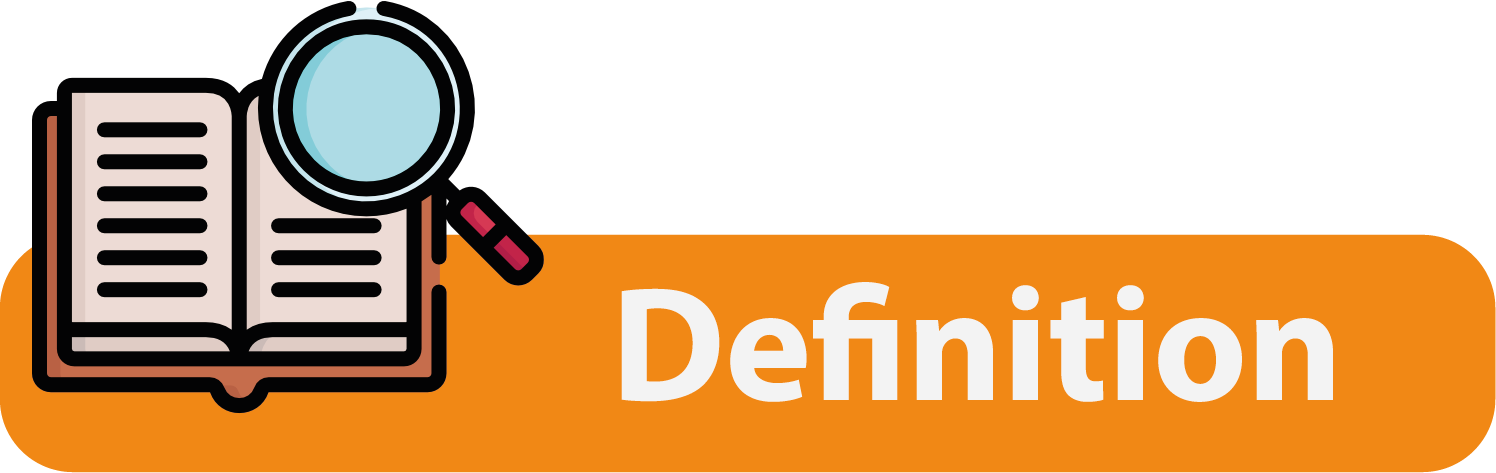
try-catch
structure is a mechanism for catching and handling exceptions, ensuring that the program execution flow can continue even after an error occurs.
Let's take a look at the syntax of such a structure and understand how it captures errors:
try
Block: This block contains the code that might generate an exception. During execution, if any statement within thetry
block throws an exception, the rest of the block's code is skipped, and the control moves to the correspondingcatch
block(s) to handle that exception;catch
Block: This block is used to handle the exception. It must follow thetry
block. If the type of exception thrown matches the type declared in thecatch
block, the code inside this block is executed. There can be multiple catch blocks associated with a singletry
block, each catching and handling different types of exceptions.
In simpler terms, we attempt to execute the code, preparing to catch an exception.
If we catch an exception, the code execution doesn't stop but continues with the operation specified in the catch
block. If there's no exception, the operation is executed successfully without entering the catch
block.
Handling ArithmeticException
In the previous chapter, we implemented a division method that throws an arithmetic exception in case of division by zero. Here's what this method looks like:
Now, let's handle this exception when calling the method using a try-catch structure:
main.java
As you can see, we attempt to perform division by zero, but we do it within the try
block. In the catch
block, we catch the ArithmeticException
and give it an alias "e
" so that we can use this object in the future (remember, exceptions are objects, right?).
This way, the exception doesn't halt the program's execution since we've handled it. Now, in the event of an exception being thrown, the program notifies the user with console output and continues its execution.
Все було зрозуміло?
Зміст курсу
Java JUnit Library. Types of Testing
Java JUnit Library. Types of Testing
Try-catch structure
How to Handle Exceptions
From the previous chapter, you may have understood that exceptions completely halt the program's execution. Such an outcome is not desirable for us because we need the program to continue running without crashing.
Simply removing exceptions is not an option, as in that case, the program would work incorrectly. Therefore, we need to learn how to handle errors using the try-catch
structure.
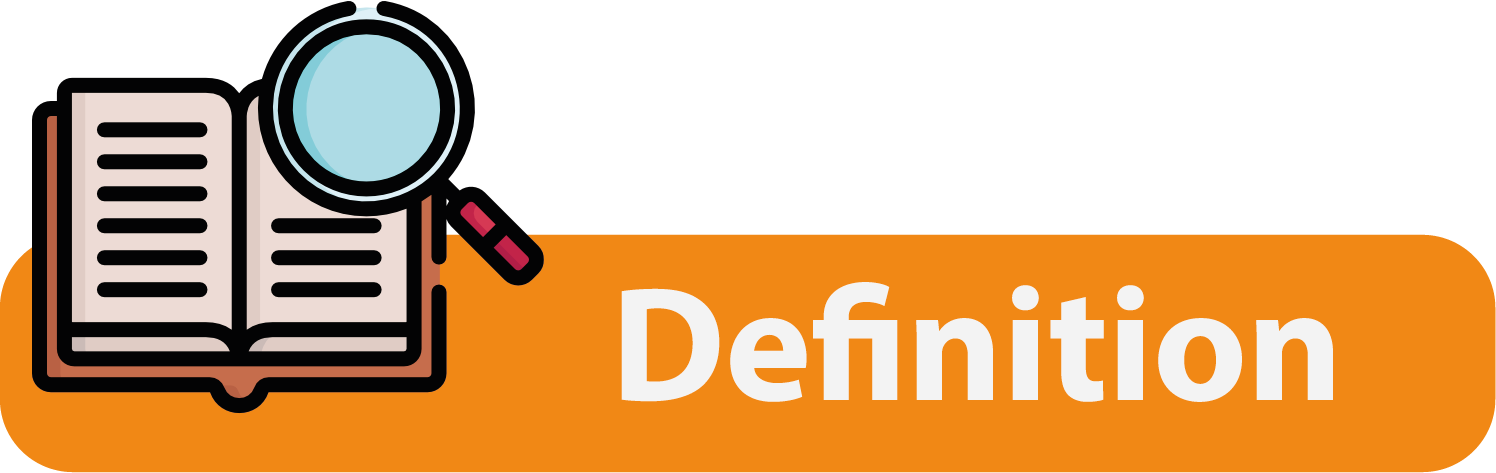
try-catch
structure is a mechanism for catching and handling exceptions, ensuring that the program execution flow can continue even after an error occurs.
Let's take a look at the syntax of such a structure and understand how it captures errors:
try
Block: This block contains the code that might generate an exception. During execution, if any statement within thetry
block throws an exception, the rest of the block's code is skipped, and the control moves to the correspondingcatch
block(s) to handle that exception;catch
Block: This block is used to handle the exception. It must follow thetry
block. If the type of exception thrown matches the type declared in thecatch
block, the code inside this block is executed. There can be multiple catch blocks associated with a singletry
block, each catching and handling different types of exceptions.
In simpler terms, we attempt to execute the code, preparing to catch an exception.
If we catch an exception, the code execution doesn't stop but continues with the operation specified in the catch
block. If there's no exception, the operation is executed successfully without entering the catch
block.
Handling ArithmeticException
In the previous chapter, we implemented a division method that throws an arithmetic exception in case of division by zero. Here's what this method looks like:
Now, let's handle this exception when calling the method using a try-catch structure:
main.java
As you can see, we attempt to perform division by zero, but we do it within the try
block. In the catch
block, we catch the ArithmeticException
and give it an alias "e
" so that we can use this object in the future (remember, exceptions are objects, right?).
This way, the exception doesn't halt the program's execution since we've handled it. Now, in the event of an exception being thrown, the program notifies the user with console output and continues its execution.
Все було зрозуміло?