while
Loop Types
Loops are structures that repeatedly execute a code block while a specific condition is true. In JavaScript, there are three types of loops:
- while;
- do-while;
- for.
Let's explore each of them in detail.
while Loop
The while
loop is the simplest type of loop. It continues to execute a code block as long as the specified condition remains true.
To create a while
loop, use the while
keyword followed by a condition in parentheses and enclose the code block in curly braces. The syntax is similar to that of an if
statement:
Here's an example:
In this example, the while
loop's code block executes six times:
- Initially, the variable
a
is set to5
; - The loop condition is
a <= 10
, which is true, so the code block is executed; - After each execution, the variable
a
is incremented by 1; - The loop continues to execute as long as the condition remains true.
Note
The
while
loop checks the condition before executing the code block. If the condition is initiallyfalse
, the code block will not be executed.
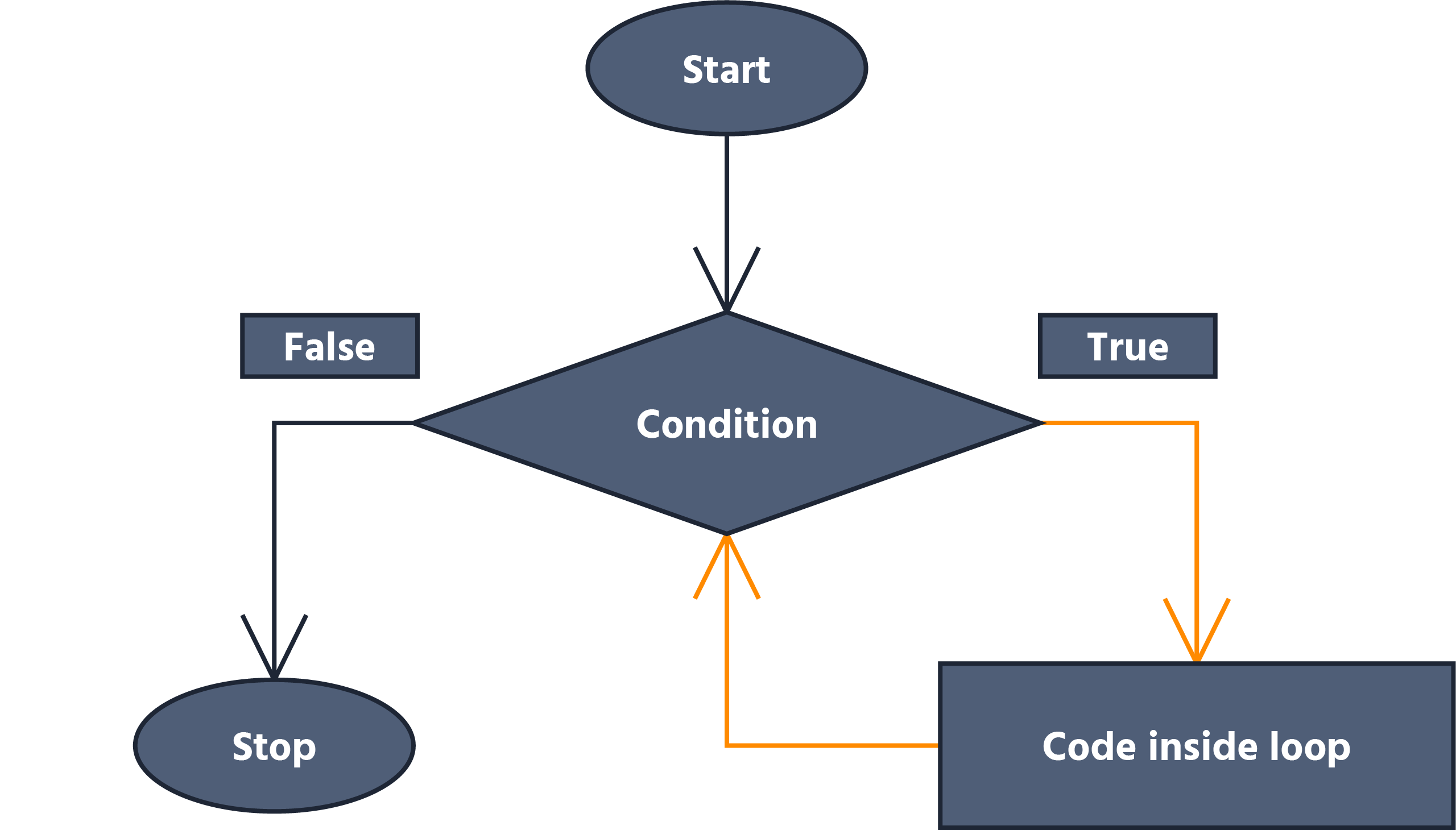
Note
Be cautious with the condition! If the condition always evaluates to
true
, the loop will never break, resulting in an infinite loop, which can cause your program to hang or become unresponsive.
Everything was clear?
Course Content
Introduction to JavaScript
Introduction to JavaScript
4. Conditional Statements
while
Loop Types
Loops are structures that repeatedly execute a code block while a specific condition is true. In JavaScript, there are three types of loops:
- while;
- do-while;
- for.
Let's explore each of them in detail.
while Loop
The while
loop is the simplest type of loop. It continues to execute a code block as long as the specified condition remains true.
To create a while
loop, use the while
keyword followed by a condition in parentheses and enclose the code block in curly braces. The syntax is similar to that of an if
statement:
Here's an example:
In this example, the while
loop's code block executes six times:
- Initially, the variable
a
is set to5
; - The loop condition is
a <= 10
, which is true, so the code block is executed; - After each execution, the variable
a
is incremented by 1; - The loop continues to execute as long as the condition remains true.
Note
The
while
loop checks the condition before executing the code block. If the condition is initiallyfalse
, the code block will not be executed.
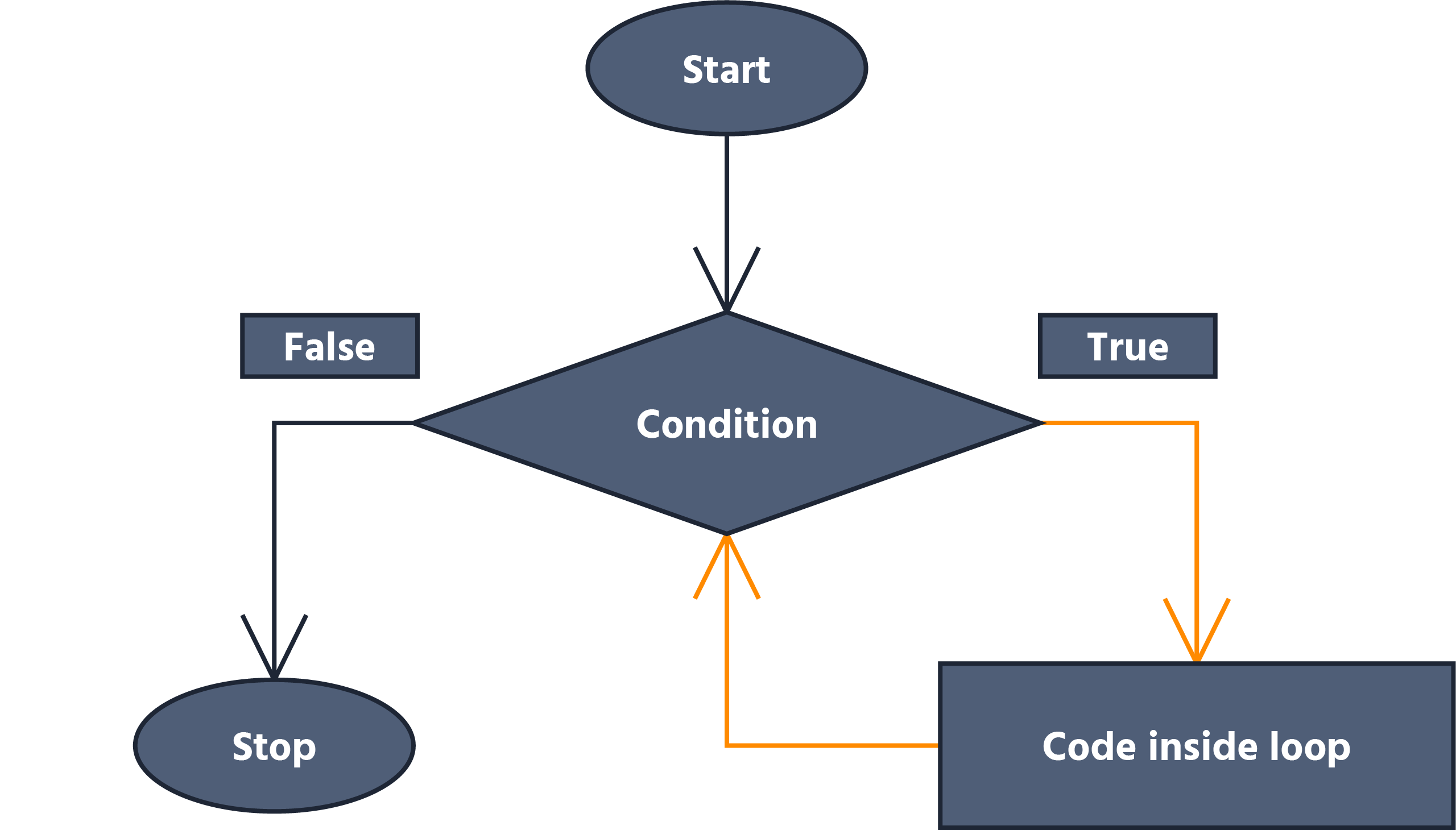
Note
Be cautious with the condition! If the condition always evaluates to
true
, the loop will never break, resulting in an infinite loop, which can cause your program to hang or become unresponsive.
Everything was clear?