Switch-Case Statement
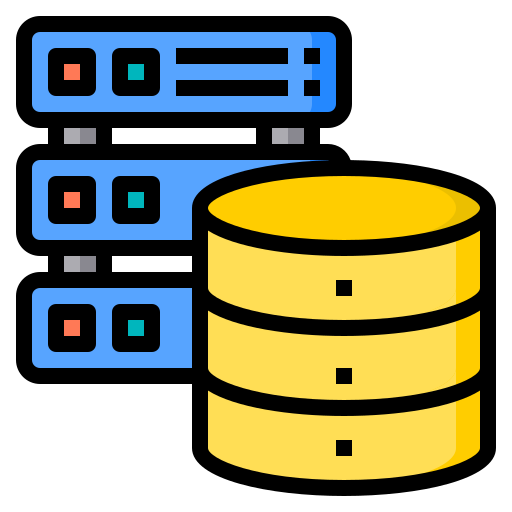
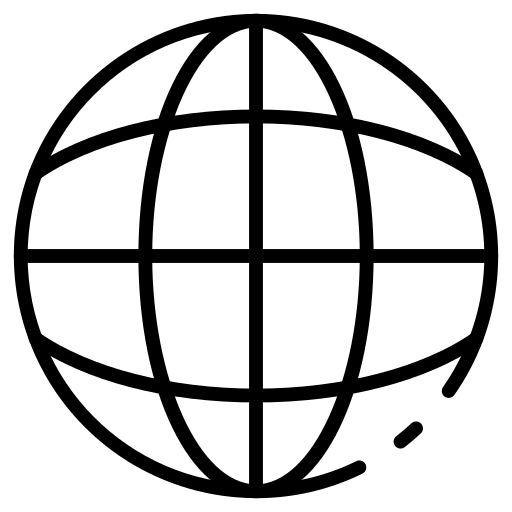
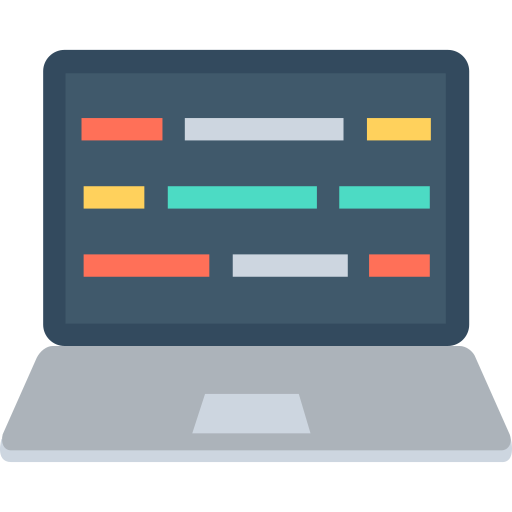
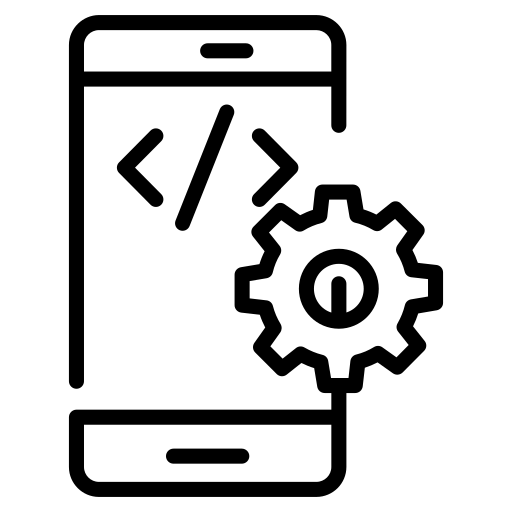
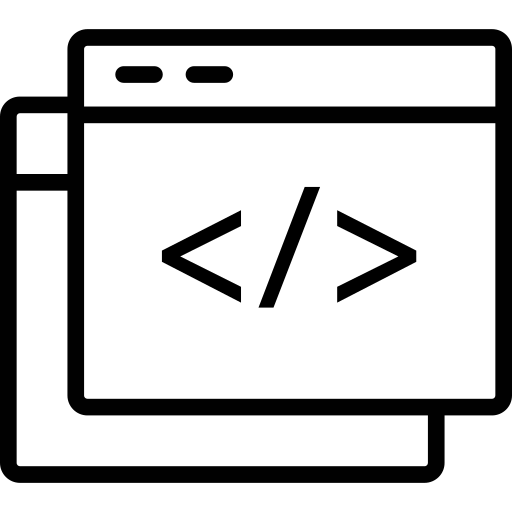
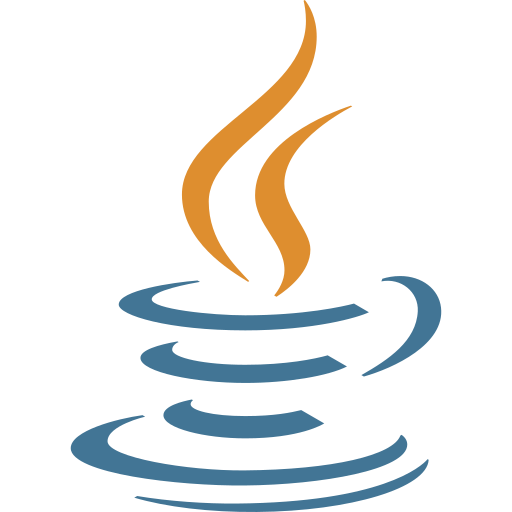
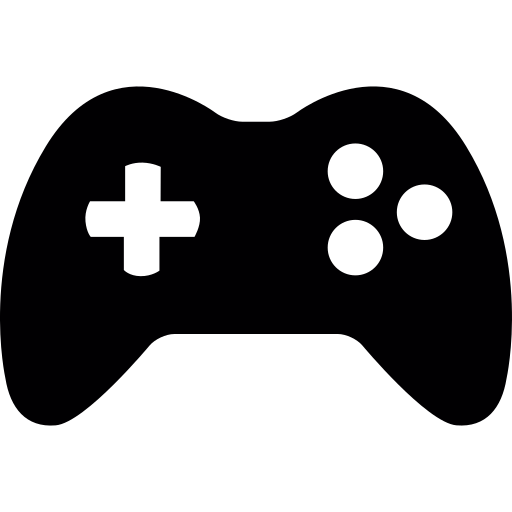
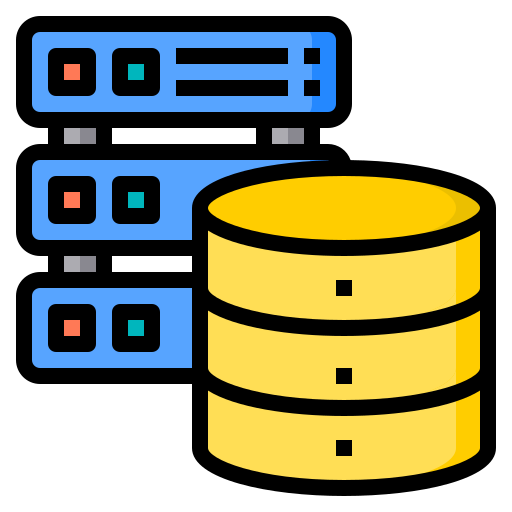
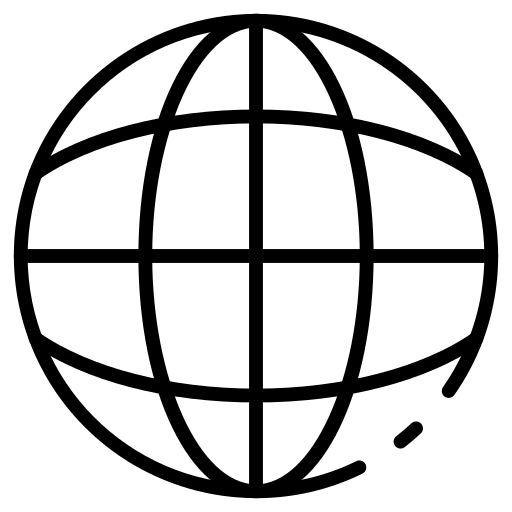
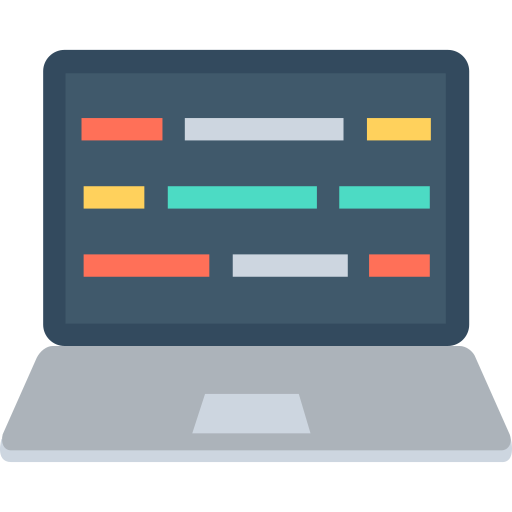
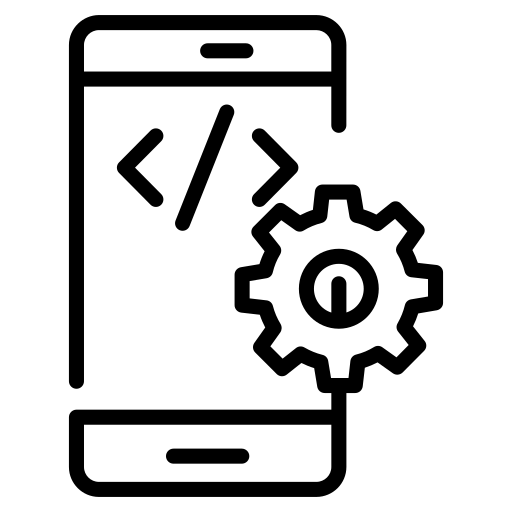
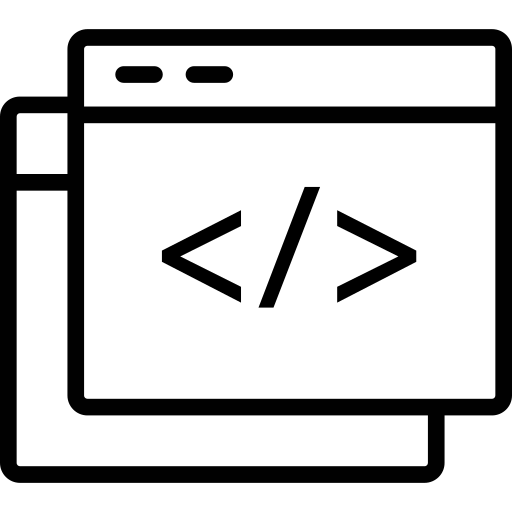
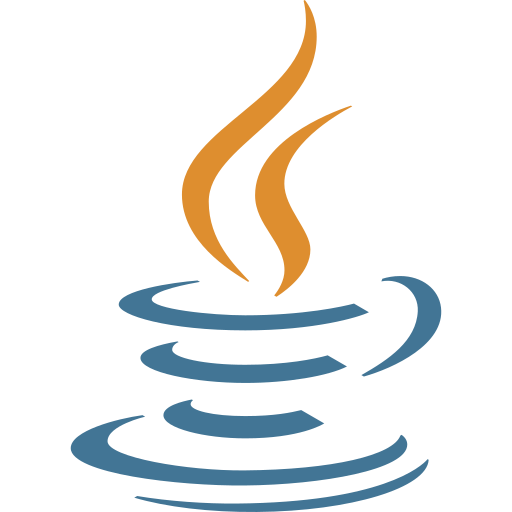
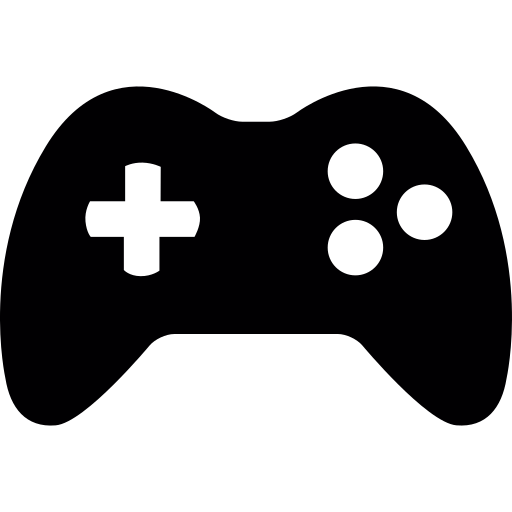
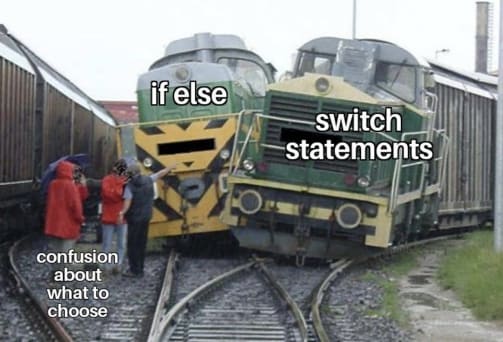
Handling Multiple Different Conditions
When we have many conditions to check, using multiple if-else
chains may not be convenient.
For example:
Main.java
We can see that this does not appear clean and professional. For such cases, Java provides the switch-case statement.
Switch - Case Statement
The switch-case
statement consists of several parts:
Main.java
In the code above, you can see that we use switch blocks to selectively execute operations. We rely on the expression, which is slightly different from a condition. There, we insert a value or an expression. For example, 10 / 2
. In this case, the case block with the signature case 5
will be executed because the expression above equals 5.
We can also use a condition here. In that case, we need to write a Boolean expression in the expression block, and it should look something like this: 10 / 2 == 5
. Then write two cases below:
But this structure will be almost indistinguishable from a regular if-else
.
The Keyword "break;"
We use this keyword to terminate the execution of a switch-case
statement and exit its body. This word is often used in loops, which we will discuss in the following chapters. Use this keyword when you need to break out of a code block and stop its execution.
Now let's improve the code we wrote above using the switch-case statement:
Main.java
We can see how the code has become much cleaner and easier to read and extend. We don't have to write another if
statement from scratch if we need to add additional conditions. Instead, we can add another case block to our switch-case
statement.
Let's look at the switch-case block scheme:
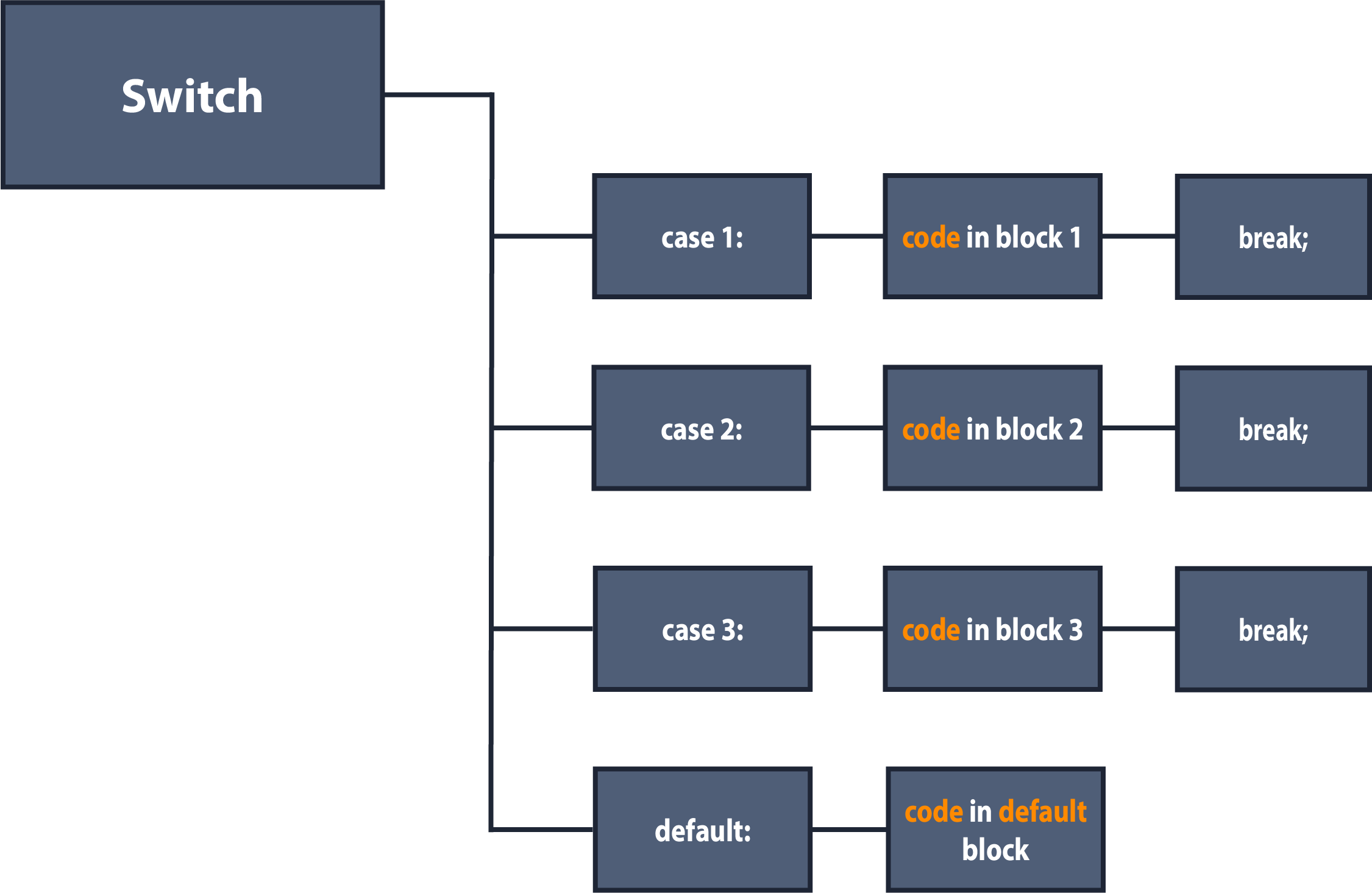
As we can see, there can be as many cases as we want. Each case requires its own condition and code, which will be executed when our program enters the case block.
It's advisable to use the break;
keyword because the program will not exit the switch block until all of the case blocks have been executed. The default block will be executed if we didn't enter any of our case blocks or didn't use the break;
keyword.
Let's look at another example without break;
keywords:
Main.java
We have received multiple values, which differ from what we expected. Additionally, we obtained information from the case 10
and default
blocks. This is precisely why we use the keyword break
after each block. In this way, the program will exit the switch
statement and continue its execution.
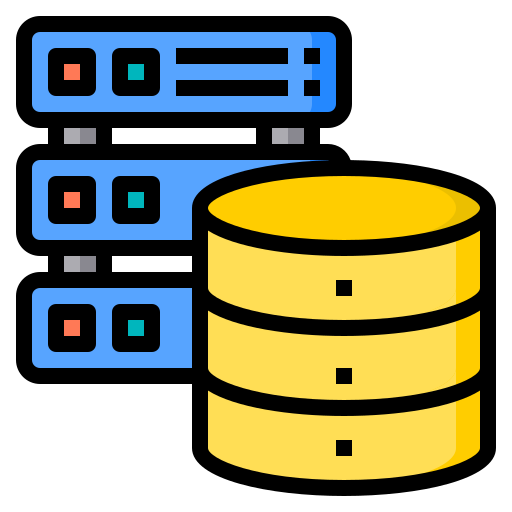
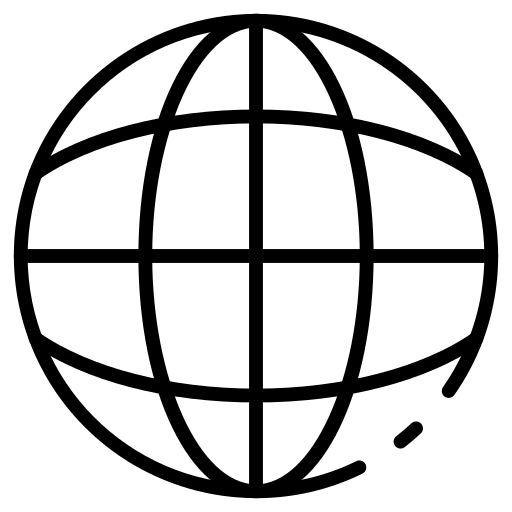
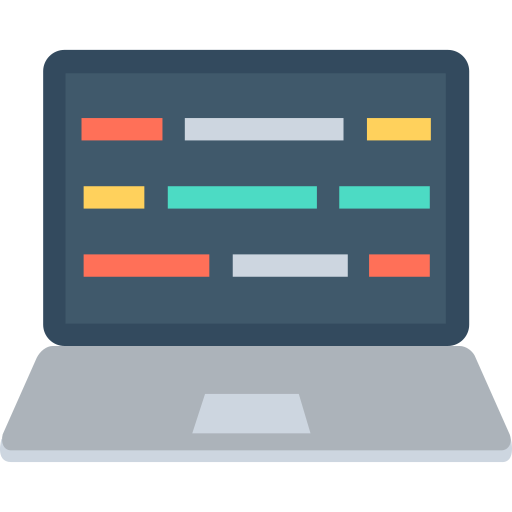
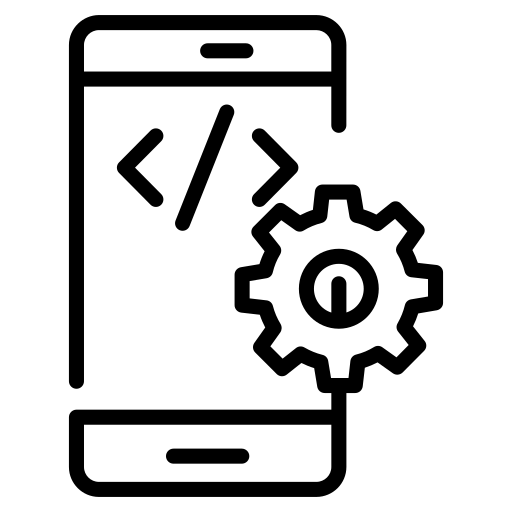
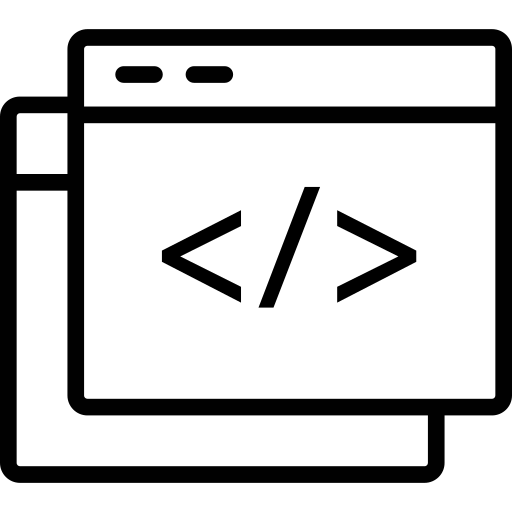
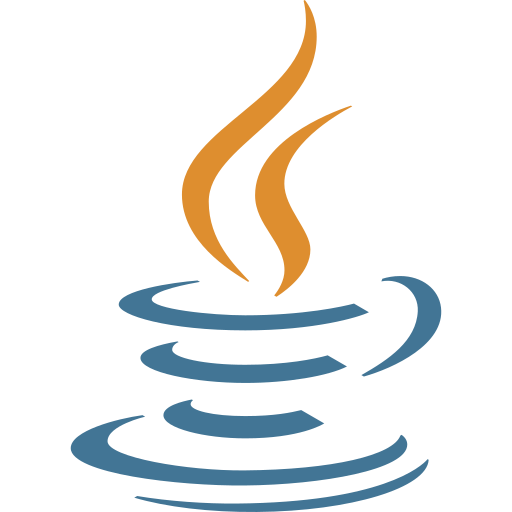
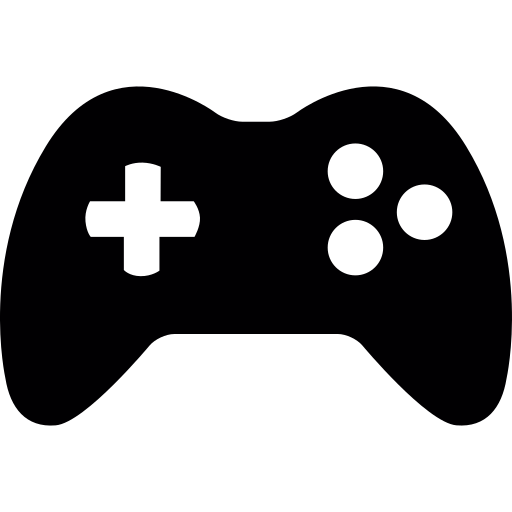
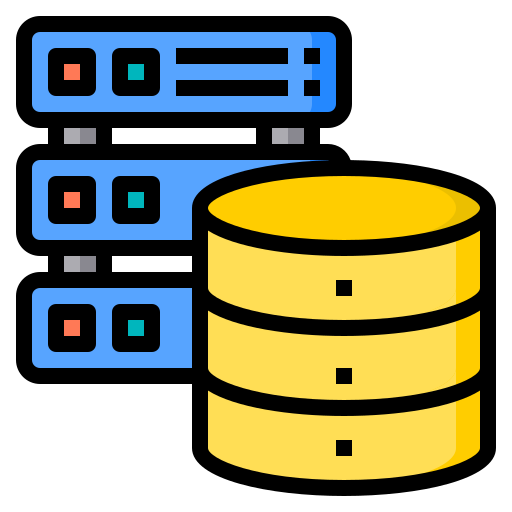
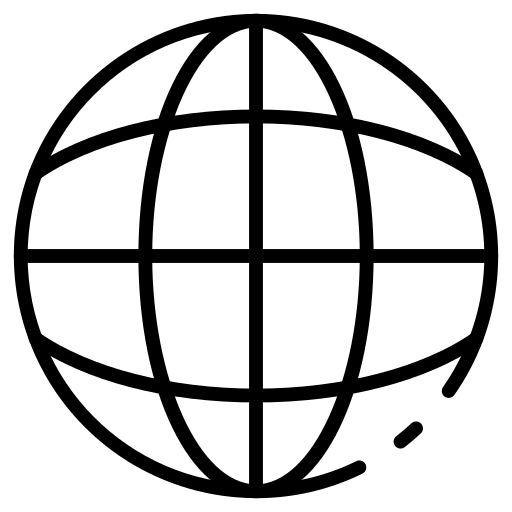
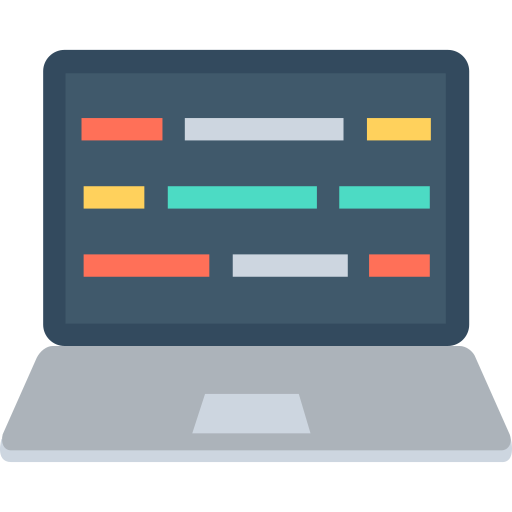
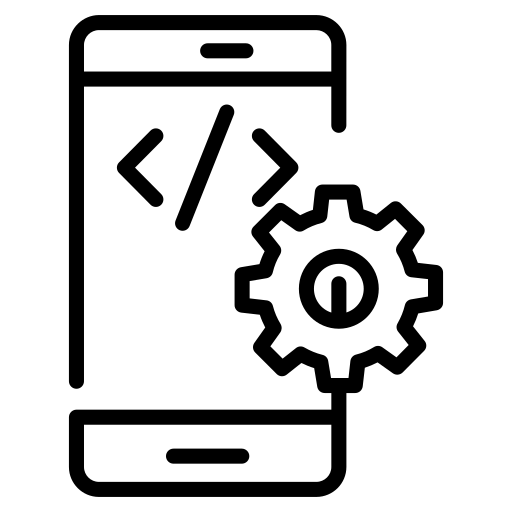
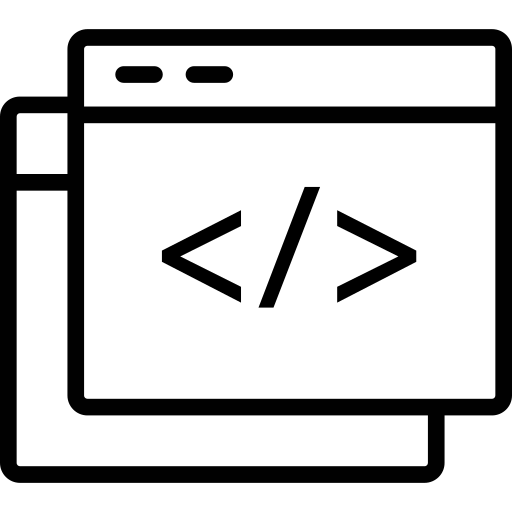
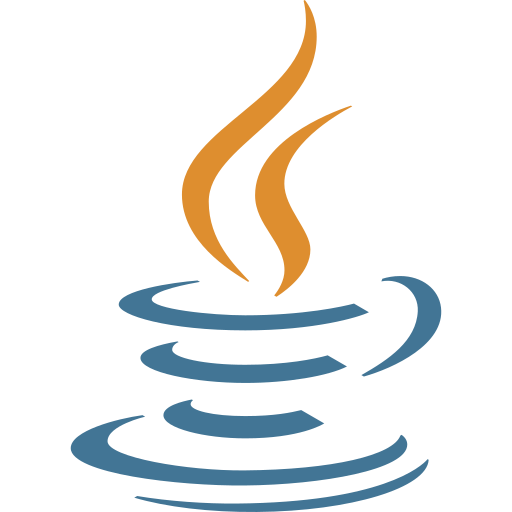
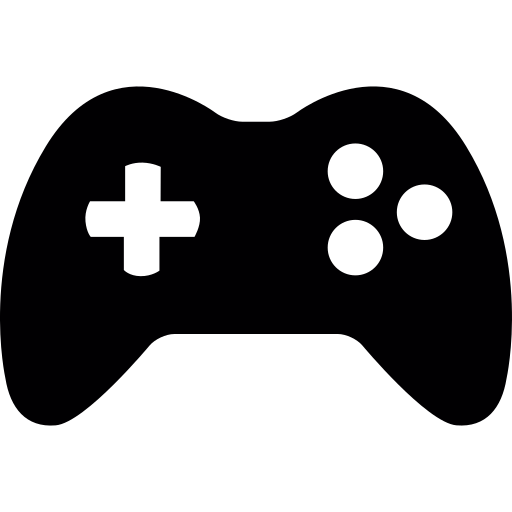
Everything was clear?
Course Content
Java Basics
Java Basics
Switch-Case Statement
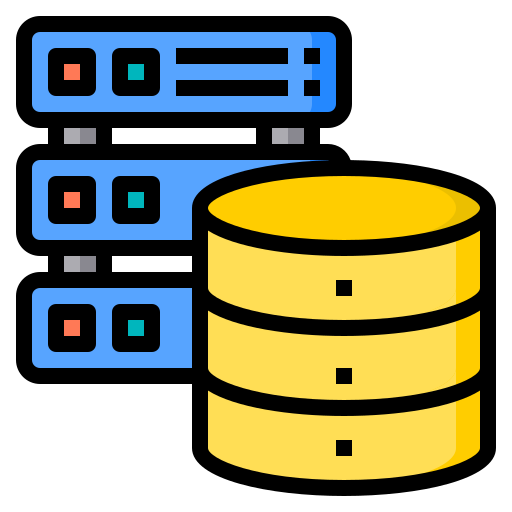
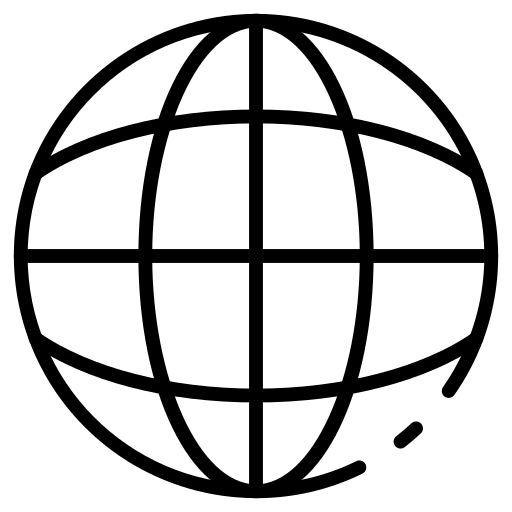
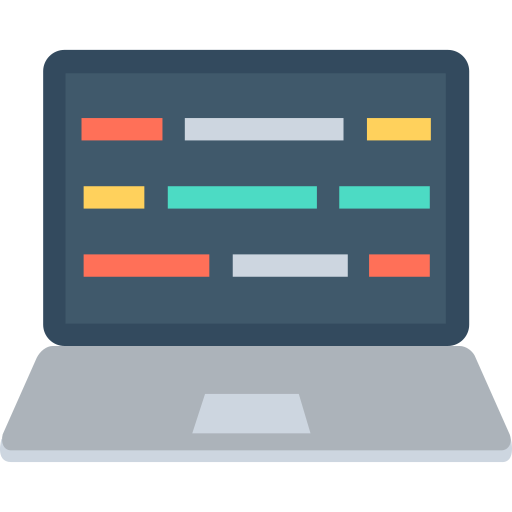
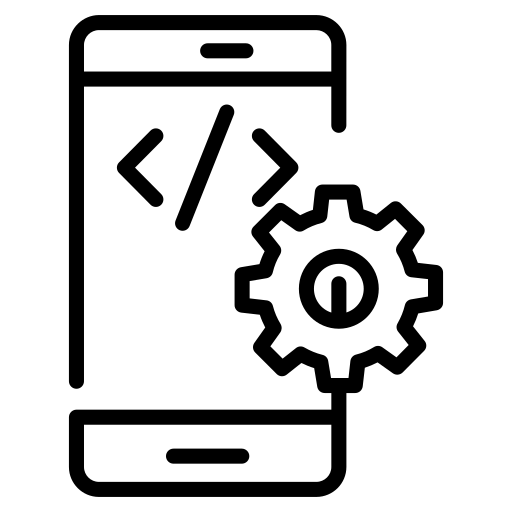
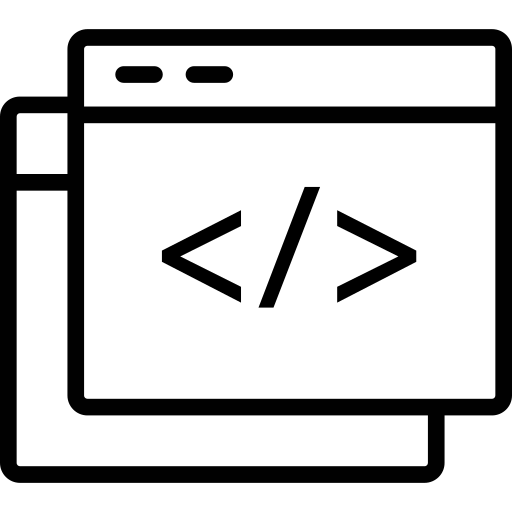
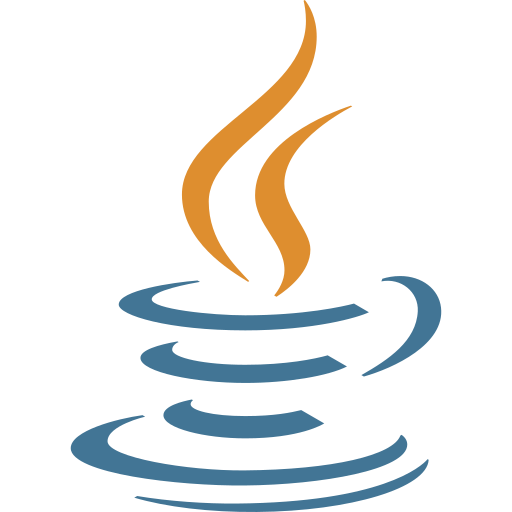
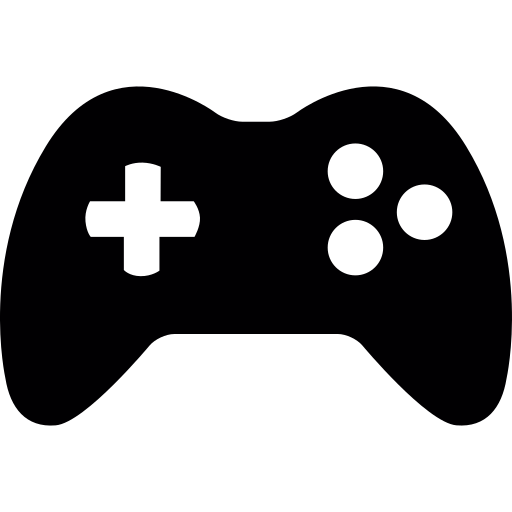
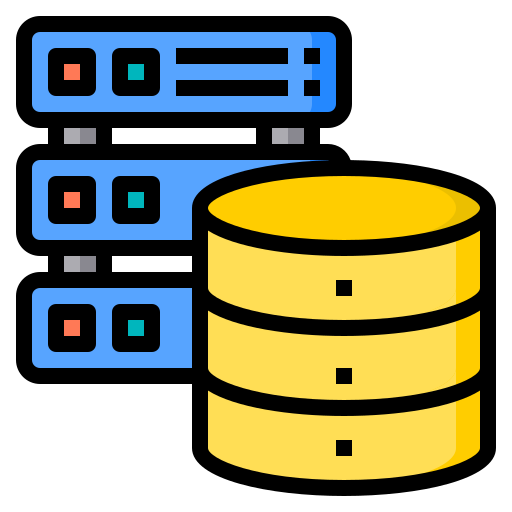
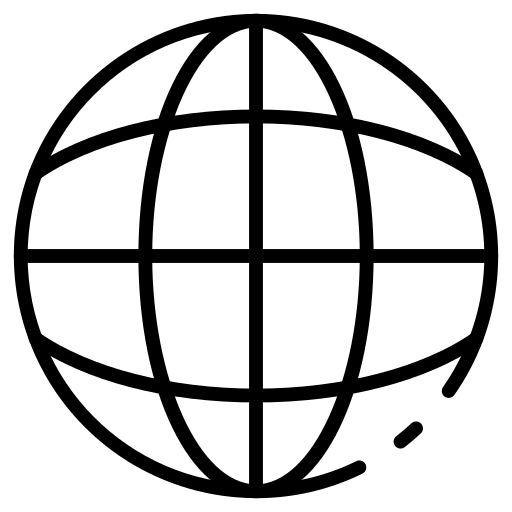
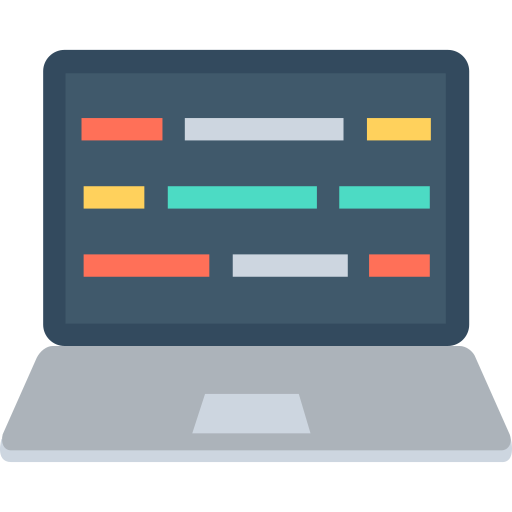
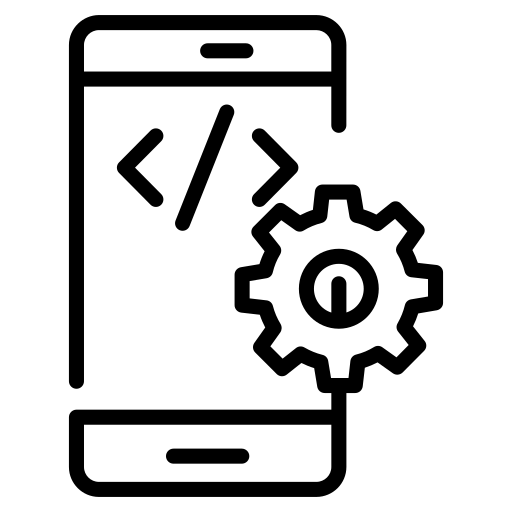
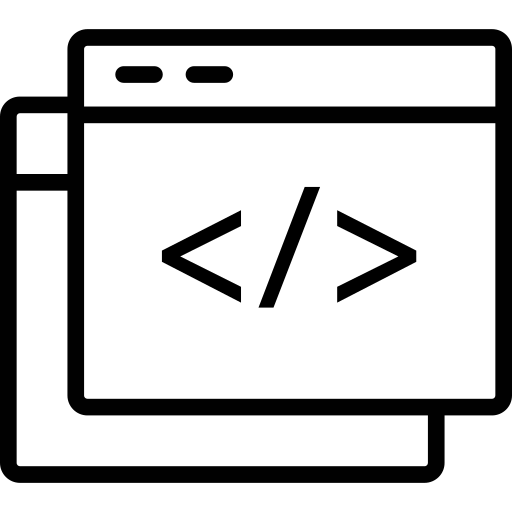
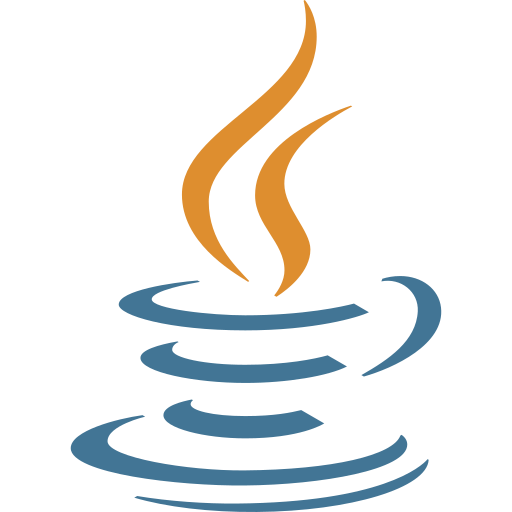
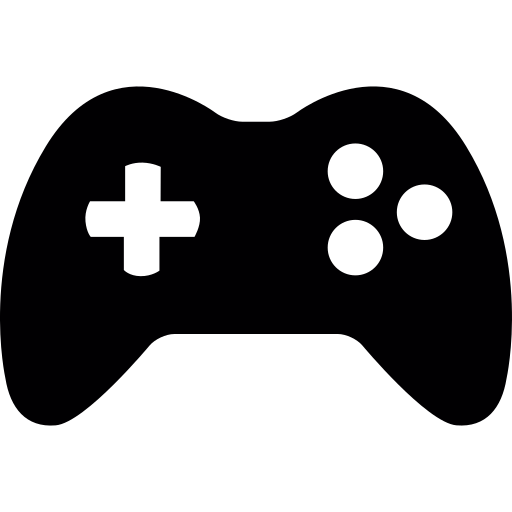
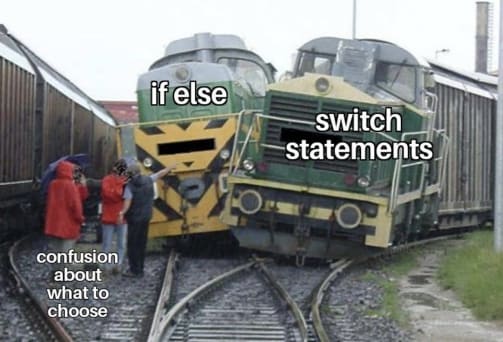
Handling Multiple Different Conditions
When we have many conditions to check, using multiple if-else
chains may not be convenient.
For example:
Main.java
We can see that this does not appear clean and professional. For such cases, Java provides the switch-case statement.
Switch - Case Statement
The switch-case
statement consists of several parts:
Main.java
In the code above, you can see that we use switch blocks to selectively execute operations. We rely on the expression, which is slightly different from a condition. There, we insert a value or an expression. For example, 10 / 2
. In this case, the case block with the signature case 5
will be executed because the expression above equals 5.
We can also use a condition here. In that case, we need to write a Boolean expression in the expression block, and it should look something like this: 10 / 2 == 5
. Then write two cases below:
But this structure will be almost indistinguishable from a regular if-else
.
The Keyword "break;"
We use this keyword to terminate the execution of a switch-case
statement and exit its body. This word is often used in loops, which we will discuss in the following chapters. Use this keyword when you need to break out of a code block and stop its execution.
Now let's improve the code we wrote above using the switch-case statement:
Main.java
We can see how the code has become much cleaner and easier to read and extend. We don't have to write another if
statement from scratch if we need to add additional conditions. Instead, we can add another case block to our switch-case
statement.
Let's look at the switch-case block scheme:
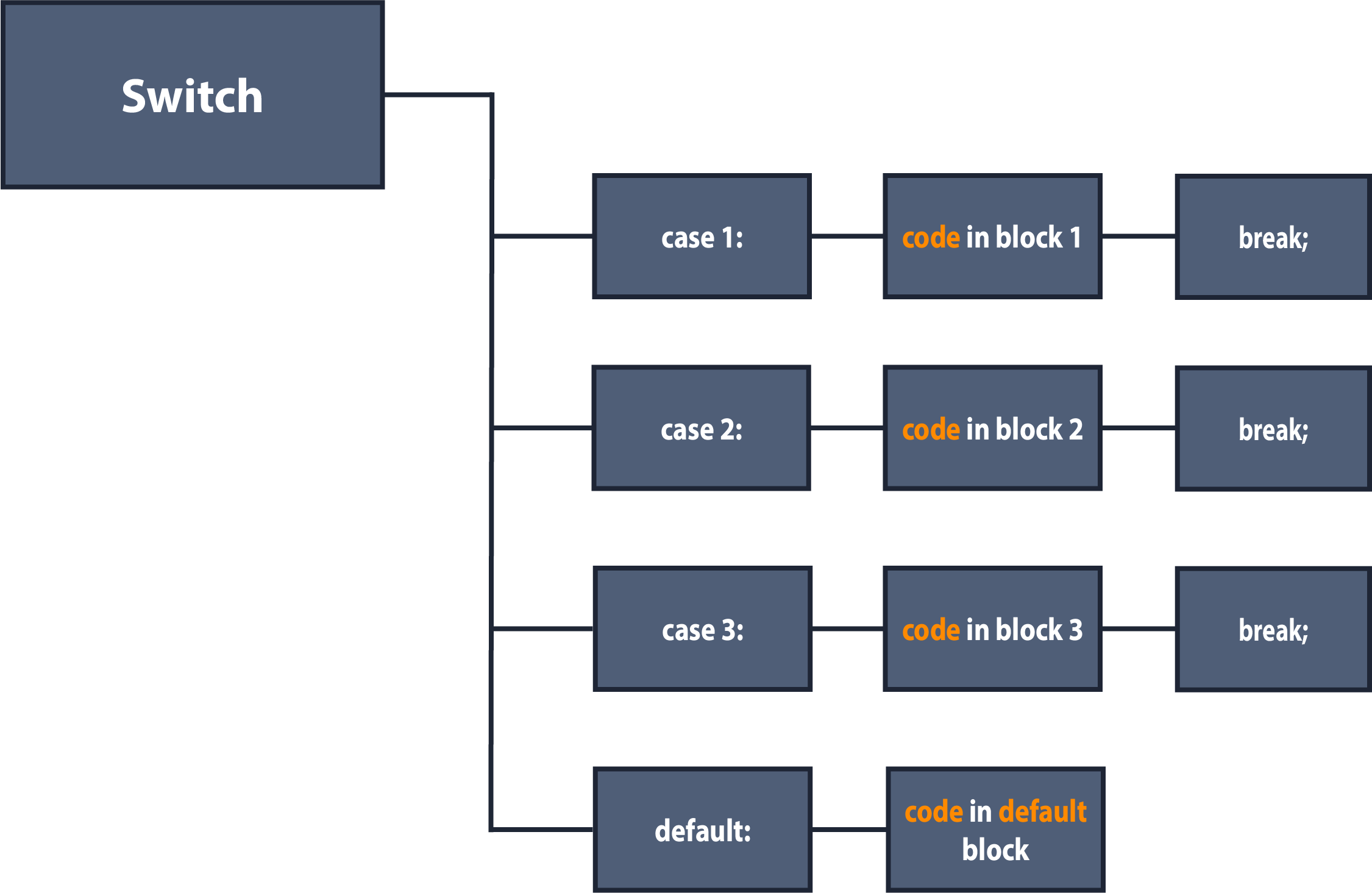
As we can see, there can be as many cases as we want. Each case requires its own condition and code, which will be executed when our program enters the case block.
It's advisable to use the break;
keyword because the program will not exit the switch block until all of the case blocks have been executed. The default block will be executed if we didn't enter any of our case blocks or didn't use the break;
keyword.
Let's look at another example without break;
keywords:
Main.java
We have received multiple values, which differ from what we expected. Additionally, we obtained information from the case 10
and default
blocks. This is precisely why we use the keyword break
after each block. In this way, the program will exit the switch
statement and continue its execution.
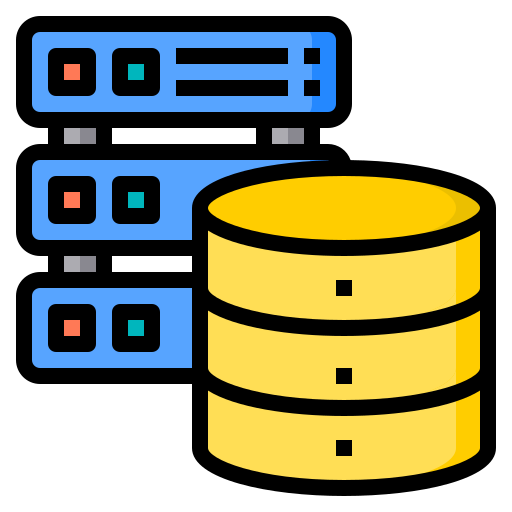
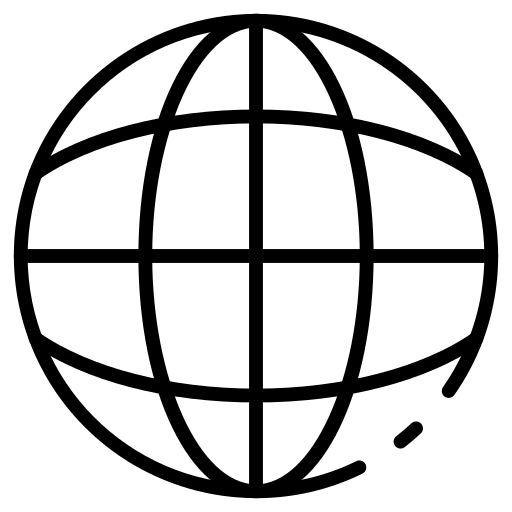
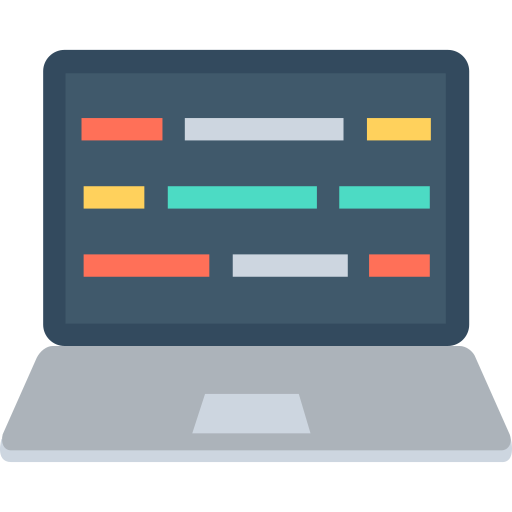
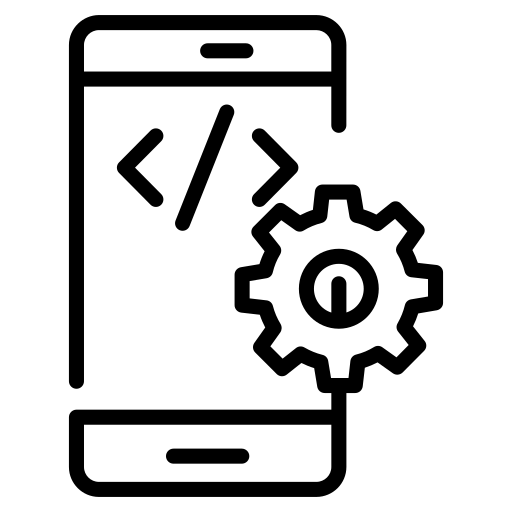
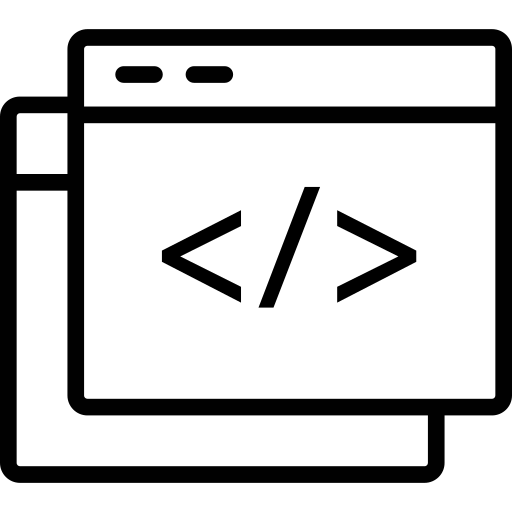
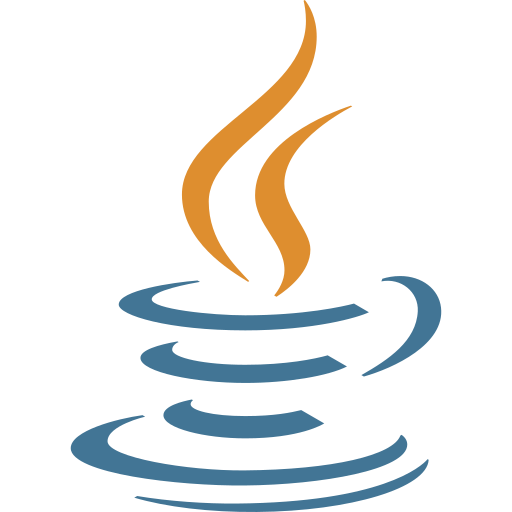
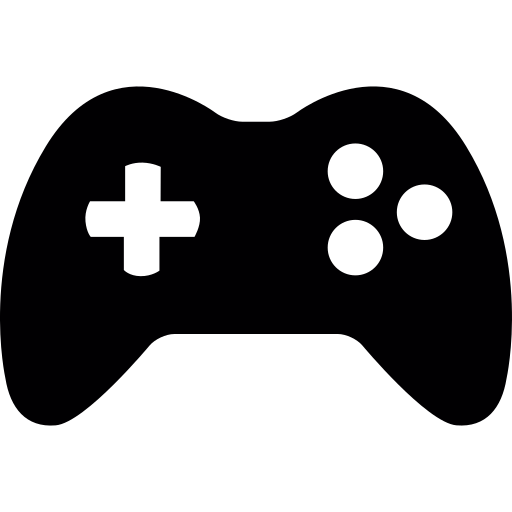
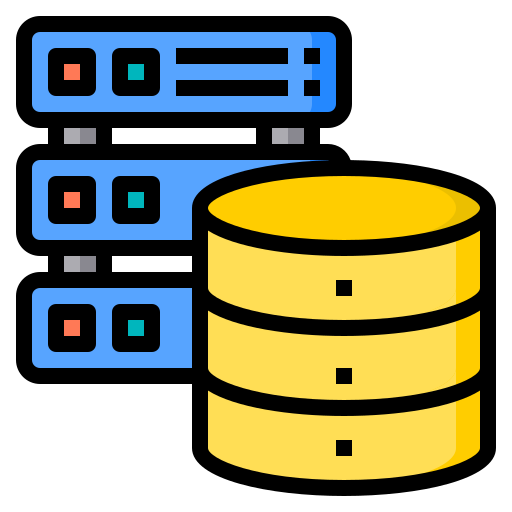
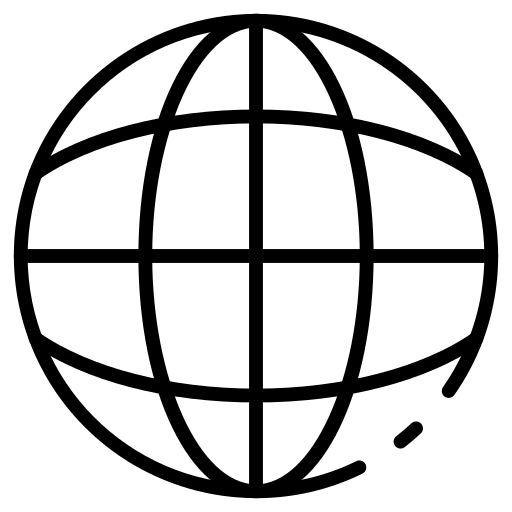
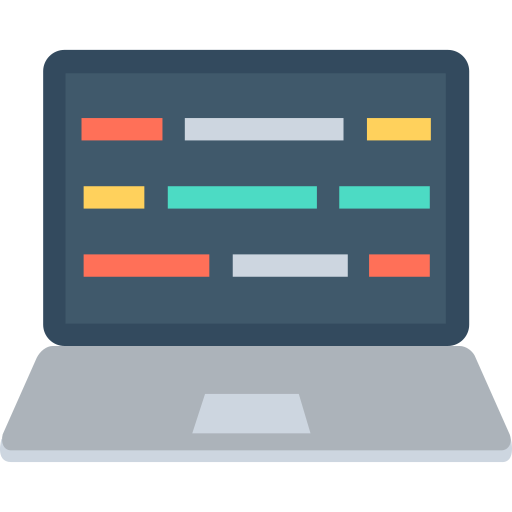
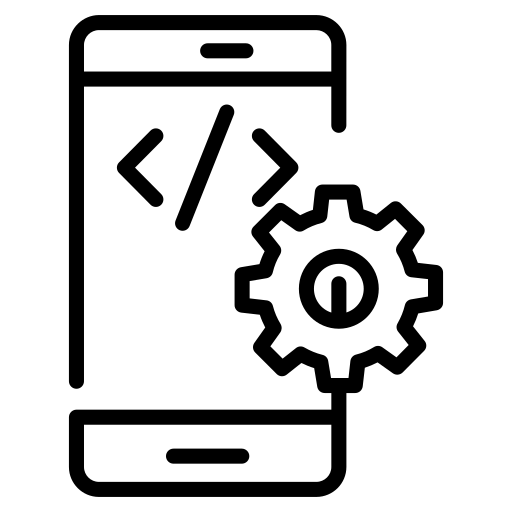
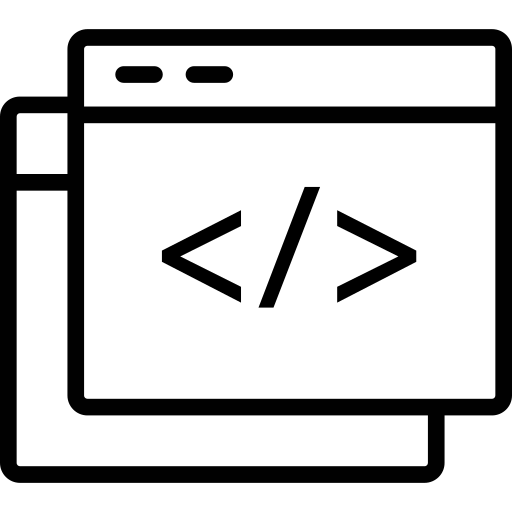
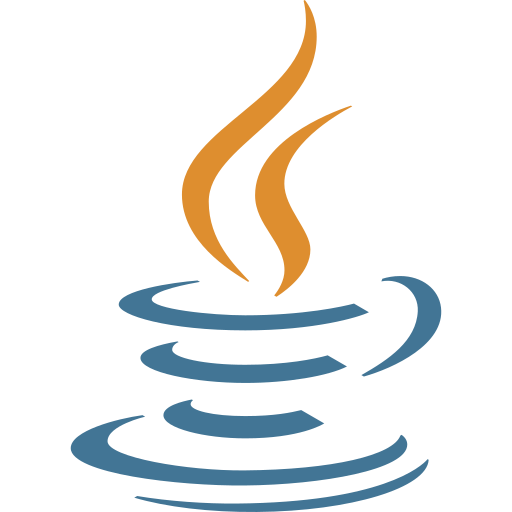
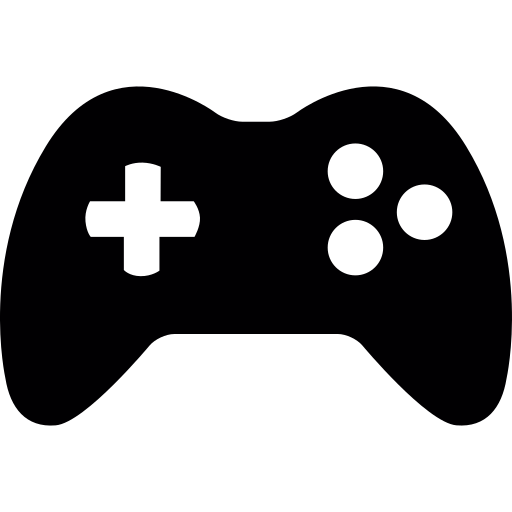
Everything was clear?