Variables
What is a Variable?
A variable is a named space in the memory that stores values. In other words, it acts a container for values in a program.
A variable must be declared before it is used. Dart uses the var
keyword to achieve this. The syntax for declaring a variable is given below.
main.dart
Code Syntax
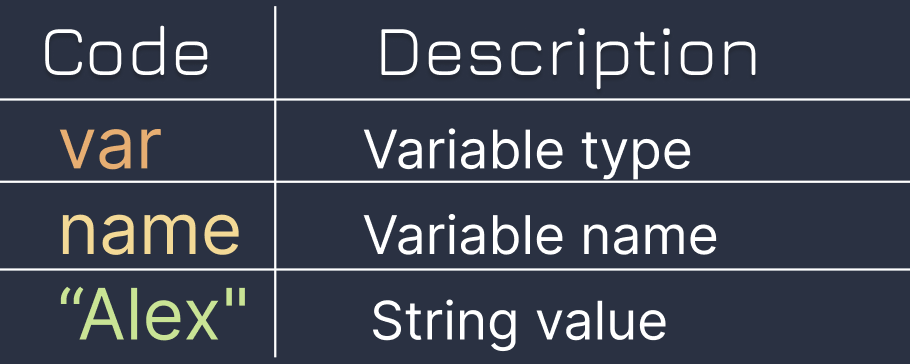
Variable Declaration Rules
- Variables name must be unique within a block of code;
- Variables name can’t be the keyword (like
var
orvoid
).; - Variables name should not start with a number;
- Variables name must not contain spaces or special symbols, except the underscore(
_
) and the dollar($
) sign.
Example Use Variable
- Thus, we work with the value stored inside the variable:
main.dart
- We can write the numbers calculation result or join strings into a variable:
main.dart
Keyword Var
When declaring a variable using var
, we do not explicitly indicate the type of data that will be stored in this variable. Dart automatically determines what data type a variable will contain when it is initialized.
With such initialization, the age
variable will store integer values.
Please note that after initializing a variable with one data type, you cannot reassign the data type for the variable!
We have successfully written the value 28
to the variable age
but cannot write the String
value 28
to this variable.
Type-checking in Dart
Type-checking in Dart is the process of verifying that the data types used in the code match the expected data types. It helps prevent errors such as assigning a value of the wrong type to a variable.
Note
Dart uses static typing, meaning the compiler checks types before running the code. It provides more safety than dynamic typing, which is used in JavaScript.
Type Annotations
To improve the readability and structure of the code, you need to use type annotations. To do this, instead of the var
keyword, we write the variable's data type.
Syntax comparison of type annotation and keyword var
:
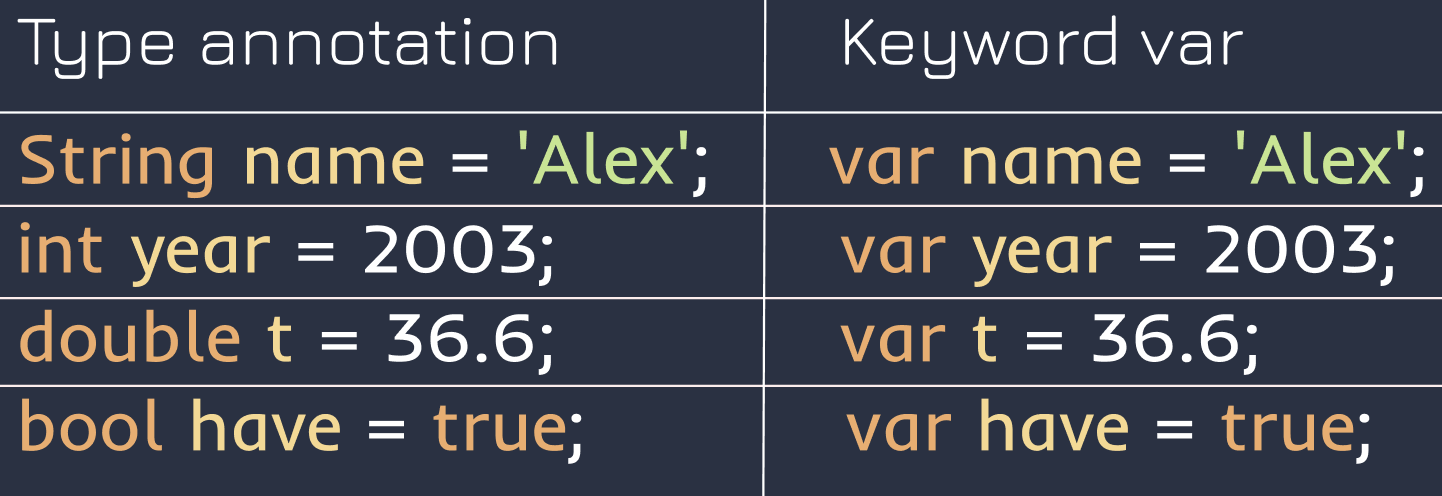
Type annotations are optional in Dart since the Dart can usually determine the type of a variable by its initial value. However, using type annotations is recommended as it can help prevent errors and make your code more readable and understandable.
Все було зрозуміло?
Зміст курсу
Introduction to Dart
1. First Acquaintance with Dart
2. Variables and Data Types
3. Conditional Statements
4. List and String
Introduction to Dart
Variables
What is a Variable?
A variable is a named space in the memory that stores values. In other words, it acts a container for values in a program.
A variable must be declared before it is used. Dart uses the var
keyword to achieve this. The syntax for declaring a variable is given below.
main.dart
Code Syntax
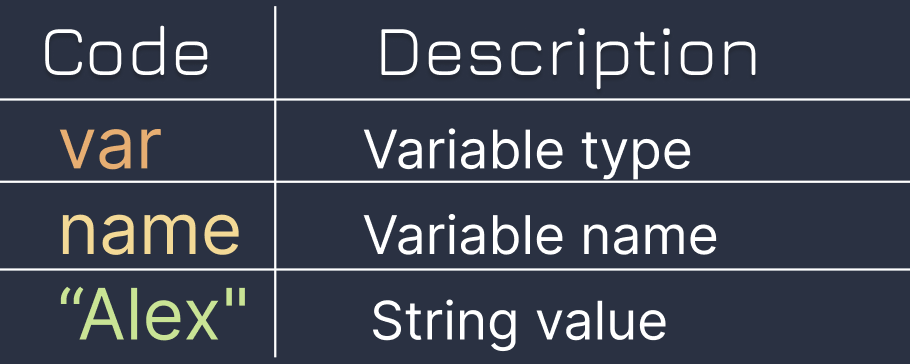
Variable Declaration Rules
- Variables name must be unique within a block of code;
- Variables name can’t be the keyword (like
var
orvoid
).; - Variables name should not start with a number;
- Variables name must not contain spaces or special symbols, except the underscore(
_
) and the dollar($
) sign.
Example Use Variable
- Thus, we work with the value stored inside the variable:
main.dart
- We can write the numbers calculation result or join strings into a variable:
main.dart
Keyword Var
When declaring a variable using var
, we do not explicitly indicate the type of data that will be stored in this variable. Dart automatically determines what data type a variable will contain when it is initialized.
With such initialization, the age
variable will store integer values.
Please note that after initializing a variable with one data type, you cannot reassign the data type for the variable!
We have successfully written the value 28
to the variable age
but cannot write the String
value 28
to this variable.
Type-checking in Dart
Type-checking in Dart is the process of verifying that the data types used in the code match the expected data types. It helps prevent errors such as assigning a value of the wrong type to a variable.
Note
Dart uses static typing, meaning the compiler checks types before running the code. It provides more safety than dynamic typing, which is used in JavaScript.
Type Annotations
To improve the readability and structure of the code, you need to use type annotations. To do this, instead of the var
keyword, we write the variable's data type.
Syntax comparison of type annotation and keyword var
:
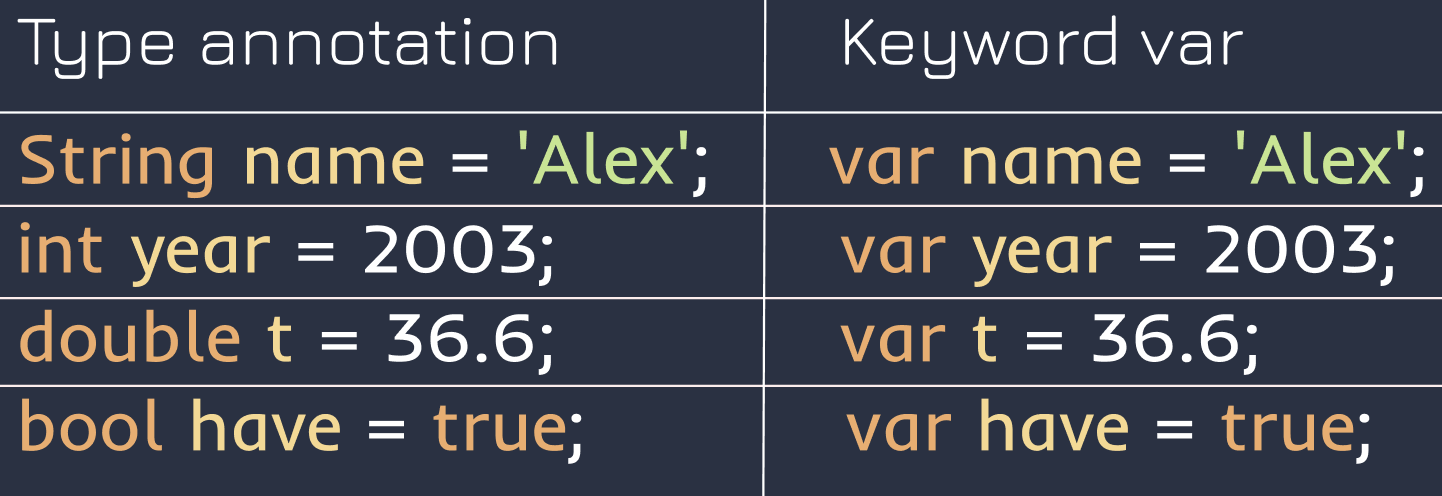
Type annotations are optional in Dart since the Dart can usually determine the type of a variable by its initial value. However, using type annotations is recommended as it can help prevent errors and make your code more readable and understandable.
Все було зрозуміло?