Course Content
Java JUnit Library. Types of Testing
Java JUnit Library. Types of Testing
Throwing Exceptions
Let's delve deeper into exceptions. You've encountered exceptions before, but we haven't explored them in detail. Now, we'll discuss them more extensively and learn how to create and handle them on our own in this section.
Why are we learning about exceptions in a testing course?
Testing code is done to make the code better and more professional. Therefore, you also need to know how to throw, catch, and create exceptions and, subsequently, how to test methods with exceptions correctly.
Let's start with the definition:
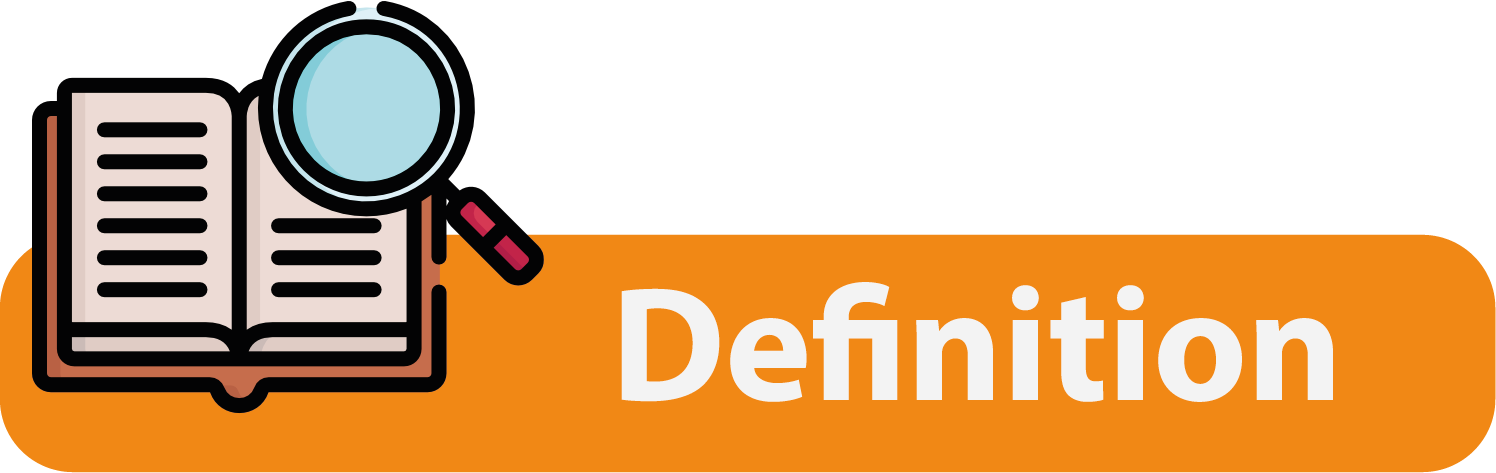
Now, let's put it in simple terms. Suppose you're writing a method for dividing two numbers. In mathematics, we know that you can't divide by zero. In the event that a user of our code tries to divide by zero, we need to tell them that it's not possible, and we'll do that using an exception. For example, our method looks like this:
In this class, we can see where we need to throw an exception. After an exception is thrown, the program execution will stop, and the user will see a console message saying that division by 0 is not allowed.
You can throw an exception using the throw
keyword. Since an exception is an object, we must create a new exception object using a constructor. In the code, it will look like this:
As you can see, we are throwing a new RuntimeException
object that will halt the program execution if we attempt to divide by zero. In the parameters, we have provided text that will be displayed on the screen if an exception is thrown. However, RuntimeException
is not the most appropriate exception for this case. Here, we can see an error related to arithmetic operations, so we should use an exception that indicates, by its name, that the error is related to arithmetic operations:
ArithmeticException
clearly indicates by its name that the error is related to arithmetic operations. This way, you can throw exceptions in your checks or code blocks. It is a good practice to specify throws ExceptionName
in a method that throws an exception. This way, you will see that this method will throw a specific exception.
Let's look at the entire class where we attempt to throw an exception:
Main.java
As you can see, when we run and perform division by zero, an error with a descriptive message about what went wrong is thrown. Later in the course, you will learn how to catch such exceptions and handle them, create your own custom exceptions, and then test methods with them.
What is the purpose of an exception in Java?
Select the correct answer
How can you throw an exception in a Java method?
Select the correct answer
Which exception should be thrown for an arithmetic error like division by zero?
Select the correct answer
Everything was clear?