Declaration and Advantages of Arrays
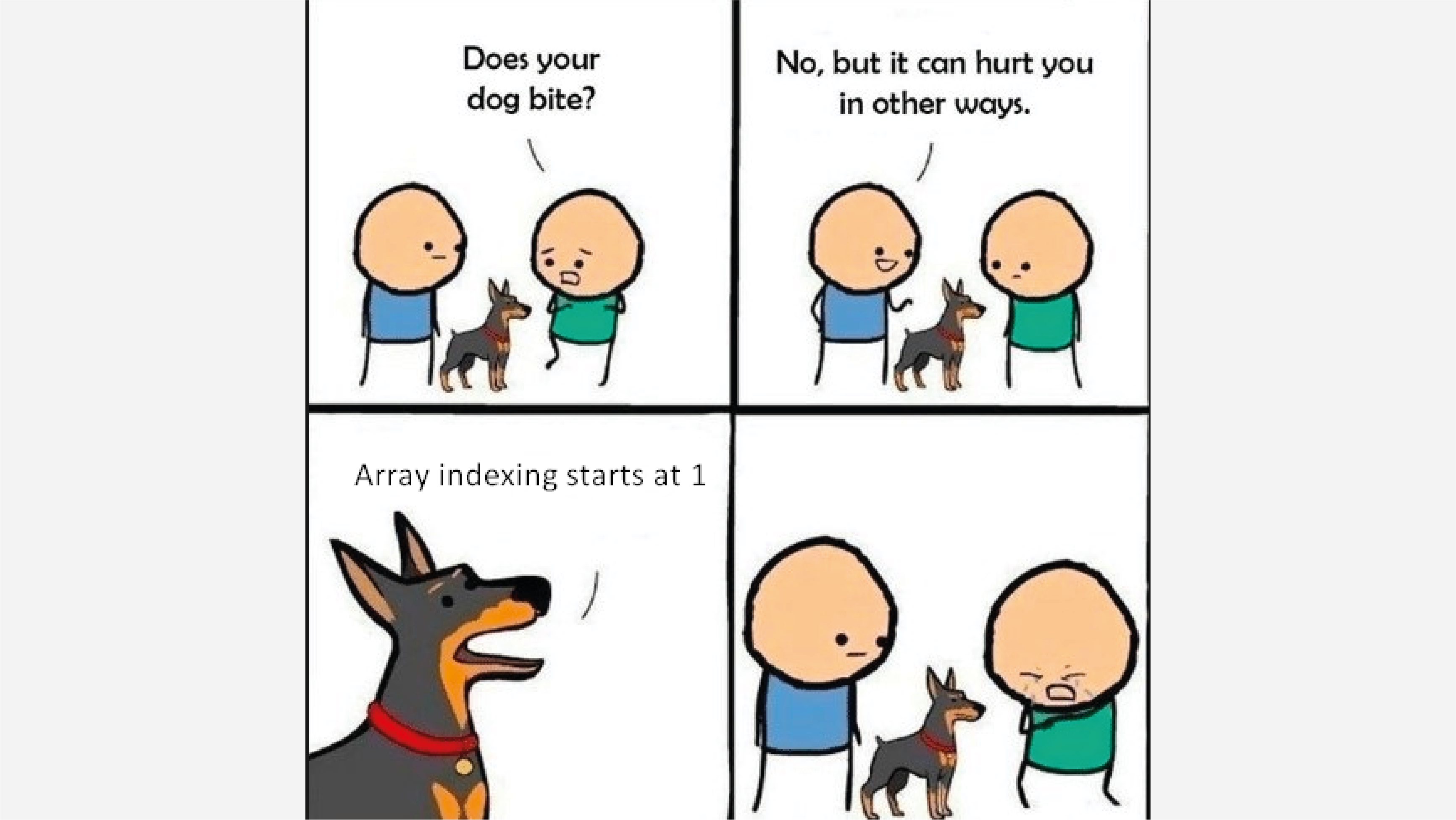
Imagine you have several books, let's say 5
of them. These books are scattered on the floor, and it's not always convenient to reach the one you need. That's how variables and data are scattered in your device's memory.
What's the solution to this problem?
Buy a bookshelf and place the books there. This bookshelf will act as an array in our code. From the shelf, we can easily take the books, seeing the titles on the covers. But in an array, a different system is used to access elements - it's called indexing. Each book will have its own index within the array, and by referring to the index, we can retrieve the desired element.
That's enough text; let's move on to the code:
We have implemented the bookshelf as an array. Let's briefly go over the syntax of how we did it, so:
let books: type[]
- Here, we define thename
andtype
of our array. We type the array with thestring[]
type since it will store book titles. Pay attention to the[]
symbols that we specify along with the type. These symbols indicate that it is indeed an array, not a simple variable;= [element1, element2, element3, ..., elementN];
- Here, we assign values to the array. Pay attention to the syntax: We assignstring
values separated by commas inside square brackets ([]
). This is our bookshelf with the titles of various books.
Q: The question arises, how do I get the book I need from the shelf?
A: The answer is - by index.
Indexing
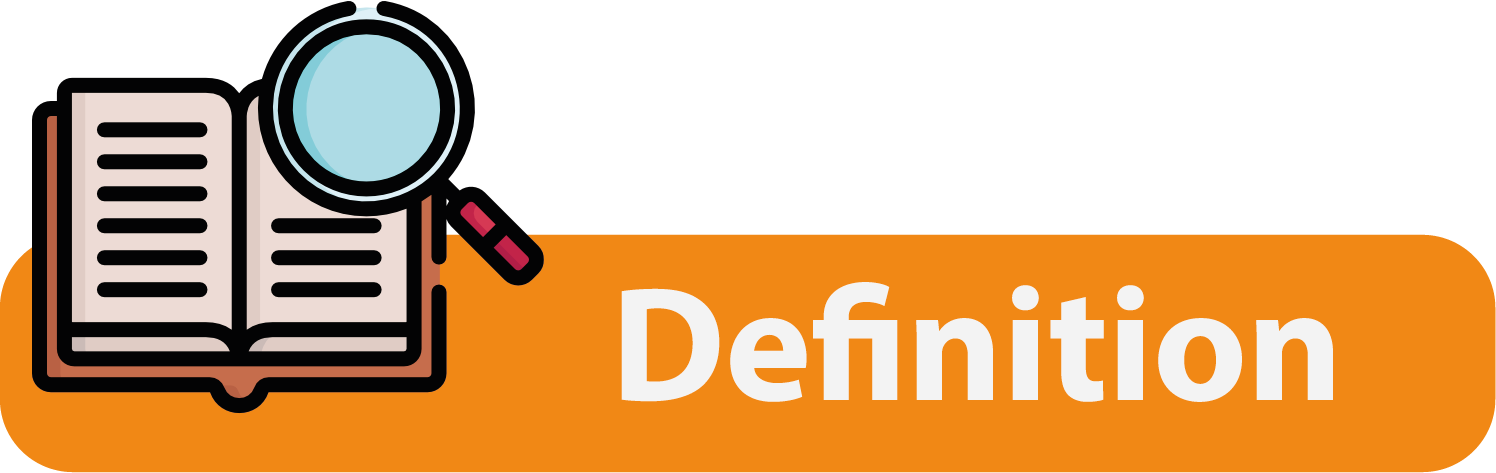
0
, the second element is at index 1
, and so on. You can use square brackets []
with the index to retrieve or modify the value of a specific element in the array. At the moment, we can retrieve an item from our bookshelf. It's done easily:
The syntax is arrayName[index]
. This way, we can access items on our 'bookshelf.'
Also, take note of the index number; we access the element at index 1
, which will be the second element in the array. This is zero-based indexing, meaning the first element has an index of 0
, the second 1
, the third 2
, and so on.
To access the last element in our 'bookshelf', we need to access the element at index 4
, as we have a total of 5
elements, making 4
the last index. This can be expressed in a formula:
index = element's_position_in_order - 1
.
Modifying Array Elements
We can replace or modify an element in the array by accessing it using its index; this is done like so:
It's so easy to do; the key is to choose the right index. But a good programmer usually knows their arrays. In the next chapter, you'll learn how to properly modify, add, and remove elements from an array. There's a lot more to discover!
Все було зрозуміло?
Зміст курсу
Introduction to TypeScript
Introduction to TypeScript
Declaration and Advantages of Arrays
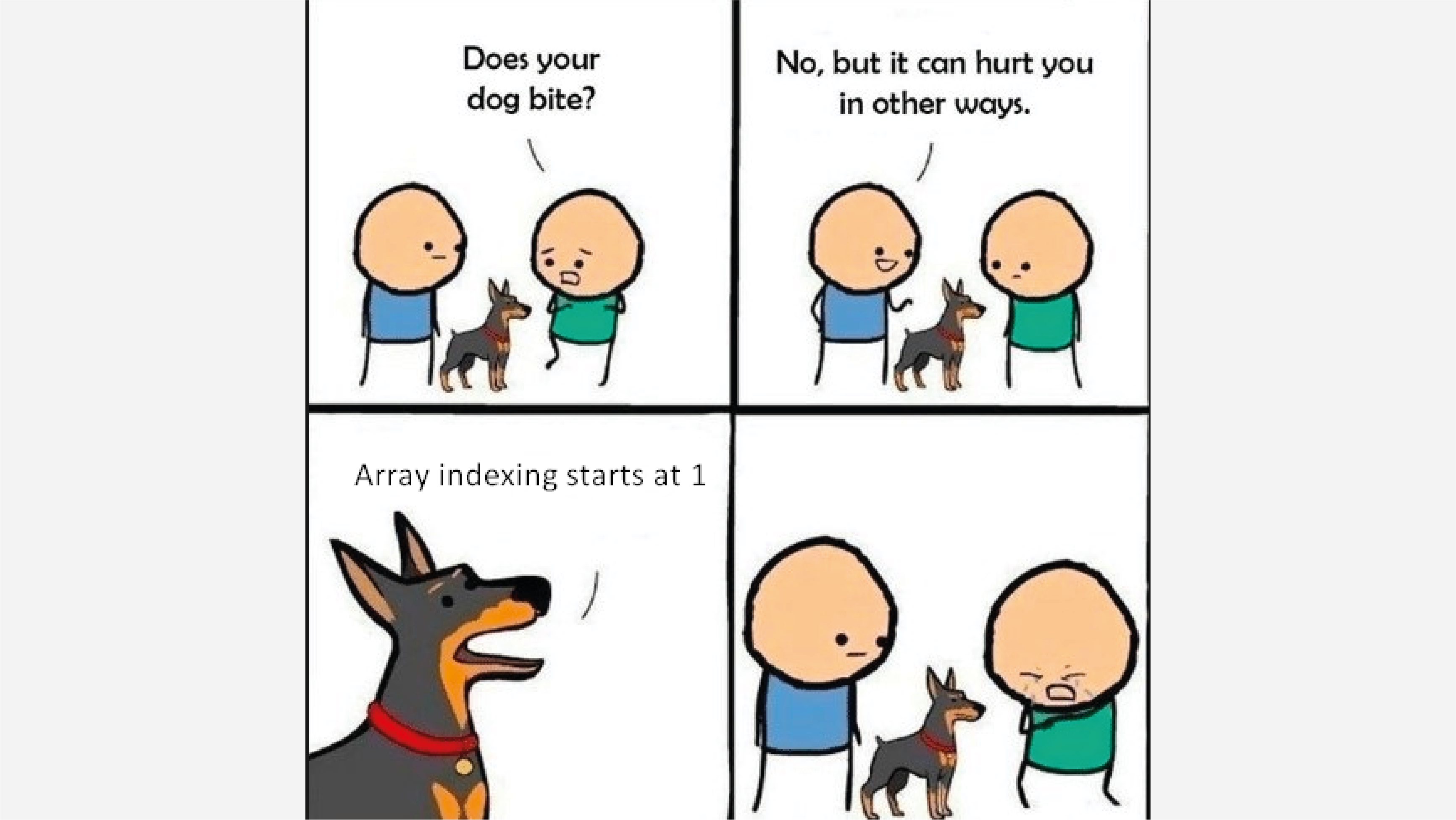
Imagine you have several books, let's say 5
of them. These books are scattered on the floor, and it's not always convenient to reach the one you need. That's how variables and data are scattered in your device's memory.
What's the solution to this problem?
Buy a bookshelf and place the books there. This bookshelf will act as an array in our code. From the shelf, we can easily take the books, seeing the titles on the covers. But in an array, a different system is used to access elements - it's called indexing. Each book will have its own index within the array, and by referring to the index, we can retrieve the desired element.
That's enough text; let's move on to the code:
We have implemented the bookshelf as an array. Let's briefly go over the syntax of how we did it, so:
let books: type[]
- Here, we define thename
andtype
of our array. We type the array with thestring[]
type since it will store book titles. Pay attention to the[]
symbols that we specify along with the type. These symbols indicate that it is indeed an array, not a simple variable;= [element1, element2, element3, ..., elementN];
- Here, we assign values to the array. Pay attention to the syntax: We assignstring
values separated by commas inside square brackets ([]
). This is our bookshelf with the titles of various books.
Q: The question arises, how do I get the book I need from the shelf?
A: The answer is - by index.
Indexing
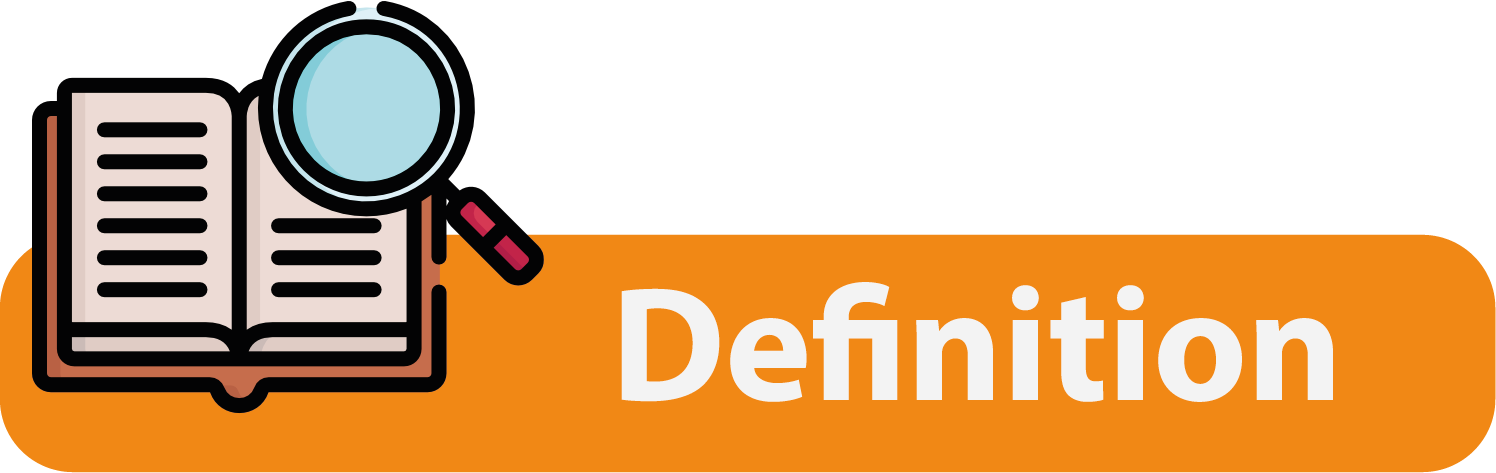
0
, the second element is at index 1
, and so on. You can use square brackets []
with the index to retrieve or modify the value of a specific element in the array. At the moment, we can retrieve an item from our bookshelf. It's done easily:
The syntax is arrayName[index]
. This way, we can access items on our 'bookshelf.'
Also, take note of the index number; we access the element at index 1
, which will be the second element in the array. This is zero-based indexing, meaning the first element has an index of 0
, the second 1
, the third 2
, and so on.
To access the last element in our 'bookshelf', we need to access the element at index 4
, as we have a total of 5
elements, making 4
the last index. This can be expressed in a formula:
index = element's_position_in_order - 1
.
Modifying Array Elements
We can replace or modify an element in the array by accessing it using its index; this is done like so:
It's so easy to do; the key is to choose the right index. But a good programmer usually knows their arrays. In the next chapter, you'll learn how to properly modify, add, and remove elements from an array. There's a lot more to discover!
Все було зрозуміло?