Class Creation
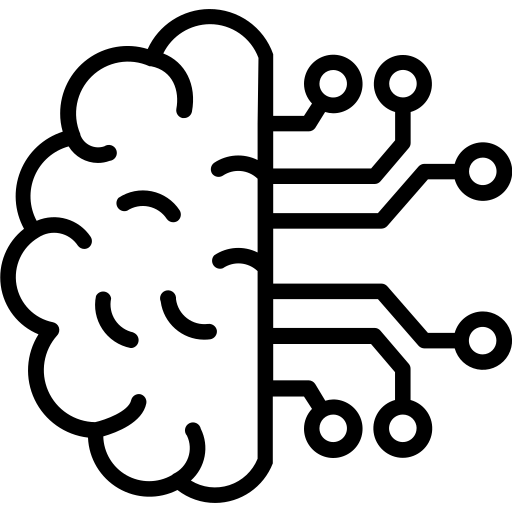
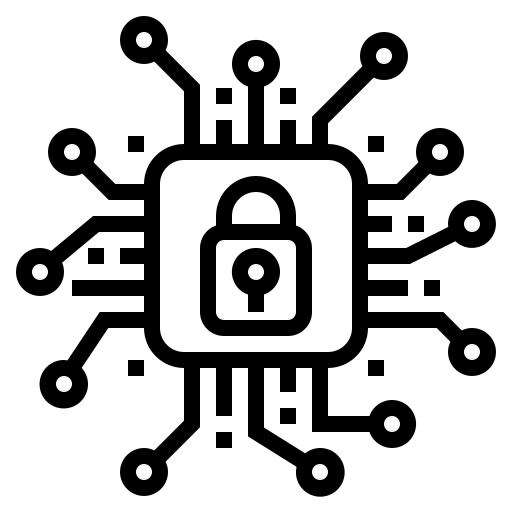
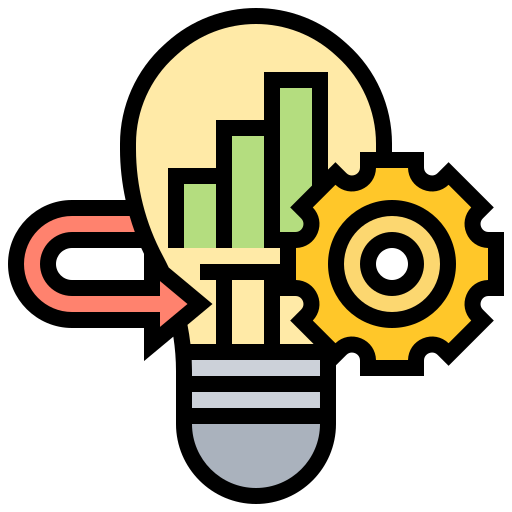
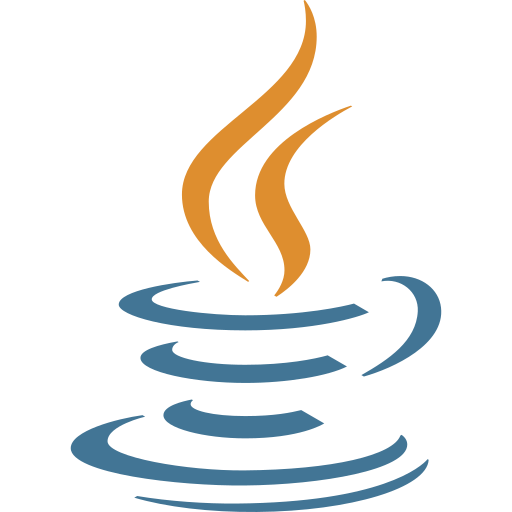
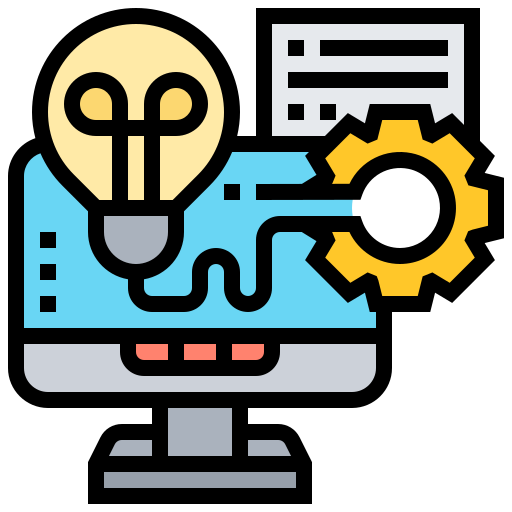
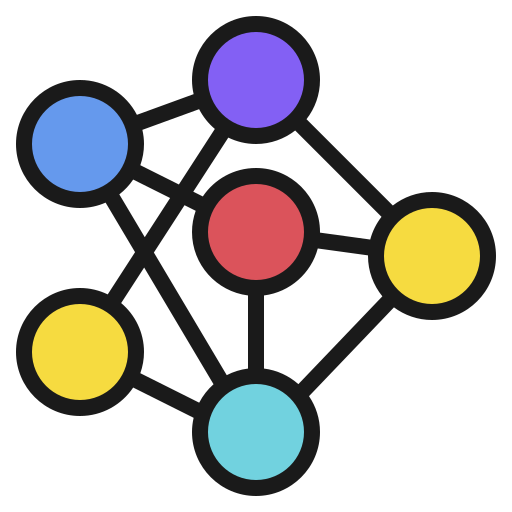
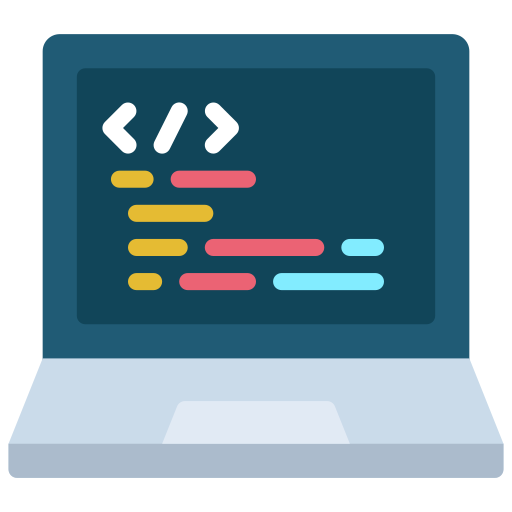
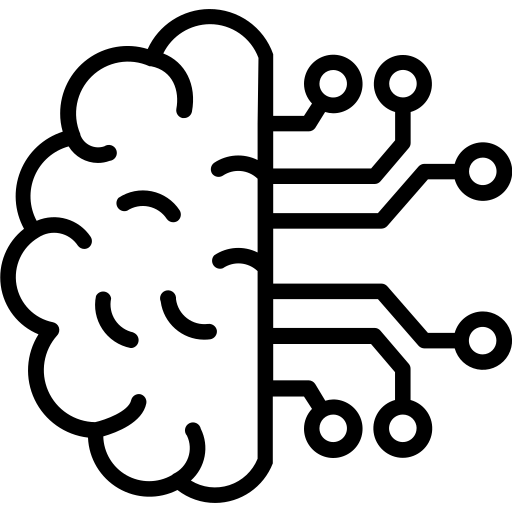
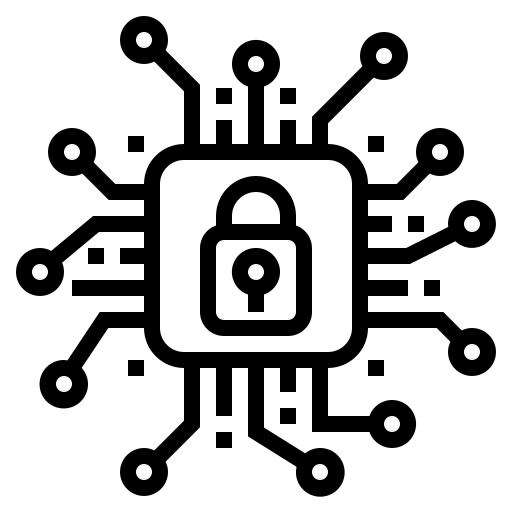
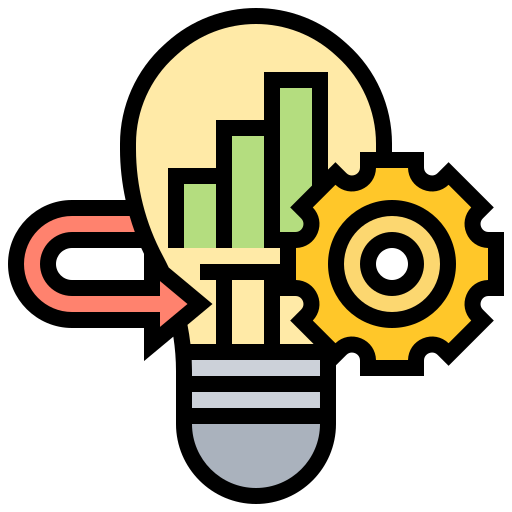
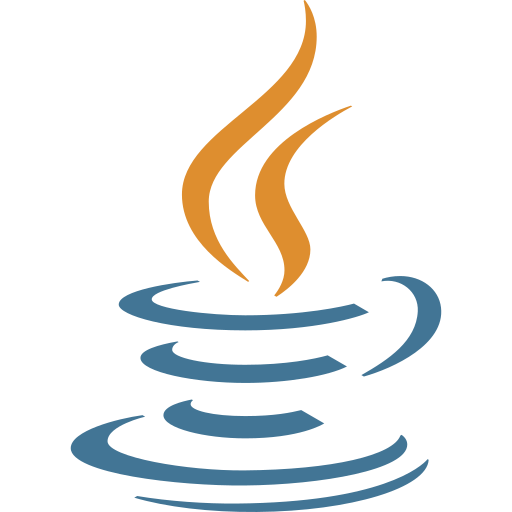
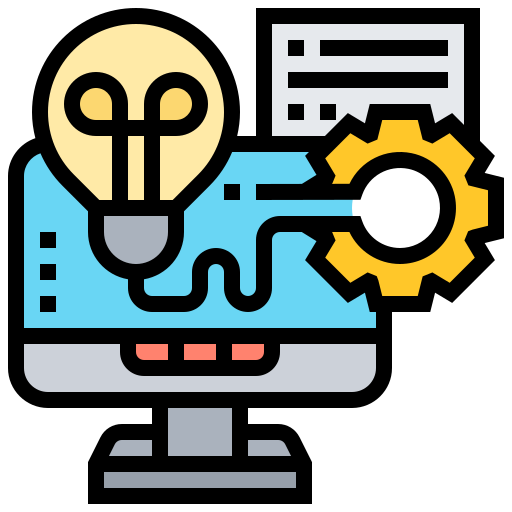
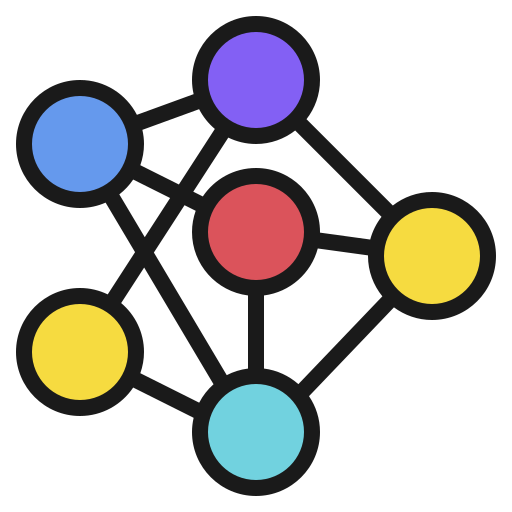
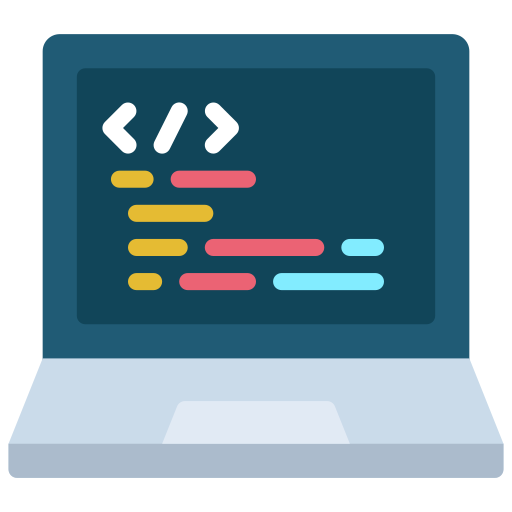
Class Syntax
The syntax for creating a class is quite simple. Let's take a look at an example with the class Person
:
Person.java
This is all it takes to create a class. However, it will be useless to us if it's empty. Therefore, we should add fields to it. Let's assume that our person has a name
, gender
, and age
:
Person.java
And now, let's write a method that allows our Person to report their name and age:
Person.java
Task
Great! Now your task is to create two objects of the Person
class in the main
method. One will be named Bob
and will be 30
years old, and the other will be named Alice
and will be 27
years old. Then, use the introduce()
method on each person to have them introduce themselves.
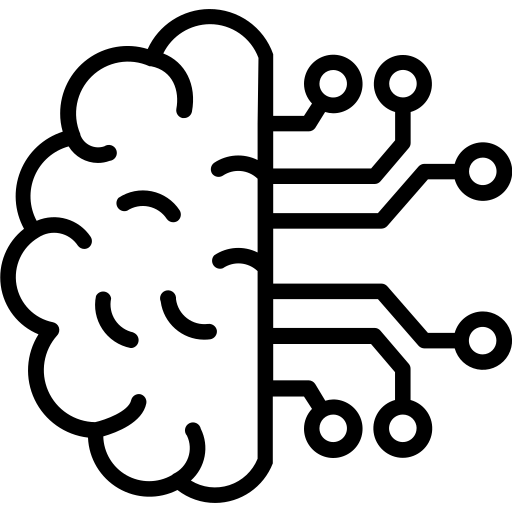
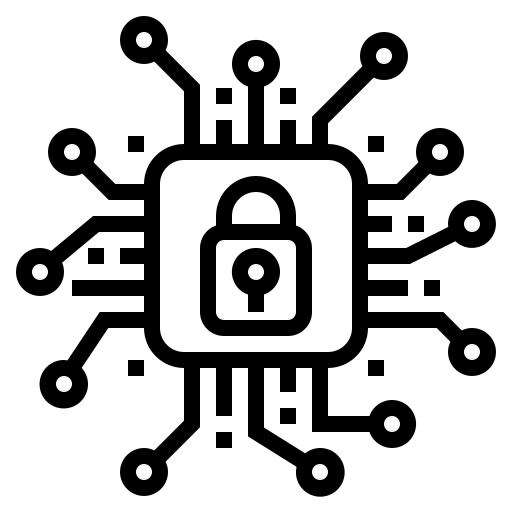
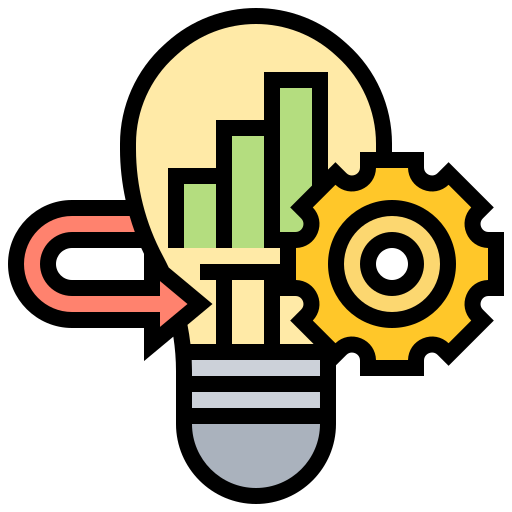
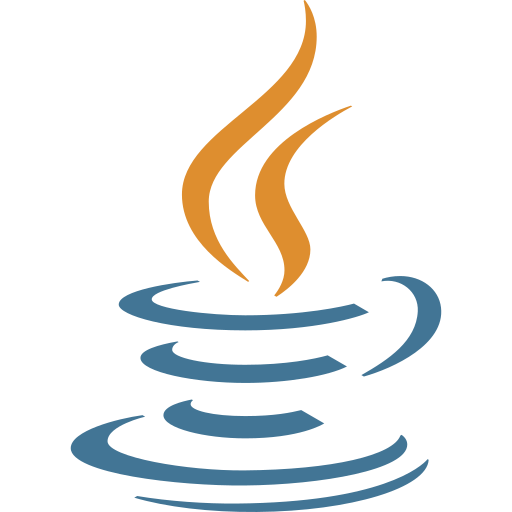
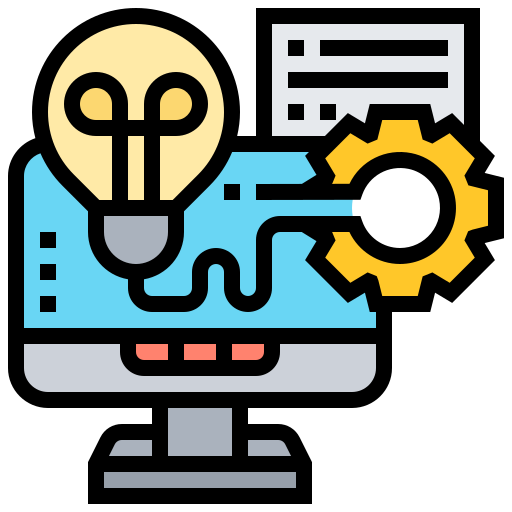
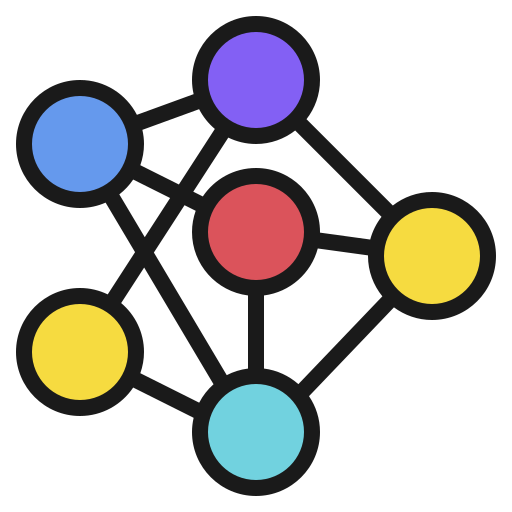
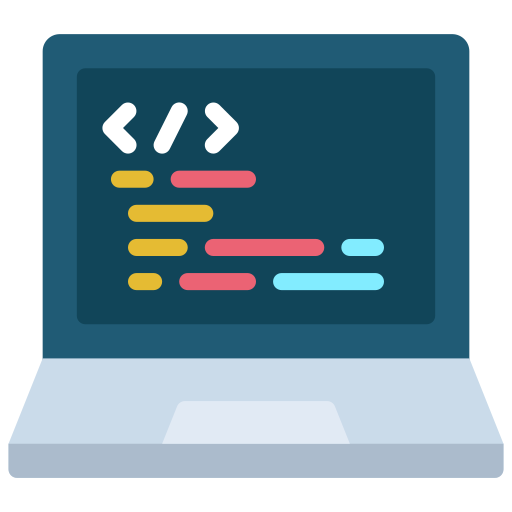
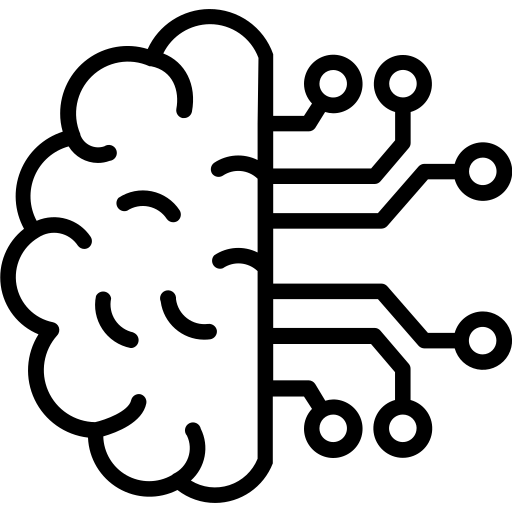
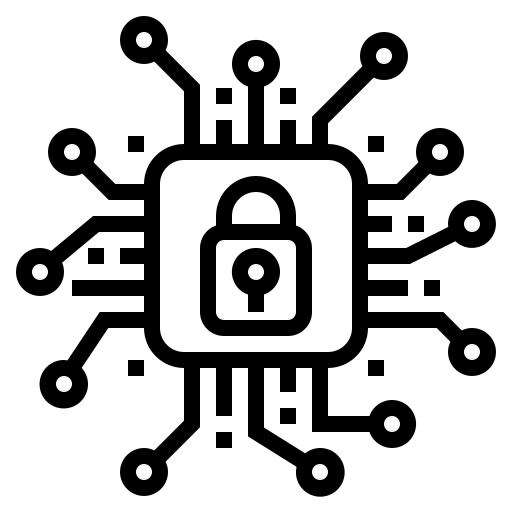
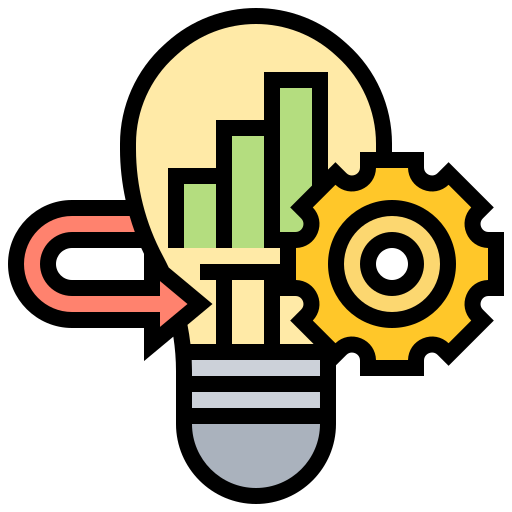
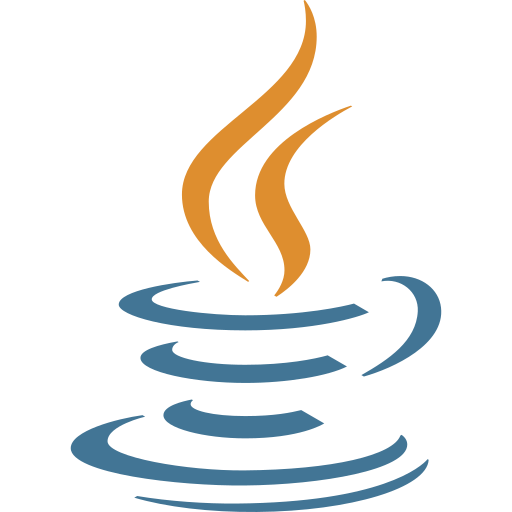
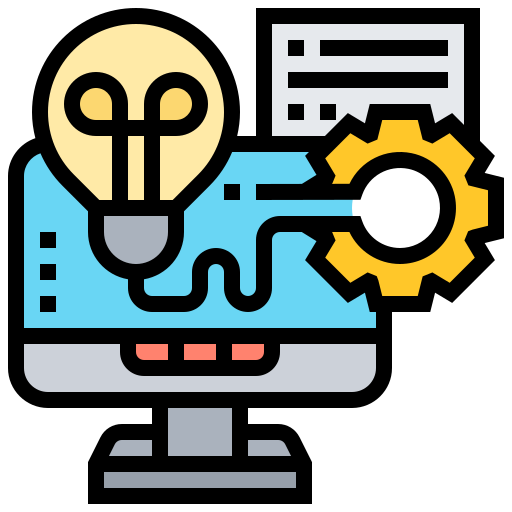
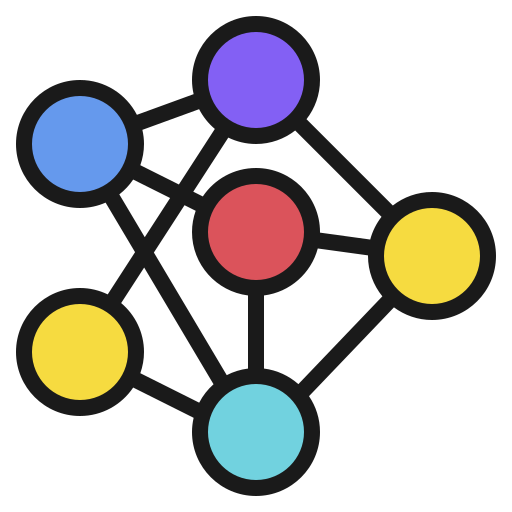
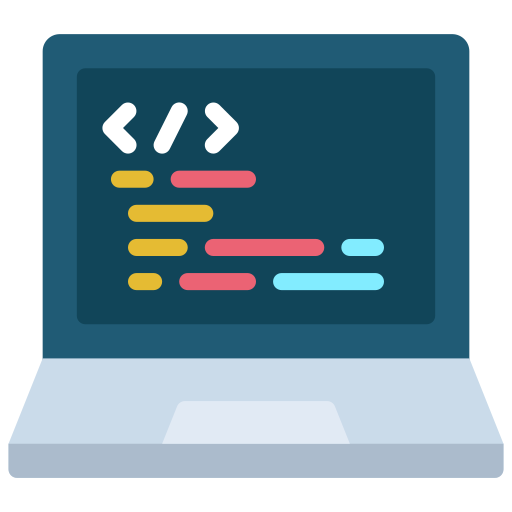
Everything was clear?
Course Content
Java Extended
Java Extended
Class Creation
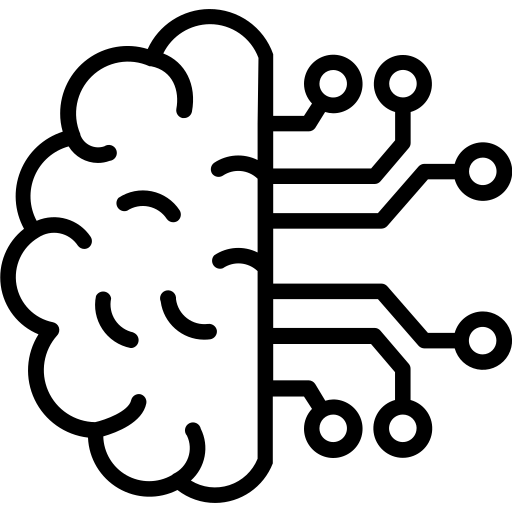
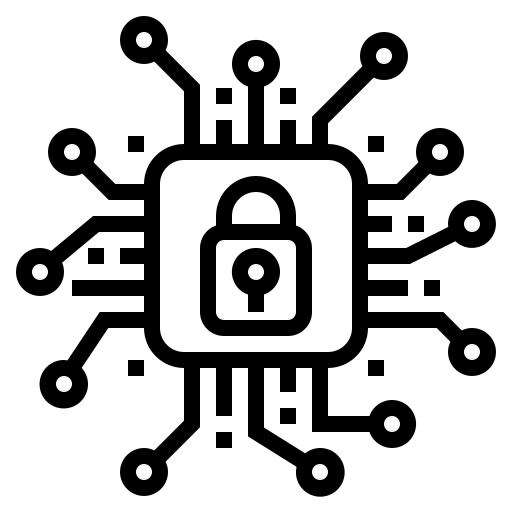
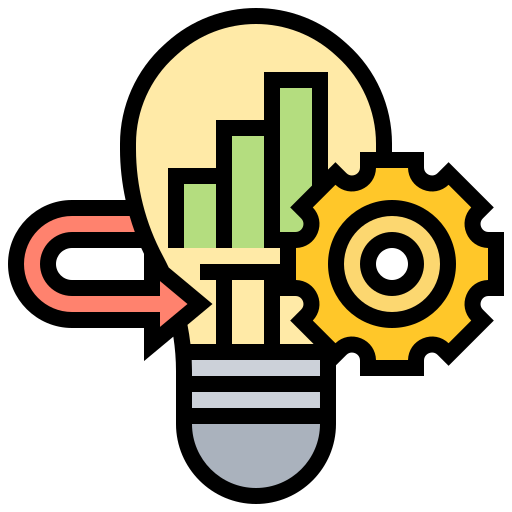
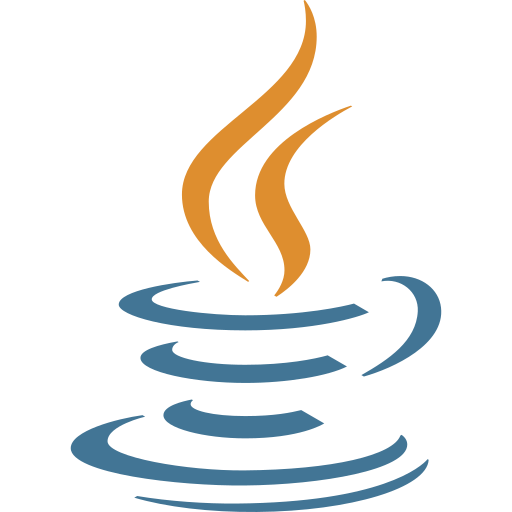
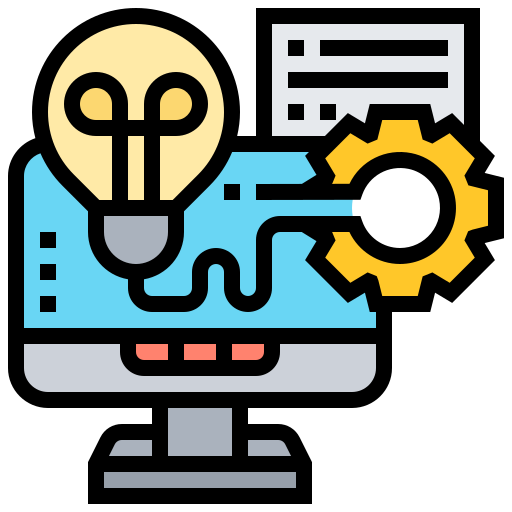
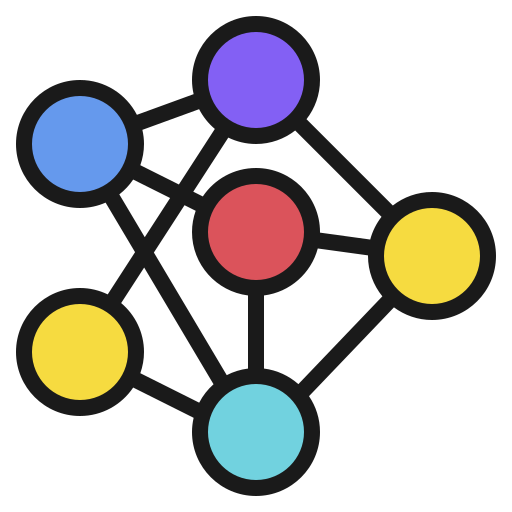
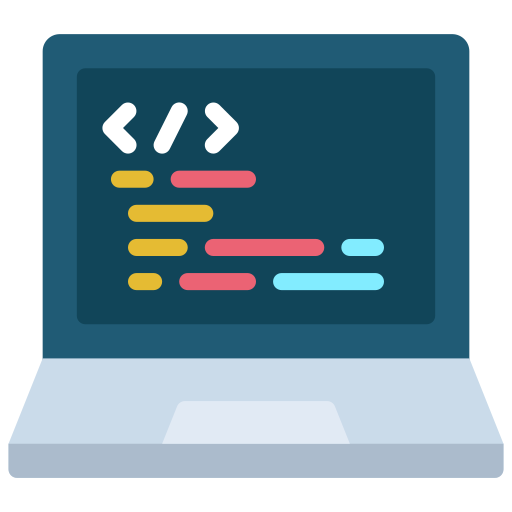
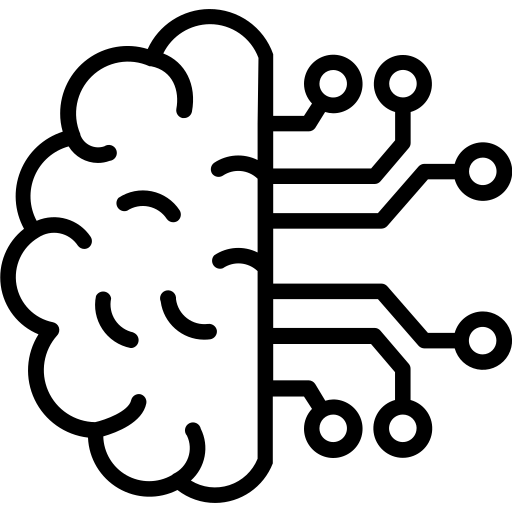
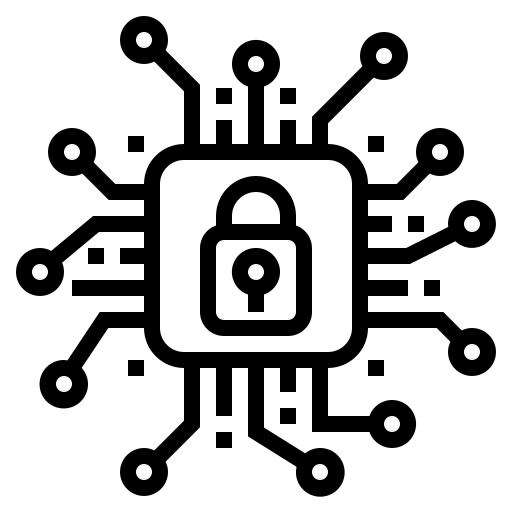
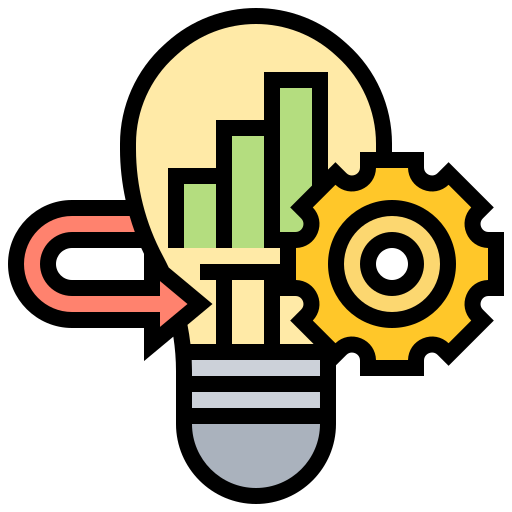
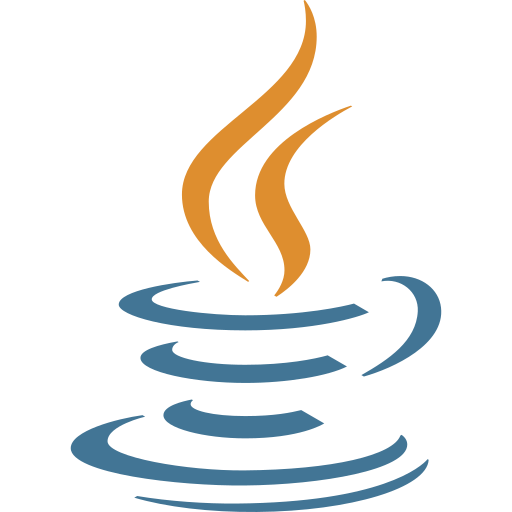
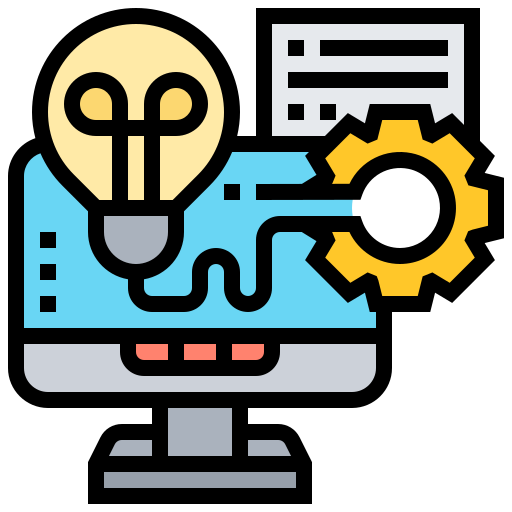
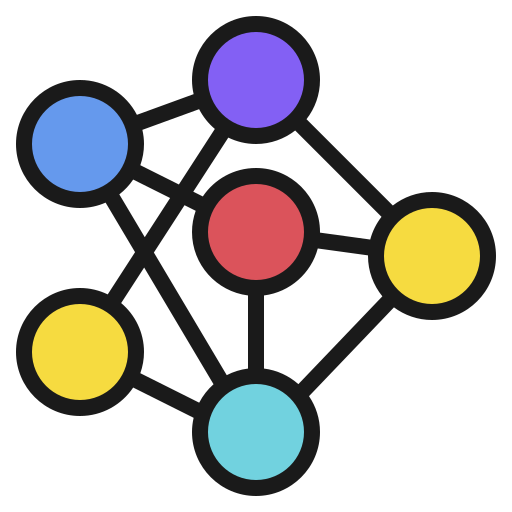
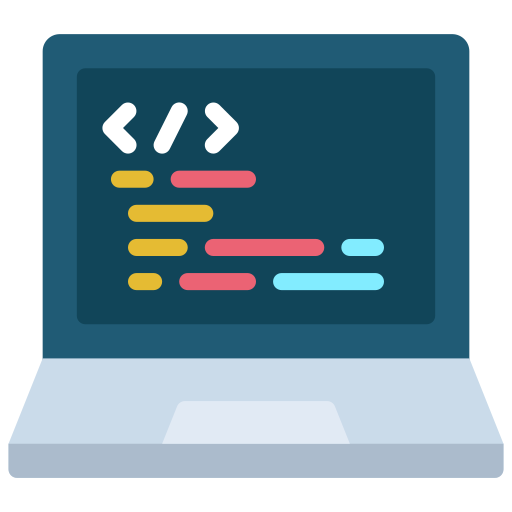
Class Syntax
The syntax for creating a class is quite simple. Let's take a look at an example with the class Person
:
Person.java
This is all it takes to create a class. However, it will be useless to us if it's empty. Therefore, we should add fields to it. Let's assume that our person has a name
, gender
, and age
:
Person.java
And now, let's write a method that allows our Person to report their name and age:
Person.java
Task
Great! Now your task is to create two objects of the Person
class in the main
method. One will be named Bob
and will be 30
years old, and the other will be named Alice
and will be 27
years old. Then, use the introduce()
method on each person to have them introduce themselves.
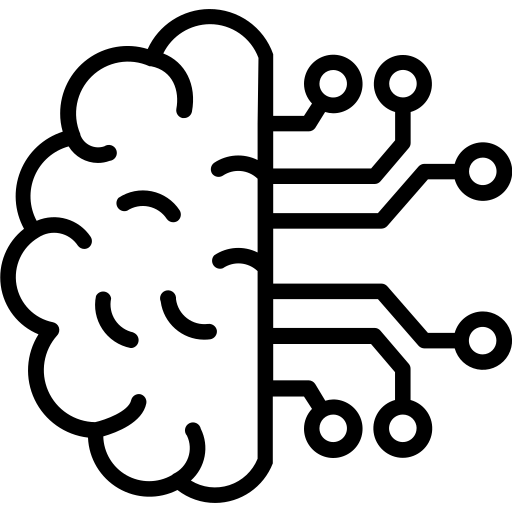
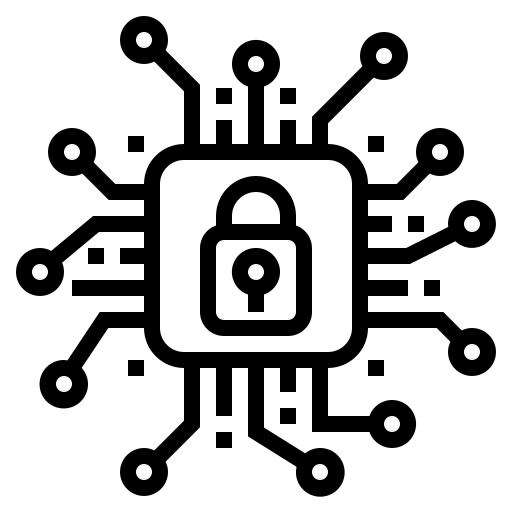
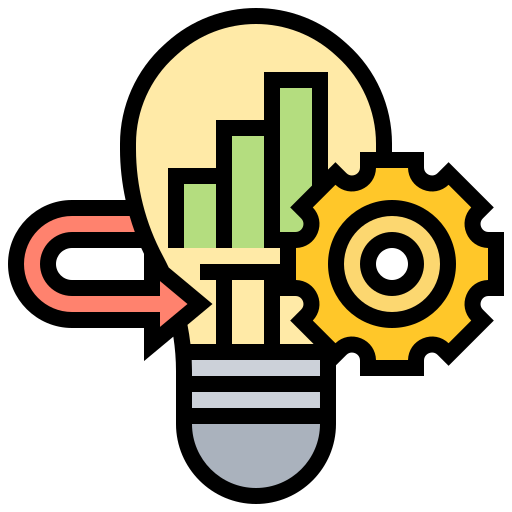
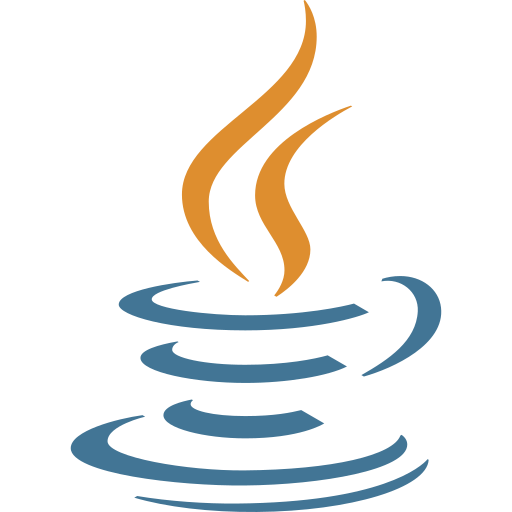
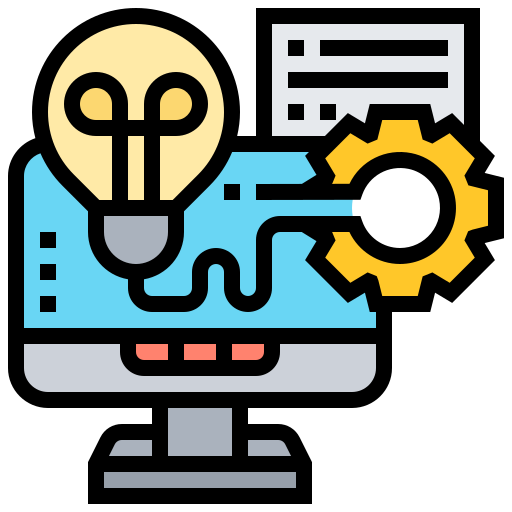
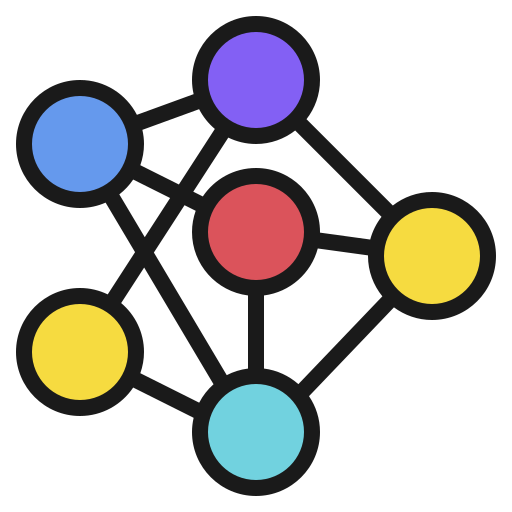
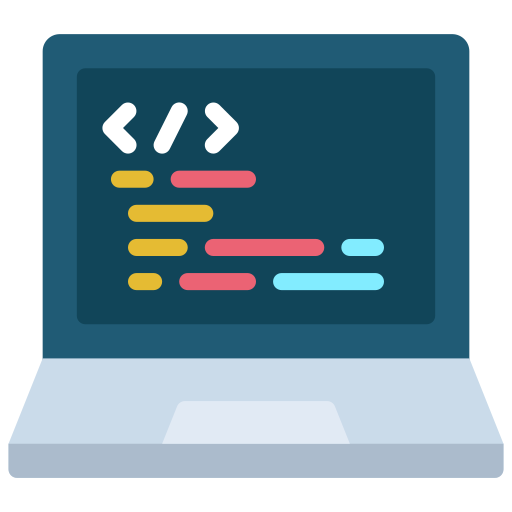
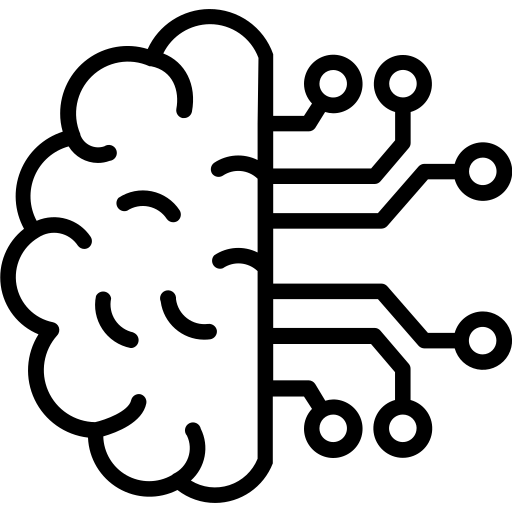
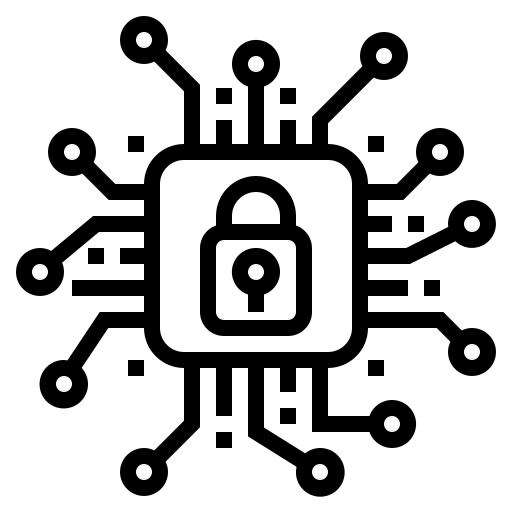
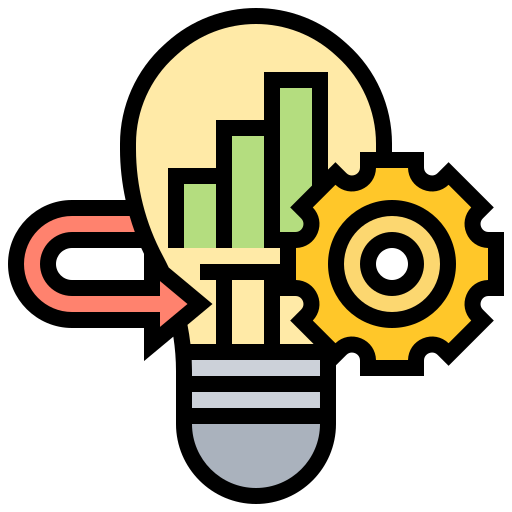
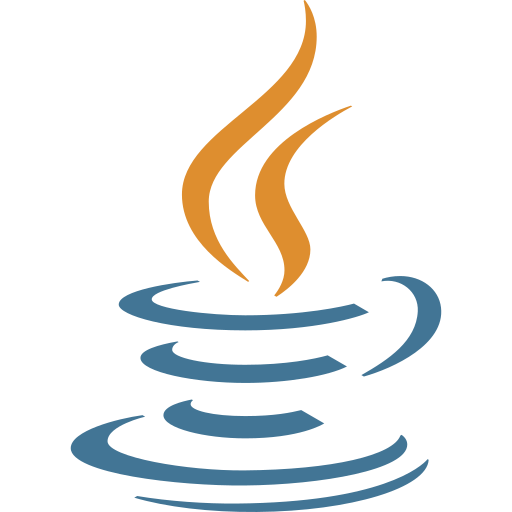
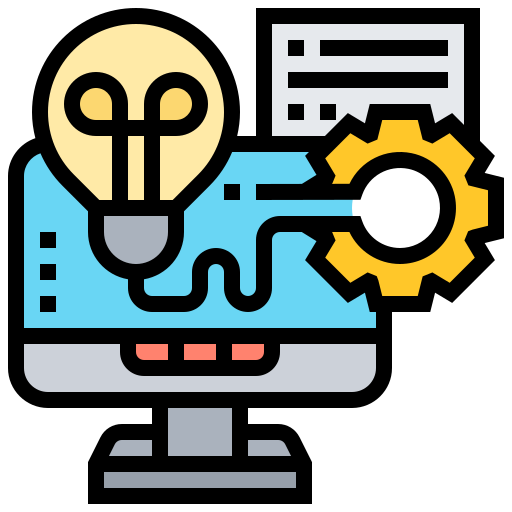
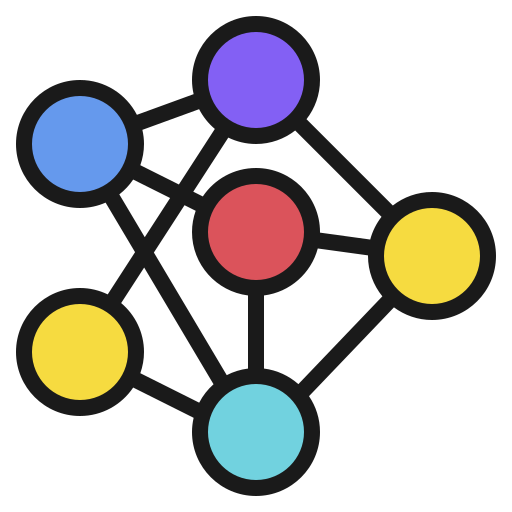
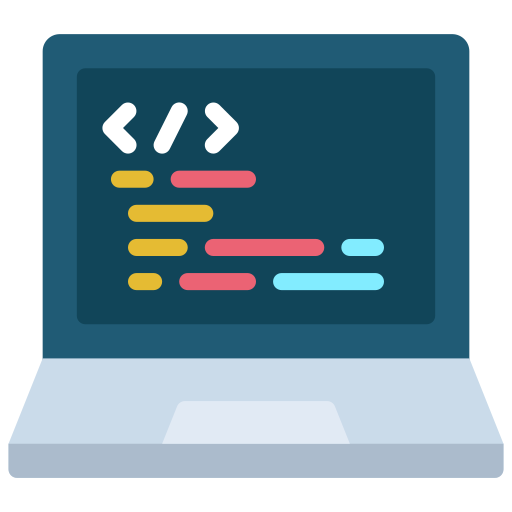
Everything was clear?