Cursos relacionados
Ver Todos os CursosIniciante
C# Basics
Get ready to embark on a thrilling coding journey with C# - the language that powers Windows applications, games, and more. Unlock the potential to build everything from dynamic web apps to powerful desktop software. With its elegance, performance, and versatility, C# is your gateway to the future of programming. Let's dive in and bring your coding dreams to life!
Avançado
Advanced C# with .NET
In this course, we will learn about some advanced C# concepts along with MAUI, which is an application development library. We will learn how to create some interesting GUI applications while keeping them quick and responsive using techniques like Threading. We will make applications that will interact user input and APIs, and apart from that, we will cover some important C# concepts like Reflection and Generics. This course will further improve our programming skills and will give us a strong foundation to move forward towards Web Development.
Intermediário
C# Beyond Basics
This is an intermediate course for C# which helps in leveling up the users skills hence enabling the user to develop more advanced applications employing more complex data structures and techniques like Object Oriented Programming.
Top 25 C# Interview Questions and Answers
Master the Essentials and Ace Your C# Interview
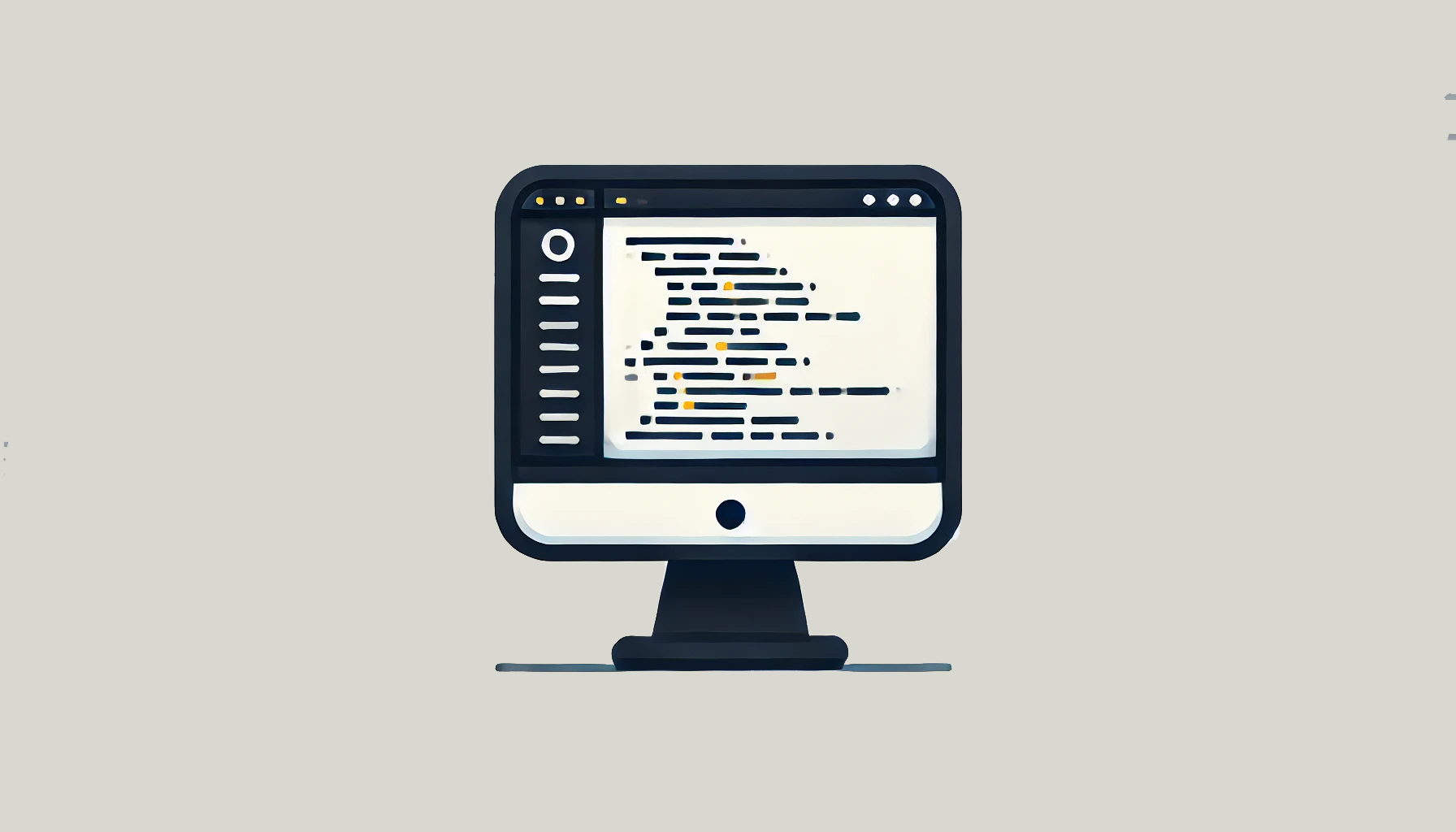
Beginner Questions
1. What is C#?
C# is a modern, object-oriented programming language developed by Microsoft as part of the .NET framework. It's widely used for building applications ranging from Windows desktop software to web services and mobile apps through Xamarin. Known for its simplicity and strong type safety, C# offers features like asynchronous programming, LINQ, and garbage collection, which automates memory management. It also supports interoperability, allowing seamless integration with other languages in the .NET ecosystem. C# runs on multiple platforms, making it a versatile choice for both small and large-scale applications.
2. What are the key features of C#?
Feature | Description |
---|---|
Object-oriented | Focuses on objects and data. |
Type safety | Ensures secure data operations. |
Interoperability | Enables language integration. |
Garbage Collection | Manages memory automatically |
3. What is the difference between C# and .NET?
C# is part of the .NET ecosystem, which provides a comprehensive framework that includes tools, libraries, and runtime environments designed to simplify the development process.
The .NET framework supports multiple programming languages, including C#, enabling developers to create cross-platform applications and access a wide range of features for building secure, scalable, and high-performance software.
4. What is a namespace in C#?
A namespace organizes classes and other types into a hierarchical structure. It prevents naming conflicts.
5. Explain using
in C#.
The using
keyword in C# serves two main purposes. First, it includes namespaces, allowing access to types without needing to specify the full namespace path. For example, using System;
lets you use Console.WriteLine()
instead of System.Console.WriteLine()
. Second, it is used for managing resources by ensuring that objects implementing the IDisposable
interface are properly cleaned up once they are no longer needed, as seen in the using
statement with file streams or database connections.
6. What is the purpose of the static
keyword?
The static
keyword in C# is used to declare members that belong to the type itself, rather than to instances of the type. This means that a static
member can be accessed without creating an instance of the class. It is commonly used for fields, methods, properties, and classes that should be shared across all instances. For example, a static
method can be called directly on the class itself, like ClassName.MethodName()
, without needing to instantiate an object.
7. What is a class in C#?
A class in C# is a blueprint for creating objects. It defines properties and methods that the objects will have.
8. What is the difference between a class and an object?
An object is an instance of a class. It represents a specific entity created based on the class blueprint, with its own set of data.
9. What are access modifiers in C#?
Access modifiers in C# define the visibility and accessibility of classes, methods, and other members. They control how and where the members of a class can be accessed. The most common access modifiers in C# are:
- public: The member is accessible from anywhere.
- private: The member is accessible only within the containing class.
- protected: The member is accessible within the containing class and derived classes.
- internal: The member is accessible within the same assembly (project).
- protected internal: The member is accessible within the same assembly and derived classes.
- private protected: The member is accessible within the same assembly and in derived classes within the same assembly.
10. What is inheritance in C#?
Inheritance in C# is a feature of object-oriented programming that allows a class to inherit properties and methods from another class. This promotes code reusability and establishes a relationship between the parent (base) class and the child (derived) class. The child class can extend or modify the behavior of the parent class.
In C#, inheritance is implemented using the : base
syntax to specify the parent class.
Run Code from Your Browser - No Installation Required
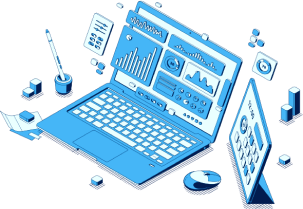
Intermediate Questions
1. What is the difference between abstract classes and interfaces in C#?
In C#, abstract classes and interfaces are used to define contracts, but differ in key ways:
-
Abstract Classes can contain both abstract methods (without implementation) and concrete methods (with implementation). A class can inherit only one abstract class and can have fields, constructors, and other members.
-
Interfaces only declare method signatures and cannot have implementation. A class can implement multiple interfaces, making them ideal for defining common functionality across unrelated classes.
In short, abstract classes are used for shared behavior with some implementation, while interfaces define a contract that can be implemented by any class.
2. Explain the concept of partial classes in C#.
In C#, partial classes allow a class to be split across multiple files. Each part of the class is defined in separate files, but they are all combined into a single class during compilation. This feature is useful for organizing large classes, managing auto-generated code (like in designer files), or for working with code in different teams or modules.
3. What is the purpose of the async and await keywords in C#?
The async
keyword marks a method as asynchronous, and await pauses the method execution until the awaited task is complete. It improves application responsiveness, especially in I/O-bound operations.
4. What is the difference between String and StringBuilder in C#?
Feature | String | StringBuilder |
---|---|---|
Immutability | Immutable (cannot be changed after creation) | Mutable (can be modified after creation) |
Performance | Slower for repeated modifications (creates new objects) | Faster for frequent modifications (modifies in place) |
Use Case | Best for fixed or infrequent changes | Best for scenarios with frequent string changes |
Memory Allocation | Each modification creates a new string object | Modifications happen within the same object |
Thread Safety | Immutable by nature, thread-safe for reading | Not thread-safe unless explicitly handled |
5. What is the difference between IEnumerable and IQueryable?
The difference between IEnumerable
and IQueryable
in C# primarily lies in how they handle data and where the processing occurs:
IEnumerable:
- Works with in-memory collections like arrays, lists, or any other collection that implements
IEnumerable
. - It performs in-memory operations (local processing) on the data.
- When you use LINQ with
IEnumerable
, the query is executed immediately and all data is retrieved from the collection into memory, which might not be efficient for large datasets.
IQueryable:
- Works with remote data sources such as databases (e.g., via LINQ to SQL or Entity Framework).
- It allows for deferred execution and translates the LINQ query into a query that can be executed on the data source (e.g., SQL query for databases).
- Queries are not executed immediately, and only the final result is sent to the server for processing, which is more efficient for large datasets.
6. Explain the concept of extension methods in C#.
Extension methods allow adding methods to existing types without modifying them or using inheritance.
7. What is dependency injection in C#?
Dependency Injection (DI) in C# is a design pattern used to achieve inversion of control (IoC), where a class's dependencies (other objects or services it needs) are provided to it rather than the class creating them itself. This makes the code more flexible, testable, and maintainable.
Instead of a class creating its own dependencies, they are "injected" into it through its constructor, properties, or methods. This way, the class doesn't need to manage its dependencies, and they can be easily replaced with different implementations if needed.
8. What are nullable types in C#, and why are they useful?
Nullable types in C# allow value types (like int
, double
, bool
, etc.) to represent null values. Normally, value types cannot be null
because they always hold a value. However, nullable types provide a way to assign null
to these value types, which is useful when dealing with situations like missing or optional data.
9. What is a sealed class in C#?
A sealed class in C# is a class that cannot be inherited. When a class is marked as sealed
, no other class can derive from it. This is useful when you want to prevent further inheritance, ensuring that the class’s behavior remains unchanged or fixed.
10. What is the difference between const and readonly?
const
fields are evaluated at compile-time, and their values cannot change. readonly
fields are assigned at runtime or in the constructor.
Advanced Questions
1. How does C# handle memory management and garbage collection? Explain the process.
C# uses automatic memory management via the Garbage Collector (GC) in the .NET runtime. The GC handles memory allocation and deallocation for managed objects, ensuring efficient use of resources and preventing memory leaks.
Objects are allocated on the managed heap, divided into three generations:
- Gen 0: Short-lived objects.
- Gen 1: Medium-lived objects.
- Gen 2: Long-lived objects.
The GC identifies unused objects by scanning for root references (e.g., local variables, static fields). Unreferenced objects are marked for removal.
- The GC reclaims memory by freeing unreferenced objects.
- Surviving objects are promoted to higher generations for future collection.
2. How does C# implement covariance and contravariance?
Covariance: Enables assignment of a more derived type to a less derived generic type. (out
keyword).
Contravariance: Enables assignment of a less derived type to a more derived generic type. (in
keyword).
3. Difference Between Assembly.Load
and Assembly.LoadFrom
Assembly.Load
loads an assembly by its fully qualified name from the Global Assembly Cache (GAC) or application base directory. It does not require a file path and is ideal when the assembly’s location is predictable.
Assembly.LoadFrom
, on the other hand, loads an assembly from a specific file path. It is useful for loading assemblies located outside standard paths, like plugins. However, it may lead to issues if the same assembly is loaded from multiple locations, as each instance is treated as distinct.
In summary, Assembly.Load
is name-based, while Assembly.LoadFrom
is path-based, with the latter offering more flexibility but requiring careful usage.
4. How the lock Statement Prevents Deadlocks in C#
The lock
statement in C# ensures that only one thread can execute a critical section of code at a time, preventing race conditions. However, preventing deadlocks—situations where two or more threads are waiting indefinitely for resources held by each other—requires careful usage and design considerations.
The lock
statement itself does not inherently prevent deadlocks. Instead, developers must follow certain best practices when using lock
to avoid creating conditions that could lead to deadlocks.
5. What is the Span type, and when should it be used?
Span<T>
provides a memory-safe, high-performance view of contiguous memory without allocations, useful for slicing arrays or buffers.
Start Learning Coding today and boost your Career Potential
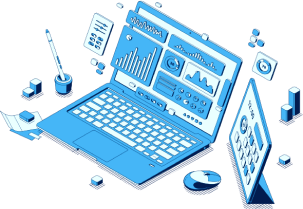
FAQs
Q: Can I use C# for mobile application development?
A: Yes, frameworks like Xamarin enable mobile development with C#.
Q: What is the role of Visual Studio in C# development?
A: Visual Studio is an Integrated Development Environment (IDE) for C#.
Q: Is C# open-source?
A: Yes, the core parts of the .NET framework are open-source.
Q: Can I use C# for web development?
A: Yes, ASP.NET allows developers to build robust web applications.
Q: What are some alternatives to C#?
A: Alternatives include Java, Python, and C++ for various use cases.
Cursos relacionados
Ver Todos os CursosIniciante
C# Basics
Get ready to embark on a thrilling coding journey with C# - the language that powers Windows applications, games, and more. Unlock the potential to build everything from dynamic web apps to powerful desktop software. With its elegance, performance, and versatility, C# is your gateway to the future of programming. Let's dive in and bring your coding dreams to life!
Avançado
Advanced C# with .NET
In this course, we will learn about some advanced C# concepts along with MAUI, which is an application development library. We will learn how to create some interesting GUI applications while keeping them quick and responsive using techniques like Threading. We will make applications that will interact user input and APIs, and apart from that, we will cover some important C# concepts like Reflection and Generics. This course will further improve our programming skills and will give us a strong foundation to move forward towards Web Development.
Intermediário
C# Beyond Basics
This is an intermediate course for C# which helps in leveling up the users skills hence enabling the user to develop more advanced applications employing more complex data structures and techniques like Object Oriented Programming.
8 In-Demand Skills to Earn Big in 2025
Skills to stay ahead
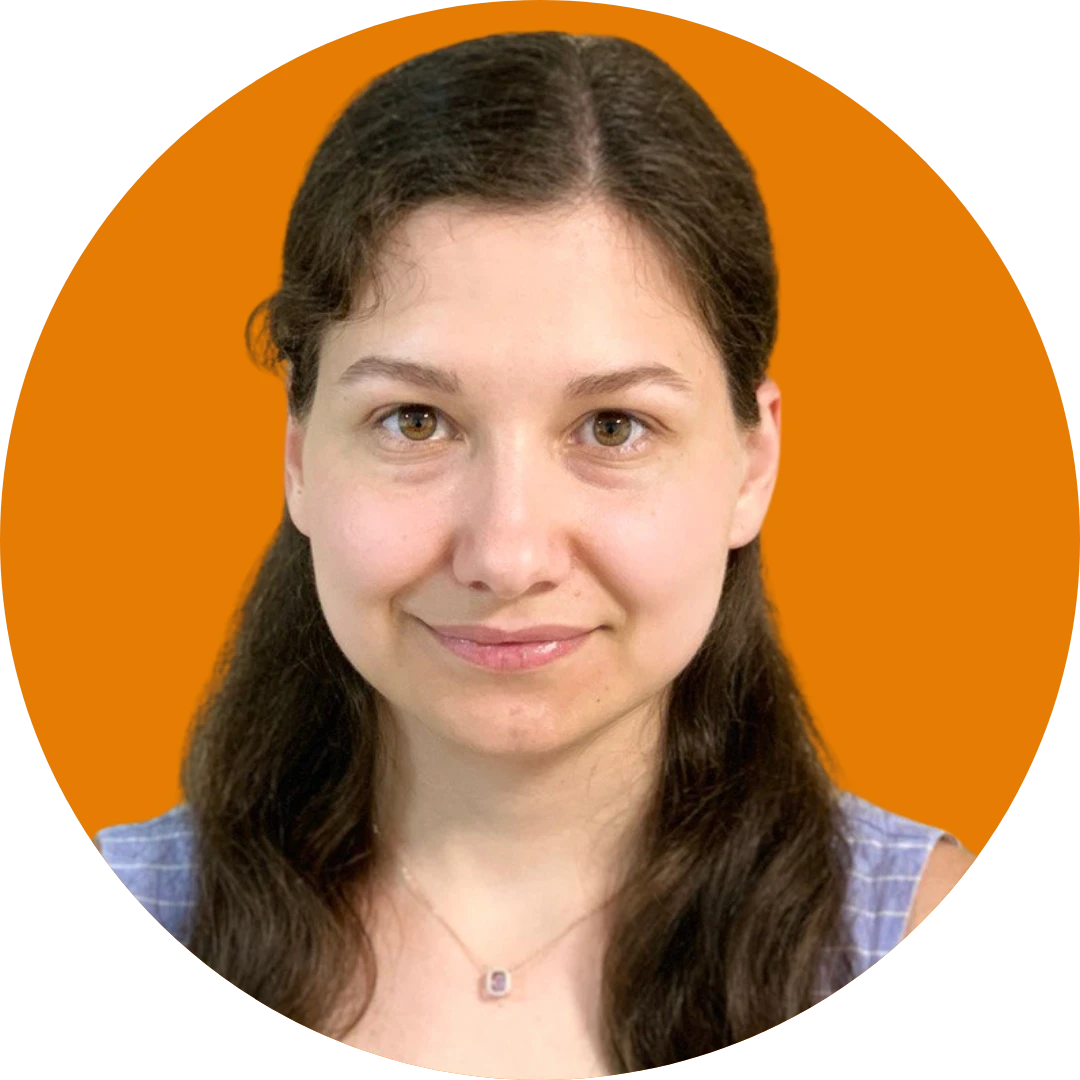
by Anastasiia Tsurkan
Backend Developer
Dec, 2024・23 min read

Top 5 IT Profession Challenges and How to Overcome Them
Navigating Though the Complexities of The Job
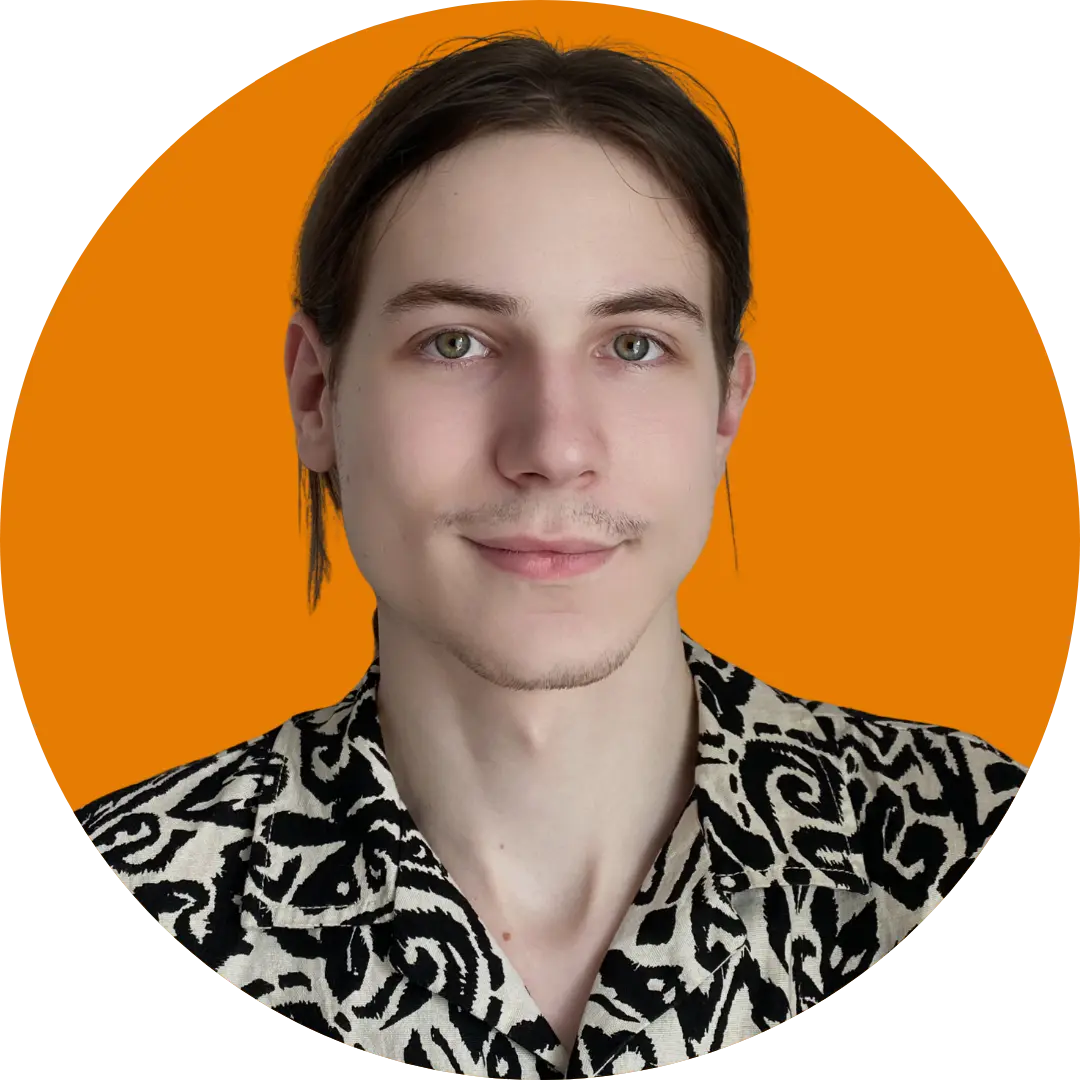
by Ihor Gudzyk
C++ Developer
Dec, 2023・5 min read
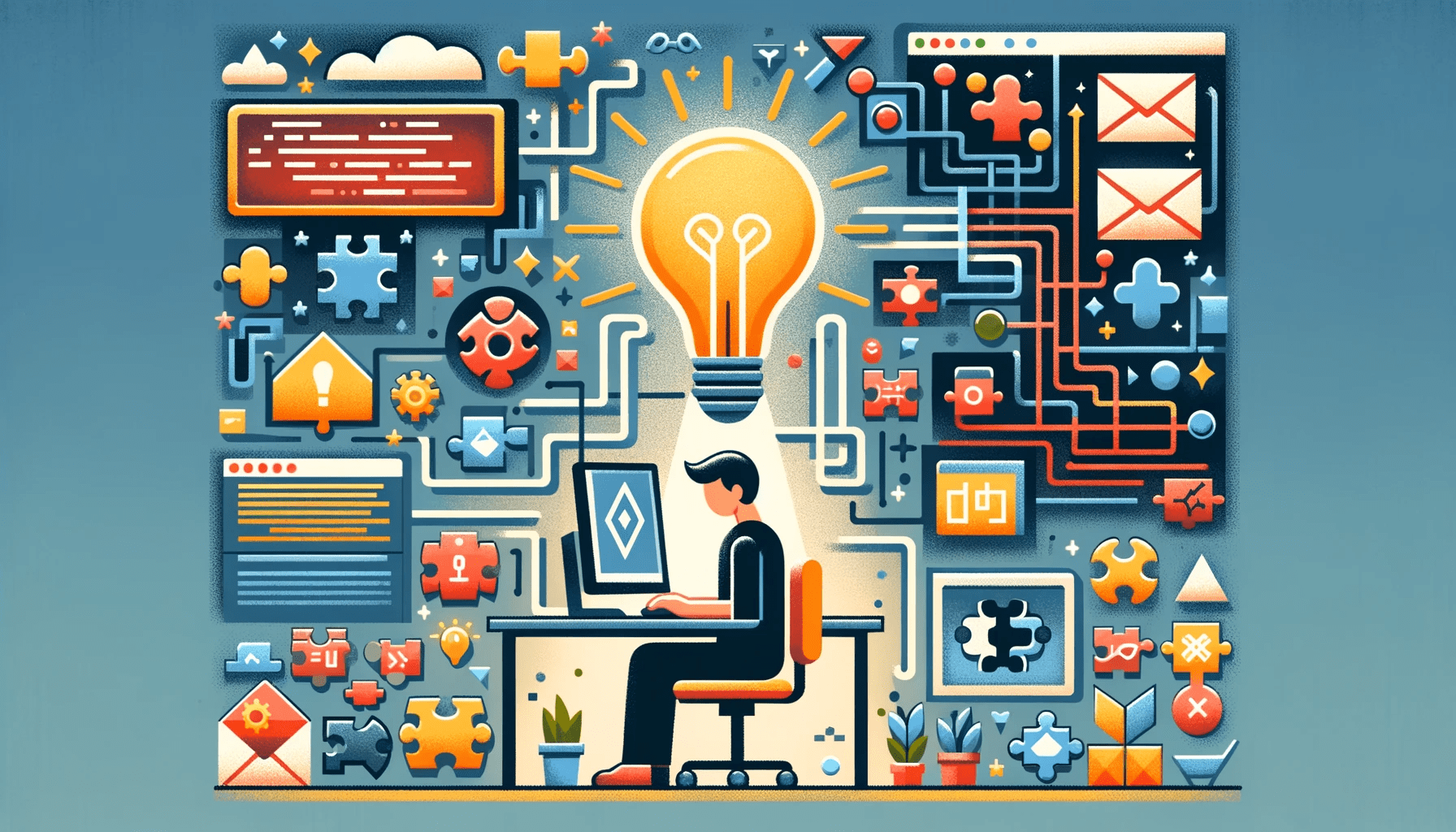
Why IT Companies Prefer Older Versions of Java
Understanding the Timeless Appeal of Established Java Editions
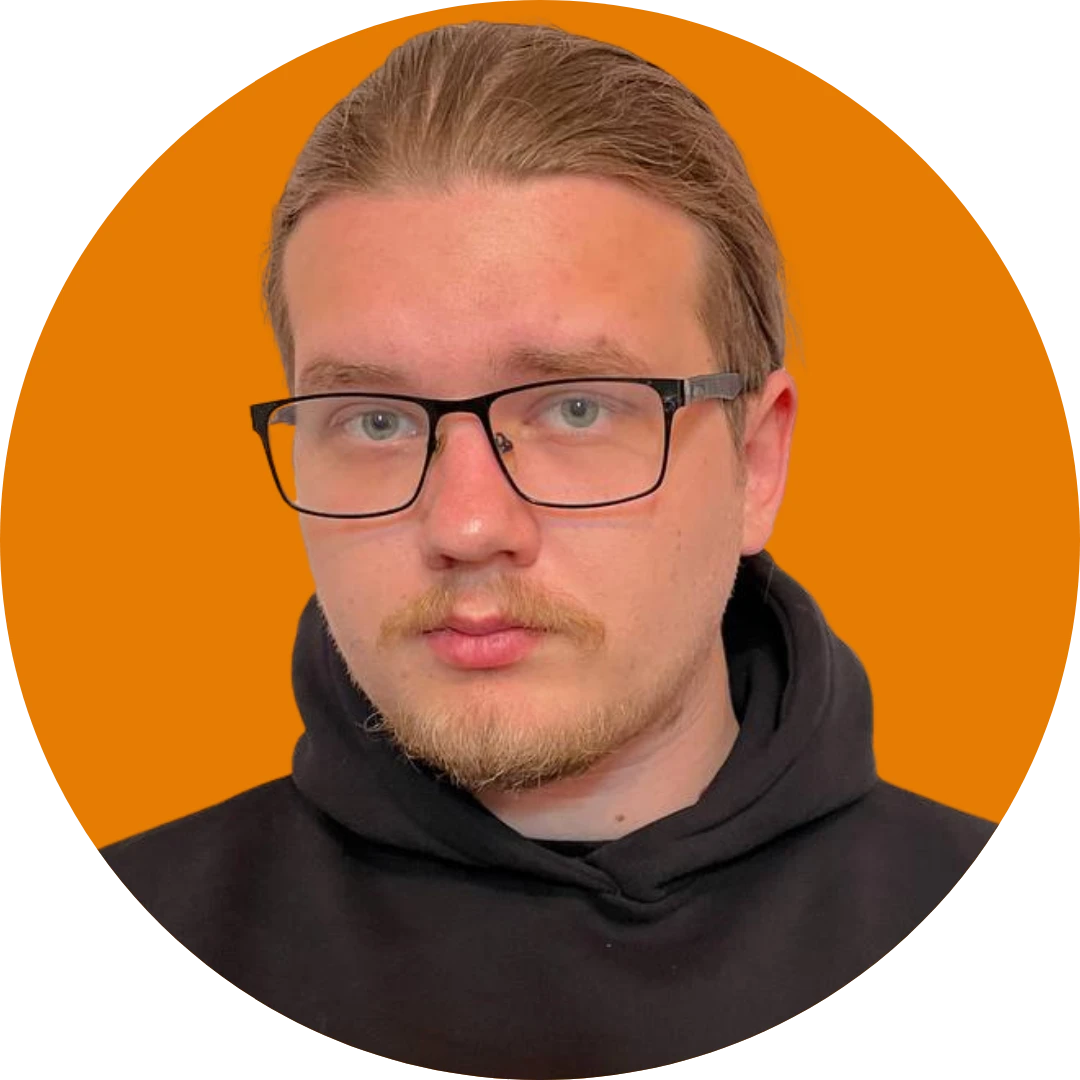
by Daniil Lypenets
Full Stack Developer
Dec, 2023・4 min read
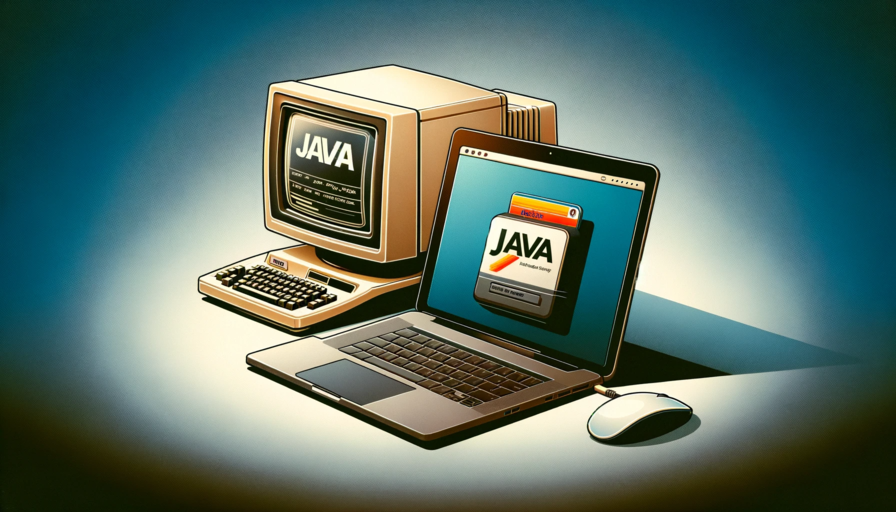
Conteúdo deste artigo