Task - Creating a Custom Event
The base program is given on the Github Repository. Clone the repository to begin solving the task.
We are aiming to make a program which displays a random image received from an API whenever the user clicks the "Get Image" button.
The program retrieves a link of a random image from the API when the user clicks the "Get Image" button, and sets the "Source" property of the "mainImage" to that retrieved link.
The GetImage
event handler method is binded to the Clicked
EventHandler of the button. So whenever the user clicks the button, the program executes that method and makes a get request to the URL https://random.imagecdn.app/v1/image
.
When the method receives a response from the API, it extracts the content from the response - which is expected to be a raw URL (link) of an image. And it passes that as a string into the OnApiResponse
method which is to be defined by you.
The OnApiResponse
method should execute the EventHandler of the apiEvent class which you're going to create. Moreover, it should pass the "content" forward into the event handler methods as an argument and for that you can use the apiEventArgs
which has already been defined for you. You can store the content in the Link
property of a new apiEventArgs
instance and pass it as a second argument to the event handler execution.
So this will be the who flow of the program:
- The user clicks Get Image;
- Program makes a GET request;
- The API responds with the URL of a random image;
- The URL is passed as a
string
toOnApiResponse
method of theapiEvent
class; - The EventHandler of
apiEvent
class is invoked, in turn invoking all binded methods (event handlers); - The URL is sent the binded Event Handlers, hence invoking the SetImage method;
- The image is shown on the screen;
Learning Objective:
You might be wondering, what was the need for creating this seemingly unnecessary "cycle". We could've simply written mainImage.Source = content
directly inside the GetImage
method where the API response is received, and by doing that we could've avoided creating the whole Event System around the API call. Moreover, In that case the program execution and code would've been much shorter:
- The user clicks Get Image;
- Program makes a GET request;
- The API responds with a URL of a random image;
- The new image is shown on the screen;
However one benefit of creating the apiEvent
is that we can use this event globally in the application. Which allows us to bind methods to the Event Handler of this event from multiple different external classes.
You basically have to replicate the "Publisher" class from the following diagram:
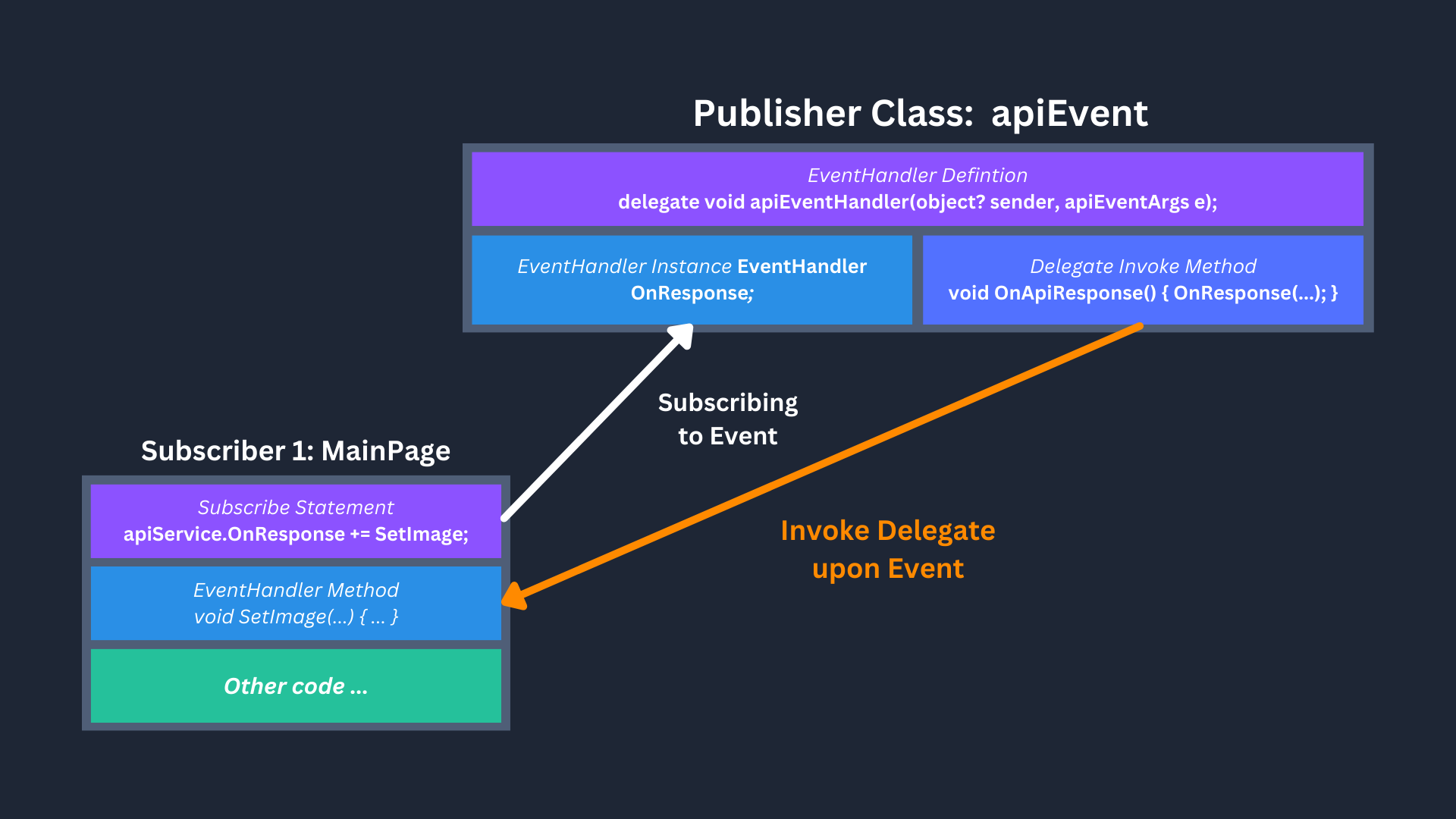
Your Task
Following is a concise description of your task:
- Create a new public class called
apiEvent
- Create a delegate type called
apiEventHandler
which can acceptapiEventArgs
as a second argument. - Create a public delegate using the recently defined delegate type. Call it
OnResponse
. - Create a delegate invoker method called
OnApiResponse
and it should accept onestring
argument. You may name the argument as "content". - Inside
OnApiResponse
, invoke the delegate if it's not null and pass the value of "content" to the event handler methods by using theapiEventArgs
method.
public delegate void delegateTypeName(object sender, EventArgs e);
Hint 2: You can pass the "content" as an argument while invoking the delegate by creating a new instance of the
apiEventArgs
class and passing the content into it's constructor, then in turn passing the instance of apiEventArgs
into the delegates execution: handler(this, new apiEventArgs(content));
using Microsoft.Maui.Controls.Shapes;
using System.Globalization;
using System.Net.Http;
namespace DataBindingPractice1
{
public class apiEvent
{
public delegate void apiEventHandler(object sender, apiEventArgs e);
public event apiEventHandler? OnResponse;
public void OnApiResponse(string content)
{
apiEventHandler? handler = OnResponse;
if (handler != null)
{
handler(this, new apiEventArgs(content));
}
}
}
public class apiEventArgs : EventArgs
{
public string Link { get; }
public apiEventArgs(string content)
{
this.Link = content;
}
}
public partial class MainPage : ContentPage
{
public apiEvent apiService = new apiEvent();
public MainPage()
{
InitializeComponent();
getImageButton.Clicked += GetImage;
apiService.OnResponse += SetImage;
}
private void SetImage(object? sender, apiEventArgs e)
{
mainImage.Source = e.Link;
}
private void GetImage(object? sender, EventArgs e)
{
string apiUrl = "https://random.imagecdn.app/v1/image";
using (HttpClient client = new HttpClient())
{
HttpResponseMessage response = client.GetAsync(apiUrl).Result;
if (response.IsSuccessStatusCode)
{
string content = response.Content.ReadAsStringAsync().Result;
apiService.OnApiResponse(content);
}
}
}
}
}
Все було зрозуміло?
Зміст курсу
Advanced C# with .NET
1. Introduction to Desktop Development with .NET
Advanced C# with .NET
Task - Creating a Custom Event
The base program is given on the Github Repository. Clone the repository to begin solving the task.
We are aiming to make a program which displays a random image received from an API whenever the user clicks the "Get Image" button.
The program retrieves a link of a random image from the API when the user clicks the "Get Image" button, and sets the "Source" property of the "mainImage" to that retrieved link.
The GetImage
event handler method is binded to the Clicked
EventHandler of the button. So whenever the user clicks the button, the program executes that method and makes a get request to the URL https://random.imagecdn.app/v1/image
.
When the method receives a response from the API, it extracts the content from the response - which is expected to be a raw URL (link) of an image. And it passes that as a string into the OnApiResponse
method which is to be defined by you.
The OnApiResponse
method should execute the EventHandler of the apiEvent class which you're going to create. Moreover, it should pass the "content" forward into the event handler methods as an argument and for that you can use the apiEventArgs
which has already been defined for you. You can store the content in the Link
property of a new apiEventArgs
instance and pass it as a second argument to the event handler execution.
So this will be the who flow of the program:
- The user clicks Get Image;
- Program makes a GET request;
- The API responds with the URL of a random image;
- The URL is passed as a
string
toOnApiResponse
method of theapiEvent
class; - The EventHandler of
apiEvent
class is invoked, in turn invoking all binded methods (event handlers); - The URL is sent the binded Event Handlers, hence invoking the SetImage method;
- The image is shown on the screen;
Learning Objective:
You might be wondering, what was the need for creating this seemingly unnecessary "cycle". We could've simply written mainImage.Source = content
directly inside the GetImage
method where the API response is received, and by doing that we could've avoided creating the whole Event System around the API call. Moreover, In that case the program execution and code would've been much shorter:
- The user clicks Get Image;
- Program makes a GET request;
- The API responds with a URL of a random image;
- The new image is shown on the screen;
However one benefit of creating the apiEvent
is that we can use this event globally in the application. Which allows us to bind methods to the Event Handler of this event from multiple different external classes.
You basically have to replicate the "Publisher" class from the following diagram:
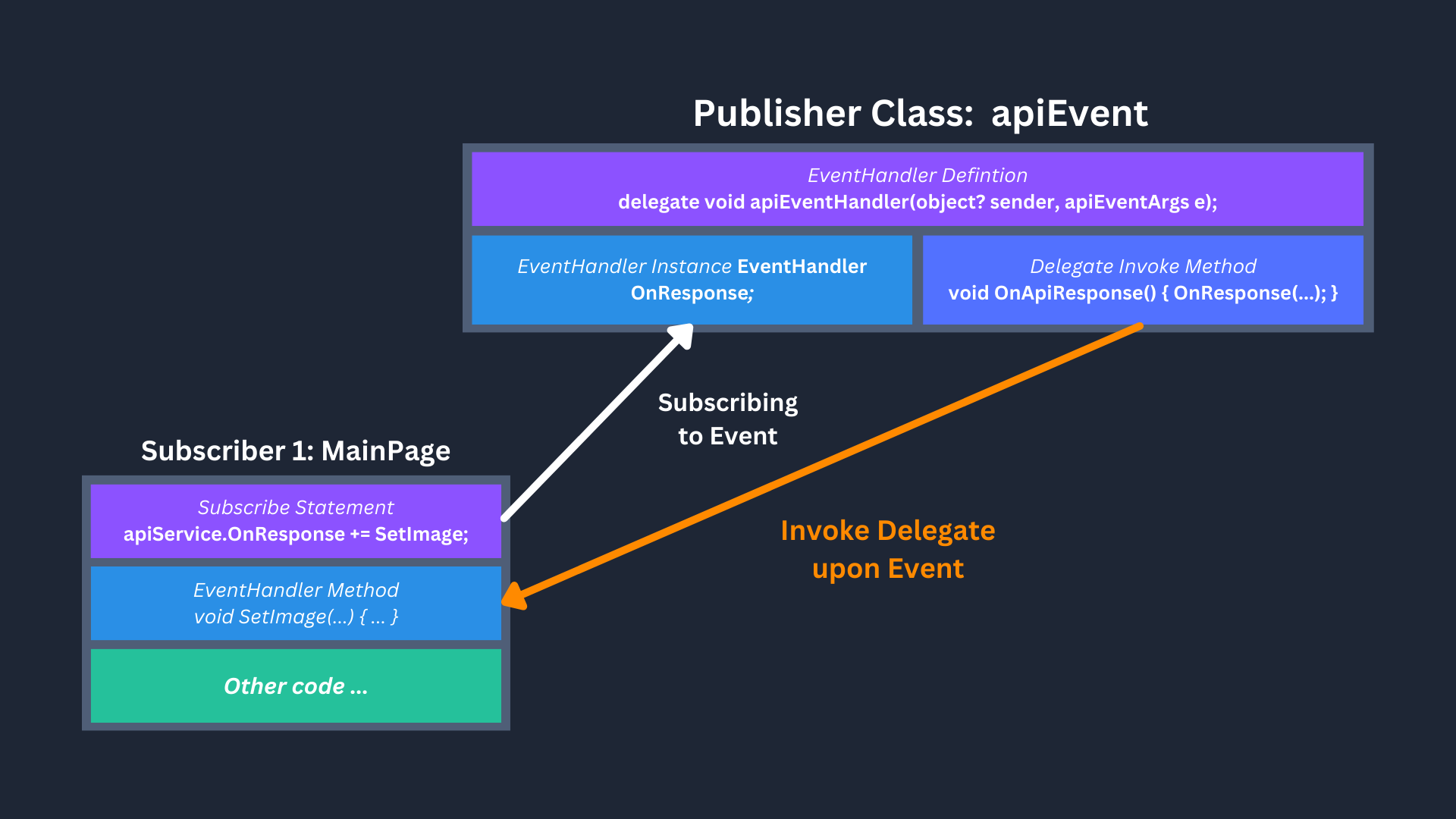
Your Task
Following is a concise description of your task:
- Create a new public class called
apiEvent
- Create a delegate type called
apiEventHandler
which can acceptapiEventArgs
as a second argument. - Create a public delegate using the recently defined delegate type. Call it
OnResponse
. - Create a delegate invoker method called
OnApiResponse
and it should accept onestring
argument. You may name the argument as "content". - Inside
OnApiResponse
, invoke the delegate if it's not null and pass the value of "content" to the event handler methods by using theapiEventArgs
method.
public delegate void delegateTypeName(object sender, EventArgs e);
Hint 2: You can pass the "content" as an argument while invoking the delegate by creating a new instance of the
apiEventArgs
class and passing the content into it's constructor, then in turn passing the instance of apiEventArgs
into the delegates execution: handler(this, new apiEventArgs(content));
using Microsoft.Maui.Controls.Shapes;
using System.Globalization;
using System.Net.Http;
namespace DataBindingPractice1
{
public class apiEvent
{
public delegate void apiEventHandler(object sender, apiEventArgs e);
public event apiEventHandler? OnResponse;
public void OnApiResponse(string content)
{
apiEventHandler? handler = OnResponse;
if (handler != null)
{
handler(this, new apiEventArgs(content));
}
}
}
public class apiEventArgs : EventArgs
{
public string Link { get; }
public apiEventArgs(string content)
{
this.Link = content;
}
}
public partial class MainPage : ContentPage
{
public apiEvent apiService = new apiEvent();
public MainPage()
{
InitializeComponent();
getImageButton.Clicked += GetImage;
apiService.OnResponse += SetImage;
}
private void SetImage(object? sender, apiEventArgs e)
{
mainImage.Source = e.Link;
}
private void GetImage(object? sender, EventArgs e)
{
string apiUrl = "https://random.imagecdn.app/v1/image";
using (HttpClient client = new HttpClient())
{
HttpResponseMessage response = client.GetAsync(apiUrl).Result;
if (response.IsSuccessStatusCode)
{
string content = response.Content.ReadAsStringAsync().Result;
apiService.OnApiResponse(content);
}
}
}
}
}
Все було зрозуміло?