Related courses
See All CoursesBeginner
Introduction to JavaScript
Learn the fundamentals of JavaScript, the backbone of dynamic web development. Discover essential concepts like syntax, variables, data types, and operators. Explore how to use conditional statements, loops, and functions to create interactive and efficient programs. Master the building blocks of JavaScript and lay the groundwork for more advanced programming skills.
Intermediate
JavaScript Data Structures
Discover the foundational building blocks of JavaScript. Immerse yourself in objects, arrays, and crucial programming principles, becoming proficient in the fundamental structures that form the basis of contemporary web development.
Error Handling with Async Await in JavaScript
Essential Techniques for Async Error Handling in JavaScript Applications
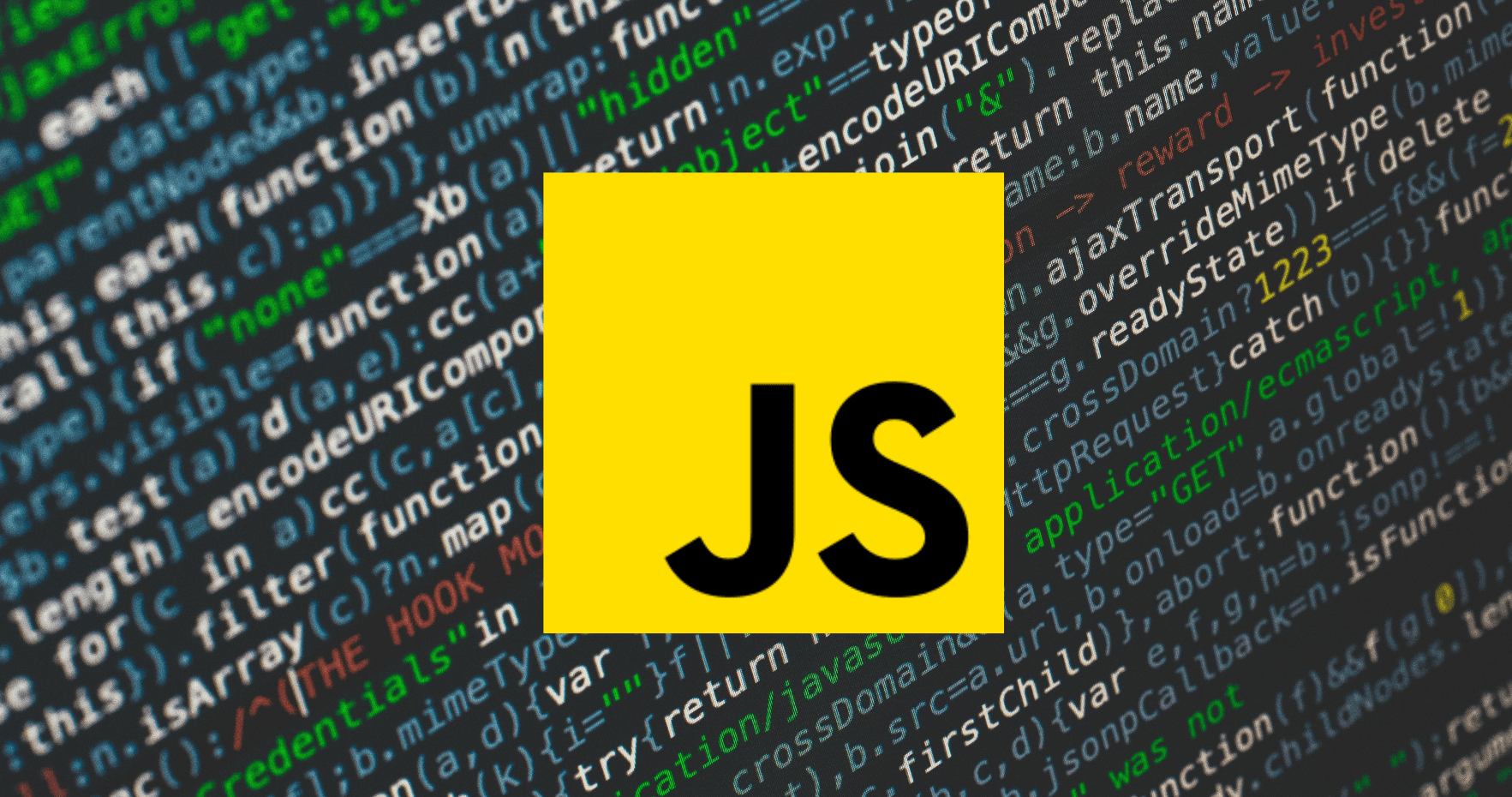
Error handling is essential in JavaScript, especially when working with asynchronous code. The async/await
syntax makes async code more readable, but handling errors effectively is key to ensuring your app doesn't fail unexpectedly.
Basic Error Handling with try-catch
The most common way to handle errors in async functions is by using a try-catch
block. If a Promise rejects or throws an error, it's caught in the catch
block, preventing your app from crashing.
js
Here, try
protects the fetch
call. If the network request fails, the error is caught, logged, and a null
fallback value is returned.
Run Code from Your Browser - No Installation Required
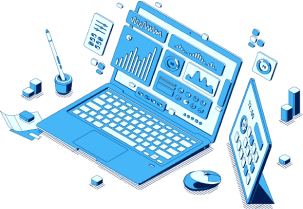
Using .catch() with Async Functions
Instead of using try-catch
inside an async function, you can handle errors by chaining a .catch()
method directly on the function call. This approach is useful when you don't need to handle errors within the function itself.
js
fetchData()
might throw an error if fetch
fails. Using .catch()
directly on the call helps catch errors without needing try-catch
inside fetchData()
.
Handling Errors in Multiple Async Operations
When you need to perform multiple async operations, you can use Promise.all()
to run them in parallel. However, if any operation fails, Promise.all()
will throw an error, stopping all other operations. Promise.allSettled()
allows each operation to complete independently, even if one fails.
js
Promise.allSettled
waits for both requests to complete, logging any errors without interrupting the other operations.
Retrying Failed Requests
Sometimes, a network request might fail due to temporary issues. A simple retry mechanism can improve reliability by trying the request again if it fails initially.
js
The function retries up to 3 times, logging each failure and retrying if it hasn't reached the maximum attempts. If all attempts fail, it throws the error.
Start Learning Coding today and boost your Career Potential
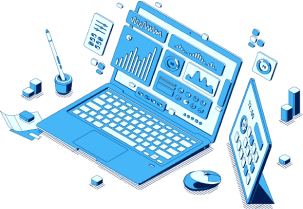
Setting Up Timeouts for Long Requests
Sometimes, a request might hang indefinitely. AbortController
lets you cancel a request if it takes too long, which is helpful for handling timeouts.
js
If the request exceeds the timeout
, it's canceled by AbortController
, logging a timeout message. This technique prevents long or unresponsive requests from blocking the app.
Conclusion
In JavaScript, handling errors with async/await
can be made simpler using these techniques:
try-catch
: catch errors within async functions, providing clear fallbacks or error messages;.catch()
: chain.catch()
for error handling outside the function;- Handling Multiple Async Operations: use
Promise.allSettled
to run operations in parallel without stopping on failure; - Retry Mechanism: retry requests in case of temporary failures;
- Timeouts: use
AbortController
to cancel requests that take too long, improving app performance.
These methods provide a foundation for managing errors effectively in async JavaScript code, making applications more resilient and user-friendly.
FAQs
Q: What is the best way to handle errors in async/await functions?
A: The most common approach is using try-catch
blocks within async functions to handle errors directly. For errors that should be managed outside the function, chaining .catch()
after calling the function can be effective.
Q: How does Promise.allSettled
differ from Promise.all
in handling errors?
A: Promise.all
will immediately reject if any Promise in the group fails, stopping all other operations. Promise.allSettled
waits for all Promises to complete, regardless of success or failure, allowing each result to be handled individually.
Q: When should I implement a retry mechanism for failed requests?
A: Retry mechanisms are beneficial for handling temporary issues like network instability or server overload. For critical API calls, using retries with exponential backoff enhances resilience, improving the chances of success without overwhelming the server.
Q: How can I set a timeout for a request in JavaScript?
A: You can use AbortController
to set a timeout for async requests, allowing you to cancel any requests that exceed a specified time limit and prevent blocking or hanging the app.
Q: Is error handling with async/await better than traditional Promises?
A: async/await
often makes error handling easier and more readable with try-catch
, especially in complex async flows. While async/await
simplifies code, both approaches have their uses depending on the scenario.
Related courses
See All CoursesBeginner
Introduction to JavaScript
Learn the fundamentals of JavaScript, the backbone of dynamic web development. Discover essential concepts like syntax, variables, data types, and operators. Explore how to use conditional statements, loops, and functions to create interactive and efficient programs. Master the building blocks of JavaScript and lay the groundwork for more advanced programming skills.
Intermediate
JavaScript Data Structures
Discover the foundational building blocks of JavaScript. Immerse yourself in objects, arrays, and crucial programming principles, becoming proficient in the fundamental structures that form the basis of contemporary web development.
Accidental Innovation in Web Development
Product Development
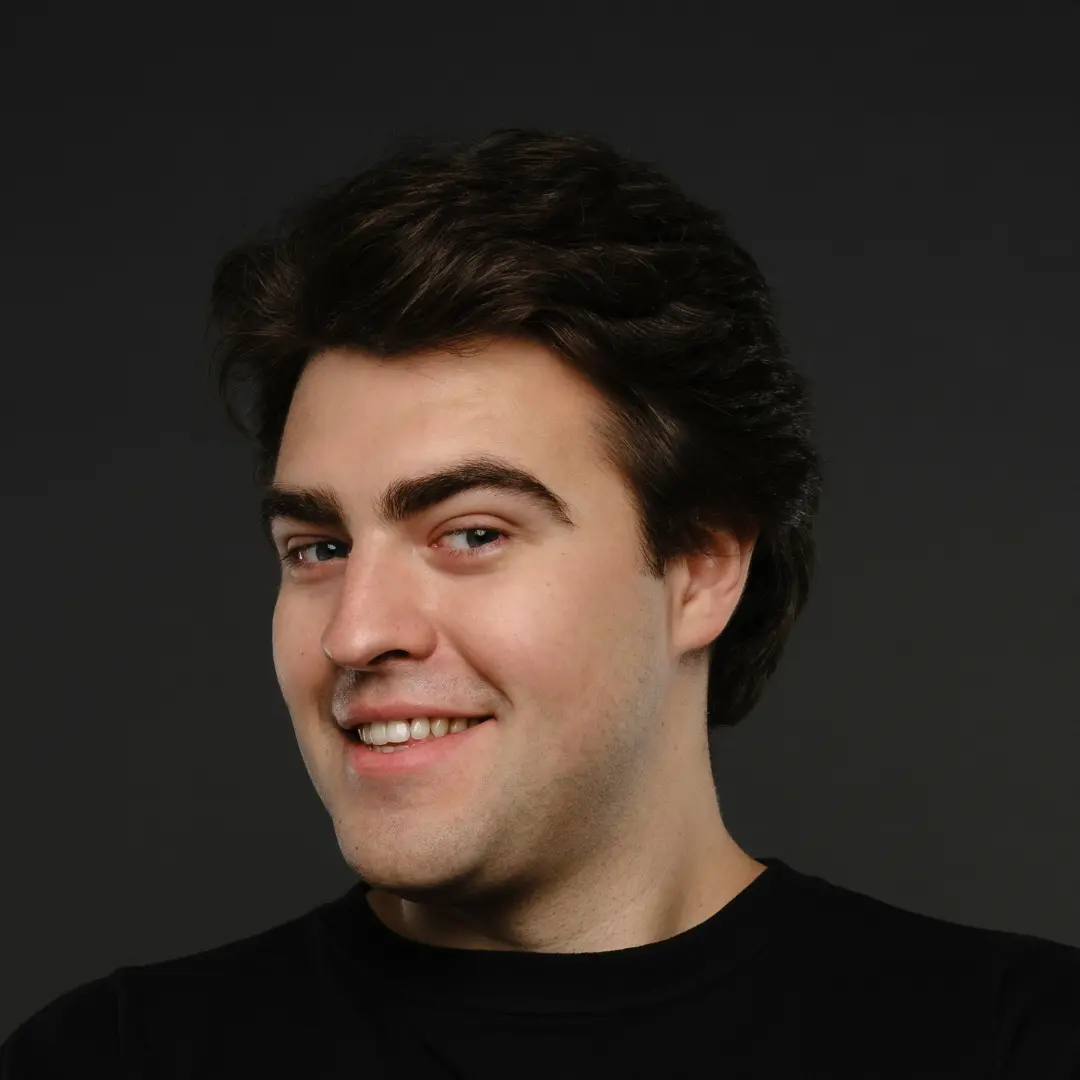
by Oleh Subotin
Full Stack Developer
May, 2024γ»5 min read
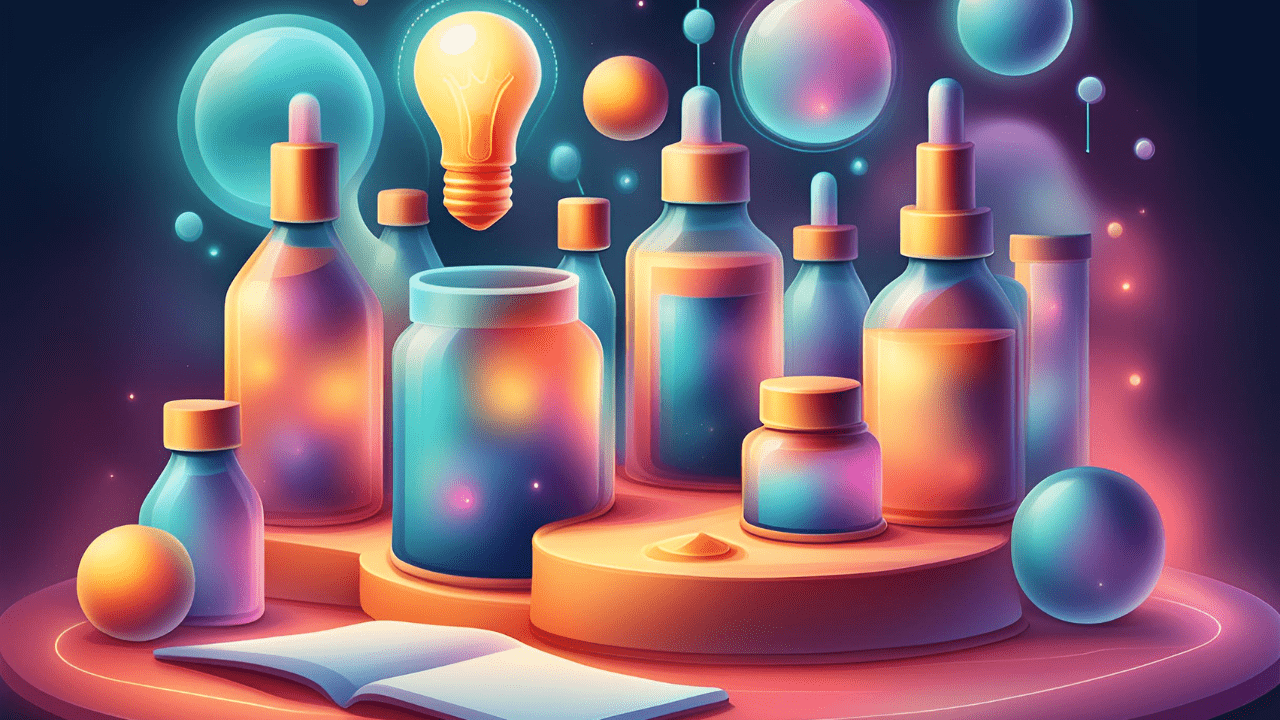
Navigating the YouTube Influencer Maze in Your Tech Career
Staying Focused and Disciplined Amidst Overwhelming Advice
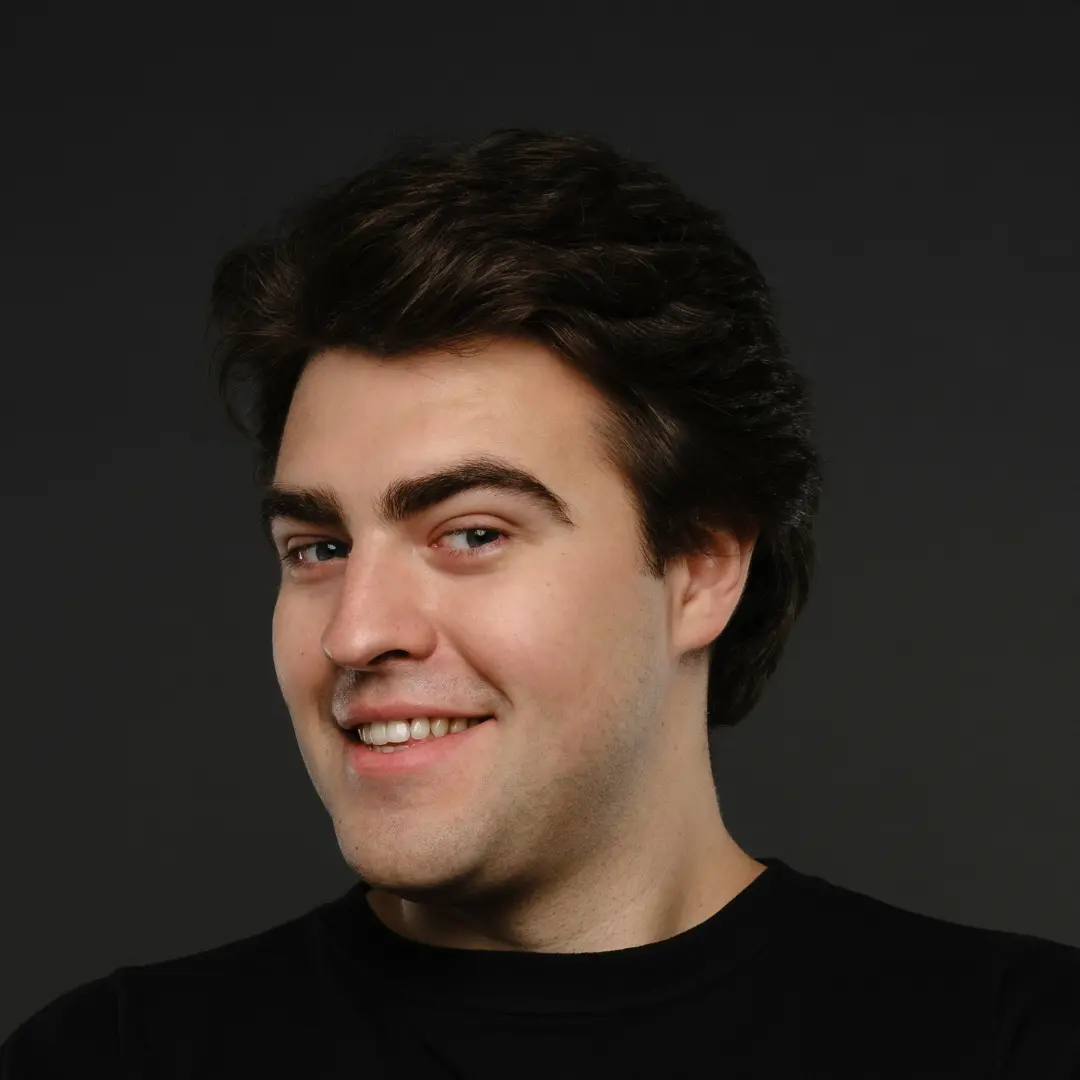
by Oleh Subotin
Full Stack Developer
Jul, 2024γ»5 min read
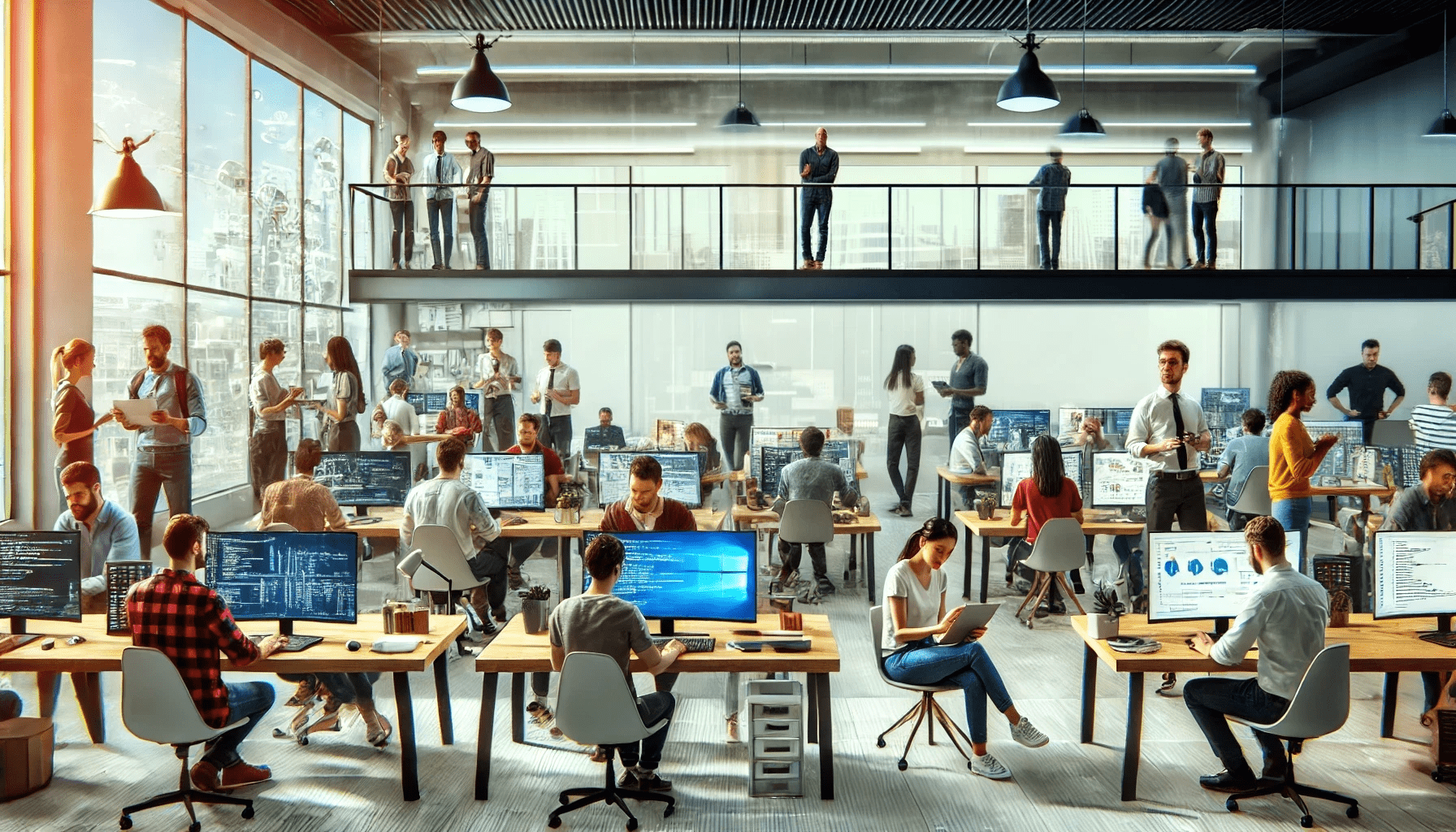
Is a College Degree Essential for a Career as a Developer
Evaluating the Role of Formal Education in Your Tech Career
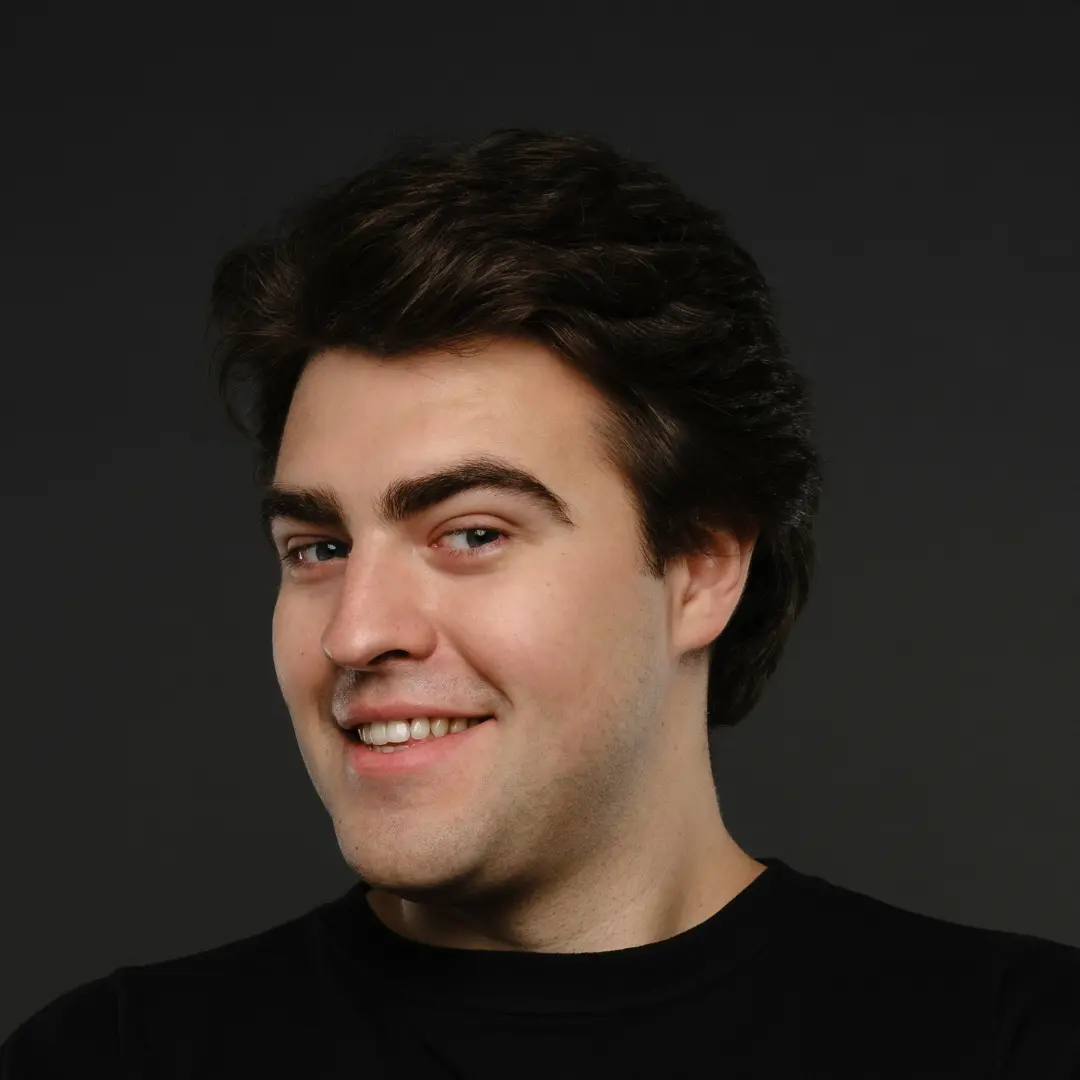
by Oleh Subotin
Full Stack Developer
Jul, 2024γ»6 min read
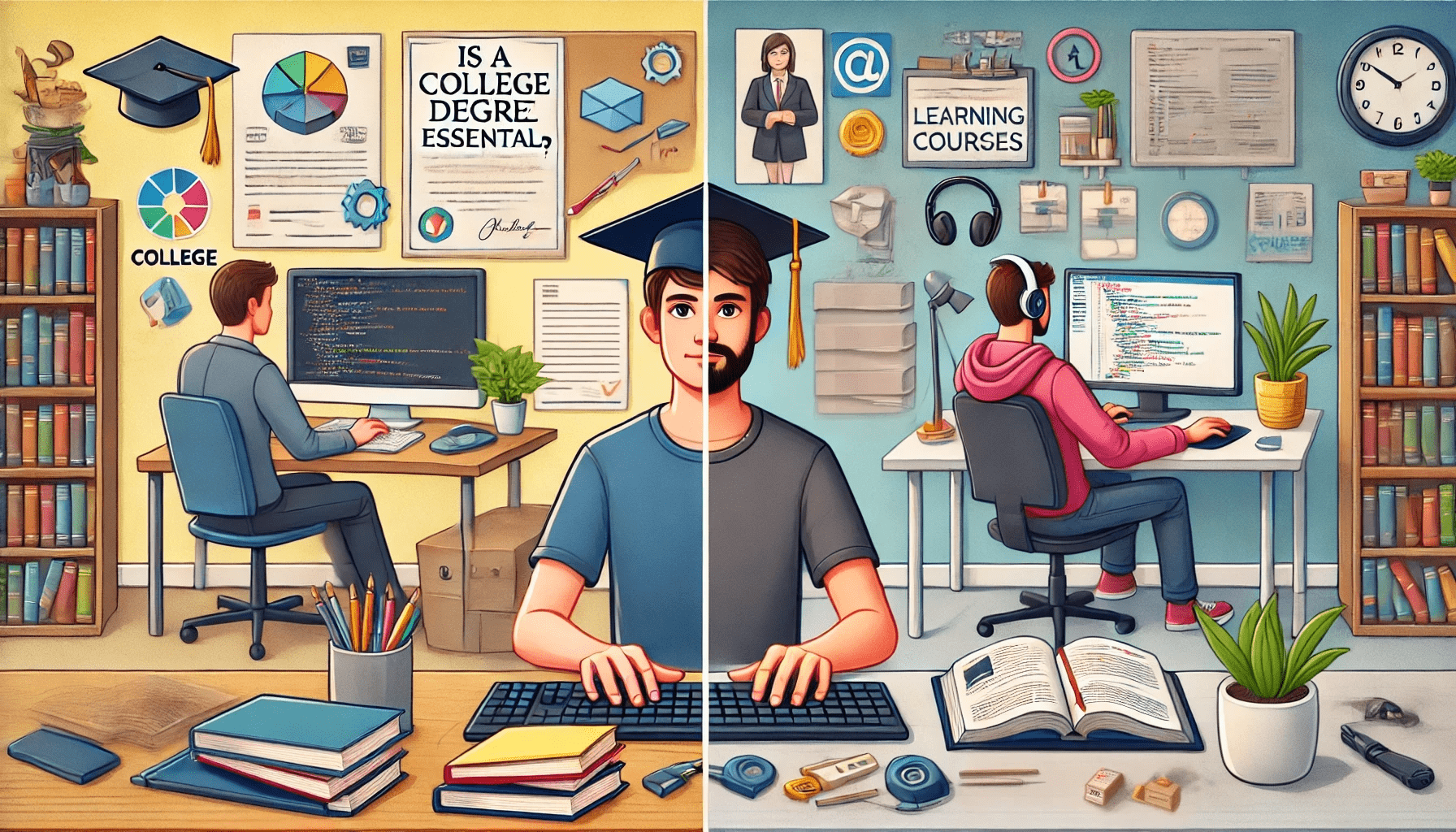
Content of this article