Related courses
See All CoursesIntermediate
Ultimate NumPy
Unlock the full potential of Python's most essential library for numerical computing, NumPy. This comprehensive course is designed to take you from a beginner's understanding to an advanced level of proficiency in NumPy. Whether you're a data scientist, engineer, researcher, or developer, mastering NumPy is essential for efficient data manipulation, scientific computing, and machine learning.
Intermediate
Pandas First Steps
Pandas is an extremely user-friendly library for data analysis. It's also designed to handle large datasets, using data structures like DataFrame and Series. This makes it an invaluable tool for Data Science. In this guide, you'll get acquainted with a range of statistical functions, including how to find correlations, modes, medians, and maximum and minimum values within a dataset. You'll also learn how to handle missing values and manipulate specific values, as well as how to remove them.
Intermediate
ML Introduction with scikit-learn
Machine Learning is now used everywhere. Want to learn it yourself? This course is an introduction to the world of Machine learning for you to learn basic concepts, work with Scikit-learn – the most popular library for ML and build your first Machine Learning project. This course is intended for students with a basic knowledge of Python, Pandas, and Numpy.
Top 50 Python Interview Questions for Data Analyst
Common Python questions for DA interview
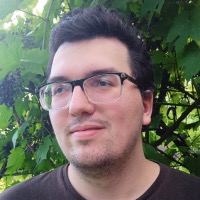
by Ruslan Shudra
Data Scientist
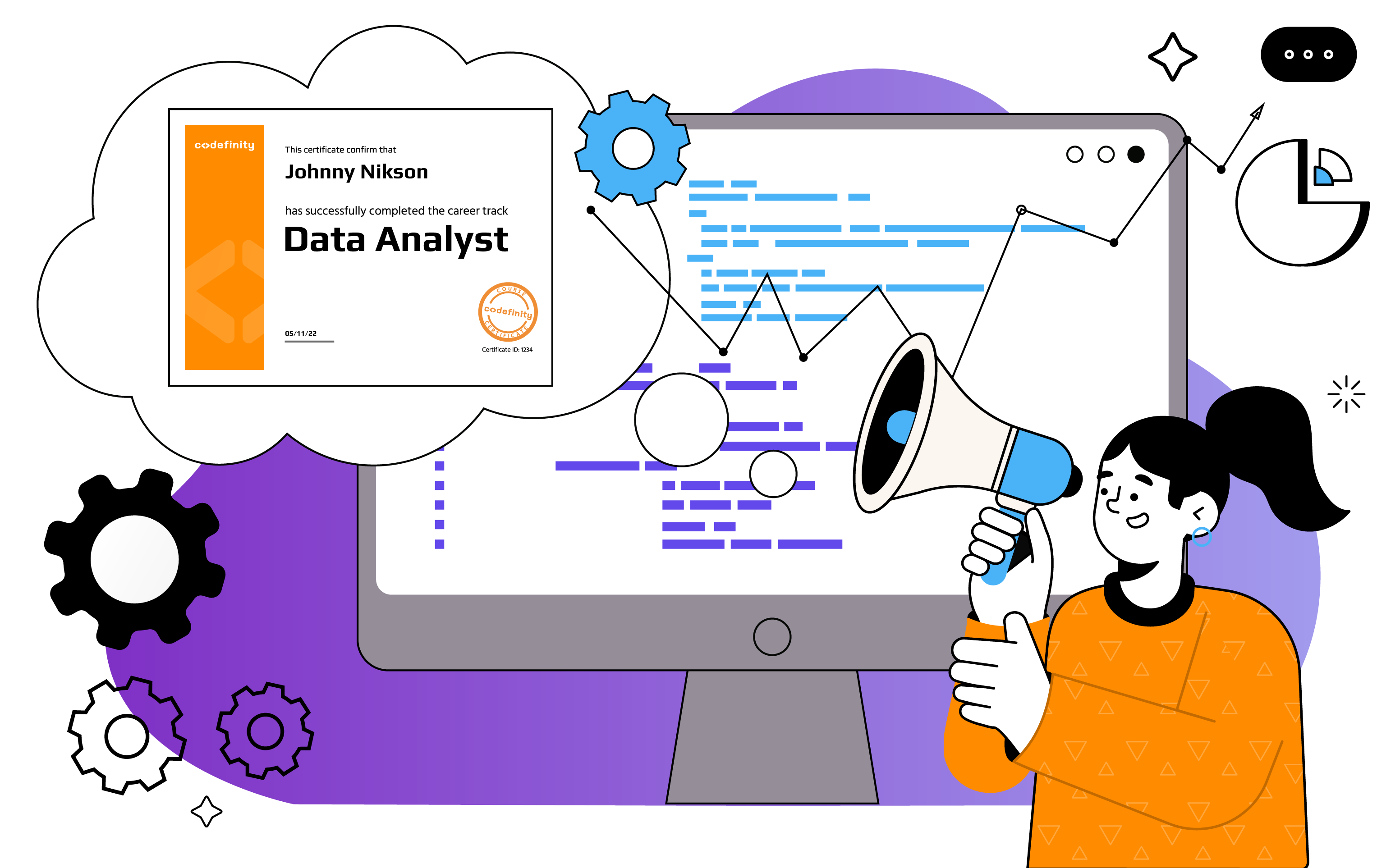
Introduction
In the rapidly evolving field of data analytics, proficiency in Python has become indispensable. Python's versatility and extensive ecosystem of libraries make it the go-to language for data manipulation, analysis, and visualization tasks. For aspiring data analysts looking to land their dream job, mastering Python is essential.
To help you prepare for your next data analyst interview, we've compiled a comprehensive list of the top 50 Python interview questions tailored specifically for data analysts. These questions are categorized into beginner, intermediate, and advanced levels, covering a wide range of topics essential for success in the field of data analytics.
Beginner Level Questions
Q1. What is Python, and why is it commonly used in data analytics?
A1. Python is a high-level programming language known for its simplicity and readability. It's widely used in data analytics due to its rich ecosystem of libraries such as Pandas, NumPy, and Matplotlib, which make data manipulation, analysis, and visualization more accessible.
Q2. How do you install external libraries in Python?
A2. External libraries in Python can be installed using package managers like pip. For example, to install the Pandas library, you can use the command pip install pandas
.
Q3. What is Pandas, and how is it used in data analysis?
A3. Pandas is a Python library used for data manipulation and analysis. It provides data structures like DataFrame and Series, which allow for easy handling and analysis of tabular data.
Q4. How do you read a CSV file into a DataFrame using Pandas?
A4. You can read a CSV file into a DataFrame using the pd.read_csv()
function in Pandas. For example:
Q5. What is NumPy, and why is it used in data analysis?
A5. NumPy is a Python library used for numerical computing. It provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays efficiently.
Q6. How do you create a NumPy array?
A6. You can create a NumPy array using the np.array()
function by passing a Python list as an argument. For example:
Q7. Explain the difference between a DataFrame and a Series in Pandas.
A7. A DataFrame is a 2-dimensional labeled data structure with columns of potentially different types. It can be thought of as a table with rows and columns. A Series, on the other hand, is a 1-dimensional labeled array capable of holding any data type.
Q8. How do you select specific rows and columns from a DataFrame in Pandas?
A8. You can use indexing and slicing to select specific rows and columns from a DataFrame in Pandas. For example:
Q9. What is Matplotlib, and how is it used in data analysis?
A9. Matplotlib is a Python library used for data visualization. It provides a wide variety of plots and charts to visualize data, including line plots, bar plots, histograms, and scatter plots.
Q10. How do you create a line plot using Matplotlib?
A10. You can create a line plot using the plt.plot()
function in Matplotlib. For example:
Q11. Explain the concept of data cleaning in data analysis.
A11. Data cleaning is the process of identifying and correcting errors, inconsistencies, and missing values in a dataset to improve its quality and reliability for analysis. It involves tasks such as removing duplicates, handling missing data, and correcting formatting issues.
Q12. How do you check for missing values in a DataFrame using Pandas?
A12. You can use the isnull()
method in Pandas to check for missing values in a DataFrame. For example:
Q13. What are some common methods for handling missing values in a DataFrame?
A13. Common methods for handling missing values include removing rows or columns containing missing values (dropna()
), filling missing values with a specified value (fillna()
), or interpolating missing values based on existing data (interpolate()
).
Q14. How do you calculate descriptive statistics for a DataFrame in Pandas?
A14. You can use the describe()
method in Pandas to calculate descriptive statistics for a DataFrame, including count, mean, standard deviation, minimum, maximum, and percentiles.
Q15. What is a histogram, and how is it used in data analysis?
A15. A histogram is a graphical representation of the distribution of numerical data. It consists of a series of bars, where each bar represents a range of values and the height of the bar represents the frequency of values within that range. Histograms are commonly used to visualize the frequency distribution of a dataset.
Q16. How do you create a histogram using Matplotlib?
A16. You can create a histogram using the plt.hist()
function in Matplotlib. For example:
Q17. What is the purpose of data visualization in data analysis?
A17. The purpose of data visualization is to communicate information and insights from data effectively through graphical representations. It allows analysts to explore patterns, trends, and relationships in the data, as well as to communicate findings to stakeholders in a clear and compelling manner.
Q18. How do you customize the appearance of a plot in Matplotlib?
A18. You can customize the appearance of a plot in Matplotlib by setting various attributes such as title, labels, colors, line styles, markers, and axis limits using corresponding functions like plt.title()
, plt.xlabel()
, plt.ylabel()
, plt.color()
, plt.linestyle()
, plt.marker()
, plt.xlim()
, and plt.ylim()
.
Q19. What is the purpose of data normalization in data analysis?
A19. The purpose of data normalization is to rescale the values of numerical features to a common scale without distorting differences in the ranges of values. It is particularly useful in machine learning algorithms that require input features to be on a similar scale to prevent certain features from dominating others.
Q20. What are some common methods for data normalization?
A20. Common methods for data normalization include min-max scaling, z-score normalization, and robust scaling. Min-max scaling scales the data to a fixed range (e.g., 0 to 1), z-score normalization scales the data to have a mean of 0 and a standard deviation of 1, and robust scaling scales the data based on percentiles to be robust to outliers.
Q21. How do you perform data normalization using scikit-learn?
A21. You can perform data normalization using the MinMaxScaler
, StandardScaler
, or RobustScaler
classes in scikit -learn. For example:
Q22. What is the purpose of data aggregation in data analysis?
A22. The purpose of data aggregation is to summarize and condense large datasets into more manageable and meaningful information by grouping data based on specified criteria and computing summary statistics for each group. It helps in gaining insights into the overall characteristics and patterns of the data.
Q23. How do you perform data aggregation using Pandas?
A23. You can perform data aggregation using the groupby()
method in Pandas to group data based on one or more columns and then apply an aggregation function to compute summary statistics for each group. For example:
Q24. What is the purpose of data filtering in data analysis?
A24. The purpose of data filtering is to extract subsets of data that meet specified criteria or conditions. It is used to focus on relevant portions of the data for further analysis or visualization.
Q25. How do you filter data in a DataFrame using Pandas?
A25. You can filter data in a DataFrame using boolean indexing in Pandas. For example, to filter rows where the 'Score' is greater than 90:
Run Code from Your Browser - No Installation Required
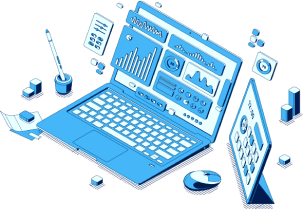
Intermediate Level Questions
Q1. What is the difference between loc and iloc in Pandas?
A1. loc
is used for label-based indexing, where you specify the row and column labels, while iloc
is used for integer-based indexing, where you specify the row and column indices.
Q2. How do you handle categorical data in Pandas?
A2. Categorical data in Pandas can be handled using the astype('category')
method to convert columns to categorical data type or by using the Categorical()
constructor. It helps in efficient memory usage and enables faster operations.
Q3. What is the purpose of the pd.concat()
function in Pandas?
A3. The pd.concat()
function in Pandas is used to concatenate (combine) two or more DataFrames along rows or columns. It allows you to stack DataFrames vertically or horizontally.
Q4. How do you handle datetime data in Pandas?
A4. Datetime data in Pandas can be handled using the to_datetime()
function to convert strings or integers to datetime objects, and the dt
accessor can be used to extract specific components like year, month, day, etc.
Q5. What is the purpose of the resample()
method in Pandas?
A5. The resample()
method in Pandas is used to change the frequency of time series data. It allows you to aggregate data over different time periods, such as converting daily data to monthly or yearly data.
Q6. How do you perform one-hot encoding in Pandas?
A6. One-hot encoding in Pandas can be performed using the get_dummies()
function, which converts categorical variables into dummy/indicator variables, where each category is represented as a binary feature.
Q7. What is the purpose of the map()
function in Python and its relevance in data analysis?
A7. The map()
function applies a given function to each item of an iterable and returns a list of the results. In data analysis, it's useful for applying functions element-wise to data structures like lists or Pandas Series.
Q8. How do you handle outliers in a DataFrame in Pandas?
A8. Outliers in a DataFrame can be handled by removing them using methods like z-score, interquartile range (IQR), or winsorization, or by transforming them using techniques like log transformation or trimming.
Q9. What is the purpose of the pd.melt()
function in Pandas?
A9. The pd.melt()
function in Pandas is used to reshape (unpivot) a DataFrame from wide format to long format, converting columns into rows. It is useful for data cleaning and analysis.
Q10. How do you perform group-wise operations in Pandas?
A10. Group-wise operations in Pandas can be performed using the groupby()
method followed by an aggregation function like sum()
, mean()
, count()
, etc., to compute summary statistics for each group.
Q11. What is the purpose of the merge()
and join()
functions in Pandas?
A11. Both merge()
and join()
functions in Pandas are used to combine DataFrames based on one or more keys (columns). merge()
is more flexible and supports different types of joins, while join()
is a convenience method for merging on indices.
Q12. How do you handle multi-level indexing (hierarchical indexing) in Pandas?
A12. Multi-level indexing in Pandas allows you to index data using multiple levels of row or column indices. It can be created using the set_index()
method or by specifying index_col
parameter while reading data from external sources.
Q13. What is the purpose of the shift()
method in Pandas?
A13. The shift()
method in Pandas is used to shift index by a specified number of periods (rows). It is commonly used to compute lag or lead values, and it can be applied to both Series and DataFrame objects.
Q14. How do you handle imbalanced datasets in Pandas?
A14. Imbalanced datasets in Pandas can be handled using techniques like resampling (oversampling minority class or undersampling majority class), using class weights in machine learning models, or using algorithms specifically designed for imbalanced datasets.
Q15. What is the purpose of the pipe()
method in Pandas?
A15. The pipe()
method in Pandas is used to apply a sequence of functions to a DataFrame or Series. It allows for method chaining and enables cleaner and more readable code by separating the data processing steps.
Advanced Level Questions
Q1. Explain the concept of method chaining in Pandas and provide an example.
A1. Method chaining involves applying multiple Pandas operations in a single line of code, often separated by dots. It improves code readability and conciseness. For example:
Q2. Describe how you would handle memory optimization for large datasets in Pandas.
A2. Memory optimization techniques include converting data types to more memory-efficient ones (e.g., using astype()
with category
dtype for categorical variables), using sparse matrices for sparse data, and processing data in chunks rather than loading it all into memory at once.
Q3. Explain the purpose of the crosstab()
function in Pandas and provide an example.
A3. The crosstab()
function computes a cross-tabulation table that shows the frequency distribution of variables. It's particularly useful for categorical data analysis. Example:
Q4. How would you efficiently handle and process large-scale time series data in Python?
A4. Efficient handling of large-scale time series data involves using specialized libraries like Dask
or Vaex
for out-of-core computation, optimizing data structures and algorithms, and leveraging parallel processing techniques.
Q5. How would you handle imbalanced datasets in a classification problem using Python?
A5. Techniques for handling imbalanced datasets include oversampling the minority class (e.g., using SMOTE), undersampling the majority class, using different evaluation metrics (e.g., F1-score, precision-recall curves), and using algorithms that are less sensitive to class imbalance (e.g., decision trees, random forests).
Q6. How would you perform feature scaling in Python, and why is it important in machine learning?
A6. Feature scaling is important for ensuring that features have the same scale, preventing some features from dominating others in algorithms like gradient descent. Common techniques include standardization (subtracting mean and dividing by standard deviation) and normalization (scaling to a range).
Q7. Explain the purpose of the rolling()
function in Pandas for time series analysis and provide an example.
A7. rolling()
is used to compute rolling statistics (e.g., rolling mean, rolling sum) over a specified window of time. Example:
Q8. Explain the purpose of the stack()
and unstack()
functions in Pandas with examples.
A8. stack()
is used to pivot the columns of a DataFrame to rows, while unstack()
pivots the rows back to columns. Example:
Q9. How would you handle multicollinearity in a regression analysis using Python?
A9. Techniques for handling multicollinearity include removing one of the correlated variables, using dimensionality reduction techniques like PCA, or using regularization methods like Ridge or Lasso regression.
Q10. Explain the purpose of the PCA
class in scikit-learn and how it can be used for dimensionality reduction.
A10. The PCA
(Principal Component Analysis) class in scikit-learn is used for linear dimensionality reduction by projecting data onto a lower-dimensional subspace. It identifies the directions (principal components) that maximize the variance of the data and reduces the dimensionality while preserving most of the variability.
Conclusion
In conclusion, this article provides a comprehensive overview of advanced topics in Python for data analysts. It covers a wide range of techniques and methodologies essential for tackling complex data analysis tasks, including ensemble learning, dimensionality reduction, anomaly detection, time series forecasting, natural language processing, feature selection, model interpretability, transfer learning, and recommender systems.
By delving into these advanced concepts and providing practical examples of their implementation using popular Python libraries and frameworks such as scikit-learn, statsmodels, Prophet, NLTK, spaCy, TensorFlow, and Keras, this article equips data analysts with the knowledge and tools necessary to extract valuable insights from diverse datasets and make informed decisions.
Start Learning Coding today and boost your Career Potential
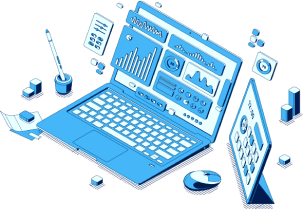
FAQs
Q: Should I learn everything covered in these Top 50 Python Interview Questions for Data Analyst?
A: While mastering every question can certainly boost your confidence and performance in Python interviews, focus on understanding the concepts thoroughly rather than memorizing answers verbatim. Prioritize topics based on your strengths and the requirements of the data analyst roles you're targeting.
Q: How can I effectively prepare for Python interviews using this article?
A: Start by going through the questions and understanding the underlying concepts. Then, practice solving related problems and reinforce your understanding by writing code. Additionally, consider discussing these topics with peers or mentors to gain different perspectives and insights.
Q: Are these questions suitable for both job seekers and hiring managers?
A: Absolutely! Job seekers can use these questions to prepare for technical interviews and showcase their Python skills. On the other hand, hiring managers can utilize this resource to assess candidates' knowledge and competence during the interview process.
Q: What if I'm new to Python? Can I still benefit from this article?
A: Definitely! This article covers questions ranging from basic to advanced levels, making it suitable for individuals at different proficiency levels. Start with the basics and gradually progress to more advanced topics as you build your understanding and expertise in Python.
Q: How can I use this article to improve my problem-solving skills?
A: Beyond memorizing answers, focus on understanding the logic behind each solution. Practice solving similar problems on your own, experiment with different approaches, and strive to write clean and efficient code. This iterative process will enhance your problem-solving abilities over time.
Q: What if I encounter a question I'm unfamiliar with during an interview?
A: Don't panic! Use the opportunity to demonstrate your problem-solving skills and willingness to learn. Analyze the question, break it down into smaller parts, and communicate your thought process with the interviewer. Employers often value candidates who can approach challenges methodically and adapt on the fly.
Q: Are there any additional resources I can explore to supplement my preparation?
A: Absolutely! Consider exploring online tutorials, documentation, coding challenges, and community forums to deepen your understanding of Python. Engaging in hands-on projects and contributing to open-source projects can also provide valuable real-world experience.
Q: How can I stay updated with the latest developments in Python?
A: Stay connected with the Python community by following influential developers on social media platforms, attending conferences, participating in webinars, and subscribing to newsletters and blogs. Additionally, regularly check updates to the Python language and popular libraries/frameworks.
Q: What if I don't perform well in my Python interview despite preparation?
A: Remember that interviews are learning experiences, and setbacks are opportunities for growth. Reflect on areas where you can improve, seek feedback from interviewers if possible, and continue refining your skills. Each interview, regardless of the outcome, contributes to your development as a Python data analyst.
Q: Any final tips for Python interview success?
A: Stay confident, stay curious, and stay humble. Approach each interview as a chance to showcase your abilities and learn from the experience. Remember that interviewers are not just evaluating your technical skills but also your attitude, communication, and problem-solving approach. Keep practicing, stay positive, and believe in your capabilities!
Related courses
See All CoursesIntermediate
Ultimate NumPy
Unlock the full potential of Python's most essential library for numerical computing, NumPy. This comprehensive course is designed to take you from a beginner's understanding to an advanced level of proficiency in NumPy. Whether you're a data scientist, engineer, researcher, or developer, mastering NumPy is essential for efficient data manipulation, scientific computing, and machine learning.
Intermediate
Pandas First Steps
Pandas is an extremely user-friendly library for data analysis. It's also designed to handle large datasets, using data structures like DataFrame and Series. This makes it an invaluable tool for Data Science. In this guide, you'll get acquainted with a range of statistical functions, including how to find correlations, modes, medians, and maximum and minimum values within a dataset. You'll also learn how to handle missing values and manipulate specific values, as well as how to remove them.
Intermediate
ML Introduction with scikit-learn
Machine Learning is now used everywhere. Want to learn it yourself? This course is an introduction to the world of Machine learning for you to learn basic concepts, work with Scikit-learn – the most popular library for ML and build your first Machine Learning project. This course is intended for students with a basic knowledge of Python, Pandas, and Numpy.
30 Python Project Ideas for Beginners
Python Project Ideas
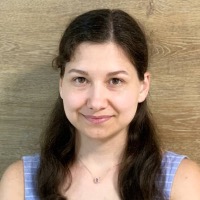
by Anastasiia Tsurkan
Backend Developer

Data Analyst vs Data Engineer vs Data Scientist
Unraveling the Roles and Responsibilities in Data-Driven Careers
by Kyryl Sidak
Data Scientist, ML Engineer

Web Scraping and Parsing in Python
Unveiling the Digital Tapestry
by Oleh Lohvyn
Backend Developer
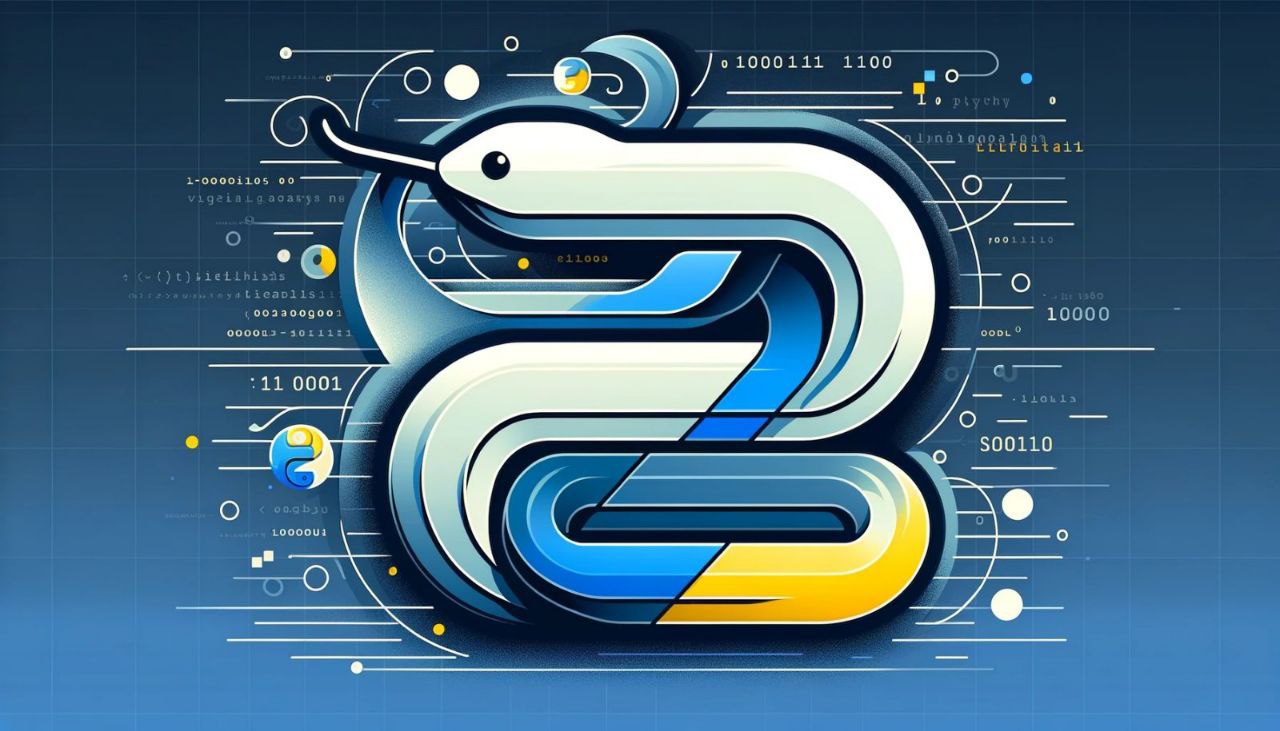
Content of this article