All About Exceptions in Python and How to Catch Them
Python Exceptions
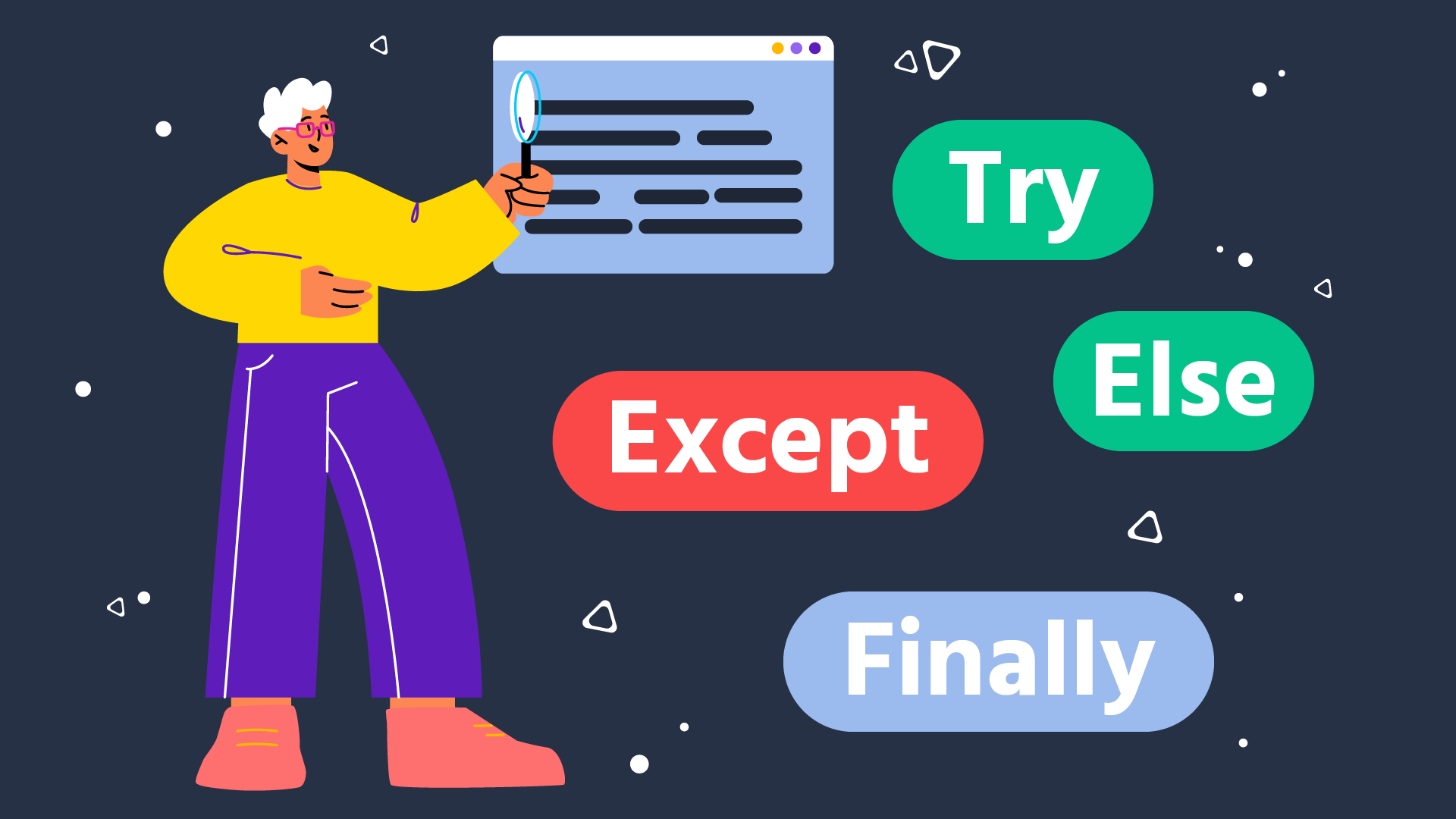
Python, a high-level programming language, is known for its clear syntax and readability, making it an excellent choice for beginners and experienced programmers alike. One of the core concepts in Python programming is the handling of exceptions. Exceptions are errors that occur during the execution of a program, and if not properly handled, can cause the program to terminate unexpectedly. This article will explore what exceptions are, the different types of exceptions in Python, and how to effectively handle them to build robust and efficient applications.
Understanding Exceptions in Python
Exceptions are events that disrupt the normal flow of a program's instructions. In Python, when an error occurs within a program, an exception is raised, which propagates backward through the stack until it is handled. If not handled, the program will terminate with an error message.
Common Types of Python Exceptions
Python comes with numerous built-in exceptions, and here are some of the most common ones:
- SyntaxError: Occurs when the parser encounters a syntax error.
- IndexError: Happens when an index of a sequence is out of range.
- KeyError: Raised when a dictionary key is not found.
- FileNotFoundError: Thrown when a file or directory is requested but doesn't exist.
- ValueError: Occurs when a function receives an argument of the correct type but an inappropriate value.
- TypeError: Raised when an operation or function is applied to an object of inappropriate type.
Understanding these exceptions is crucial for debugging and error handling in Python programs.
Run Code from Your Browser - No Installation Required
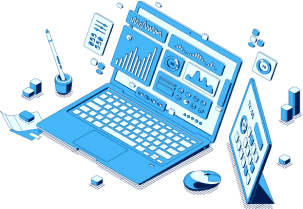
Exception Handling: Try, Except, Else, Finally
Python provides several keywords to handle exceptions gracefully: try, except, else, and finally.
-
The 'try' Block You wrap the code that might cause an exception in a try block. Python executes this block, and if an exception occurs, it immediately stops the current process and jumps to the except block.
-
The 'except' Block The except block catches and handles the exception. You can specify which exception you want to catch after the except keyword. If you don't specify any exception, it will catch all exceptions, which is not considered good practice because it can hide errors.
python
-
The 'else' Block The else block is optional and, if present, must follow all except blocks. It's used for code that should run if the try block doesn't raise an exception.
-
The 'finally' Block The finally block is optional as well. It is intended for cleanup actions that must be executed under all circumstances, such as closing a file or releasing a resource.
Raising Exceptions
In Python, you can also manually raise an exception using the raise keyword. This is useful when you want to enforce certain conditions within your code and provide more informative error messages.
python
Custom Exceptions
To create your own custom exceptions, you can define a class that derives from Python's built-in Exception class.
python
Start Learning Coding today and boost your Career Potential
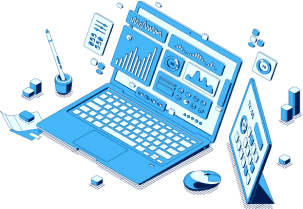
Best Practices for Exception Handling
- Catch Specific Exceptions: Always catch specific exceptions instead of a general 'except:' clause to avoid unintentionally handling unexpected errors.
- Use the else Clause: Utilize the 'else' clause to separate code that should run if no exceptions are raised from the code in the try block.
- Cleanup with finally: Use the 'finally' block to ensure that resources are released even if an error occurs.
- Keep Exception Handlers Minimal: Keep the code inside your exception handlers to a minimum, focusing only on the relevant error-handling logic.
- Use Logging: Instead of using 'print()' statements, consider logging exceptions, which offer more flexibility and can be easily configured for different environments.
Conclusion
In conclusion, understanding and properly handling exceptions in Python is crucial for building robust and reliable applications. By following best practices and utilizing Python's exception-handling constructs, you can control the flow of your program and deal with unexpected situations gracefully.
The SOLID Principles in Software Development
The SOLID Principles Overview
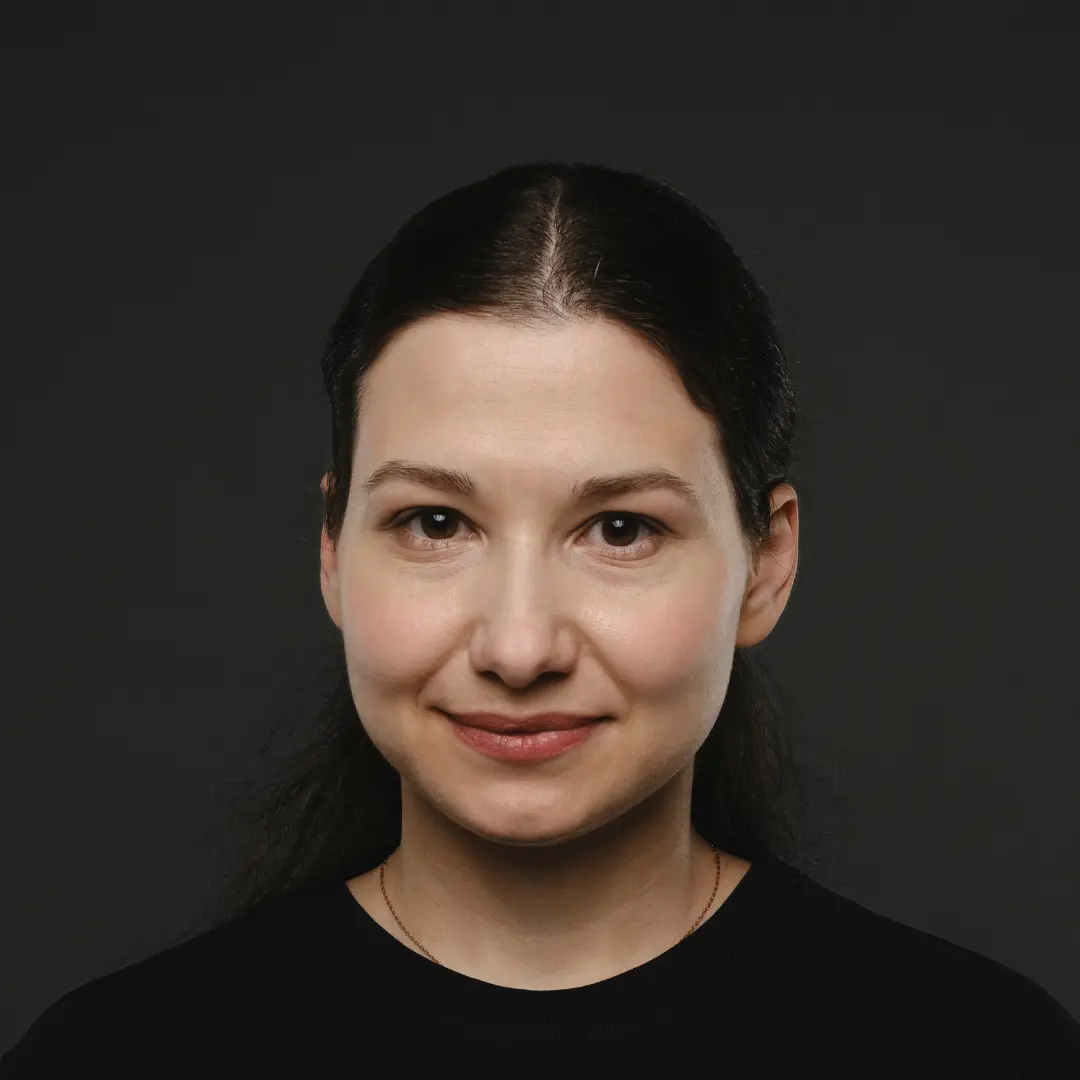
by Anastasiia Tsurkan
Backend Developer
Nov, 2023・8 min read
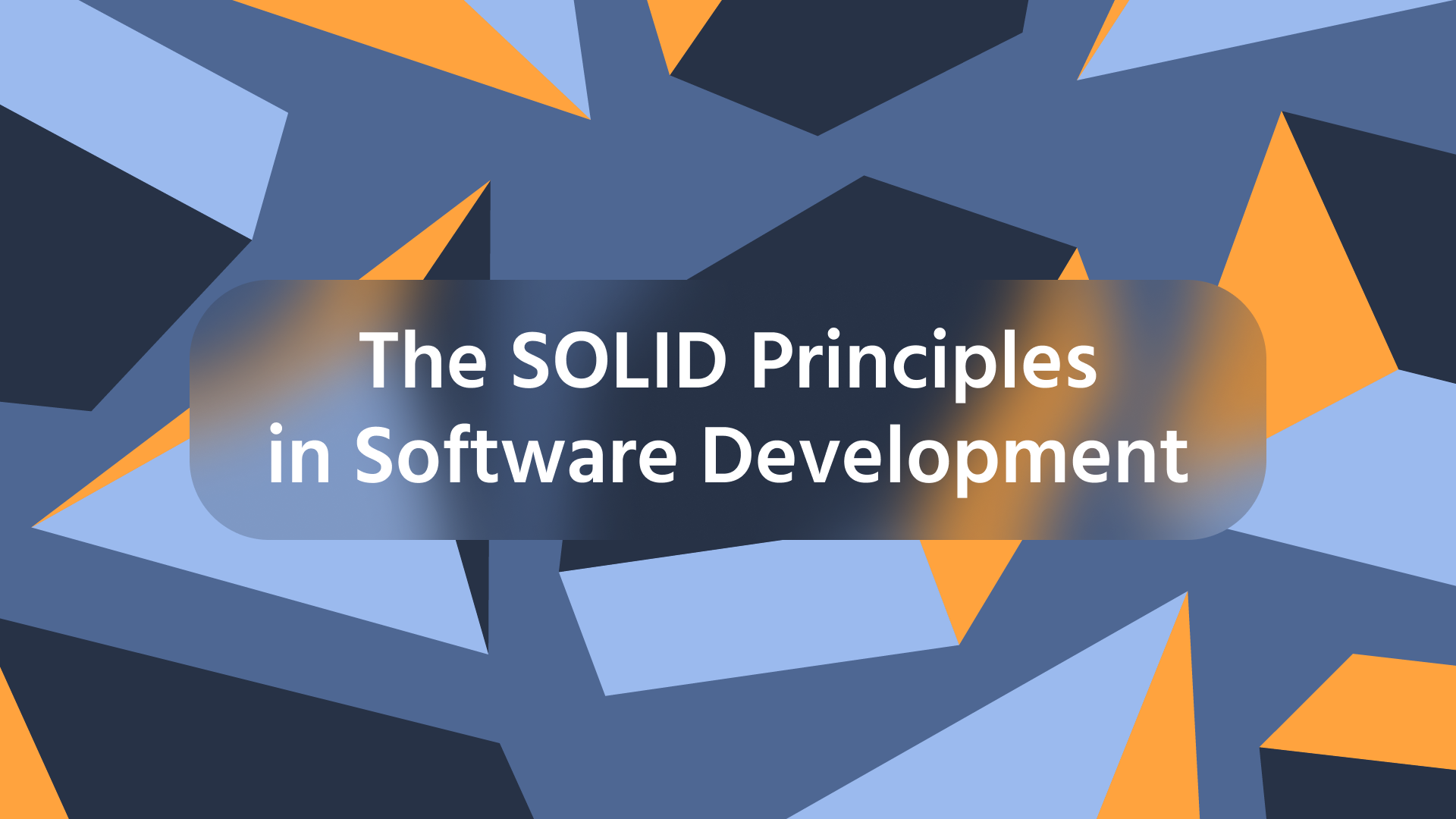
30 Python Project Ideas for Beginners
Python Project Ideas
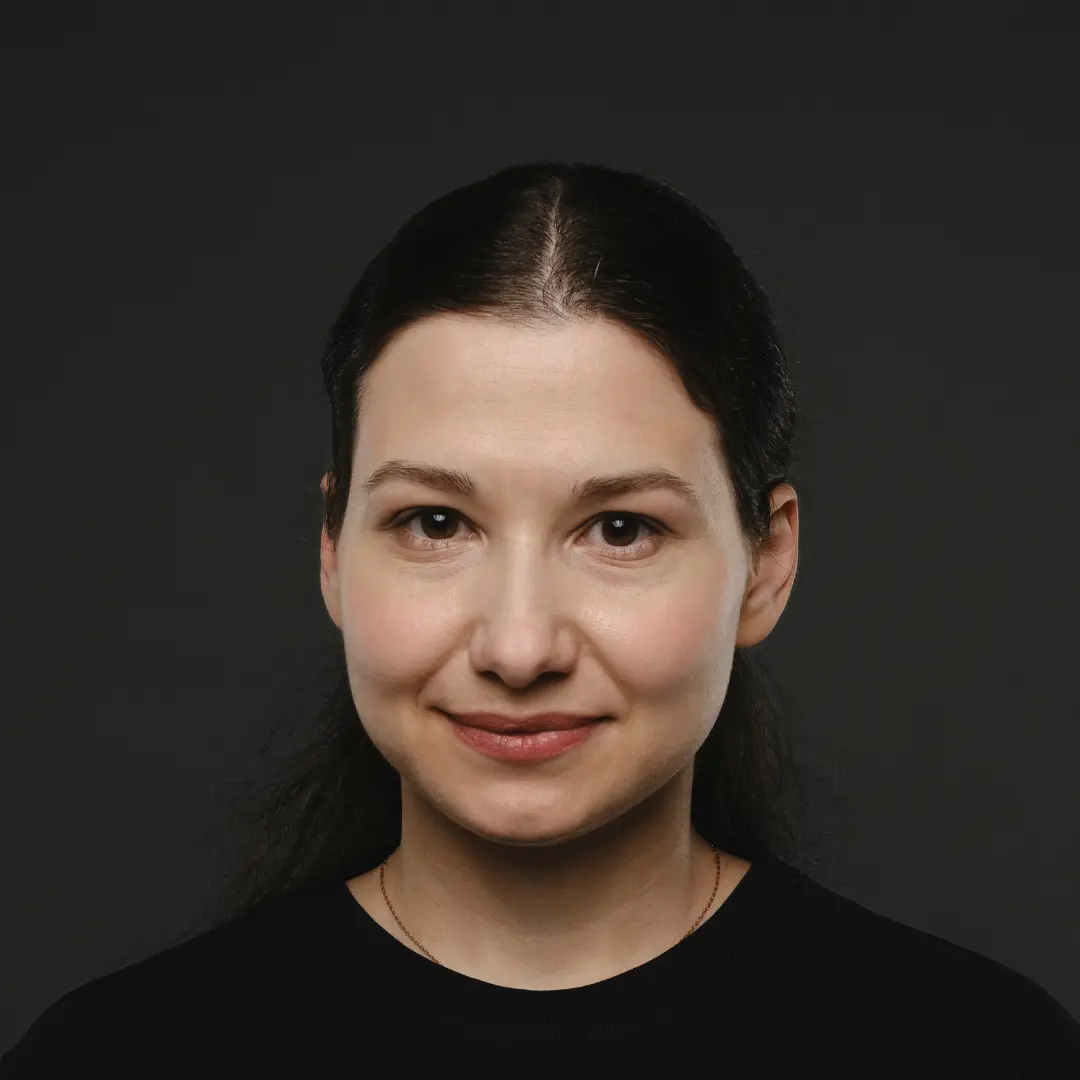
by Anastasiia Tsurkan
Backend Developer
Sep, 2024・14 min read
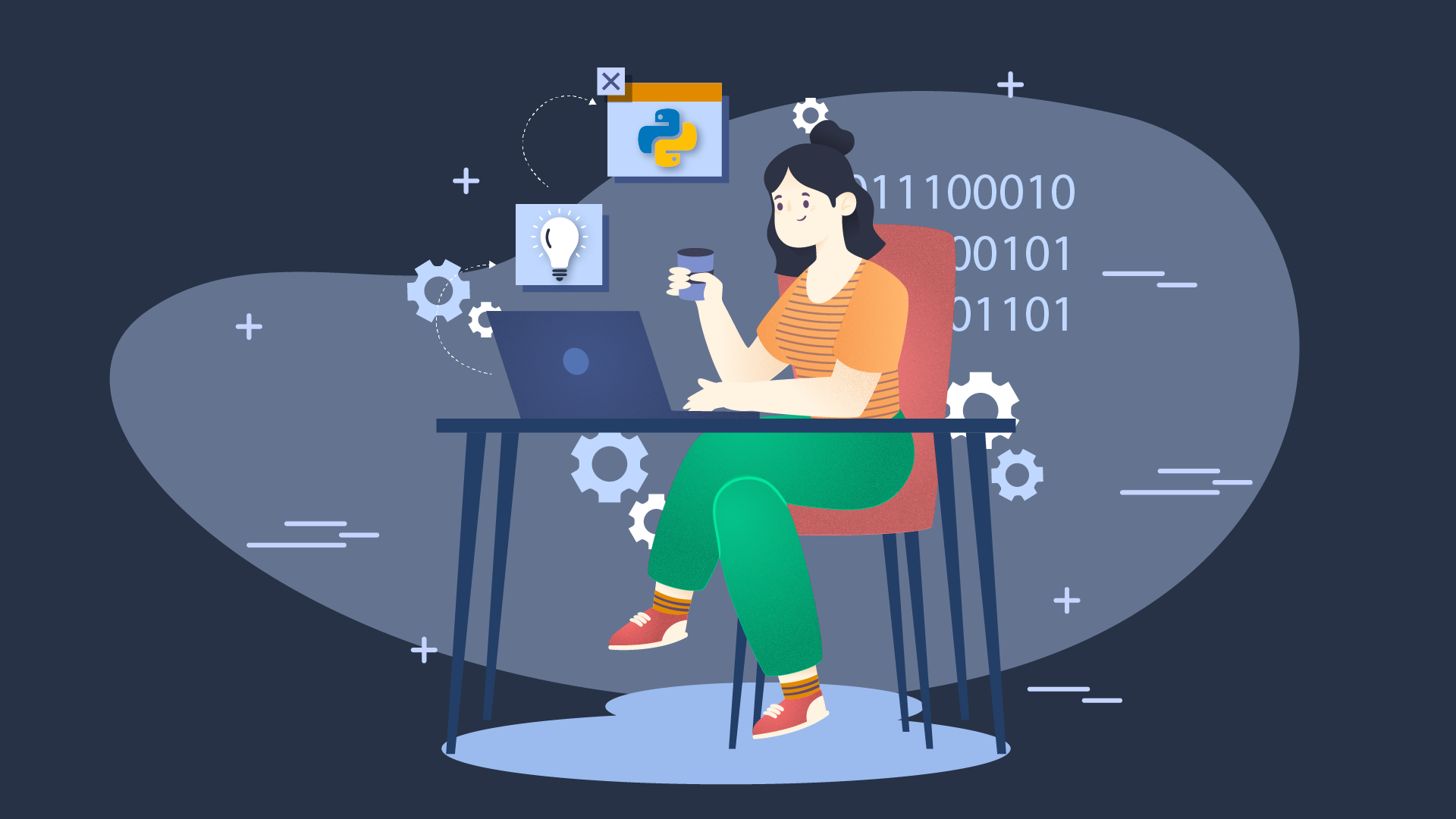
Python For Loops Beyond the Basics
For Loops Additional Functionality
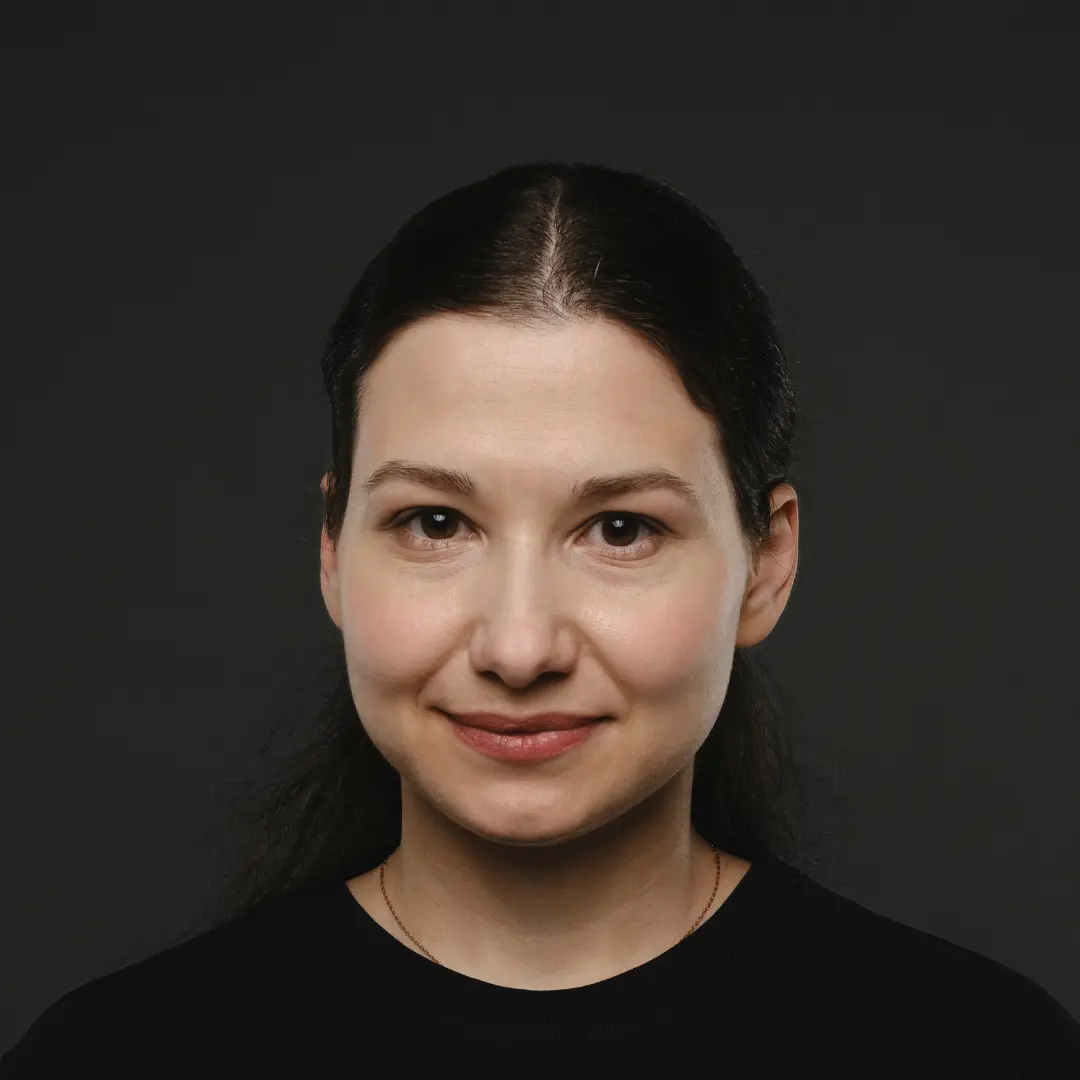
by Anastasiia Tsurkan
Backend Developer
Dec, 2023・3 min read
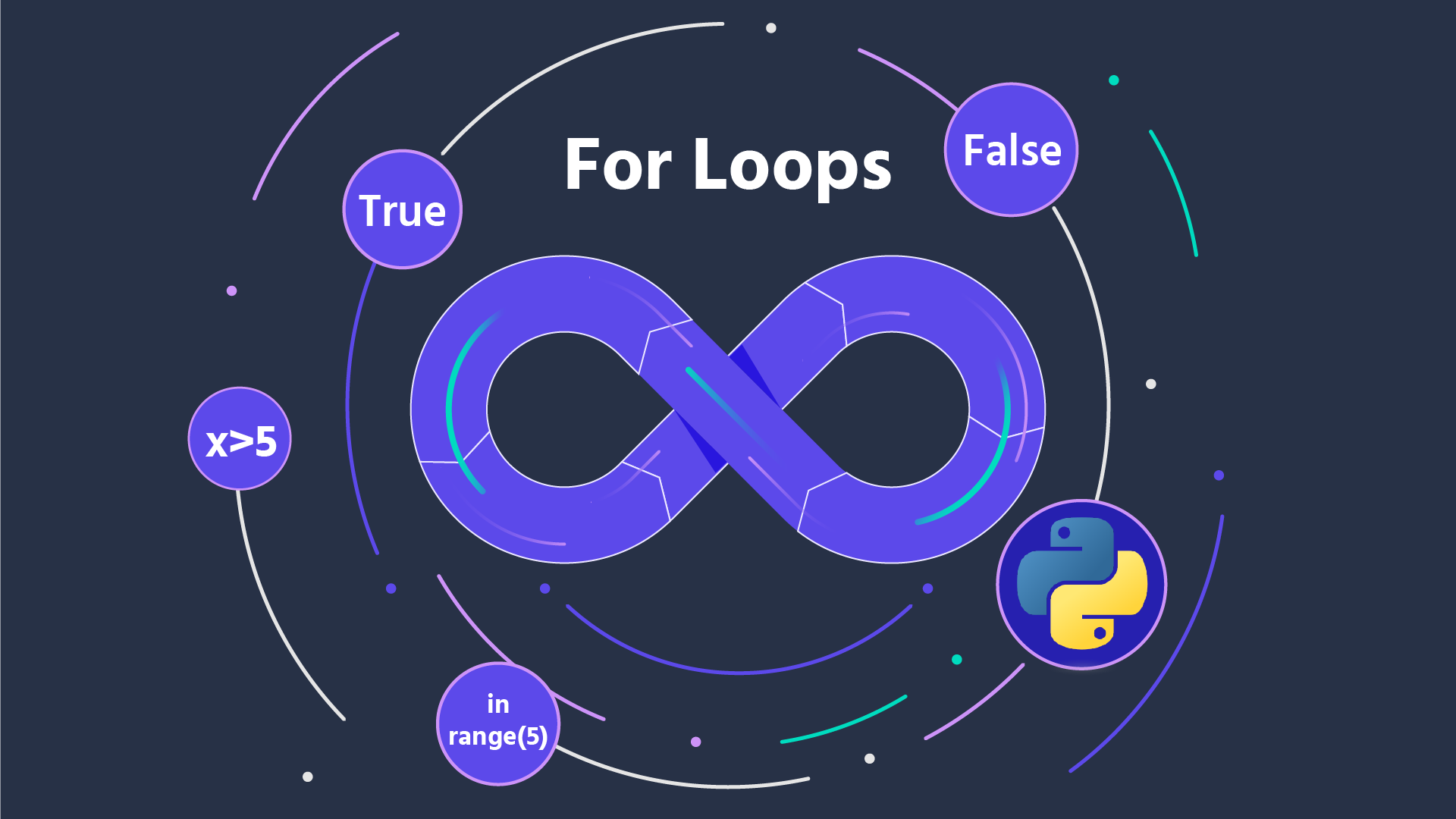
Indhold for denne artikel