Курси по темі
Всі курсиБазовий
Introduction to Python
Python is an interpreted high-level general-purpose programming language. Unlike HTML, CSS, and JavaScript, which are primarily used for web development, Python is versatile and can be used in various fields, including software development, data science, and back-end development. In this course, you'll explore the core aspects of Python, and by the end, you'll be crafting your own functions!
Середній
Intermediate Python Techniques
In this course, you will learn advanced topics such as function arguments, iterators, generators, closures, and decorators. Dozens of practice exercises will help you grasp these topics.
Asynchronous Programming in Python
Brief Intro to Asynchronous Programming
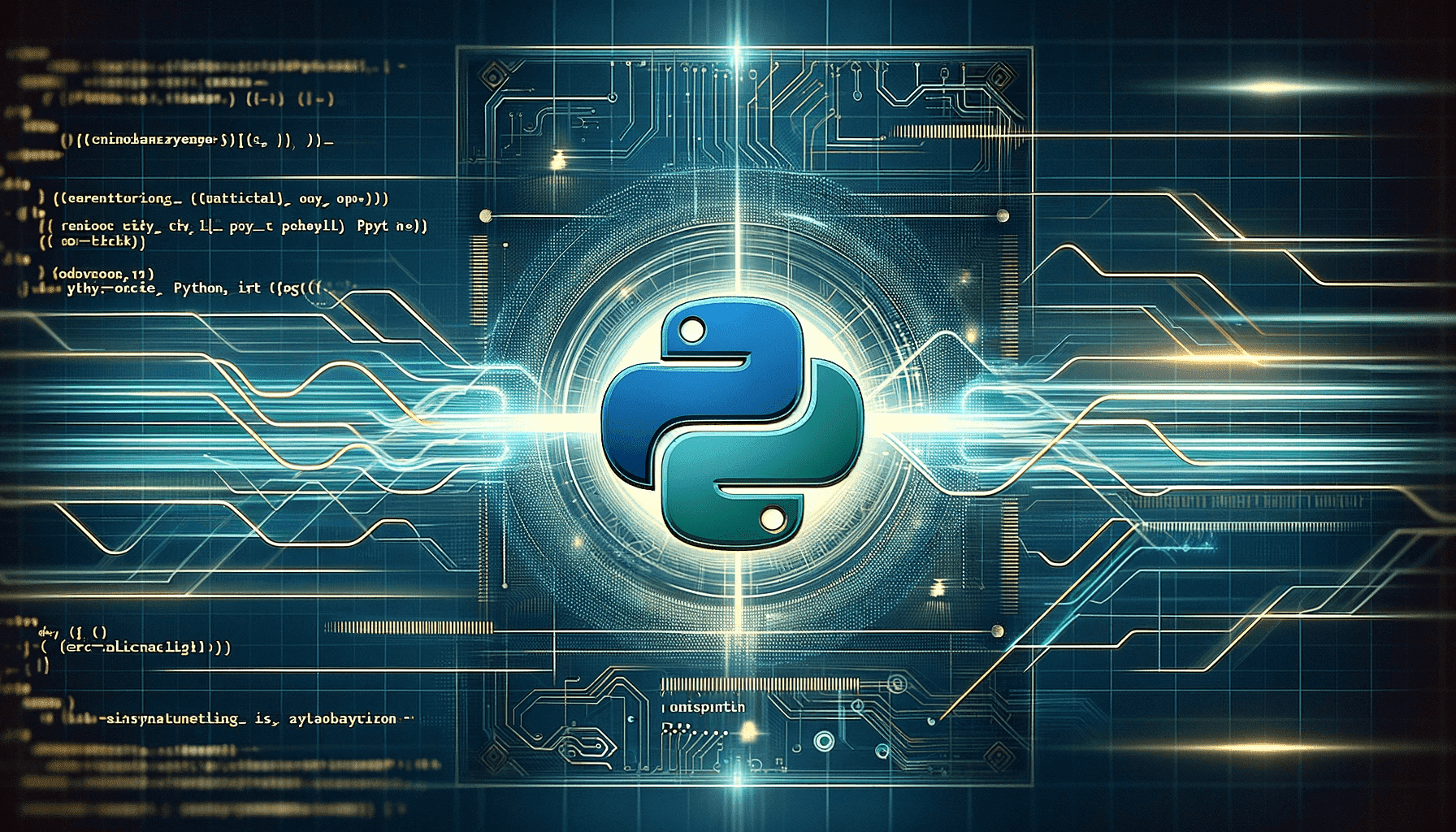
Introduction
Asynchronous programming is a method of concurrency that allows a program to execute multiple tasks simultaneously. In Python, this is particularly important for improving the performance of I/O-bound and high-latency applications. This article will guide you through the fundamentals of asynchronous programming in Python, exploring its benefits, key concepts, and how it differs from traditional synchronous programming.
Understanding Asynchronous vs. Synchronous Programming
- Synchronous Programming
- Linear execution
- Each task must complete before the next starts
- Simple but can be inefficient
- Asynchronous Programming
- Tasks run independently
- Allows for simultaneous execution of tasks
- Better resource utilization
Code Example: Synchronous vs. Asynchronous
Run Code from Your Browser - No Installation Required
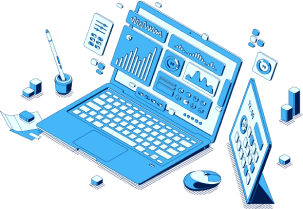
The Role of the asyncio Library
Python's asyncio
library is a cornerstone of asynchronous programming. It provides tools to run asynchronous tasks and handle concurrency.
Key Components of asyncio
- Event Loop: Orchestrates the execution of asynchronous tasks.
- Coroutines: Functions that can pause and resume their execution.
- Futures and Tasks: Represent the eventual result of an asynchronous operation.
Writing Asynchronous Code in Python
To utilize asynchronous programming in Python, you must understand how to write and manage coroutines.
- Creating Coroutines
- Use
async def
to define a coroutine. await
is used to call other asynchronous functions.
- Use
- Managing Coroutines
- Coroutines are executed in an event loop.
- Use
asyncio.run()
to run the main coroutine.
Code Example: Basic Asynchronous Program
Handling I/O-bound and High-latency Operations
Asynchronous programming excels in I/O-bound and high-latency scenarios.
Advantages for I/O-bound Processes
- Non-blocking I/O calls
- Improved application responsiveness
- Handling Network Requests Asynchronously
- Use
aiohttp
for asynchronous HTTP requests.
- Use
Code Example: Asynchronous HTTP Request
Start Learning Coding today and boost your Career Potential
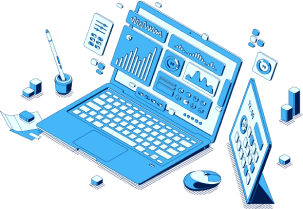
Advanced Asynchronous Programming Techniques
- Error Handling in Asynchronous Code
- Use
try-except
blocks within coroutines.
- Use
- Concurrent Execution of Coroutines
- Utilize
asyncio.gather()
to run multiple coroutines concurrently.
- Utilize
Code Example: Concurrent Execution
FAQs
Q: What is asynchronous programming in Python?
A: Asynchronous programming is a programming paradigm that allows the execution of non-blocking code, enabling tasks to run concurrently. In Python, it is commonly implemented using the asyncio
module.
Q: How does asynchronous programming differ from synchronous programming?
A: In synchronous programming, tasks are executed sequentially, one after the other. In asynchronous programming, tasks can be executed concurrently, allowing the program to continue executing other tasks while waiting for certain operations to complete.
Q: What is the role of the async
and await
keywords in Python asynchronous programming?
A: The async
keyword is used to define asynchronous functions, and the await
keyword is used to call asynchronous functions, indicating that the program should wait for the result without blocking other tasks.
Q: Can you give an example of an asynchronous function in Python?
A: Certainly! Here's a simple example:
Курси по темі
Всі курсиБазовий
Introduction to Python
Python is an interpreted high-level general-purpose programming language. Unlike HTML, CSS, and JavaScript, which are primarily used for web development, Python is versatile and can be used in various fields, including software development, data science, and back-end development. In this course, you'll explore the core aspects of Python, and by the end, you'll be crafting your own functions!
Середній
Intermediate Python Techniques
In this course, you will learn advanced topics such as function arguments, iterators, generators, closures, and decorators. Dozens of practice exercises will help you grasp these topics.
The SOLID Principles in Software Development
The SOLID Principles Overview
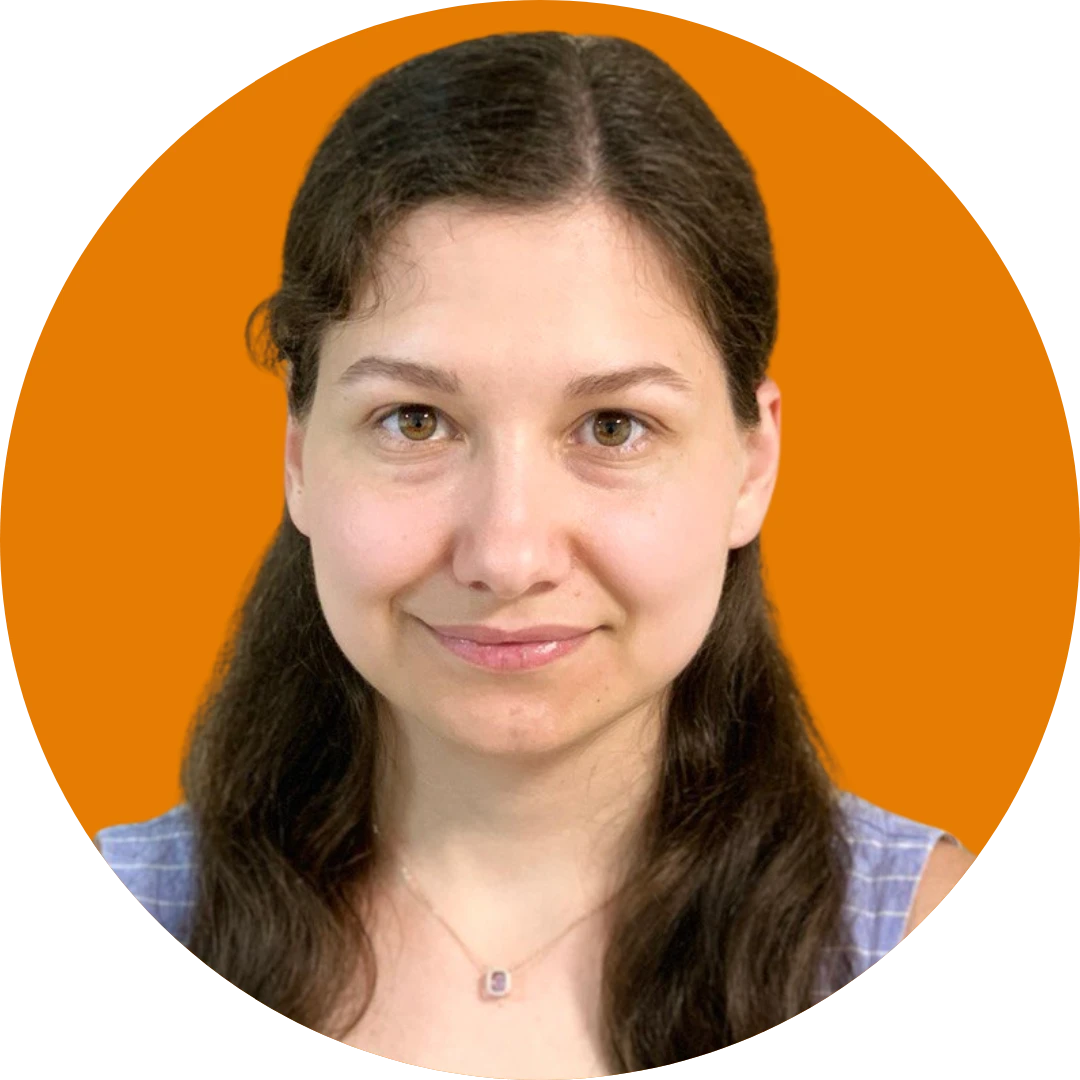
by Anastasiia Tsurkan
Backend Developer
Nov, 2023・8 min read
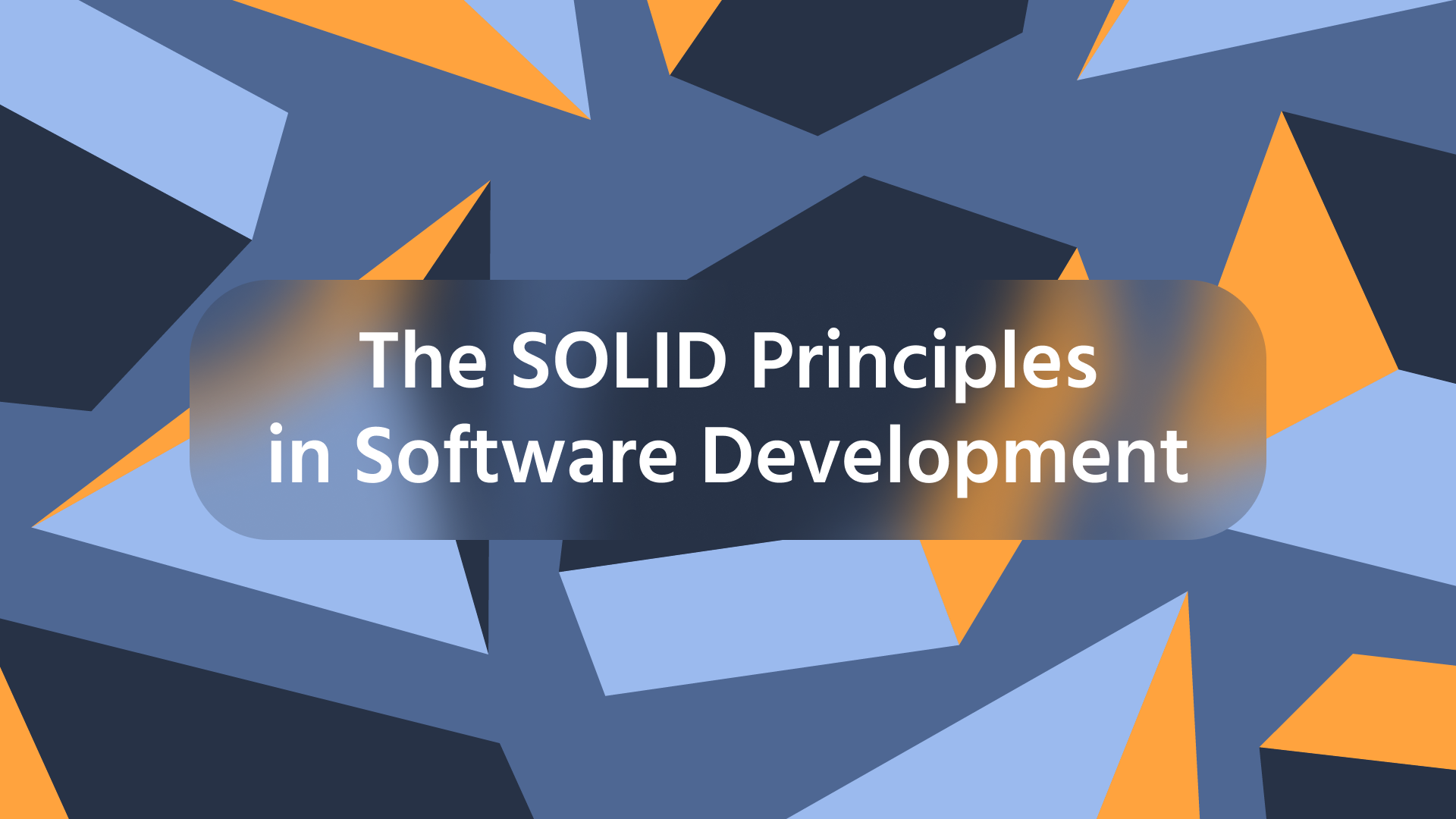
30 Python Project Ideas for Beginners
Python Project Ideas
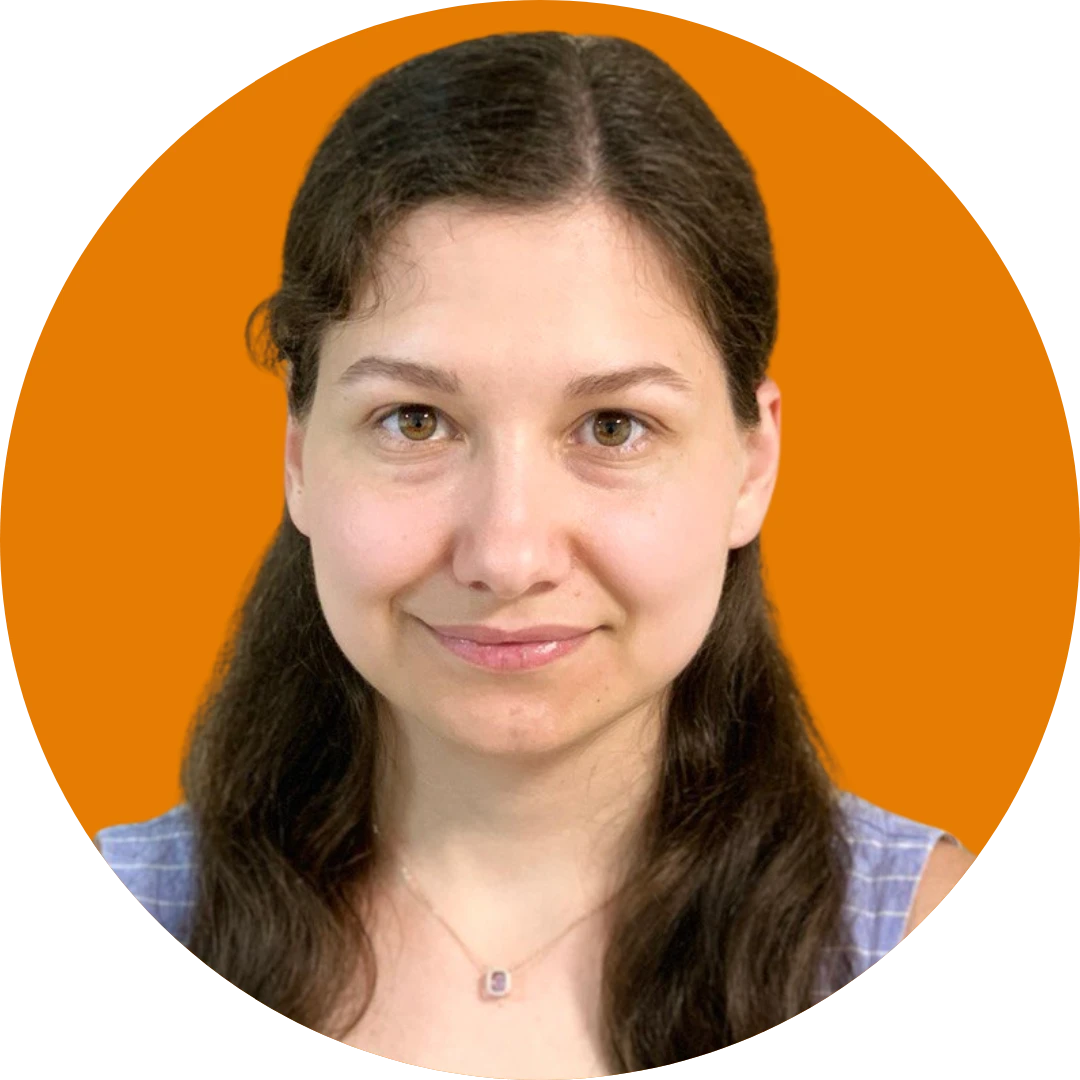
by Anastasiia Tsurkan
Backend Developer
Sep, 2024・14 min read
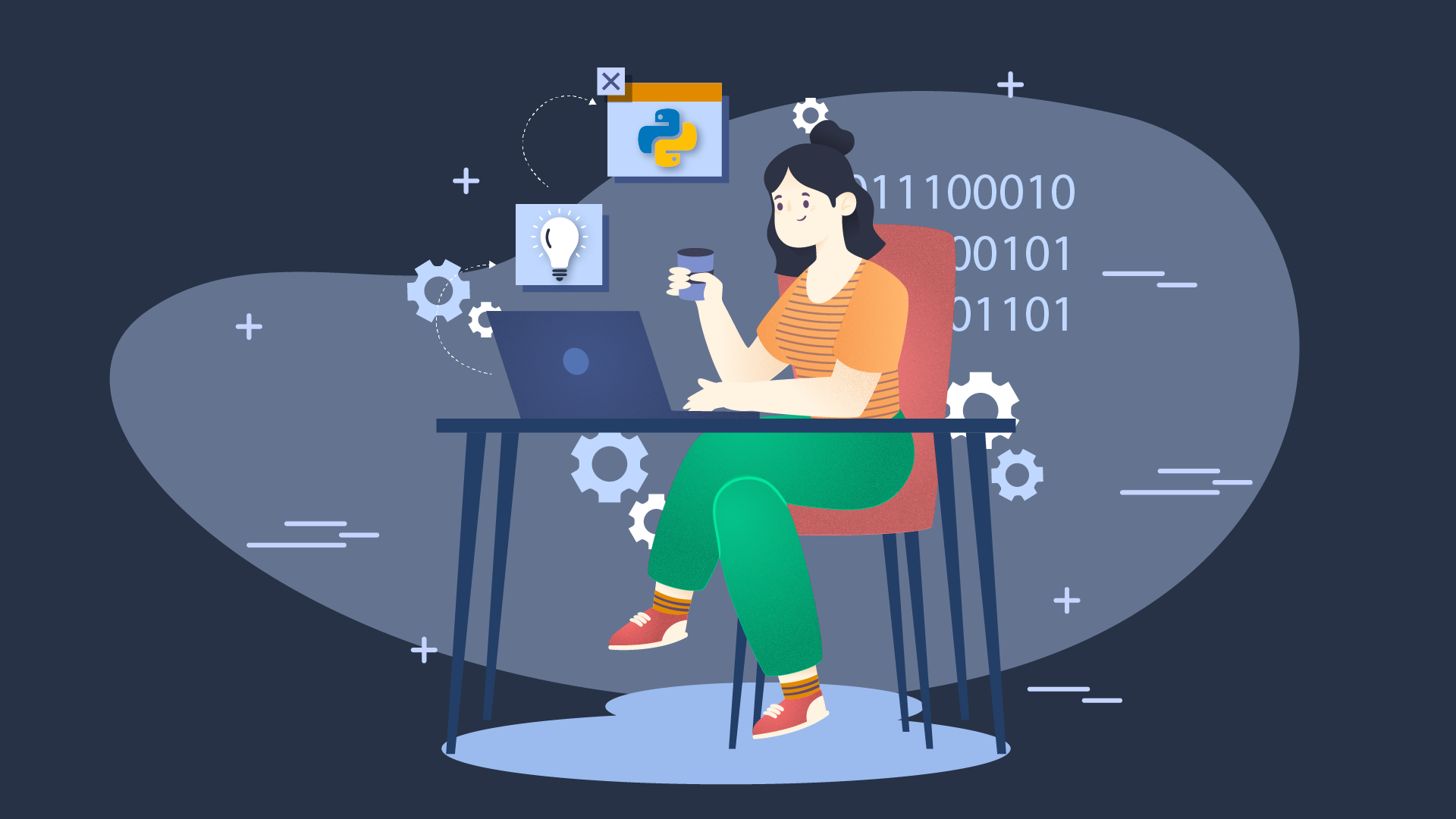
The Standardization Process of C++
Navigating Through the Evolution of a Core Programming Language
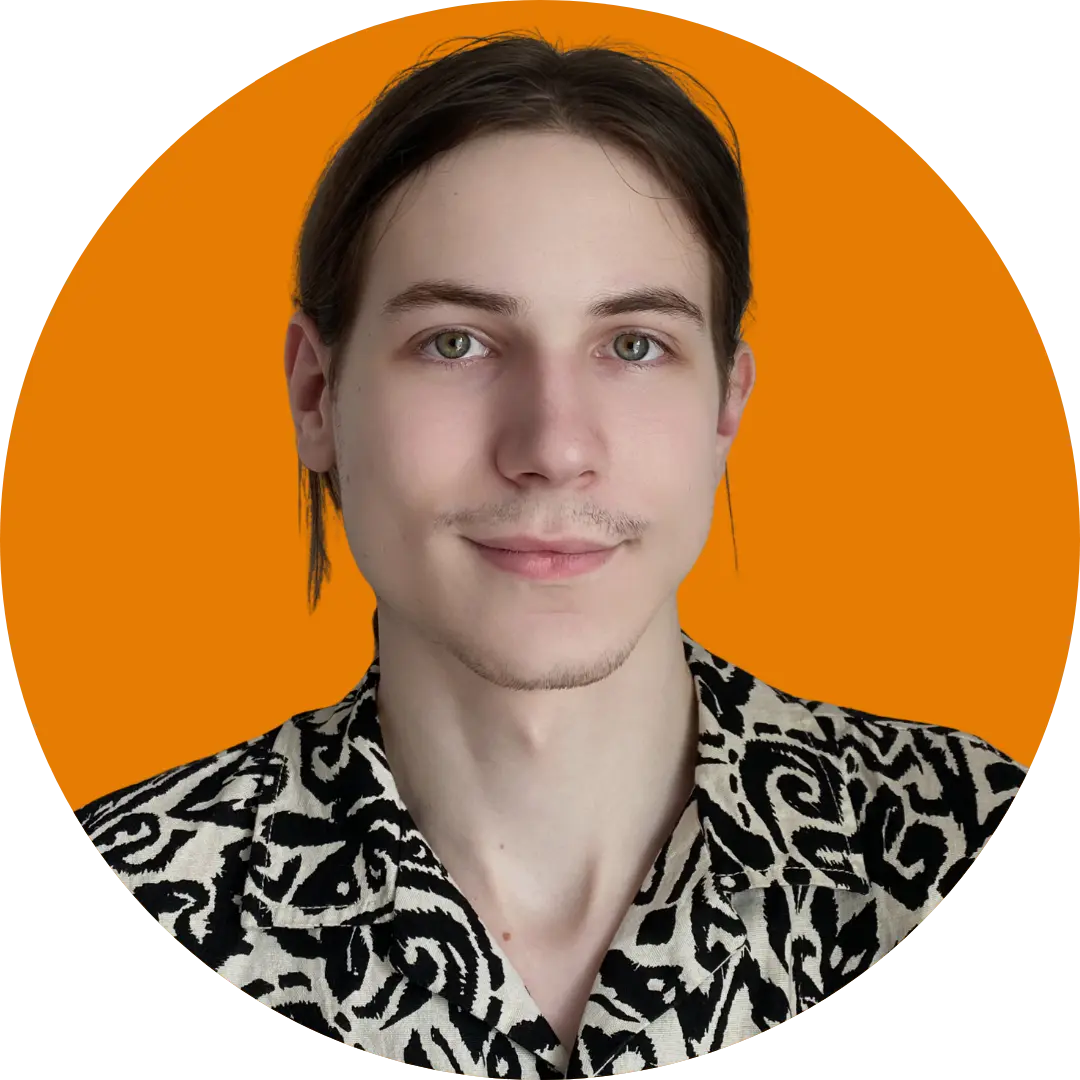
by Ihor Gudzyk
C++ Developer
Feb, 2024・7 min read
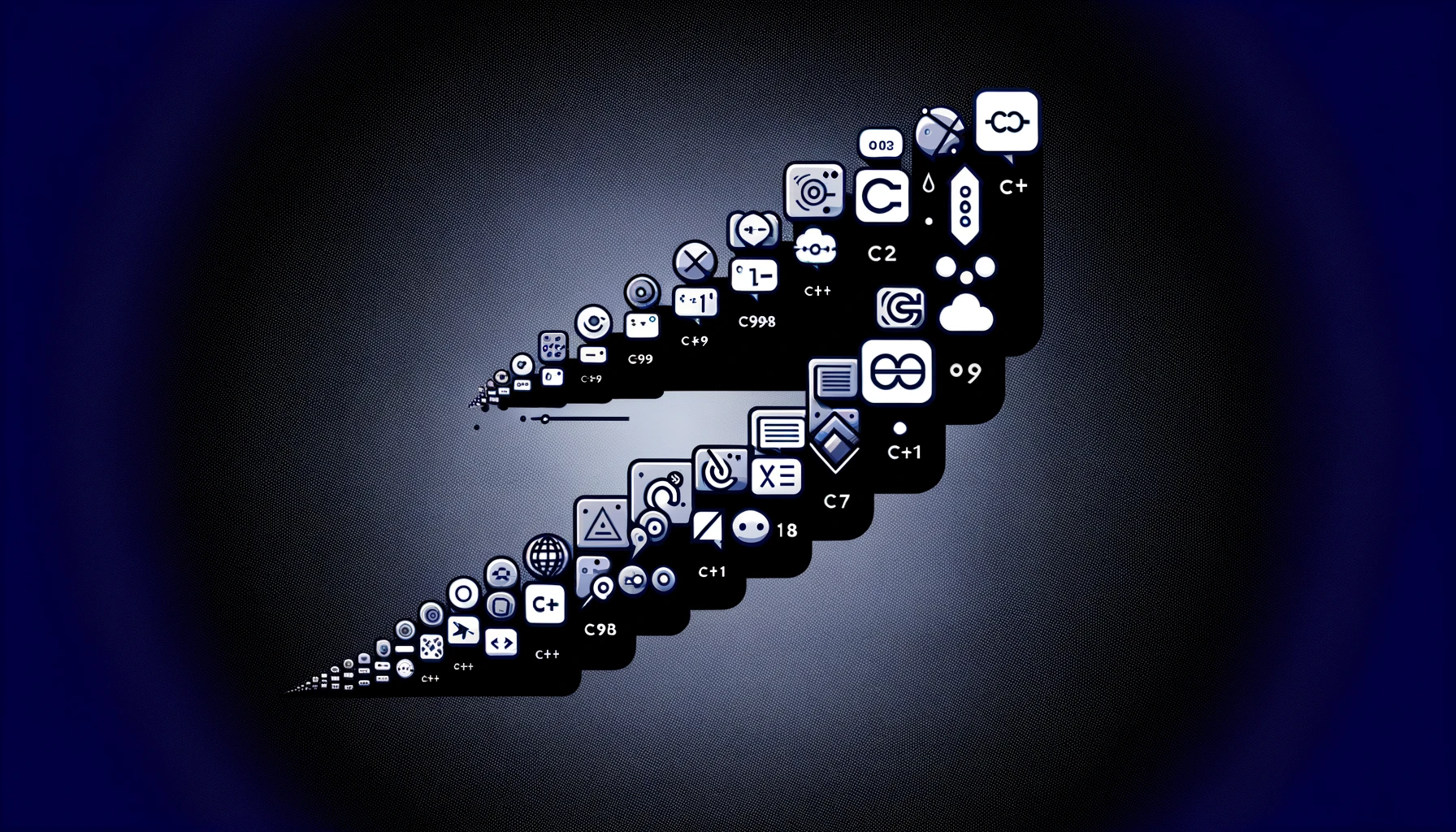
Зміст