Related courses
See All CoursesBeginner
Introduction to Python
Python is an interpreted high-level general-purpose programming language. Unlike HTML, CSS, and JavaScript, which are primarily used for web development, Python is versatile and can be used in various fields, including software development, data science, and back-end development. In this course, you'll explore the core aspects of Python, and by the end, you'll be crafting your own functions!
Beginner
C++ Introduction
Start your path to becoming a skilled developer by mastering the foundational principles of programming through C++. Whether you're starting from scratch or already have some coding experience, this course will provide you with the solid foundation needed to become a proficient developer and open the doors to a wide range of career opportunities in software development and engineering. Let's study C++!
Beginner
Java Basics
This course will familiarize you with Java and its features. After completing the course, you will be able to solve simple algorithmic tasks and understand how basic console Java applications work.
The SOLID Principles in Software Development
The SOLID Principles Overview
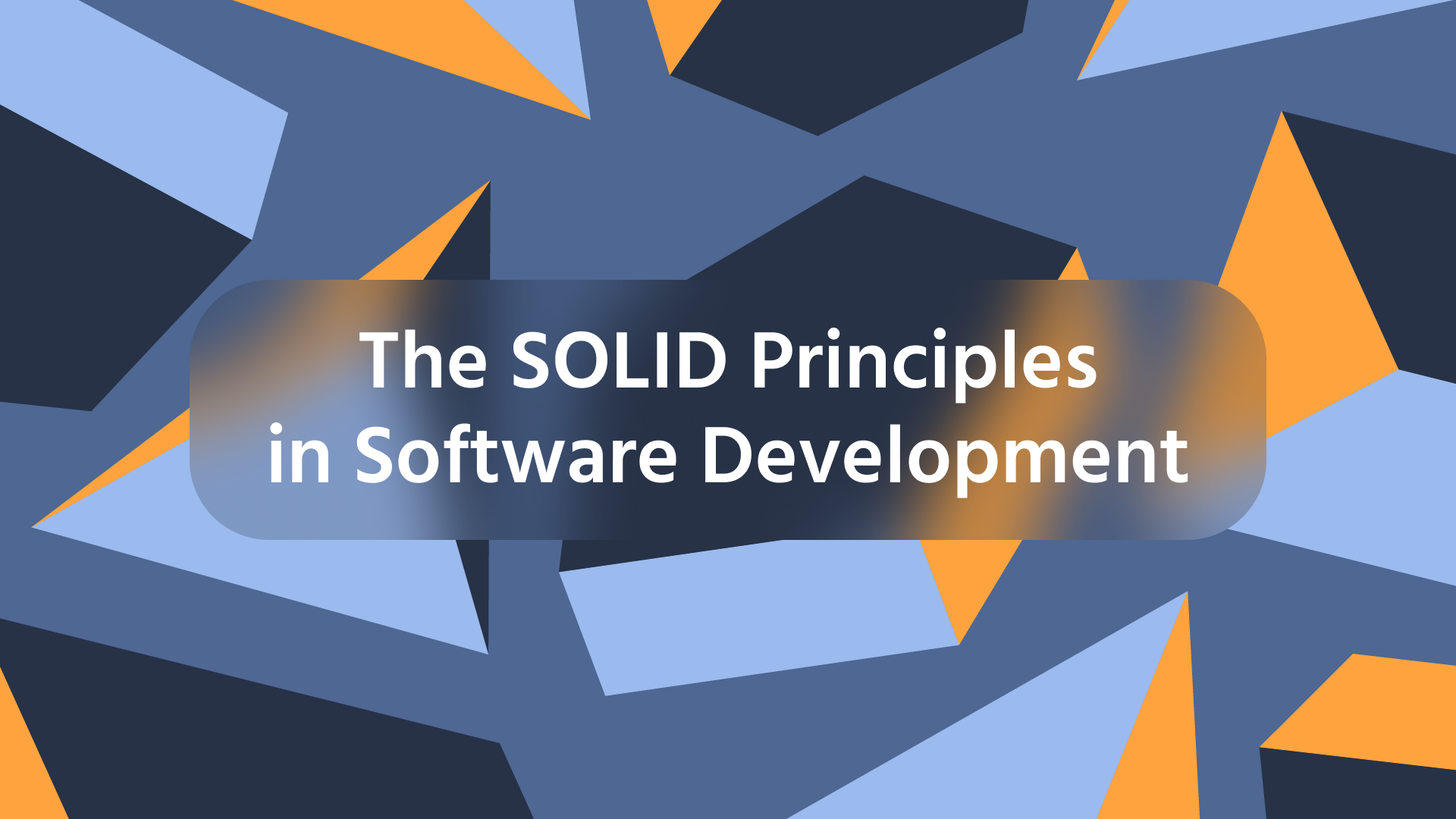
Understanding SOLID coding principles is essential for creating maintainable and scalable software. SOLID principles coding focuses on five key design principles: Single Responsibility, Open-Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion. These principles form the foundation of solid software development, especially for those working in object-oriented programming. By applying SOLID principles software, developers can create systems that are more flexible, easier to understand, and maintain over time.
Single Responsibility Principle (SRP)
Concept:
The Single Responsibility Principle states that a class should have only one reason to change, meaning it should only have one job or responsibility.
Benefits:
- Easier to Test: Classes with a single responsibility are simpler to understand and test.
- Reduced Complexity: Limits the impact of changes, as each class is only focused on one task.
Example:
Consider a class Report. Instead of giving it methods for both generating and printing a report, separate these functions into two classes: ReportGenerator and ReportPrinter.
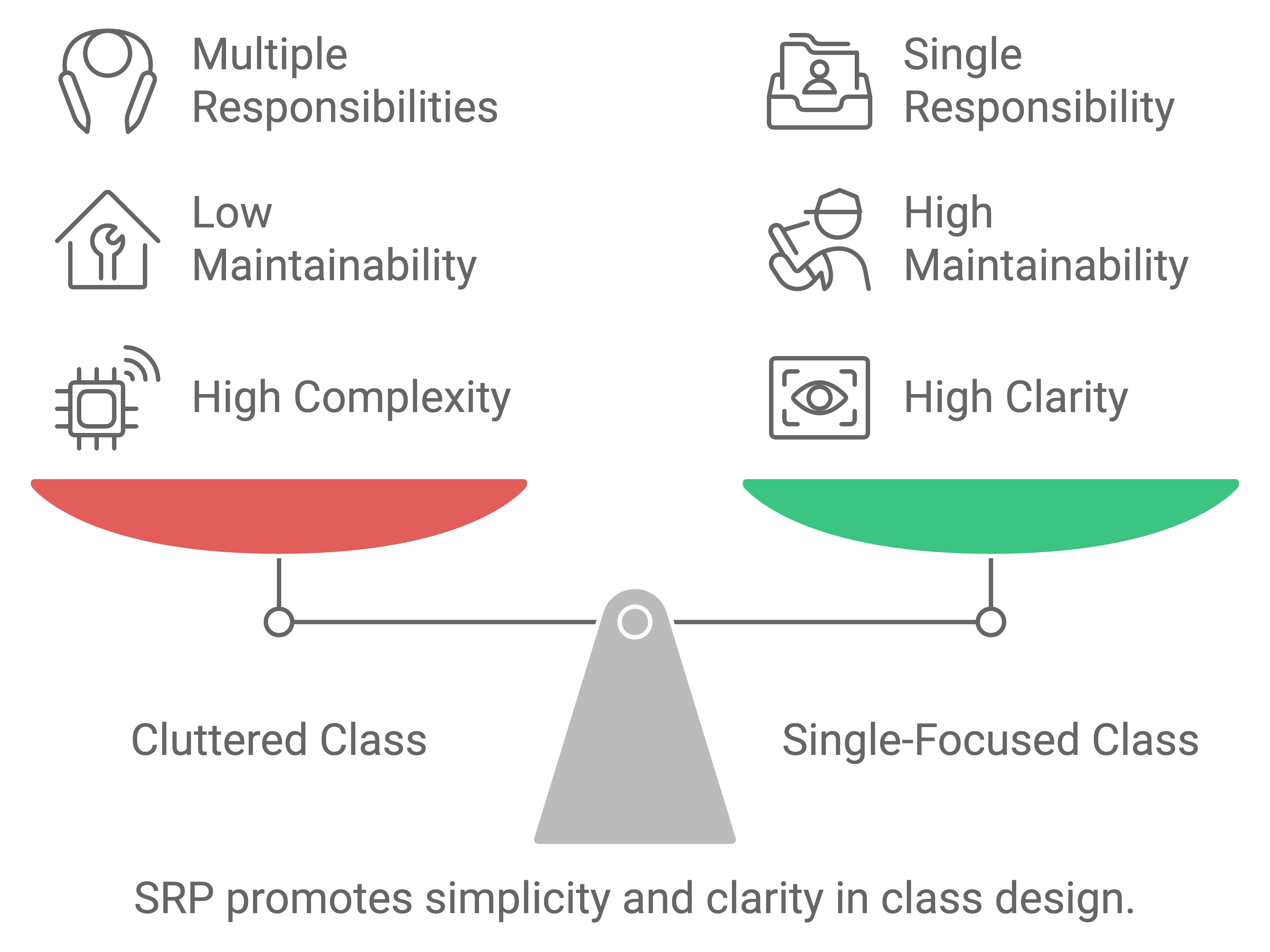
Open-Closed Principle (OCP)
Concept:
Software entities should be open for extension but closed for modification. This means you should be able to add new functionality without changing the existing code.
Benefits:
- Scalability: Facilitates the addition of new features without modifying existing code.
- Stability: Reduces the risk of breaking existing functionality.
Example:
An Invoice class can be extended to support different types of invoices, like ProformaInvoice or CreditInvoice, without modifying the original Invoice class.
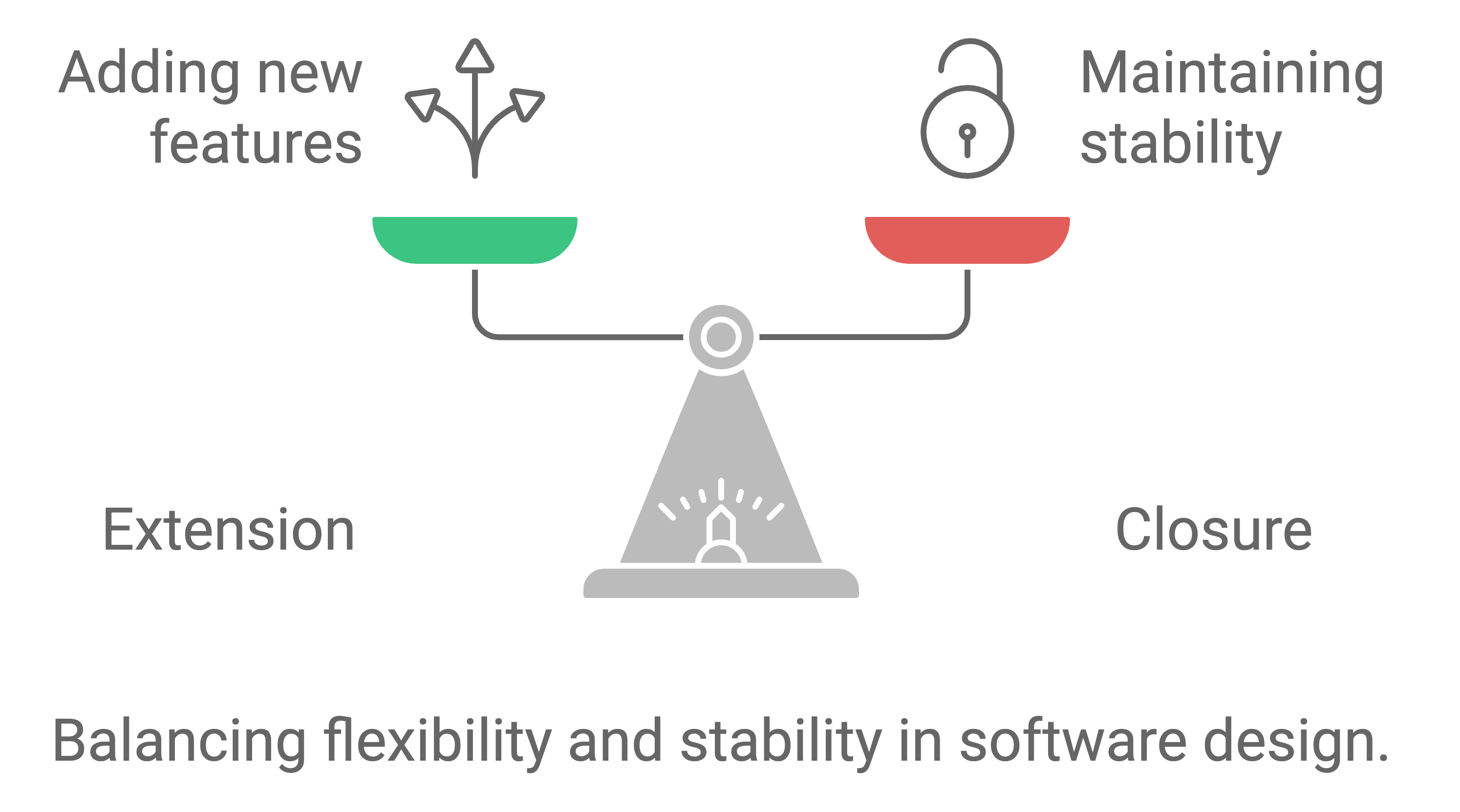
Run Code from Your Browser - No Installation Required
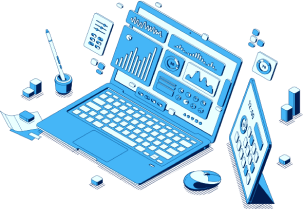
Liskov Substitution Principle (LSP)
Concept:
Objects of a superclass should be replaceable with objects of its subclasses without affecting the correctness of the program.
Benefits:
- Interchangeability: Ensures that subclasses can stand in for their parent classes.
- Robust Design: Promotes the correctness of inheritance hierarchies.
Example:
If Bird is a superclass, and Duck is a subclass, then you should be able to replace Bird with Duck without altering the program's behavior.
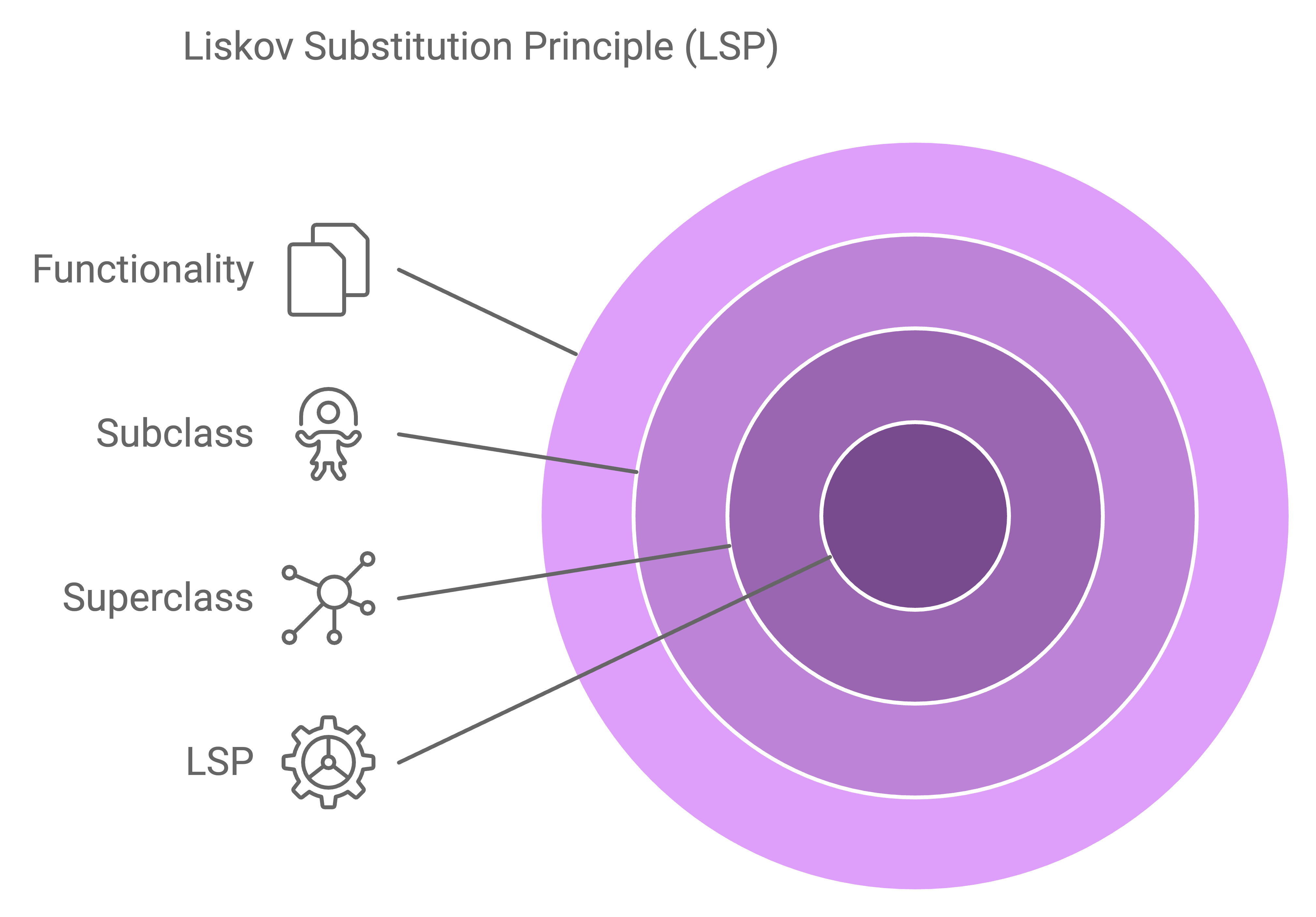
Interface Segregation Principle (ISP)
Concept:
Clients should not be forced to depend on interfaces they do not use. This principle suggests splitting large interfaces into smaller ones.
Benefits:
- Flexibility: Clients only need to know about the methods that are of interest to them.
- Maintainability: Easier to make changes as changes in one part of the system are less likely to affect other parts.
Example:
Instead of one large Worker interface, have separate interfaces like Workable, Feedable, Maintainable for different types of work.
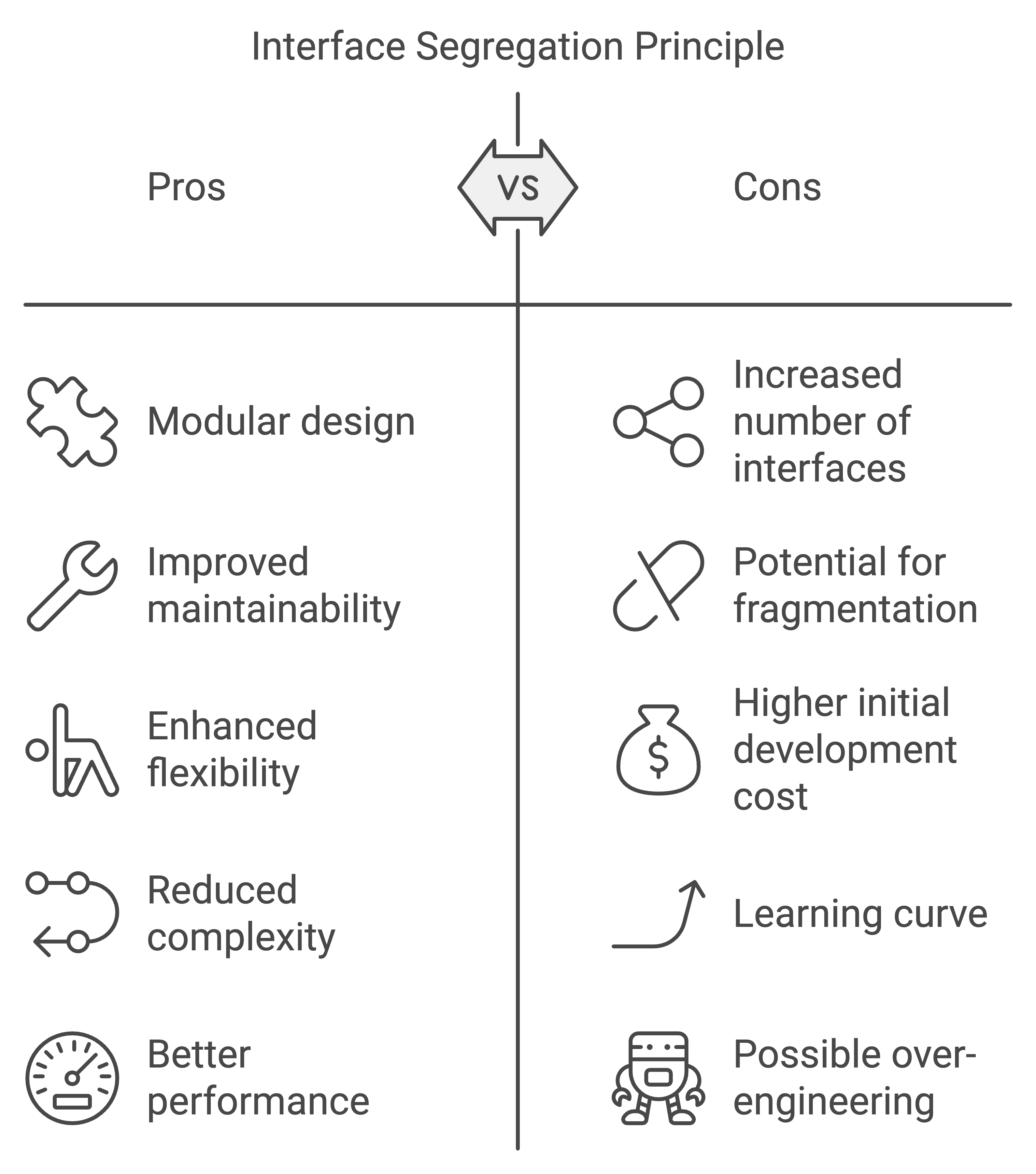
Dependency Inversion Principle (DIP)
Concept:
High-level modules should not depend on low-level modules. Both should depend on abstractions. Additionally, abstractions should not depend on details, but details should depend on abstractions.
Benefits:
- Decoupling: Reduces the dependency between different parts of the code.
- Flexibility: Easier to refactor, change, and deploy.
Example:
An OrderProcessor class should depend on an IOrder interface, not on a concrete Order class. This makes it easy to introduce new types of orders.
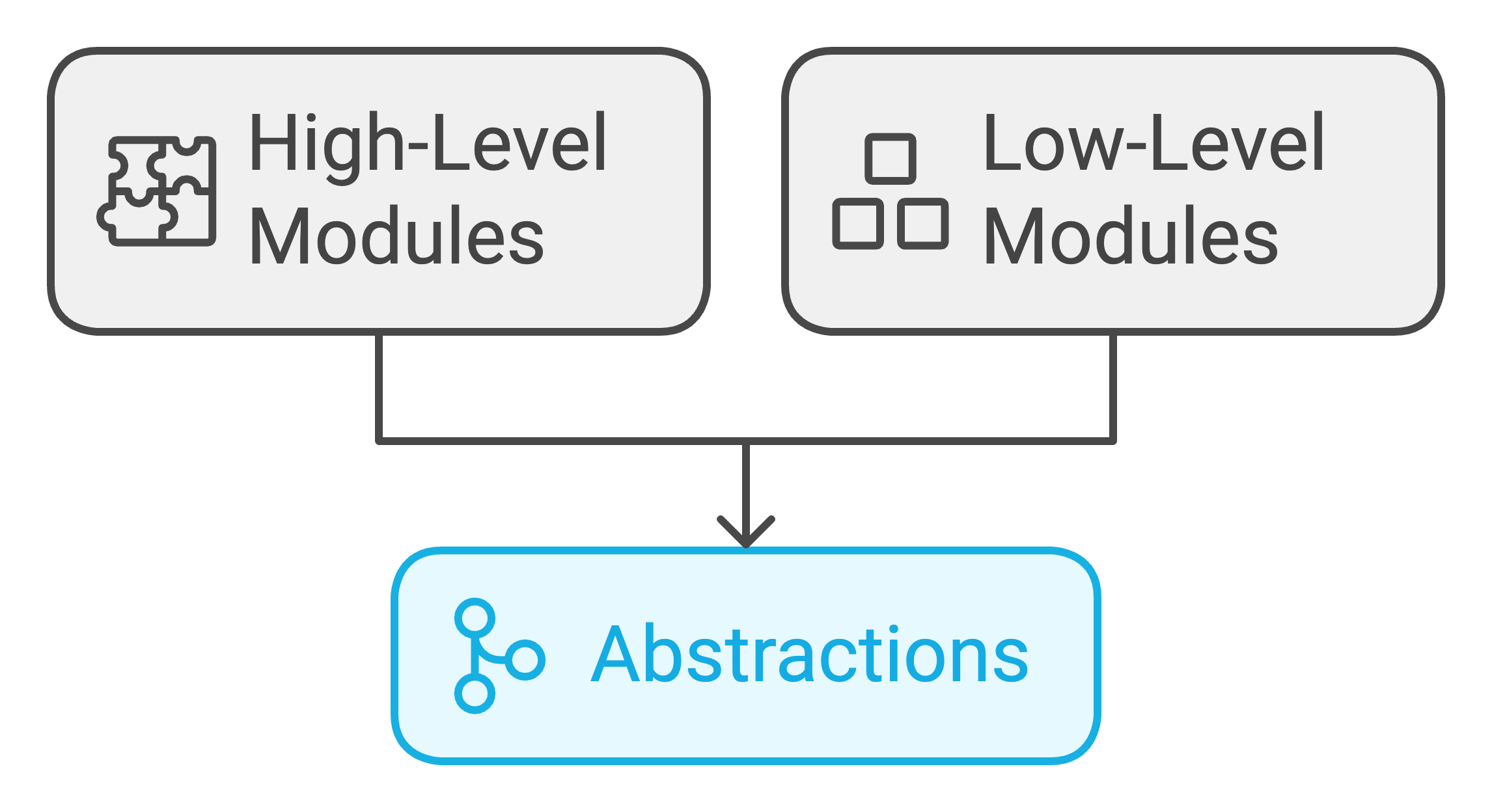
Conclusion
Incorporating SOLID coding principles into your development process is crucial for building robust and scalable software. These principles, from Single Responsibility to Dependency Inversion, provide a framework for solid software development that ensures flexibility, maintainability, and a clear structure. Mastering SOLID principles coding not only improves your coding practices but also contributes to better SOLID principles software design, making your applications easier to modify and extend in the long run.
Start Learning Coding today and boost your Career Potential
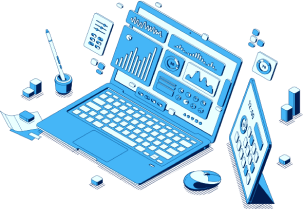
FAQ
Q: Why are SOLID principles important in software development?
A: SOLID coding principles help in creating software that is easier to maintain, understand, and extend. They encourage developers to create more modular, scalable, and robust systems by promoting best practices in design.
Q: Can SOLID principles be applied to other programming paradigms apart from OOP?
A: While SOLID principles coding are primarily designed for object-oriented programming, the core concepts of modularity, maintainability, and scalability can be adapted to other paradigms such as functional programming or procedural programming.
Q: How do SOLID principles impact team collaboration?
A: By adhering to SOLID principles software, teams can ensure that their codebase is clean, consistent, and easy to manage. This improves communication between team members, reduces bugs, and allows for faster onboarding of new developers.
Q: Are there any downsides to using SOLID principles?
A: Although SOLID coding principles are highly valuable, over-applying them can lead to over-engineering. It's important to strike a balance, applying these principles in situations where they are most beneficial, rather than forcing them into every aspect of the project.
Q: Can SOLID principles be applied to modern software development practices like microservices?
A: Yes, SOLID principles software can be applied in modern architectures like microservices. However, microservices are often more focused on decoupling entire services rather than individual classes, so the principles should be applied with that larger context in mind. For example, the Single Responsibility Principle can be used to design microservices with a specific, well-defined purpose.
Q: How do SOLID principles interact with other design patterns?
A: SOLID principles coding often complement design patterns such as Factory, Singleton, or Strategy. These principles help guide when and how to use design patterns effectively to create modular and flexible systems.
Q: Are SOLID principles still relevant for newer programming languages and frameworks?
A: Absolutely. SOLID software development principles remain relevant regardless of language or framework, as they promote general best practices for design that stand the test of time. Even in modern frameworks like React, Vue, or Angular, understanding these principles can guide developers in writing better-structured and more maintainable code.
Q: What should I prioritize if I can't apply all SOLID principles?
A: It’s not always feasible to apply every SOLID principle in every situation. Focus on the ones that provide the most immediate benefit to your project. Single Responsibility and Dependency Inversion are often a good starting point, as they address common challenges in software design.
Learn More
- Martin, Robert C. Agile Software Development, Principles, Patterns, and Practices. Prentice Hall, 2002.
Robert C. Martin (Uncle Bob) – The creator of the SOLID principles. His book is a foundational resource for understanding these principles.
- Feathers, Michael. Working Effectively with Legacy Code. Prentice Hall, 2004.
The person who actually coined the acronym SOLID to describe Martin’s five principles in the context of object-oriented design.
- Gamma, Erich, Richard Helm, Ralph Johnson, and John Vlissides. Design Patterns: Elements of Reusable Object-Oriented Software. Addison-Wesley, 1994.
This seminal work on design patterns forms a base for understanding object-oriented design, which is essential for SOLID principles.
Related courses
See All CoursesBeginner
Introduction to Python
Python is an interpreted high-level general-purpose programming language. Unlike HTML, CSS, and JavaScript, which are primarily used for web development, Python is versatile and can be used in various fields, including software development, data science, and back-end development. In this course, you'll explore the core aspects of Python, and by the end, you'll be crafting your own functions!
Beginner
C++ Introduction
Start your path to becoming a skilled developer by mastering the foundational principles of programming through C++. Whether you're starting from scratch or already have some coding experience, this course will provide you with the solid foundation needed to become a proficient developer and open the doors to a wide range of career opportunities in software development and engineering. Let's study C++!
Beginner
Java Basics
This course will familiarize you with Java and its features. After completing the course, you will be able to solve simple algorithmic tasks and understand how basic console Java applications work.
30 Python Project Ideas for Beginners
Python Project Ideas
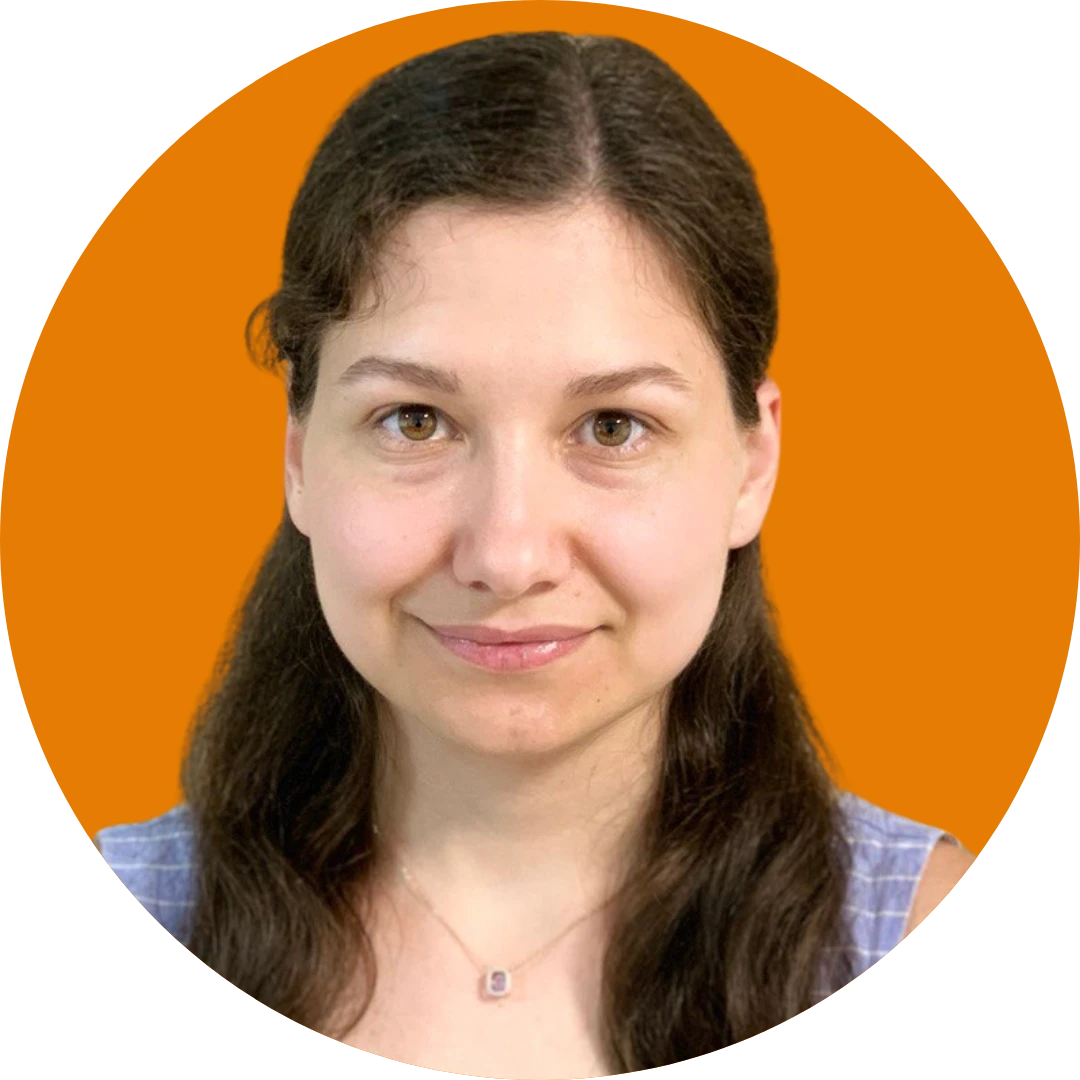
by Anastasiia Tsurkan
Backend Developer
Sep, 2024・14 min read
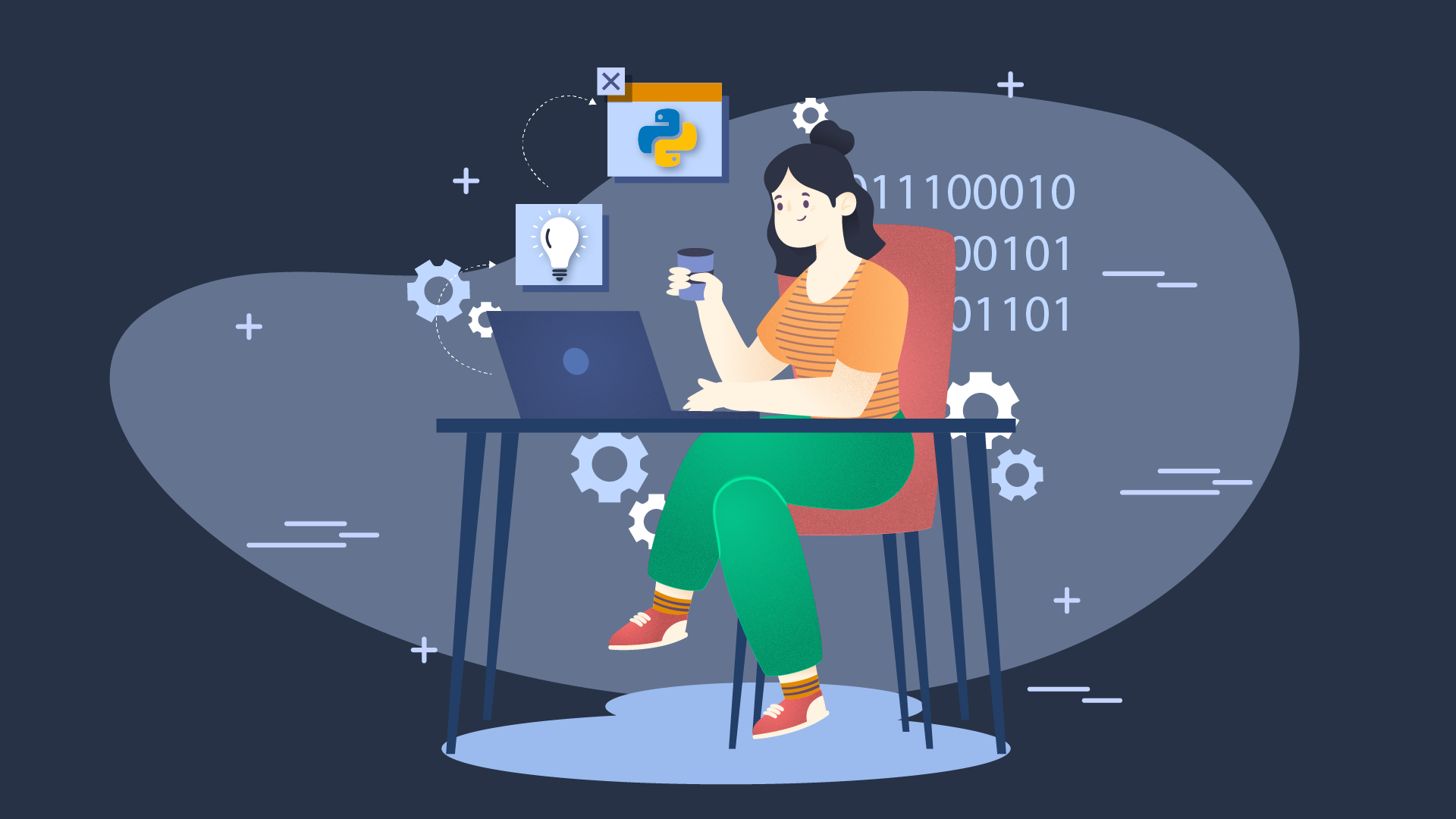
Coding for Beginners The Ultimate Guide on How to Start
Road map for beginer
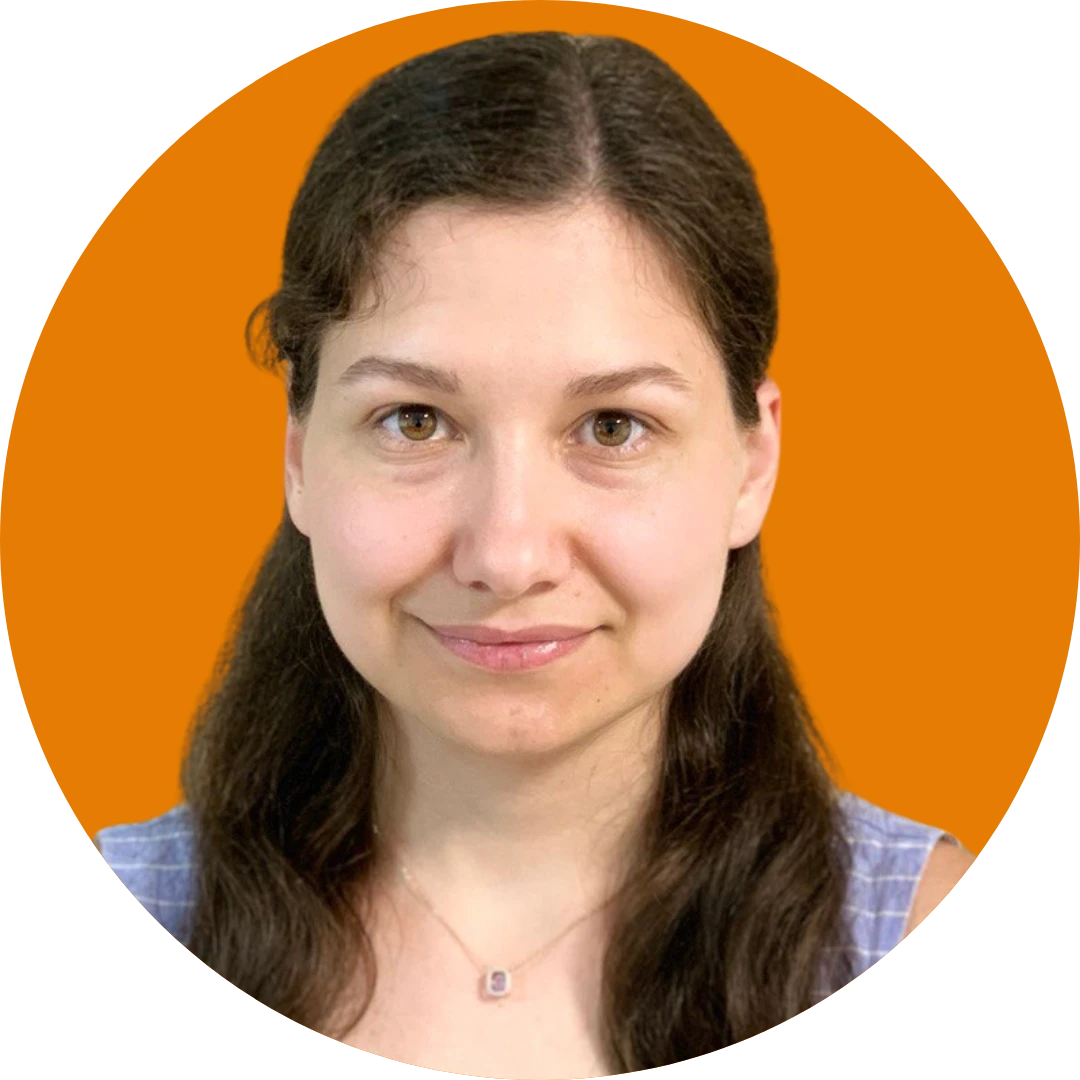
by Anastasiia Tsurkan
Backend Developer
Nov, 2023・10 min read
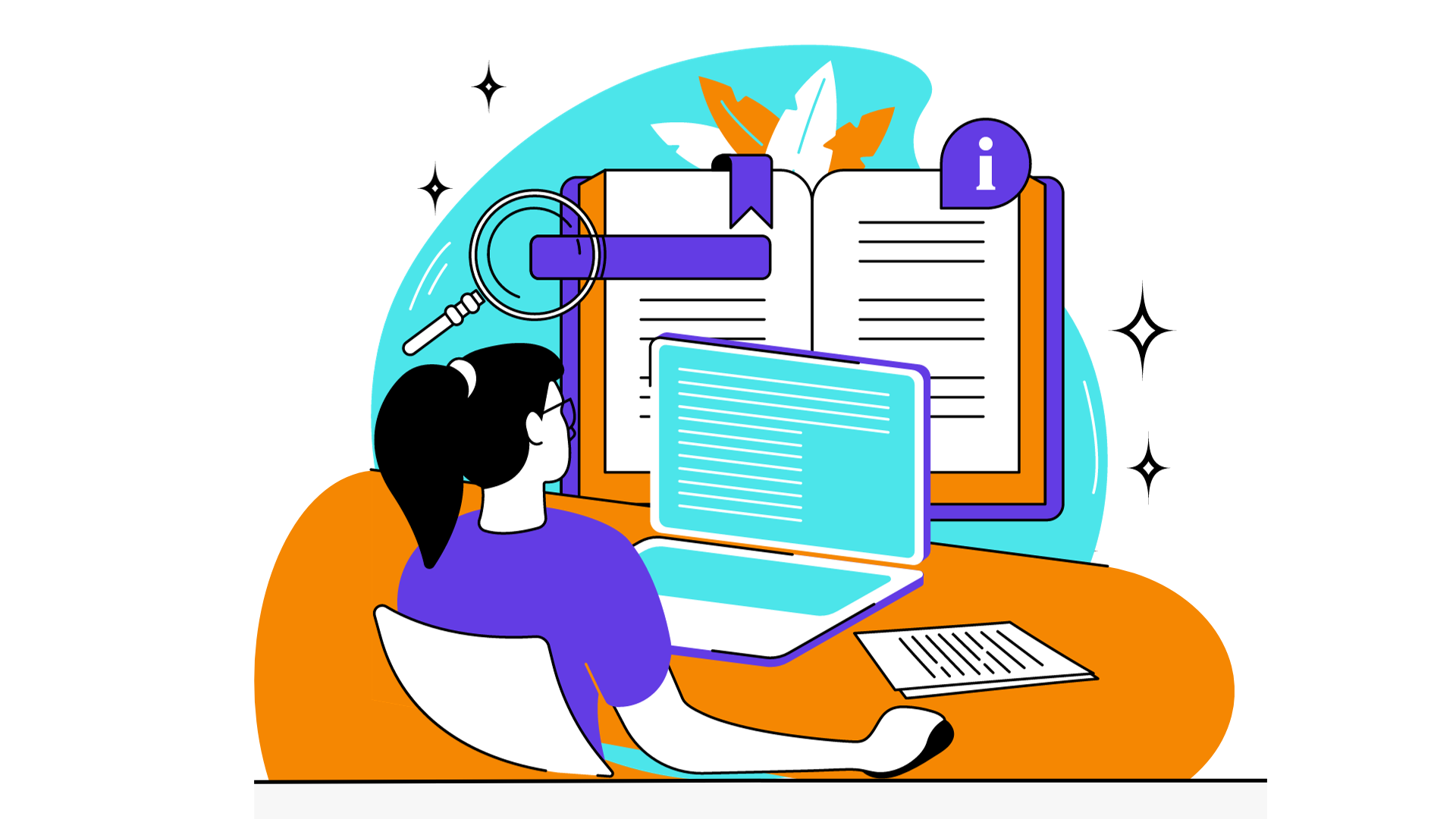
How Information Moves Across the Internet
Understanding Data Movement Through OSI and TCP/IP Models
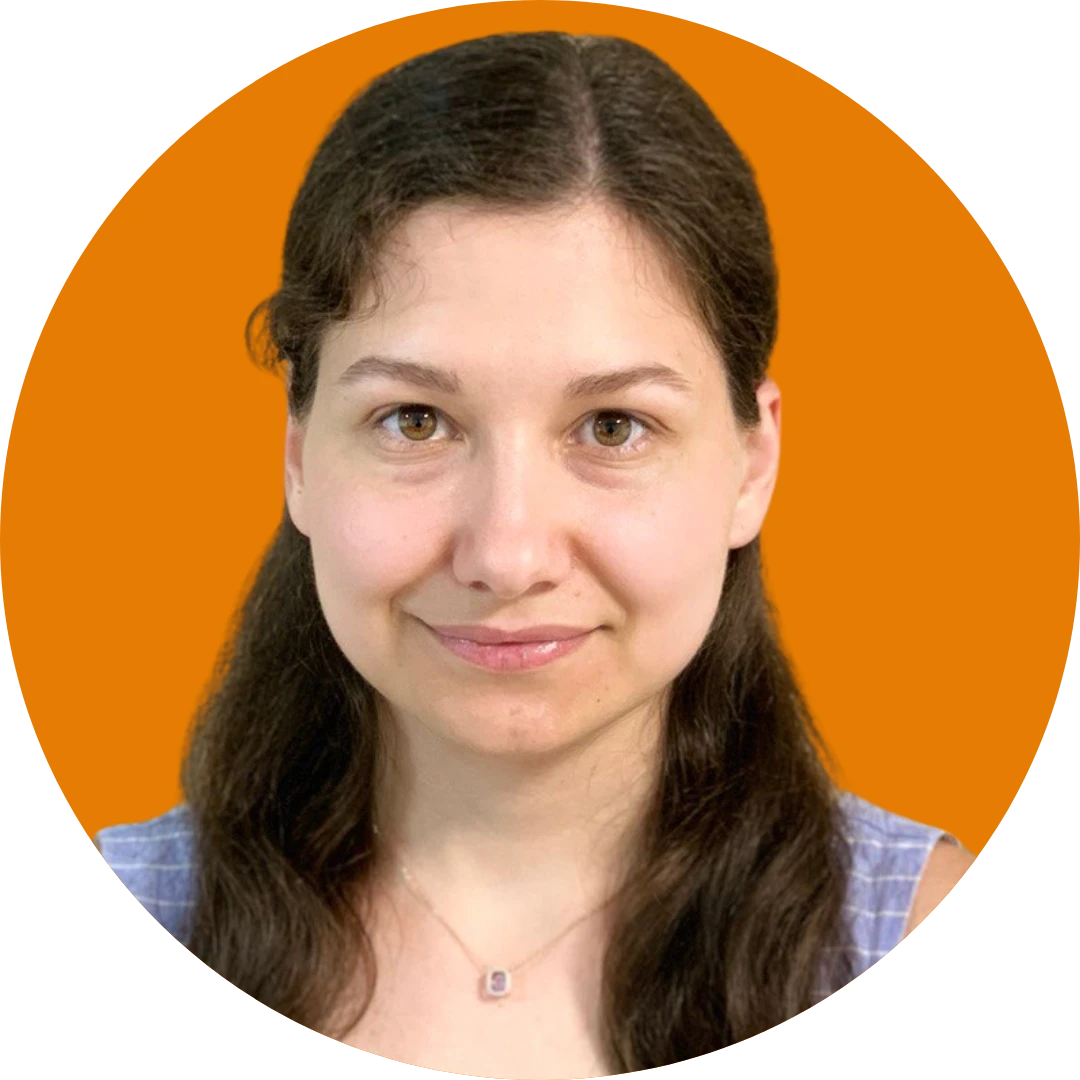
by Anastasiia Tsurkan
Backend Developer
Jan, 2024・6 min read
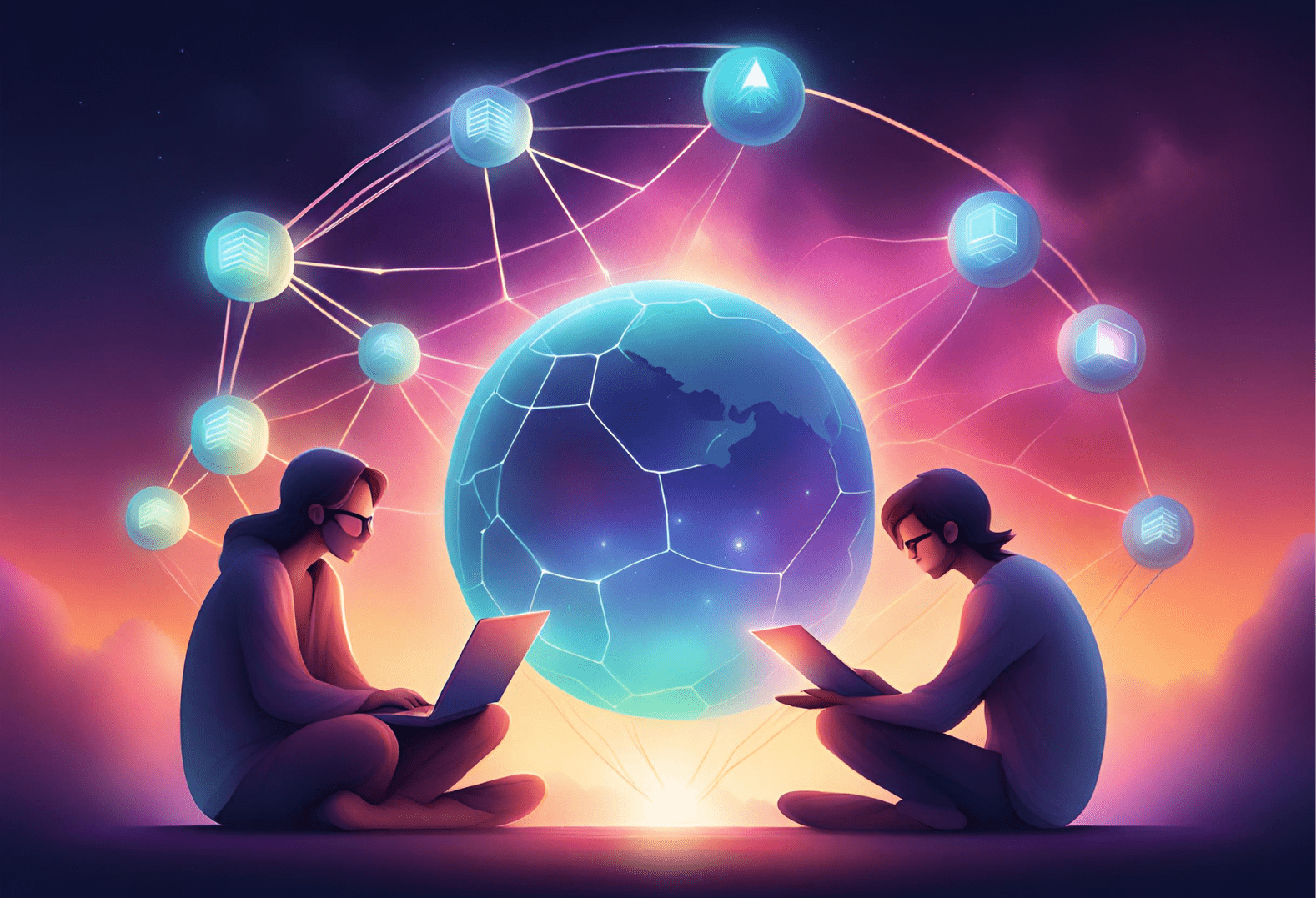
Content of this article