Related courses
See All CoursesBeginner
C# Basics
Get ready to embark on a thrilling coding journey with C# - the language that powers Windows applications, games, and more. Unlock the potential to build everything from dynamic web apps to powerful desktop software. With its elegance, performance, and versatility, C# is your gateway to the future of programming. Let's dive in and bring your coding dreams to life!
Intermediate
C# Beyond Basics
This is an intermediate course for C# which helps in leveling up the users skills hence enabling the user to develop more advanced applications employing more complex data structures and techniques like Object Oriented Programming.
What is the StringBuilder in C#
The Power of StringBuilder in C#
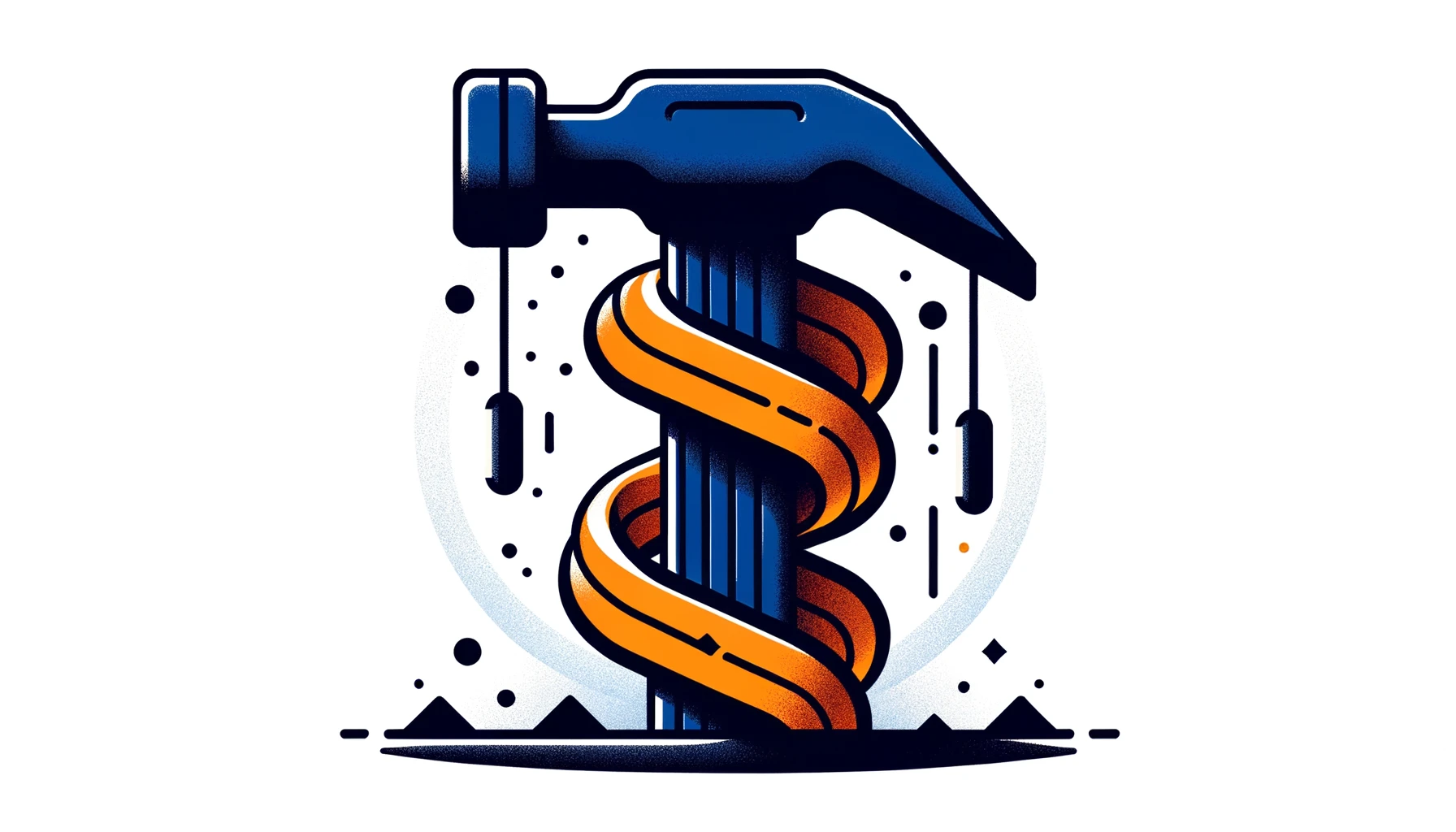
When it comes to optimizing string manipulation in C#, the StringBuilder class is a powerful tool that can significantly improve performance and memory usage compared to traditional string concatenation methods. In this article, we'll explore what StringBuilder is, how it works, and when to use it in your C# code.
What is StringBuilder
StringBuilder is a class in the System.Text namespace in C# that represents a mutable string of characters. Unlike the traditional string class, which is immutable (meaning once a string is created, it cannot be changed), StringBuilder allows you to modify the content of the string without creating a new string object every time a modification is made.
Run Code from Your Browser - No Installation Required
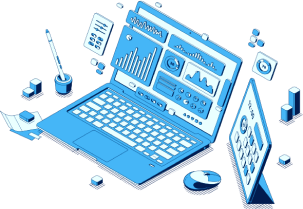
How is StringBuilder Works
When you manipulate strings using the standard concatenation operator (+
), a new string object is created for each concatenation operation. This can lead to performance issues, especially when dealing with large strings or a large number of concatenations.
StringBuilder, on the other hand, uses a resizable buffer to store the string content. When you append or modify the content of a StringBuilder object, it directly manipulates this buffer, avoiding the need to create new string objects. This results in more efficient string manipulation, especially for operations that involve multiple concatenations.
Using StringBuilder in C#
Here's a basic example of how you can use StringBuilder in C#:
In this example, we create a StringBuilder object sb
and append two strings to it. Finally, we use the ToString()
method to convert the StringBuilder object back to a regular string and print it to the console.
When to Use StringBuilder
StringBuilder is particularly useful in scenarios where you need to concatenate multiple strings or modify a string multiple times, such as building a long string from smaller parts or constructing dynamic SQL queries. By using StringBuilder, you can avoid the performance overhead associated with creating new string objects for each operation.
However, it's important to note that StringBuilder is not always necessary. For simple string manipulations or when performance is not a critical factor, using the standard string class or string interpolation ($""
) may be more appropriate for readability and simplicity.
Start Learning Coding today and boost your Career Potential
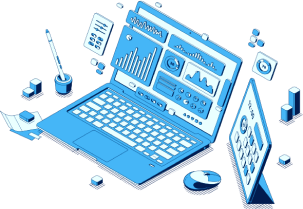
Conclusion
In conclusion, StringBuilder is a powerful tool in C# for efficient string manipulation. By using StringBuilder, you can improve the performance and memory usage of your string operations, especially when dealing with large strings or a large number of concatenations. While StringBuilder is not always necessary, understanding how and when to use it can help you write more efficient and optimized C# code.
FAQs
Q: What is StringBuilder in C#?
A: StringBuilder is a class in the System.Text namespace in C# that represents a mutable string of characters. Unlike the traditional string class, which is immutable, StringBuilder allows you to modify the content of the string without creating a new string object every time a modification is made.
Q: How does StringBuilder improve string manipulation performance?
A: When you manipulate strings using the standard concatenation operator (+
), a new string object is created for each concatenation operation. StringBuilder uses a resizable buffer to store the string content. When you append or modify the content of a StringBuilder object, it directly manipulates this buffer, avoiding the need to create new string objects. This results in more efficient string manipulation, especially for operations that involve multiple concatenations.
Q: When should I use StringBuilder in my C# code?
A: StringBuilder is particularly useful in scenarios where you need to concatenate multiple strings or modify a string multiple times, such as building a long string from smaller parts or constructing dynamic SQL queries. By using StringBuilder, you can avoid the performance overhead associated with creating new string objects for each operation. However, it's important to note that StringBuilder is not always necessary. For simple string manipulations or when performance is not a critical factor, using the standard string class or string interpolation ($""
) may be more appropriate for readability and simplicity.
Q: Can I convert a StringBuilder object back to a regular string?
A: Yes, you can convert a StringBuilder object back to a regular string using the ToString()
method. This method returns the contents of the StringBuilder object as a regular string, allowing you to use it in string operations or to display it to the user.
Q: Is StringBuilder the only way to manipulate strings efficiently in C#?
A: No, StringBuilder is not the only way to manipulate strings efficiently in C#. Depending on your requirements, there are other methods and classes you can use. For example, string interpolation ($""
) can be more readable and convenient for simple string concatenations. In some cases, using the String.Concat()
method or String.Join()
method may also be suitable. It's important to choose the right approach based on the specific needs of your application.
Related courses
See All CoursesBeginner
C# Basics
Get ready to embark on a thrilling coding journey with C# - the language that powers Windows applications, games, and more. Unlock the potential to build everything from dynamic web apps to powerful desktop software. With its elegance, performance, and versatility, C# is your gateway to the future of programming. Let's dive in and bring your coding dreams to life!
Intermediate
C# Beyond Basics
This is an intermediate course for C# which helps in leveling up the users skills hence enabling the user to develop more advanced applications employing more complex data structures and techniques like Object Oriented Programming.
The DRY Principle
Maximizing Efficiency in Code and Development
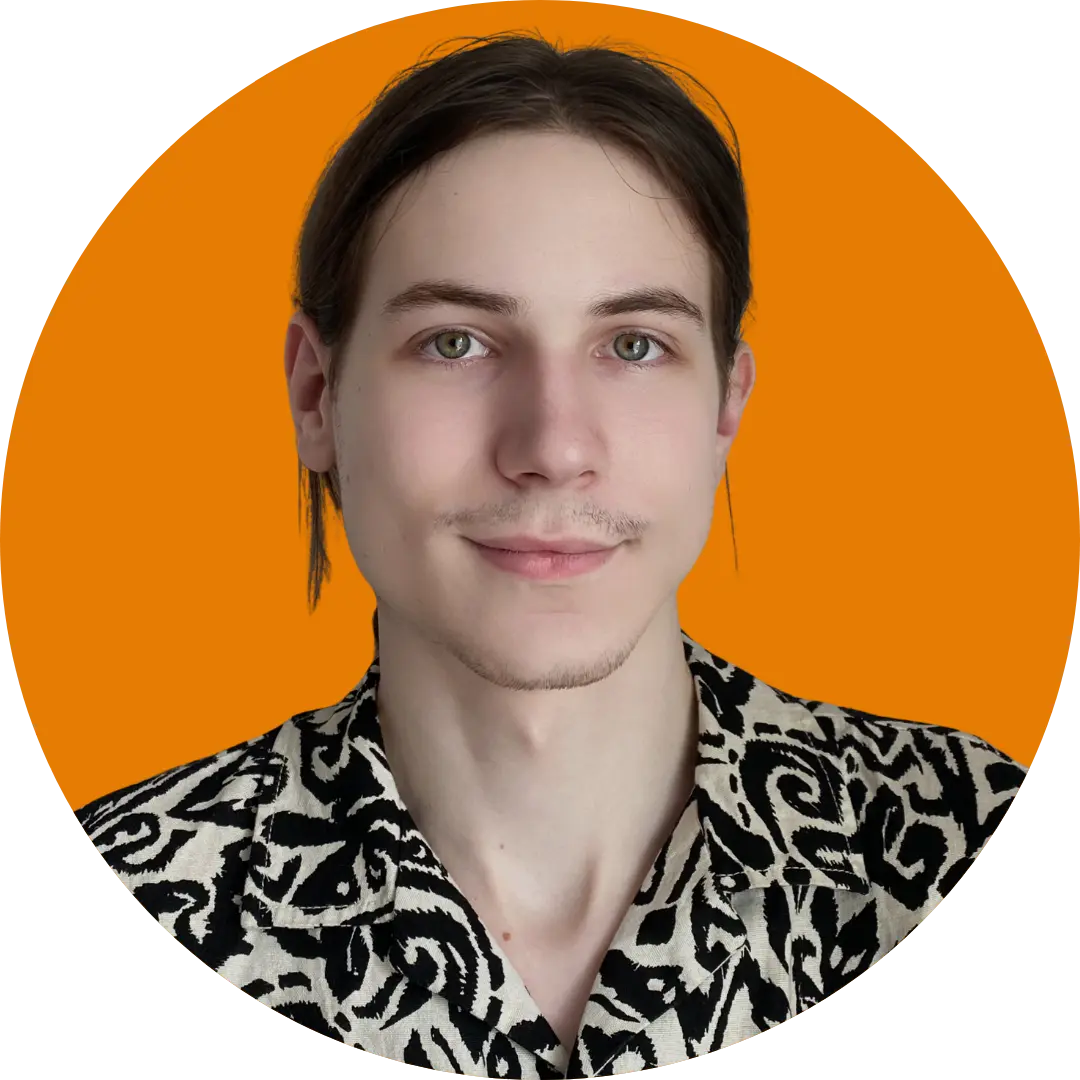
by Ihor Gudzyk
C++ Developer
Aug, 2024・9 min read
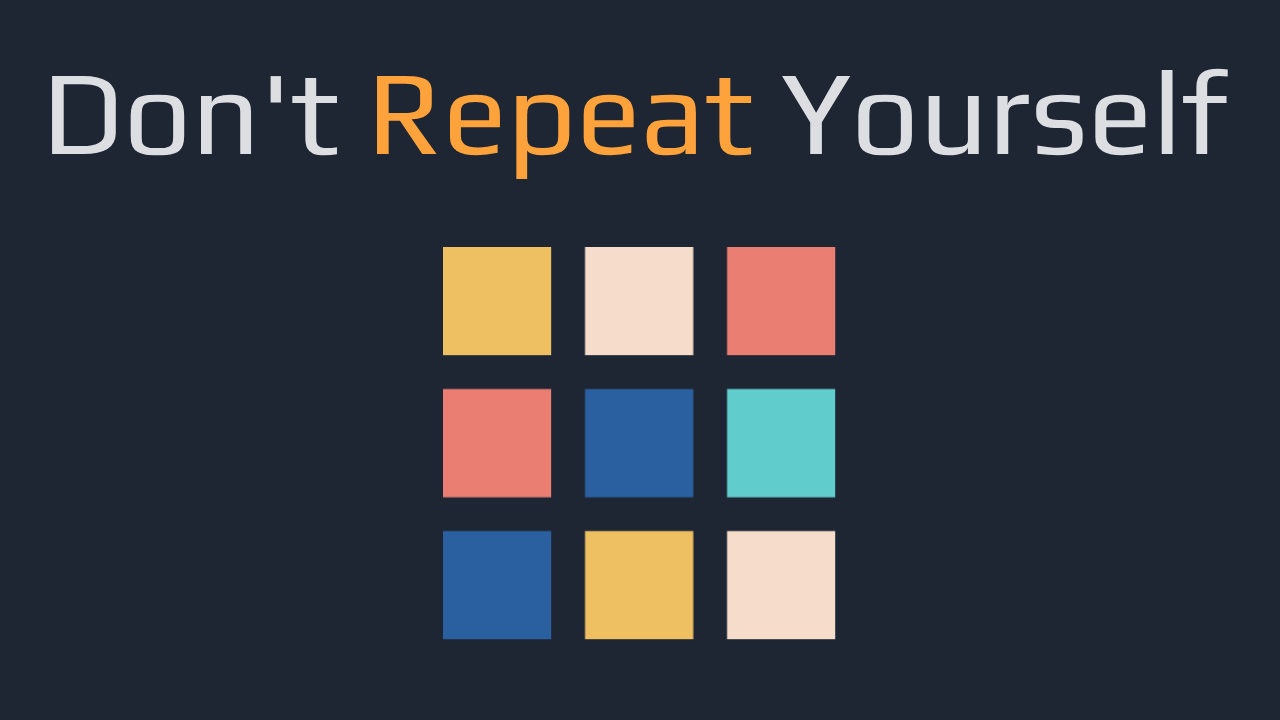
Introduction to Regular Expressions for Beginners
A Guide to Understanding and Using Regex in Programming
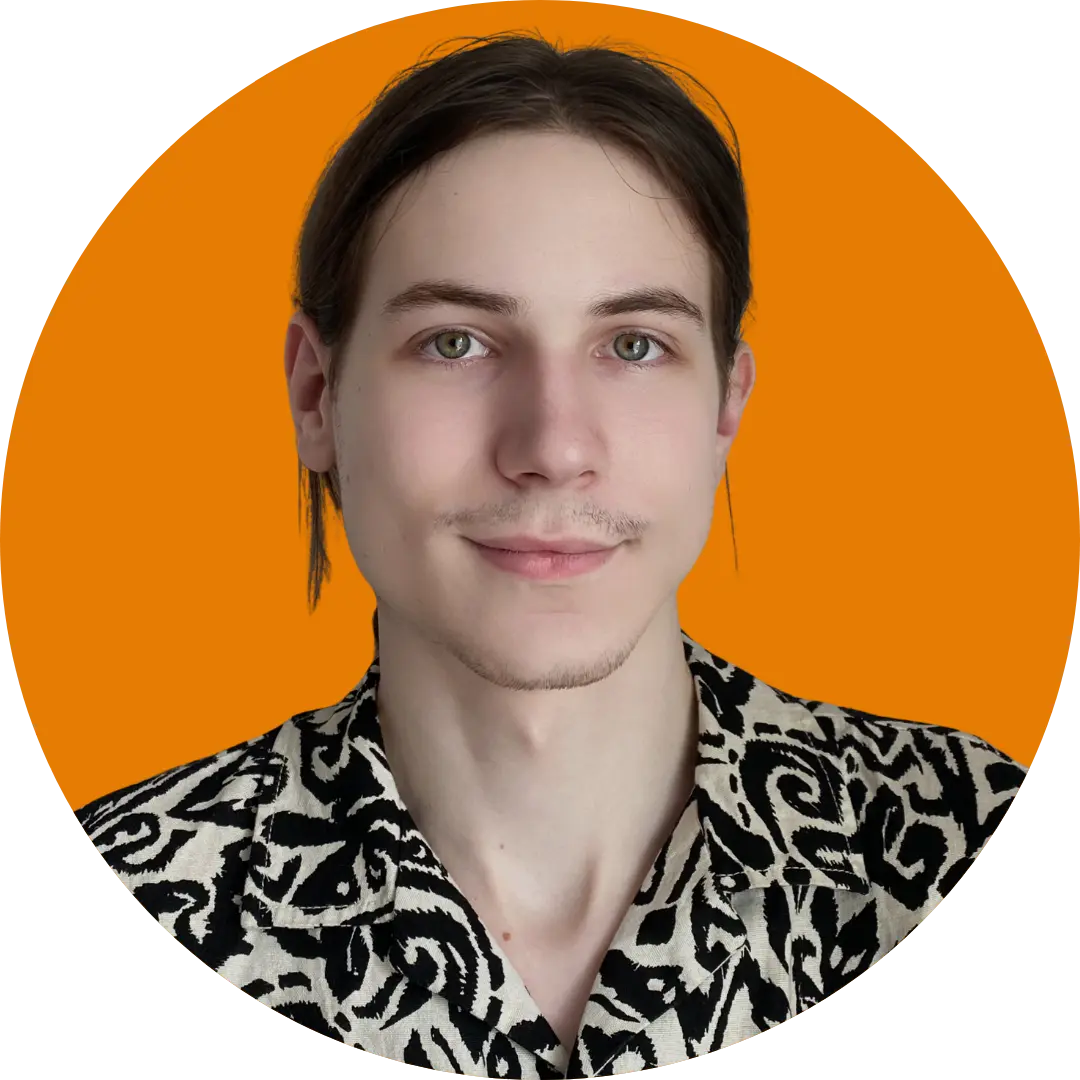
by Ihor Gudzyk
C++ Developer
Sep, 2024・13 min read
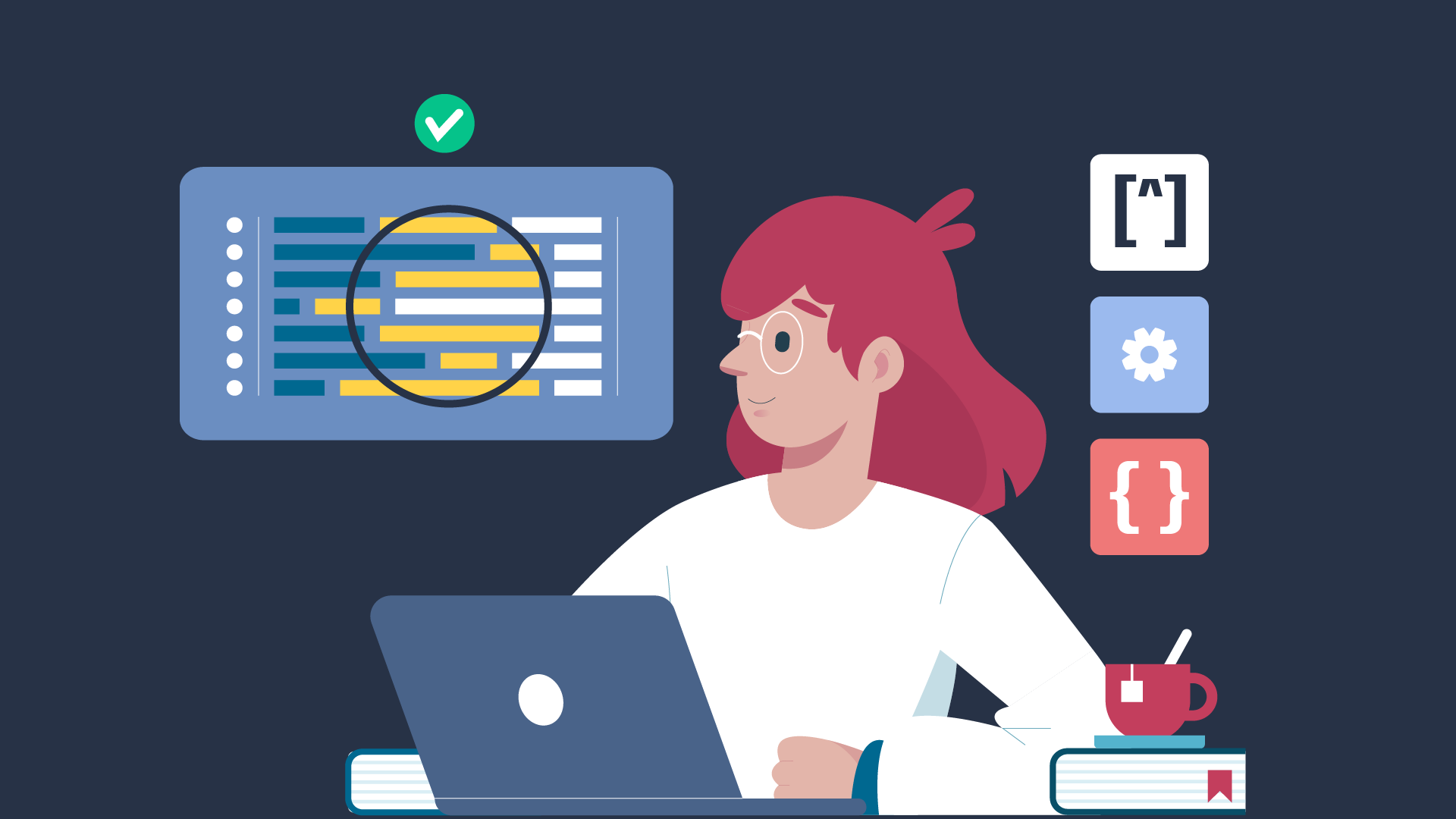
Understanding Preprocessor Directives in C++
The Cornerstone of Efficient C++ Coding
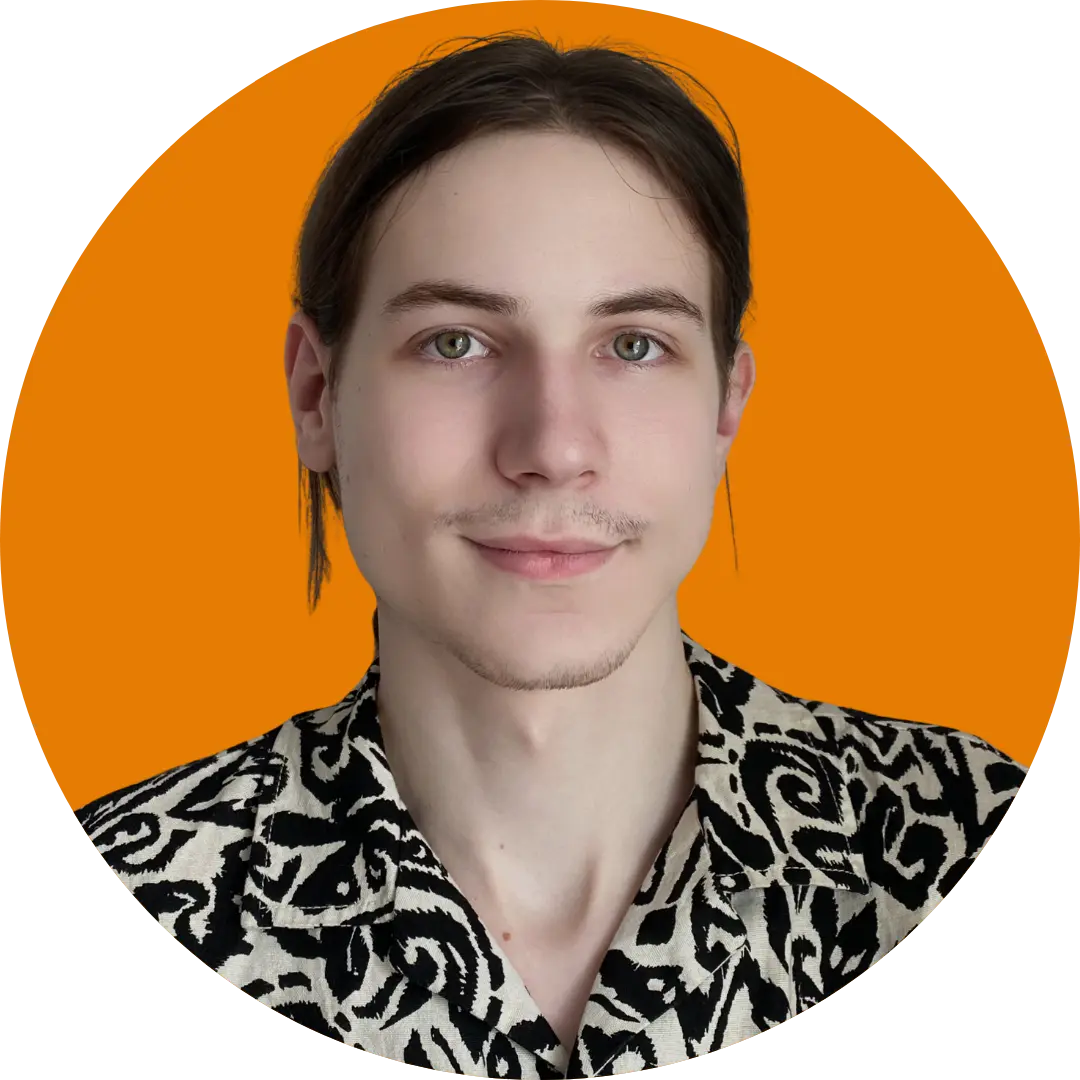
by Ihor Gudzyk
C++ Developer
Dec, 2023・4 min read
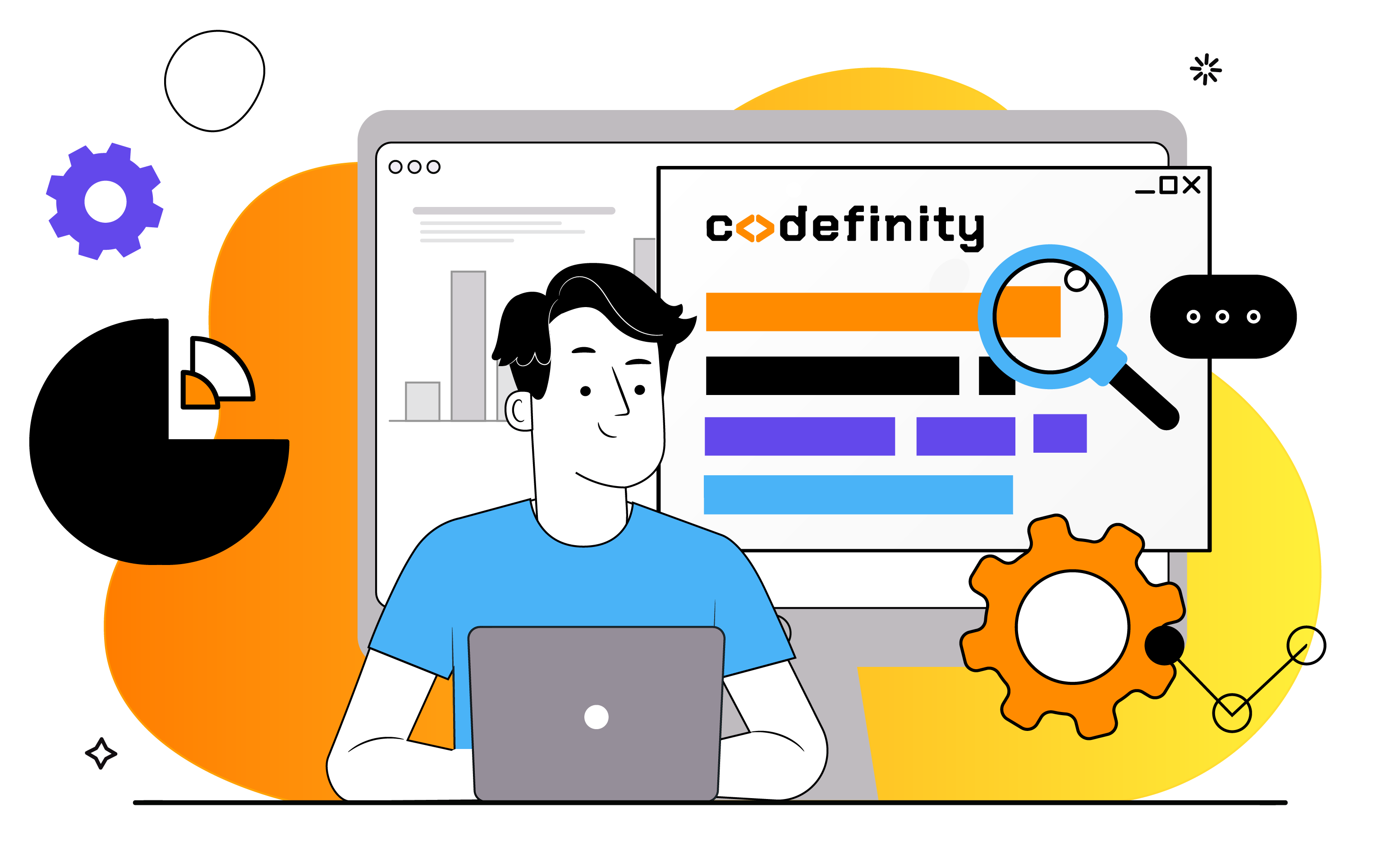
Content of this article