Course Content
C++ OOP
C++ OOP
Introduction to Inheritance
Why do We Need Inheritance
To better understand the concepts of inheritance, let’s look at some practical examples. The primary benefit of inheritance is code reuse. For example, Student and Teacher classes reuse the code from Person (name, age, and display_info method). This reduces redundancy and makes the code more maintainable.
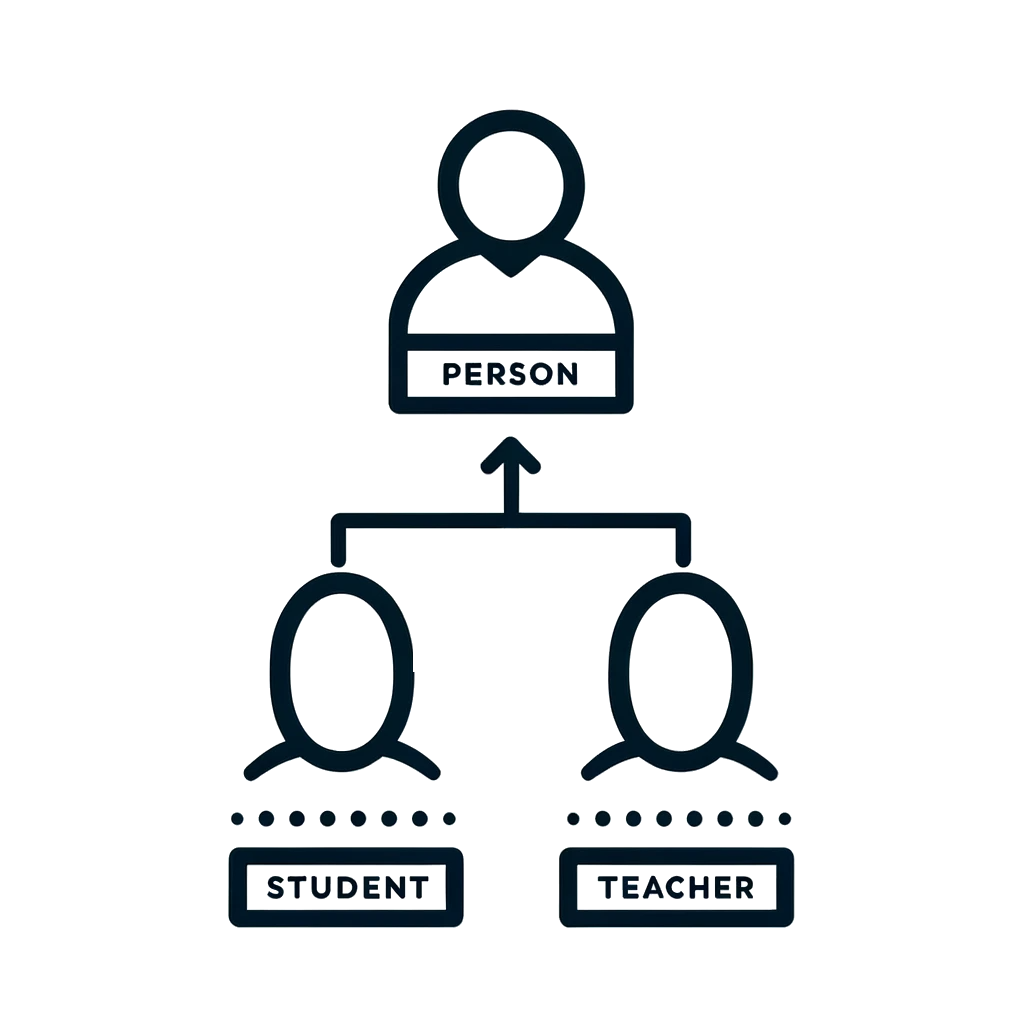
Syntax of inheritance
Derived class is declared using a class declaration that specifies the base class from which it inherits. This is done using a colon followed by the access specifier (public, protected, or private) and the base class name.
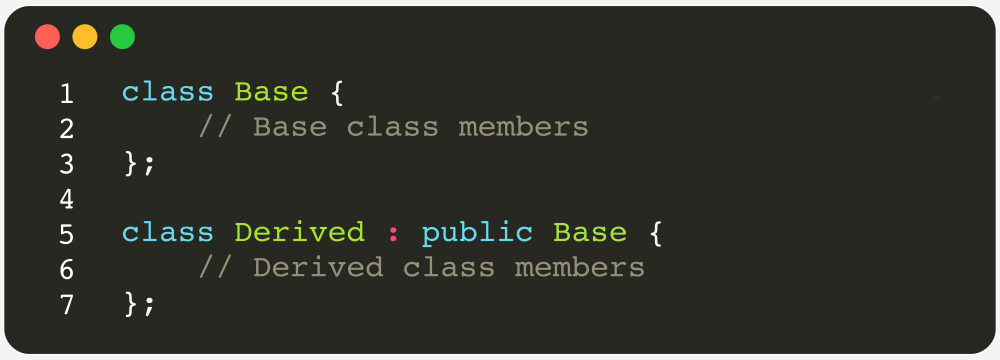
- Base Class (superclass): the class whose properties and functions are inherited. It is also known as the parent or superclass.
- Derived Class (subclass): the class that inherits from the parent. It is also known as the child or subclass.
- Access Specifier: this specifier determines how the members of the base class are inherited by the derived class.
- Inheritance: the colon (:) followed by the accessSpecifier and BaseClass indicates that DerivedClass is inheriting from BaseClass.
Note
The role and impact of access specifiers in inheritance will be covered in a later section of this chapter
Types of Inheritance
There are multiple times of inheritance. Each type offers a unique way of establishing relationships between classes, thereby providing a foundation for effective object-oriented design. Here are the main types of inheritance:
Single Inheritance | Involves one base class and one derived class. |
Multiple Inheritance | A derived class inherits from more than one base class. |
Multilevel Inheritance | Involves a chain of classes where a class is derived from another derived class. |
Hierarchical Inheritance | Multiple classes are derived from a single base class. |
Hybrid Inheritance | A combination of two or more types of inheritance. |
What is inheritance in object-oriented programming?
Select the correct answer
How is a derived class declared
Select the correct answer
Which classes is suitable to reuse code from the Person class?
Select a few correct answers
Everything was clear?