Cursos relacionados
Ver Todos os CursosIniciante
Introduction to Python
Python is a high-level, interpreted, general-purpose programming language. Distinguished from languages such as HTML, CSS, and JavaScript, which are mainly utilized in web development, Python boasts versatility across multiple domains, including software development, data science, and back-end development. This course will guide you through Python's fundamental concepts, equipping you with the skills to create your own functions by the conclusion of the program.
Intermediário
Intermediate Python Techniques
In this course, you will learn advanced topics such as function arguments, iterators, generators, closures, and decorators. Dozens of practice exercises will help you grasp these topics.
Intermediário
In-Depth Python OOP
This course covers Object-Oriented Programming (OOP) in Python, starting with the basics and progressing to advanced concepts. You will implement a User model to gain practical experience and understanding of OOP features. By the end of the course, you will have a strong foundation in OOP and a deeper knowledge of Python's inner workings.
Understanding Data Classes in Python
Simplifying Data Handling with Python's Data Classes
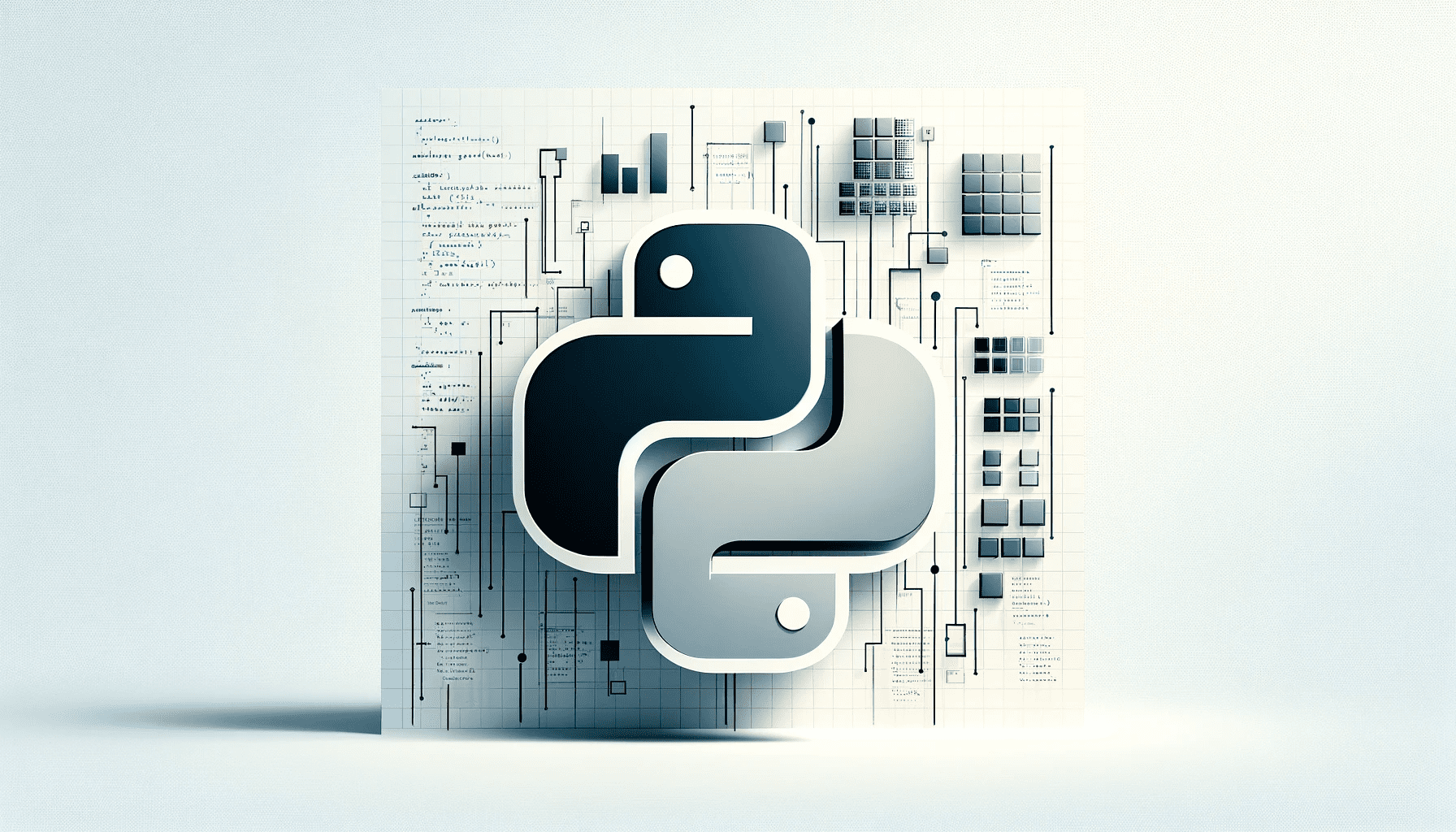
Data Classes in Python offer a streamlined way to handle data-rich objects. By reducing boilerplate code, they allow developers to focus more on functionality than on repetitive setup.
Introduction to Data Classes
Python is known for its simplicity and readability, and Data Classes, introduced in Python 3.7, further these characteristics. They are a way to automate common tasks associated with classes, making your code more maintainable and readable.
A Data Class in Python is a regular class but is geared towards storing state and data rather than containing a lot of logic. By using the @dataclass
decorator, Python automatically creates methods like __init__()
, __repr__()
, and __eq__()
based on the class attributes you define. This automation reduces the need for boilerplate code, which is especially beneficial in large projects where simplicity and readability are key.
Defining a Data Class
Creating a data class is straightforward. First, import the dataclass
decorator from the dataclasses
module. Then, use this decorator above your class definition. Inside the class, you define attributes with type annotations.
python
In this example, Point
is a data class with two attributes, x
and y
. Python automatically generates the __init__
and __repr__
methods.
Advantages of Using Data Classes
- Reduction in Boilerplate Code: The automatic generation of common methods like
__init__
and__repr__
means less code to write and maintain. - Immutability Support: You can make instances immutable, thus preventing them from being altered after creation, which is beneficial for maintaining data integrity.
- Type Hints Enhancement: By encouraging the use of type hints, data classes improve code quality, readability, and aid in debugging.
Common Methods in Data Classes
Python generates several dunder methods for data classes:
__init__
: Initializes the data class with given values.__repr__
: Provides a human-readable representation of the class instance.__eq__
: Compares two instances of the class for equality.
These methods can be overridden like in any other class for custom behavior.
Run Code from Your Browser - No Installation Required
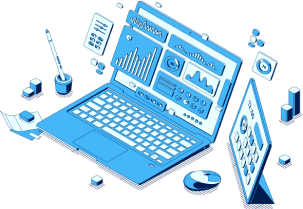
Customizing Data Classes
Data Classes can be extended with custom methods. This feature makes them versatile, as they are not just limited to storing data.
python
This Rectangle
class has a custom method area()
which calculates the area of the rectangle.
Default Factory Functions
The default_factory
attribute allows you to specify a function that returns the default value for a field.
python
Post-Initialization Processing
The __post_init__
method can be used for additional initialization and is called after the built-in __init__
method.
python
Compatibility with Typing
Data Classes work well with the typing
module, allowing for more complex types like List
, Dict
, and Optional
.
python
Inheritance in Data Classes
Data Classes support inheritance, allowing you to extend and customize base data classes.
python
Start Learning Coding today and boost your Career Potential
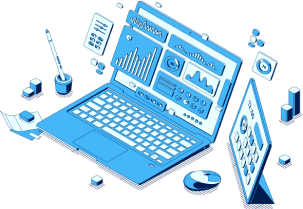
Best Practices
Avoid using Data Classes when:
- The class contains a lot of business logic.
- You need control over the data encapsulation and internal representation.
Speaking of using the Data Classes, the best practices are the following:
- Use type annotations for clarity.
- Utilize
default_factory
for mutable default values. - Override the
__repr__
method for meaningful output if the default is not sufficient.
Overall, Data Classes are best when you need a container for data attributes. Regular classes are more suitable for complex scenarios where control over behavior is necessary.
Integration with External Libraries
Data Classes can seamlessly integrate with serialization libraries, ORM frameworks, and more, making them versatile in various applications.
python
FAQs
Q: How does immutability work in data classes?
A: You can make a data class immutable by setting the frozen
parameter to True
. This prevents modification of instances after creation.
Q: Can data classes have private attributes?
A: Yes, you can define private attributes in data classes using the underscore naming convention (e.g., _private_attribute
).
Q: How do data classes handle optional attributes?
A: Optional attributes can be defined using typing.Optional
and setting a default value of None
.
Q: What are the limitations in inheritance with data classes?
A: While data classes support inheritance, default values in derived classes can override those in base classes, which requires careful design.
Q: Can data classes be nested within each other?
A: Yes, data classes can be nested, allowing for complex data structures that are still easy to manage.
Cursos relacionados
Ver Todos os CursosIniciante
Introduction to Python
Python is a high-level, interpreted, general-purpose programming language. Distinguished from languages such as HTML, CSS, and JavaScript, which are mainly utilized in web development, Python boasts versatility across multiple domains, including software development, data science, and back-end development. This course will guide you through Python's fundamental concepts, equipping you with the skills to create your own functions by the conclusion of the program.
Intermediário
Intermediate Python Techniques
In this course, you will learn advanced topics such as function arguments, iterators, generators, closures, and decorators. Dozens of practice exercises will help you grasp these topics.
Intermediário
In-Depth Python OOP
This course covers Object-Oriented Programming (OOP) in Python, starting with the basics and progressing to advanced concepts. You will implement a User model to gain practical experience and understanding of OOP features. By the end of the course, you will have a strong foundation in OOP and a deeper knowledge of Python's inner workings.
The SOLID Principles in Software Development
The SOLID Principles Overview
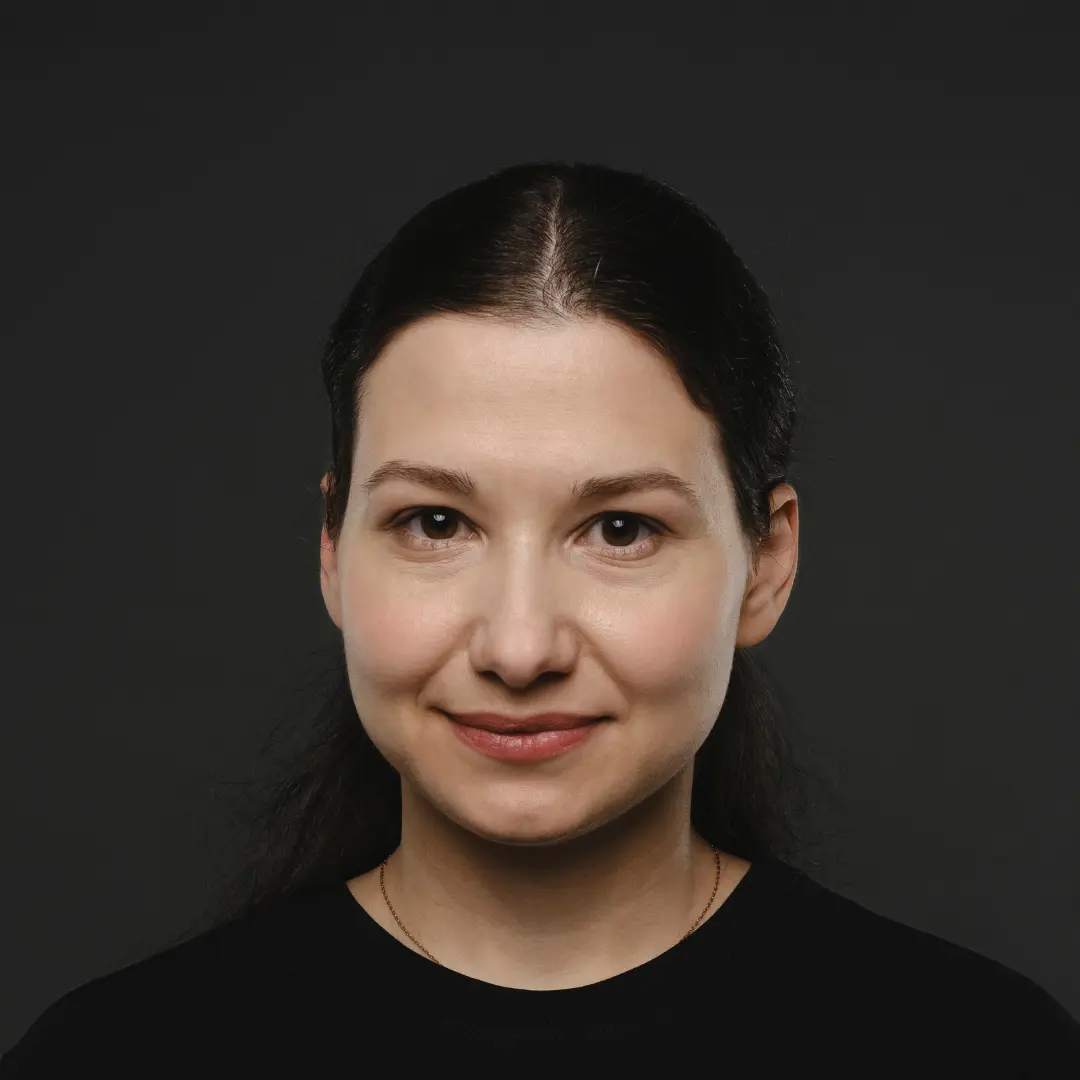
by Anastasiia Tsurkan
Backend Developer
Nov, 2023・8 min read
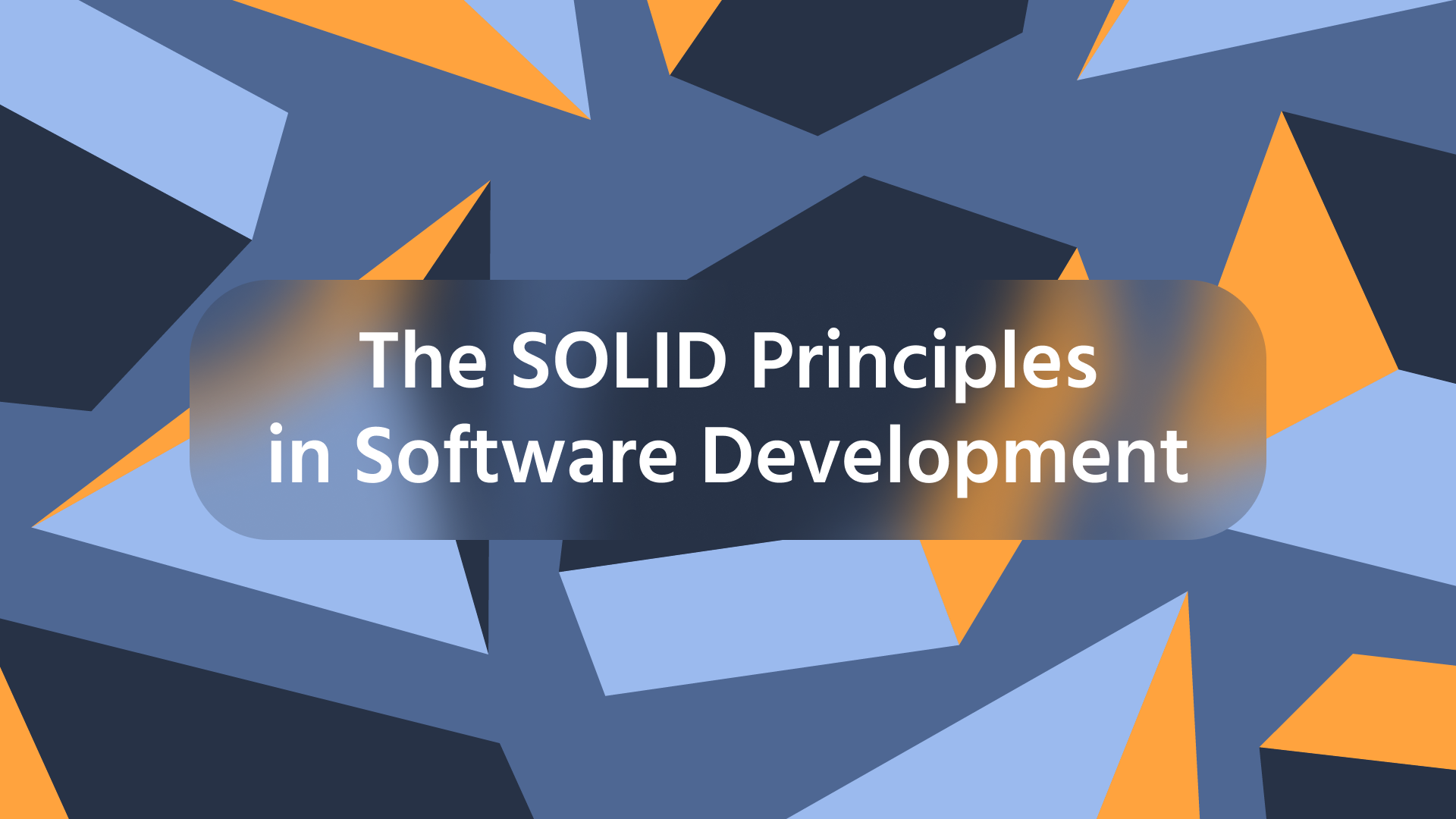
Match-case Operators in Python
Match-case Operators vs if-elif-else statements
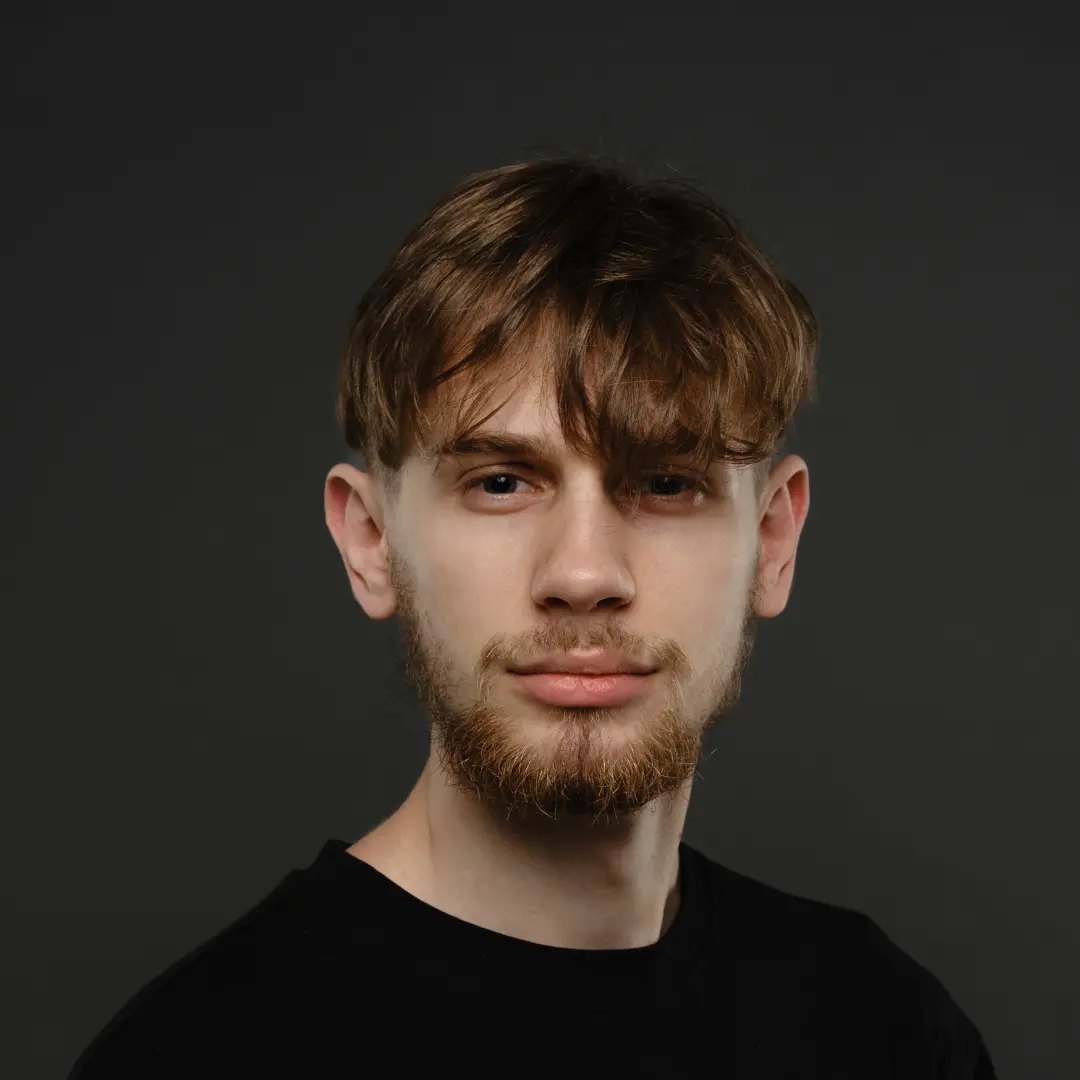
by Oleh Lohvyn
Backend Developer
Dec, 2023・6 min read
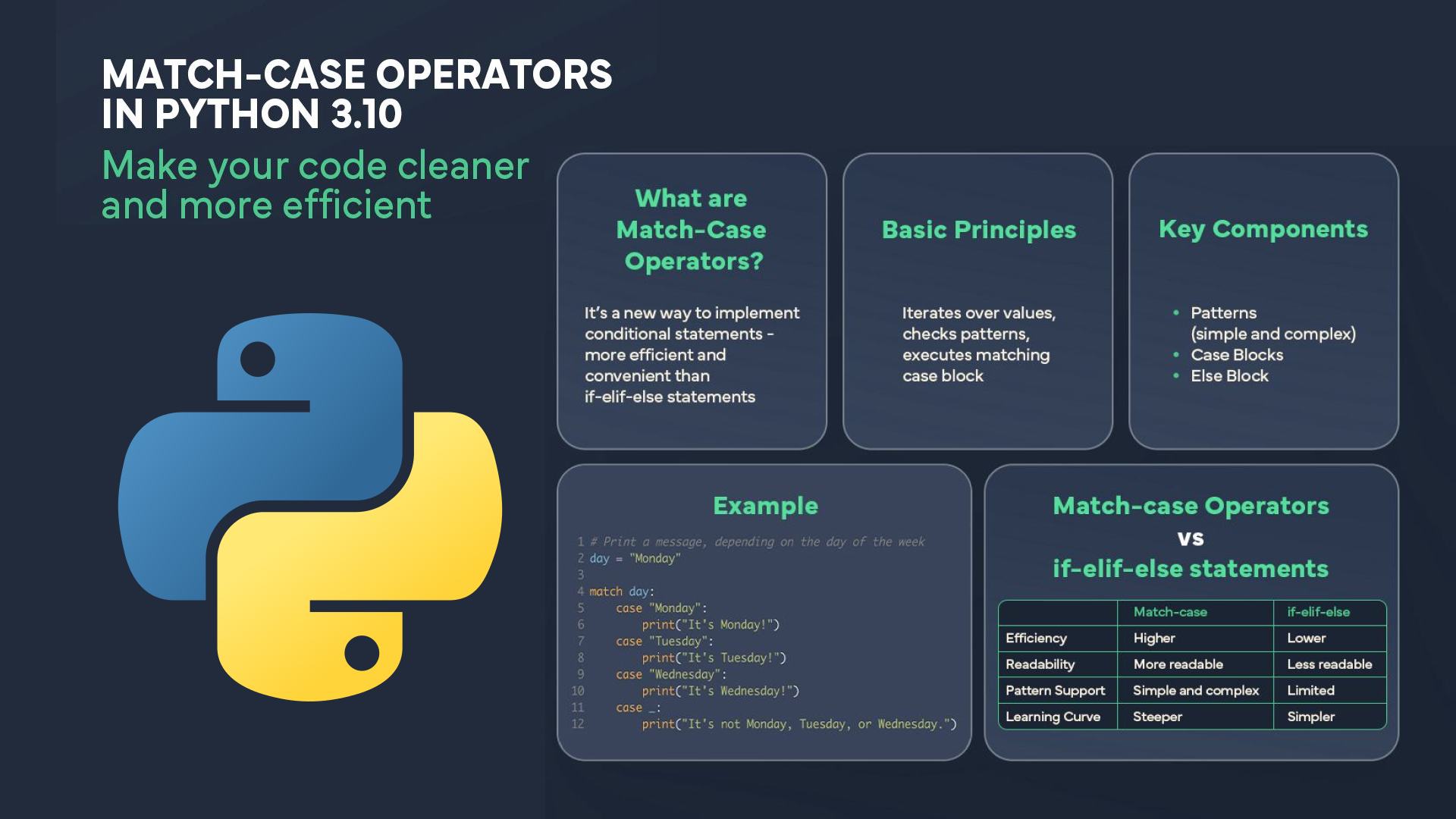
30 Python Project Ideas for Beginners
Python Project Ideas
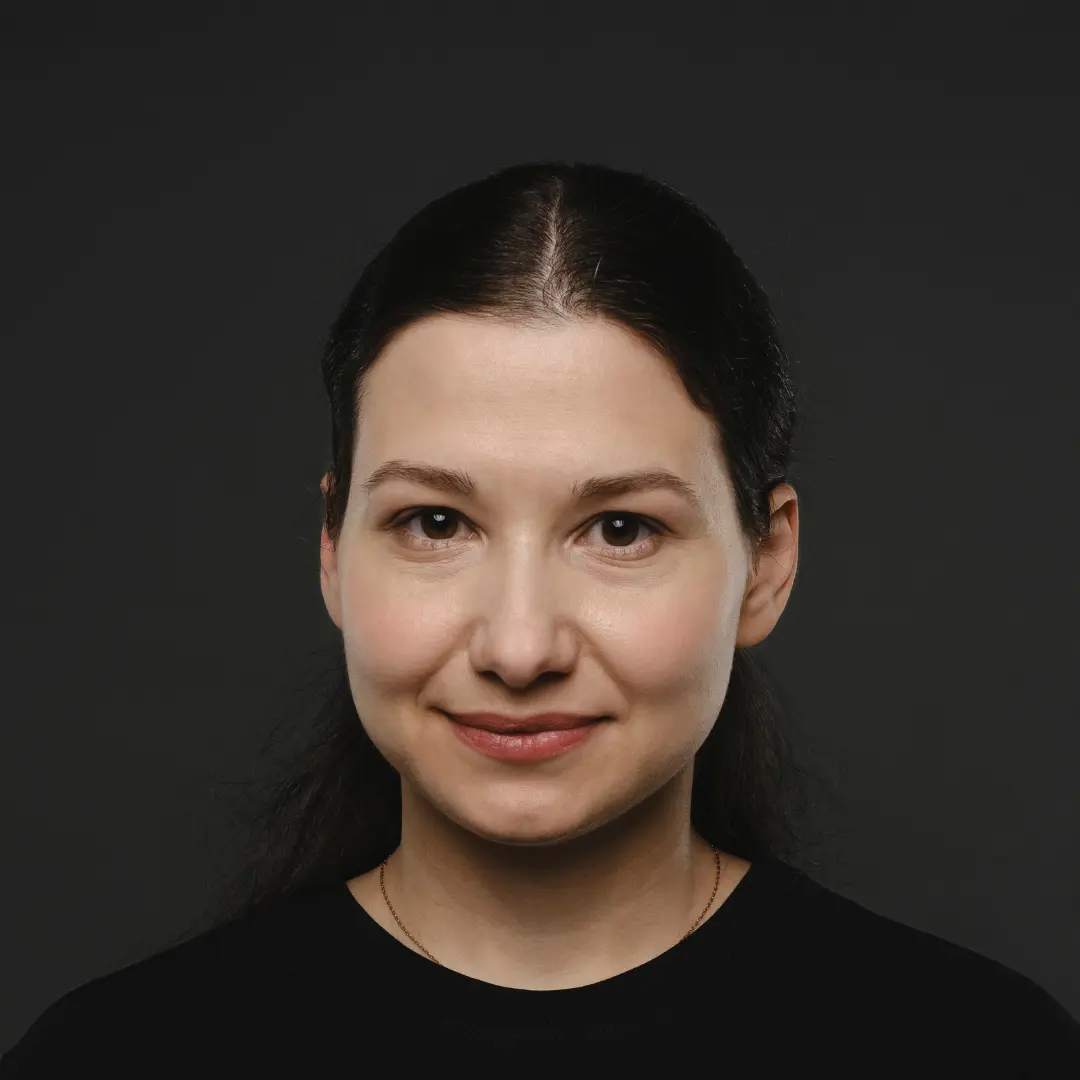
by Anastasiia Tsurkan
Backend Developer
Sep, 2024・14 min read
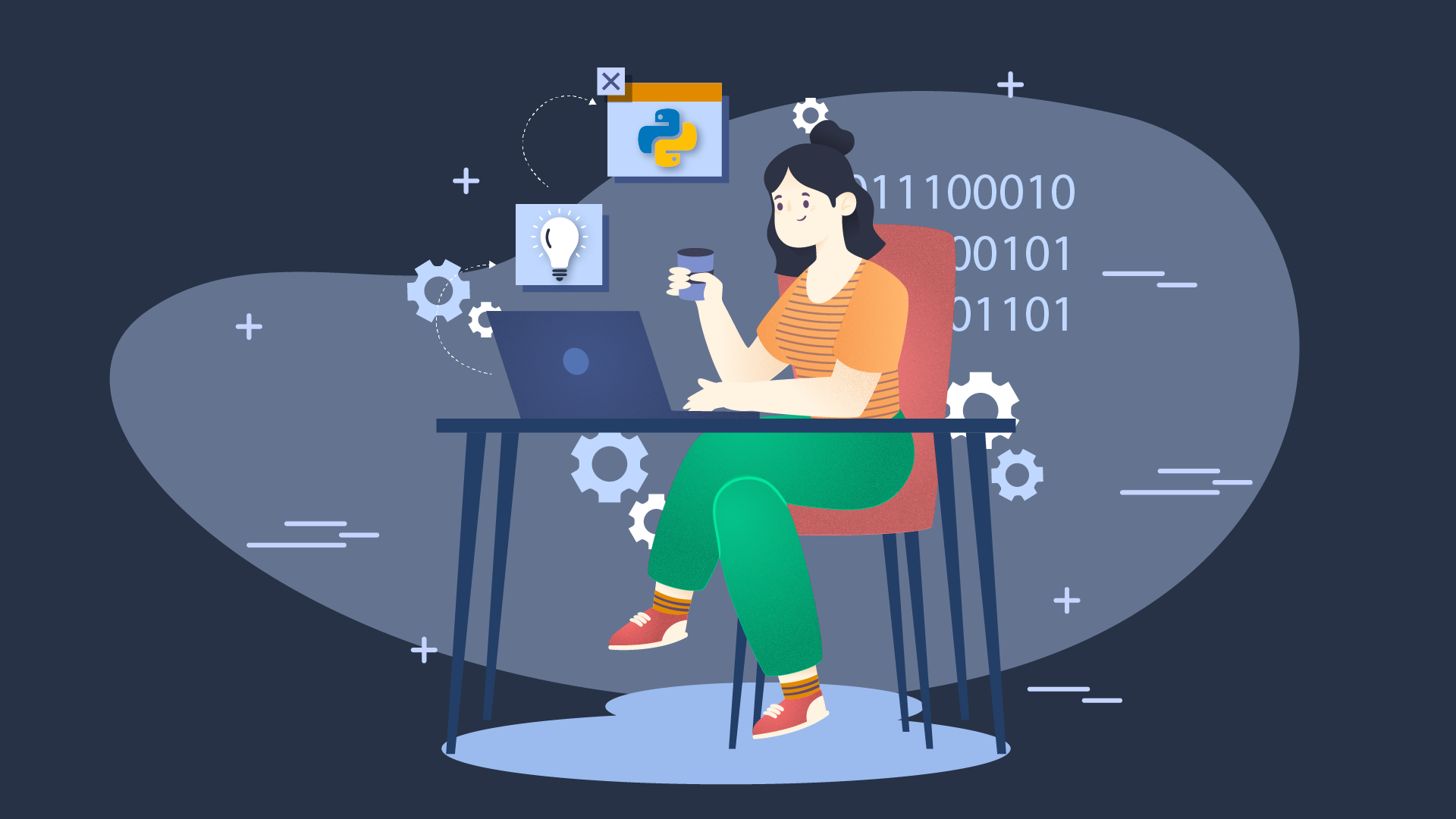
Conteúdo deste artigo