Cursos relacionados
Ver Todos os CursosIniciante
Introduction to Python
Python is a high-level, interpreted, general-purpose programming language. Distinguished from languages such as HTML, CSS, and JavaScript, which are mainly utilized in web development, Python boasts versatility across multiple domains, including software development, data science, and back-end development. This course will guide you through Python's fundamental concepts, equipping you with the skills to create your own functions by the conclusion of the program.
Intermediário
Intermediate Python Techniques
In this course, you will learn advanced topics such as function arguments, iterators, generators, closures, and decorators. Dozens of practice exercises will help you grasp these topics.
Understanding Garbage Collection in Python
Overview of Python Garbage Collection
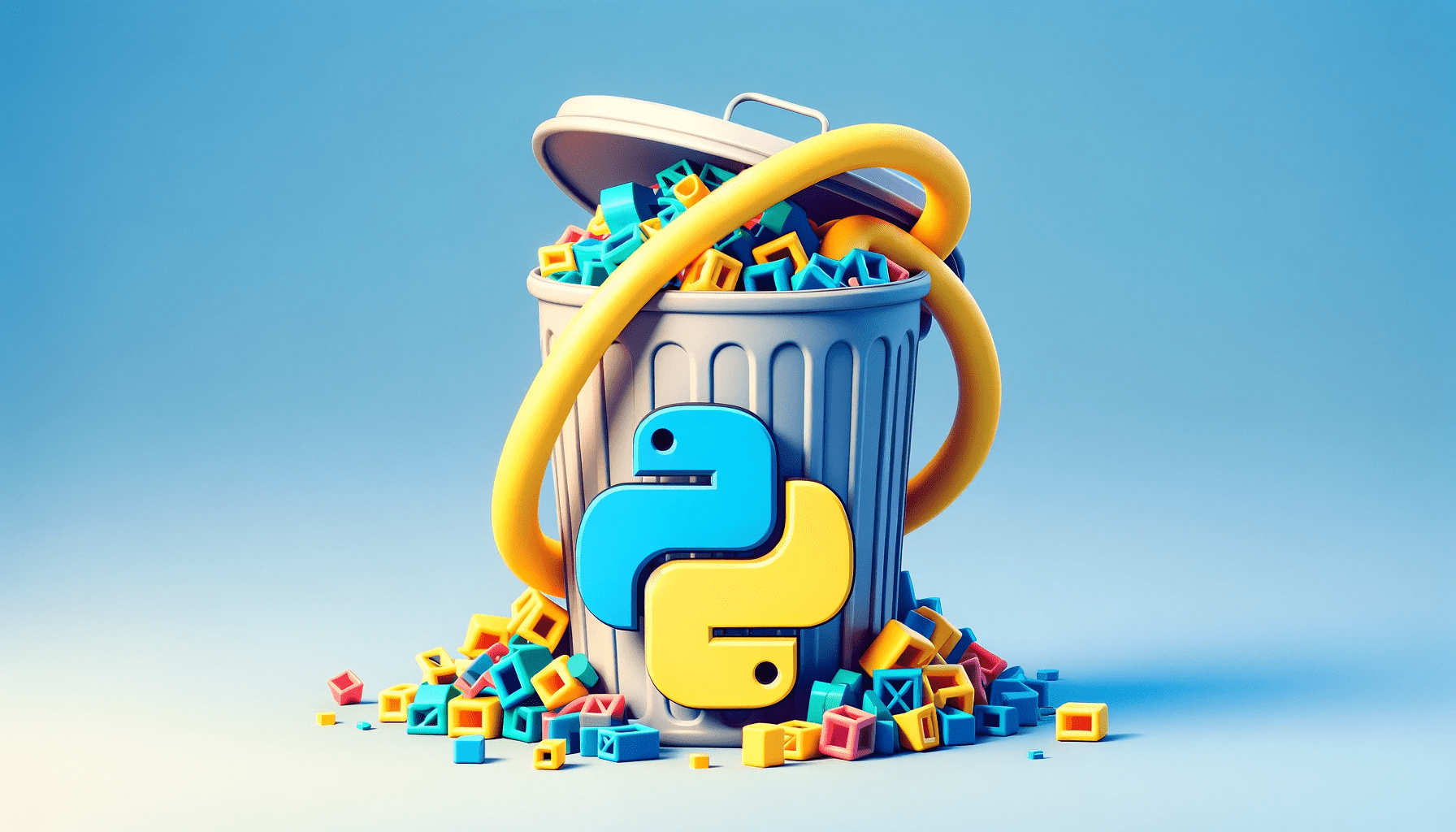
Garbage collection in Python is a critical process for automatic memory management. This article delves deeper into the concept, focusing particularly on the generational approach, and provides insights to help both beginners and seasoned programmers better understand and optimize their Python code.
Introduction to Garbage Collection
Key Points:
- Automated Process: Python manages memory allocation and deallocation without the need for manual intervention.
- Reference Counting: The primary method of garbage collection in Python.
- Generational Collection: A sophisticated approach to managing memory in Python.
Deep Dive into Generational Garbage Collection
Python employs a generational garbage collection system that categorizes objects into three generations, reflecting their lifespan and likelihood of being garbage collected:
-
Generation 0: This is the youngest generation. It contains newly created objects. Since many objects are short-lived, the garbage collector checks this generation more frequently than the others. Objects that survive the garbage collection process in generation 0 are promoted to generation 1.
-
Generation 1: This generation holds objects that have survived one round of garbage collection. In other words, these objects have lived longer than those in generation 0 but are not as old as those in generation 2. The garbage collector scans this generation less frequently than generation 0. Objects that are not collected and are still alive after a garbage collection pass are then promoted to generation 2.
-
Generation 2: This is the oldest generation and contains long-lived objects. The garbage collector checks this generation even less frequently than generation 1. It’s assumed that if an object has survived several rounds of garbage collection, it might be needed for the entire lifetime of the program, so the cost of repeatedly checking these objects is saved.
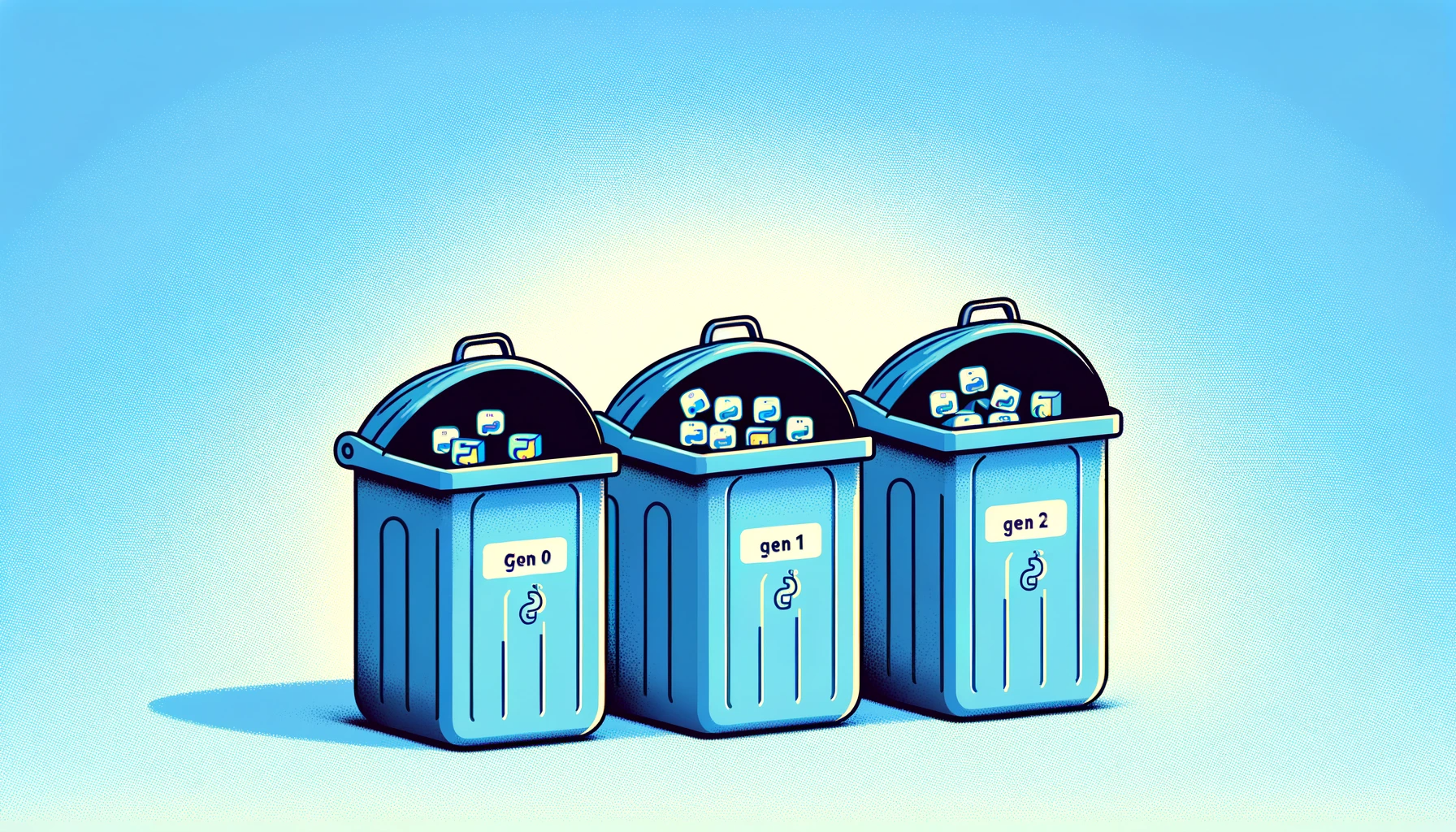
Run Code from Your Browser - No Installation Required
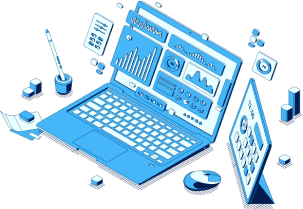
How Generations Work
- Allocation and Promotion: Objects are initially allocated to Generation 0. If they survive a garbage collection cycle, they are promoted to the next generation.
- Thresholds and Collection: Each generation has a threshold that determines when it will be collected. When the number of allocations minus the number of deallocations exceeds this threshold, a garbage collection cycle begins for that generation.
Benefits of the Generational Approach
The generational approach in garbage collection, particularly as implemented in Python, offers several benefits that enhance both the efficiency and effectiveness of memory management:
-
Increased Efficiency in Memory Reclamation: By focusing more on younger generations, where most objects are short-lived, the generational approach allows for more frequent and efficient reclaiming of memory. This is because younger generations are usually smaller and contain a higher percentage of garbage, making it quicker to scan and clean.
-
Improved Performance: Since older objects are collected less frequently, the time spent on garbage collection is reduced, especially for larger and older generations. This leads to improved overall performance, as the garbage collector is less likely to interrupt program execution.
-
Optimized Resource Utilization: The generational strategy helps in reducing the overhead of memory management. By categorizing objects based on their age and allocating resources accordingly, it ensures that the garbage collection process is more targeted and less resource-intensive.
-
Minimization of Memory Fragmentation: This approach can also help in reducing memory fragmentation. Since older objects are moved less frequently and younger objects are more likely to be quickly collected, it maintains a cleaner and more organized memory structure.
-
Reduced Risk of Premature Collection: In systems without generational garbage collection, there's a risk of prematurely collecting objects that are still in use. The generational approach minimizes this risk by keeping newer objects separate and focusing more on objects that have been around long enough to be likely unused.
-
Adaptive Thresholds: Python's garbage collector adapts the thresholds for triggering collection based on the program's behavior. This adaptiveness helps in balancing the need for timely garbage collection against the need to minimize the impact on program performance.
-
Handling of Long-Lived Objects: By moving long-lived objects into an older generation, the system recognizes that these objects are less likely to be garbage, thereby avoiding unnecessary checks and freeing up resources to focus on more probable areas of memory waste.
-
Circular Reference Detection: The generational approach, combined with Python's reference counting, effectively handles circular references. Objects involved in circular references may not be freed through reference counting alone, but the generational garbage collector can identify and clear these circular dependencies.
-
Better for Real-Time Applications: While Python is not typically used for real-time applications, the principles of generational garbage collection are advantageous in such contexts, as they allow for more predictable and less disruptive garbage collection cycles.
Best Practices for Memory Management
Implementing best practices can lead to more efficient memory usage in your Python programs.
Tips:
- Avoid Circular References: They can hinder the garbage collection process.
- Utilize Context Managers: For example, the
with
statement for resource management. - Regularly Monitor Memory: Tools like
tracemalloc
can identify potential issues early.
Start Learning Coding today and boost your Career Potential
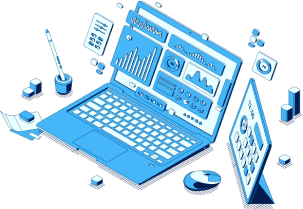
Advanced Topics in Garbage Collection
- Customizing Garbage Collection: Python's
gc
module allows developers to interact with the garbage collector. - Forced Garbage Collection: Manually triggering garbage collection in specific scenarios.
FAQs
Q: How often does Python perform garbage collection?
A: Python periodically checks the number of allocations and deallocations. When a certain threshold is crossed, a garbage collection cycle for a particular generation is triggered.
Q: Can we modify the garbage collection process?
A: Yes, Python's gc
module allows you to customize various aspects of the garbage collection process, including disabling it, though this is generally not recommended.
Q: How does garbage collection impact Python's performance?
A: While necessary, garbage collection can impact performance. Optimizing the creation and lifetime of objects can minimize this impact.
Q: Is manual memory management required in Python?
A: Python handles most memory management tasks automatically, but understanding how it works can help you write more efficient code.
Q: How can I diagnose memory leaks in Python?
A: Python provides tools like tracemalloc
to track memory allocation. Regularly monitoring your application can help detect and diagnose memory leaks.
Q: What are the differences in the thresholds for each generation?
A: Thresholds for each generation are typically set higher as the generation number increases, as older generations are expected to have less frequent, but larger, collections.
Q: Can objects skip generations?
A: Generally, objects are promoted to the next generation only after surviving a collection. However, certain large objects might be allocated directly to an older generation to optimize performance.
Cursos relacionados
Ver Todos os CursosIniciante
Introduction to Python
Python is a high-level, interpreted, general-purpose programming language. Distinguished from languages such as HTML, CSS, and JavaScript, which are mainly utilized in web development, Python boasts versatility across multiple domains, including software development, data science, and back-end development. This course will guide you through Python's fundamental concepts, equipping you with the skills to create your own functions by the conclusion of the program.
Intermediário
Intermediate Python Techniques
In this course, you will learn advanced topics such as function arguments, iterators, generators, closures, and decorators. Dozens of practice exercises will help you grasp these topics.
The SOLID Principles in Software Development
The SOLID Principles Overview
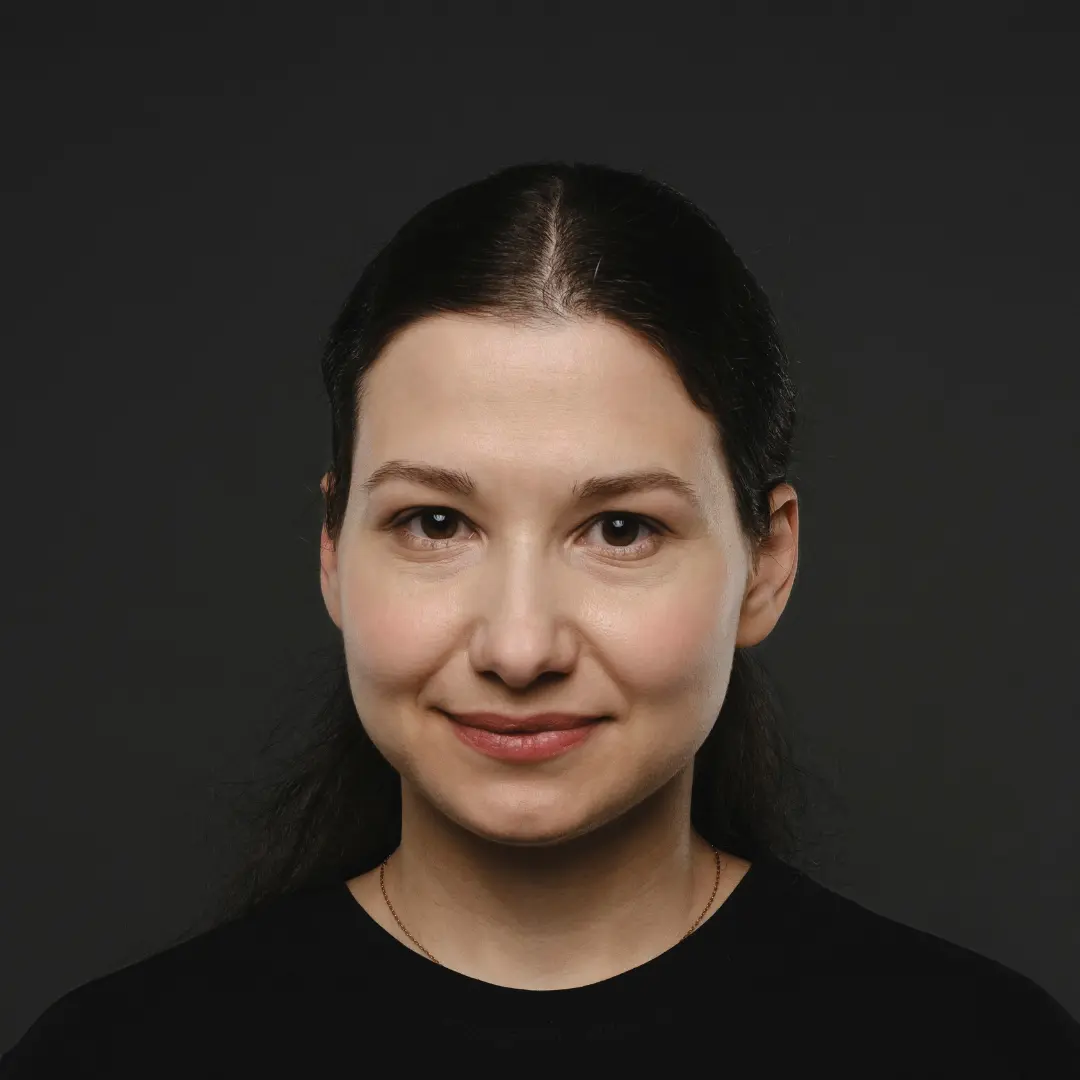
by Anastasiia Tsurkan
Backend Developer
Nov, 2023・8 min read
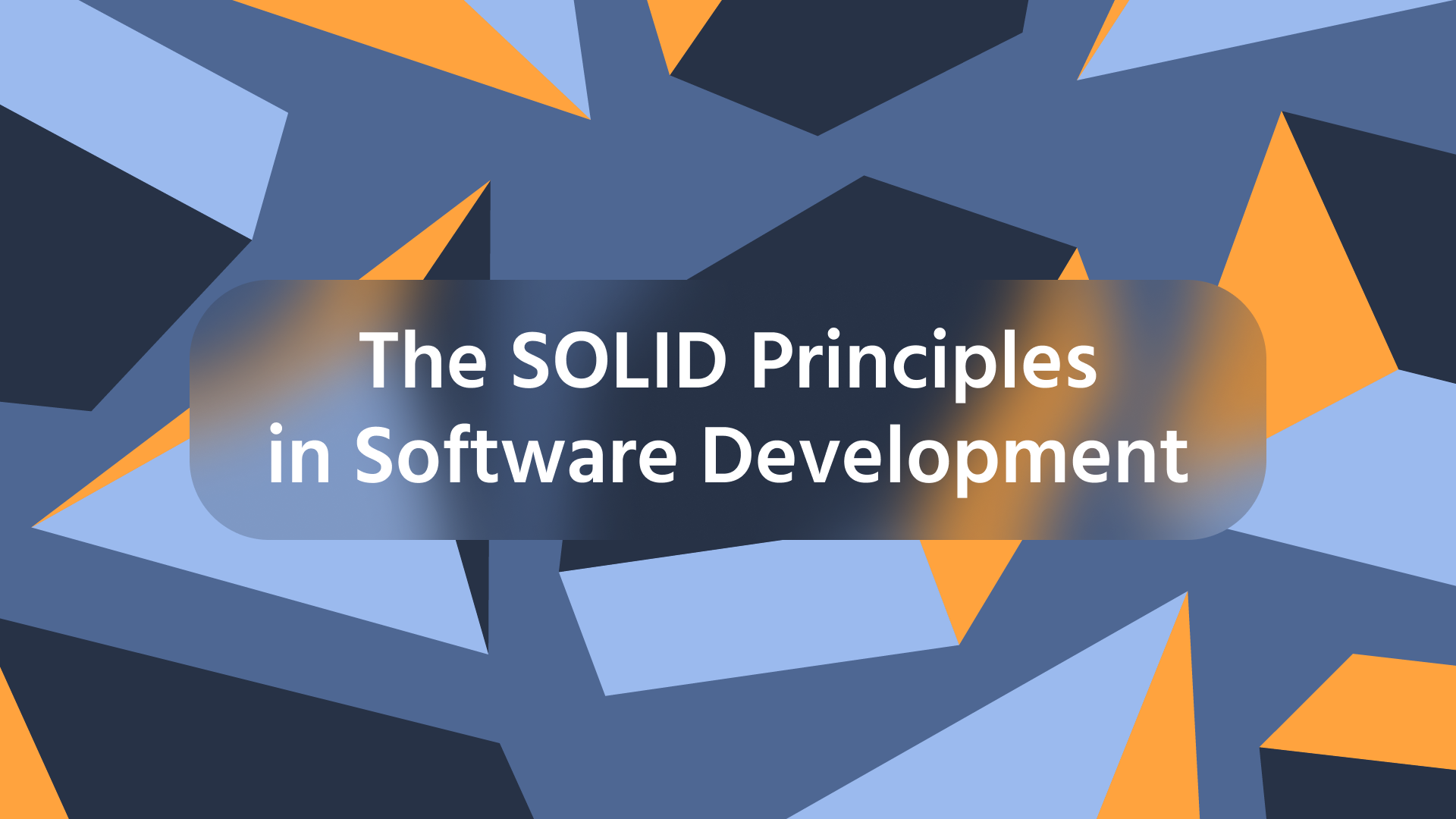
The Facade Pattern Explained
Simplify Complex Systems with a Unified Interface
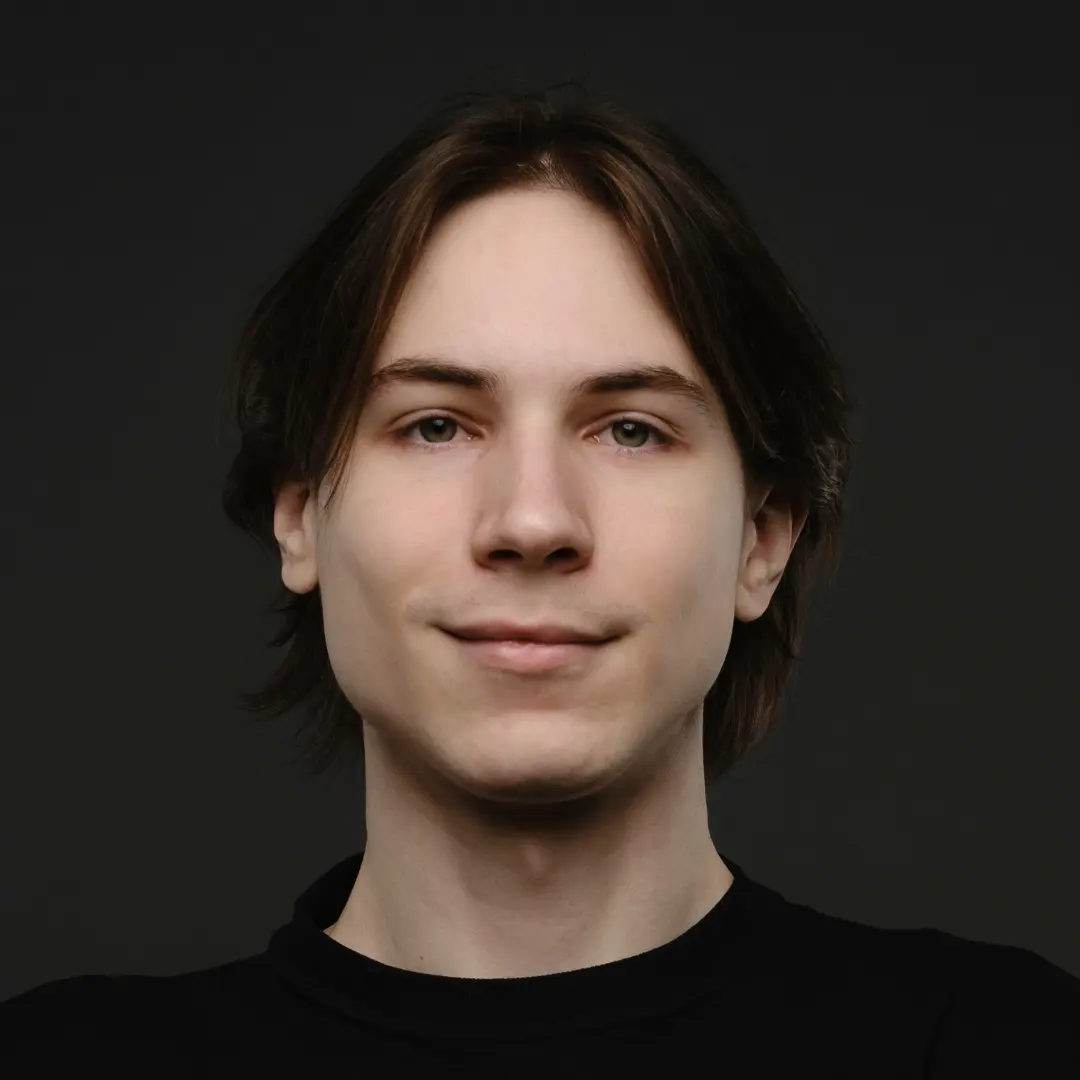
by Ihor Gudzyk
C++ Developer
May, 2025・6 min read
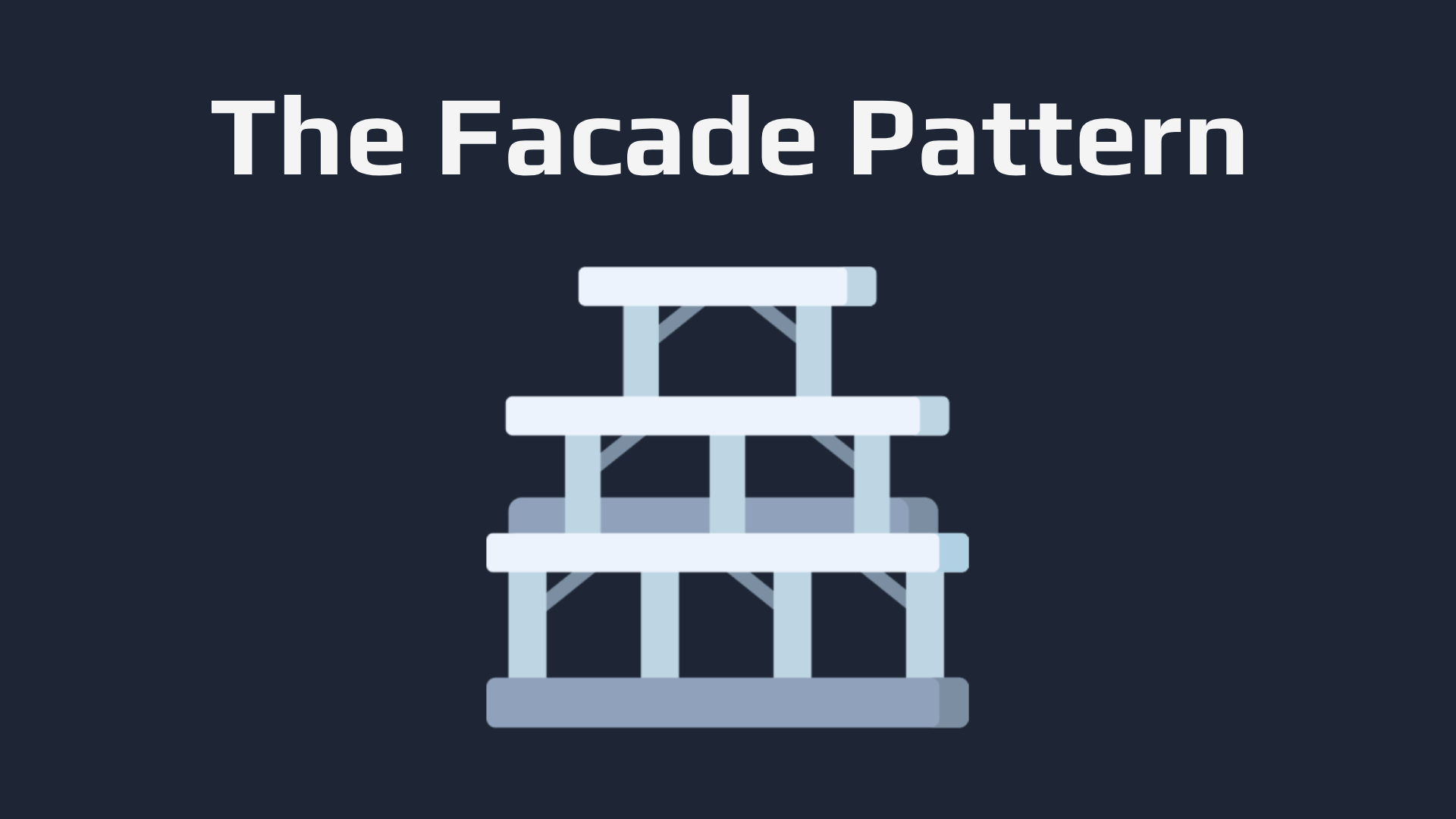
30 Python Project Ideas for Beginners
Python Project Ideas
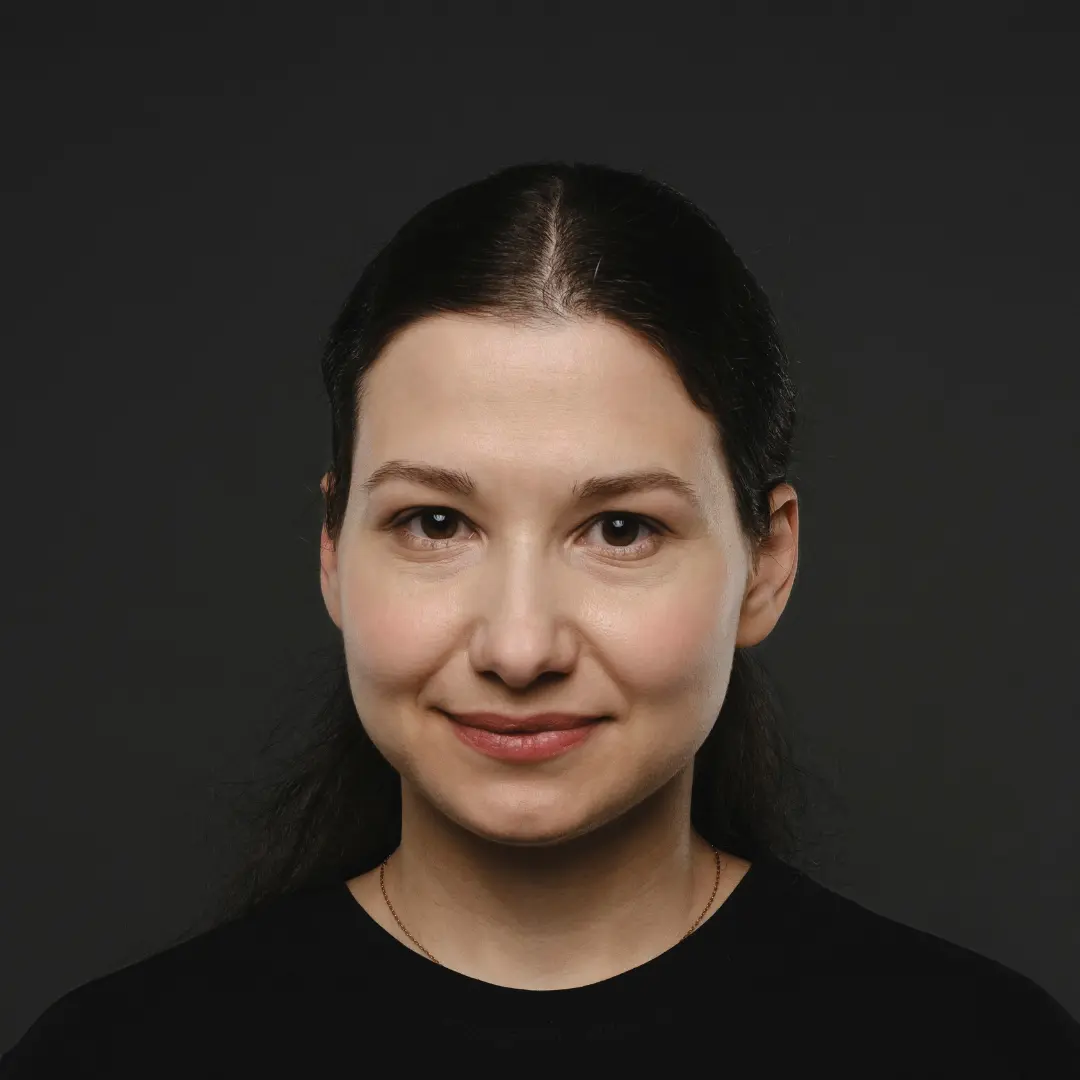
by Anastasiia Tsurkan
Backend Developer
Sep, 2024・14 min read
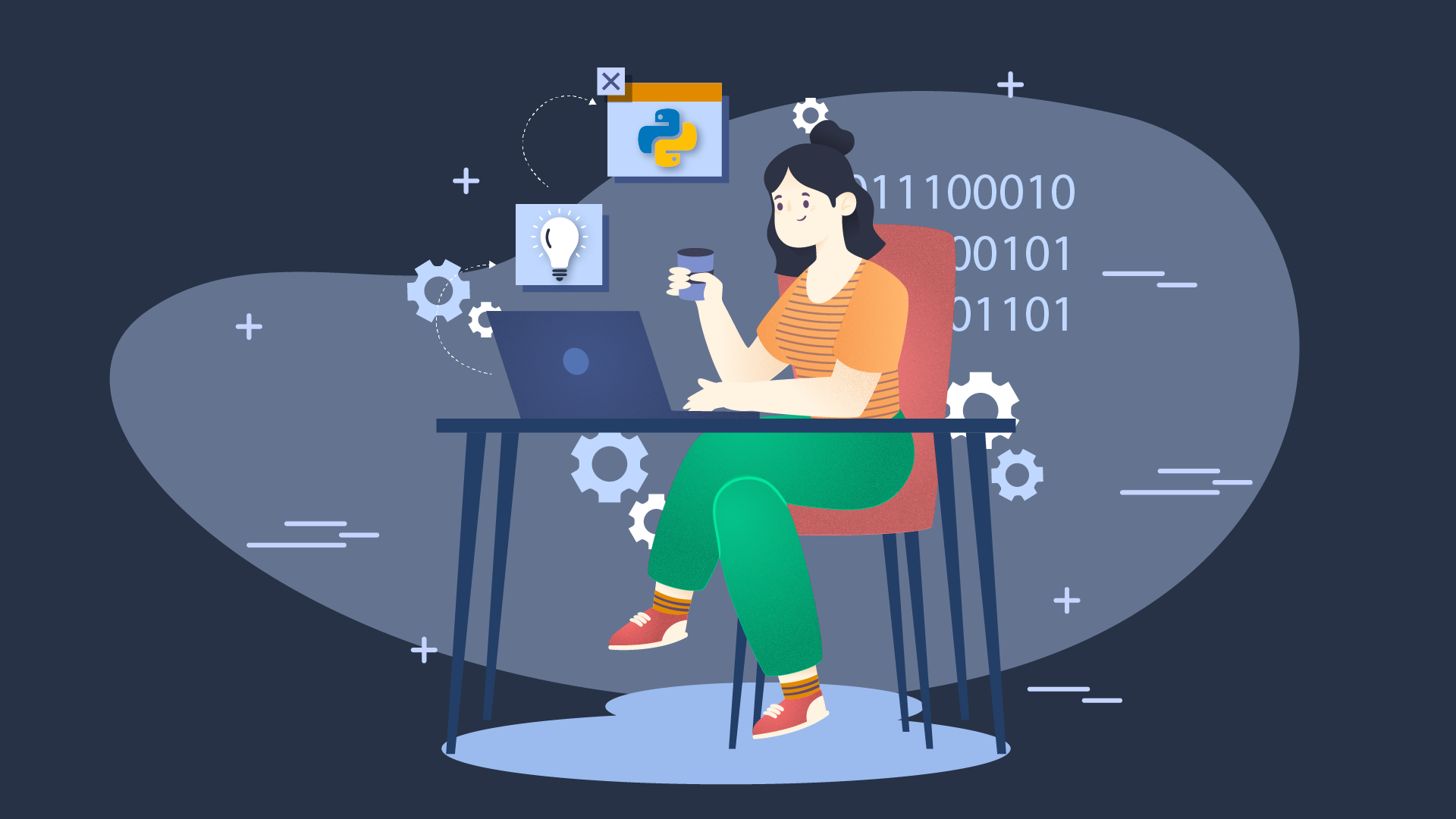
Conteúdo deste artigo