Golang 10 Best Practices
Golang Coding Like a Professional
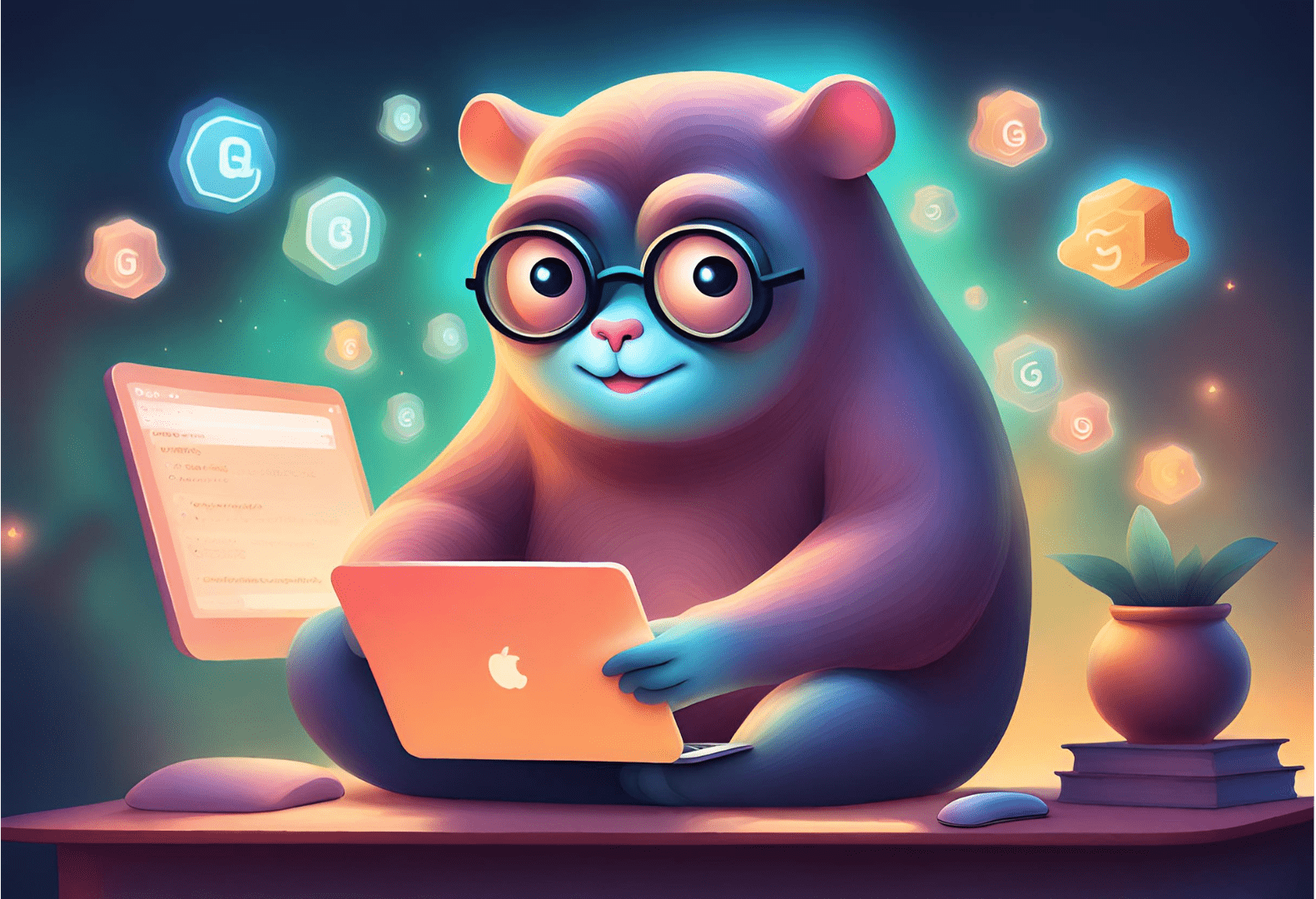
Golang, commonly referred to as Go, is a modern programming language developed by Google, known for its simplicity, efficiency, and robustness. Since its inception, Go has gained significant popularity for its ability to handle large-scale distributed systems, making it an ideal choice for cloud-based applications, web servers, and microservices architecture. It's also used in data processing pipelines, networking tools, and even in the development of command-line utilities. Go’s growing popularity is largely due to its powerful concurrency model, straightforward syntax, and a strong standard library.
In the rapidly evolving landscape of software development, adhering to golang best practices is crucial. These practices provide a framework for writing code that is not only functional but also optimized for performance, easier to maintain, and more secure. By following these guidelines, developers can:
- Maximize Performance ⚡: Efficiently utilize Go's capabilities for high-speed execution.
- Enhance Maintainability 🛠️: Ensure code is clean, readable, and easy to update or scale.
- Improve Reliability ✅: Reduce the likelihood of errors and bugs in the codebase.
- Boost Security 🔒: Adhere to secure coding standards to prevent vulnerabilities.
1. Efficient Use of Goroutines
Goroutines are a fundamental aspect of Go, offering a lightweight and efficient means of handling concurrency. Unlike threads in other languages, goroutines are managed by the Go runtime, not the operating system. This design makes them extremely lightweight and scalable. However, understanding how to use them efficiently is key to harnessing their full potential.
Best practices for starting and managing Goroutines involve careful consideration of their lifecycle and communication. It's crucial to avoid spawning excessive goroutines, as this can lead to resource exhaustion. Instead, developers should focus on the task's nature and determine whether it benefits from parallel execution. Proper synchronization mechanisms, such as channels or wait groups, are essential to manage goroutines effectively, ensuring that resources are not wasted and data races are avoided.
Common pitfalls with goroutines include memory leaks, race conditions, and deadlocks. These can be mitigated by understanding the nature of concurrency in Go and using tools like the race detector during development. Careful design of goroutine interaction with other parts of the program is vital to prevent these issues, making it essential to comprehend the nuances of goroutine lifecycles and intercommunication.
2. Proper Error Handling
Error handling in Go is uniquely straightforward yet powerful. In Go, errors are treated as values, and functions that can fail typically return an error as their last return value. This approach forces developers to explicitly handle errors where they occur, leading to more reliable and predictable code.
Robust error handling patterns in Go involve checking for errors and handling them gracefully. This can include logging the error, retrying the operation, or even failing fast, depending on the context and severity of the error. It's important to avoid ignoring errors, as this can lead to more complex issues down the line. Additionally, wrapping errors with contextual information can greatly aid in debugging and maintenance.
Examples of good error handling include checking for errors after each operation that can produce them, and handling them appropriately. Bad error handling, conversely, might involve ignoring errors or panicking unnecessarily. A well-handled error in Go not only prevents the program from failing unexpectedly but also provides meaningful insight into what went wrong, aiding in quick resolution and robustness of the application.
Good Error Handling Example
go
☑️ Error Check: The function checks for the division by zero error and returns an error if it occurs.
☑️ Error Handling: In main()
, the error is checked and logged, providing feedback to the user, while the program fails gracefully.
Bad Error Handling Example
go
☑️ No Error Check: The function does not check for division by zero, leading to a potential runtime panic or unexpected results.
☑️ No Error Handling: The error condition is ignored, which could cause the program to fail in unpredictable ways.
3. Effective Use of Interfaces
Understanding Go interfaces is crucial for writing flexible and maintainable Go code. In Go, interfaces are types that specify sets of methods, but unlike in many other languages, they are implicitly implemented. This means a type implements an interface simply by implementing its methods, without any explicit declaration. This feature promotes a design philosophy known as "composition over inheritance," leading to more modular and decoupled code.
Designing clean and reusable interfaces in Go involves focusing on simplicity and relevance. Interfaces should be small and focused, typically defining only one or two methods. This practice, often called the "interface segregation principle," ensures that implementations are not burdened with methods they don't need. Additionally, by designing interfaces that are narrowly defined and closely aligned with specific behaviors, you encourage code reuse and improve the clarity of your codebase.
Examples of interface misuse include overgeneralizing an interface or defining an interface with too many methods. Overgeneralized interfaces can lead to weakly defined roles for types, making the code harder to understand and maintain. An interface with too many methods can lead to bloated implementations, where types must implement methods they don't need, violating the principle of minimalism in interface design.
Good Practice Example:
go
In this example, the Writer
interface is small and focused, defining only a single method. This allows for flexible implementations, like the FileWriter
struct, which adheres to the interface without unnecessary overhead. The LogToFile
function can accept any type that implements the Writer
interface, promoting code reuse and flexibility.
Bad Practice Example:
go
In this case, the Device
interface is overgeneralized, containing multiple methods that may not all be relevant for every implementation. The Printer
struct, for example, must implement all methods, even though it may only be relevant for the Start
, Stop
, and Write
methods. This leads to bloated and less maintainable code, as the implementation is forced to include methods that are unnecessary or irrelevant.
- Small, Focused Interfaces 😊: As shown in the good example, interfaces should be small and specific, focusing on one or two methods to maintain simplicity and relevance.
- Avoid Overgeneralization ⚠️: The bad example highlights the pitfalls of defining interfaces with too many methods, leading to complex and difficult-to-maintain code.
- Encourage Flexibility and Reuse 🔄: Well-designed interfaces promote code reuse and flexibility, as different types can implement them with minimal overhead.
Run Code from Your Browser - No Installation Required
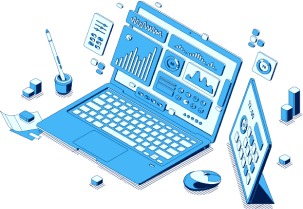
4. Memory Management
Understanding the basics of memory management in Go is essential for writing efficient programs. Go uses an automatic garbage collection (GC) system, which simplifies memory management and eliminates the need for manual memory deallocation as in languages like C and C++. However, this doesn't mean a Go programmer can ignore memory management; understanding how memory allocation works in Go is key to writing efficient, memory-safe programs.
Tips for optimizing memory usage in Go include understanding how and when memory allocation occurs and how to control it. For instance, being mindful of how variables are passed — by value or by reference — can significantly affect memory usage. Avoiding unnecessary allocations, such as reusing buffers instead of creating new ones, and being aware of the memory implications of certain data structures, like slices and maps, are also crucial.
Tools for memory profiling in Go, such as pprof, are indispensable for identifying memory-related issues. These tools can help pinpoint where in your code memory is being allocated and can assist in tracking down memory leaks. Regular use of profiling in development and testing phases can lead to more efficient memory usage, reducing the overhead of garbage collection and improving the overall performance of your applications. By continually analyzing and refining the memory usage of your Go programs, you can ensure they are both efficient and robust.
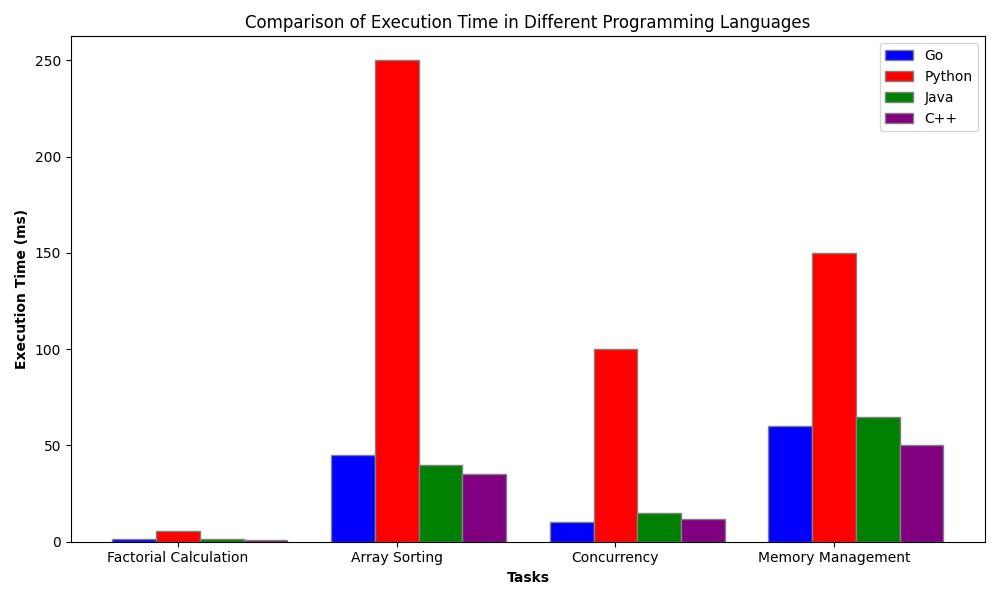
Go provides high memory management efficiency, outperforming Python and Java due to its optimized garbage collection (GC) system, making it an excellent choice for performance-critical applications. However, C++ remains the most efficient in this area, thanks to manual memory management, which offers maximum control and performance.
5. Concurrency Patterns
Go's concurrency model is one of its most distinctive features, built around goroutines and channels. This model is based on the concept of "communicating sequential processes" (CSP). Goroutines are lightweight threads managed by the Go runtime, and channels are the conduits through which goroutines communicate, synchronizing execution and sharing data. This model allows developers to write concurrent code that is easier to understand and maintain compared to traditional thread-based concurrency models.
Implementing common concurrency patterns in Go often revolves around the effective use of goroutines and channels. For instance, the 'worker pool' pattern, where a number of workers execute tasks concurrently, can be efficiently implemented using goroutines. Channels are instrumental in distributing tasks and collecting results. Another common pattern is the 'producer-consumer', where one or more goroutines produce data and others consume it, with channels facilitating the data flow.
Avoiding race conditions and deadlocks is crucial in concurrent programming. Race conditions, where multiple goroutines access shared resources without proper synchronization, can lead to unpredictable results. Go's race detector tool is invaluable in identifying such issues. Deadlocks, on the other hand, occur when goroutines are waiting on each other, leading to a standstill. Careful design of goroutine interactions and channel usage is essential to avoid these issues. Ensuring that all channels are properly closed and avoiding unbuffered channels when not necessary are good practices in this regard.
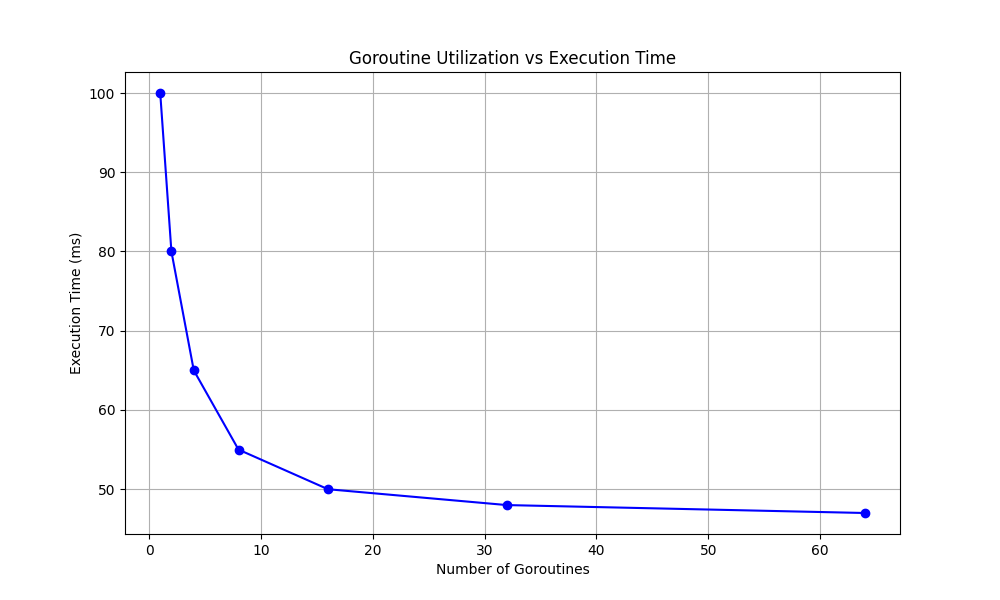
This graph illustrates how the number of goroutines affects task execution time in Go programming. As the number of goroutines increases, execution time gradually decreases, highlighting the efficiency of concurrent task execution in Go. However, beyond a certain point, the gains in efficiency diminish, indicating an optimal number of goroutines for the task. This underscores the importance of balancing the number of goroutines with system resources to achieve maximum performance.
6. Code Formatting and Style
The importance of code readability in Go cannot be overstated. Readable code is not just easier to understand and maintain; it's also more accessible to other developers, which is vital in collaborative environments. Go emphasizes simplicity and clarity in its design, and this extends to how Go code should be written and formatted.
Go provides several tools to help maintain a consistent code style, with gofmt being the most prominent. gofmt
automatically formats Go code according to the standard style guidelines, ensuring that Go codebases have a consistent look and feel. This eliminates debates over formatting styles in teams and keeps the focus on the code's functionality.
Community-accepted styling conventions in Go extend beyond formatting to include naming, program structure, and idiomatic patterns. For instance, idiomatic Go favors short, concise names, especially for local variables with limited scopes. The effective use of package structures, keeping them small and focused, is also encouraged. Additionally, the Go community values documentation highly, and tools like godoc
rely on comments being well-written and informative. Adhering to these conventions not only makes the code more readable but also ensures it aligns well with the broader Go ecosystem, making it easier for others to understand and contribute.
7. Dependency Management
Managing dependencies in Go projects is a critical aspect of modern software development. As Go applications grow in complexity, they often rely on various external libraries and packages. Effective management of these dependencies ensures that your project remains stable, maintainable, and easy to collaborate on.
Using Go modules effectively is central to handling dependencies. Introduced in Go 1.11, modules provide an official dependency management solution, allowing you to track and control the specific versions of external packages your project depends on. A Go module is defined by a go.mod
file at the root of your project, which lists the required packages along with their versions. Modules ensure reproducible builds by explicitly stating which dependencies and versions are needed, thus avoiding the "it works on my machine" problem.
Tips for versioning and package management include adhering to semantic versioning principles and being cautious with major version upgrades. Semantic versioning (or SemVer) is a versioning scheme that reflects the nature of changes in the version number. For instance, updating the minor version number for backwards-compatible changes, and the major version for breaking changes. It's essential to regularly update dependencies to incorporate bug fixes and security patches, but major version changes should be approached carefully, as they may introduce breaking changes. Regularly auditing your dependencies with tools like go list -m all
or go mod tidy
helps in maintaining a clean and up-to-date go.mod
file.
8. Testing and Benchmarking
Writing effective tests in Go is foundational to producing reliable and maintainable code. The Go standard library provides extensive support for testing, making it straightforward to write and execute tests. Go's testing philosophy encourages writing tests alongside your code, in _test.go
files. This approach promotes test-driven development (TDD) and makes it easy to maintain tests as the codebase evolves.
Benchmarking Go code is an important practice, especially for performance-critical applications. Go's built-in testing framework includes support for writing benchmark tests, which measure the performance of your code in terms of execution time. These benchmarks are invaluable for identifying performance bottlenecks and validating the impact of optimizations. They are defined similarly to unit tests but use the Benchmark
function prefix and are run using the go test -bench
command.
Tools and frameworks for testing in Go extend beyond the standard library. Tools like GoMock or Testify can be used for mocking and asserting, providing more sophisticated capabilities for testing complex scenarios. Additionally, coverage tools integrated into the Go toolchain, such as go test -cover
, help in identifying parts of the codebase that are not adequately tested, ensuring thorough test coverage across your project. By leveraging these tools and practices, Go developers can ensure that their code is not only functionally correct but also performs efficiently under various scenarios.
Start Learning Coding today and boost your Career Potential
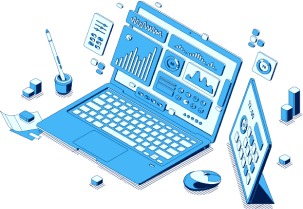
9. Documentation and Comments
Best practices for documenting Go code are integral to its understandability and maintainability. In Go, documentation is not just a good practice but a part of the language's culture. Go provides a built-in tool, godoc
, which generates documentation directly from the code. The convention in Go is to write documentation comments in a specific format, starting with the name of the element being described. This makes the documentation easy to read both in the source code and in the generated documentation web pages.
Writing meaningful comments involves explaining the 'why' behind the code, not just the 'what'. Comments should clarify the intent behind a block of code, any non-obvious reasons for why it's written a certain way, or describe the broader context. Avoid redundant comments that simply restate what the code is doing. Instead, focus on providing insights that are not immediately apparent from the code itself.
Tools for generating documentation, like 'godoc', play a significant role in Go's ecosystem. godoc
extracts comments from the code and presents them in a readable, web-based format. Developers should strive to write comments that are clear and informative to make the most of this tool, as it is often the first place others will look for understanding a codebase or library.
For further reading, check out the official Go documentation and popular community resources:
10. Performance Optimization
Techniques for profiling Go applications are vital for understanding and improving their performance. Profiling involves measuring the resource usage of your program (like CPU time, memory usage, and more) to identify bottlenecks. Go provides several built-in profiling tools, such as the pprof
package, that can be used to gather detailed performance data. This data is invaluable for understanding how your program behaves in a production-like environment and where optimizations will have the most impact.
Identifying and fixing performance bottlenecks often starts with CPU and memory profiling. CPU profiling helps in identifying functions that are taking up most of the CPU time. Memory profiling, on the other hand, helps in finding areas where memory is being used inefficiently. Once bottlenecks are identified, the focus shifts to optimizing these areas, which could involve algorithmic changes, more efficient data structures, or concurrency optimizations.
Case studies of performance optimization in Go can provide valuable insights. Many Go community blogs and forums share real-world stories of how performance issues were identified and resolved. These case studies can be a rich resource for learning about practical performance tuning in Go.
Conclusion
Go offers several unique advantages compared to Python and Java. Its lightweight goroutines provide efficient concurrency, which is more resource-effective than Python’s Global Interpreter Lock (GIL) and Java’s threads. Go’s explicit error handling simplifies error management compared to exceptions in Python and checked exceptions in Java, resulting in more maintainable code.
Dependency management in Go is more straightforward with its built-in module system, whereas Python and Java use more complex tools. Go’s performance optimization through machine code compilation and efficient garbage collection ensures high execution speed compared to Python’s interpreted nature and Java’s JVM overhead.
Go’s philosophy emphasizes simplicity and clarity in code, setting it apart from the more feature-rich approaches of Python and Java. To master Go, explore the official Go website, community blogs, forums, and open-source projects. Staying updated with golang best practices and continuous learning are essential for leveraging Go’s full potential.
The SOLID Principles in Software Development
The SOLID Principles Overview
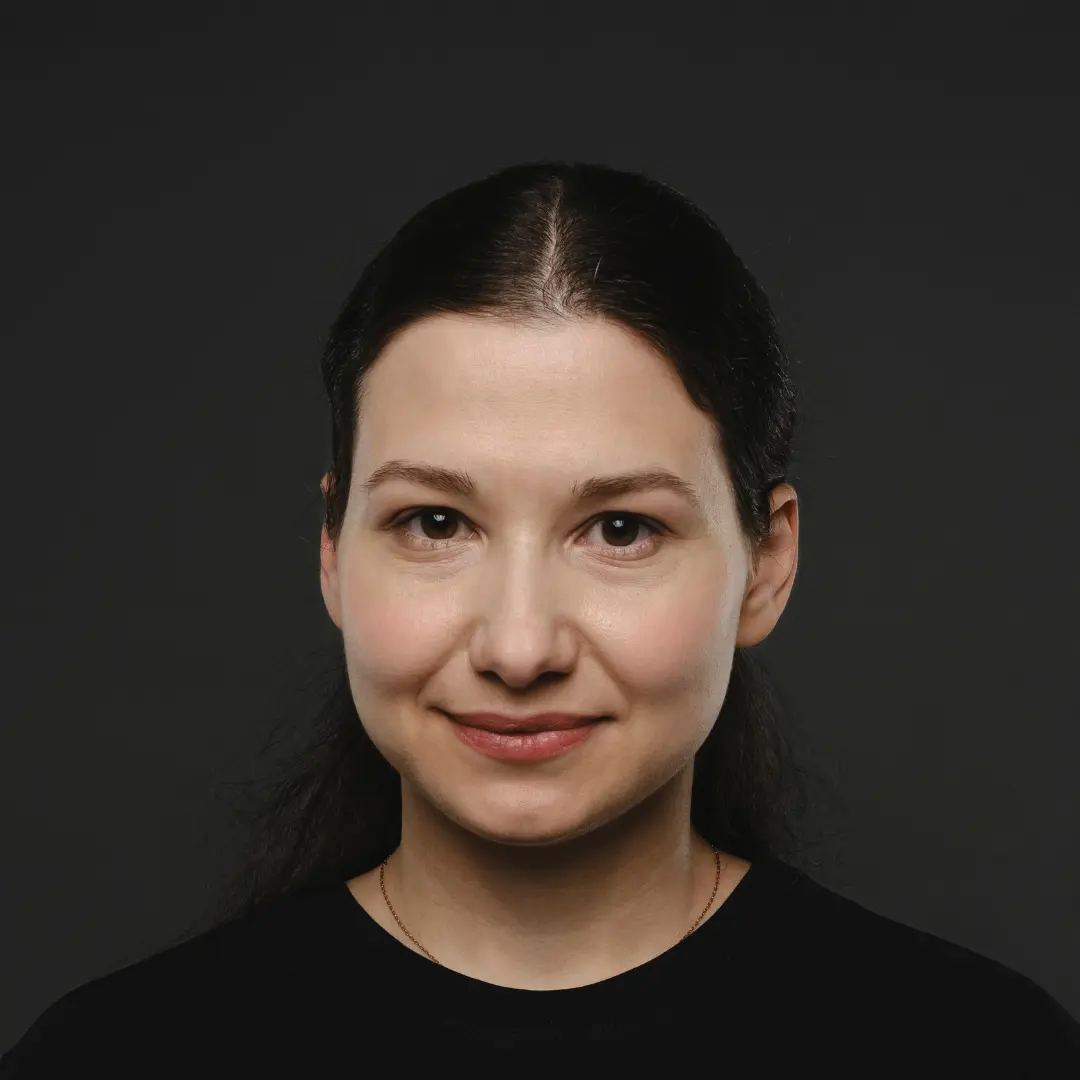
by Anastasiia Tsurkan
Backend Developer
Nov, 2023・8 min read
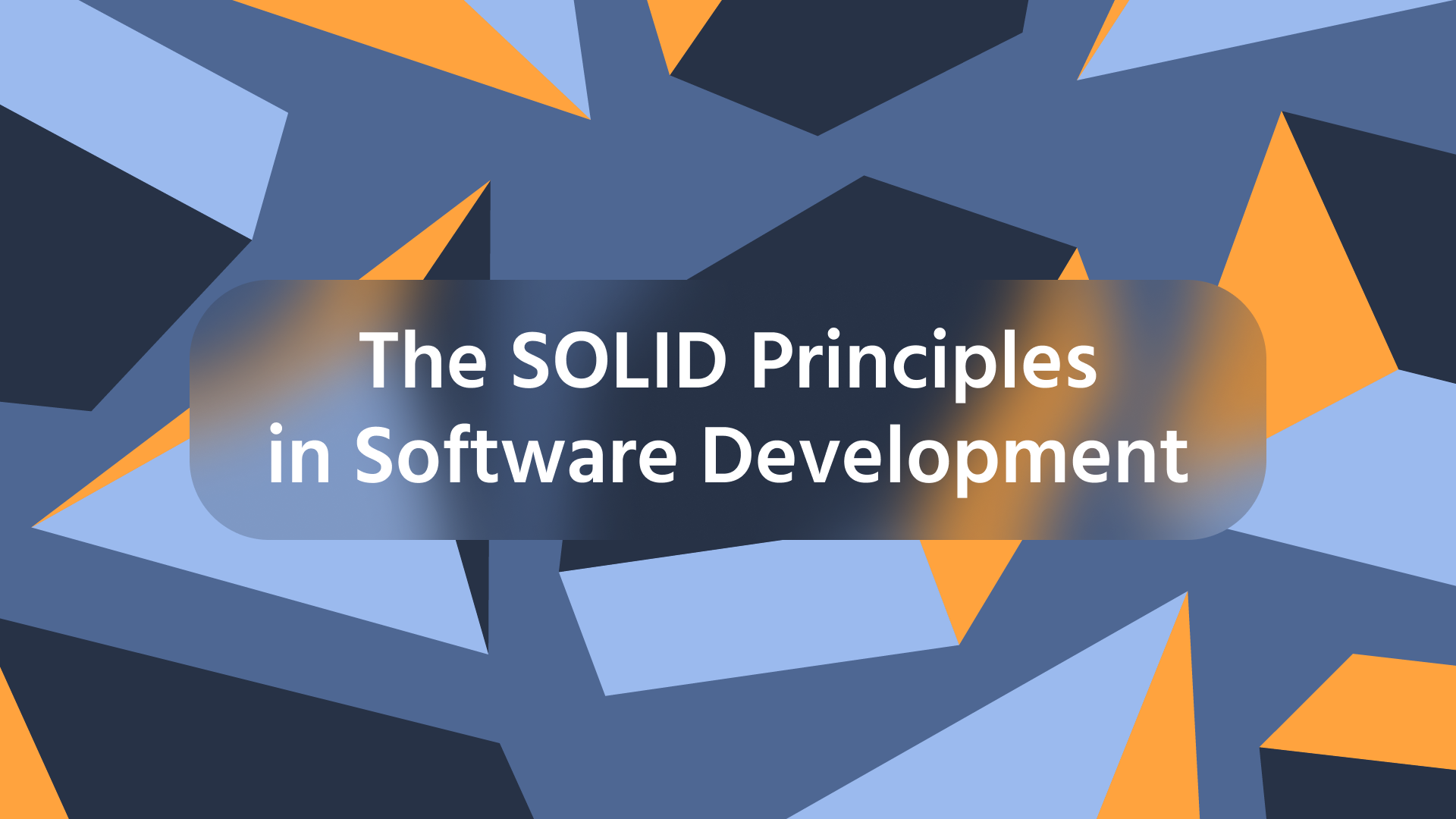
Match-case Operators in Python
Match-case Operators vs if-elif-else statements
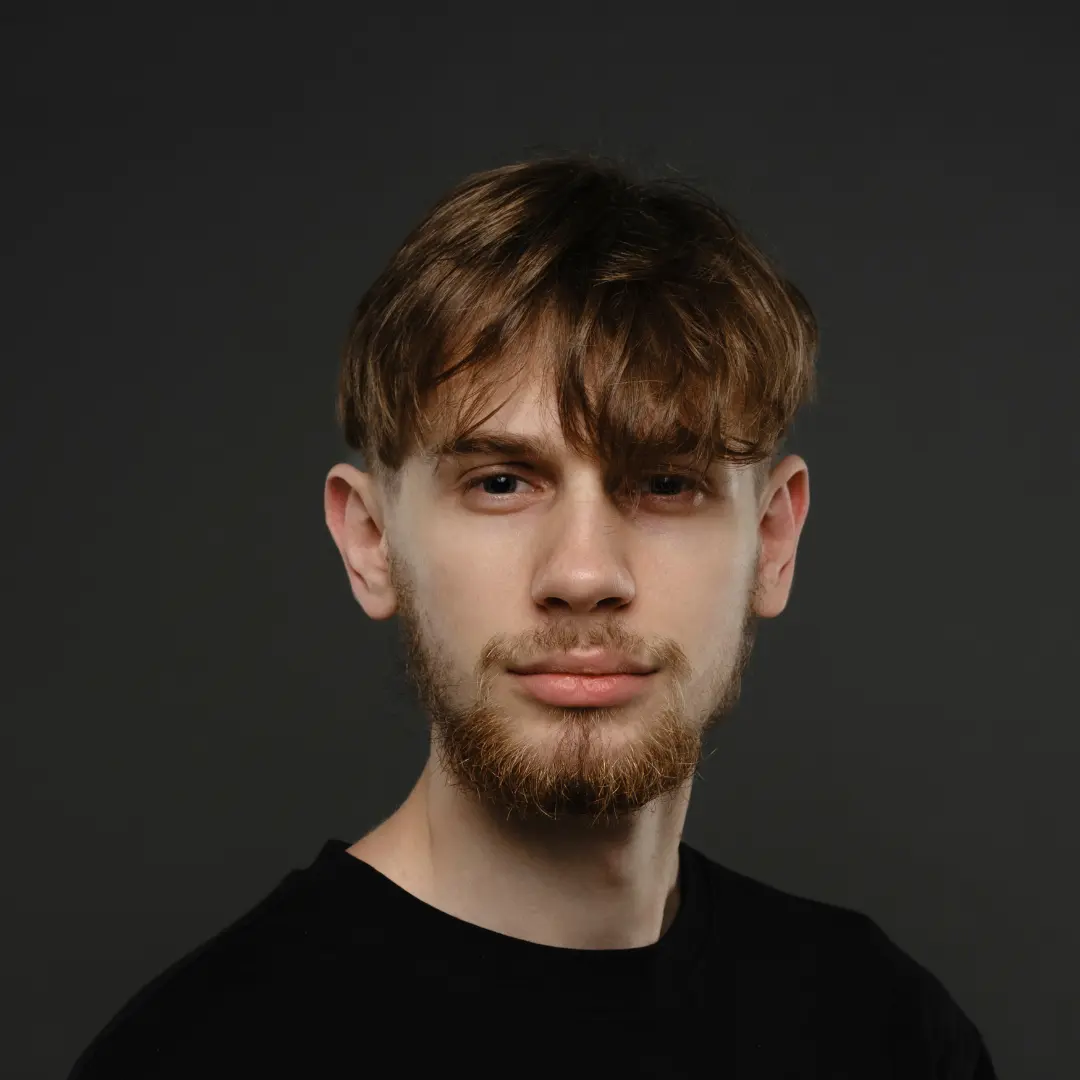
by Oleh Lohvyn
Backend Developer
Dec, 2023・6 min read
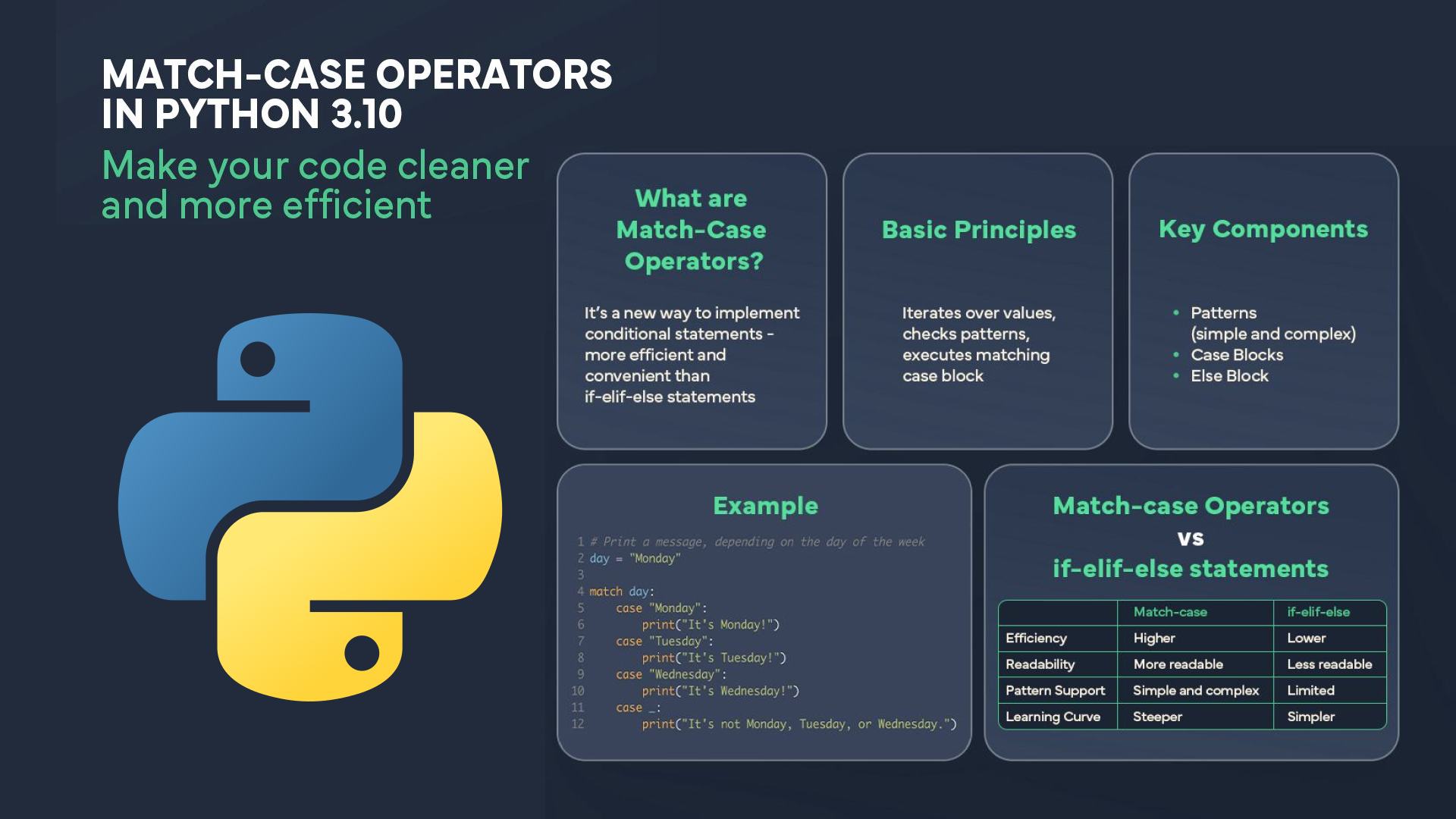
30 Python Project Ideas for Beginners
Python Project Ideas
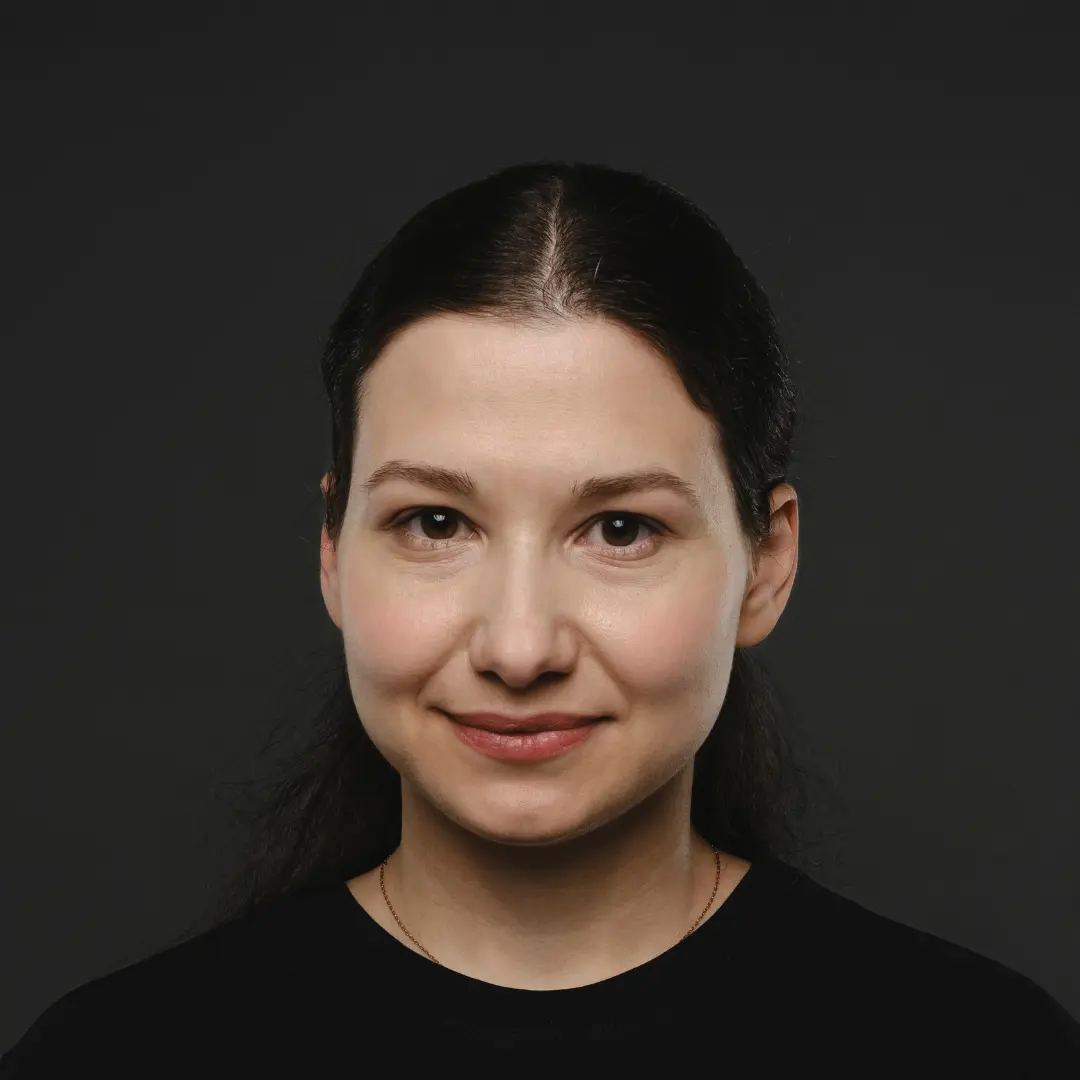
by Anastasiia Tsurkan
Backend Developer
Sep, 2024・14 min read
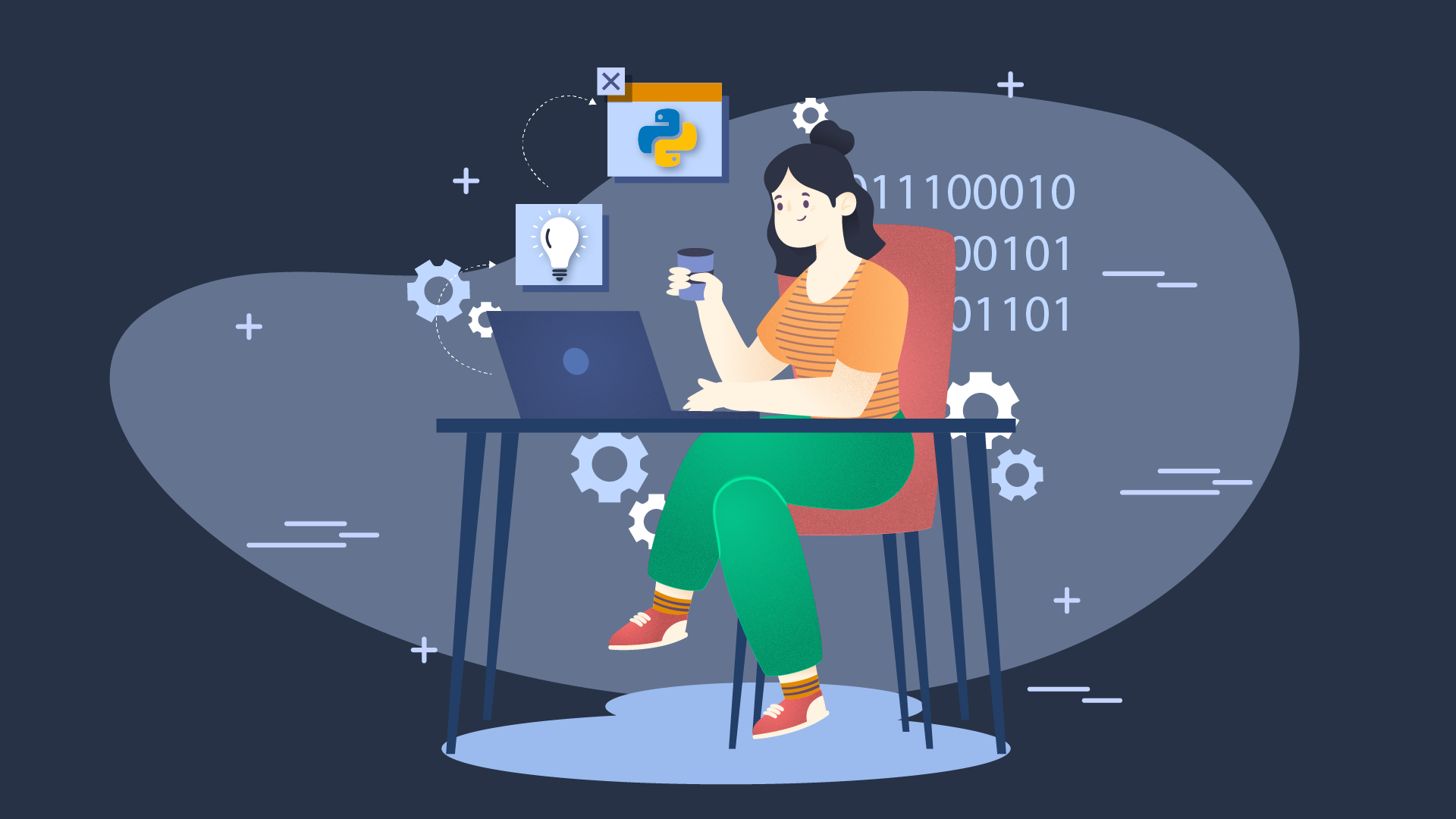
Зміст