Golang 2.0 tutorial
What is Golang and why do we need it?
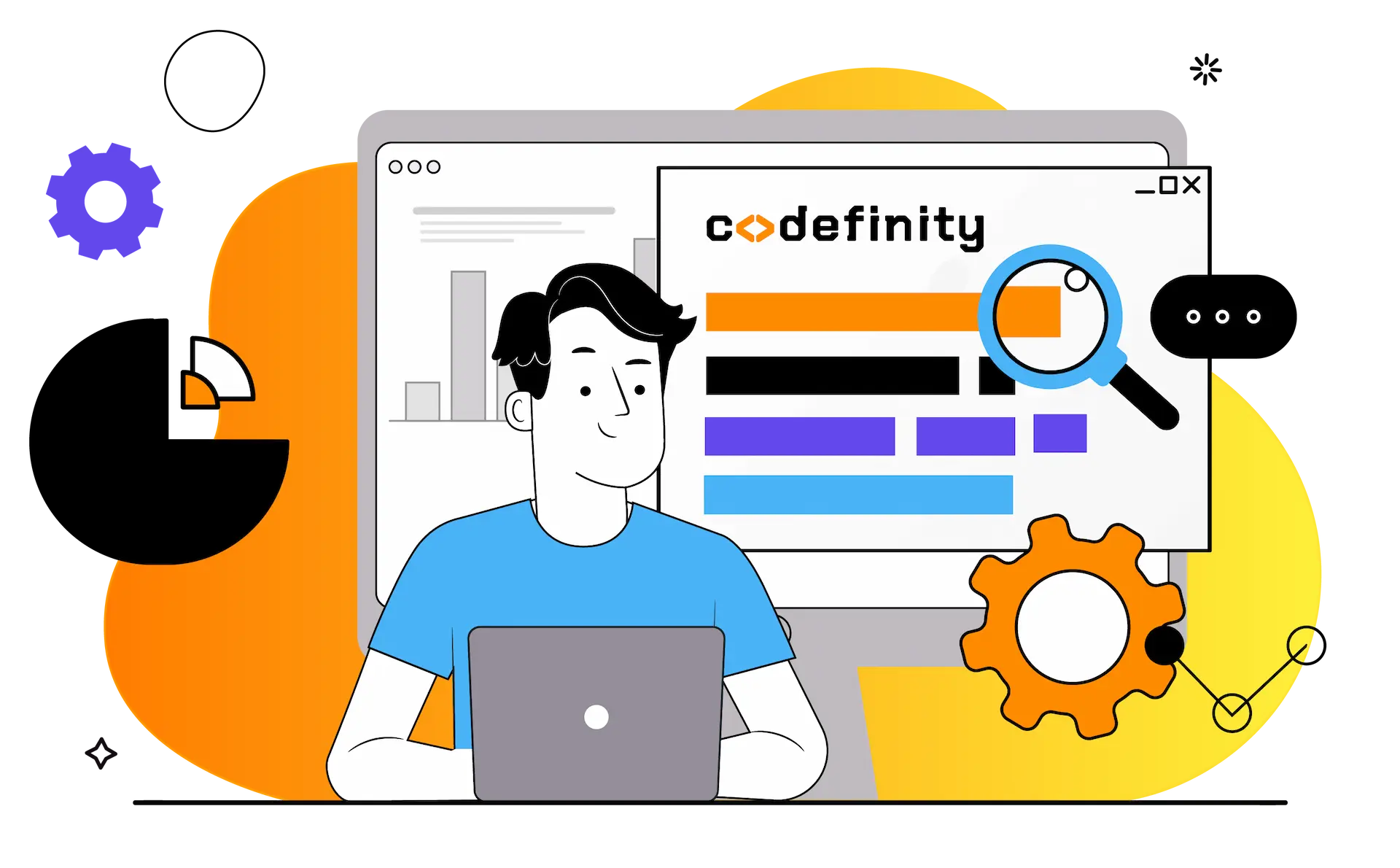
What is Golang and why do we need it?
Go is a programming language created to make programs fast, reliable, and easy to develop. It's helpful for things like:
- Speed: Go allows you to create programs that run very fast. This is important when you need to process a lot of data or respond to requests on the internet.
- Simple Syntax: Go has a fairly simple and understandable syntax, which helps newcomers to programming easily understand and write code.
- Concurrent Development: Go allows you to create programs that utilize multiple cores of your computer, speeding up task processing.
- Efficient Memory Management: Go makes memory management an automatic process, so you don't have to worry about many memory details.
- Abundance of Useful Tools: Go has many tools and libraries that help you do various things, such as creating websites, working with databases, developing network applications, and more.
- Reliability: Go makes it easy to detect and fix errors in your code, making your programs more reliable.
In essence, Go helps developers create programs that are fast, reliable, and easy to develop, even if you are a beginner in programming.
The basic syntax
The main function
in the Go programming language is the entry point for your program. It's where the execution of your program begins. Here's how it works:
package main
: The packagemain
statement tells the Go compiler that this is an executable program, not a library.import "fmt"
: You can import other packages to use their functionality. In this case, we're importing the"fmt"
package, which provides functions for formatted input and output.func main()
: This line declares the main function, which is the starting point of your program. It's a required function, and it will be executed when you run your program.{}
: The curly braces{}
enclose the code that should be executed when themain
function is called.
Here's how the main function works:
- When you run your Go program, the Go compiler finds the main function and starts executing the code inside it.
- You can place any code you need inside the
main function
, such as printing messages to the screen, calling other functions, creating variables, and working with those variables, and more. - When all the instructions inside the
main function
have been executed, the program's execution finishes. - All other functions you create in your program can be called from the
main function
or from other functions, depending on your program's structure and logic.
Run Code from Your Browser - No Installation Required
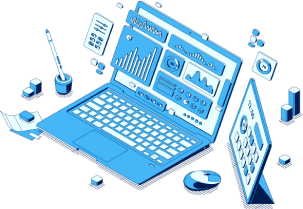
Key aspects of Go's syntax
The syntax of the Go programming language is characterized by its simplicity and clarity, making it user-friendly for both beginners and experienced developers. Here are some key aspects of Go's syntax:
- Declaration and Assignment: In Go, variable declaration and assignment are straightforward. You use the
var
keyword for declaration and the:=
operator for assignment. For example: - Conditional Statements
if
: Go uses theif
statement for conditional execution of code based on a specified condition. For example: - Loops: Go supports two primary types of loops:
for
andrange
.for
is used for iterating a specific number of times, andrange
is used for iterating through elements in collections. An example of afor
loop: - Functions: Functions in Go are declared using the
func
keyword. Parameters are listed within parentheses, and the return type follows the parameter declaration. Here's an example of a function: - Packages: Go uses packages to organize code. Each file should belong to a specific package, import other packages, and functions and variables should be visible within the package.
- Concurrency: Go supports concurrency through goroutines and channels, making it easy to create parallel tasks.
- Simplified Error Handling: In Go, error handling is streamlined with error values, enhancing code reliability.
- Built-in Types and Structures: Go includes various built-in data types like integers, strings, booleans, as well as structures, interfaces, and arrays, making it a versatile language.
The syntax of Go is simplified and easy to understand, making it an excellent choice for both beginners and experienced developers seeking a language with a developer-friendly environment.
On our platform, you can learn the basics of the go language in a course Introduction to GoLang
The conclusions
In conclusion, the Go programming language, often referred to as Golang, stands out as a powerful and approachable tool for software development. Its syntax is known for its simplicity and clarity, making it a favored choice for both newcomers and experienced developers alike.
One of Go's notable features is its efficiency and speed. It excels at executing tasks swiftly, thanks to its effective memory management and support for concurrent code execution. Concurrency, a vital aspect of modern software development, is seamlessly integrated into Go through goroutines and channels, enabling developers to create parallel programs with ease.
Go doesn't abstract away low-level control, which makes it suitable for system-level programming and empowers developers with a level of control over system resources that some other languages might not offer.
Additionally, Go boasts a rich collection of standard libraries, making it easy to tackle various tasks, from handling networking and I/O to cryptography and testing. The language's active and supportive community, along with an abundance of learning resources, fosters skill development and collaboration.
Due to these advantages, Go has gained popularity for diverse application development, including web applications, microservices, system software, development tools, and much more. It enables developers to produce efficient and reliable code, establishing itself as an invaluable asset in the toolkit of programmers.
Start Learning Coding today and boost your Career Potential
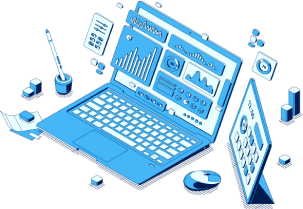
FAQs
Q: What is the Go programming language (Golang)?
A: The Go programming language, often known as Golang, is an open-source programming language developed by Google. It is characterized by its high execution speed, simple syntax, and built-in support for concurrent code execution.
Q: What tasks is Go typically used for?
A: Go is used for developing a variety of applications, including web applications, microservices, system software, development tools, handling large volumes of data, and many other tasks.
Q: Why is Go popular among developers?
A: Go has gained popularity due to its efficiency, fast execution, straightforward syntax, and a large developer community. It empowers developers to create productive and reliable code.
Q: How can I start learning Go?
A: To begin learning Go, you'll need a computer where you can install Go development tools. Then, you can utilize the official Go documentation and online courses to learn the language.
Q: Does Go support object-oriented programming (OOP)?
A: Go has limited support for object-oriented programming (OOP) compared to some other languages. It uses structures and interfaces to implement OOP concepts in a more straightforward manner.
Q: Is Go a free programming language?
A: Yes, Go is a free and open-source programming language, and you can use it freely for software development.
Q: How can I get support and help with Go-related questions?
A: You can get support and help from the Go community through forums, chat channels, and other online resources. The official Go documentation is also a valuable source of information.
The SOLID Principles in Software Development
The SOLID Principles Overview
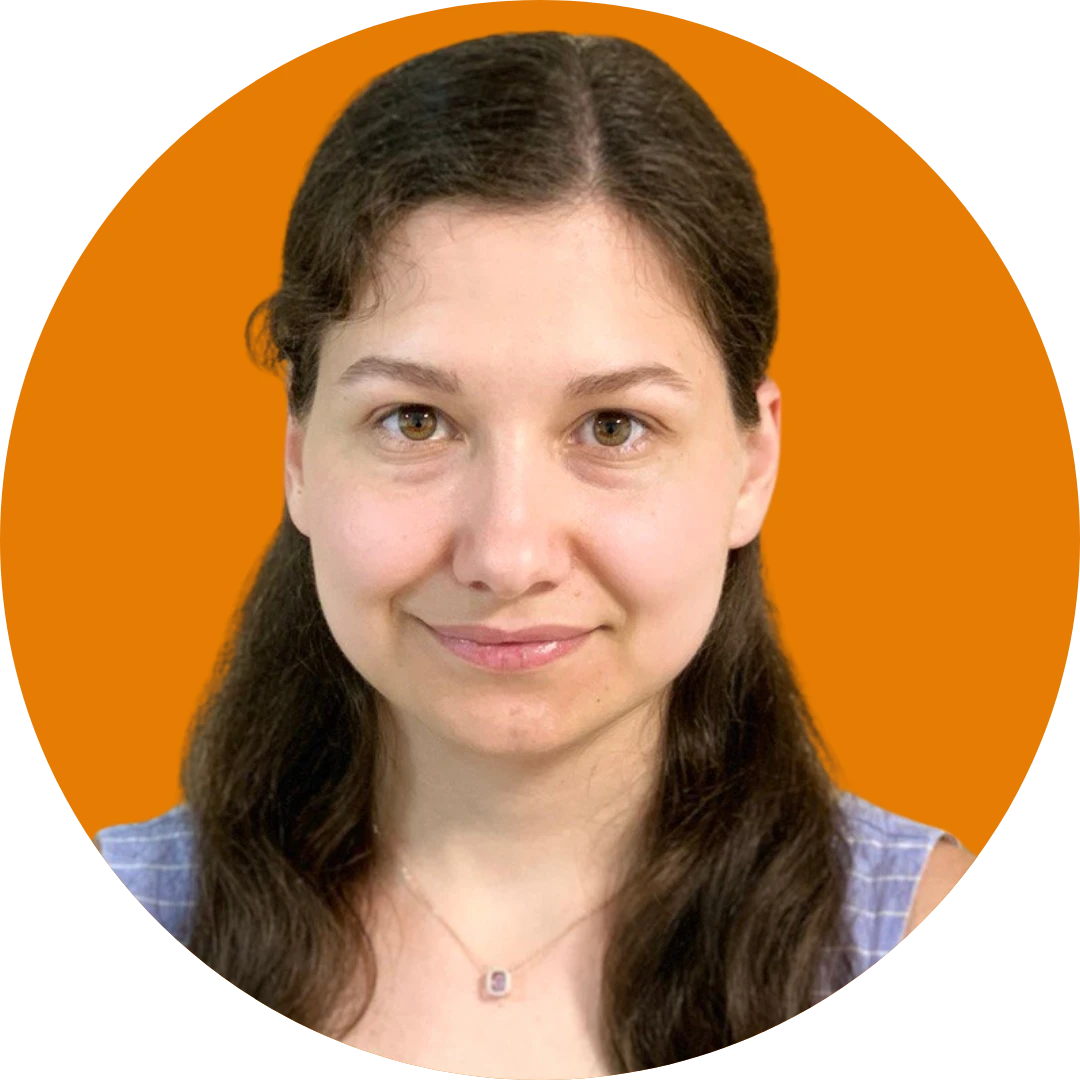
by Anastasiia Tsurkan
Backend Developer
Nov, 2023・8 min read
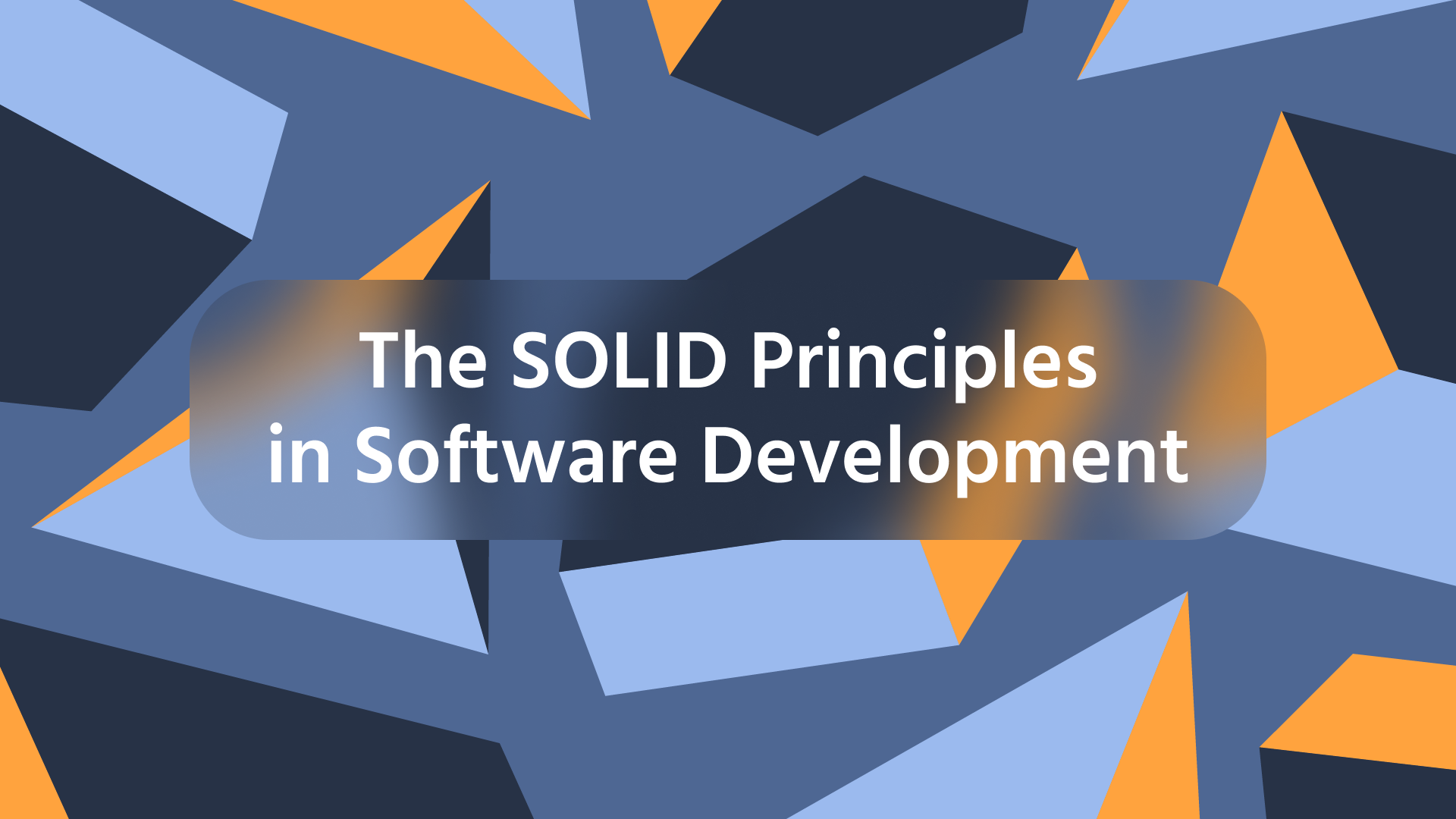
Match-case Operators in Python
Match-case Operators vs if-elif-else statements
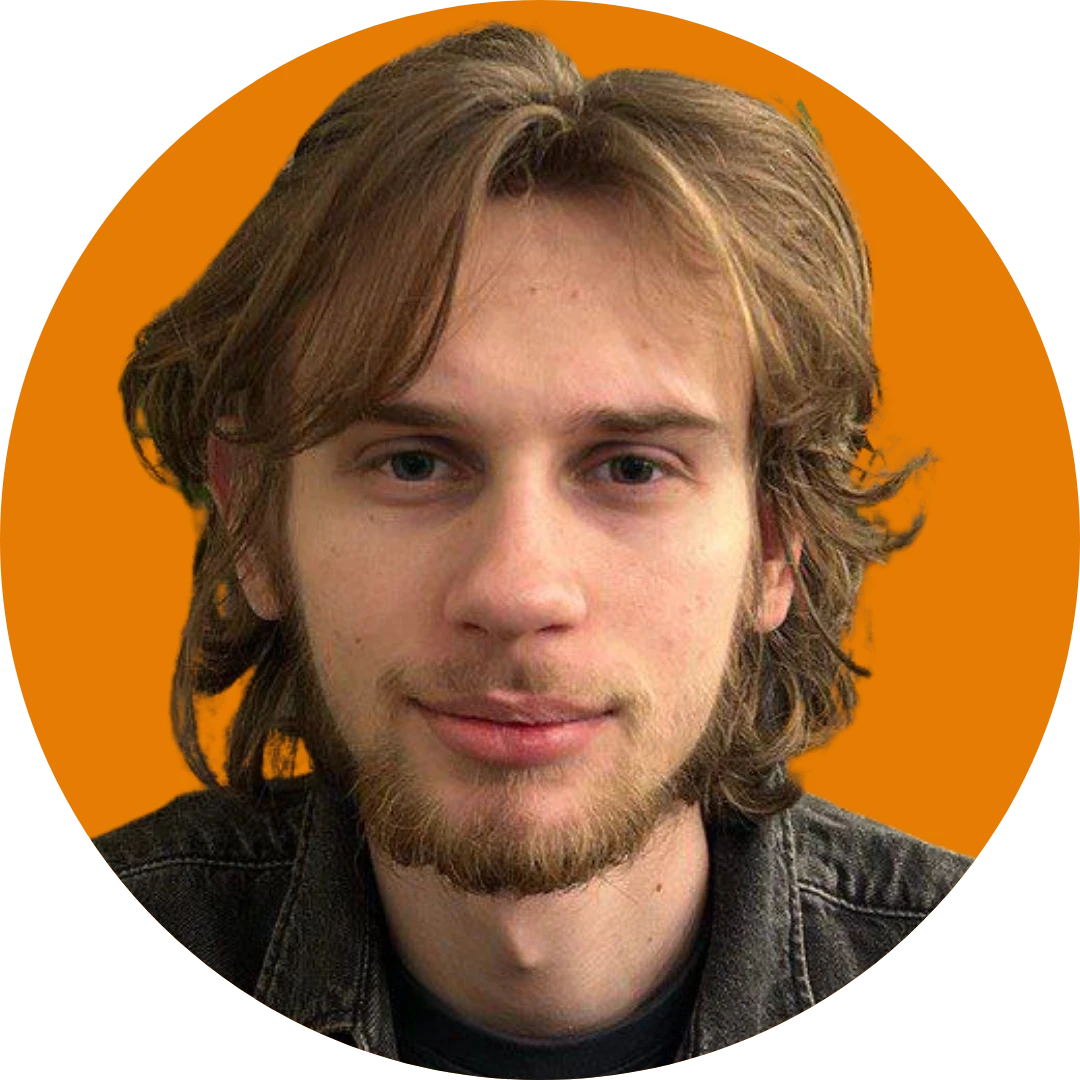
by Oleh Lohvyn
Backend Developer
Dec, 2023・6 min read
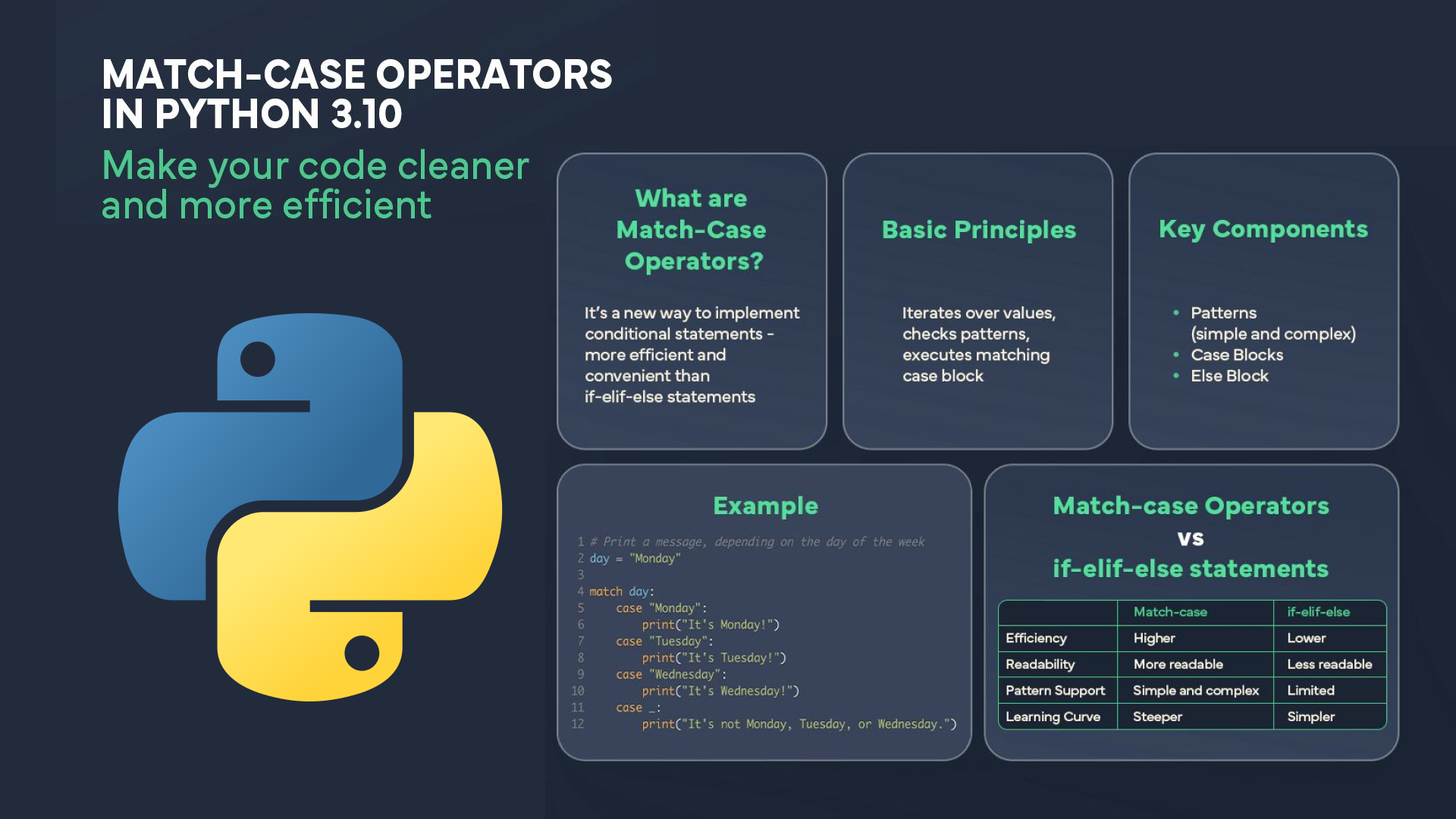
30 Python Project Ideas for Beginners
Python Project Ideas
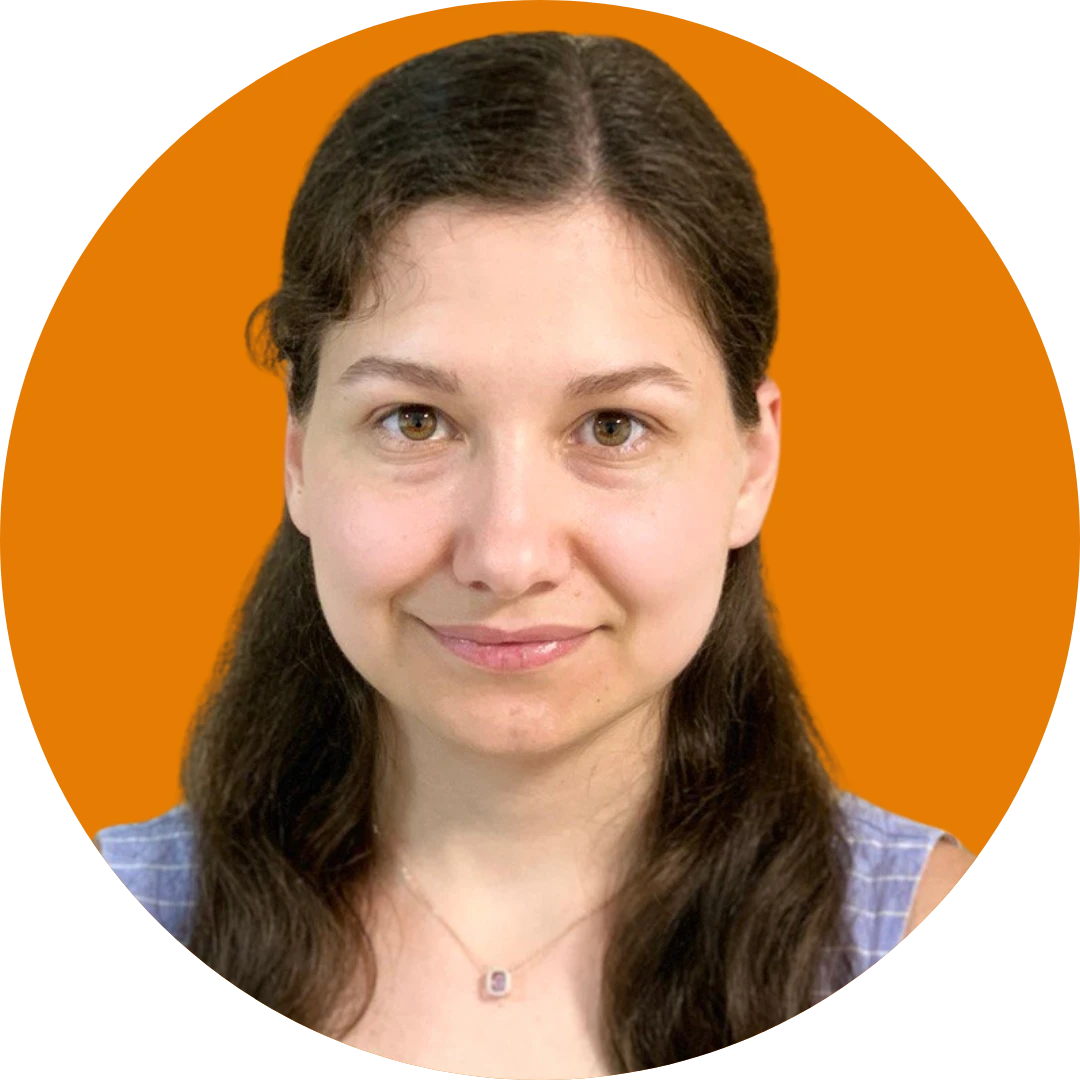
by Anastasiia Tsurkan
Backend Developer
Sep, 2024・14 min read
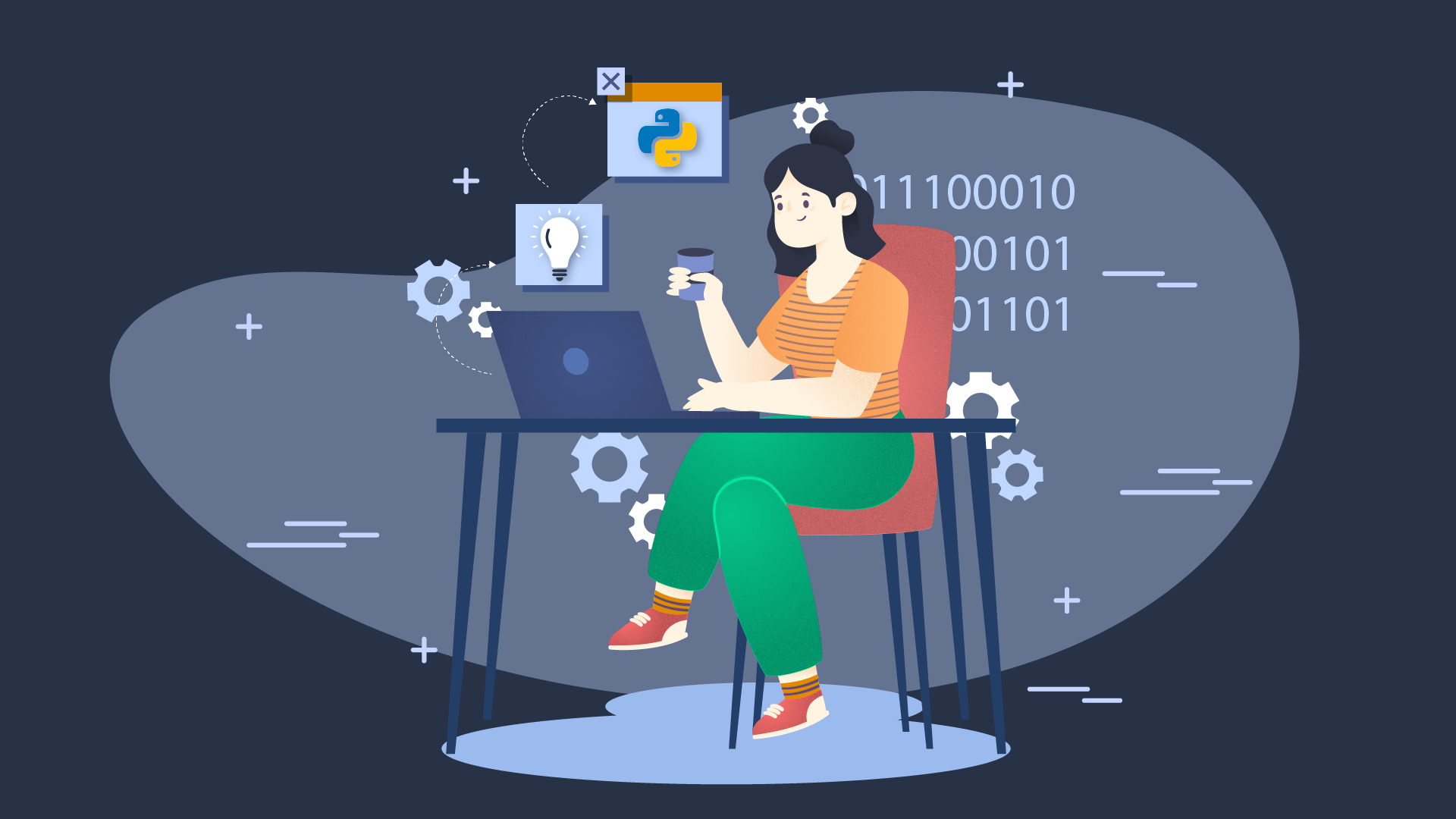
Зміст