Introduction to GoLang
Course
1268 Learners Already enrolled- Gain foundational knowledge of the Go programming language.
- Understand variables, their modification, and the use of constants.
- Grasp basic operators, expressions, and type casting for effective data manipulation.
- Become proficient with control structures, including loops and conditional statements.
Equip your business with cutting-edge Data and AI expertise.
Share it on social media and in your performance review
There are 6 modules in this course
This course is an introduction to the Go language for absolute beginners. It covers all the essential topics that are necessary to start coding in Go.- What is Go Programming Language?Preview
- Hello WorldPreview
- Comments in GoPreview
- Basics of VariablesPreview
- Modifying Variable ValuesPreview
- ConstantsPreview
- Basics of Operators & ExpressionsPreview
- Challenge: Hello WorldPreview
- Challenge: Comments in GoPreview
- Challenge: Basics of VariablesPreview
- Challenge: ConstantsPreview
- Declaring ArraysPreview
- Accessing and Modifying Array ElementsPreview
- SlicesPreview
- Length vs CapacityPreview
- Multi-Dimensional ArraysPreview
- Iterating Over ArraysPreview
- Passing Variable Number of Arguments into FunctionsPreview
- Passing Arrays into FunctionsPreview
- Challenge: Finding the Largest Element in a GridPreview
Chosen by students of top schools
Why people choose Codefinity for their career
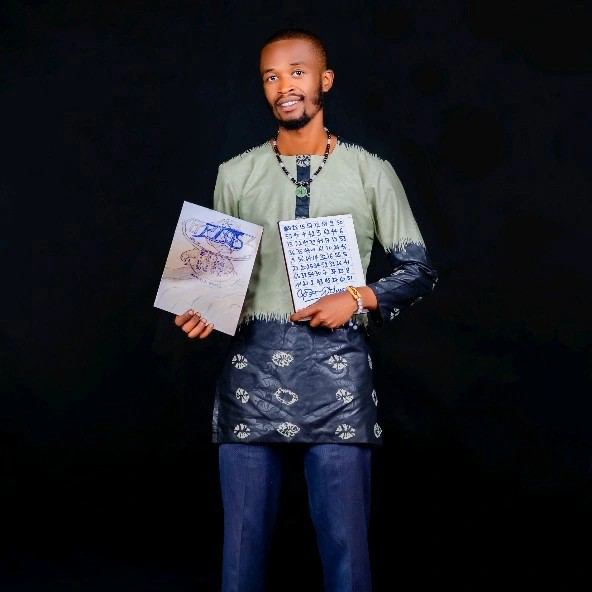
Kwizera Mugisha
The teaching methodology at Codefinity is excellent, and I particularly appreciate how it has prepared me to handle real-world coding problems. Currently, I am delving into Node.js and eagerly anticipate building full-stack projects that integrate all the knowledge I have gained.
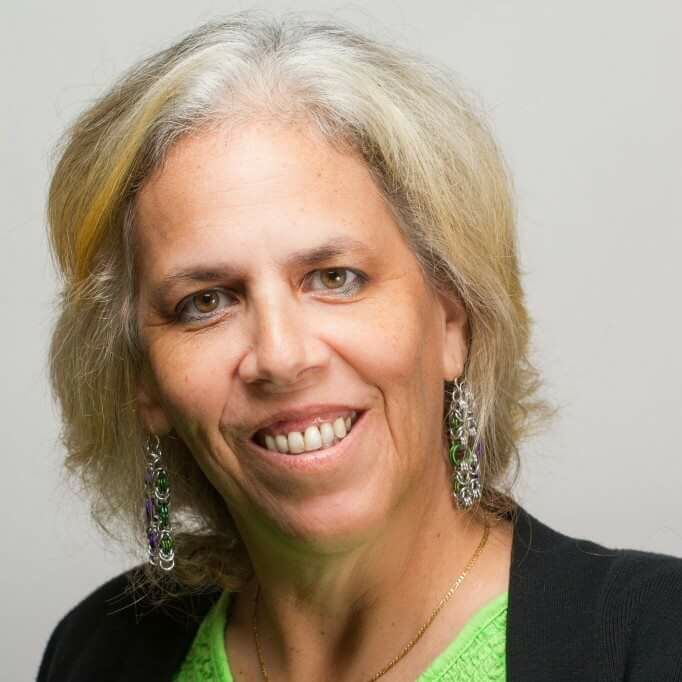
Sherry Barnes-Fox
My first course was 4 hours, I did it in a few days, "nugget-style. The instructions are very clear and easy to understand. There is even a hint to help you get the answer, and if you still cannot get the answer, then you can display the answer. I love the learning style that is used, it engages me.

Bill Wagner
I have really liked the browser-based lessons that allow me to code within the lesson. The RUN button allows me to test the code I write before submitting for a grade.
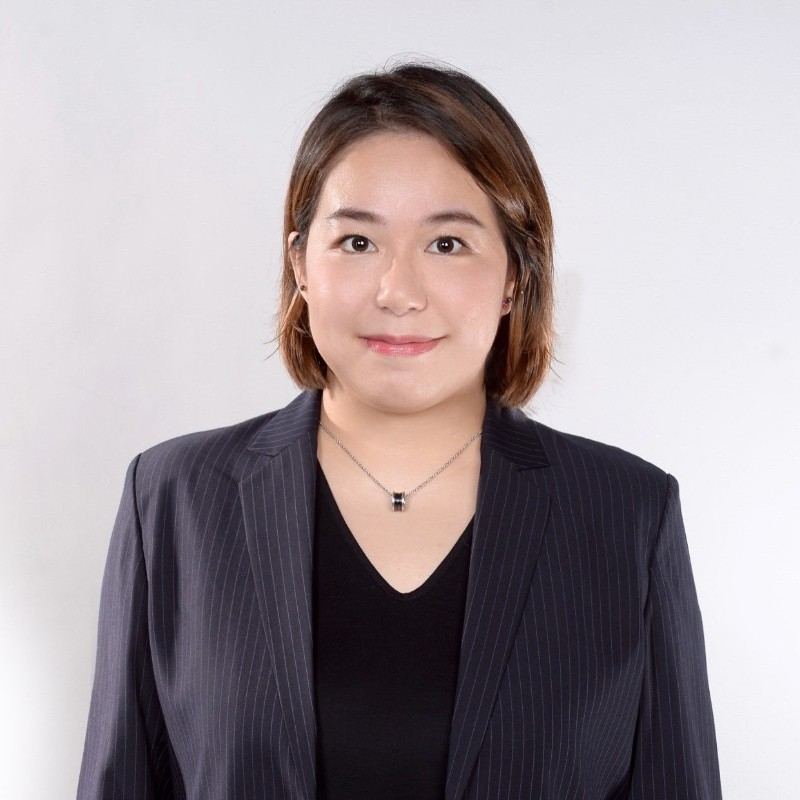
Stephanie Chan
As I went through the first course of the Python track, I liked the way the course was lay out (in easy and digestible modules) with little exercises at the end of each concept.
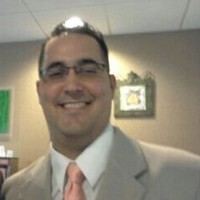
Daniel Chinea
I have gained a lot of practical and logical thinking skills, along with patience for myself and confidence in myself that I can learn programming.
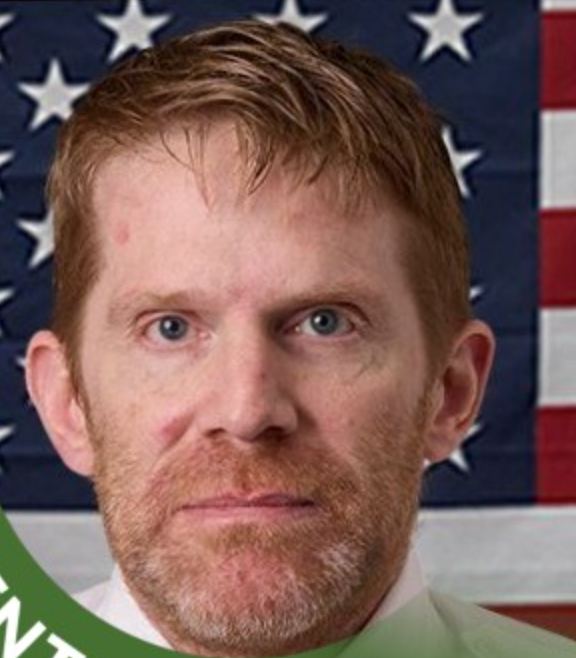
Steve Bruening
The learning was progressive and made it easy to follow along and make progress. I could feel my skills increasing and building on each other as the course went along.
Learner reviews
4.8
16 reviews
5
81%
4
13%
3
6%
2
0%
1
0%
Showing 3 of 16 reviews
Michael Stack
5
Reviewed on Feb 24, 2025
Well put together course. Some examples questions could be a little more revamped for clearer understanding but not a deal killer. A test for completion would be nice.
Jayden Harris
5
Reviewed on Oct 20, 2024
5
Reviewed on Sep 18, 2024
evolutive way to code
Recommended if you're interested in learning Golang
Embrace the fascination of Tech Skills! Our AI-assistant provides real-time feedback, personalized hints, and error explanations, empowering you to learn with confidence.
With Workspaces, you can create and share projects directly on our platform. We've prepared templates for your convenience
Take control of your career development and commence your path into mastering the latest technologies
Real-world projects elevate your portfolio, showcasing practical skills to impress potential employers
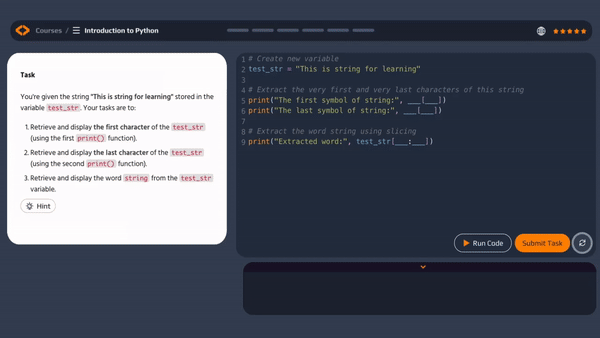

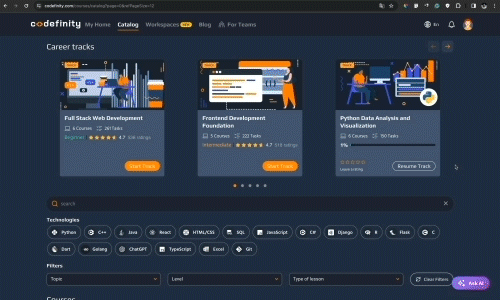
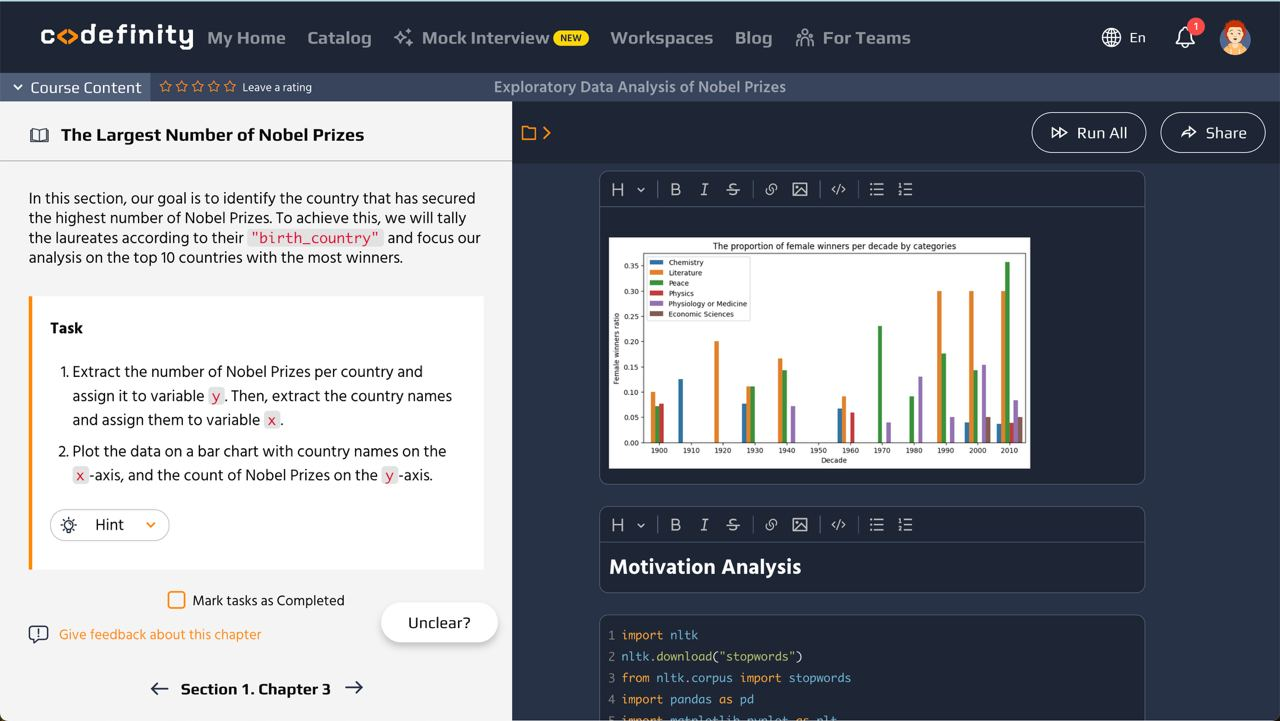
Full catalog access
One subscription opens up this course and our entire catalog of projects and skills.Your subscription also includes:
Frequently asked questions
Still have questions?
Write your question here