Курси по темі
Всі курсиБазовий
Introduction to Python
Python is a high-level, interpreted, general-purpose programming language. Distinguished from languages such as HTML, CSS, and JavaScript, which are mainly utilized in web development, Python boasts versatility across multiple domains, including software development, data science, and back-end development. This course will guide you through Python's fundamental concepts, equipping you with the skills to create your own functions by the conclusion of the program.
Середній
Databases in Python
"Databases in Python" is a hands-on course designed to teach you the fundamentals of working with databases using Python, focusing on the sqlite3 library and SQLAlchemy. You’ll learn how to store, modify, and retrieve data, build efficient queries, and configure databases for your projects. The course covers both SQL basics and the ORM (Object-Relational Mapping) approach, which allows you to interact with databases through Python objects. This course is perfect for beginners looking to deepen their skills in data management, application development, and information handling.
Top 50 Technical Interview Questions for Django Developers
Key Points to Consider When Preparing for an Interview
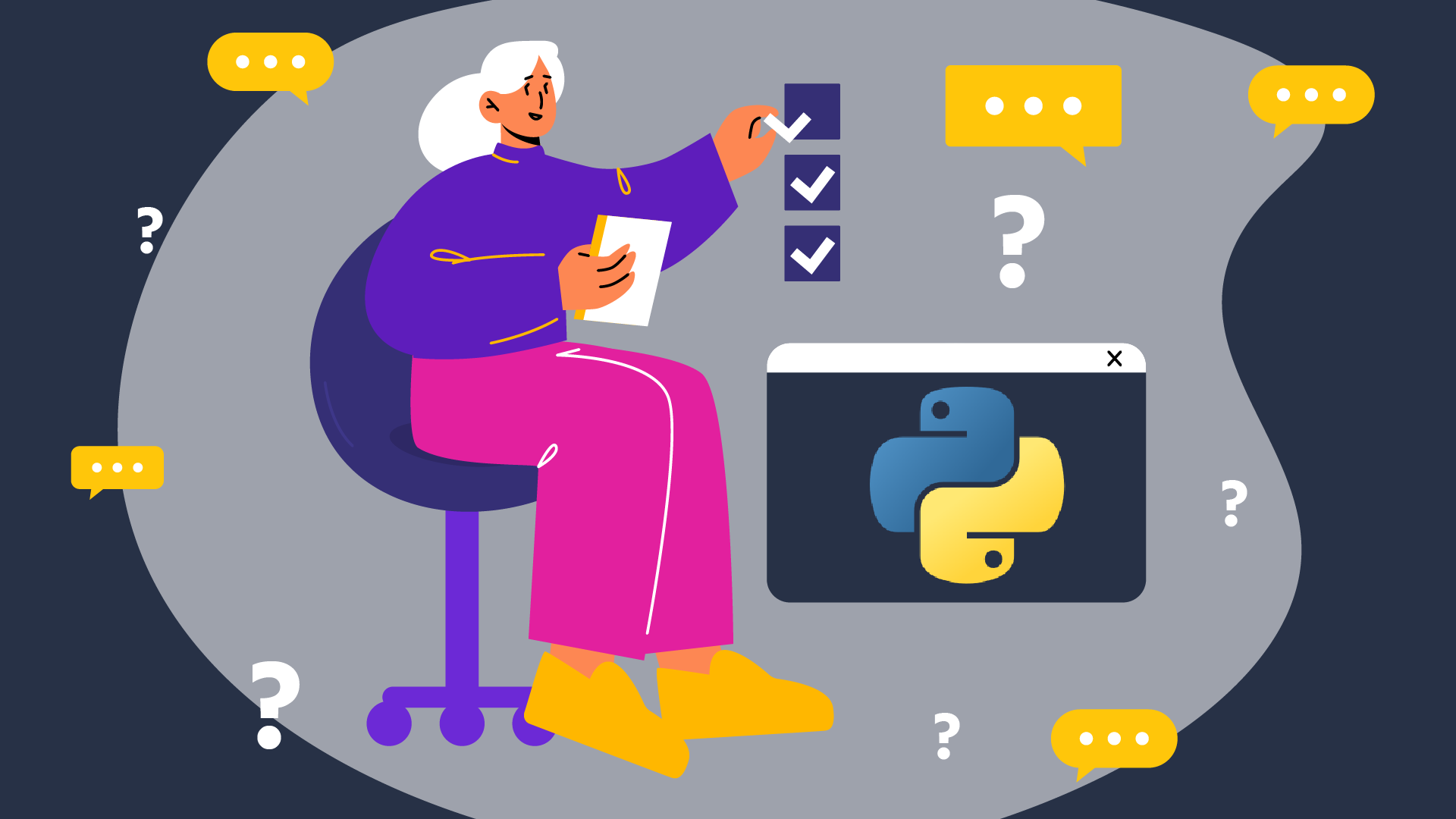
Django is one of the most popular web frameworks, enabling developers to quickly build scalable, secure, and feature-rich web applications. Its flexibility and extensive capabilities make it the go-to choice for businesses of all sizes, from small startups to global corporations.
Mastering Django is an essential skill for any Python developer. However, landing a job requires more than just basic knowledge. Many employers assess your expertise through technical interviews that cover theory, practical skills, and real-world use cases of the framework.
In this article, we’ve compiled the Top 50 Technical Interview Questions for Django Developers to help you prepare. These questions cover all aspects of Django development: from setting up a project to creating models, working with templates, ensuring security, and optimizing applications. This guide will help you confidently ace your interview and deepen your understanding of the framework.
Django Basics
1. What is Django?
Django is a high-level web framework written in Python that allows developers to quickly build secure, scalable, and feature-rich web applications. It was created to simplify the development of complex, data-driven websites by providing built-in solutions for common tasks such as URL routing, database management, user authentication, and more. Django follows the "Don't Repeat Yourself" (DRY) principle, encouraging code reuse and efficiency.
2. What are the advantages of Django?
The key advantages of Django include:
- Speed of development: Django provides many built-in solutions and libraries, allowing developers to rapidly create web applications without having to build everything from scratch.
- Security: Django has built-in features that protect against common web vulnerabilities such as SQL injection, Cross-Site Request Forgery (CSRF), and Cross-Site Scripting (XSS).
- Scalability: Django easily scales for large applications, which is why it’s used by companies like Instagram and Pinterest.
- Active community: Django has a large and active community, ensuring regular updates, support, and contributions.
- Flexibility: Although Django enforces certain standards, it also allows for customization and extension to meet specific needs.
3. How does the Django MVT architecture work?
Django follows the MVT (Model-View-Template) architecture, which is a variation of the more common MVC (Model-View-Controller) architecture:
- Model: Represents the data of the application and handles interactions with the database. Models define the structure of the data and handle data processing through Django's ORM.
- View: Handles the logic of processing requests and returns responses. Views get data from the models and send it to the templates for rendering.
- Template: Displays the data to the user in the form of HTML. Templates can include dynamic content that is passed from the view to be rendered in the browser.
4. What is ORM in Django, and how does it work?
ORM (Object-Relational Mapping) in Django is a tool that allows developers to interact with the database using object-oriented programming rather than writing raw SQL queries. Django's ORM automatically generates SQL code based on the defined models, enabling developers to interact with the database through Python objects.
- For example, when creating a model class in Django, it is automatically mapped to a database table. Instead of writing SQL queries, developers use Python methods to create, update, delete, and retrieve data from the database.
5. How do you create a new project in Django?
To create a new Django project, follow these steps:
- Install Django using pip:
bash
- Create a new project using the
django-admin
command:
This command creates a folder with the project name, which contains the basic structure of a Django project.bash - Navigate into the project directory:
bash
- Start the development server to check if Django is set up correctly:
You should see a message indicating that the server is running atbashhttp://127.0.0.1:8000/
, and you can check the project in your browser.
These steps will help you create and run a basic Django project.
6. What are Django apps?
In Django, an app is a self-contained module or component that provides specific functionality to a Django project. An app typically consists of models, views, templates, and other resources related to a specific feature of the project. For example, you might have an app for user authentication, another for blog posts, and another for a shopping cart. Django encourages a modular approach, allowing you to organize different features into separate apps, making it easier to maintain and scale the project. Apps can be reused in multiple projects.
7. What is the Django admin site?
The Django admin site is a built-in, web-based interface that allows administrators to manage and interact with the data of the Django project. It is automatically generated based on the models defined in the project. Through the admin site, users can perform CRUD (Create, Read, Update, Delete) operations on the models without writing any additional code. The admin interface is highly customizable and can be extended with custom fields, forms, and actions to suit specific needs.
8. What is a Django middleware?
Middleware in Django is a lightweight, low-level function that sits between the web server and the Django application. It processes requests before they reach the view and processes responses before they are sent back to the client. Middleware can be used for various purposes, such as request/response processing, session management, authentication, security checks, and more. Django provides built-in middleware for handling things like security, sessions, and caching, but you can also write custom middleware to implement specific functionality.
9. How do you handle static files in Django?
In Django, static files refer to files such as CSS, JavaScript, and images that are not dynamically generated by the application but are used in the presentation layer. To manage static files:
- During development, Django automatically serves static files from a folder named
static
inside the app or project. - For production, you need to collect static files from various apps into a single location using the
collectstatic
management command:bash - Django will then serve these files from a designated directory specified in the settings (
STATIC_ROOT
), and they can be served by a web server like Nginx or Apache.
10. What is Django’s URL dispatcher?
Django’s URL dispatcher is responsible for mapping incoming requests to the appropriate view. It works by using URL patterns defined in the urls.py
file, which is part of each Django app or project. The dispatcher matches the requested URL to one of the patterns and then calls the corresponding view function to handle the request.
- URL patterns are defined using regular expressions or path converters, and you can also include parameters in the URL, which can be passed to the view function for further processing.
- For example, a simple URL pattern might look like this:
python
These are the answers to the next five questions in the "Basics of Django" section.
Run Code from Your Browser - No Installation Required
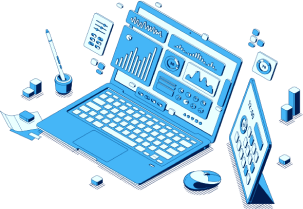
Django Models
1. What is a model in Django?
A model in Django is a Python class that defines the structure of the data for the application. It acts as a blueprint for database tables, mapping the fields of the model to columns in the corresponding database table. Models are the central part of Django's ORM (Object-Relational Mapping) system, allowing you to interact with the database using Python code instead of raw SQL. Models define fields (e.g., CharField
, IntegerField
, DateField
) and methods for accessing and manipulating the data.
2. How do you create a model and apply migrations?
To create a model in Django:
- Define a class that inherits from
django.db.models.Model
. - Add fields to the class, using Django's built-in field types.
- After defining the model, run migrations to apply the changes to the database.
- Step 1: Create a migration for the model by running the following command:
bash
- Step 2: Apply the migration to the database:
bash
- Step 1: Create a migration for the model by running the following command:
3. What is a migration, and how do you run it?
A migration in Django is a way of propagating changes you make to your models (like adding, deleting, or modifying fields) into the database schema. Migrations are stored in Python files within the migrations
folder of each Django app. To create and apply migrations:
makemigrations
generates migration files based on changes made to models.migrate
applies the migrations to the database. Migrations help ensure that your database schema stays in sync with your model definitions.
4. How do you define "one-to-one," "one-to-many," and "many-to-many" relationships?
In Django models, relationships between models are defined using specific fields:
- One-to-One: Use
OneToOneField
to define a one-to-one relationship, where one object in a model is related to exactly one object in another model.python - One-to-Many: Use
ForeignKey
to define a one-to-many relationship, where one object in a model is related to many objects in another model.python - Many-to-Many: Use
ManyToManyField
to define a many-to-many relationship, where multiple objects in one model are related to multiple objects in another model.python
5. How do ForeignKey and ManyToManyField work in models?
- ForeignKey is used to define a many-to-one relationship between two models. It means that each instance of the model with the
ForeignKey
can be linked to one instance of another model. Theon_delete
parameter specifies what happens when the referenced object is deleted (e.g.,CASCADE
will delete all related objects).python - ManyToManyField is used to define a many-to-many relationship. This field creates an intermediary table in the database that stores the relationship between two models.
python
6. How do you define standard and custom model managers?
A manager in Django is a class that manages database query operations for model instances. The default manager, objects
, is automatically created for every model. You can also define custom managers by inheriting from models.Manager
and adding methods that return customized querysets:
- Standard manager: The default manager for a model.
python
- Custom manager: You can add methods to the custom manager to filter or return specific querysets.
python
7. What is a QuerySet in Django?
A QuerySet in Django represents a collection of database queries that retrieve data from the database. QuerySets are lazy, meaning they don’t hit the database until they are evaluated (e.g., through iteration, slicing, or converting to a list). You can filter, exclude, or modify QuerySets using methods like filter()
, exclude()
, and get()
. QuerySets allow you to retrieve, modify, and perform other operations on model instances in an efficient and flexible manner.
8. How do filters (filter(), exclude(), get()) work in QuerySet?
filter()
returns a new QuerySet with objects that match the given criteria:pythonexclude()
returns a new QuerySet with objects that do not match the given criteria:pythonget()
retrieves a single object based on the given parameters. If no match is found, it raisesDoesNotExist
; if multiple objects are found, it raisesMultipleObjectsReturned
:python
9. How do you implement the save() and delete() methods in a model?
- The
save()
method in Django is used to save an instance of a model to the database:python - The
delete()
method is used to delete a model instance from the database:python
10. How does cascade deletion work in Django?
Cascade deletion is implemented using the on_delete=models.CASCADE
option in a ForeignKey
field. When an object referenced by a ForeignKey
is deleted, all related objects are also deleted automatically. For example:
python
If a User
object is deleted, all Post
objects that reference that user will also be deleted automatically.
These answers should help explain key concepts related to Django models and working with data in the Django ORM.
Django Templates
1. What is a template in Django?
A template in Django is an HTML file that contains dynamic content and is used to render the final output that will be displayed in the browser. It allows you to separate the presentation (HTML) from the logic of your application. Templates can include variables, logic (like loops and conditions), and template tags and filters to display dynamic data based on what is passed from the views.
2. How do you pass data from a view to a template?
In Django, data is passed from the view to the template through a context. The context is a dictionary that contains keys and values, where the keys are the variable names that will be used in the template, and the values are the data that you want to display. To pass the data to the template:
- In the view, you use
render()
to send the context to the template:python
3. How do tags and filters work in Django templates?
-
Tags are used to control the logic within templates, such as loops, conditions, and other template-related actions. For example:
{% if %}
: Used for conditional logic.{% for %}
: Used for loops to iterate over a list.{% include %}
: Includes another template in the current template.
html -
Filters are used to modify the output of variables before displaying them in the template. Filters are applied using the
|
symbol. For example:{{ value|lower }}
: Converts text to lowercase.{{ value|length }}
: Gets the length of a list or string.
html
4. What is context in templates?
In Django templates, context refers to the data passed from the view to the template. It is a dictionary where the keys are variable names, and the values are the actual data or objects that the template can use. For example:
python
Within the template, you can access the context data as follows:
html
5. How does template inheritance work in Django?
Django templates support template inheritance, allowing you to create a base template and extend it in other templates. This promotes reusability and keeps your code DRY (Don’t Repeat Yourself). The base template contains common elements (like headers, footers, or navigation), and child templates override specific blocks.
- Base template (
base.html
):html - Child template (
home.html
):html
6. How do you use static files in templates?
Django provides a way to include static files (CSS, JavaScript, images) in your templates using the {% static %}
tag. This ensures that static files are served correctly in both development and production environments.
- First, include
{% load static %}
at the beginning of your template. - Then, you can reference static files like this:
html
7. How do templates work for forms in Django?
Django provides a convenient way to generate and render HTML forms using templates. You can render form fields manually or use Django's built-in form rendering methods.
- Automatic rendering of forms:
html
form.as_p
renders the form fields as<p>
elements. - You can also manually render individual fields:
html
8. How do you handle template errors?
Django provides error handling mechanisms in templates:
- Template syntax errors: Django will raise a
TemplateSyntaxError
, which includes the line number and a message about the error. - Displaying custom error messages: You can display error messages in forms using Django’s
{{ form.errors }}
:html
9. How do you add dynamic content in templates?
Django templates allow you to add dynamic content using variables and template tags. You can display dynamic content like data from the view, such as user details, date and time, or results from a database query.
- Displaying variables:
html
- Using template tags:
html
10. How do you implement custom tags and filters?
You can create custom template tags and filters by writing a Python file in a templatetags
directory within your app.
-
Custom filter: To create a custom filter:
- Create a file called
custom_filters.py
in thetemplatetags
folder of your app.
python- In your template:
html - Create a file called
-
Custom tag: To create a custom tag:
- Create a file called
custom_tags.py
in thetemplatetags
folder of your app.
python- In your template:
html - Create a file called
These answers should help clarify the usage of templates in Django, including key features like context, inheritance, and custom tags/filters.
Django Views
1. What is a view in Django?
A view in Django is a Python function or class that receives a web request and returns a web response. The response can include HTML content, redirections, JSON data, or other content types. Views handle the business logic, such as querying the database, processing forms, and returning the appropriate response to the user.
- Example of a simple view:
python
2. What is the difference between function-based views (FBVs) and class-based views (CBVs)?
-
Function-based views (FBVs): These are simple Python functions that take a request object as an argument and return a response. They are more straightforward and easier to understand for simple use cases.
- Example:
python
- Example:
-
Class-based views (CBVs): These are more structured and object-oriented, allowing you to create reusable views by subclassing Django's generic view classes. They are better suited for more complex views with reusable patterns like handling forms, lists, or CRUD operations.
- Example:
python
- Example:
3. How to implement a redirect in Django?
In Django, you can redirect users to a different URL using the redirect()
function from django.shortcuts
. This is useful when you want to send users to another page after a form submission or other action.
- Example of redirecting to another URL:
python
- You can also pass a URL as a string:
python
4. How do you handle HTTP requests (GET, POST) in views?
Django views can handle different HTTP methods, such as GET
and POST
, using simple conditional logic or through class-based views.
-
Function-based views:
python -
Class-based views: Use methods like
get()
andpost()
to handle respective HTTP methods.python
5. What is JsonResponse and how do you use it?
JsonResponse
is a subclass of Django's HttpResponse
that allows you to return JSON-encoded data as a response. It's commonly used when creating APIs or sending data to a frontend application.
- Example of using
JsonResponse
:python
6. How to handle 404 and 500 errors in Django?
Django provides a built-in way to handle common HTTP errors such as 404 (Page Not Found) and 500 (Server Error).
-
404 Error: To handle 404 errors (when a page is not found), you can create a custom template named
404.html
. Django will use this template if a page is not found.- Example:
- Create a
404.html
file in your templates directory to display a custom 404 error page.
- Create a
- Example:
-
500 Error: Similarly, you can create a custom template named
500.html
to handle server errors.- Example:
- Create a
500.html
file in your templates directory to display a custom 500 error page.
- Create a
- Example:
Additionally, you can raise a Http404
exception manually in your views:
python
7. How do you use mixins in class-based views?
Mixins are used in class-based views to add common functionality without needing to rewrite code. They allow you to reuse pieces of logic across different views.
- Example of a mixin:
python
8. How do the dispatch()
and get_context_data()
methods work in class-based views?
-
dispatch(): This method is called when a request is made to a class-based view. It determines which method (such as
get()
,post()
, etc.) should be called based on the HTTP method of the request. You can overridedispatch()
to add custom logic before or after the request is processed.Example:
python -
get_context_data(): This method is used to add extra context data to a template. It is commonly used in views that render templates (such as
ListView
orDetailView
). You can override it to pass additional context.Example:
python
9. What is Middleware in Django, and how do you implement it?
Middleware is a way to process requests globally before they reach the view and after the view has processed them. Middleware can be used for various tasks like authentication, logging, and security.
-
Example of implementing middleware:
- Create a custom middleware class that implements
__init__()
and__call__()
methods.
python - Create a custom middleware class that implements
-
Add it to the
MIDDLEWARE
list insettings.py
:python
10. How to add custom Middleware?
To add custom middleware in Django, you can create a class that inherits from MiddlewareMixin
(for Django versions before 2.0) or implement the necessary methods in a class (for Django 2.0+).
-
For Django 2.0+:
python -
Add the middleware to the
MIDDLEWARE
setting insettings.py
:python
These answers cover key aspects of handling views, HTTP requests, and middleware in Django, which will be useful for building robust applications.
Start Learning Coding today and boost your Career Potential
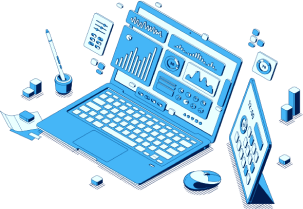
Forms and Validation in Django
1. How do you create a form in Django?
In Django, forms are created by defining a form class that inherits from django.forms.Form
. This class defines the fields and their types, such as CharField
, IntegerField
, DateField
, etc.
- Example of creating a basic form:
python
Once the form is created, you can use it in a view to render the form in a template and handle user input.
2. How is form data validation handled?
Django provides built-in validation for form fields, such as ensuring the correct data type (e.g., email format or minimum length). Validation is automatically applied when you call form.is_valid()
.
- Example of validation:
python
You can also define custom validation for individual fields using the clean_<field_name>()
method, which allows you to write additional checks (e.g., checking if the email already exists in the database).
3. What are ModelForms in Django?
A ModelForm is a special form class that is linked directly to a Django model. It automatically generates form fields based on the model's fields, simplifying the creation of forms that correspond to model data. ModelForms are useful when you want to create, update, or delete model instances via forms.
- Example of using a
ModelForm
:python
Once you create a ModelForm
, you can use it to create or update model instances:
python
4. How do you implement custom validation in forms?
Custom validation can be implemented by overriding the clean()
method or by defining specific clean_<field_name>()
methods for individual fields. The clean()
method is useful when you need to perform validation that involves multiple fields, while clean_<field_name>()
is used for field-specific validation.
-
Example of field-specific validation:
python -
Example of overriding
clean()
for custom form-wide validation:python
5. How do you handle file uploads in forms?
To handle file uploads in Django, you need to use FileField
or ImageField
in your form and ensure that your view is set up to handle file uploads. Additionally, you need to include enctype="multipart/form-data"
in your HTML form tag.
-
Example of a form with a file field:
python -
Example of handling file upload in a view:
python
In the HTML template, make sure to include enctype="multipart/form-data"
:
html
By following these steps, you can handle file uploads seamlessly in Django forms.
Security and Authentication in Django
1. How does Django’s authentication system work?
Django’s authentication system is designed to handle user login, logout, password management, and user permissions. It provides a set of built-in views, forms, and models to manage authentication. The core of Django's authentication system is the User
model, which includes fields like username
, password
, email
, and is_authenticated
. It also includes functions like login()
, logout()
, and authenticate()
.
- To authenticate a user, Django checks the provided credentials (username and password) and verifies them against the
User
model in the database. - The
login()
method creates a session, allowing users to stay logged in, and thelogout()
method destroys the session.
Django's authentication system also includes decorators and mixins for restricting access to views based on user authentication and permissions.
2. How to implement a user registration system?
To implement a user registration system, you can use Django's UserCreationForm
or create a custom registration form. Here's an example using the built-in form:
-
views.py:
python -
register.html:
html
This allows users to create an account with a username
, password
, and password confirmation
. After a successful registration, users can log in with their credentials.
3. What is CSRF, and how do you prevent it in Django?
CSRF (Cross-Site Request Forgery) is an attack where a malicious actor tricks a user into performing an unwanted action on a website where they are authenticated, such as submitting a form.
Django provides built-in protection against CSRF attacks by requiring a special token (CSRF token
) to be included in any POST, PUT, DELETE, or PATCH requests. This token is added automatically to forms using the {% csrf_token %}
template tag.
- To prevent CSRF attacks:
- Always include
{% csrf_token %}
inside any<form>
tag that modifies data (e.g., POST requests). - In views handling POST requests, Django verifies that the CSRF token matches the session.
- Always include
Example:
html
Django will check if the token is present and correct in the request before processing it. If not, it will block the request and raise a 403 Forbidden
error.
4. How to implement role-based authorization in Django?
In Django, you can implement role-based authorization by using permissions and groups. A Group
is a collection of permissions, and you can assign users to different groups based on their role (e.g., Admin, Editor, Viewer). You can also assign individual permissions directly to users.
-
Create Groups and Permissions: Django provides a model called
Group
where you can assign multiple permissions. You can create a group in the Django admin panel or programmatically. -
Assign Permissions to Users: You can assign users to groups, and groups will have certain permissions that allow or restrict access to different views. You can also use Django’s built-in
user.has_perm('permission_name')
method to check if a user has a specific permission. -
Example of using decorators for role-based authorization:
python
In this example, the dashboard
view requires the user to be logged in and have the view_dashboard
permission. You can create and assign permissions in Django's admin interface.
5. How to protect a Django application from SQL injections?
SQL injection is an attack where a user can inject malicious SQL code into queries. Django ORM (Object-Relational Mapping) helps protect your application from SQL injection by automatically escaping parameters and creating safe SQL queries.
- Django ORM: When using Django’s
QuerySet
API (e.g.,filter()
,exclude()
,get()
), the ORM automatically escapes user inputs, so there is no risk of SQL injection.
Example of safe query:
python
Django automatically handles the SQL query safely, even if the input ('john_doe'
) contains special characters.
- Avoid Raw SQL Queries: If you need to execute raw SQL, use Django’s
connection.cursor()
method and ensure that user input is parameterized, so it doesn’t get executed directly in SQL.
Example of using params
for safe raw SQL queries:
python
By using Django's ORM and ensuring all user input is handled properly, you can effectively protect your application from SQL injection attacks.
Сonclusion
In conclusion, preparing for a Django developer interview involves mastering a wide range of topics, from basic concepts like Django’s architecture and ORM to more advanced subjects like security, authentication, and form handling. As you study, focus on understanding the core principles of Django and how to apply them in real-world projects. Practice building applications, working with models and views, and understanding how to manage user authentication and authorization. Make sure to familiarize yourself with common best practices, such as preventing SQL injections and utilizing Django's built-in tools for security. Before your interview, review key topics, try to solve common problems using Django, and be ready to demonstrate your understanding of both fundamental and advanced concepts. Finally, remember that interviews not only test your technical knowledge but also your problem-solving skills, so stay calm, think critically, and communicate your ideas clearly.
Курси по темі
Всі курсиБазовий
Introduction to Python
Python is a high-level, interpreted, general-purpose programming language. Distinguished from languages such as HTML, CSS, and JavaScript, which are mainly utilized in web development, Python boasts versatility across multiple domains, including software development, data science, and back-end development. This course will guide you through Python's fundamental concepts, equipping you with the skills to create your own functions by the conclusion of the program.
Середній
Databases in Python
"Databases in Python" is a hands-on course designed to teach you the fundamentals of working with databases using Python, focusing on the sqlite3 library and SQLAlchemy. You’ll learn how to store, modify, and retrieve data, build efficient queries, and configure databases for your projects. The course covers both SQL basics and the ORM (Object-Relational Mapping) approach, which allows you to interact with databases through Python objects. This course is perfect for beginners looking to deepen their skills in data management, application development, and information handling.
The 80 Top Java Interview Questions and Answers
Key Points to Consider When Preparing for an Interview
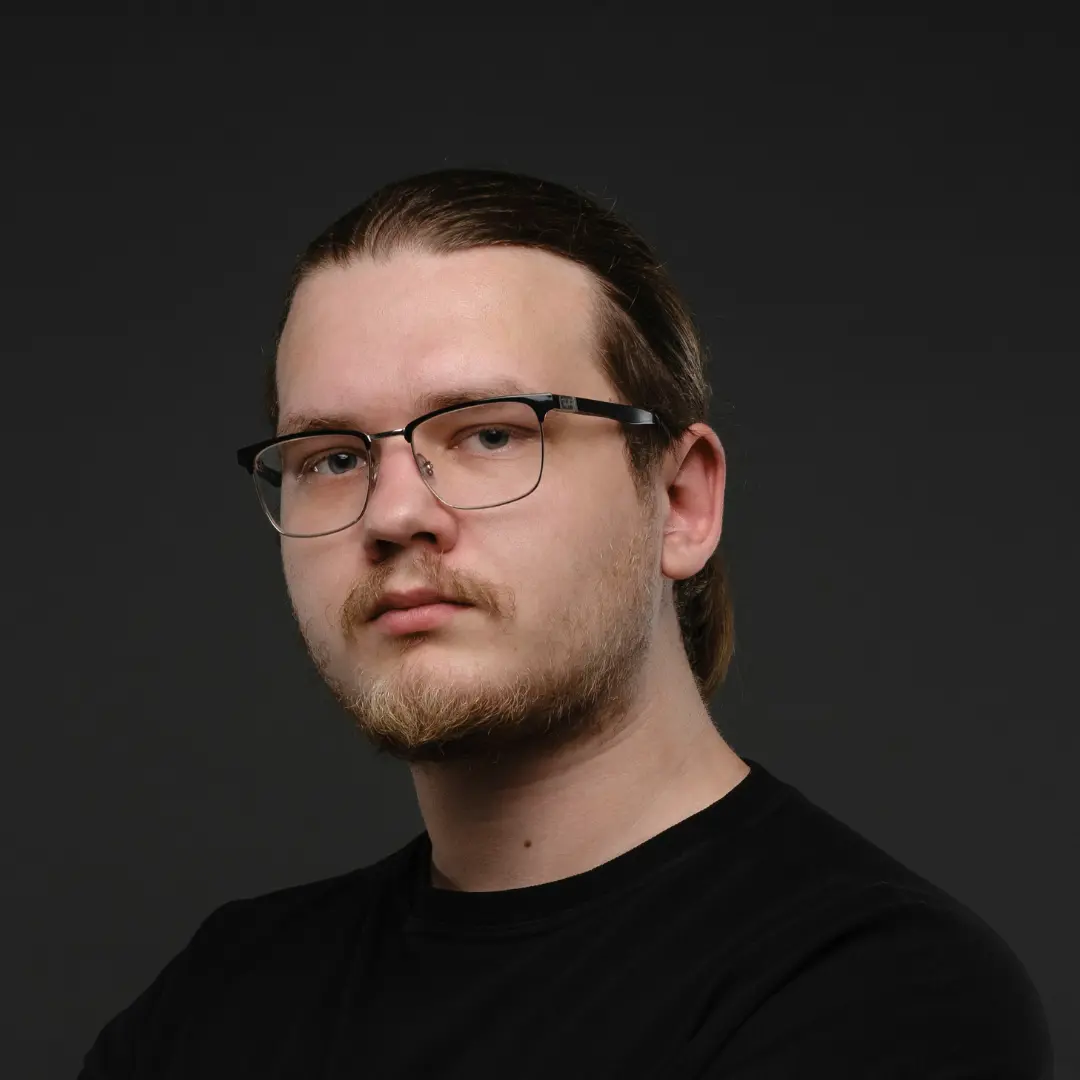
by Daniil Lypenets
Full Stack Developer
Apr, 2024・30 min read
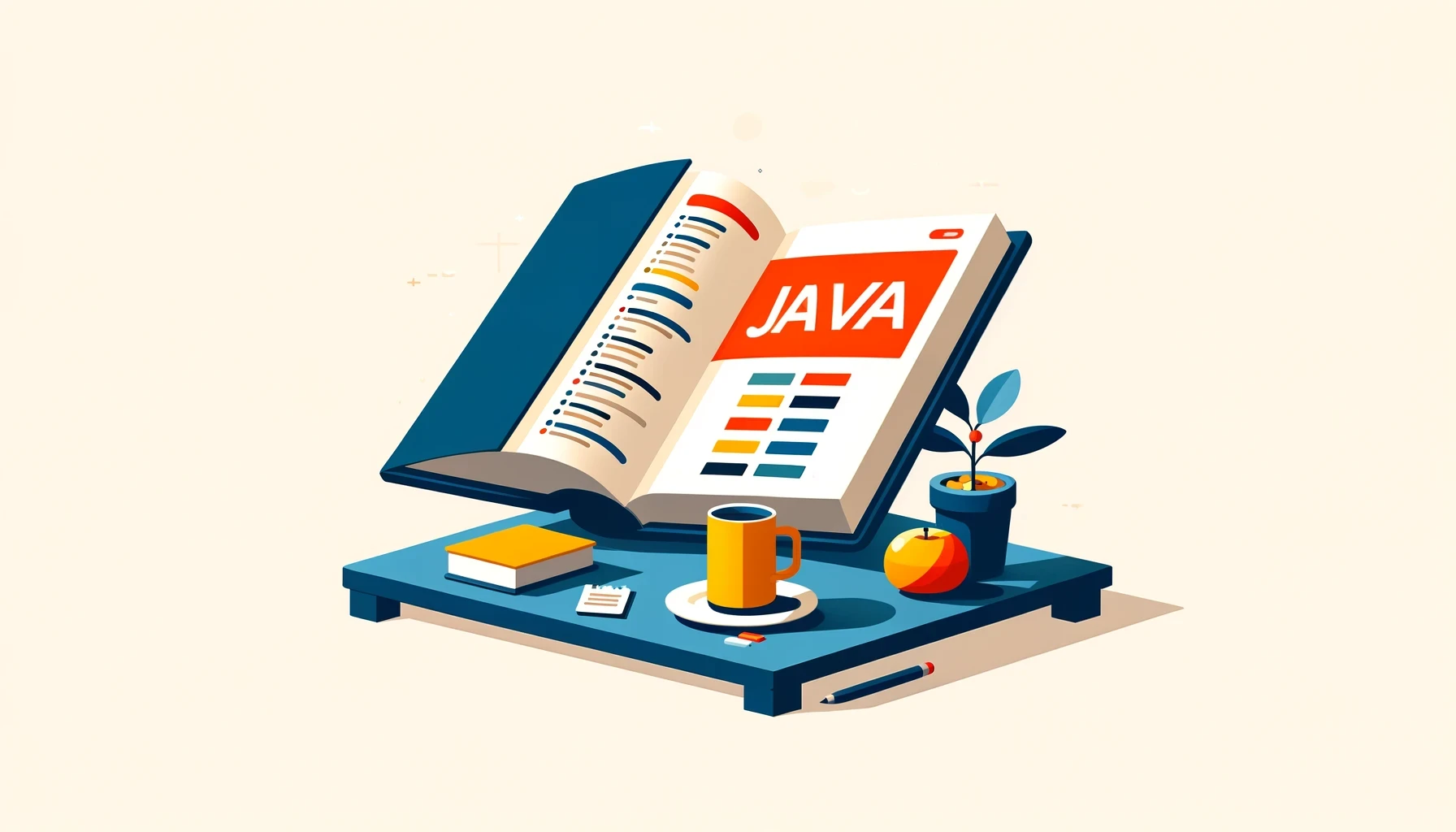
The 50 Top SQL Interview Questions and Answers
For Junior and Middle Developers
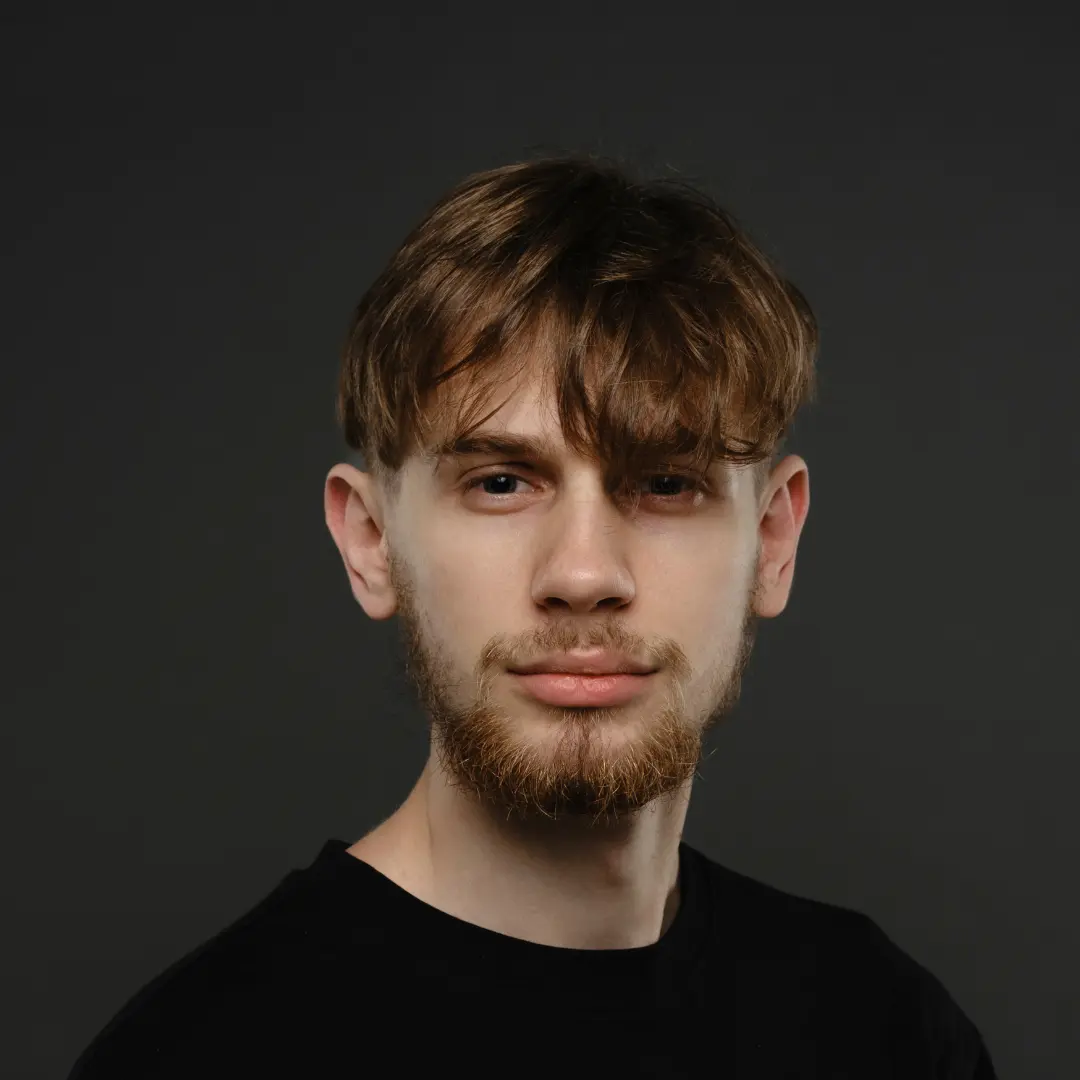
by Oleh Lohvyn
Backend Developer
Apr, 2024・31 min read
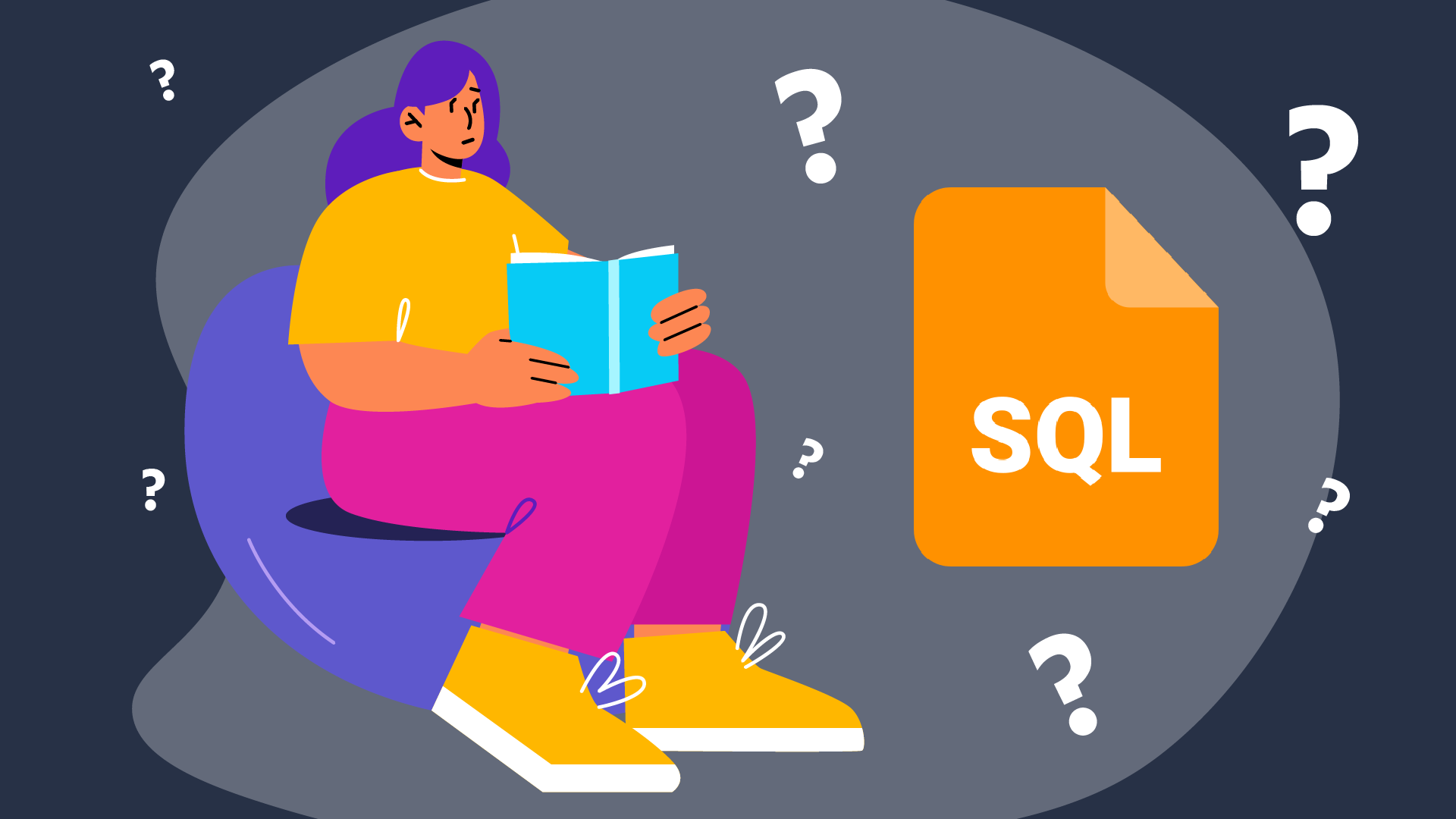
The SOLID Principles in Software Development
The SOLID Principles Overview
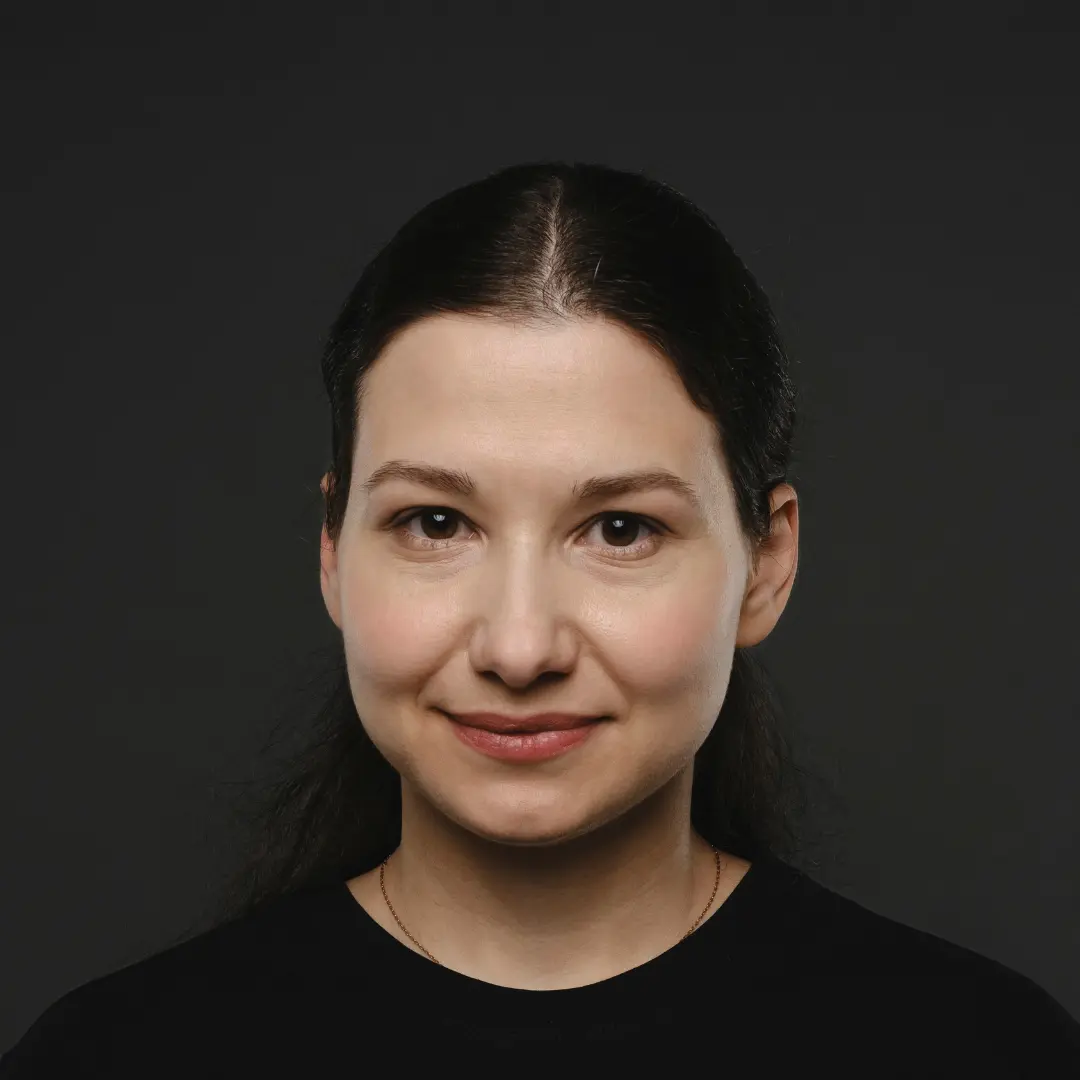
by Anastasiia Tsurkan
Backend Developer
Nov, 2023・8 min read
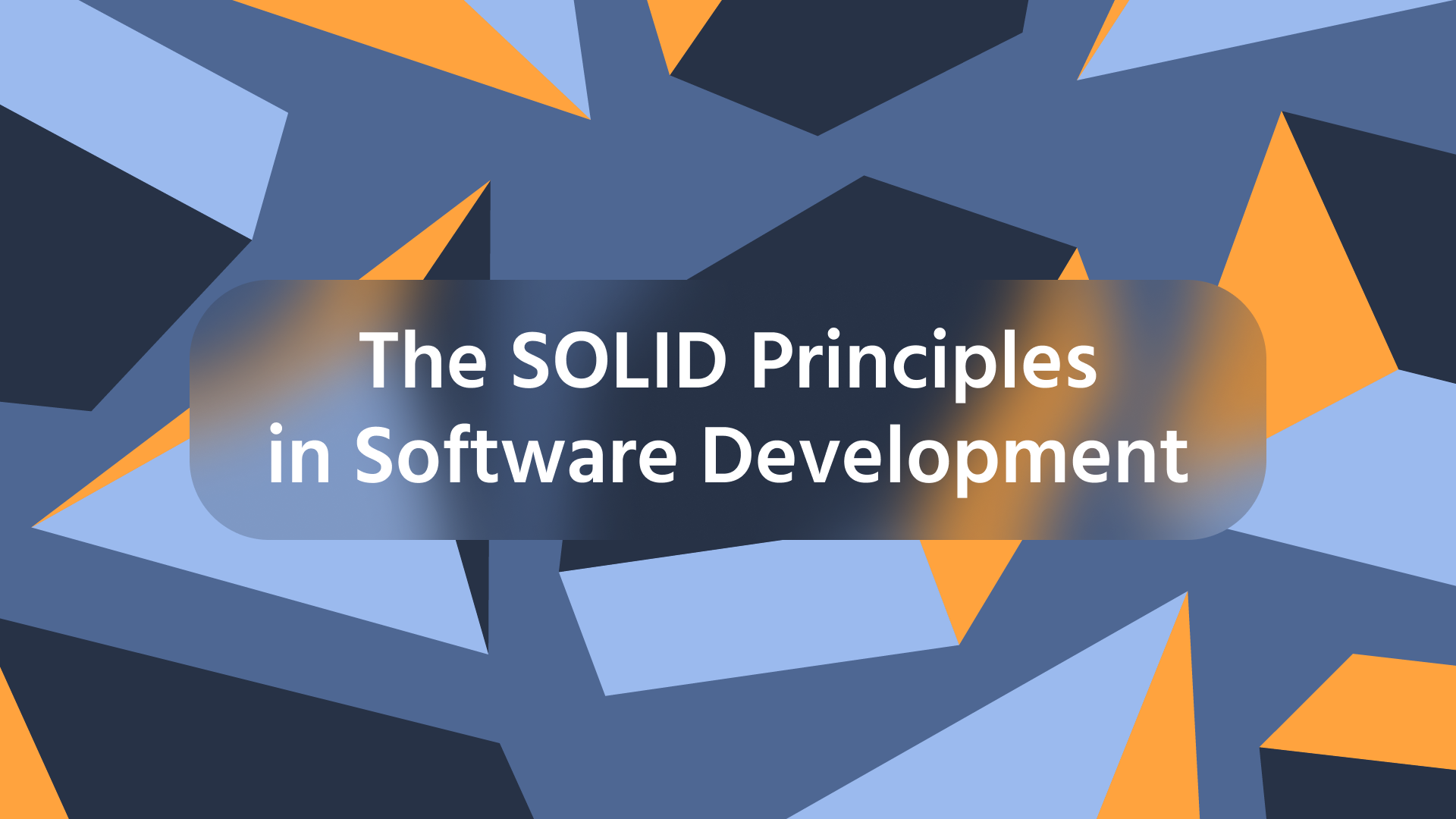
Зміст