Курси по темі
Всі курсиСередній
React Mastery
Take your web development skills to the next level with the course on building modern web applications using React. Learn to create dynamic user interfaces, efficiently manage state and data, and leverage the latest technologies and best practices in web development. Join now and unlock the full potential of React to create highly interactive and engaging web apps that meet the demands of today's users.
Просунутий
React Router Essentials
Delve into the world of routing within React applications and learn how React Router streamlines the management of navigation, URL state, and dynamic component rendering, all in response to the current URL.
Ultimate React Router Guide
React Router in React
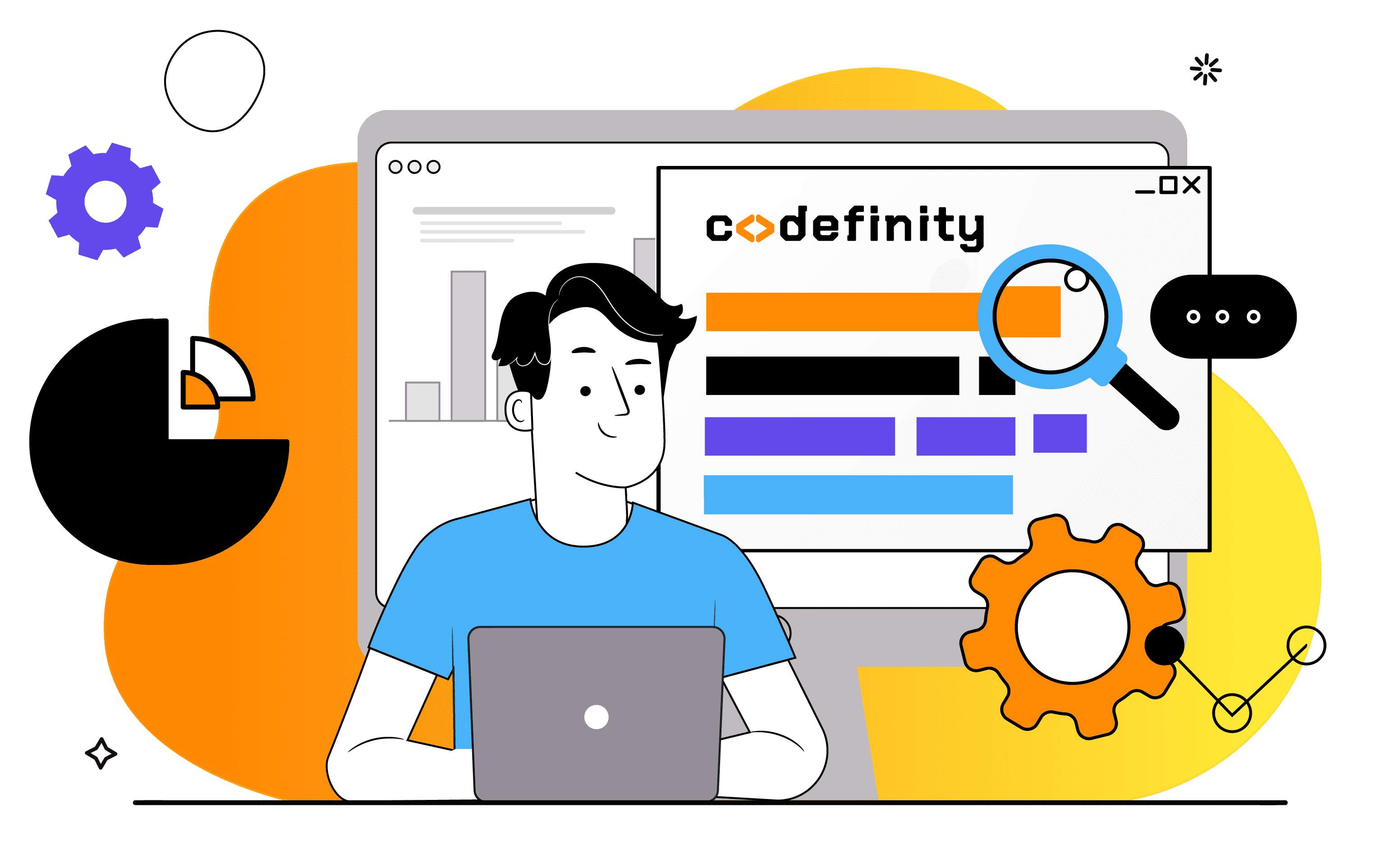
One of the defining features of web applications is the ability to navigate to specific parts of the application using URLs. In this guide, we'll explore routing in React applications and how React Router makes it easy to handle navigation, manage URL states, and display different components based on the current URL.
The Role of URLs in Web Applications
URLs, or Uniform Resource Locators, play a pivotal role by functioning as digital addresses that guide users to specific sections or pages within your application. URLs like street addresses enable users to bookmark, share, and revisit specific views or functionalities within your web application. Understanding the anatomy of URLs is crucial for building robust routing systems.
A standard URL comprises several essential components:
- Protocol: This component determines how data is transmitted and can be represented by protocols like HTTP or HTTPS. Example:
https://
(Protocol) - Host: The host component identifies the server where your application resides. For example, it might appear as "mysite.com." Example:
https://mysite.com
(Host) - Path: This part of the URL specifies the location within your application, such as "/books/e3q76gm9lzk." Example:
https://mysite.com/books/e3q76gm9lzk
(Path) - URL Parameters: These parameters can be either dynamic or static and serve the purpose of passing data via URLs. They can be structured like "?category=adventure&status=unread." Example:
https://mysite.com/books/e3q76gm9lzk?category=adventure&status=unread
(URL Parameters) - Query String: Beginning with a "?", the query string comprises key-value pairs separated by "&". For instance, "category=adventure." Example:
https://mysite.com/books/e3q76gm9lzk?category=adventure&sort=price
(Query String) - Anchor (Hash): The anchor component, denoted by a "#" symbol, is employed for navigating to specific sections within a page. It often appears as "#comments." Example:
https://mysite.com/books/e3q76gm9lzk#comments
(Anchor)
React Router: A Routing Library for React
While React provides the foundational tools for building user interfaces and managing application states, it lacks a built-in routing solution. This is where React Router steps in. React Router is a widely adopted library crafted explicitly to address the routing needs of React applications. It provides a comprehensive suite of components and hooks dedicated to simplifying routing, managing navigation history, and streamlining component rendering based on the current URL.
In essence, React Router seamlessly integrates with your React application, allowing you to define routes and map them to specific components. It ensures that the right components are rendered when users navigate to different URLs within your application. This powerful library empowers developers to create smooth and structured navigation experiences, enhancing the overall usability of React applications.
Run Code from Your Browser - No Installation Required
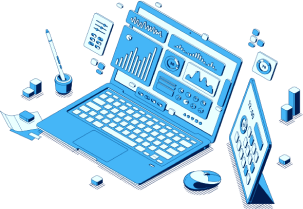
Getting Started with React Router
To begin using React Router in your React application, you'll first need to install the react-router-dom
package. Here's a step-by-step guide on how to set up routing:
- Install
react-router-dom
:
- Wrap your entire application with the
<BrowserRouter>
component:
Wrapping your application with <BrowserRouter>
is essential to enable routing functionality throughout your app.
- Define routes using the
<Route>
component. For example:
You can define routes by using the <Route>
component. In this example, when the URL path matches "/about", it renders the About
component.
- Use links provided by React Router (
<Link>
or<NavLink>
) to navigate between pages without reloading the entire page:
React Router offers convenient components like <Link>
and <NavLink>
for navigation within your application. These components allow users to move between pages seamlessly without reloading the entire page.
Dynamic Routing with URL Parameters
Working with dynamic data and routing to specific resources, such as individual blog posts, is a common requirement in many applications. Rather than creating separate routes for each resource, React Router allows you to use URL parameters to define a dynamic pattern. For example:
In this example, :postId
serves as a placeholder for a dynamic value that can represent any specific blog post. React Router captures this value from the URL, making it accessible for your components.
You can access the parameter's value using the useParams
hook like this:
This approach simplifies fetching data based on the parameter value and rendering the appropriate content. With dynamic routing and URL parameters, you can create versatile and data-driven applications that respond dynamically to user interactions and provide a seamless user experience.
Organizing Content with Nested Routes
Nested routes are a powerful way to hierarchize and structure your React application. For example, if you have an "About" page with subpages like "Mission," "Team," and "Reviews," you can nest routes as follows:
In this example, the "About" page is the parent route, and "Mission", "Team", and "Reviews" are child routes. When a user navigates to "/about", the content of the "About" component is displayed. However, if they navigate to "/about/mission", the content of the "Mission" component is rendered within the "About" component.
Shared Layouts and Index Routes
You can implement shared layouts to prevent duplicating common layout elements across multiple pages in your React application. These layouts typically include headers, navigation menus, and footers that remain consistent across different pages. React Router offers the ability to create index routes responsible for rendering their child routes and sharing a common layout.
For instance, you can establish a shared layout for your entire application like this:
In this example, the <SharedLayout>
component serves as the common layout for your application. When a user navigates to the root URL ("/"), the <SharedLayout>
component is rendered, and the <Home>
component is displayed within it as the index route.
Start Learning Coding today and boost your Career Potential
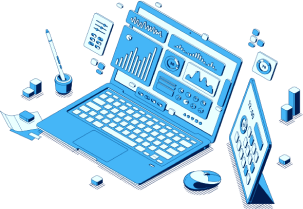
Conclusion
Routing is a critical aspect of modern web development, and React Router empowers you to manage it effectively in your React applications. Armed with this knowledge, you can create web applications that provide a seamless and intuitive user experience.
FAQs
Q: What is React Router, and why do I need it in my React application?
A: React Router is a library designed for handling routing in React applications. It's essential because it enables navigation, URL management, and dynamic components rendering based on the current URL.
Q: What are URL parameters, and how do they work in React Router?
A: URL parameters in React Router allow you to capture dynamic values from the URL and use them in your components. For example, you can define a route like "/users/:userId" and then access the userId
parameter using the useParams
hook.
Q: How can I create shared layouts for my React application using React Router?
A: Shared layouts can be created in React Router by using index routes. An index route renders its child routes and provides a common layout. For instance, you can define a layout for your entire application using a route like "/"
and render child routes within it. This approach ensures that common layout elements like headers and footers are shared across multiple pages.
Q:Are nested routes the same as child routes in React Router?
A: Yes, nested routes and child routes refer to the same concept in React Router. They allow you to define routes, creating a hierarchical structure for your application.
Q: What advanced routing techniques can I explore with React Router?
A: React Router provides advanced routing features, including route guards, lazy loading, and route transitions. Route guards allow you to control access to specific routes based on conditions. Lazy loading enables you to load components asynchronously, improving performance. Route transitions allow smooth transitions between different pages in your application.
Q: Is React Router the only routing library available for React applications?
A: While React Router is the most widely used routing library for React, other alternatives are available, such as Reach Router. The choice of routing library depends on your specific project requirements and preferences.
Курси по темі
Всі курсиСередній
React Mastery
Take your web development skills to the next level with the course on building modern web applications using React. Learn to create dynamic user interfaces, efficiently manage state and data, and leverage the latest technologies and best practices in web development. Join now and unlock the full potential of React to create highly interactive and engaging web apps that meet the demands of today's users.
Просунутий
React Router Essentials
Delve into the world of routing within React applications and learn how React Router streamlines the management of navigation, URL state, and dynamic component rendering, all in response to the current URL.
useState Hook in React with TypeScript
Guide to Using useState in React with TypeScript
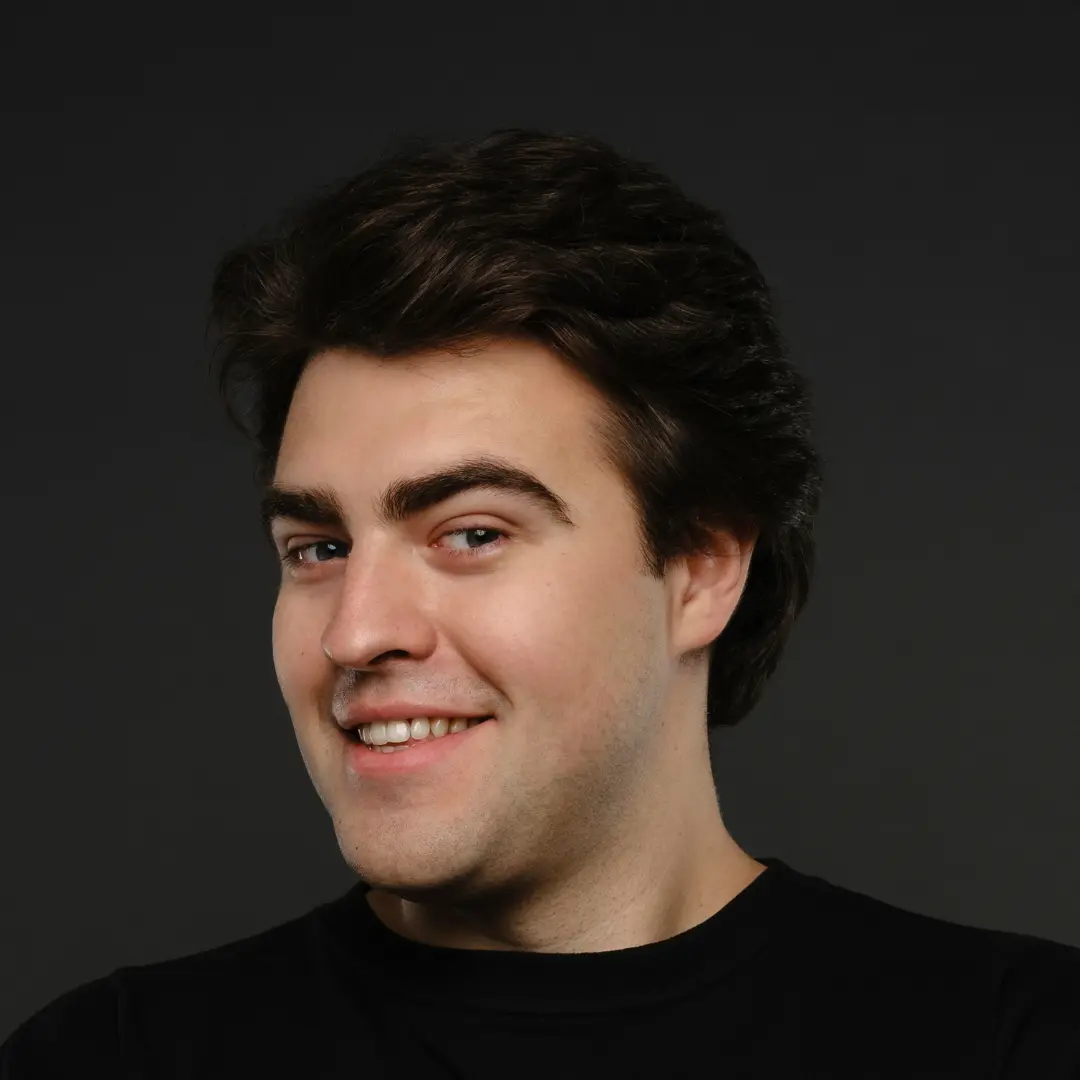
by Oleh Subotin
Full Stack Developer
May, 2024・9 min read
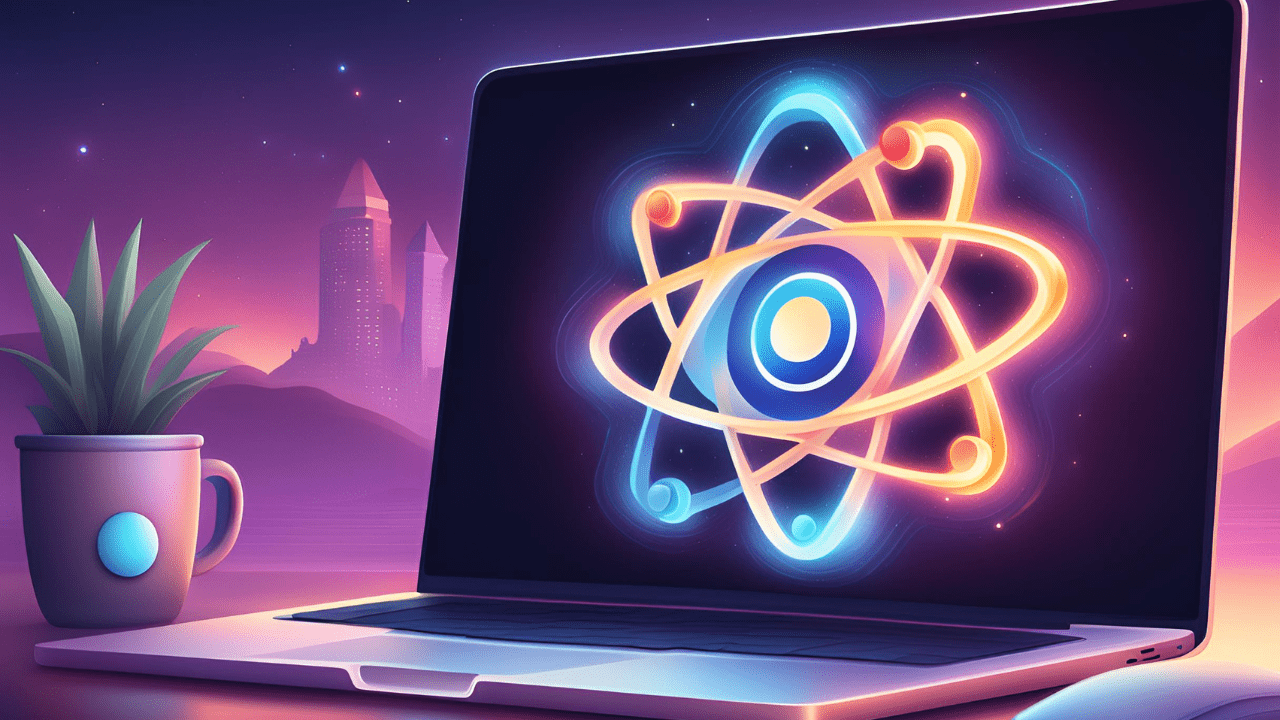
Accidental Innovation in Web Development
Product Development
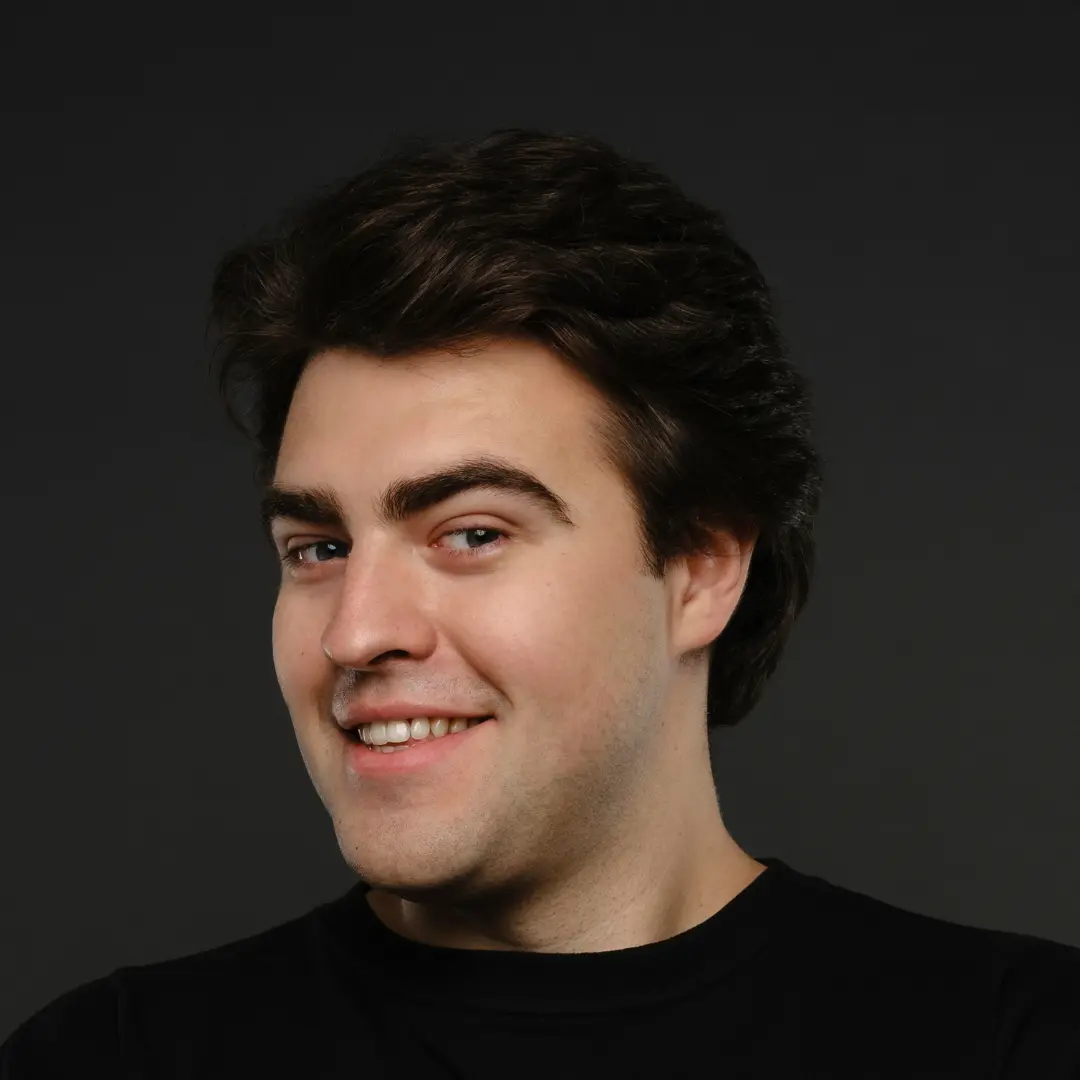
by Oleh Subotin
Full Stack Developer
May, 2024・5 min read
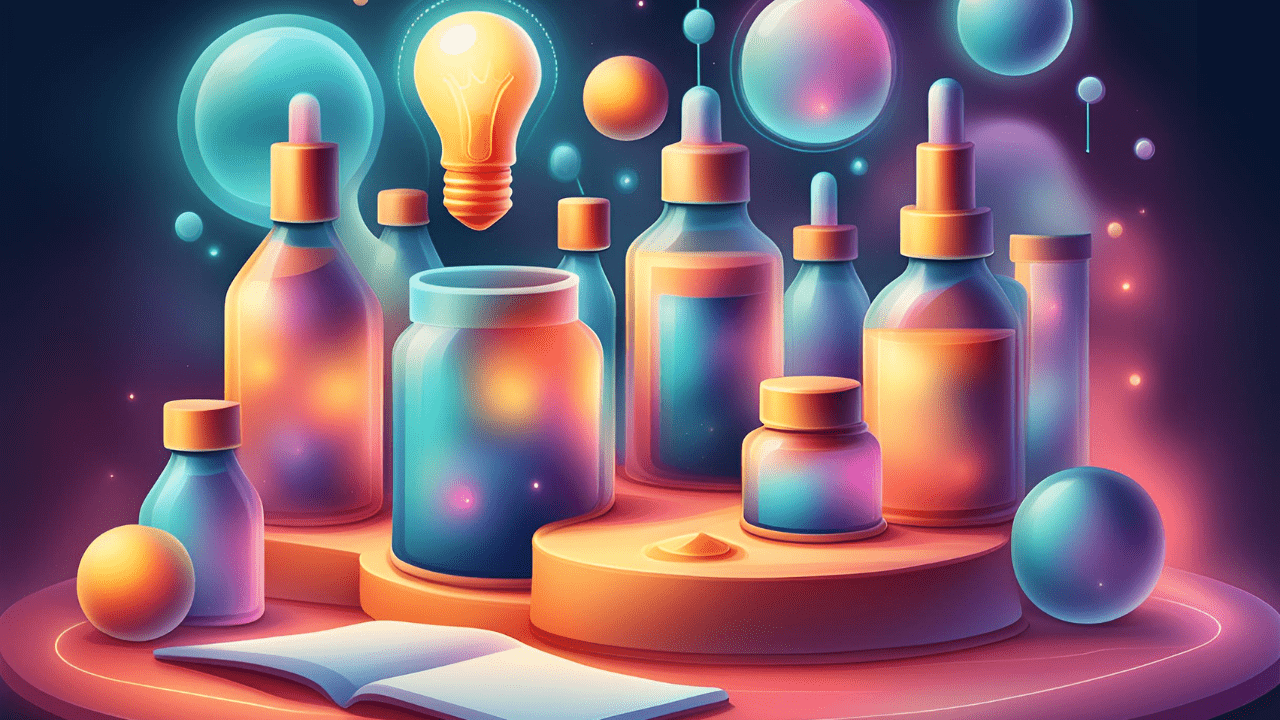
Navigating the YouTube Influencer Maze in Your Tech Career
Staying Focused and Disciplined Amidst Overwhelming Advice
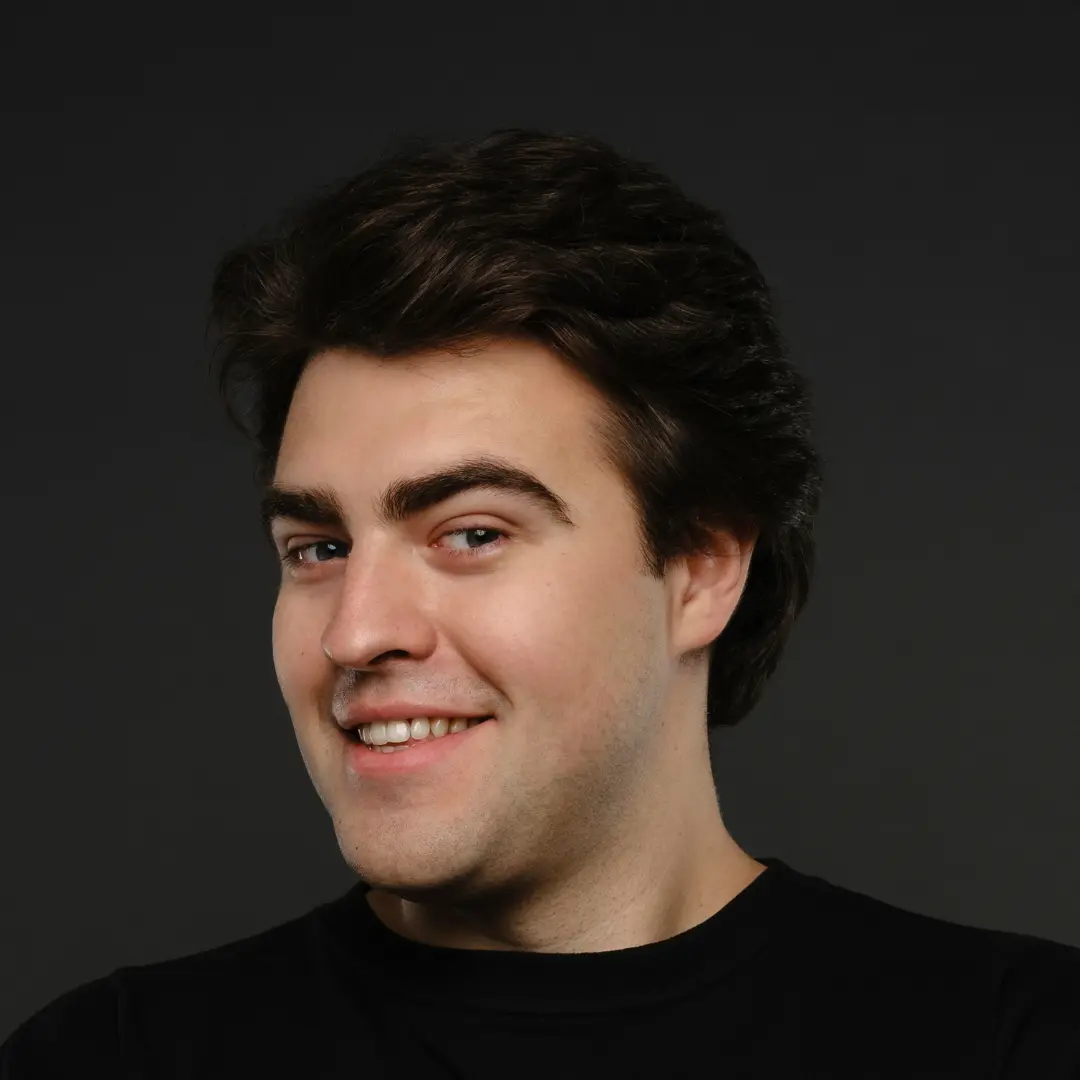
by Oleh Subotin
Full Stack Developer
Jul, 2024・5 min read
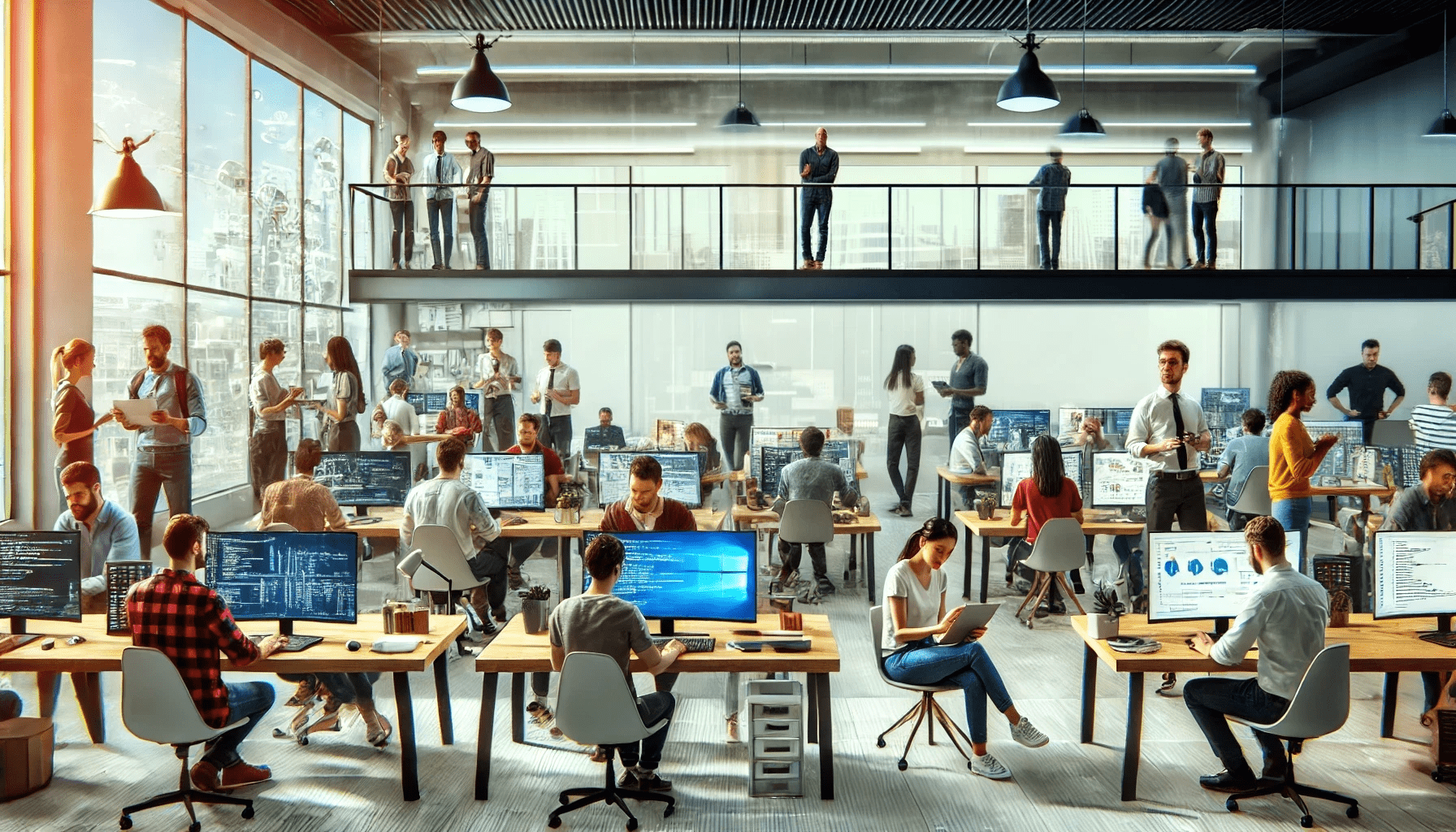
Зміст