Курси по темі
Всі курсиБазовий
Introduction to JavaScript
Learn the fundamentals of JavaScript, the backbone of dynamic web development. Discover essential concepts like syntax, variables, data types, and operators. Explore how to use conditional statements, loops, and functions to create interactive and efficient programs. Master the building blocks of JavaScript and lay the groundwork for more advanced programming skills.
Середній
JavaScript Data Structures
Discover the foundational building blocks of JavaScript. Immerse yourself in objects, arrays, and crucial programming principles, becoming proficient in the fundamental structures that form the basis of contemporary web development.
Understanding the Spread and Rest Operators in JavaScript
Spread and Rest Operators
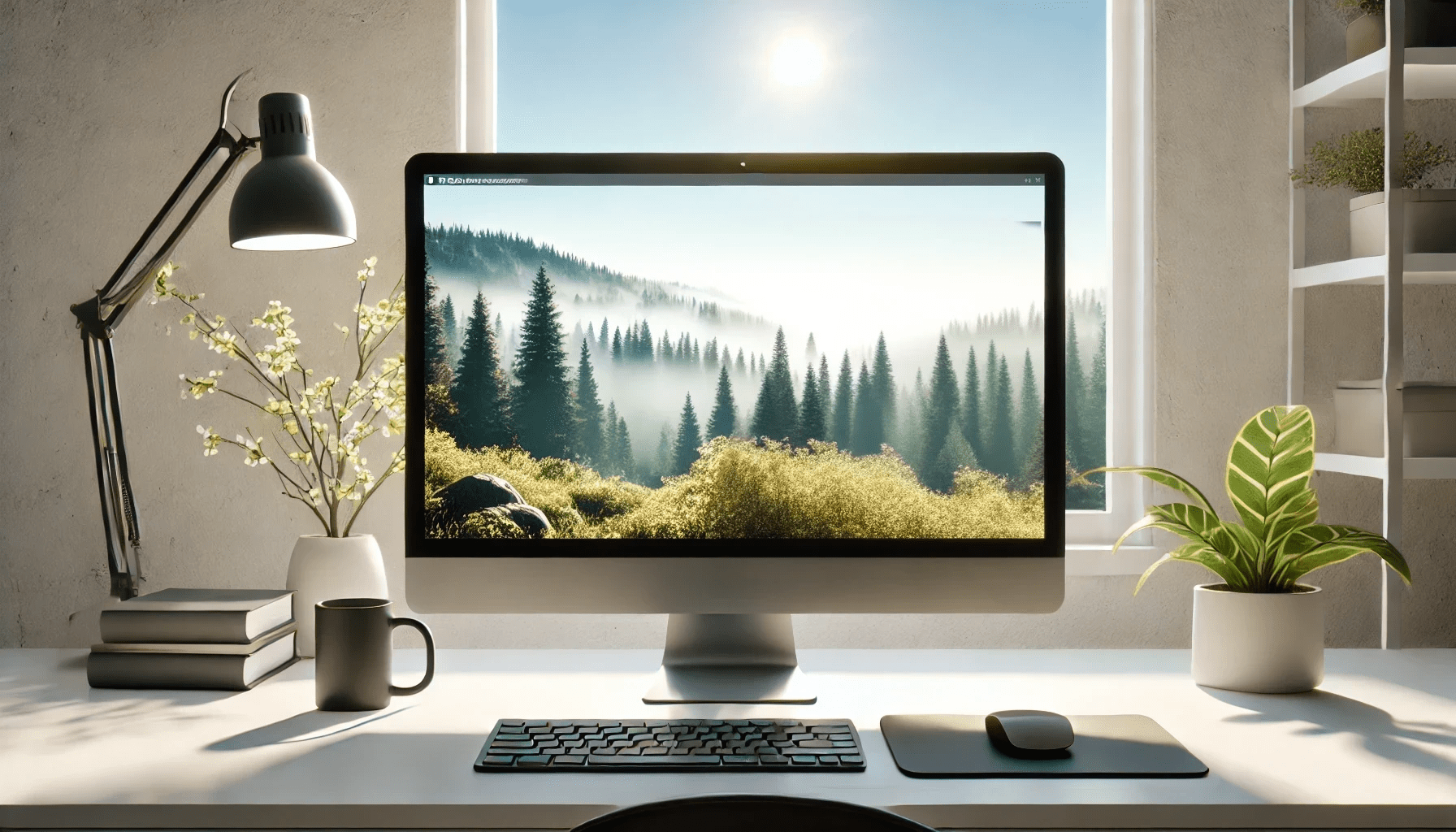
JavaScript introduced two powerful operators in ES6, the spread (...
) and rest (...
) operators. While they look identical, their purposes are distinct. Both of these operators simplify working with arrays, objects, and function arguments, making code more readable and expressive. This article will explore how to use both spread and rest operators in various contexts.
What is the Spread Operator?
The spread operator (...
) allows you to spread the elements of an array or the properties of an object into another array or object. Essentially, it breaks apart iterable elements (like arrays or objects) and spreads their contents.
Spreading Arrays
One of the most common uses of the spread operator is to clone or merge arrays.
Cloning an Array:
Here, the spread operator copies all elements of originalArray
into clonedArray
.
Merging Arrays:
Spreading Objects
Similarly, you can use the spread operator to clone or merge objects.
Cloning an Object:
Merging Objects:
Using Spread in Function Calls
The spread operator can also be used to spread elements as arguments in a function call.
This is especially useful when you have an array and want to pass its values as individual arguments to a function.
Run Code from Your Browser - No Installation Required
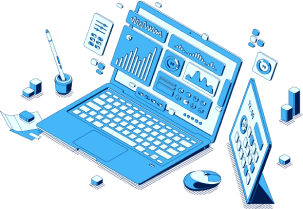
What is the Rest Operator?
The rest operator (...
) does the opposite of the spread operator: it gathers multiple elements and consolidates them into a single array or object. This is often used in function parameters to handle variable numbers of arguments.
Rest Parameters in Functions
The most common use of the rest operator is in function definitions, where it allows you to collect all remaining arguments into an array.
Rest in Function Parameters:
In this example, ...numbers
collects all arguments passed to the sum
function into an array, making it possible to work with them using array methods.
Combining Rest with Regular Parameters
You can mix regular parameters with rest parameters in function definitions.
Here, name
and age
are regular parameters, while ...otherInfo
collects any remaining arguments into an array.
Rest in Destructuring
The rest operator can also be used when destructuring arrays or objects.
Array Destructuring:
Object Destructuring:
Key Differences Between Spread and Rest Operators
While both the spread and rest operators use the same ...
syntax, they have different purposes:
Operator | Description | Context |
---|---|---|
Spread Operator | Expands an array or object into individual elements or properties. | Used in expression contexts (like passing arguments or merging arrays/objects). |
Rest Operator | Gathers individual elements or properties into an array or object. | Used in assignment contexts (like function parameters or destructuring). |
Conclusion
The spread and rest operators in JavaScript provide a flexible, clean way to work with arrays, objects, and function arguments. By learning how to effectively use these operators, you can make your code more concise and expressive.
While they share the same syntax, it's essential to understand their different use cases: the spread operator is used to unpack elements, while the rest operator gathers elements together. These operators are incredibly powerful, and mastering them can significantly enhance your JavaScript coding skills.
Start Learning Coding today and boost your Career Potential
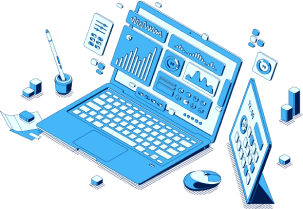
FAQs
Q: What is the spread operator in JavaScript?
A: The spread operator (...
) is used to expand an array or object into its individual elements or properties.
Q: What is the rest operator in JavaScript?
A: The rest operator (...
) is used to collect multiple elements or properties into an array or object, often in function parameters.
Q: How do I use the spread operator to clone an array?
A: You can clone an array by writing const newArray = [...originalArray];
.
Q: Can I use both spread and rest operators with objects?
A: Yes, both operators can be used with objects to clone, merge, or collect properties.
Q: What is the key difference between the spread and rest operators?
A: The spread operator expands elements, while the rest operator gathers elements into an array or object.
Курси по темі
Всі курсиБазовий
Introduction to JavaScript
Learn the fundamentals of JavaScript, the backbone of dynamic web development. Discover essential concepts like syntax, variables, data types, and operators. Explore how to use conditional statements, loops, and functions to create interactive and efficient programs. Master the building blocks of JavaScript and lay the groundwork for more advanced programming skills.
Середній
JavaScript Data Structures
Discover the foundational building blocks of JavaScript. Immerse yourself in objects, arrays, and crucial programming principles, becoming proficient in the fundamental structures that form the basis of contemporary web development.
Accidental Innovation in Web Development
Product Development
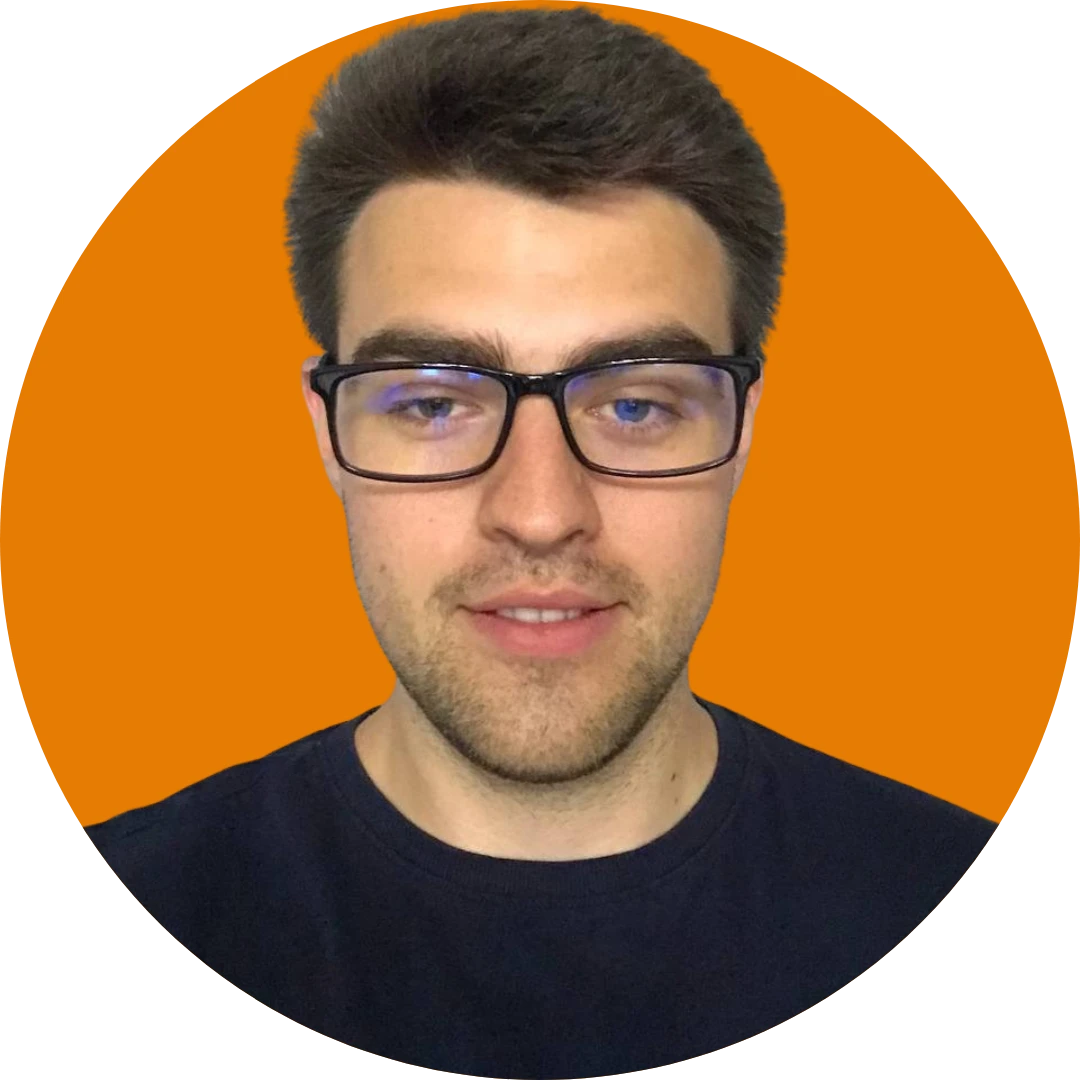
by Oleh Subotin
Full Stack Developer
May, 2024・5 min read
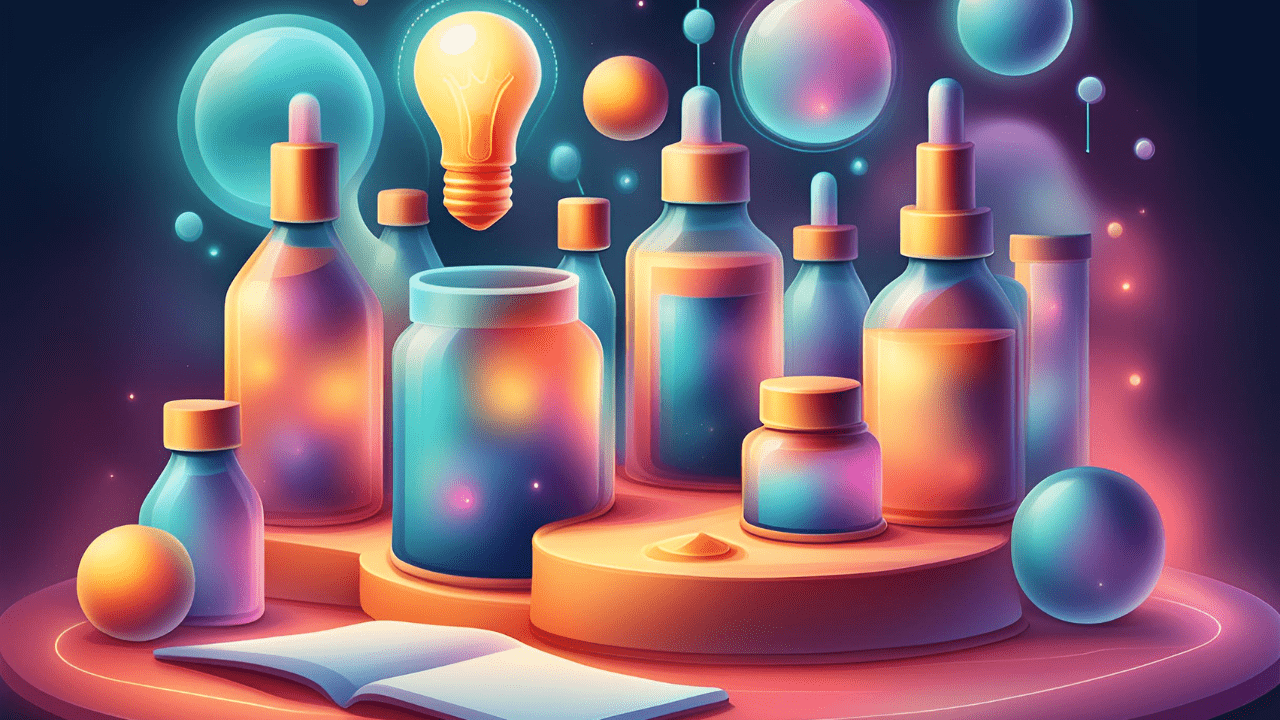
Navigating the YouTube Influencer Maze in Your Tech Career
Staying Focused and Disciplined Amidst Overwhelming Advice
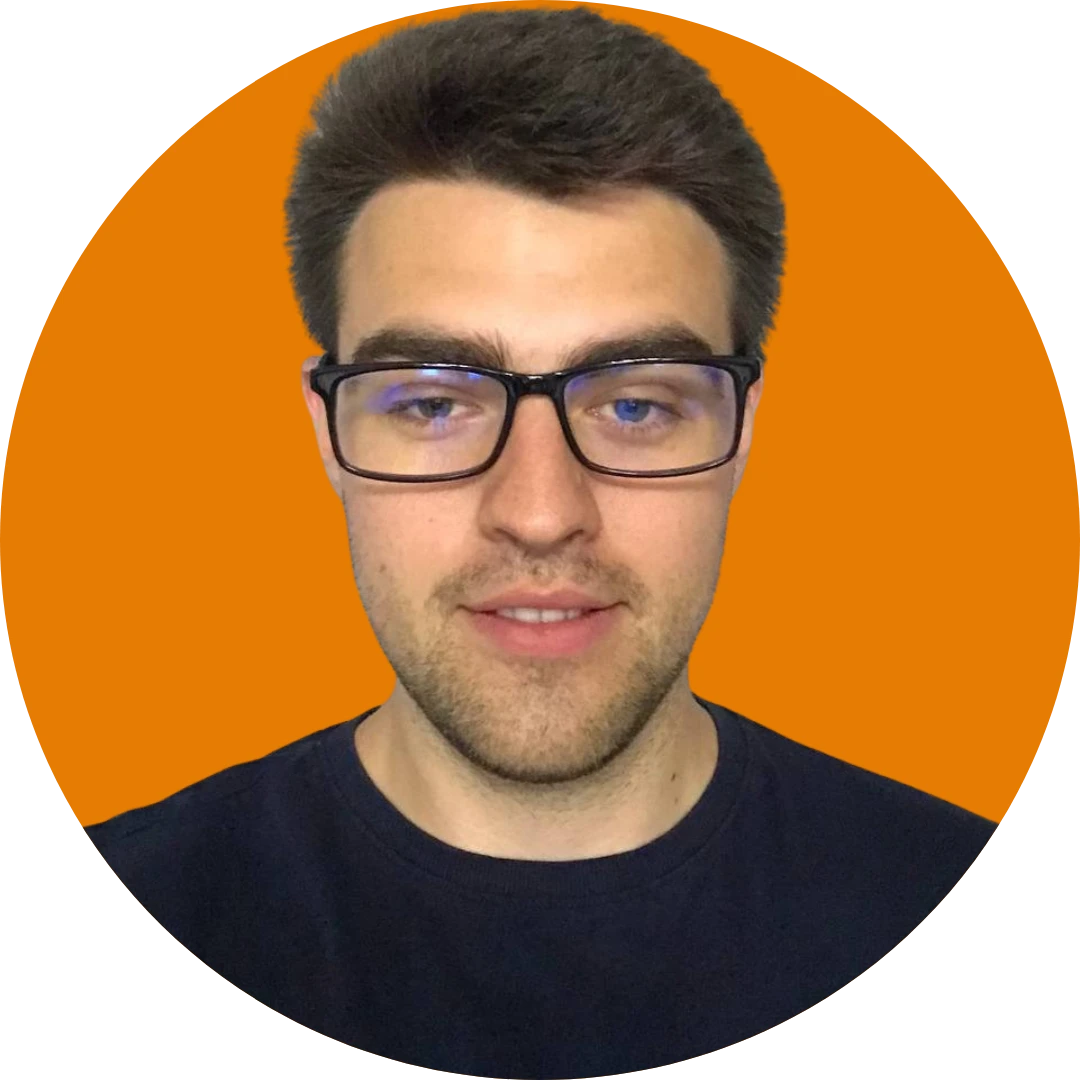
by Oleh Subotin
Full Stack Developer
Jul, 2024・5 min read
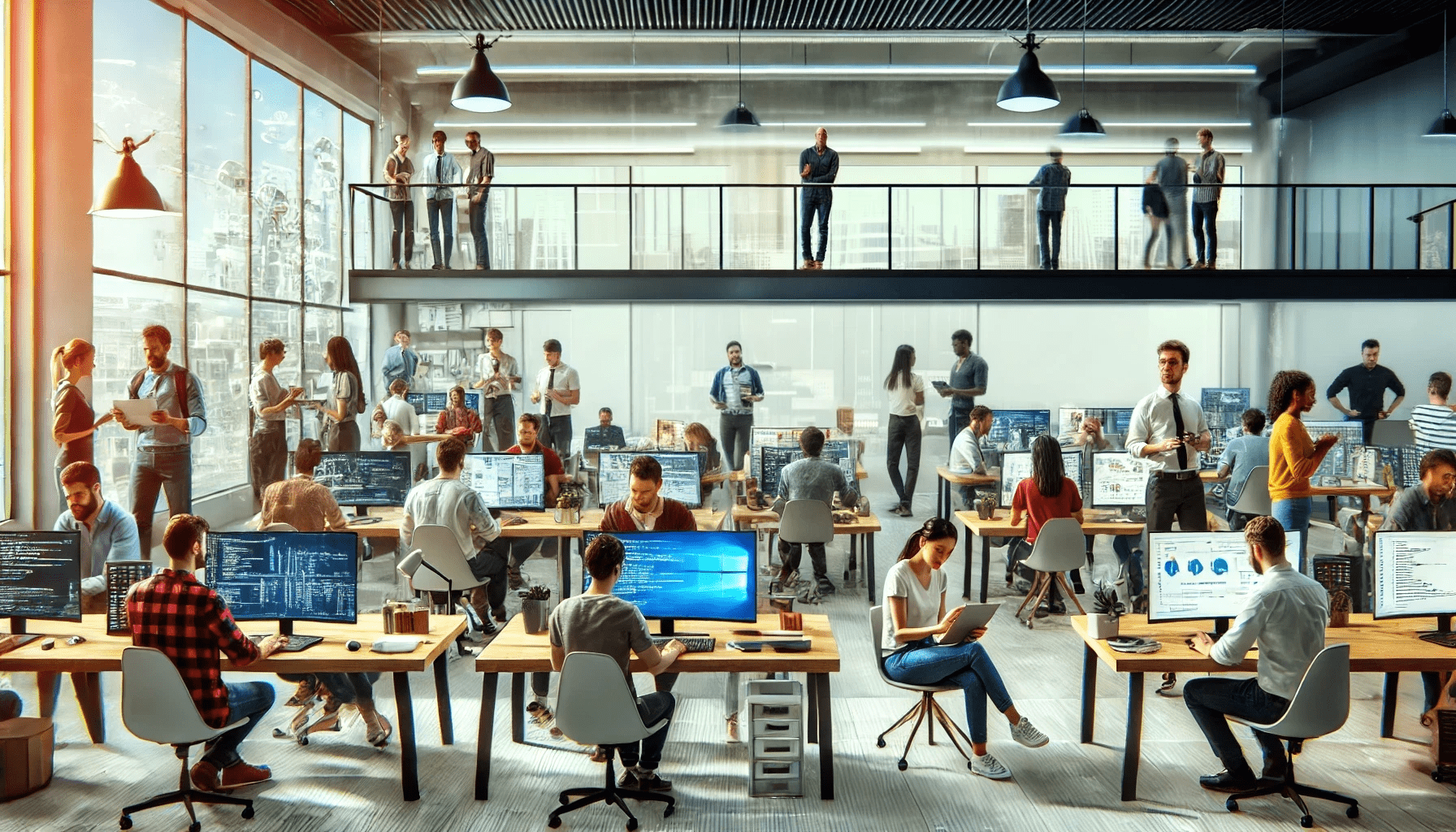
Managing JavaScript Packages with npm
Node Package Manager
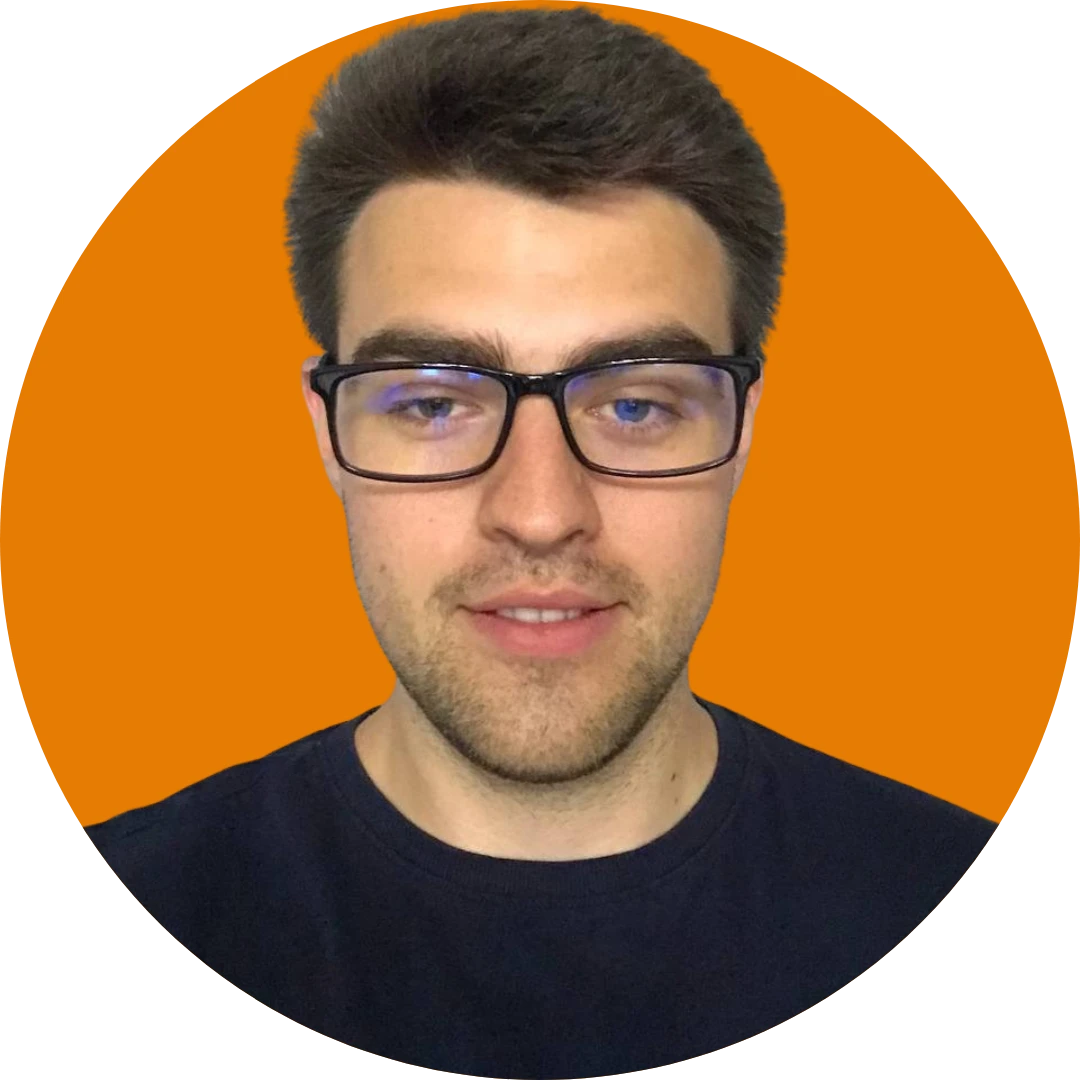
by Oleh Subotin
Full Stack Developer
Jun, 2024・4 min read
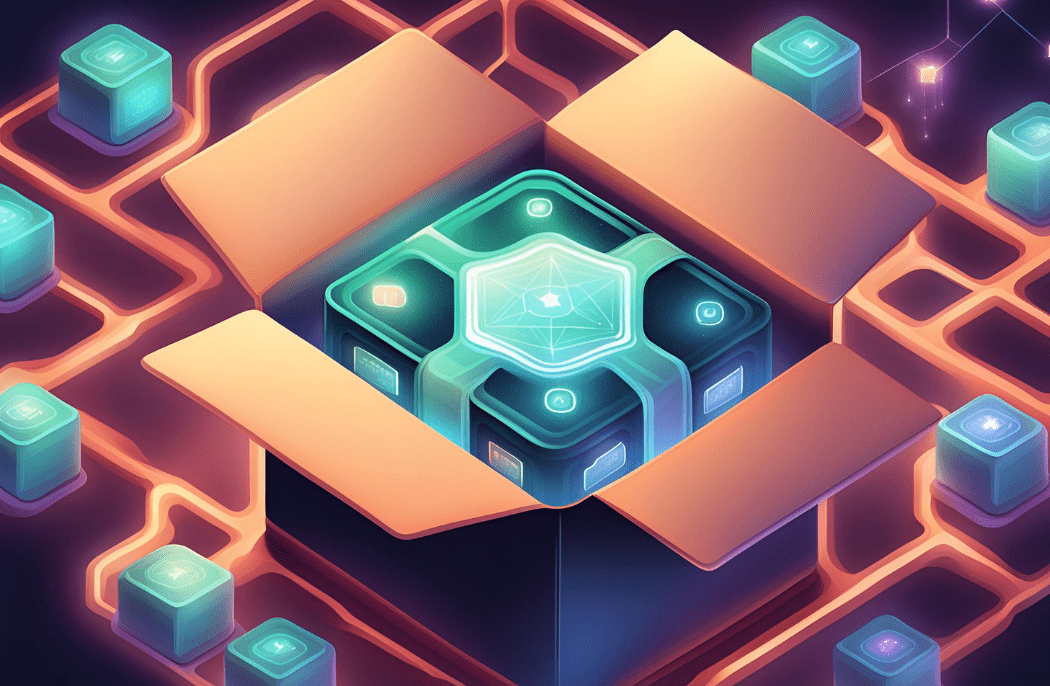
Зміст