Related courses
See All CoursesAchieving Peak Performance with Python Using Optimization Techniques
Unlocking Efficiency in Python Code
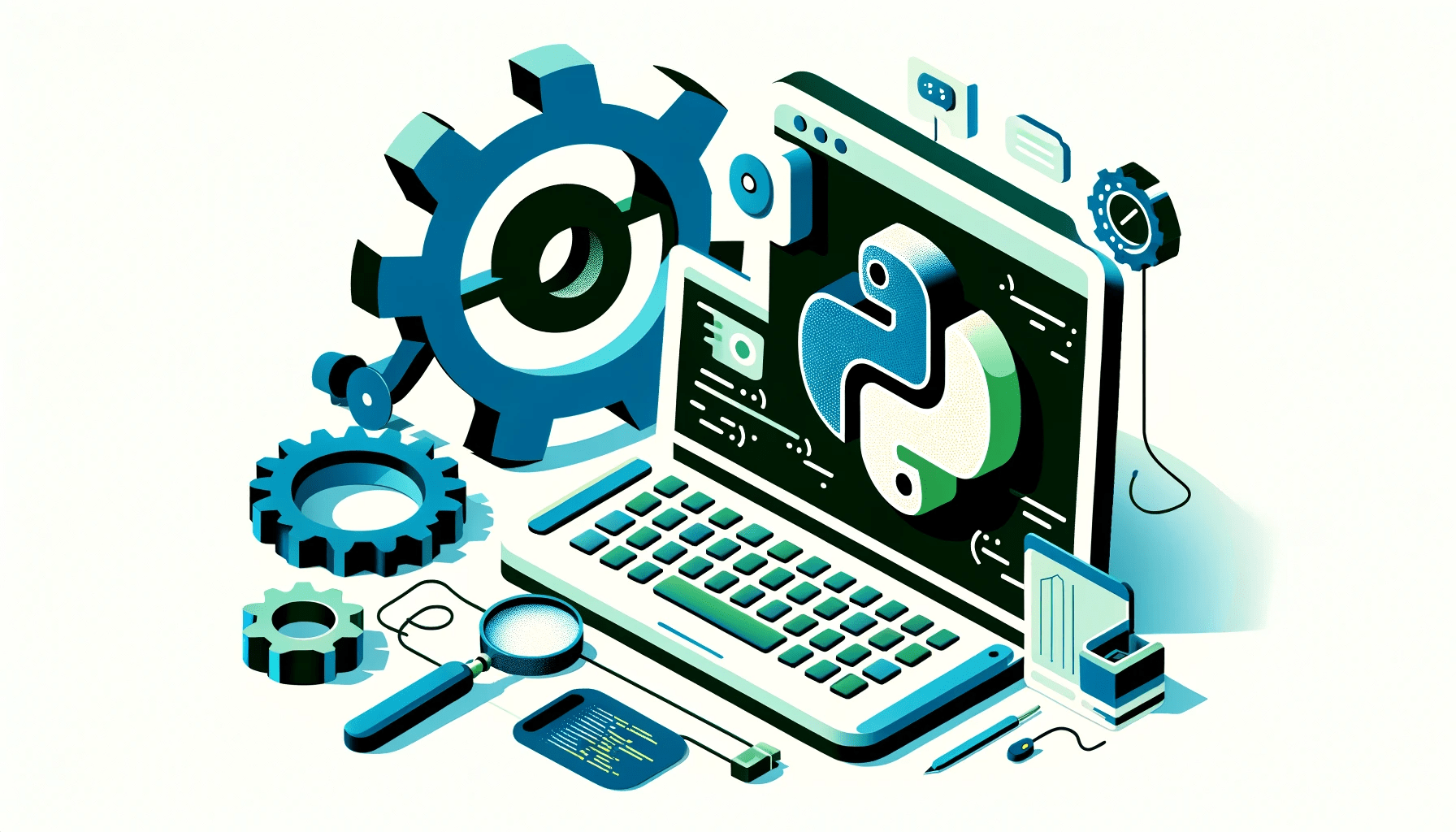
Python's popularity stems from its readability and simplicity, making it a favorite among developers for a wide range of applications. However, writing efficient Python code is essential to achieve peak performance, especially in data-intensive or time-sensitive applications. This article explores various optimization techniques to enhance the efficiency and speed of your Python code.
Understanding Python's Performance Characteristics
To optimize Python code effectively, it's crucial to understand the language's inherent performance characteristics:
-
Interpreted Nature: Python is an interpreted language, meaning the Python interpreter reads and executes code line by line. This can lead to slower execution compared to compiled languages like C++ or Java. However, Python's development speed and ease of use often compensate for this. Just-In-Time (JIT) compilers like PyPy can improve execution speed by compiling parts of the code to machine code dynamically.
-
Dynamic Typing: Python is dynamically typed, allowing you to write more flexible code. However, this means that the type of a variable can change over time, and type checking happens at runtime, which can add overhead.
-
Global Interpreter Lock (GIL): The GIL is a mutex that allows only one thread to execute in the interpreter at once. This can be a bottleneck in multi-threaded CPU-bound programs, as it prevents the concurrent execution of Python bytecode.
Profiling Python Code
Effective optimization starts with identifying bottlenecks using profiling tools:
-
cProfile and line_profiler: These tools help you understand the time spent in each part of your code. They are invaluable for identifying slow functions and lines of code.
-
Memory Profilers: Tools like
memory_profiler
help track memory usage, which is crucial for optimizing memory-intensive applications.
python
This code snippet demonstrates using cProfile
to profile my_function()
. It provides insights into the function call counts and execution times.
Optimization Techniques
Now, let's delve deeper into various optimization techniques which you can use to make your code more efficient in terms of speed and memory as well.
Run Code from Your Browser - No Installation Required
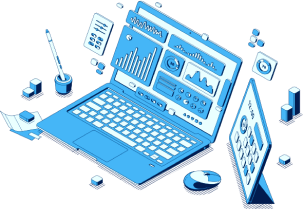
Efficient Data Structures
Choosing the right data structure is a fundamental step in optimizing Python code:
-
Lists vs. Sets: Lists are ordered collections, while sets are unordered collections of unique elements. Sets offer O(1) complexity for membership tests, making them faster than lists for this purpose.
-
Dictionaries: They are ideal for key-value storage with O(1) average-case complexity for lookups.
Algorithm Optimization
Algorithm selection significantly impacts performance, especially in terms of time and space complexity:
-
Sorting Algorithms: Python's built-in
sorted()
function and the.sort()
method on lists are highly optimized and should be used instead of writing custom sorting logic. -
Search Algorithms: For large datasets, algorithms like binary search (O(log n)) are more efficient than linear search (O(n)).
Use of Built-in Functions and Libraries
Pythonβs built-in functions and standard libraries are optimized for performance:
-
Built-in Functions: Functions like
map()
,filter()
, list comprehensions, and generator expressions are usually faster and more memory-efficient than their manual equivalents. -
Standard Libraries: Libraries like
itertools
andfunctools
provide efficient implementations of common tasks and algorithms.
Avoiding Unnecessary Computations
Optimizing Python code often involves reducing unnecessary operations:
-
Caching Results: Functions like
functools.lru_cache
can be used to cache the results of expensive function calls, avoiding redundant computations. -
Avoiding Global Variables: Accessing global variables in Python is slower than accessing local variables. Therefore, minimizing their use can result in performance gains.
Start Learning Coding today and boost your Career Potential
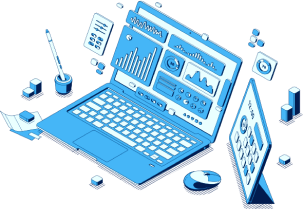
Using Third-party Libraries for Computationally Intensive Tasks
For data-intensive tasks, leveraging optimized third-party libraries can be highly effective:
- NumPy and Pandas: These libraries are optimized with C extensions, offering significant performance improvements for numerical computations and data manipulation.
Dealing with Multithreading and Multiprocessing
Python's GIL can limit the effectiveness of multi-threading in CPU-bound tasks. However, there are strategies to work around this:
-
Multithreading: This is most effective for I/O-bound tasks where the program spends time waiting for external resources. Python threads can be used to handle multiple I/O-bound tasks concurrently.
-
Multiprocessing: For CPU-bound tasks, the multiprocessing module can help by creating separate Python processes, each with its own Python interpreter and memory space, thus bypassing the GIL.
FAQs
Q: Do I need to always optimize my Python code?
A: Optimization should be focused on parts of the code that are critical performance bottlenecks. Premature optimization can lead to unnecessary complexity.
Q: Can Python be as fast as compiled languages like C++?
A: Python's interpreted nature generally makes it slower than compiled languages. However, with optimization techniques and JIT compilers, it can achieve performance close to compiled languages for certain tasks.
Q: How important is algorithm choice in Python optimization?
A: Algorithm choice is crucial in optimization. Efficient algorithms can dramatically reduce both time and space complexity, leading to better performance.
Q: Is it worth using third-party libraries for optimization?
A: Yes, especially for computationally intensive tasks. Libraries like NumPy and Pandas are optimized at a low level and can provide significant performance benefits.
Q: How can I manage memory usage in Python?
A: Effective memory management involves using appropriate data structures, avoiding unnecessary data copies, and utilizing generators for iterating over large data sets to reduce memory footprint.
Related courses
See All CoursesThe SOLID Principles in Software Development
The SOLID Principles Overview
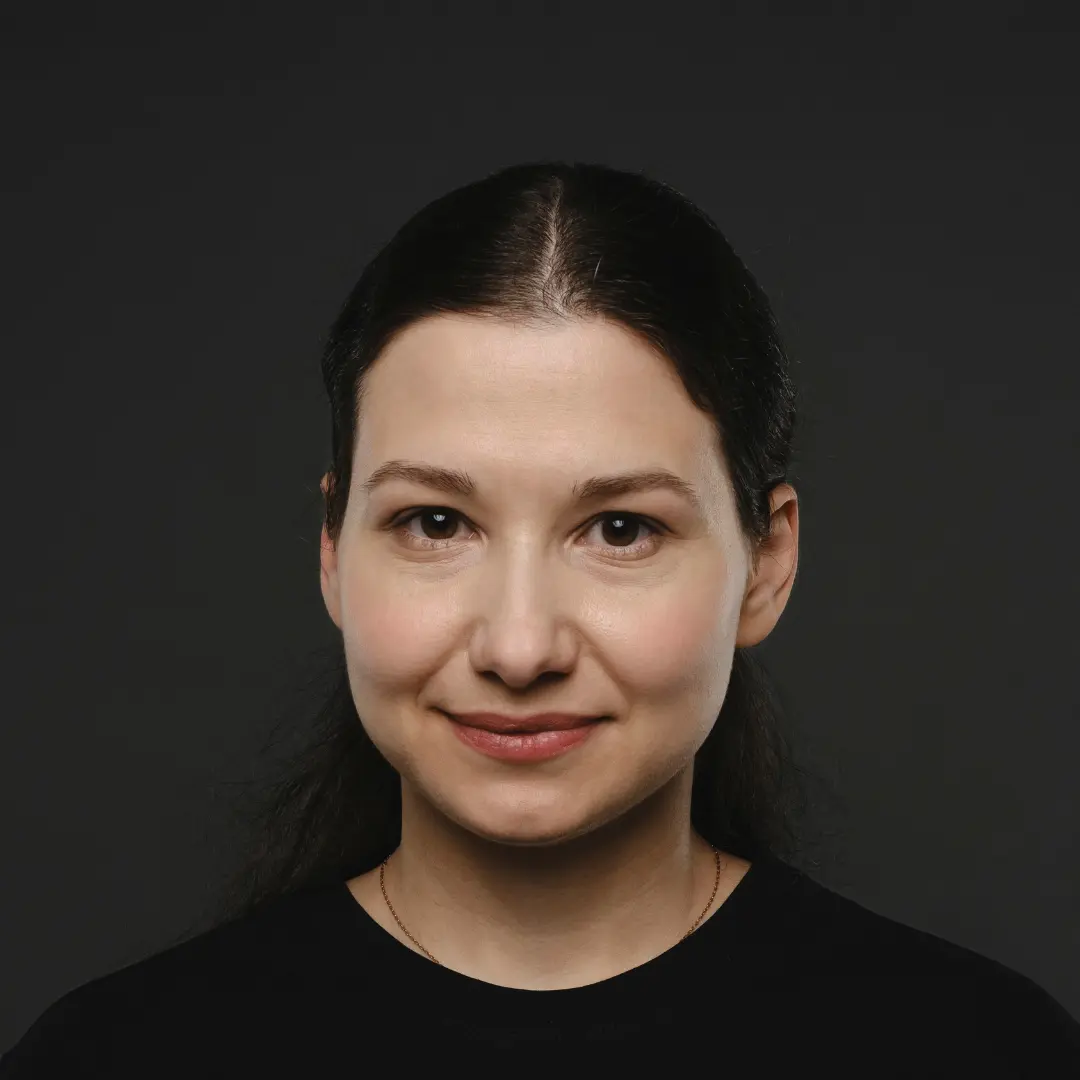
by Anastasiia Tsurkan
Backend Developer
Nov, 2023γ»8 min read
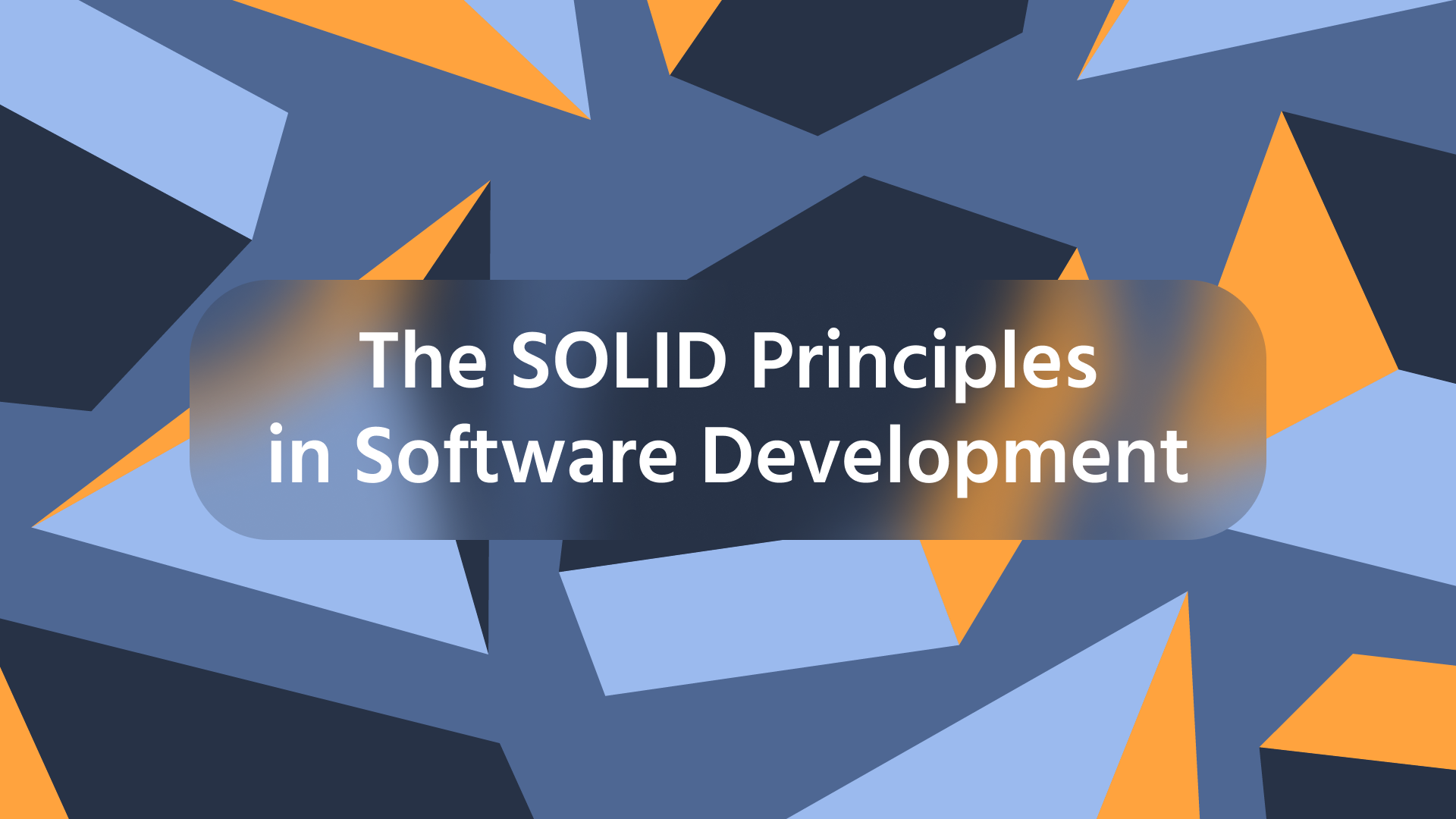
Match-case Operators in Python
Match-case Operators vs if-elif-else statements
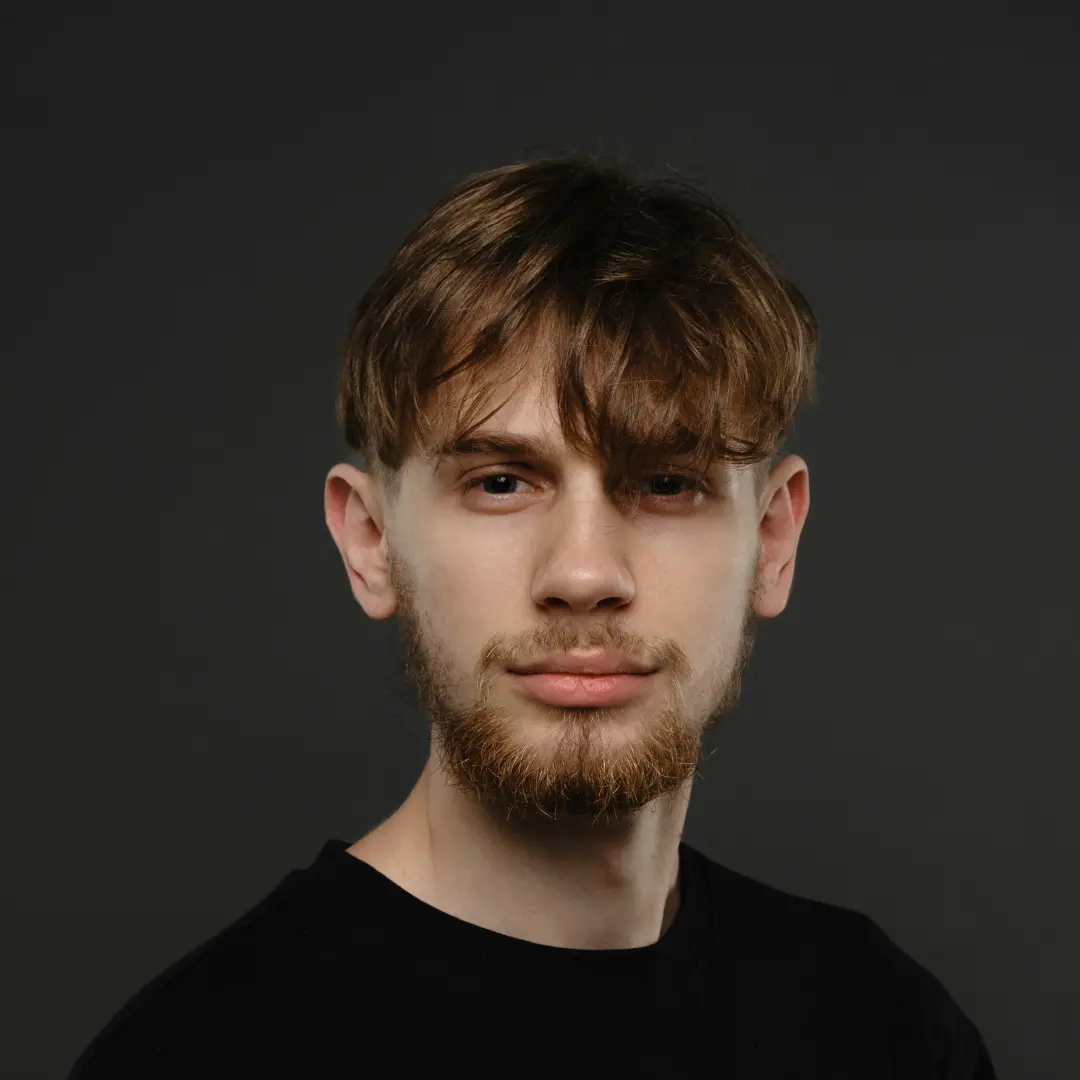
by Oleh Lohvyn
Backend Developer
Dec, 2023γ»6 min read
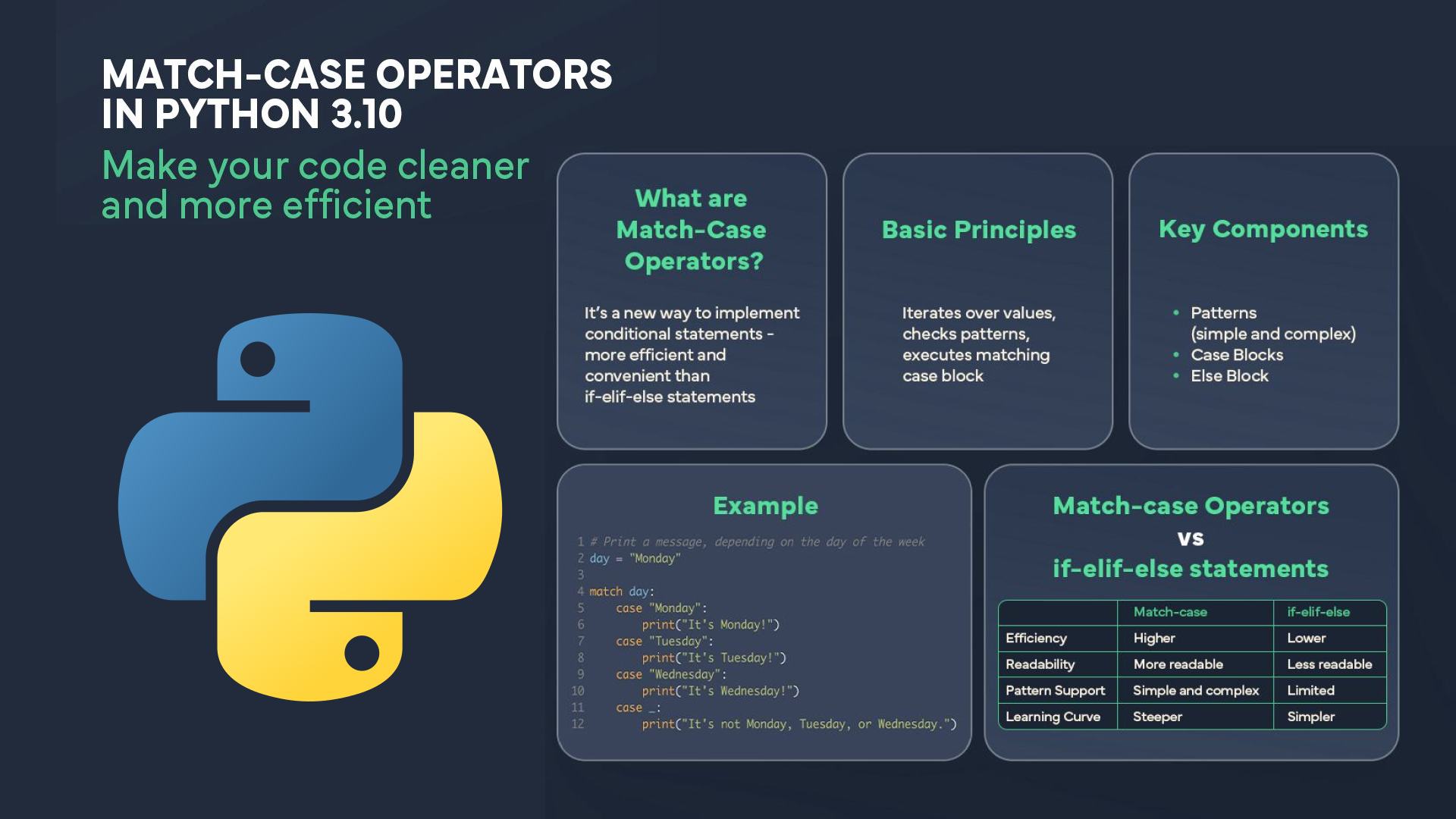
30 Python Project Ideas for Beginners
Python Project Ideas
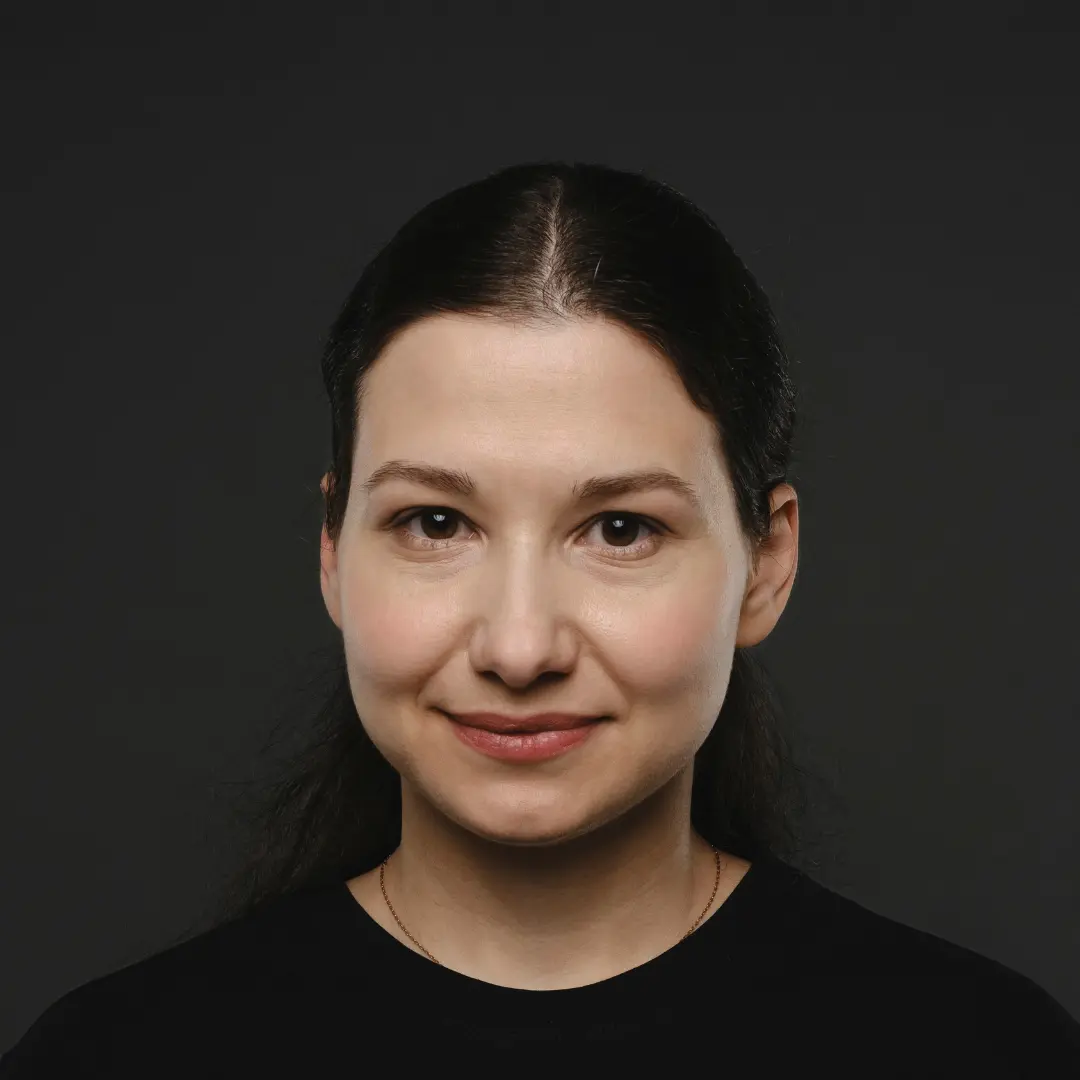
by Anastasiia Tsurkan
Backend Developer
Sep, 2024γ»14 min read
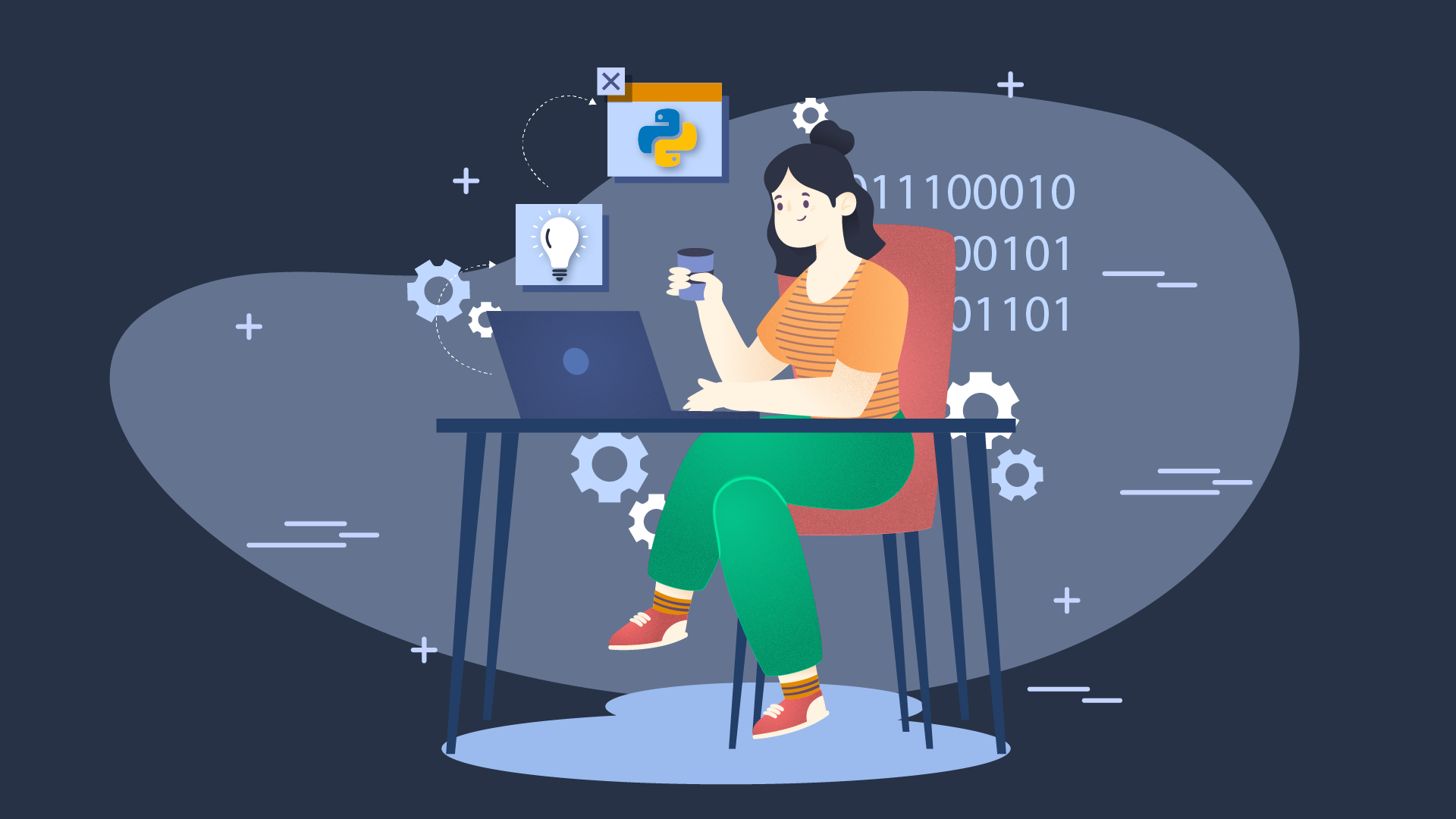
Content of this article