Related courses
See All CoursesBeginner
Introduction to Python
Python is a high-level, interpreted, general-purpose programming language. Distinguished from languages such as HTML, CSS, and JavaScript, which are mainly utilized in web development, Python boasts versatility across multiple domains, including software development, data science, and back-end development. This course will guide you through Python's fundamental concepts, equipping you with the skills to create your own functions by the conclusion of the program.
Intermediate
Intermediate Python Techniques
In this course, you will learn advanced topics such as function arguments, iterators, generators, closures, and decorators. Dozens of practice exercises will help you grasp these topics.
Asynchrony vs Multithreading
Asynchrony vs Multithreading
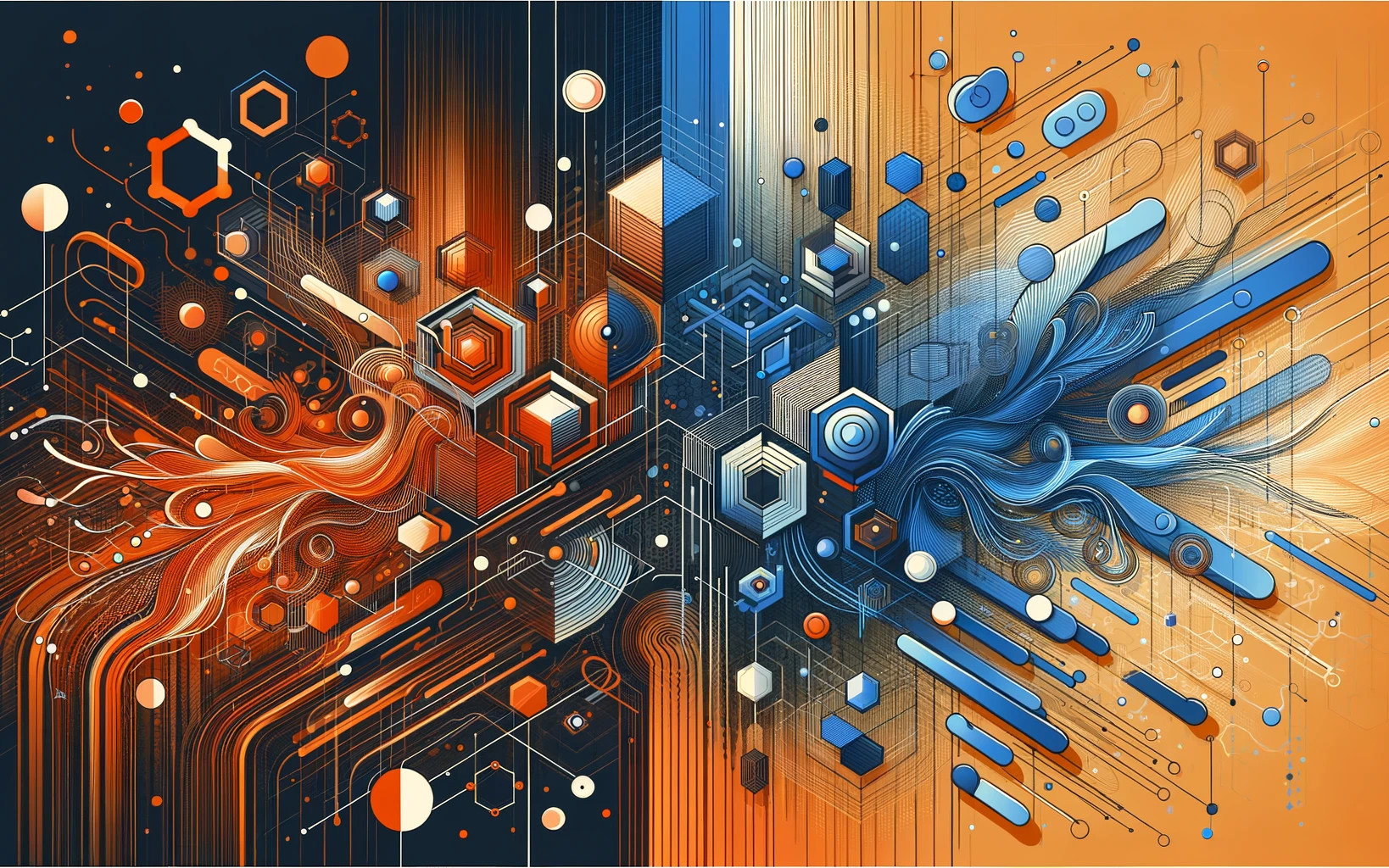
Introduction
In the realm of software development, particularly in handling concurrent operations, two prevalent concepts emerge: asynchrony and multithreading. While both are designed to optimize the execution of tasks and improve application performance, they operate on fundamentally different principles and architectures. Understanding the distinctions between asynchrony and multithreading is crucial for developers to make informed decisions when architecting software solutions.
Understanding Asynchrony
What is Asynchrony?
Asynchrony refers to the execution of tasks in a non-blocking manner, allowing a program to initiate a potentially long-running task and continue executing other tasks without waiting for the first task to complete. Asynchronous operations are typically handled using callbacks, promises, or async/await syntax, depending on the programming language.
Key Features
- Non-blocking Execution: Asynchronous tasks do not block the main execution thread, enhancing responsiveness and performance.
- Event Loop: Many asynchronous environments, like Node.js, use an event loop to manage asynchronous operations, executing tasks as their corresponding events or conditions are met.
- Suitability: Ideal for I/O-bound tasks, such as web requests, file operations, or network communications, where waiting for the operation to complete would otherwise idle the system.
Run Code from Your Browser - No Installation Required
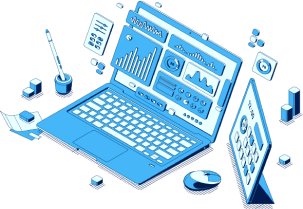
Understanding Multithreading
What is Multithreading?
Multithreading involves the concurrent execution of multiple threads within a single process, allowing the program to perform multiple operations simultaneously. Each thread represents a separate path of execution, sharing the process's memory space but running independently.
Key Features
- Concurrency: Multithreading enables the concurrent execution of tasks, utilizing multiple CPU cores to perform computations in parallel.
- Context Switching: Threads may undergo context switching, where the CPU switches between different threads, allowing for multitasking within the same application.
- Suitability: Best suited for CPU-bound tasks that require significant computation, where parallelizing the workload can significantly reduce execution time.
Comparing Asynchrony and Multithreading
Execution Model
- Asynchrony: Relies on single-threaded event loops or non-blocking I/O operations, suitable for handling a large number of tasks that are I/O-bound.
- Multithreading: Utilizes multiple threads to achieve parallel execution, ideal for tasks that are computationally intensive and can be divided into parallel workloads.
Resource Utilization
- Asynchrony: Efficient in handling I/O-bound operations with minimal CPU usage, as tasks are executed sequentially in a non-blocking manner.
- Multithreading: Can increase CPU utilization by distributing tasks across multiple cores, but also incurs overhead from context switching and synchronization.
Complexity and Overhead
- Asynchrony: Can simplify the handling of concurrent I/O-bound operations, but managing asynchronous code flow can become complex, especially with nested callbacks or error handling.
- Multithreading: Introduces complexity in ensuring thread safety and managing synchronization between threads, requiring careful design to avoid issues like deadlocks or race conditions.
Use Cases
Asynchrony
- Web servers handling multiple client requests simultaneously.
- Applications performing background data fetching or file I/O operations.
Multithreading
- Data processing applications requiring intensive computation, such as image or video processing.
- Applications that need to perform several unrelated tasks concurrently, like a GUI application running background tasks while remaining responsive to user input.
Start Learning Coding today and boost your Career Potential
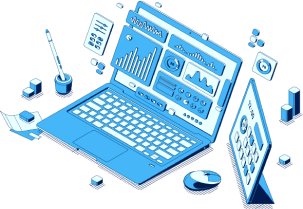
Conclusion
Choosing between asynchrony and multithreading depends on the specific requirements of the application, including the nature of the tasks (I/O-bound vs. CPU-bound), performance considerations, and the desired complexity of implementation. Understanding the strengths and limitations of each approach enables developers to architect more efficient, responsive, and scalable applications.
FAQs
Q: Can asynchrony and multithreading be used together?
A: Yes, it's possible and sometimes beneficial to use both in the same application. For example, an asynchronous I/O operation might run on a separate thread to avoid blocking the main thread, combining the advantages of non-blocking I/O operations with parallel execution.
Q: How does error handling differ between asynchronous operations and multithreading?
A: Asynchronous operations typically use mechanisms like promises or try/catch blocks (in languages that support async/await) to handle errors. In multithreading, error handling must consider synchronization and thread safety, often requiring more complex mechanisms to propagate errors across threads.
Q: Is one approach more efficient than the other?
A: Efficiency depends on the application's specific tasks. Asynchrony is more efficient for I/O-bound operations, minimizing idle time and resource utilization. Multithreading is more efficient for CPU-bound tasks that can be parallelized to reduce overall execution time.
Q: Does multithreading always improve performance?
A: Not necessarily. While multithreading can improve performance for CPU-bound tasks, the overhead of thread management and synchronization can outweigh the benefits for I/O-bound tasks or when used excessively in applications not designed for parallel execution.
Q: Are there programming languages that favor one approach over the other?
A: Yes. For example, Node.js is designed around asynchronous I/O operations, making it highly suitable for I/O-bound tasks. In contrast, languages like Java and C# offer extensive support for multithreading, making them well-suited for developing applications that require parallel computation.
Related courses
See All CoursesBeginner
Introduction to Python
Python is a high-level, interpreted, general-purpose programming language. Distinguished from languages such as HTML, CSS, and JavaScript, which are mainly utilized in web development, Python boasts versatility across multiple domains, including software development, data science, and back-end development. This course will guide you through Python's fundamental concepts, equipping you with the skills to create your own functions by the conclusion of the program.
Intermediate
Intermediate Python Techniques
In this course, you will learn advanced topics such as function arguments, iterators, generators, closures, and decorators. Dozens of practice exercises will help you grasp these topics.
The SOLID Principles in Software Development
The SOLID Principles Overview
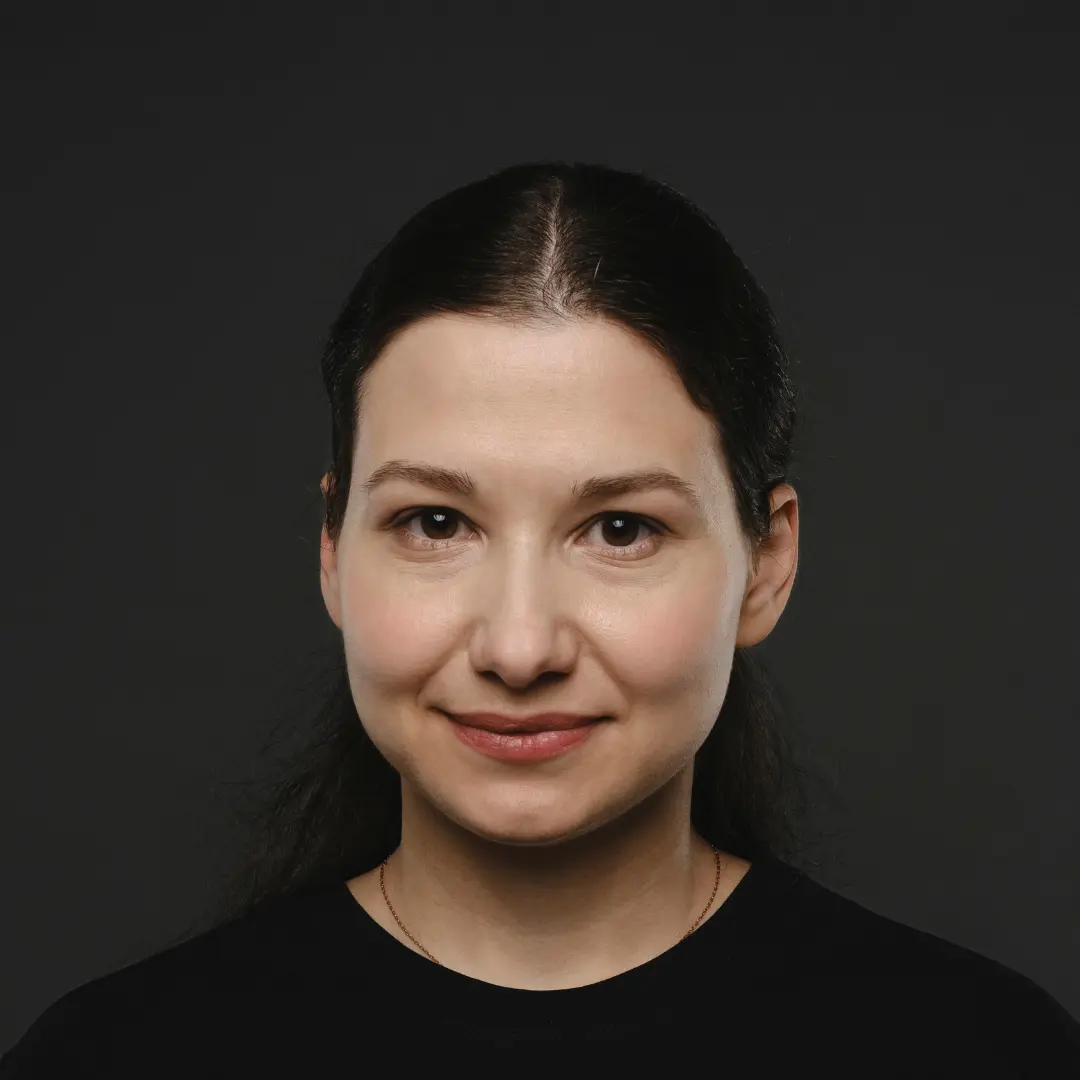
by Anastasiia Tsurkan
Backend Developer
Nov, 2023γ»8 min read
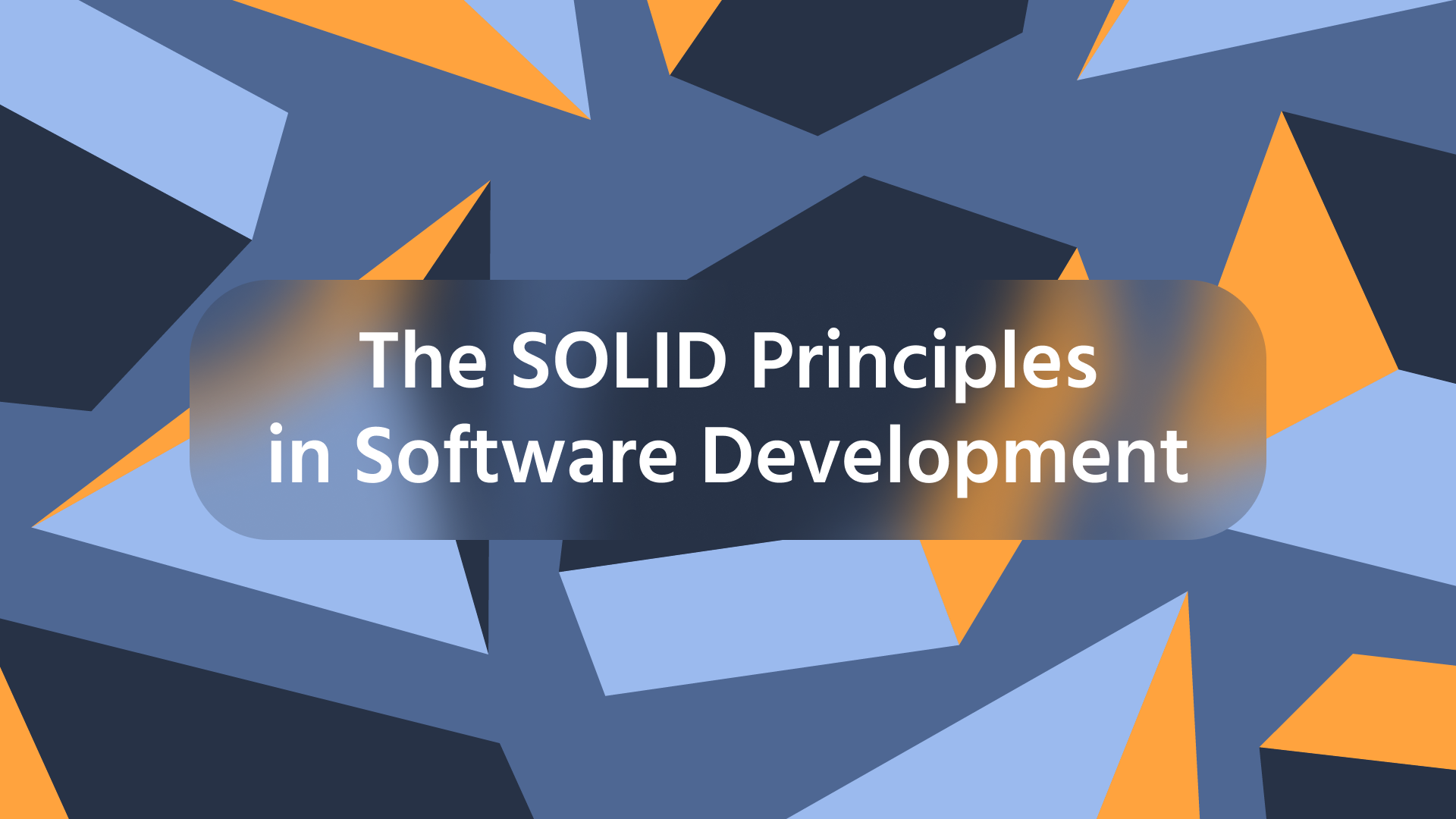
30 Python Project Ideas for Beginners
Python Project Ideas
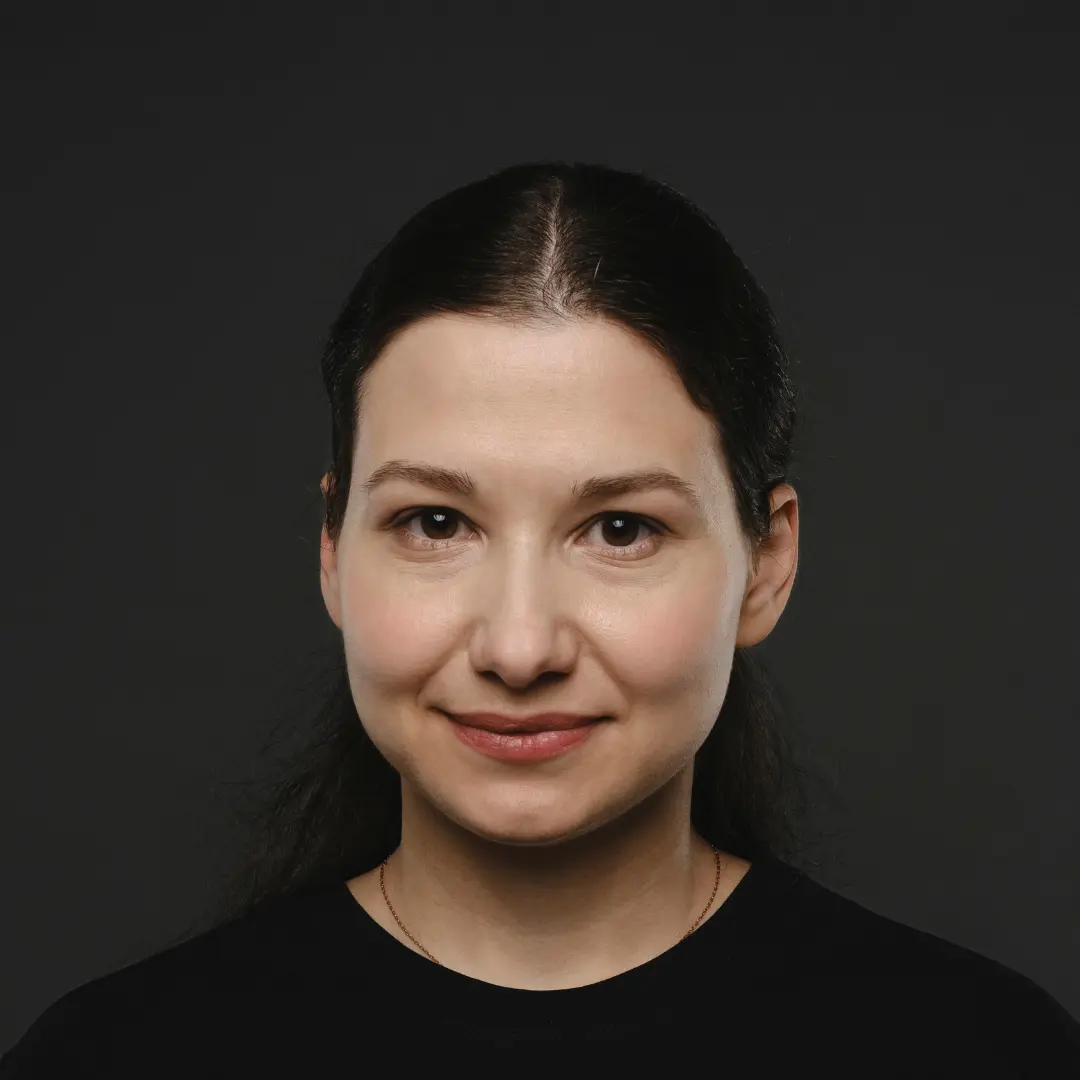
by Anastasiia Tsurkan
Backend Developer
Sep, 2024γ»14 min read
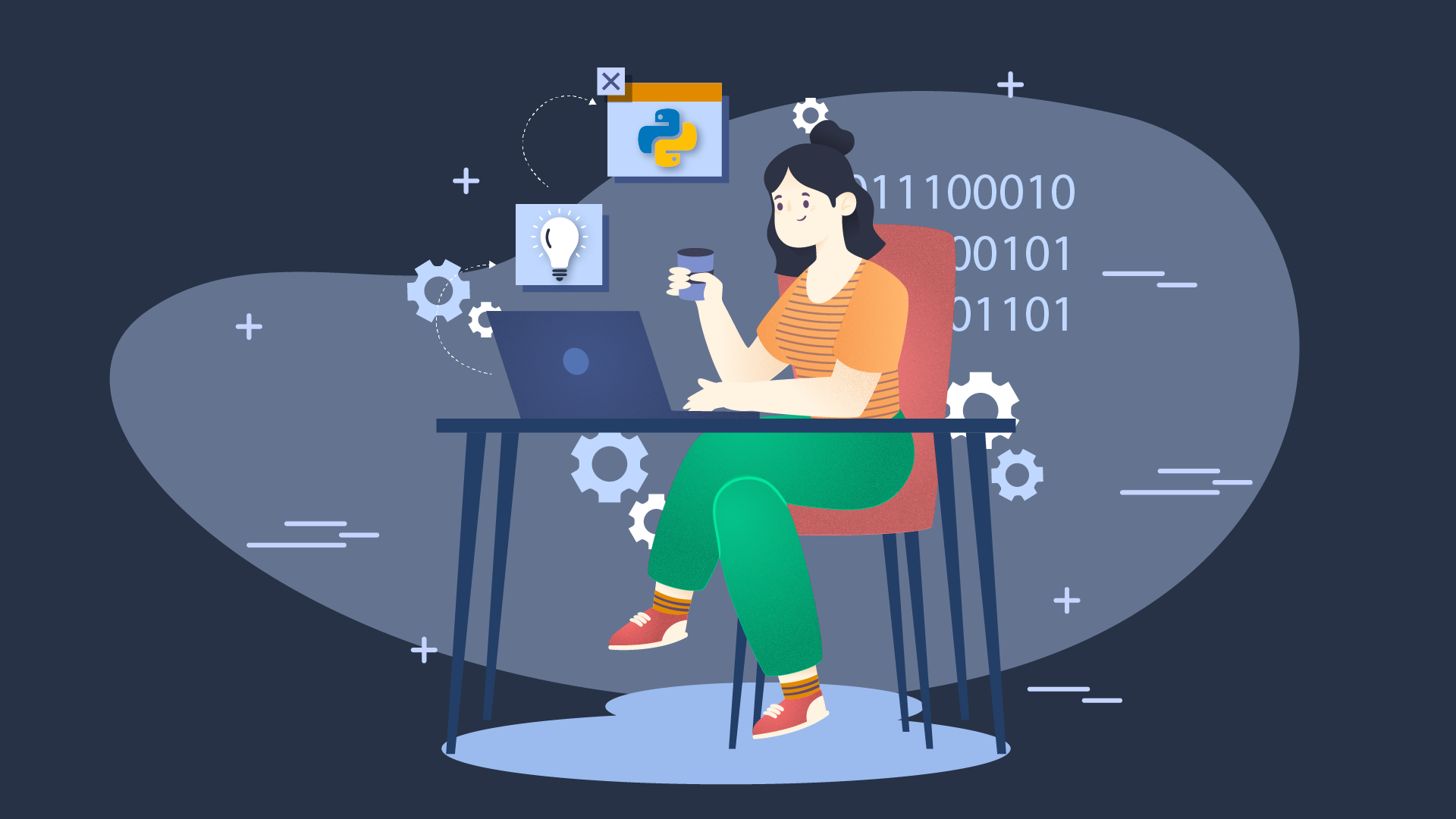
Asynchronous Programming in Python
Brief Intro to Asynchronous Programming
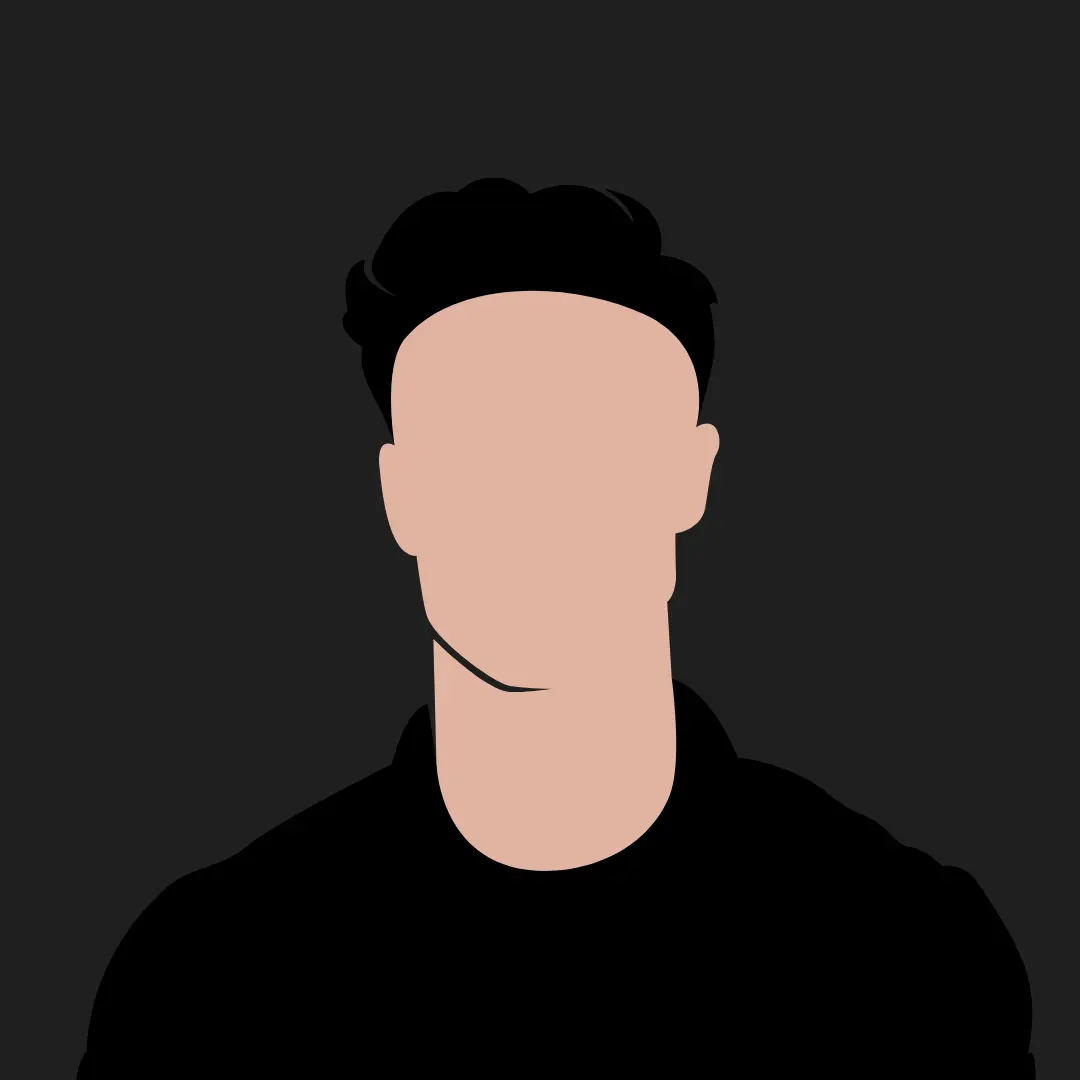
by Ruslan Shudra
Data Scientist
Dec, 2023γ»5 min read
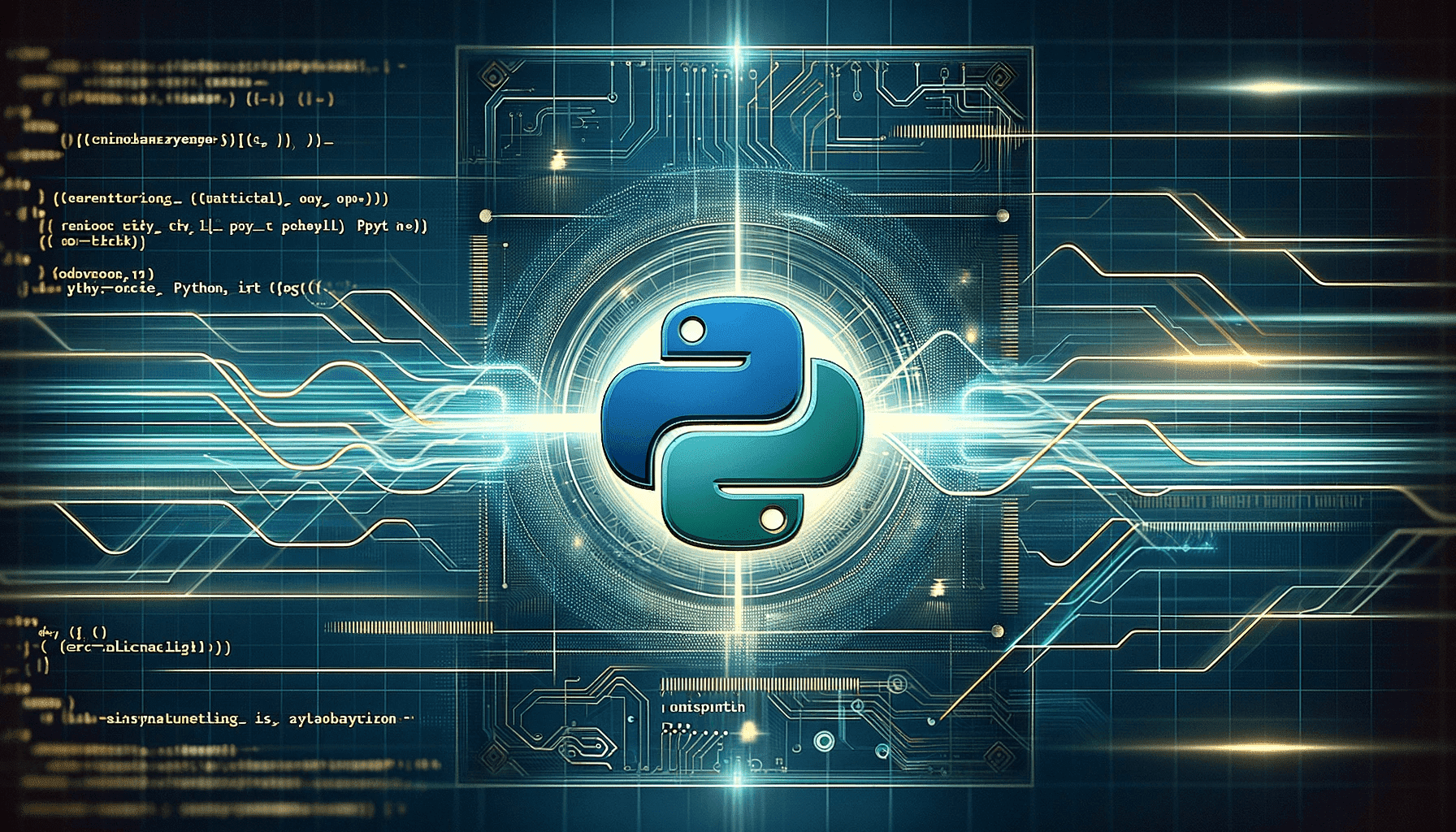
Content of this article