Курси по темі
Всі курсиПросунутий
Java OOP
Those who know OOP can program well. That's what many programmers say. Get ready for an important part of your Java learning journey, mastering which will greatly boost your programming skills in general. You will learn how to effectively use the Java development environment, the principles of Object-Oriented Programming (OOP), and best practices in OOP. You will learn to make your code flexible and deepen your knowledge of previously covered topics. Let's get started!
Середній
Середній Рівень Python: Аргументи, Генератори та Декоратори
У цьому курсі ви вивчите такі складні теми, як аргументи функцій, ітератори, генератори, замикання та декоратори. Десятки практичних вправ допоможуть вам засвоїти ці теми.
Середній
C++ Functions
Explore the world of C++ functions and delve into their fundamental concepts and practical applications. Gain proficiency in declaring, using, and optimizing functions, including parameter handling, scope management, and function overloading. Learn about recursion, templates, and lambda expression for robust C++ programming. Develop the skills to create modular, efficient, and reusable functions for real-world coding challenges.
Functional Programming vs Object-Oriented Programming
A Comparative Guide for Beginners
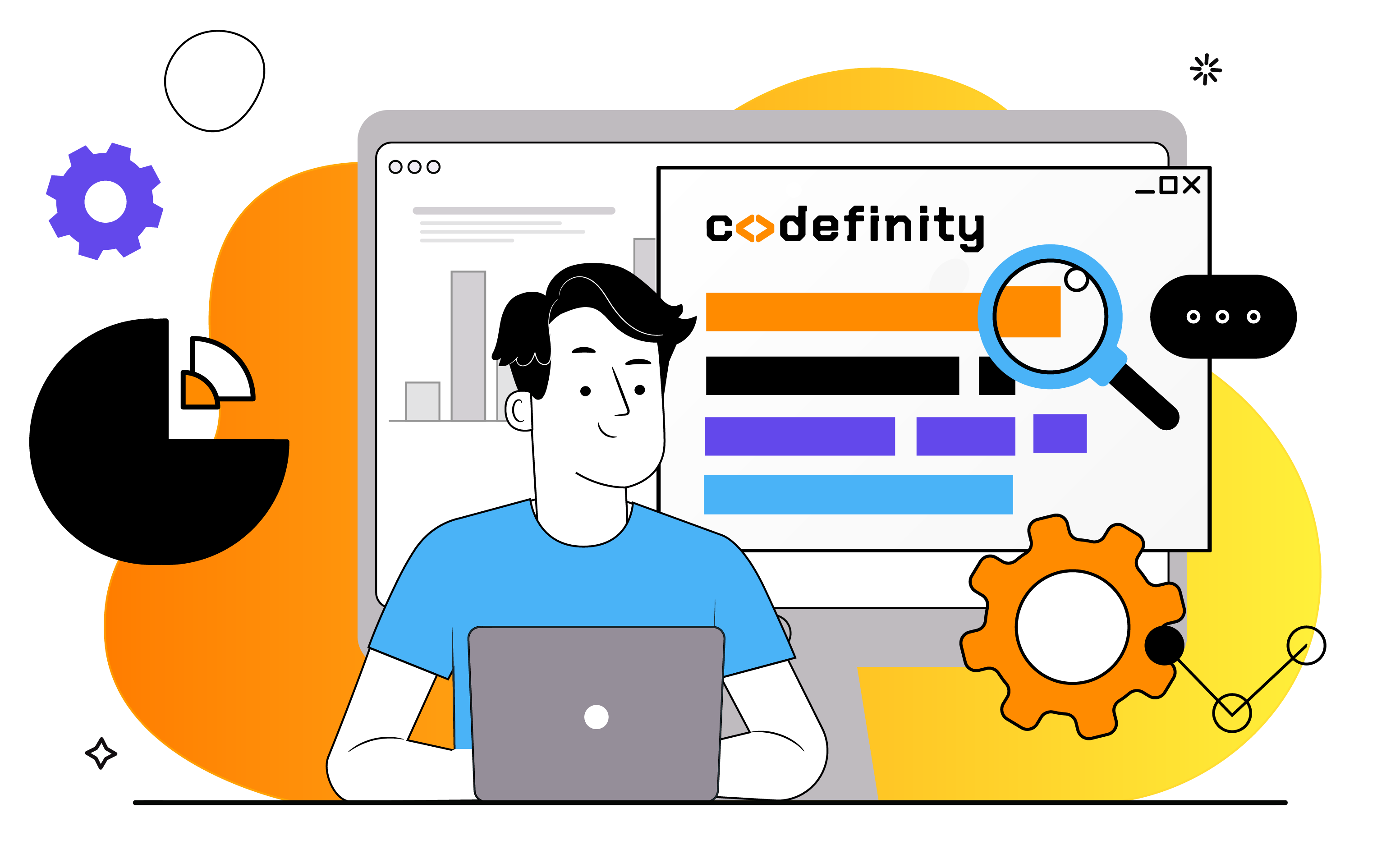
In the world of software development, the approach you take to write and organize code can significantly affect both the process and the outcome of your projects. Among the myriad of programming paradigms, Functional Programming (FP) and Object-Oriented Programming (OOP) stand out due to their unique approaches and widespread use. This article aims to provide a comprehensive understanding of both paradigms, focusing on their principles, advantages, challenges, and applications, particularly for beginners in programming.
Understanding Functional Programming (FP)
Functional Programming, inspired by mathematical functions, is a style of building the structure and elements of computer programs—that treats computation as the evaluation of mathematical functions and avoids changing-state and mutable data. This paradigm emphasizes the application of functions, in contrast to the imperative programming paradigm, which emphasizes changes in state.
Key Concepts in Detail:
-
Immutability: In FP, once a data structure is created, it cannot be altered. Any modification leads to the creation of a new data structure. This principle reduces the likelihood of bugs related to state changes and makes the code more predictable.
-
Pure Functions: A cornerstone of FP, pure functions always produce the same output for the same set of inputs and do not cause any observable side effects, such as modifying a global object or a parameter passed by reference.
-
First-Class and Higher-Order Functions: Functions in FP are treated as first-class citizens, meaning they can be assigned to variables, passed as arguments to other functions, and returned from other functions. Higher-order functions either take one or more functions as arguments or return a function as their result, enabling powerful ways to compose software.
Examples of FP Languages and Their Usage:
- Haskell: A standard-bearer for FP, Haskell is used in academia and industry for its strong static typing and rich function composition capabilities.
- Scala: Combining OOP and FP, Scala is a good choice for scalable concurrent applications, such as Twitter’s backend.
- Clojure: A modern Lisp variant, Clojure is known for its simplicity and focus on concurrency, making it suitable for multi-threaded applications.
Practical Applications of FP
- Data Analysis and Processing: The immutable data structures and higher-order functions in FP make it ideal for data transformation and analysis.
- Concurrent Systems: With its immutable data and pure functions, FP excels in concurrent programming, making it a good choice for applications requiring high concurrency.
Run Code from Your Browser - No Installation Required
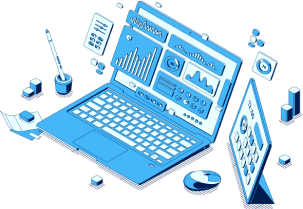
Diving into Object-Oriented Programming (OOP)
Object-Oriented Programming organizes software design around data, or objects, rather than functions and logic. An object can be defined as a data field that has unique attributes and behavior. OOP's primary aim is to increase the flexibility and maintainability of code through its core concepts.
Key Concepts in Detail:
-
Encapsulation: This is about bundling the data (variables) and the methods (functions) that operate on the data into single units, known as objects. Encapsulation helps in hiding the internal state of an object from the outside world.
-
Inheritance: It allows a new class to inherit the properties and methods of an existing class. This helps in code reusability and in creating a hierarchy of classes.
-
Polymorphism: This principle allows objects of different classes to be treated as objects of a common superclass. It is the ability of different objects to respond, each in its own way, to identical messages.
Examples of OOP Languages and Their Usage:
- Java: Renowned for its portability across platforms, Java is widely used in enterprise environments, Android app development, and web applications.
- C++: Known for its performance and efficiency, C++ is used in software development for systems where hardware access and performance are critical, such as game development and real-time systems.
- Python: Python’s simplicity and readability make it ideal for web development, data analysis, artificial intelligence, and more.
Practical Applications of OOP
- Game Development: The ability to model complex entities as objects makes OOP ideal for game development.
- GUI Applications: The encapsulation of data and operations into objects suits the development of applications with graphical user interfaces, where elements can be treated as objects.
Functional Programming in Practice
Key Advantages and Challenges:
- Testability and Debugging: Pure functions are easier to test and debug due to their predictability and lack of side effects. However, the abstract nature of FP can be challenging to grasp initially, and debugging can be difficult due to the lack of state changes to track.
- Concurrency and Performance: FP's immutable data and stateless nature make it excellent for concurrent programming. However, applications requiring intensive data mutation may suffer performance penalties due to the overhead of creating new instances for every data change.
Object-Oriented Programming in Practice
Key Advantages and Challenges:
- Modularity and Reusability: OOP's encapsulation allows for modular code, and inheritance promotes reusability. However, large OOP systems can become complex and hard to maintain.
- Efficiency for Specific Tasks: While OOP is efficient for structuring complex programs, it can be less efficient and more cumbersome for tasks requiring extensive data transformation, which FP handles more elegantly.
Start Learning Coding today and boost your Career Potential
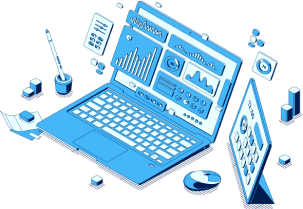
Choosing Between FP and OOP
Factors to Consider:
- Project Requirements: The nature of the project often dictates the more suitable paradigm. For example, projects requiring high levels of concurrency may benefit from FP, whereas projects with complex data models and behaviors may be better suited for OOP.
- Team Expertise: The skill set and familiarity of the development team with either paradigm can influence the choice. A team experienced in OOP might find it more productive to stick with that approach, and vice versa.
- Performance Considerations: Some tasks are more efficiently solved with one paradigm over the other, and choosing the right one can lead to better performance and easier maintenance.
Balancing FP and OOP:
Many modern programming languages, such as Python, Scala, and Kotlin, support both paradigms. This flexibility allows developers to combine the best aspects of both FP and OOP in a single project, leveraging the strengths of each to achieve more robust, efficient, and maintainable software solutions.
FAQs
Q: Which programming paradigm is better for beginners?
A: Both paradigms offer valuable perspectives. OOP might be more intuitive for beginners due to its tangible representation of concepts as objects. FP, while more abstract, introduces powerful concepts that promote clean and maintainable code.
Q: Can I use both FP and OOP in the same project?
A: Absolutely. Many languages support both paradigms, allowing you to utilize FP for parts of the project that benefit from its features, and OOP for others where its characteristics are more advantageous.
Q: Is FP or OOP more popular in the industry?
A: OOP has been traditionally more popular and is extensively used in many fields, including web and application development. However, FP has seen a surge in popularity, especially in systems that require high levels of concurrency and reliability.
Q: Do I need to choose one paradigm over the other?
A: No, a good understanding of both paradigms can make you a more versatile and effective programmer. In many cases, using a combination of both paradigms can lead to better software design.
Q: Are there programming languages that support both paradigms?
A: Yes, languages like Scala, Kotlin, and Python support both FP and OOP paradigms. These languages allow developers to choose the most appropriate paradigm for the task at hand, or even blend the two.
Курси по темі
Всі курсиПросунутий
Java OOP
Those who know OOP can program well. That's what many programmers say. Get ready for an important part of your Java learning journey, mastering which will greatly boost your programming skills in general. You will learn how to effectively use the Java development environment, the principles of Object-Oriented Programming (OOP), and best practices in OOP. You will learn to make your code flexible and deepen your knowledge of previously covered topics. Let's get started!
Середній
Середній Рівень Python: Аргументи, Генератори та Декоратори
У цьому курсі ви вивчите такі складні теми, як аргументи функцій, ітератори, генератори, замикання та декоратори. Десятки практичних вправ допоможуть вам засвоїти ці теми.
Середній
C++ Functions
Explore the world of C++ functions and delve into their fundamental concepts and practical applications. Gain proficiency in declaring, using, and optimizing functions, including parameter handling, scope management, and function overloading. Learn about recursion, templates, and lambda expression for robust C++ programming. Develop the skills to create modular, efficient, and reusable functions for real-world coding challenges.
The SOLID Principles in Software Development
The SOLID Principles Overview
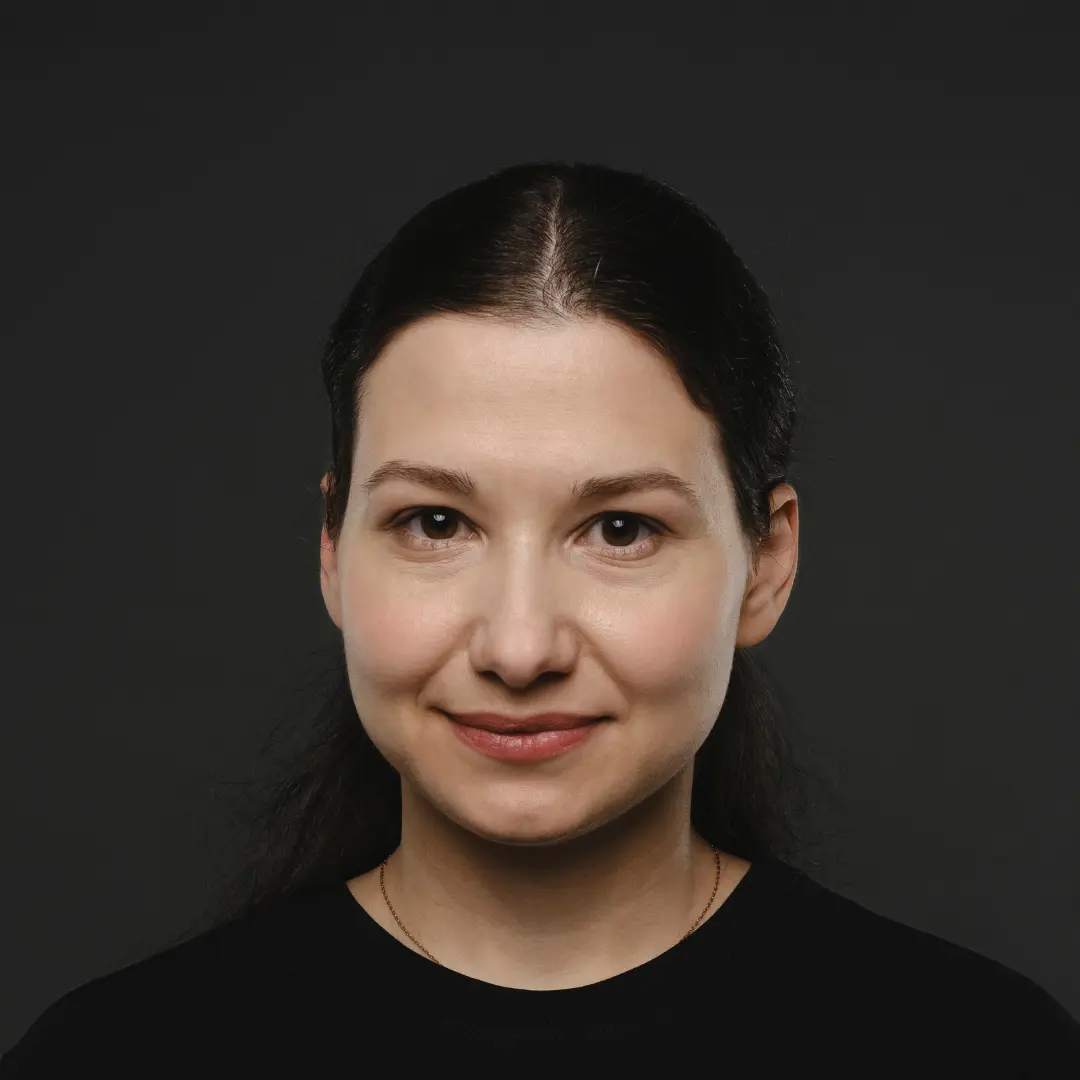
by Anastasiia Tsurkan
Backend Developer
Nov, 2023・8 min read
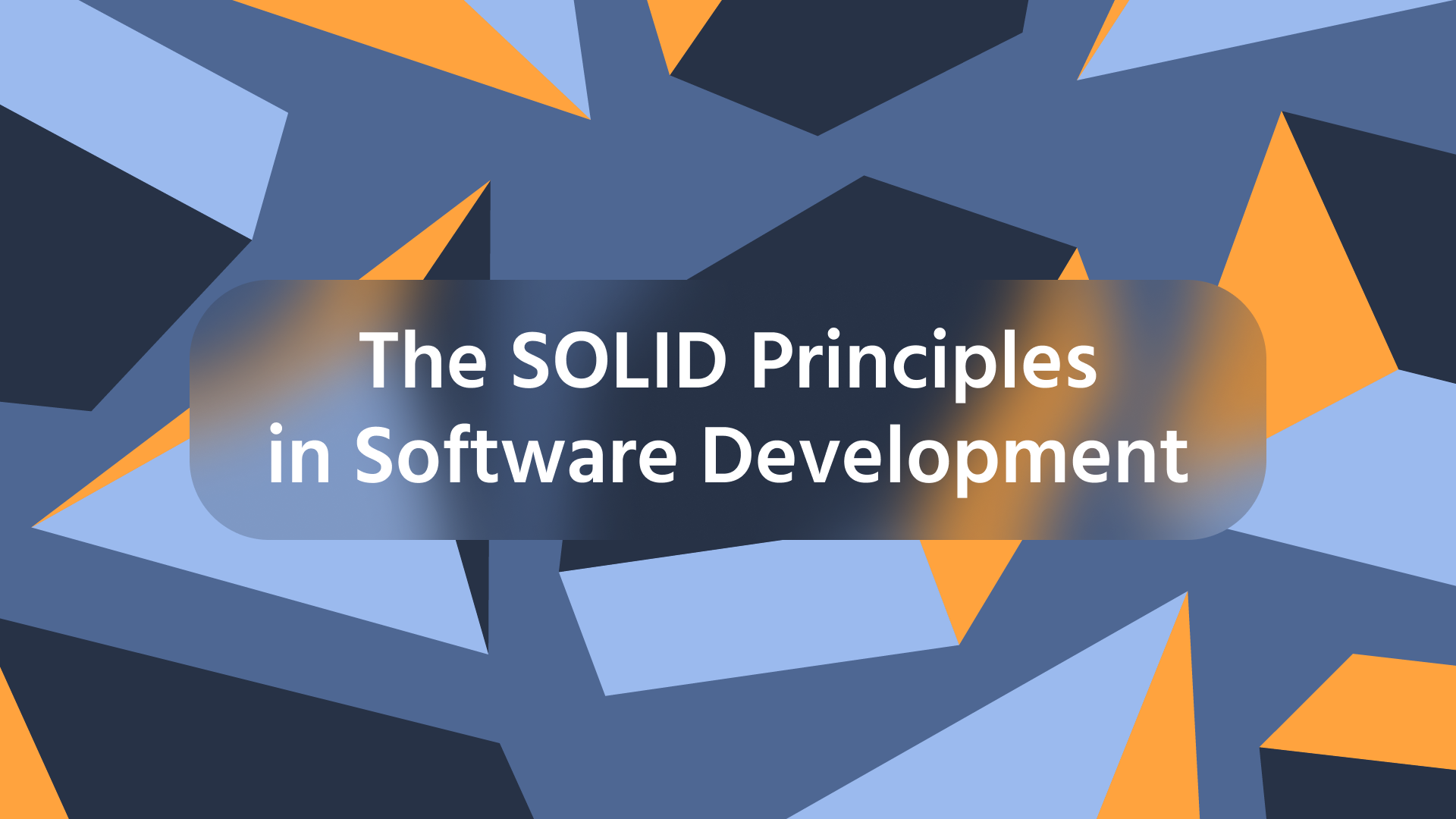
Match-case Operators in Python
Match-case Operators vs if-elif-else statements
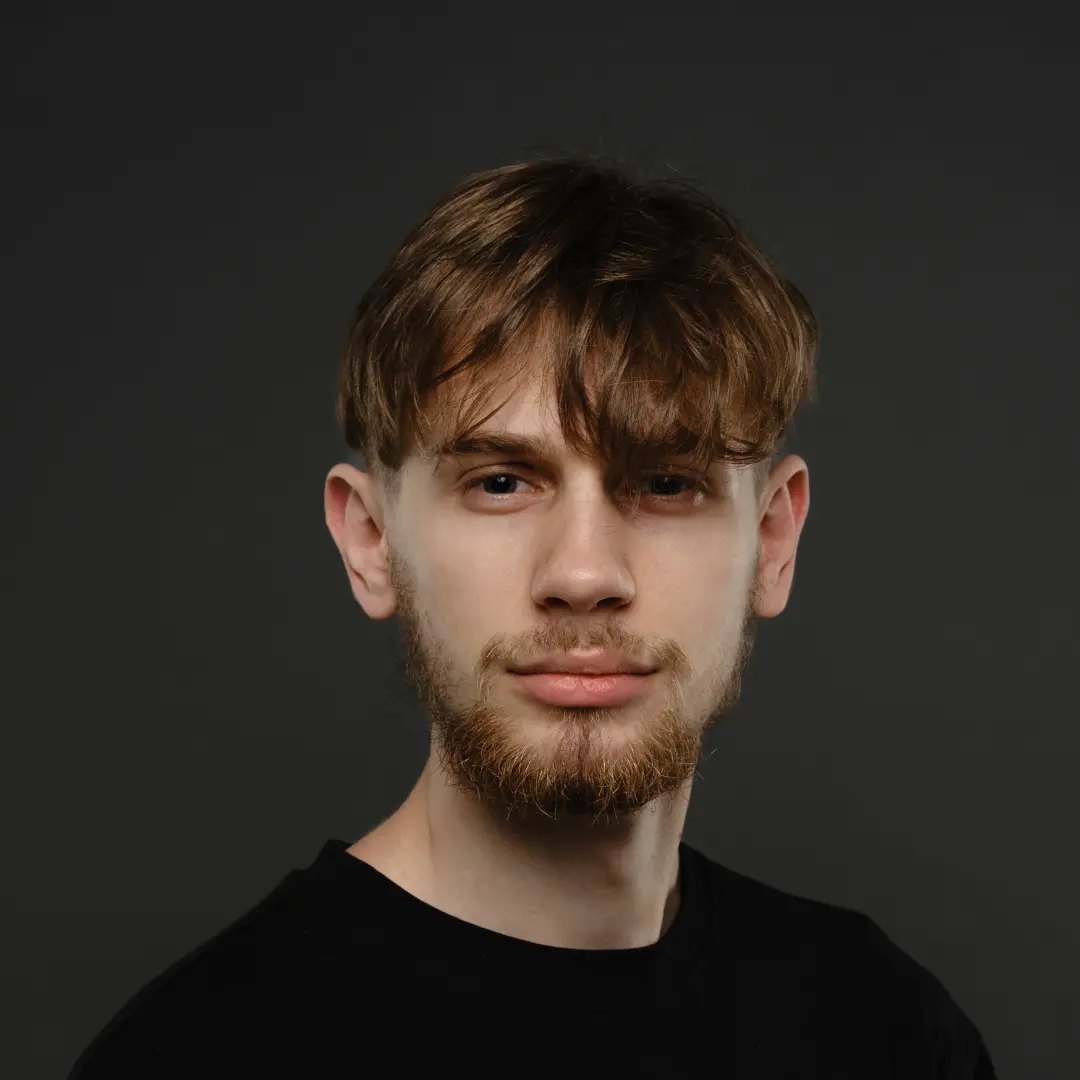
by Oleh Lohvyn
Backend Developer
Dec, 2023・6 min read
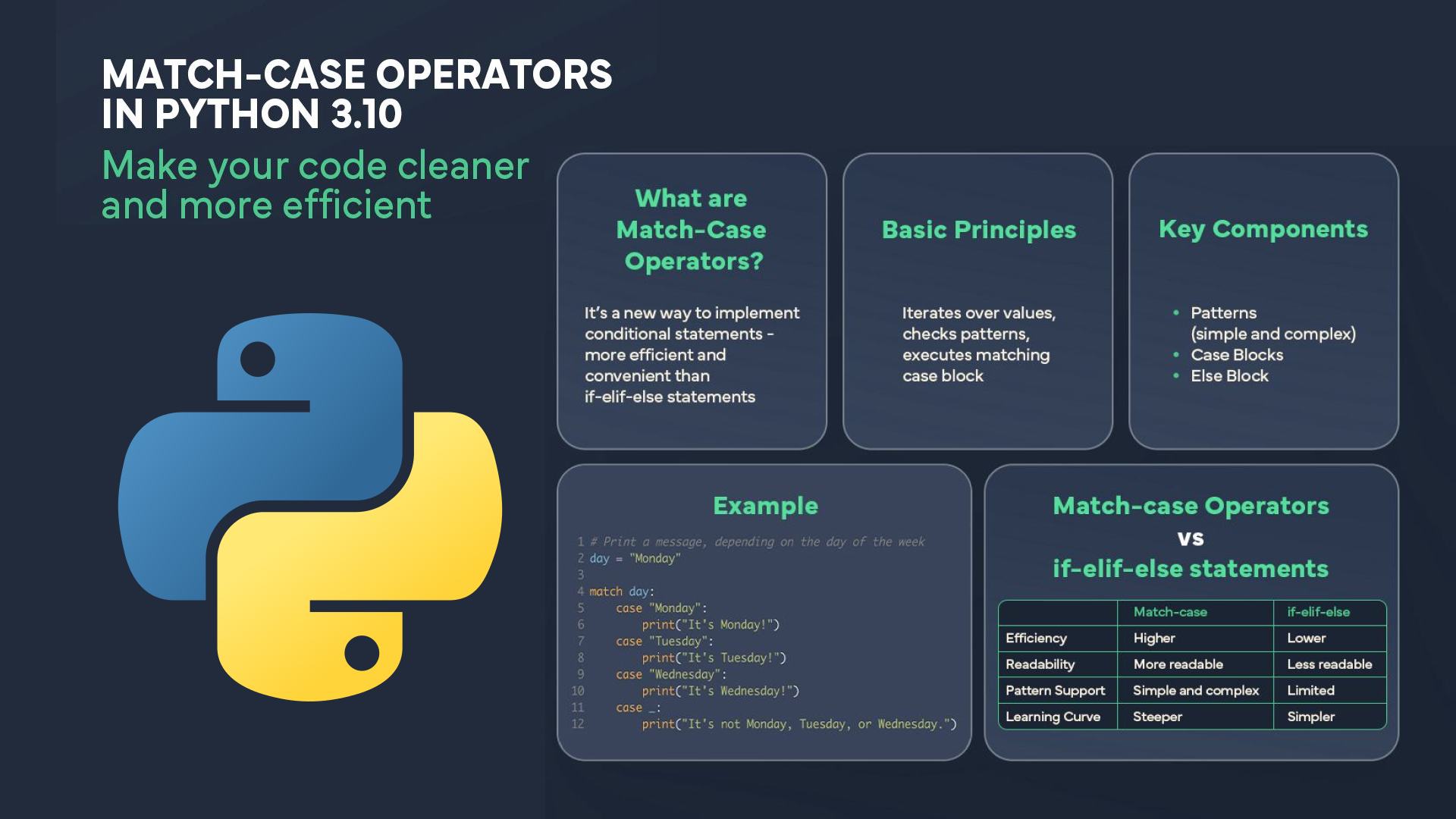
30 Python Project Ideas for Beginners
Python Project Ideas
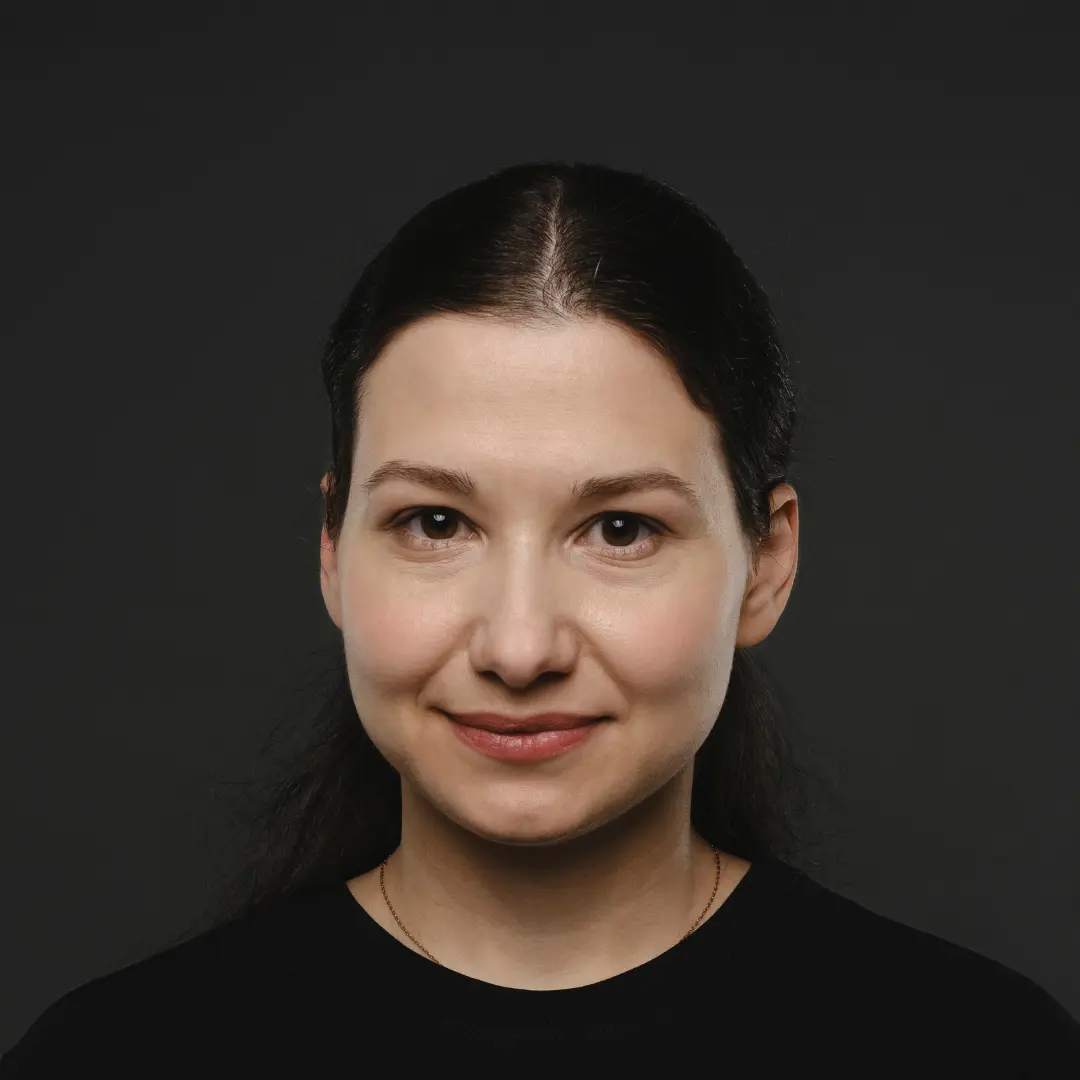
by Anastasiia Tsurkan
Backend Developer
Sep, 2024・14 min read
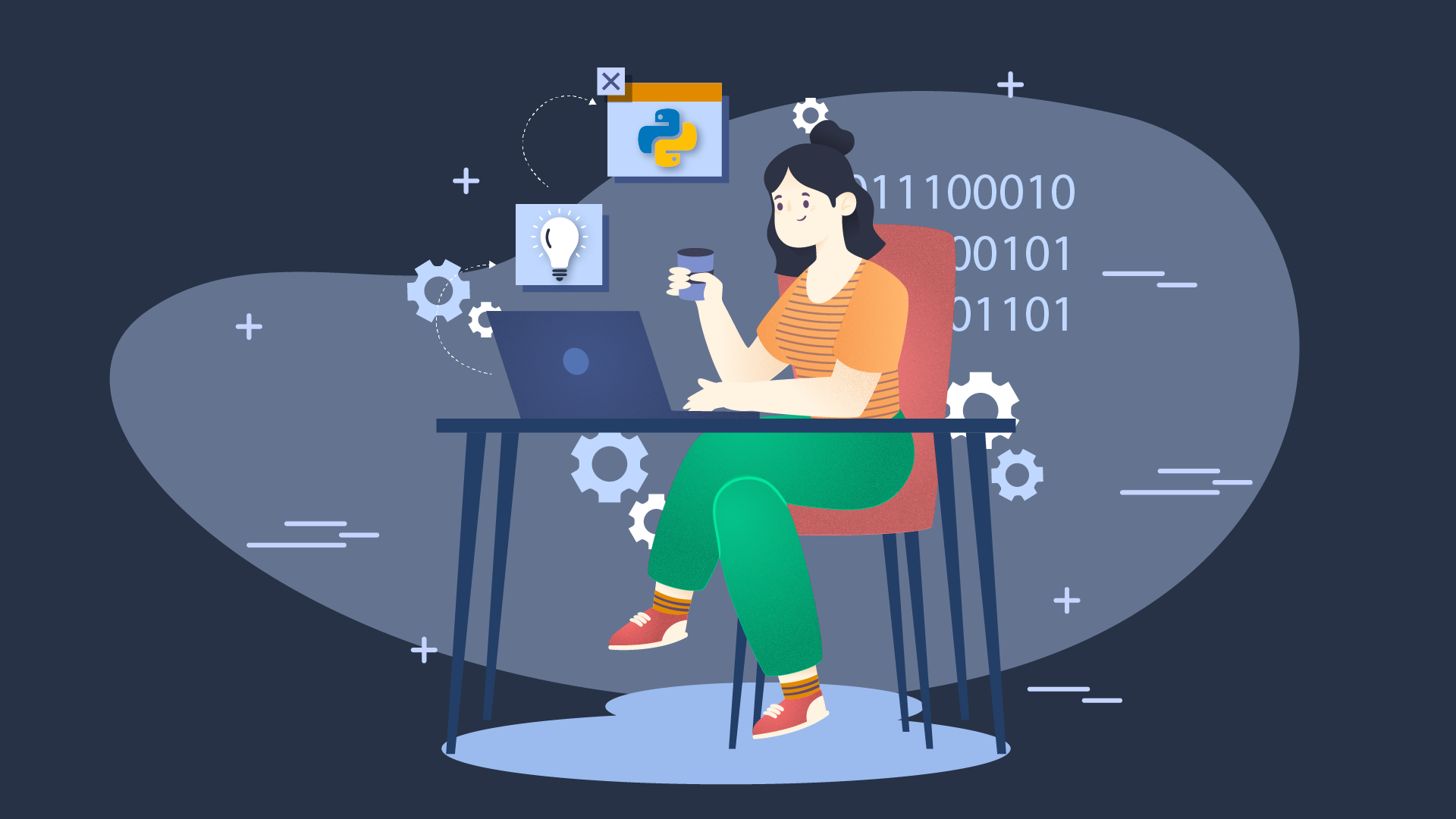
Зміст