Related courses
See All CoursesIntermediate
C++ Pointers and References
Unlock the Power of Memory Manipulation. Dive deep into the fundamentals of programming with this comprehensive course designed for beginners and individuals seeking to strengthen their knowledge on pointers and references in C++. Mastering these essential concepts is crucial for unleashing the full potential of your programming skills. Let's start!
Beginner
C++ Introduction
Start your path to becoming a skilled developer by mastering the foundational principles of programming through C++. Whether you're starting from scratch or already have some coding experience, this course will provide you with the solid foundation needed to become a proficient developer and open the doors to a wide range of career opportunities in software development and engineering. Let's study C++!
Memory Management in C++
How Memory works in C plus plus
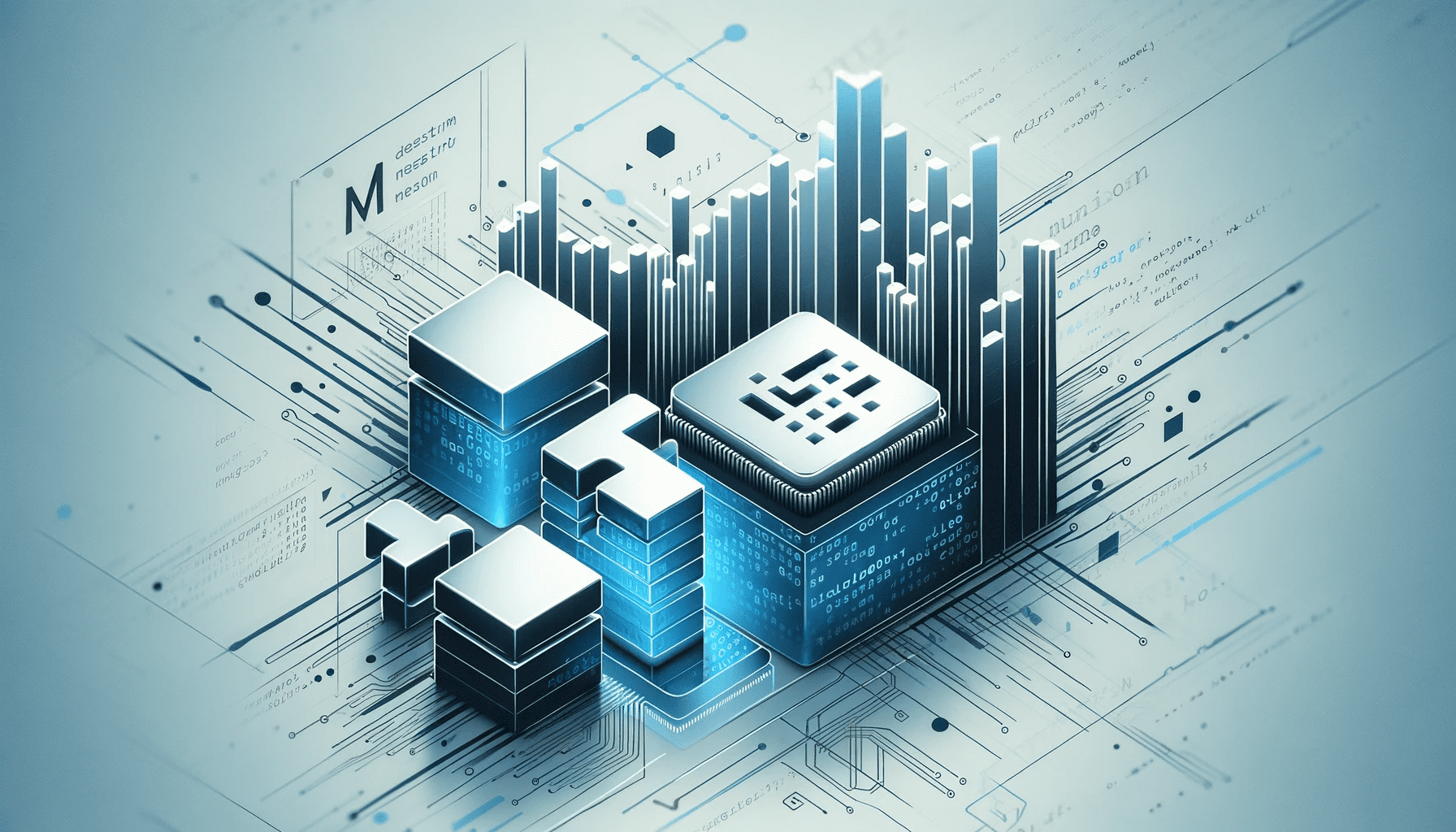
Memory management is a critical aspect of programming in C++. It involves the allocation, use, and deallocation of memory in an application. Effective memory management ensures optimal use of resources, prevents memory leaks, and enhances application performance.
Understanding memory
Before diving into best practices, it's essential to understand the basics of memory in C++. Memory in C++ can be broadly categorized into four segments:
Memory | Description | Management | Characteristics |
---|---|---|---|
Stack | Stores local variables and information about function calls. | Managed by the compiler. | - Operates on a Last-In-First-Out (LIFO) principle. - Fixed and relatively small size. - Not suitable for large data structures to avoid overflow. |
Heap | Used for dynamic memory allocation. | Managed by the programmer using new and delete . | - Requires explicit allocation and deallocation. - Prone to memory leaks if not managed properly. - Larger in size than the stack, but with slower allocation and deallocation. |
Global/Static | Stores global variables, static variables, and constants. | Allocated at program start and deallocated at program end. | - Lifetime spans the entire execution of the program. - Scope depends on the declaration location. - Can complicate debugging and testing if overused. |
Code | Contains the binary code of the program. | Managed by the operating system and the compiler. | - Usually read-only to prevent accidental modification. - No direct management needed by the programmer. |
Run Code from Your Browser - No Installation Required
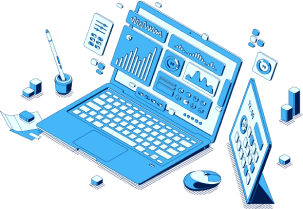
Avoid Memory Leaks
A memory leak in computing refers to a situation where a computer program incorrectly manages memory allocations, resulting in reduced performance and potentially causing system crashes or slowdowns. Here's a more detailed explanation:
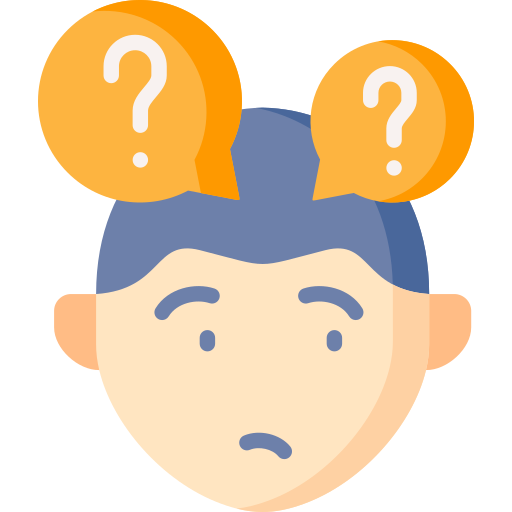
Forgetting to Free Memory | Not using delete for memory allocated with new . |
Double Free | Deallocating memory more than once. |
Dangling Pointers | Using pointers that point to deallocated memory. |
Memory fragmentation can occur in long-running applications, where the heap becomes cluttered with allocated and deallocated memory blocks.
Conclusion
Remember, memory management is not just about preventing leaks; it's about understanding how different types of memory (stack, heap, global/static) work and using them appropriately. The power of C++ lies in its ability to give programmers close control over memory usage, but this also requires a higher level of responsibility to ensure resources are managed correctly.
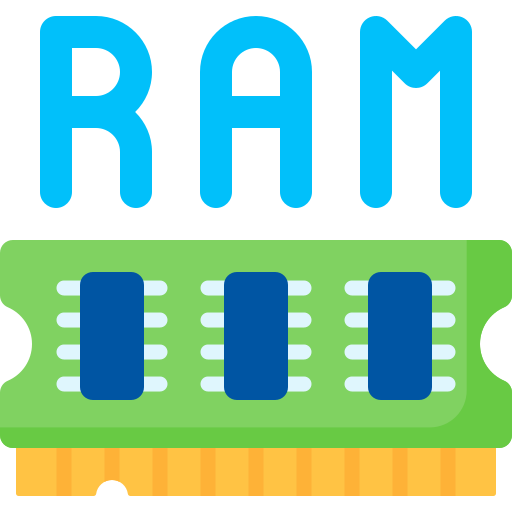
Efficient memory management is fundamental in C++ for crafting robust, high-performance applications. As with any aspect of programming, continuous learning and practice are key.
Start Learning Coding today and boost your Career Potential
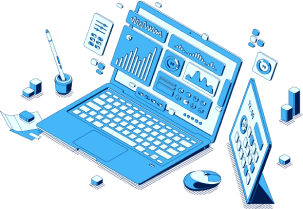
FAQs
Q: Is memory management in C++ more complex compared to other languages?
A: Yes, C++ gives programmers more control over memory management, which can be complex but allows for greater efficiency and performance optimization.
Q: Can smart pointers be used for all types of memory allocation?
A: Smart pointers are ideal for most dynamic memory allocations, but specific scenarios might require manual memory management.
Q: How does RAII help in memory management?
A: RAII ties resource allocation to object lifetime, ensuring that allocated resources are automatically released when an object goes out of scope.
Q: Are there tools to help detect memory leaks in C++?
A: Yes, tools like Valgrind, Visual Studio Diagnostics Tools, and others can detect memory leaks and analyze memory usage.
Q: What is the difference between stack and heap memory?
A: Stack memory is managed by the system and stores local variables and function calls, while heap memory is managed by the programmer for dynamic memory allocation.
Related courses
See All CoursesIntermediate
C++ Pointers and References
Unlock the Power of Memory Manipulation. Dive deep into the fundamentals of programming with this comprehensive course designed for beginners and individuals seeking to strengthen their knowledge on pointers and references in C++. Mastering these essential concepts is crucial for unleashing the full potential of your programming skills. Let's start!
Beginner
C++ Introduction
Start your path to becoming a skilled developer by mastering the foundational principles of programming through C++. Whether you're starting from scratch or already have some coding experience, this course will provide you with the solid foundation needed to become a proficient developer and open the doors to a wide range of career opportunities in software development and engineering. Let's study C++!
Introduction to Regular Expressions for Beginners
A Guide to Understanding and Using Regex in Programming
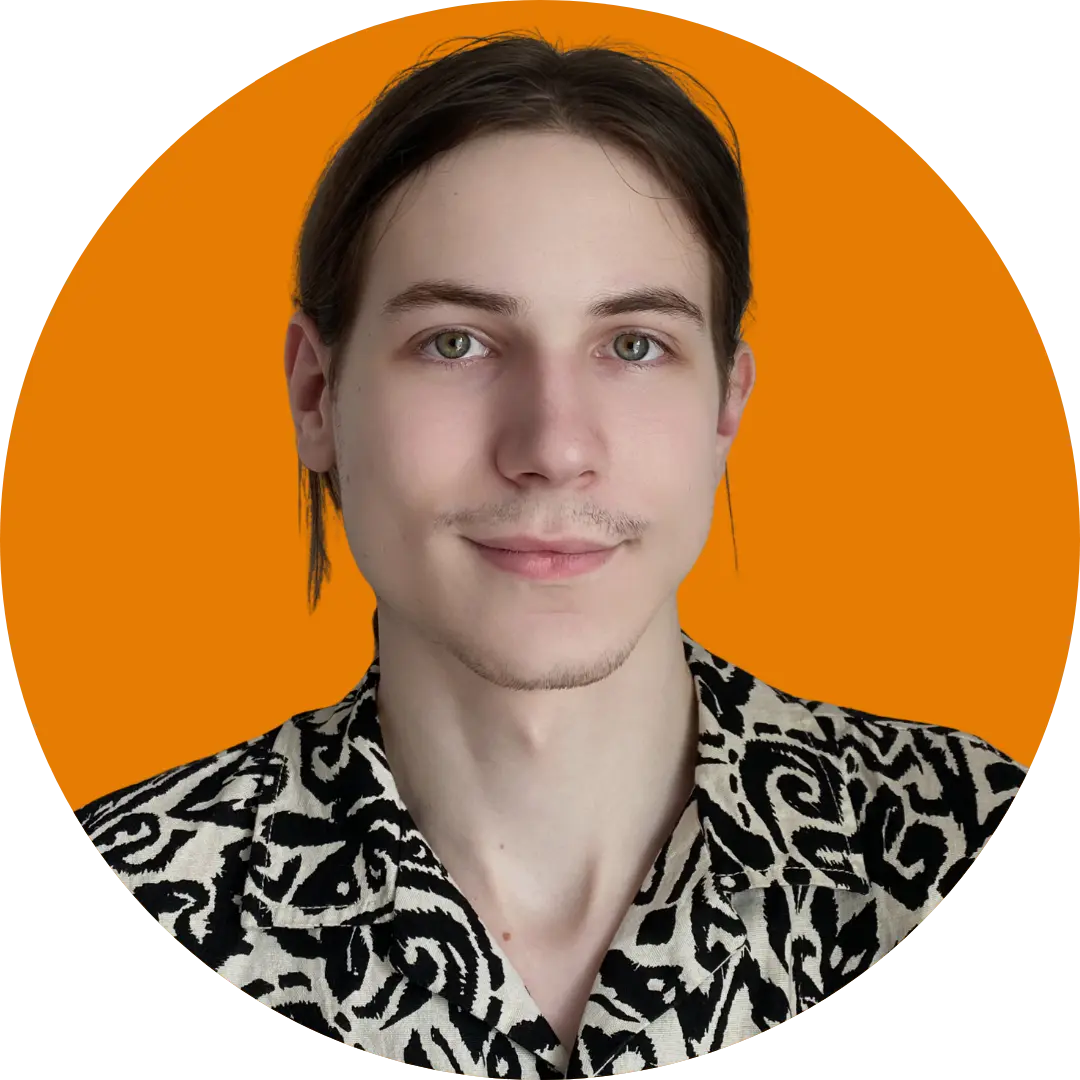
by Ihor Gudzyk
C++ Developer
Sep, 2024・13 min read
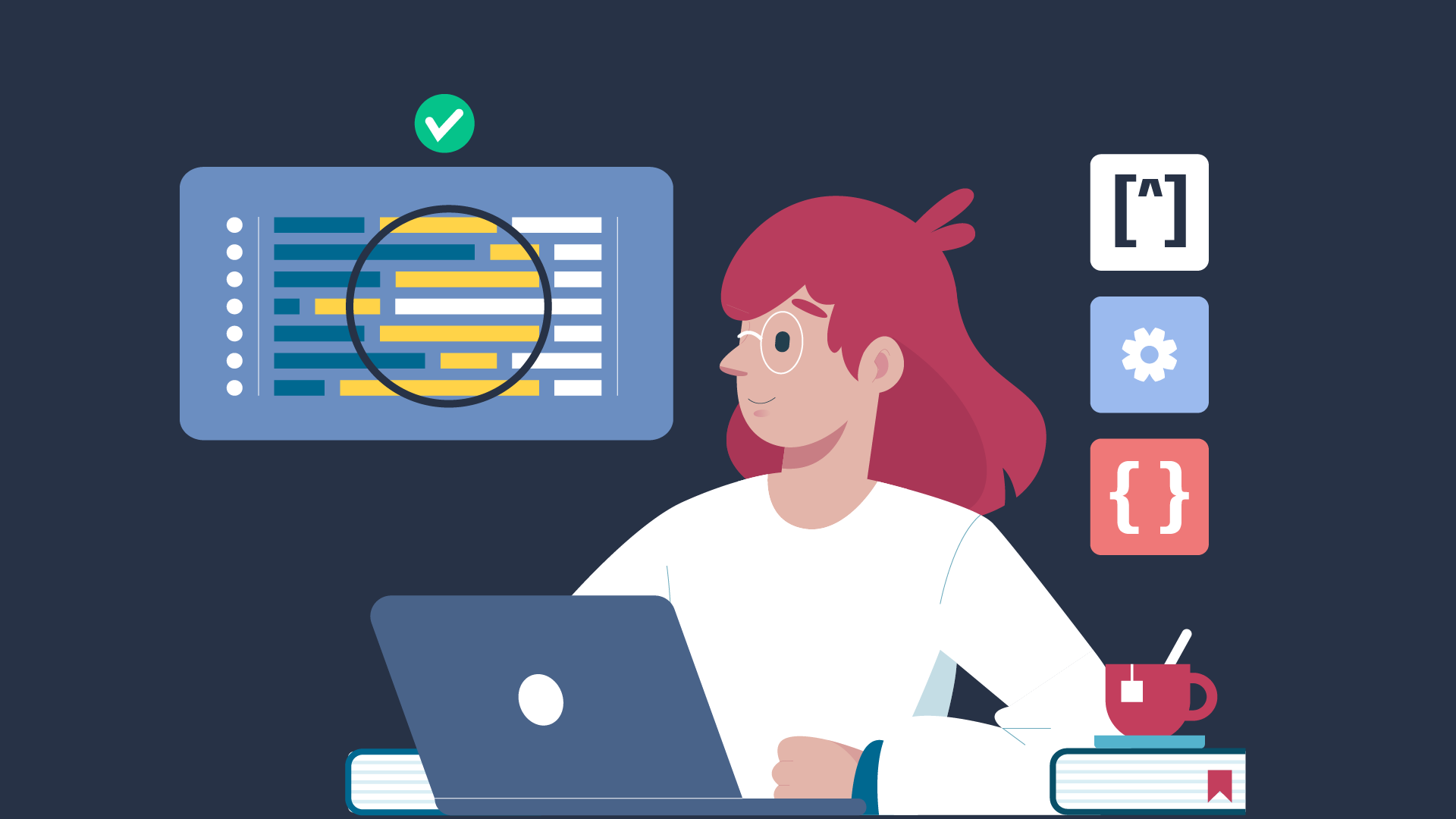
How Learning Coding Can Boost Your Career
Unlocking New Opportunities Through Coding Skills
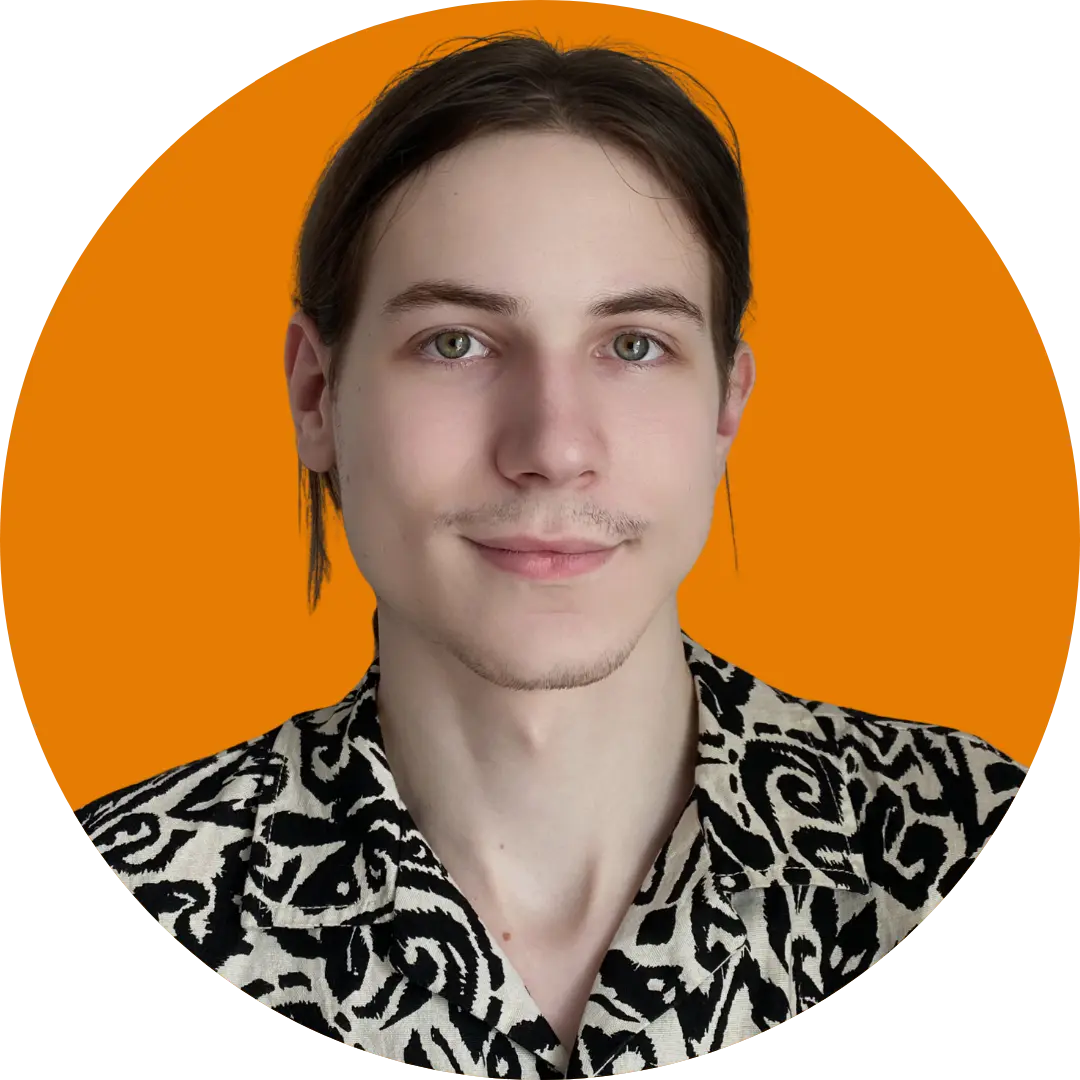
by Ihor Gudzyk
C++ Developer
Dec, 2023・4 min read
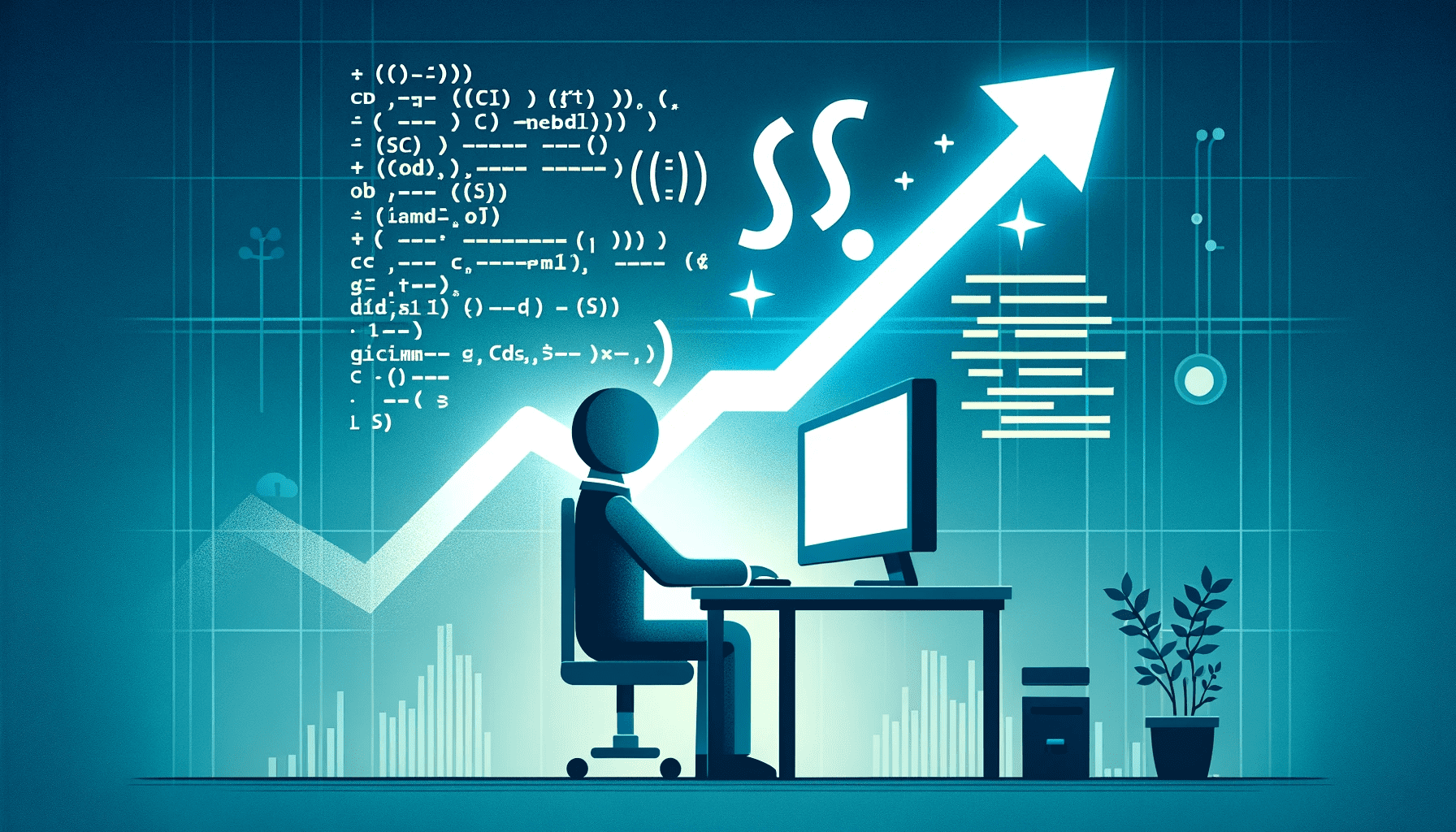
The DRY Principle
Maximizing Efficiency in Code and Development
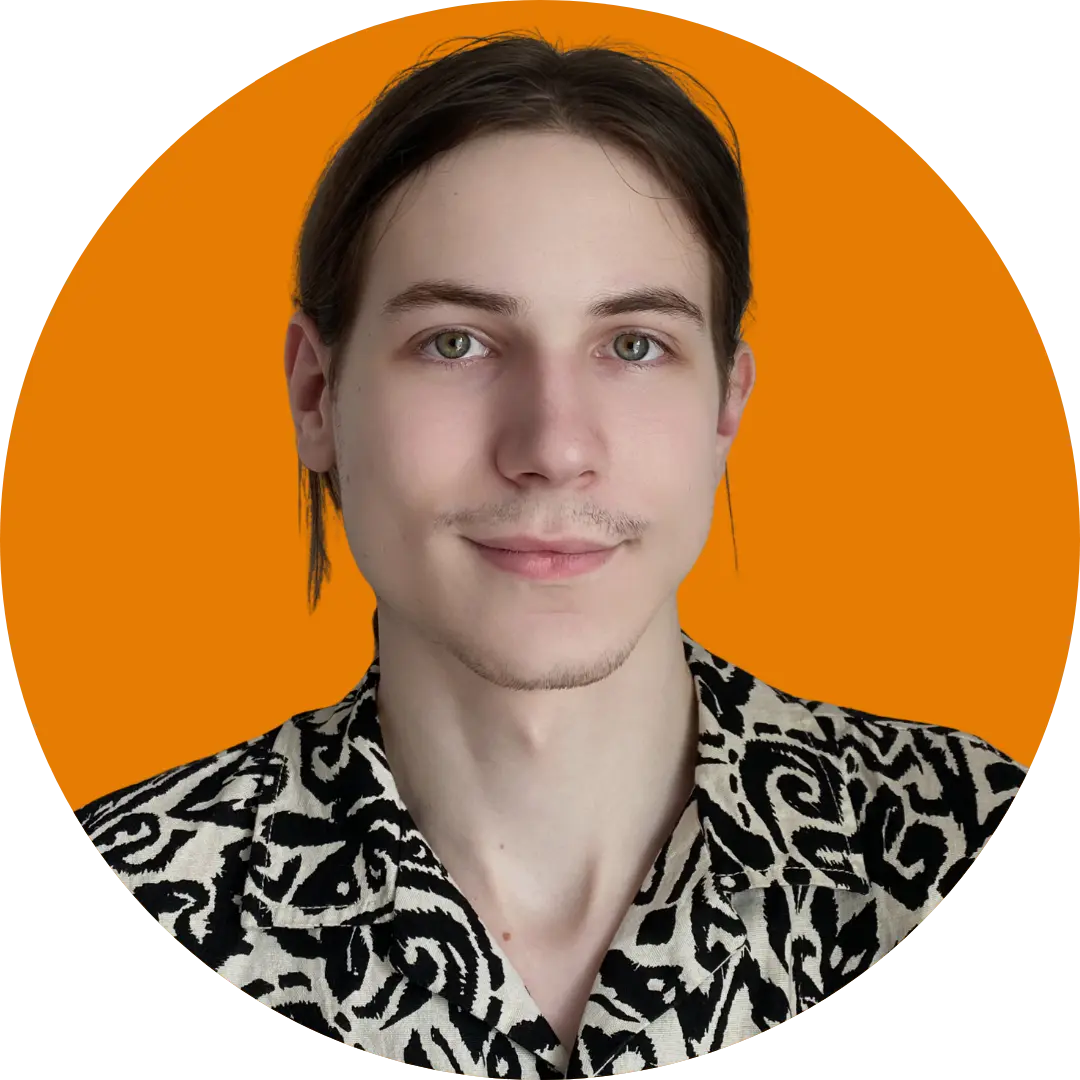
by Ihor Gudzyk
C++ Developer
Aug, 2024・9 min read
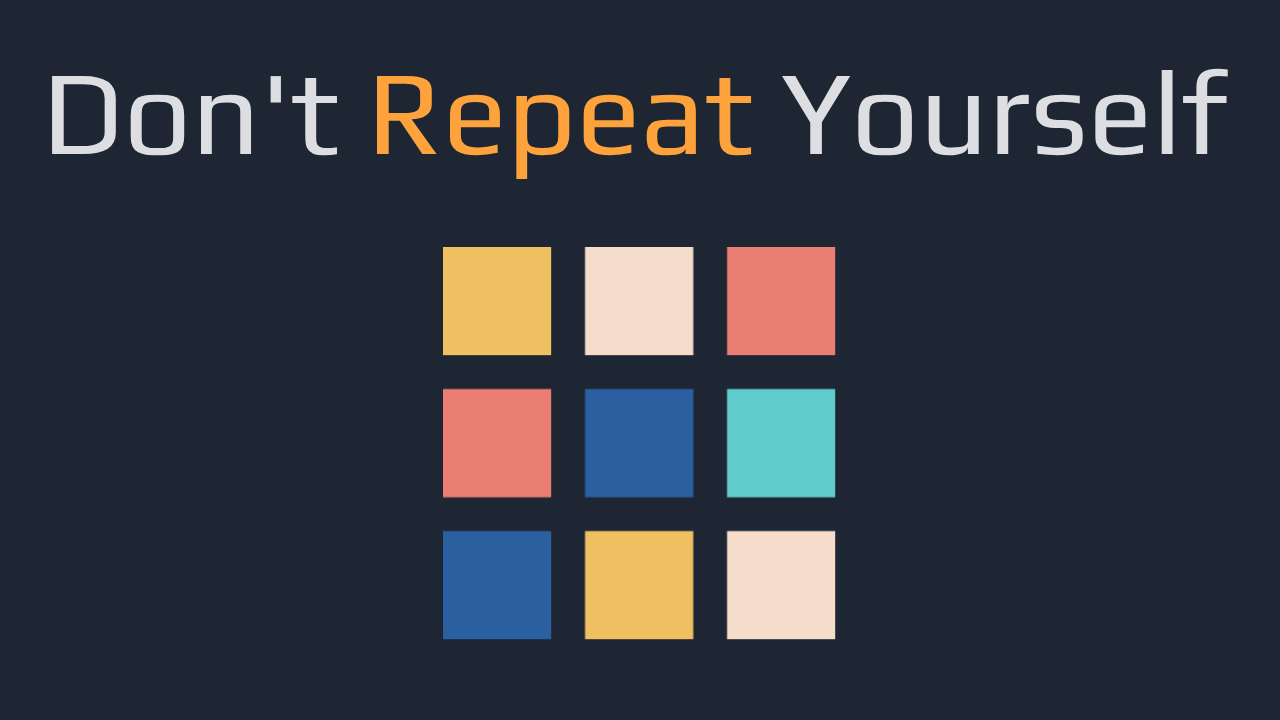
Content of this article