Related courses
See All CoursesAdvanced
State Management with Redux Toolkit in React
Discover the power of Redux Toolkit in conjunction with React, and supercharge your state management skills. This course comprehensively introduces Redux Toolkit, a powerful library for managing state. Learn how to streamline your Redux setup, simplify complex state logic, and create efficient, scalable React applications.
Intermediate
React Mastery
Take your web development skills to the next level with the course on building modern web applications using React. Learn to create dynamic user interfaces, efficiently manage state and data, and leverage the latest technologies and best practices in web development. Join now and unlock the full potential of React to create highly interactive and engaging web apps that meet the demands of today's users.
Advanced
Next.js 14 Mastery for Building Modern Web Apps
Next.js is a React framework designed for creating full-stack web applications. With Next.js, you utilize React to construct user interfaces while benefiting from additional features and optimizations provided by the framework.
React vs Angular - A Comprehensive Comparison
React vs Angular
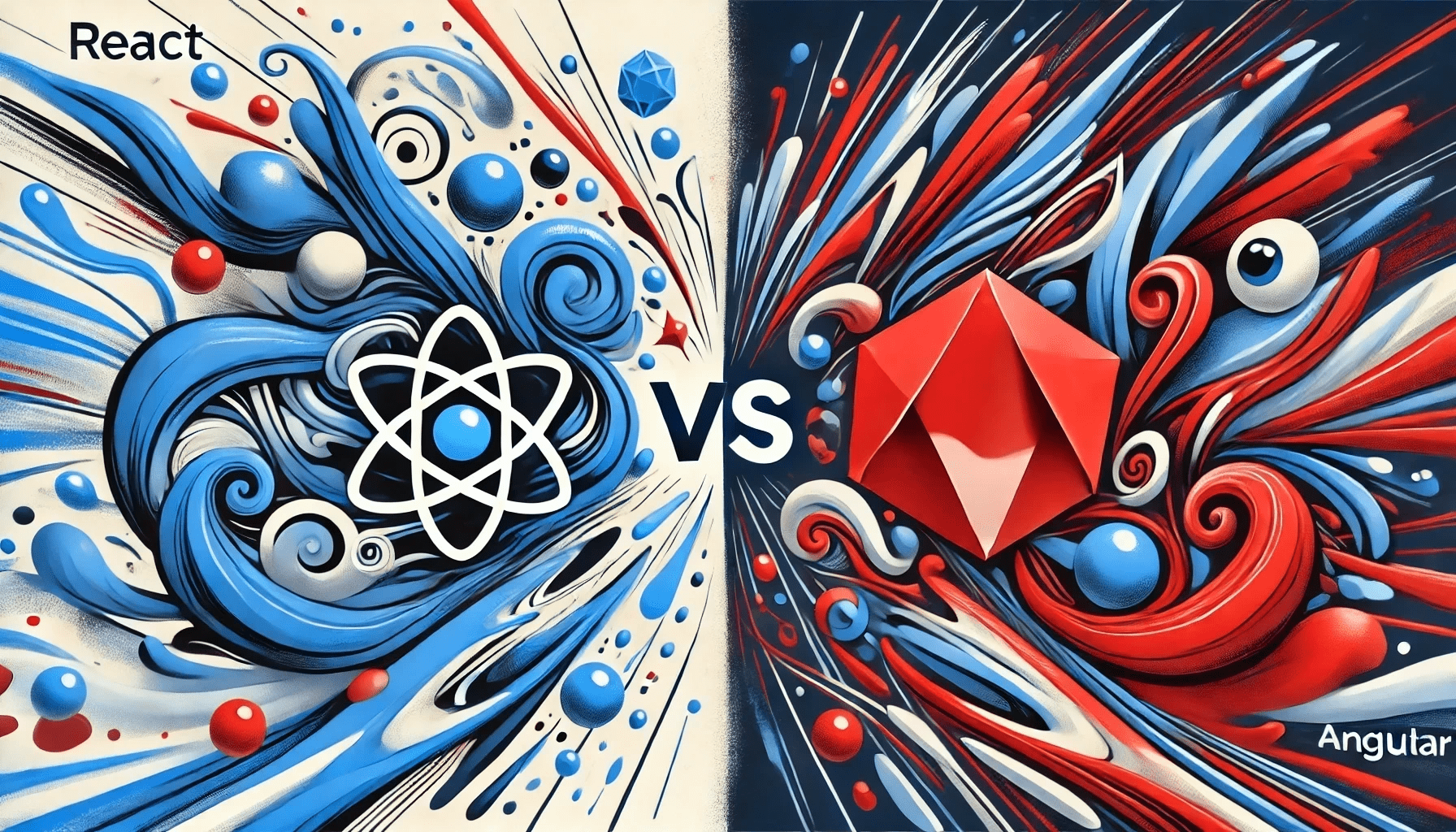
Introduction to React and Angular
What is React?
React is a JavaScript library developed by Facebook for building user interfaces. It is designed to make it easy to create interactive UIs with a component-based architecture, allowing developers to build encapsulated components that manage their own state. React is often used for single-page applications and aims to provide high performance and a responsive user experience.
What is Angular?
Angular is a comprehensive JavaScript framework developed by Google for building web applications. Unlike React, which is a library, Angular provides a full-fledged framework solution with built-in tools and libraries for routing, state management, and form validation. Angular is well-suited for creating complex, large-scale applications with its modular structure and two-way data binding capabilities.
History and Evolution of React and Angular
React was first released in 2013 and has undergone significant updates over the years. The release of React 16 introduced major improvements in performance and developer experience. Angular, originally released in 2010 as AngularJS, experienced a complete rewrite and rebranding in 2016 with Angular 2, now commonly referred to as Angular. This shift brought substantial changes, including a transition from a Model-View-Controller (MVC) architecture to a more component-based structure similar to React.
Core Concepts and Architecture
Component-Based Architecture in React
React's architecture revolves around components, which are reusable pieces of UI. Components can be either class-based or functional, with functional components gaining popularity due to the introduction of React Hooks, which allow state and side effects to be managed within functional components. This modular approach promotes reusability and maintainability.
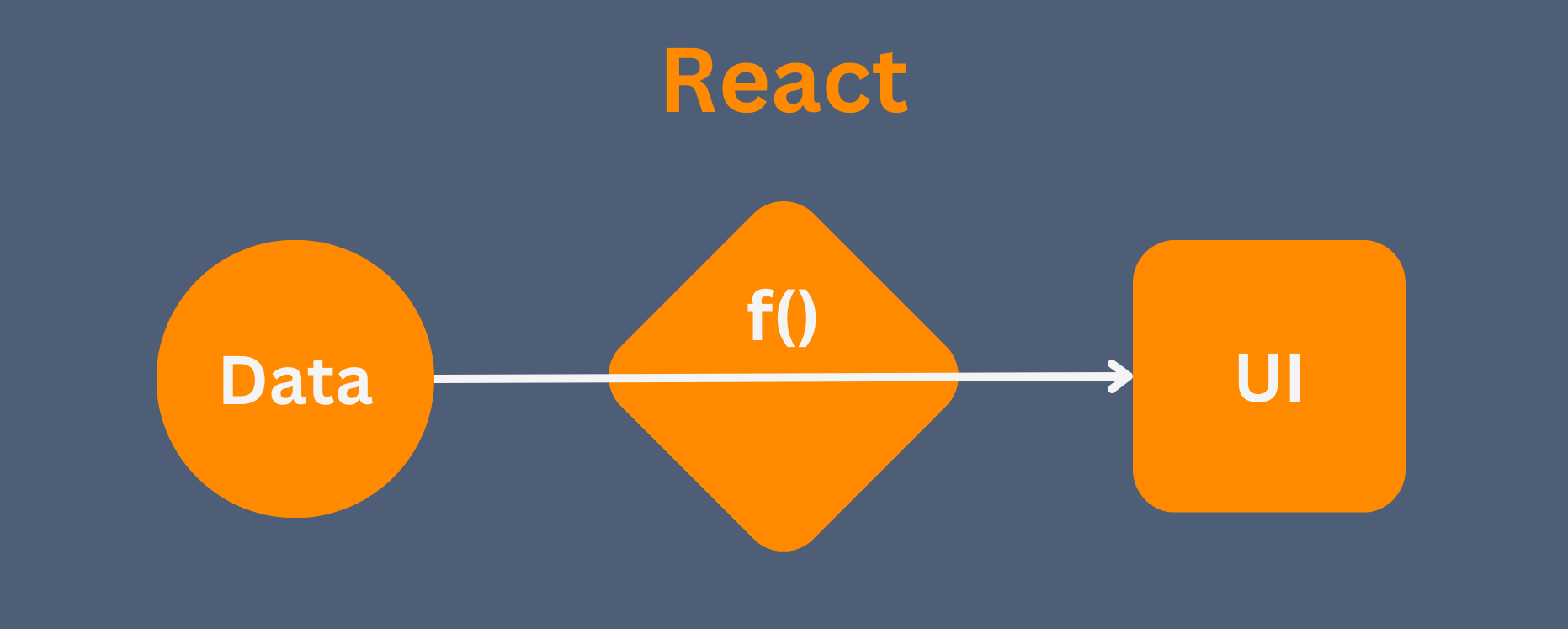
Module-Based Architecture in Angular
Angular uses a module-based architecture where an application is divided into distinct modules. Each module can contain components, services, directives, and pipes, encapsulating a specific functionality of the application. This modular structure helps in organizing and scaling large applications effectively.
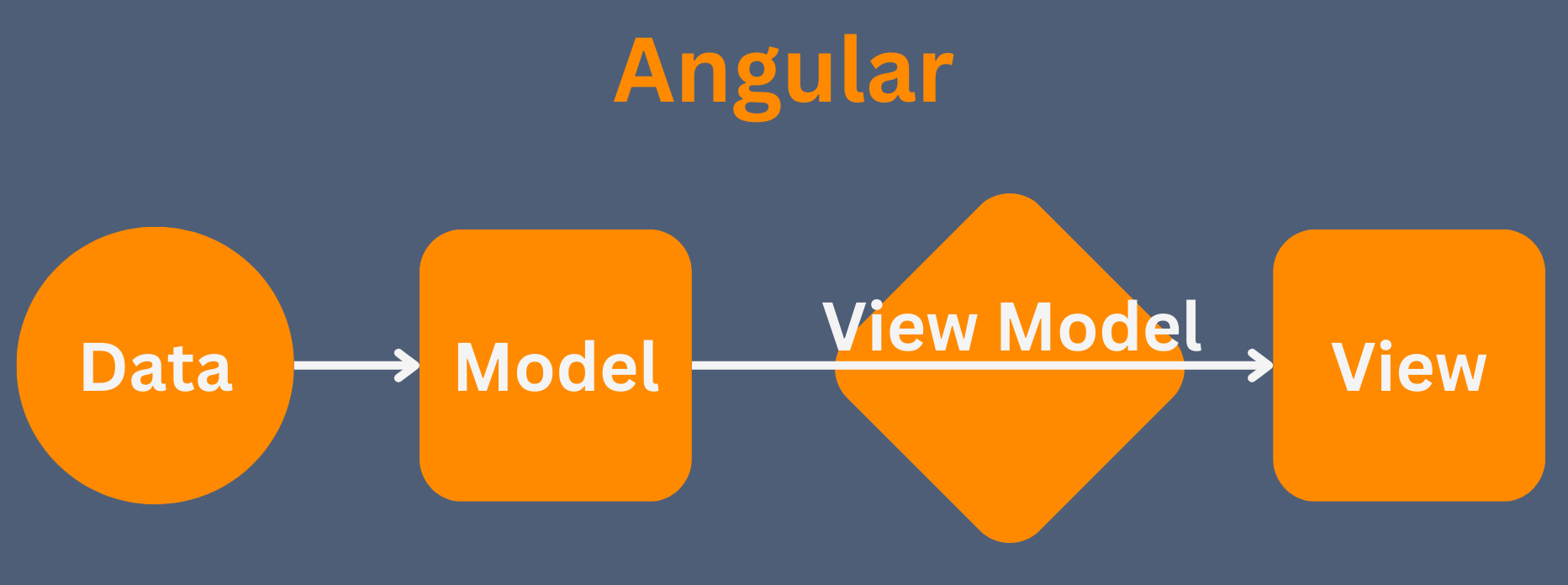
Data Binding: One-Way vs Two-Way
React employs one-way data binding, where data flows in a single direction from parent to child components. This unidirectional flow simplifies debugging and enhances performance, as changes in state are predictable and traceable.
Angular, on the other hand, supports two-way data binding, which allows for automatic synchronization of data between the model and the view. This means that changes in the UI are instantly reflected in the model and vice versa. While two-way data binding can accelerate development by reducing boilerplate code, it can also introduce complexity in larger applications.
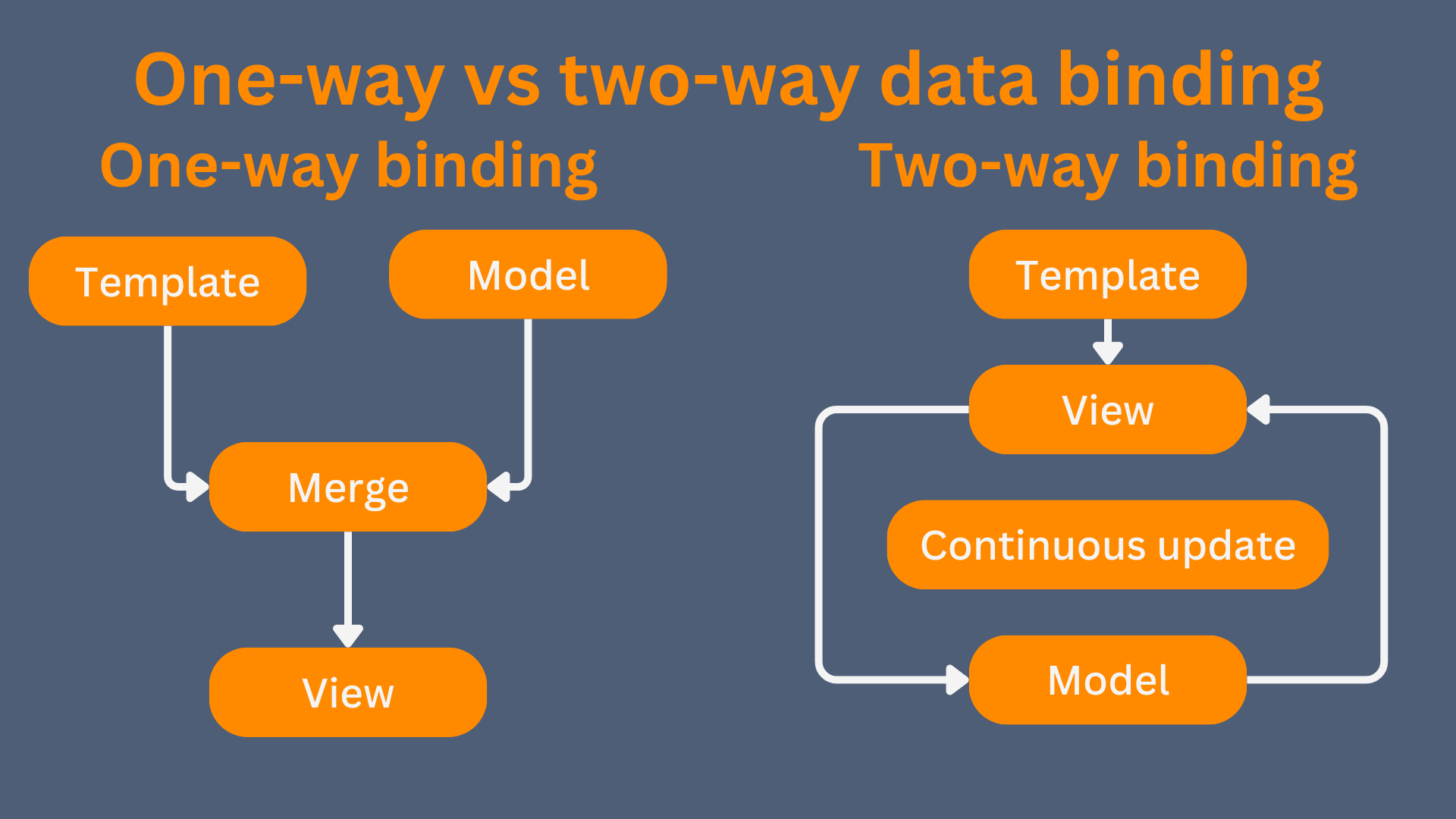
Performance and Optimization
React's Virtual DOM and Performance Benefits
React uses a virtual DOM (Document Object Model) to optimize rendering performance. The virtual DOM is a lightweight copy of the actual DOM, and React efficiently updates the real DOM only when necessary by comparing changes with the virtual DOM. This approach minimizes direct manipulation of the DOM, leading to faster updates and a smoother user experience.
Angular's Change Detection Mechanism
Angular employs a change detection mechanism to keep the UI in sync with the underlying data. This involves checking the state of the application and updating the DOM accordingly. Angular's change detection is optimized through techniques such as tree-shaking and Ahead-of-Time (AOT) compilation, which reduce the application's bundle size and improve runtime performance.
Performance Comparison: When to Use Each Framework
React's performance benefits make it ideal for applications with complex, interactive UIs and frequent updates. Its virtual DOM and efficient reconciliation process ensure high performance in these scenarios. Angular's strength lies in its comprehensive framework capabilities, making it suitable for large-scale applications with intricate data management requirements. The choice between React and Angular often depends on the specific needs and scale of the project.
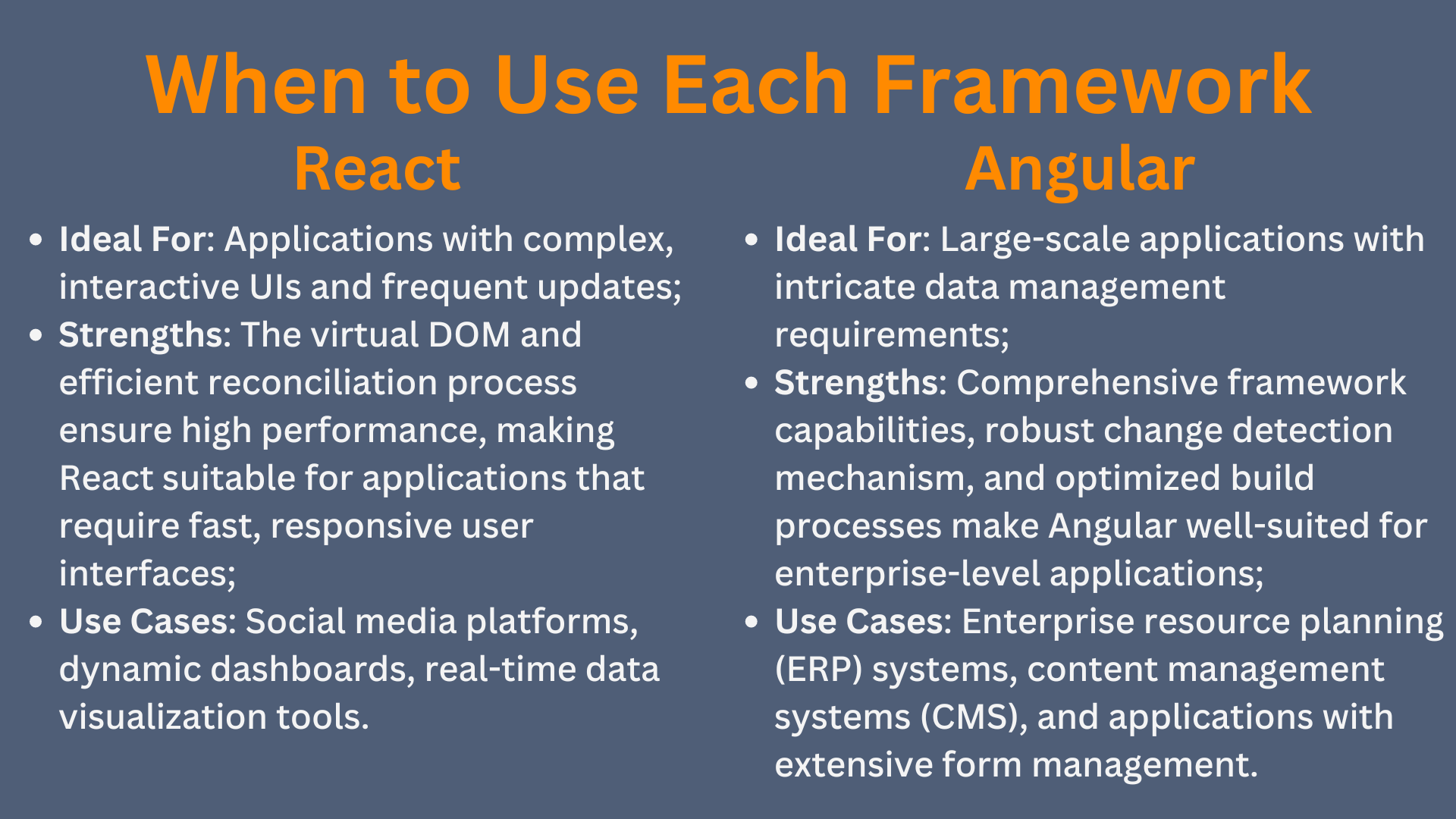
For a detailed performance comparison between React and Angular, including statistical analysis and practical insights, check out this Medium article on Angular VS React: Performance comparison with Google Web Vitals.
Run Code from Your Browser - No Installation Required
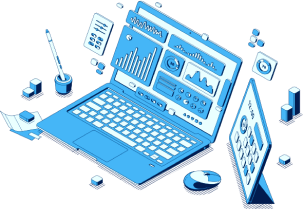
Development Experience
Learning Curve: React vs Angular
React's learning curve is relatively gentle, especially for developers familiar with JavaScript. Its simplicity and focus on building UI components make it accessible to beginners. On the other hand, Angular, with its comprehensive framework and more complex syntax, presents a steeper learning curve. However, Angular's extensive documentation and structured approach can be beneficial for developers seeking a robust, all-in-one solution.
To help you get started, here are some valuable resources for further learning:
React Learning Resources
- React Documentation - Comprehensive guide to all aspects of React, from basics to advanced topics;
- React Official Tutorial: Tic-Tac-Toe - A hands-on tutorial for building a small React application;
- Reactiflux Community - A large community of React developers discussing various topics;
- Stack Overflow React Tag - A vast collection of questions and answers about React.
Angular Learning Resources
- Angular Documentation - Detailed and structured documentation covering all features and best practices of Angular;
- Angular Official Tutorials - The official Angular tutorials that guide you through building Angular applications;
- Angular Community Discord - An active community of Angular developers;
- Stack Overflow Angular Tag - A comprehensive repository of questions and answers related to Angular.
Tooling and Ecosystem: Libraries and Frameworks
Both React and Angular boast robust ecosystems that provide a plethora of tools and libraries to enhance development efficiency and application performance. Here's a comparative overview of some of the most popular libraries and frameworks for each:
React Ecosystem
- Create React App
- Purpose: Sets up a new React project with a minimal configuration;
- Key Features: Boilerplate setup, build system, linting, and testing;
- Link: Create React App.
- Next.js
- Purpose: Framework for server-side rendering and static site generation;
- Key Features: API routes, dynamic routing, and optimized performance;
- Link: Next.js.
- Redux
- Purpose: State management library;
- Key Features: Centralized state, easy debugging, predictable state transitions;
- Link: Redux.
- React Router
- Purpose: Routing library for navigation;
- Key Features: Declarative routing, dynamic routing, nested routes;
- Link: React Router.
- Material-UI
- Purpose: UI framework following Material Design principles;
- Key Features: Pre-designed components, theming, responsive design;
- Link: Material UI.
Angular Ecosystem
- Angular CLI
- Purpose: Command-line interface tool;
- Key Features: Scaffolding, building, testing, and maintaining Angular applications;
- Link: The Angular CLI.
- NgRx
- Purpose: State management library;
- Key Features: Reactive state management, inspired by Redux;
- Link: NgRx.
- Angular Material
- Purpose: UI component library;
- Key Features: Material Design components, accessibility, and theming;
- Link: Angular Material.
- RxJS
- Purpose: Reactive programming library;
- Key Features: Observables for asynchronous programming, event handling;
- Link: RxJS.
- PrimeNG
- Purpose: Rich UI component suite;
- Key Features: Comprehensive set of UI components, themes, and templates;
- Link: PrimeNG.
Community Support and Resources
React and Angular both enjoy strong community support and extensive resources. React's community is known for its active contributions and a vast array of tutorials, courses, and third-party libraries. Angular's community is equally supportive, with comprehensive official documentation, community-driven blogs, and conferences dedicated to Angular development.
Aspect | React | Angular |
---|---|---|
Architecture | Library for building UI components. Uses a virtual DOM for efficient updates. React components are reusable and managed using state and props. | Full-fledged MVC (Model-View-Controller) framework. Uses real DOM. Angular provides a comprehensive solution with services, directives, and dependency injection. |
Data Binding | One-way data binding. Data flows in one direction, making it easier to debug and understand. | Two-way data binding. Changes in the UI and model are synchronized automatically, which simplifies development but can impact performance. |
Performance | High performance due to virtual DOM. React updates only the parts of the DOM that have changed. | Performance can be affected by two-way data binding and real DOM, but Angular's change detection and Ahead-of-Time (AOT) compilation improve performance. |
Learning Curve | Generally easier to learn, especially for developers with JavaScript experience. JSX syntax is straightforward. | Steeper learning curve due to the complexity of the framework and the requirement to learn TypeScript and Angular-specific concepts. |
Use Cases and Applications
When to Choose React
React is an excellent choice for applications that require high interactivity and dynamic user interfaces. Its component-based architecture and virtual DOM make it ideal for scenarios where performance and user experience are paramount. Key use cases include:
- Social Media Platforms: React's efficient updating and rendering capabilities make it well-suited for applications with frequent user interactions and real-time updates;
- E-commerce Sites: The ability to create interactive and responsive user interfaces helps enhance the shopping experience, driving engagement and conversions;
- Dashboards: React's modular components allow for the development of highly customizable and data-driven dashboards that can handle complex visualizations and real-time data updates.
When to Choose Angular
Angular excels in building enterprise-level applications with complex workflows and data management needs. Its modular architecture, built-in features, and strong typing with TypeScript make it suitable for large-scale projects. Key use cases include:
- Content Management Systems (CMS): Angular's structured approach and powerful form handling capabilities make it ideal for developing robust and scalable CMS solutions;
- Enterprise Resource Planning (ERP) Systems: The comprehensive framework and modular architecture of Angular support the development of intricate and integrated ERP systems that require strong data management and extensive functionality;
- Progressive Web Apps (PWAs): Angular's built-in tools and libraries facilitate the development of PWAs that offer a native app-like experience with offline capabilities and enhanced performance.
Case Studies: Successful Applications Built with React and Angular
React
Application | Description |
---|---|
The primary platform for React, leveraging its performance and flexibility to manage complex user interactions and real-time updates. | |
Utilizes React to deliver a fast, responsive user experience with real-time features like live stories and feed updates. | |
Implements React for its web application, providing seamless communication with a rich, interactive interface. | |
Airbnb | Uses React to create dynamic, interactive listings and booking interfaces, enhancing user engagement. |
Angular
Application | Description |
---|---|
Google Cloud Console | Built with Angular to manage complex workflows and provide a powerful, integrated interface for cloud services. |
Microsoft Office 365 | Utilizes Angular to create a cohesive, responsive interface for its suite of office applications. |
Upwork | Implements Angular for its robust, scalable platform, handling extensive functionality and large user bases. |
Forbes | Uses Angular to deliver a fast, engaging content management experience with efficient handling of large amounts of data and real-time updates. |
Feature/Use Case | React | Angular |
---|---|---|
Development Speed | Faster setup and development due to its component-based architecture and JSX syntax. | Steeper learning curve due to its comprehensive framework and TypeScript. |
Scalability | Highly scalable with a flexible architecture, ideal for building complex UIs. | Built-in tools and strong typing via TypeScript make it scalable for large enterprise applications. |
Learning Curve | Easier for developers with JavaScript experience; JSX syntax is straightforward. | Steeper learning curve due to TypeScript and extensive framework features. |
Performance | Virtual DOM ensures high performance for dynamic and interactive UIs. | Two-way data binding can impact performance, but Angular's change detection can optimize it. |
Data Binding | One-way data binding promotes unidirectional data flow, making debugging easier. | Two-way data binding simplifies form handling and real-time updates. |
State Management | Requires external libraries like Redux or Context API for state management. | Built-in services and dependency injection simplify state management. |
SEO Optimization | React can be optimized for SEO with server-side rendering (Next.js). | Angular Universal provides server-side rendering for SEO optimization. |
Flexibility and Customization | Highly flexible, allowing the use of different libraries and tools. | Provides a comprehensive solution out-of-the-box, but with less flexibility. |
Conclusion
Summary of Key Differences
Aspect | React | Angular |
---|---|---|
Architecture | Component-based architecture, promoting reusability and modularity. | Module-based architecture, ideal for organizing and scaling large applications. |
Data Binding | One-way data binding, simplifying debugging and enhancing performance. | Two-way data binding, enabling automatic synchronization between the model and the view. |
Performance | Utilizes a virtual DOM for efficient updates and a smoother user experience. | Employs a sophisticated change detection mechanism for real-time data synchronization. |
Learning Curve | Simpler and easier to learn, especially for developers familiar with JavaScript. | Comprehensive but more complex, requiring familiarity with TypeScript and its extensive features. |
Use Cases | Best suited for highly interactive UIs, such as social media platforms and dynamic dashboards. | Ideal for large-scale enterprise applications with complex workflows and data management needs. |
Final Recommendations for Developers
Choosing between React and Angular ultimately depends on the specific needs of your project. Here are some recommendations to guide your decision:
- Choose React if
- You need a flexible, high-performance solution for interactive UIs;
- Your project involves frequent updates and dynamic content;
- Your team prefers a simpler, component-based architecture with a gentler learning curve.
- Choose Angular if
- You are developing a large-scale application with complex data management requirements;
- You need a comprehensive framework with built-in solutions for routing, state management, and form validation;
- Your team is comfortable with TypeScript and requires a more structured development approach.
Ultimately, the best choice will depend on your project requirements, team expertise, and long-term maintenance considerations. Both React and Angular are powerful tools, and selecting the right one can significantly impact the success of your project.
Start Learning Coding today and boost your Career Potential
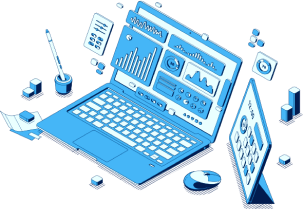
FAQs
What are the main differences in syntax between React and Angular?
React
React uses JSX, a syntax extension that allows HTML to be written within JavaScript. This approach makes it easier to create UI components by combining JavaScript logic and HTML structure in a single file. JSX enhances code readability and provides a seamless integration of JavaScript functionalities within the HTML.
Example: React Component using JSX
javascript
Angular
Angular uses TypeScript, which introduces static typing and additional features to JavaScript. It also employs Angular-specific syntax for templates and decorators. TypeScript's static typing helps catch errors at compile time and improves code maintainability. Angular templates use directives and bindings to create dynamic and responsive UIs.
Example: Angular Component using TypeScript
typescript
Β
Can I use React and Angular together in a project?
While it is technically possible to use both React and Angular in a single project, it is generally not recommended due to potential conflicts and increased complexity. Each framework has its ecosystem and best practices, and combining them can lead to maintenance challenges. If you need features from both, consider integrating them through micro frontends or isolating specific functionalities within separate modules.
What are the best practices for optimizing performance in React and Angular?
For React
- Use React.memo and useMemo: Prevent unnecessary re-renders by memoizing components and values;
- Optimize State Management: Utilize tools like Redux or the Context API to efficiently manage state;
- Code-Splitting and Lazy Loading: Reduce initial load time by splitting code and loading components only when needed.
For Angular
- OnPush Change Detection Strategy: Improve performance by using the OnPush strategy, which minimizes change detection cycles;
- Angular CLI's Optimization Features: Leverage built-in features for performance optimization, such as Ahead-of-Time (AOT) compilation and tree-shaking;
- Optimize Template Expressions: Avoid complex calculations within templates to reduce processing overhead.
How do React and Angular handle state management?
React
React relies on external libraries like Redux, MobX, or the Context API for state management. These libraries offer various approaches to managing application state and handling side effects. Redux provides a centralized state container, MobX facilitates reactive programming, and the Context API allows for lightweight state sharing across components.
Angular
Angular has built-in services and dependency injection for state management, often using RxJS for reactive state management. Services provide a way to share data and logic across components, while RxJS enables powerful reactive programming patterns to handle asynchronous data streams.
What are the job market trends for React and Angular developers?
Both React and Angular developers are in high demand, with distinct market trends for each:
React
React developers are often more popular for front-end roles due to React's widespread adoption, ease of learning, and flexibility. Many startups and tech companies prefer React for its simplicity and performance, leading to a high demand for React developers.
Angular
Angular is favored for enterprise-level applications, and its demand is strong in companies looking for comprehensive framework solutions. Organizations with complex, large-scale projects often choose Angular for its robustness and extensive built-in features. Angular developers are sought after in industries like finance, healthcare, and government.
For a more in-depth exploration of the popularity of both React and Angular, it is recommended to refer to the Stack Overflow survey, especially focusing on:
- Most Popular Technologies Chart - Stack Overflow Survey 2024 - Most Popular Technologies;
- Admired and Desired Chart - Stack Overflow Survey 2024 - Admired and Desired;
- Worked with vs Want to Work with Chart - Stack Overflow Survey 2024 - Worked with vs Want to Work With.
Related courses
See All CoursesAdvanced
State Management with Redux Toolkit in React
Discover the power of Redux Toolkit in conjunction with React, and supercharge your state management skills. This course comprehensively introduces Redux Toolkit, a powerful library for managing state. Learn how to streamline your Redux setup, simplify complex state logic, and create efficient, scalable React applications.
Intermediate
React Mastery
Take your web development skills to the next level with the course on building modern web applications using React. Learn to create dynamic user interfaces, efficiently manage state and data, and leverage the latest technologies and best practices in web development. Join now and unlock the full potential of React to create highly interactive and engaging web apps that meet the demands of today's users.
Advanced
Next.js 14 Mastery for Building Modern Web Apps
Next.js is a React framework designed for creating full-stack web applications. With Next.js, you utilize React to construct user interfaces while benefiting from additional features and optimizations provided by the framework.
Navigating the YouTube Influencer Maze in Your Tech Career
Staying Focused and Disciplined Amidst Overwhelming Advice
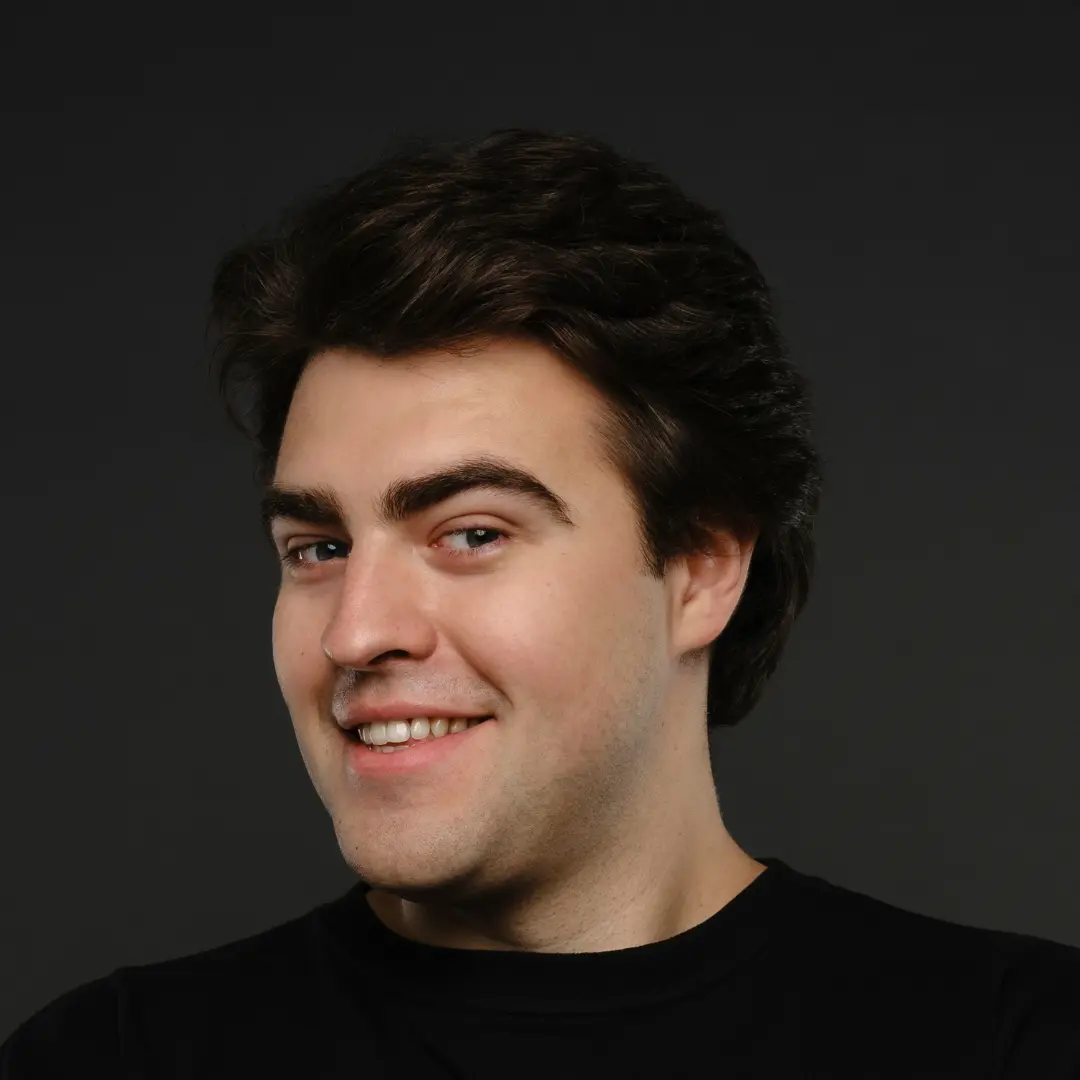
by Oleh Subotin
Full Stack Developer
Jul, 2024γ»5 min read
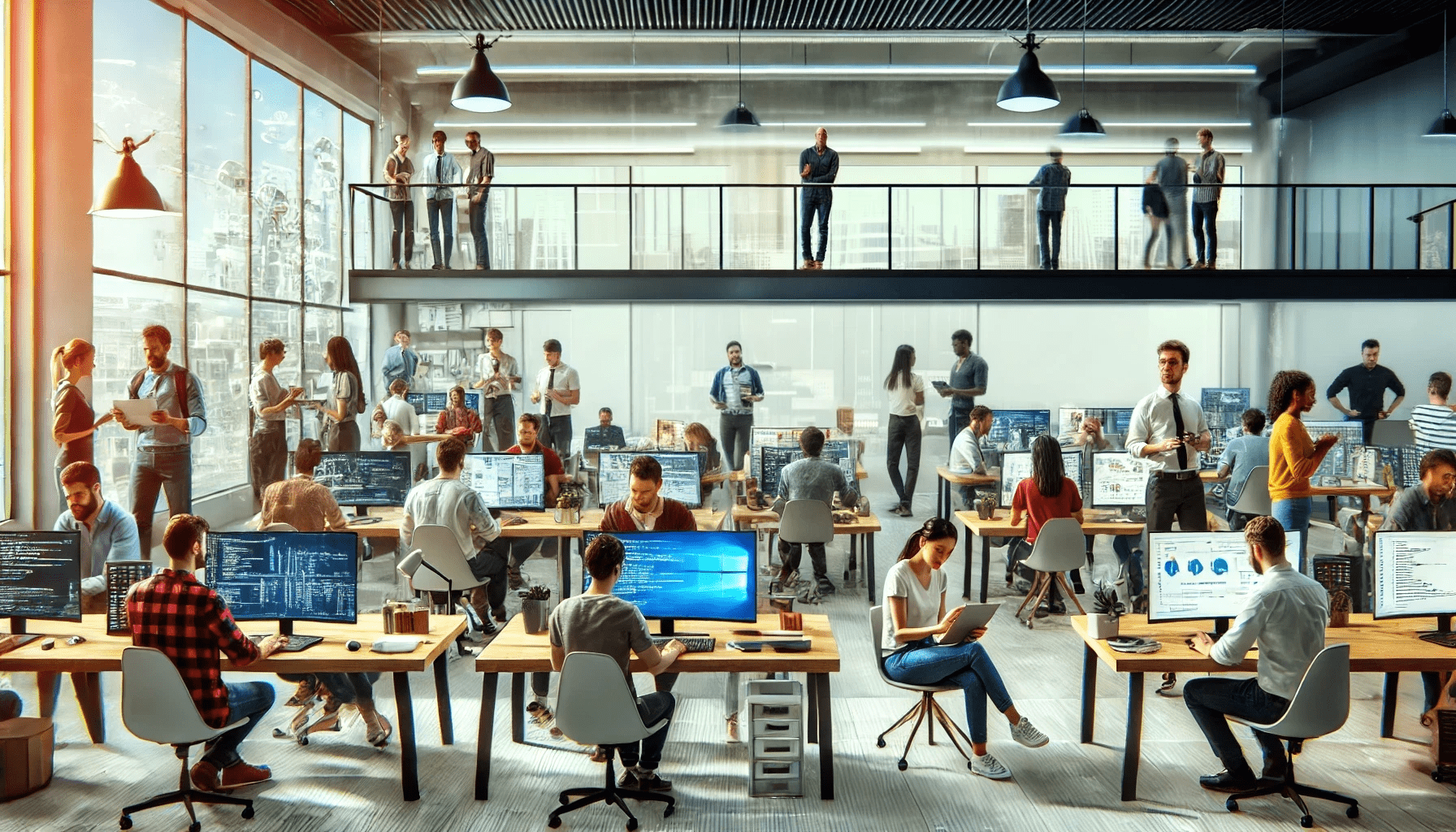
Accidental Innovation in Web Development
Product Development
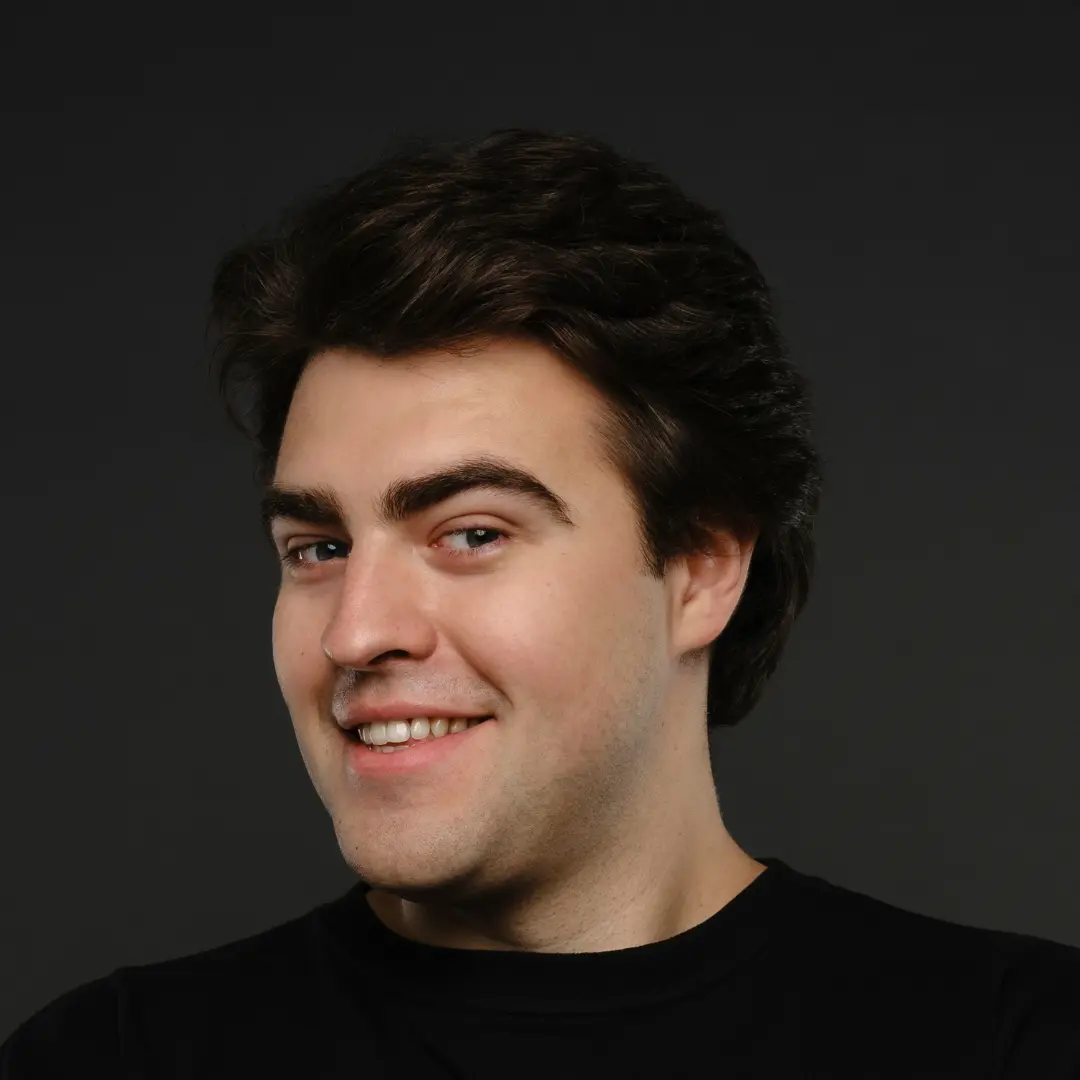
by Oleh Subotin
Full Stack Developer
May, 2024γ»5 min read
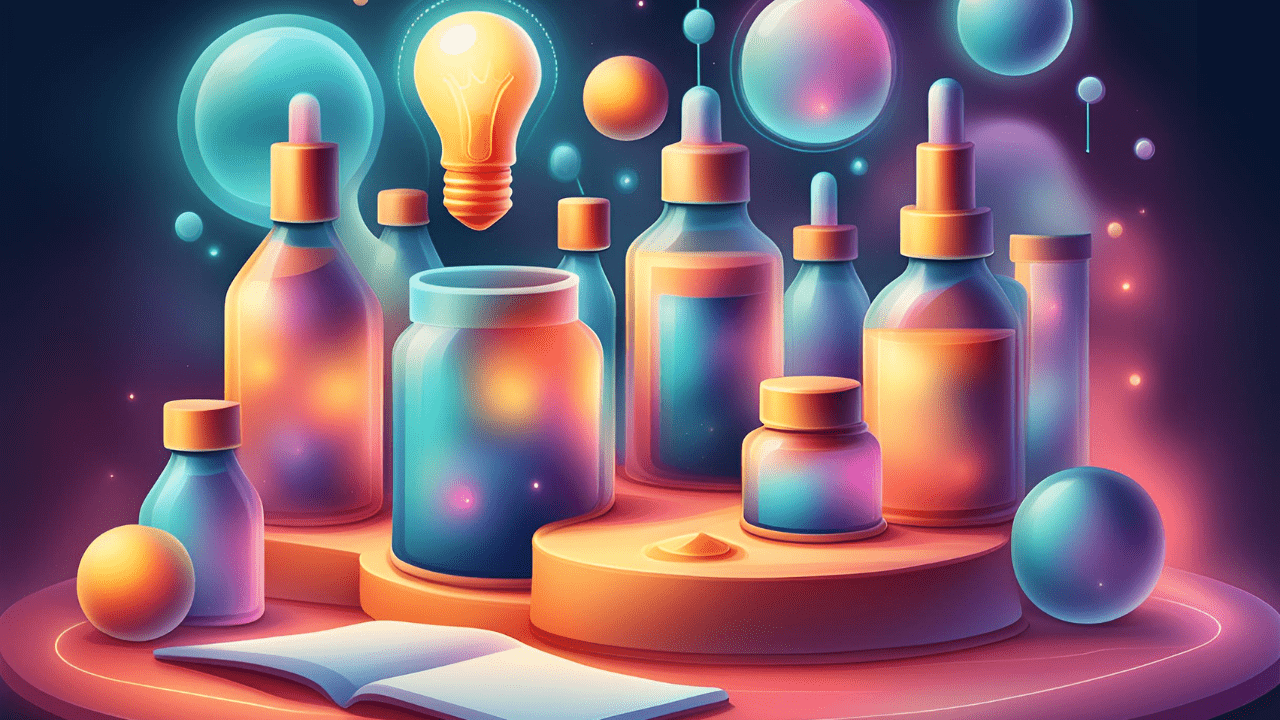
Is a College Degree Essential for a Career as a Developer
Evaluating the Role of Formal Education in Your Tech Career
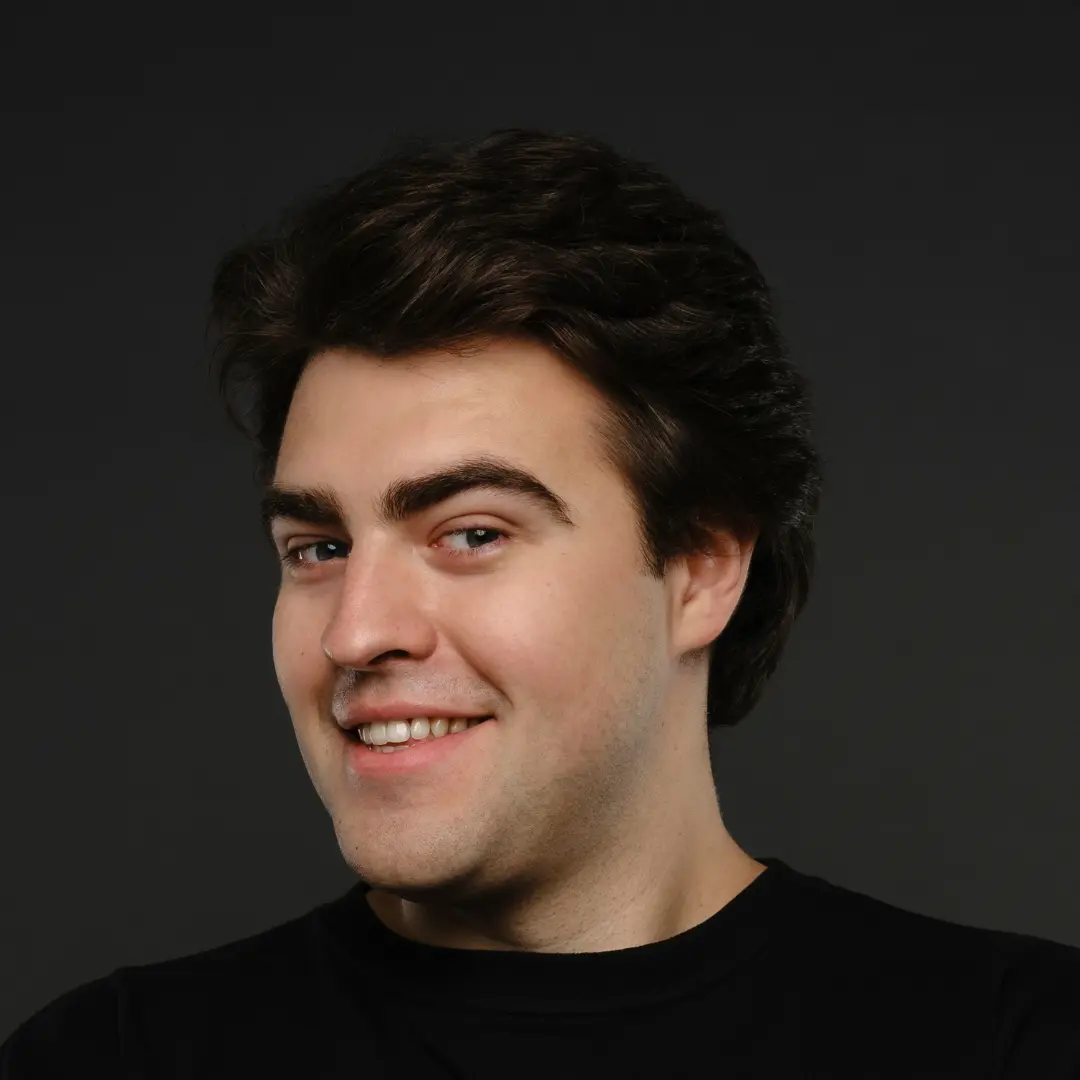
by Oleh Subotin
Full Stack Developer
Jul, 2024γ»6 min read
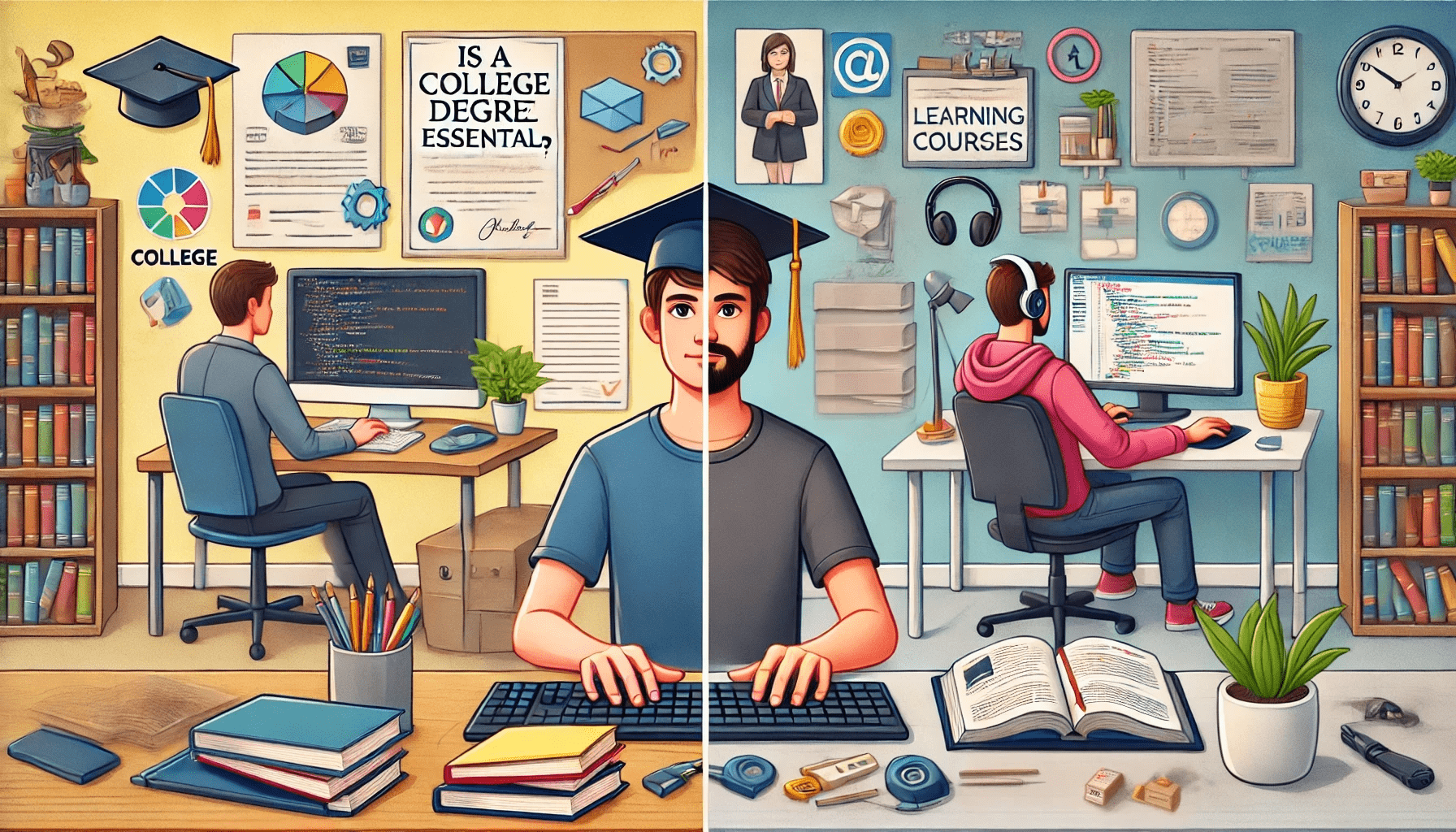
Content of this article