Related courses
See All CoursesReal-Time Notification System with Node.js and WebSockets
Implementing Real-Time Notifications for Targeted User Updates Across Multiple Servers
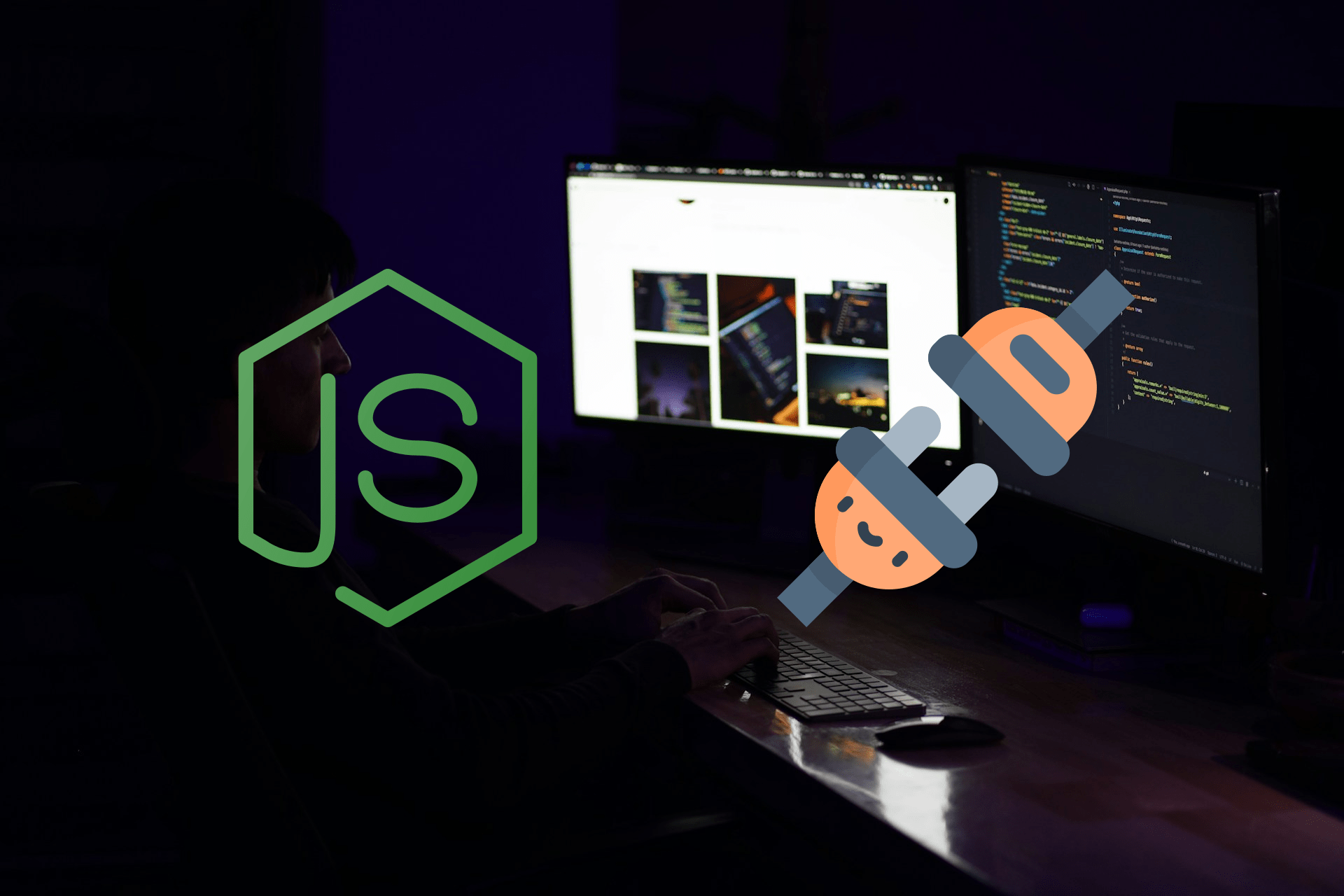
Let's walk through building a notification system where users receive real-time messages, like updates or alerts, instantly. We'll use Node.js and WebSockets to manage real-time communication, creating a simple yet effective setup for delivering instant notifications to connected clients.
System Overview
Set Up the Project and Install Dependencies
To get started, let's create a new project and install the necessary libraries.
bash
Install required packages.
bash
express
: a lightweight web framework to handle HTTP requests;ws
: a WebSocket library for Node.js that enables us to set up a WebSocket server.
Run Code from Your Browser - No Installation Required
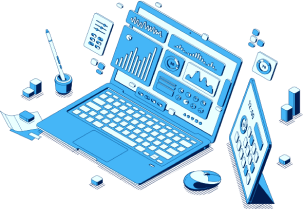
Setting Up the Server with WebSocket Connections
Now, we'll set up a Node.js server that will handle WebSocket connections. This server will allow clients to connect and stay connected, enabling the server to push notifications in real time.
Create server.js
and add the following code. This file will contain our server code.
js
Explanation
- WebSocket Connections: When a client connects to the server via WebSocket, the server keeps that connection open, allowing real-time communication. The
wss.on('connection', ...)
function handles these connections; - Notification Endpoint: The
POST /notify
endpoint accepts a notification message and broadcasts it to all connected clients, ensuring that each client receives updates immediately.
Creating a Client to Display Notifications
To test the notification system, let's create a simple HTML file that connects to our WebSocket server and displays notifications as they arrive.
Create a file called index.html
in the same project folder and add the following code to index.html
:
html
Explanation
- Client Connection: The client connects to the WebSocket server at
ws://localhost:8080
; - Real-Time Display: Each time a message is received, it is displayed in the
notifications
div, providing immediate feedback on notifications.
By opening this file in multiple tabs, you can simulate different users connected to the server, each able to receive notifications in real time.
Running and Testing the Notification System
- In the terminal, run the server:
We should seebashServer is listening on port 8080
in the terminal, confirming that the server is running; - Open the Client (
index.html
) in a Browser: Open this file in multiple tabs to simulate multiple users connected to the WebSocket server; - Send a Notification Using
curl
: Open a new terminal and use the following curl command to send a notificationbash
Each open tab with index.html
should display the notification message "New alert!" in real time, demonstrating the immediate, server-to-client communication.
Start Learning Coding today and boost your Career Potential
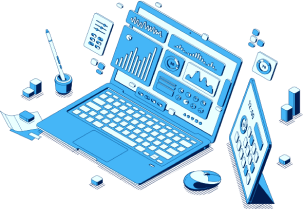
Real-World Applications and Considerations
- Real-Time Updates: This setup is ideal for applications that require real-time updates, such as chat systems, alert notifications, or live comments;
- Scalability: For more complex, high-traffic systems, you could extend this system by adding Redis or using a message broker. Load balancers or an API gateway could also be added to distribute WebSocket connections across multiple servers;
- Authentication: In production systems, you would typically secure WebSocket connections by authenticating users before allowing them to connect, ensuring only authorized clients receive notifications.
With these foundations, you have a functional notification system that is efficient and suited for real-time applications requiring instantaneous updates.
Conclusion
We built a real-time notification system using Node.js and WebSockets, providing a simple yet effective way to deliver instant updates to users. This setup, ideal for applications that require immediate notifications, lays a foundation for scalable real-time systems.
FAQs
Q: What is the main purpose of WebSockets in this system?
A: WebSockets allow persistent, two-way communication between the server and clients, enabling the server to push notifications instantly without requiring the client to keep requesting updates.
Q: Can this setup send notifications to specific users instead of all clients?
A: This basic setup broadcasts to all clients, but you can add logic to identify users and send messages to specific clients based on user IDs or session information.
Q: How can I secure WebSocket connections in this setup?
A: In production, use HTTPS (wss://) to encrypt WebSocket data and authenticate users before allowing WebSocket connections.
Q: How would I store past notifications?
A: Integrate a database (like MongoDB or PostgreSQL) to store notifications, enabling clients to access a history of alerts or messages.
Q: What's the best way to test this system?
A: Open multiple tabs with index.html
as different clients, then send notifications using a curl
command to confirm that each client receives real-time updates.
Related courses
See All CoursesAccidental Innovation in Web Development
Product Development
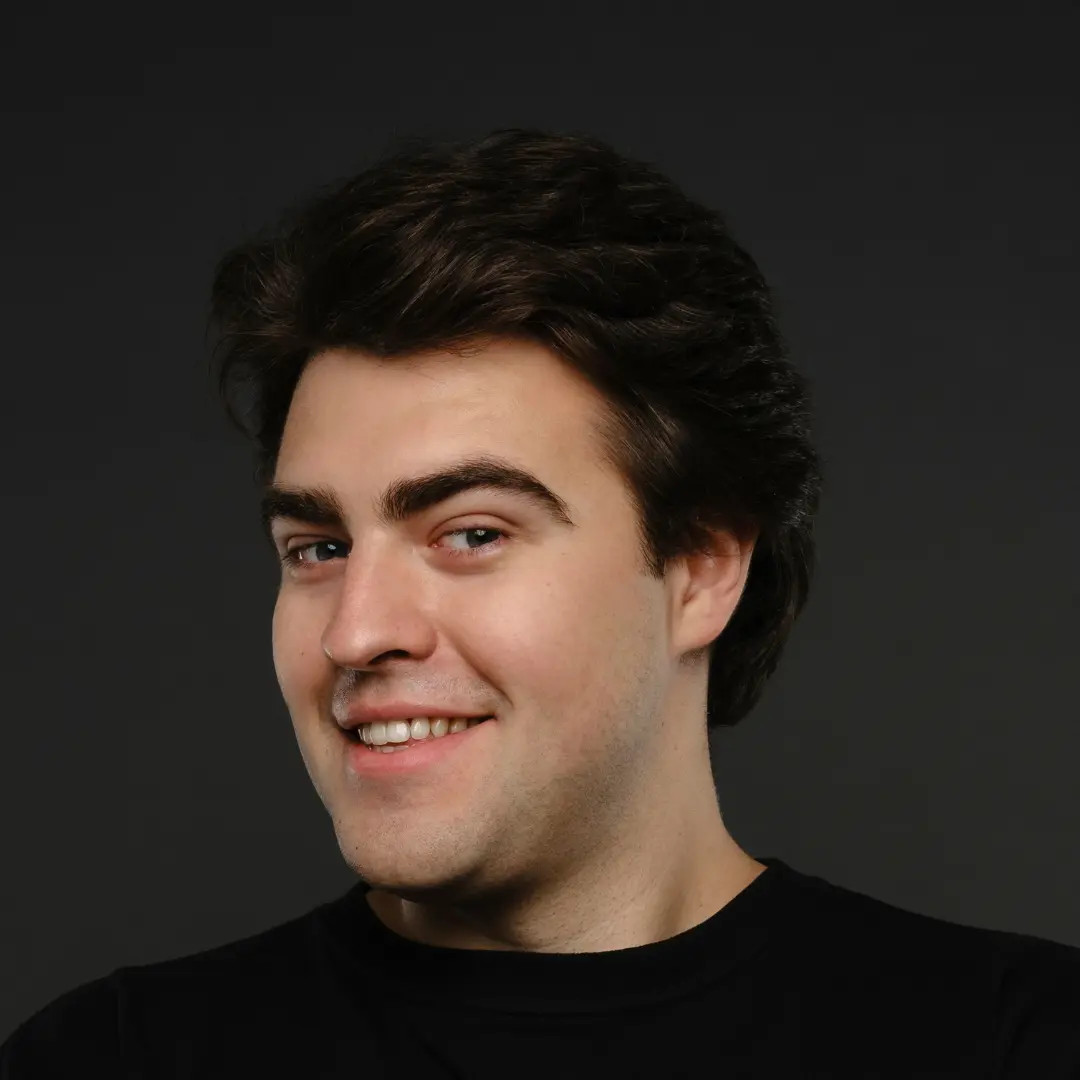
by Oleh Subotin
Full Stack Developer
May, 2024γ»5 min read
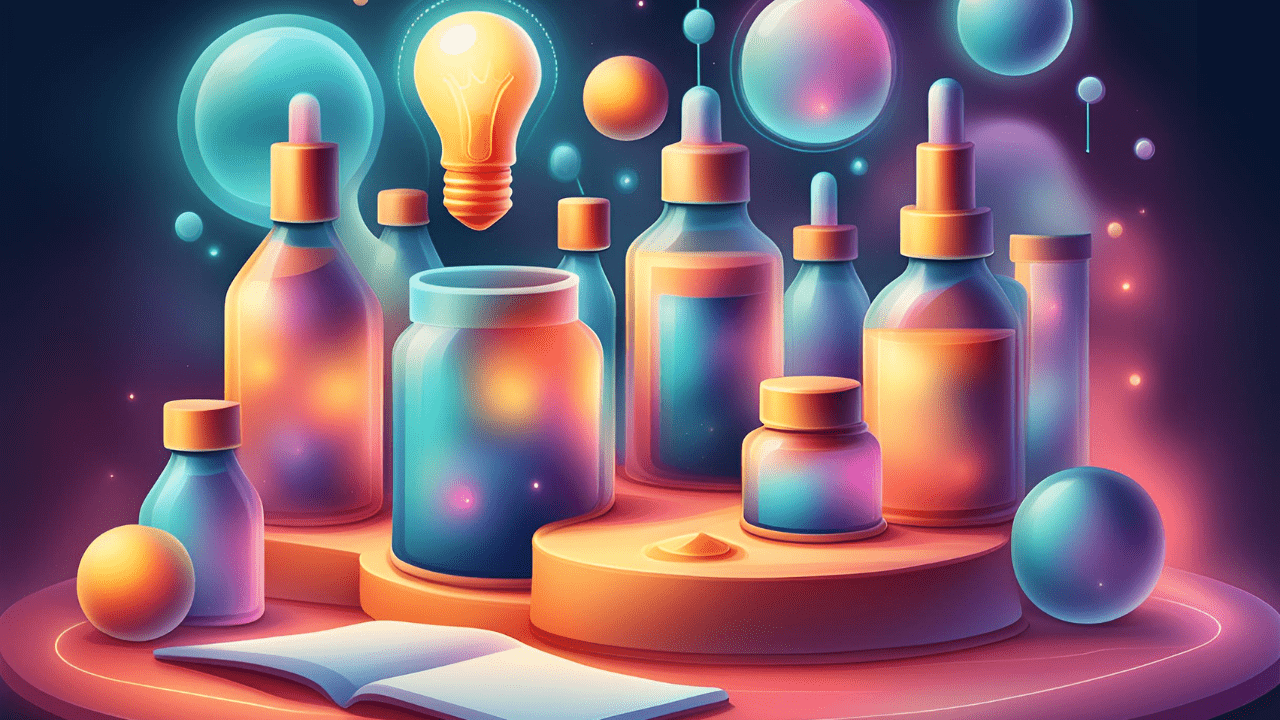
Navigating the YouTube Influencer Maze in Your Tech Career
Staying Focused and Disciplined Amidst Overwhelming Advice
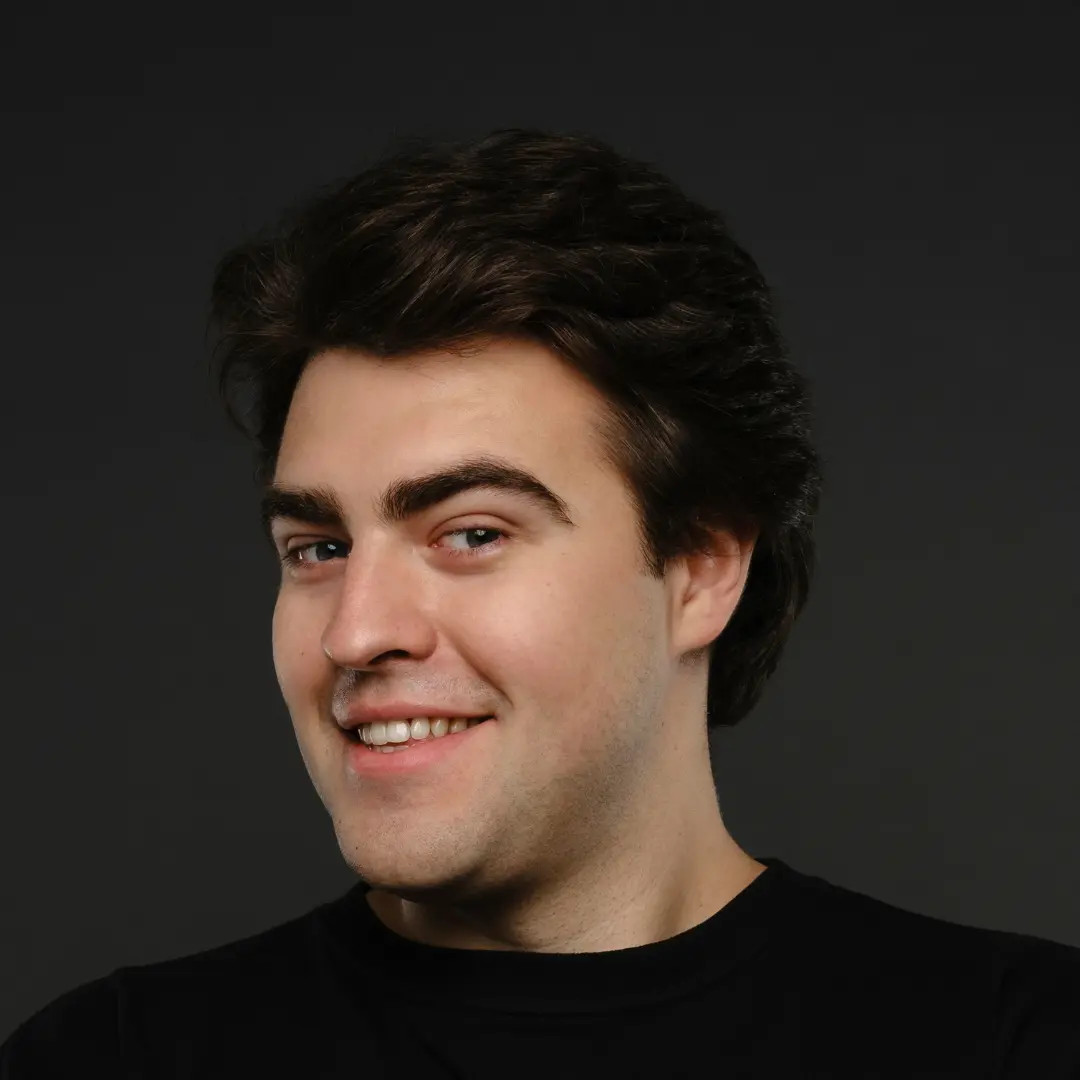
by Oleh Subotin
Full Stack Developer
Jul, 2024γ»5 min read
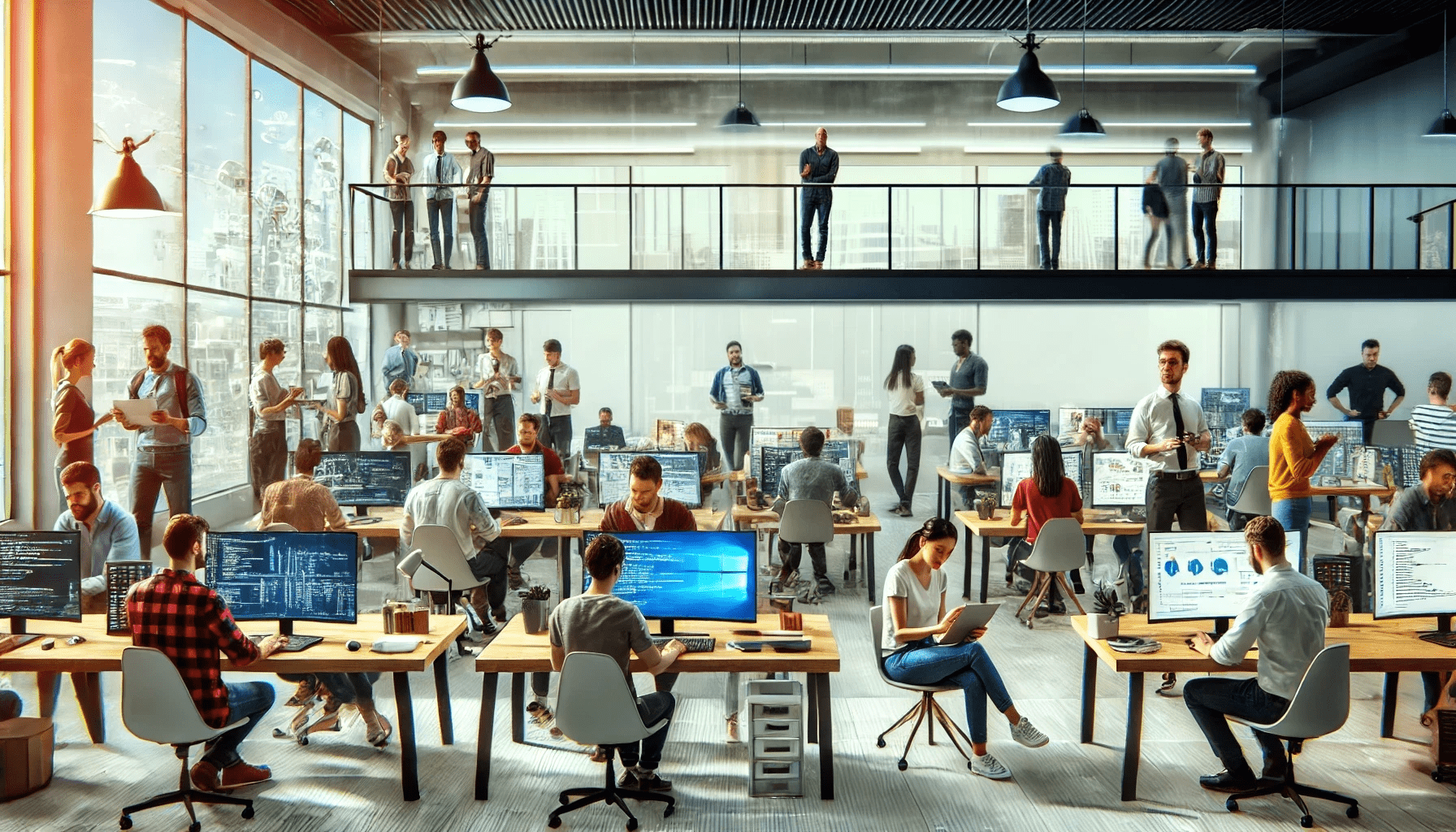
Is a College Degree Essential for a Career as a Developer
Evaluating the Role of Formal Education in Your Tech Career
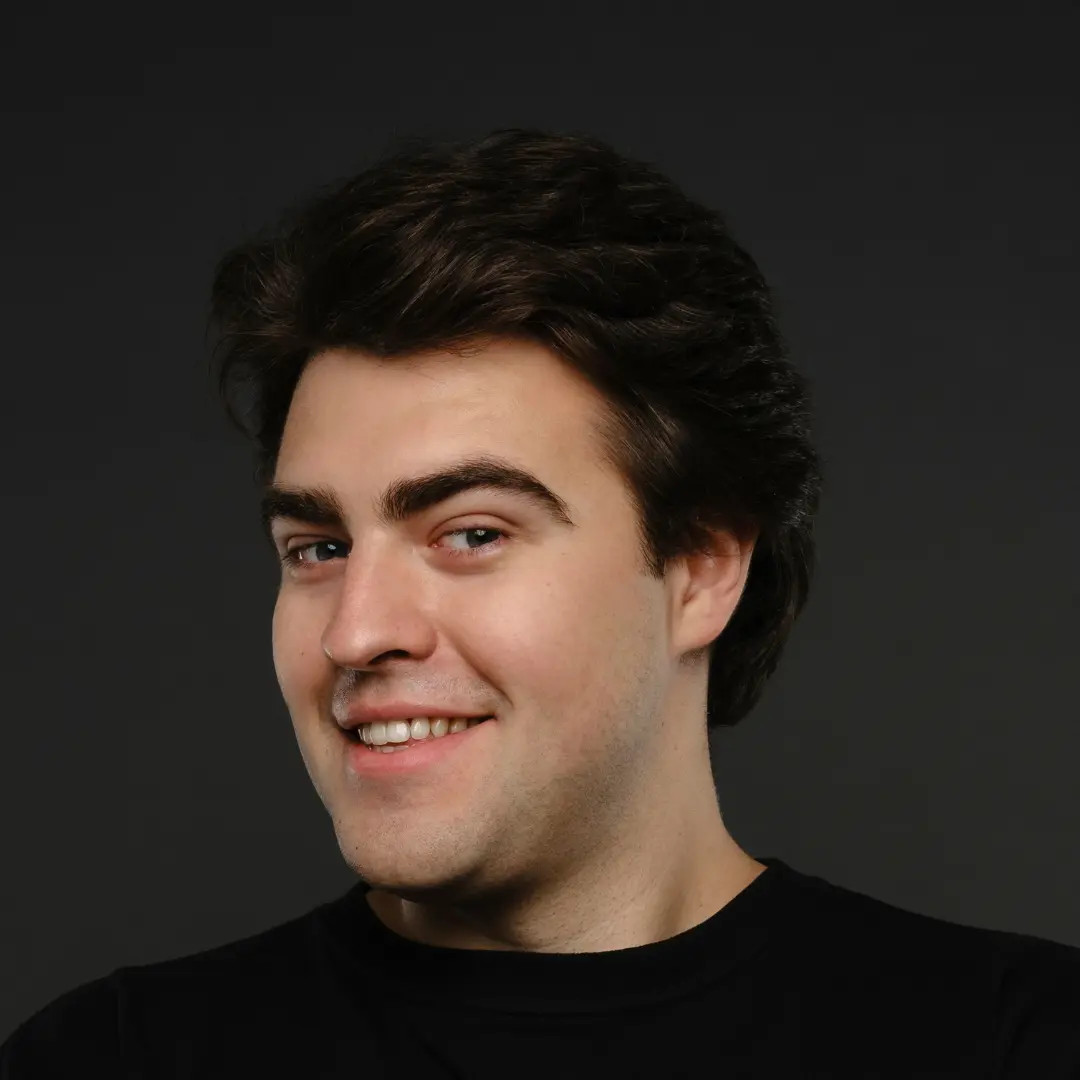
by Oleh Subotin
Full Stack Developer
Jul, 2024γ»6 min read
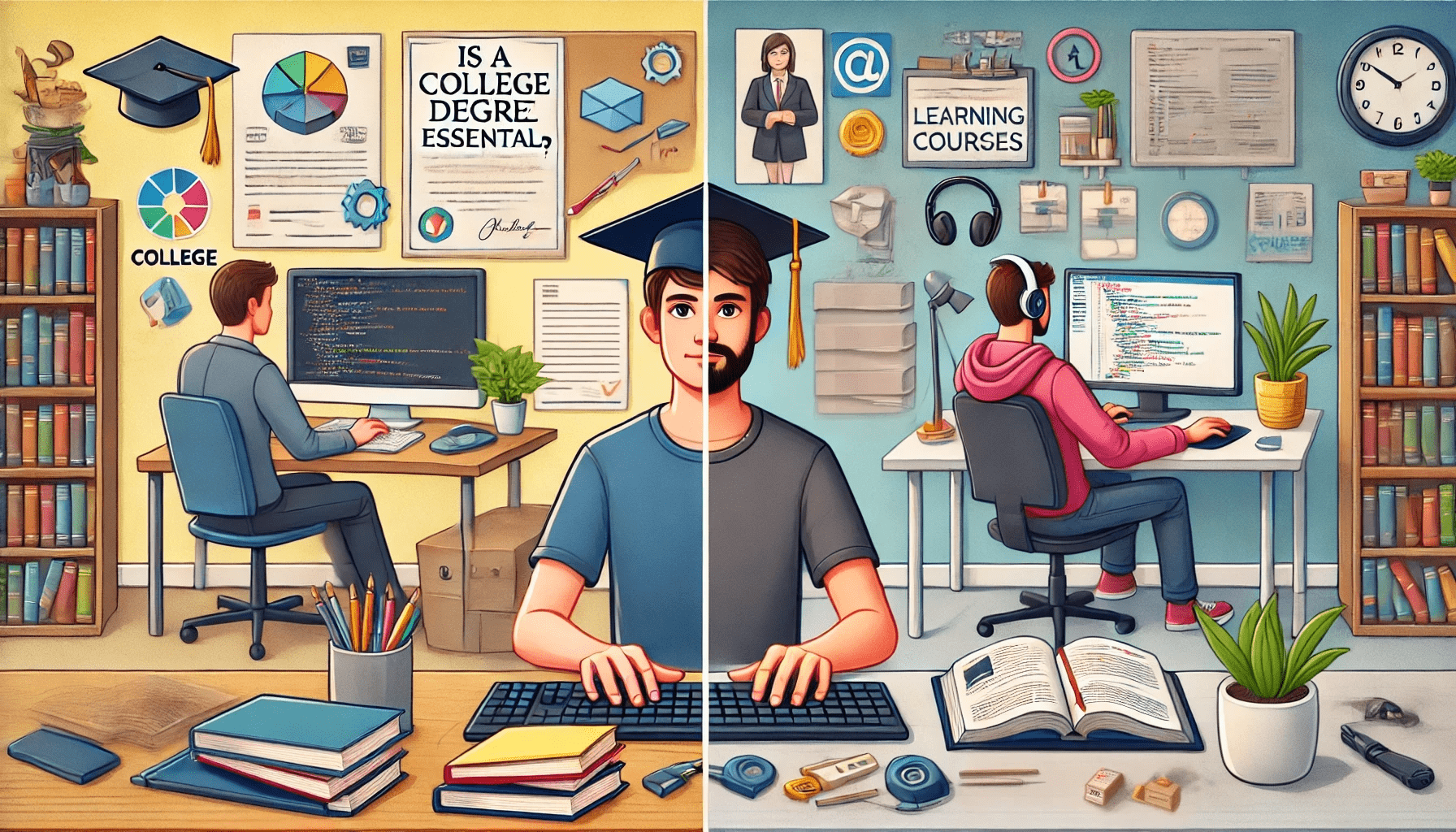
Content of this article