Related courses
See All CoursesIntermediate
Intermediate SQL
This course is perfect for those who already have a basic understanding of SQL and want to delve into more advanced concepts to craft more powerful queries. Throughout the course, you will become familiar with data grouping and filtering grouped data. You will also learn how to work with multiple tables simultaneously, including how to combine them. Additionally, you will explore different types of table joins and how to apply them in practice.
Intermediate
Databases in Python
"Databases in Python" is a hands-on course designed to teach you the fundamentals of working with databases using Python, focusing on the sqlite3 library and SQLAlchemy. Youβll learn how to store, modify, and retrieve data, build efficient queries, and configure databases for your projects. The course covers both SQL basics and the ORM (Object-Relational Mapping) approach, which allows you to interact with databases through Python objects. This course is perfect for beginners looking to deepen their skills in data management, application development, and information handling.
Beginner
Introduction to SQL
This course is perfect for beginners ready to explore the world of SQL. Whether you're just starting out in database management or aiming to use SQL for your application development projects, this course covers the essentials. You'll quickly learn how to leverage the full potential of SQL, from querying and managing data to seamlessly integrating it into real-world applications. By the end of the course, you'll have the confidence and skills to solve practical problems with SQL and enhance your development process.
The 50 Top SQL Interview Questions and Answers
For Junior and Middle Developers
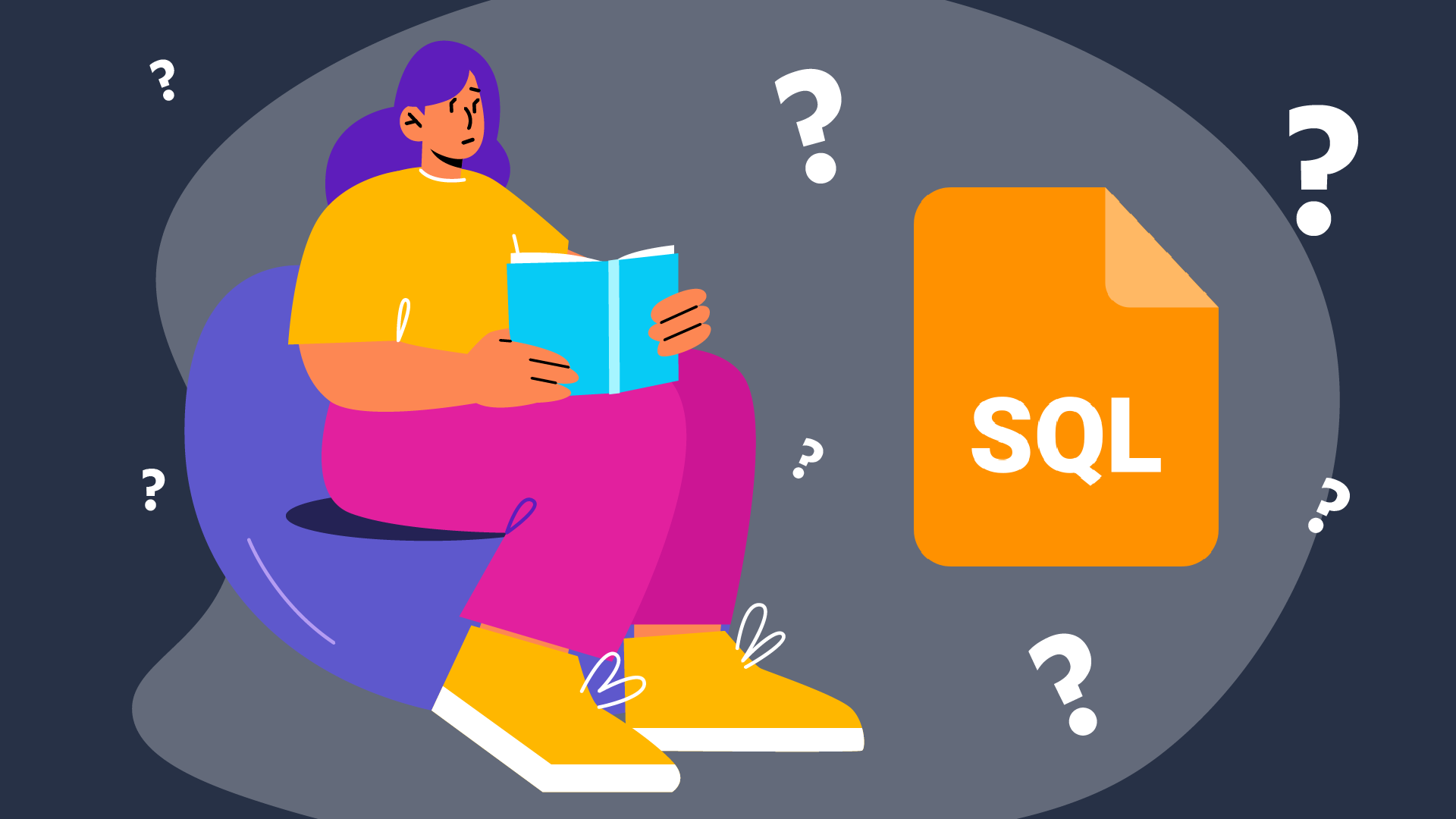
SQL (Structured Query Language) is a critical skill for anyone working in data analytics and software development. Preparing for an interview for a position involving SQL can be challenging. This article presents you with the top 50 questions to help you prepare for the interview and demonstrate your knowledge and skills in SQL.
SQL and NoSQL
Advantages and Disadvantages
Q1: What are the advantages of SQL compared to NoSQL?
A: SQL offers a structured approach and the ability to perform complex operations on data, making it an excellent choice for projects where strict consistency and high data accuracy are required. Many large enterprises rely on SQL for critical systems as it provides reliability and stability.
Q2: Why do you consider SQL more suitable for certain cases compared to NoSQL?
A: I believe SQL is more suitable in cases where ensuring data stability and integrity is crucial, such as database management systems for financial institutions or medical records. SQL allows for complex data operations and ensures consistency, which is critical for such applications.
Q3: What disadvantages of SQL and NoSQL could impact your project?
A: A disadvantage of SQL could be the complexity of horizontally scaling, especially with large volumes of data. Additionally, using SQL may require higher expenses for hardware and database administration.
How Not to Answer:
- Avoid emphasizing the disadvantages of NoSQL, as it may create the impression that you don't fully understand both technologies or have a bias towards one type of database.
- Avoid making categorical conclusions that SQL is always better than NoSQL or vice versa. It's better to highlight in which cases each of them may be more suitable.
Based on the discussion of the advantages and disadvantages of SQL and NoSQL, it's important to emphasize in the interview your ability to adapt technologies to specific project requirements. The interviewer expects you to demonstrate a deep understanding of both types of databases and the ability to make informed choices between them depending on the project's needs. It's important to show readiness to consider each case individually, taking into account the product's requirements, scale, and projected workload. The key is the ability to work with both technologies and use them according to the business needs.
Basic SQL Commands
CRUD
Q4: What are the primary SQL commands you are familiar with, and how are they used?
A: The main SQL commands I am familiar with are SELECT
, INSERT
, UPDATE
, and DELETE
. Each of these commands serves specific functions: SELECT
is used to retrieve data from a database, INSERT
is for adding new records, UPDATE
is for modifying existing records, and DELETE
is for removing data.
Q5: Can you provide examples of using the SELECT, INSERT, UPDATE, and DELETE commands in SQL?
A: For example, the SELECT
command can be used to retrieve specific columns or rows from a table. INSERT
is utilized to add a new row of data into a table. UPDATE
allows for modifying existing data within a table, while DELETE
removes specific rows from a table.
Q6: How do you utilize conditional operators in SQL queries, such as WHERE, to filter query results?
A: Conditional operators like WHERE are used to filter query results based on specified conditions. For instance, I often use WHERE
to select rows where a certain column value is greater than a specified value.
Q7: What are CRUD operations in the context of databases, and how are they utilized?
A: CRUD stands for Create, Read, Update, Delete, representing the basic operations that can be performed on data in a database. Create is used to add new records, Read retrieves data, Update modifies existing records, and Delete removes data.
How to Answer:
-
Provide specific examples that best showcase your expertise. For instance: "When using the
SELECT
command, I often utilize subqueries to retrieve complex data from multiple tables." -
Emphasize the importance of using appropriate conditional operators for optimizing queries. For example: "Using
WHERE
effectively in SQL queries can significantly enhance the performance and efficiency of database operations." -
Explain how you apply CRUD operations in your work and their significance. For instance: "Implementing CRUD operations allows for efficient data management, ensuring data integrity and security within the system."
Your responses to questions about basic SQL commands and CRUD operations should demonstrate a clear understanding of each command's functionality and practical applications. Interviewers expect candidates to not only list commands but also explain their usage with examples and discuss their impact on database operations. Highlighting your ability to effectively utilize SQL commands and CRUD operations will showcase your preparedness and suitability for the position.
Run Code from Your Browser - No Installation Required
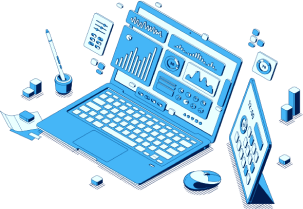
Data Conditions and Filtering
WHERE, ORDER BY, GROUP BY, HAVING
Q8: How would you describe the data filtering process in SQL?
A: Data filtering in SQL typically occurs using the key WHERE
clause. This clause allows specifying conditions under which data is selected from tables. For example:
sql
Will retrieve all records from the Employees table where the salary is greater than 50,000
.
Q9: What is the ORDER BY clause and how is it used?
A: The ORDER BY
clause is used to sort the results of a query by specific fields in either ascending or descending order. For example:
sql
Sorts the records in the Employees table by salary in descending order.
Q10: What is the difference between the GROUP BY and HAVING clauses?
A: The GROUP BY
clause is used to group rows from query results based on the values of a specific field. For example:
sql
groups rows by department and calculates the average salary for each department. The HAVING
clause is used to filter groups of results based on conditions that cannot be expressed using the WHERE
clause. For example:
sql
will select only those department groups where the average salary is greater than 50,000
.
How Not to Answer:
- Avoid misunderstanding the difference between the
GROUP BY
andHAVING
clauses. Do not confuse their functions. - Do not use the
GROUP BY
clause without using aggregate functions if you do not intend to group the data. - Avoid omitting specifying the sorting order using
ORDER BY
if the order is important for the query results.
Based on the discussion of the WHERE
, ORDER BY
, GROUP BY
, HAVING
clauses, it is important to emphasize in the interview your ability to understand and effectively use these constructs for data processing. Consider each query individually, taking into account its requirements and needs, as well as the ability to optimize queries to improve database performance and efficiency.
Joins
INNER JOIN, LEFT JOIN, RIGHT JOIN
Q11: Can you explain what a SQL join is and how it's used?
A: A SQL join is used to combine rows from two or more tables based on a related column between them. It allows retrieving data from multiple tables in a single query. For example:
sql
Retrieves the order ID and customer name from the Orders and Customers tables, joining them on the CustomerID column.
Q12: What are the different types of joins in SQL?
A: There are several types of joins in SQL:
INNER JOIN
: Returns rows when there is a match in both tables.LEFT JOIN
(or LEFT OUTER JOIN): Returns all rows from the left table and matching rows from the right table.RIGHT JOIN
(or RIGHT OUTER JOIN): Returns all rows from the right table and matching rows from the left table.FULL JOIN
(or FULL OUTER JOIN): Returns rows when there is a match in one of the tables.CROSS JOIN
: Returns the Cartesian product of the two tables, meaning it returns all possible combinations of rows from both tables.
Q13: When would you use an INNER JOIN versus an OUTER JOIN?
A: You would use an INNER JOIN
when you only want to retrieve rows that have matching values in both tables. This is useful when you need to retrieve data that exists in both tables. On the other hand, you would use an OUTER JOIN
when you want to retrieve all rows from one or both tables, regardless of whether there is a match between the tables. This is useful when you need to include unmatched rows from one or both tables in the result set.
How Not to Answer:
- Avoid using joins without specifying the join condition, as it can result in a Cartesian product, leading to unexpected and inefficient results.
- Do not assume that one type of join is always better than another. Consider the specific requirements of the query and choose the appropriate join type accordingly.
- Avoid forgetting to alias columns when joining tables with common column names to avoid ambiguity in the result set.
When discussing joins in an interview, it's important to demonstrate a clear understanding of how joins work and when to use each type of join. Highlight your ability to construct complex queries involving multiple tables and your familiarity with optimizing join queries for performance when working with large datasets.
Aggregate Operators
SUM, AVG, COUNT, MAX, MIN
Q14: What are aggregate operators in SQL, and how are they used?
A: Aggregate operators in SQL are functions used to perform calculations on a set of values and return a single value. They are often used in combination with the GROUP BY
clause to calculate summary statistics for groups of rows. For example, SUM
calculates the total of a set of values, AVG
calculates the average, COUNT
counts the number of rows, MAX
finds the maximum value, and MIN
finds the minimum value.
Q15: Can you provide an example of each aggregate operator in a SQL query?
A: Certainly:
SUM
: Calculates the total salary of all employees.
sql
AVG
: Calculates the average salary of all employees.
sql
COUNT
: Counts the total number of employees.
sql
MAX
: Finds the highest salary among all employees.
sql
MIN
: finds the lowest salary among all employees.
sql
Q16: When would you use each aggregate operator?
A: These operators should be used as follows:
- Use
SUM
when you need to find the total of a set of values, such as total sales. - Use
AVG
when you need to find the average of a set of values, such as average test scores. - Use
COUNT
when you need to count the number of rows, such as the total number of orders. - Use
MAX
when you need to find the maximum value in a set, such as the highest temperature recorded. - Use
MIN
when you need to find the minimum value in a set, such as the lowest stock price.
How Not to Answer:
- Avoid using aggregate operators without considering the grouping of data, as it may lead to incorrect results.
- Do not forget to handle cases where aggregate functions return
NULL
values, especially when dealing with outer joins or empty result sets. - Avoid using aggregate functions unnecessarily, especially in cases where simpler alternatives, such as
COUNT(*)
, could achieve the desired result more efficiently.
When discussing aggregate operators in an interview, demonstrate a solid understanding of their functions and how to use them effectively to derive insights from data. Highlight your ability to construct queries that utilize aggregate functions to calculate meaningful summary statistics and your awareness of potential pitfalls, such as NULL
values and performance considerations.
Start Learning Coding today and boost your Career Potential
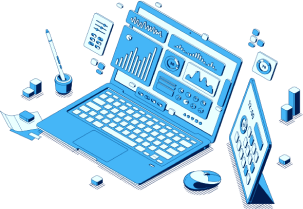
Indexes
Their Impact on Query Performance
Q17: What are indexes in databases, and what are they used for?
A: Indexes in databases are data structures that speed up the retrieval and querying of data by allowing quick access to specific rows within a table. They are created on one or more columns of a table and enable the database to efficiently find and sort data. Indexes significantly enhance query performance, especially when dealing with large volumes of data.
Q18: What types of indexes exist in SQL, and how do they differ?
A: In SQL, there are various types of indexes, including:
- Unique indexes: Ensure that values in the indexed columns are unique.
- Clustered indexes: Order the physical arrangement of rows in a table based on the indexed column's values.
- Non-clustered indexes: Do not affect the physical order of rows in a table.
- Full-text indexes: Used for fast searching of large amounts of textual data.
Q19: How do indexes impact query performance?
A: Indexes significantly enhance query performance by accelerating data retrieval and sorting. They enable the database to quickly locate answers to queries containing conditions that match indexed columns. However, improper use or over-indexing can lead to decreased performance as indexes require additional resources for storage and maintenance.
How Not to Answer:
- Avoid providing generic answers without specific examples of indexes' impact on query performance.
- Avoid underestimating the importance of optimizing indexes and their impact on performance.
- Remember that effective index usage requires analyzing specific queries and application requirements.
During discussions on indexes and their impact on query performance in an interview, it's crucial to demonstrate a deep understanding of the role of indexes in database management and their effect on query execution efficiency. Highlight your ability to analyze and optimize indexes to achieve maximum performance in database management systems.
Other Popular Questions
Q20: What are the advantages of using transactions in a database?
A: Transactions allow grouping database operations, ensuring atomicity, consistency, isolation, and durability (ACID) to maintain data in a stable and secure state.
Q21: How can SQL queries be optimized to improve database performance?
A: SQL query optimization involves using indexes, selecting the right operations, avoiding full table scans, and using statistics to enhance query performance.
Q22: What are views in SQL and how are they used?
A: Views in SQL are virtual tables stored in the database that contain the results of queries. They are used to simplify complex queries and provide a virtual representation of data.
Q23: What methods can be used to ensure database security?
A: To ensure database security, one can use parameterized queries to prevent SQL injections, access control, data encryption, and logging events to detect breaches.
Q24: What strategies are used for concurrency control in a database?
A: Concurrency control strategies include locking, optimistic conflict resolution, and using isolated transactions to maintain data integrity during simultaneous access.
Q25: What types of indexes exist in a database, and how do they impact performance?
A: Types of indexes include single-level and multi-level B-trees, hash indexes, full-text search, etc. Indexes improve performance by speeding up data retrieval and sorting.
Q26: How do you use stored procedures to optimize database operations?
A: Stored procedures are used to store a set of SQL instructions in the database. They allow preparing and executing complex operations, reducing network traffic, and improving performance.
Q27: How do you respond to SQL injections in the programs you develop?
A: To prevent SQL injections, I use parameterized queries, input validation, and filtering, as well as restrict access to the database from users.
Q28: How do you ensure database backup and recovery?
A: I ensure regular database backups and transaction logging to enable data recovery to a specific point in time.
Q29: What tools do you use for monitoring and tuning database performance?
A: I use various monitoring tools such as SQL Server Management Studio, Oracle Enterprise Manager, as well as queries to gather performance metrics.
Q30: What is a primary key in a database table, and why is it important?
A: A primary key in a database table is a unique identifier for each record in the table. It ensures that each row has a unique value that can be used to uniquely identify the record. For example, in a users table, the primary key could be an ID, which is unique for each user and allows for quick and efficient access to a specific record. Using a primary key is an important practice as it ensures data integrity and helps avoid duplication of information.
Q31: What types of relationships exist between tables in databases?
A: In databases, there are different types of relationships between tables, such as one-to-one, one-to-many, and many-to-many. For example, a one-to-many relationship is used when each record in one table can have multiple corresponding records in another table, but each record in the second table can only have one corresponding record in the first table.
Q32: What data types are used in SQL, and how do they differ from each other?
A: The data types in SQL include integers, floats, strings, date and time, boolean values, and others. Each data type has its own characteristics and limitations. For example, integers are used to store whole numbers without decimal places, while floats have a decimal part.
Q33: How can data be edited in a database table?
A: Data in a database table can be edited using the UPDATE
statement, which allows for changing values in existing rows of the table. For example, the UPDATE
command can be used to change a user's name or address in the users table.
Q34: How does the LIMIT function work in SQL and what is it used for?
A: The LIMIT
function in SQL is used to limit the number of rows returned by a query. It is often used to retrieve a specific number of rows from a table. For example, SELECT * FROM table LIMIT 10
will return the first 10 rows from the table.
Q35: How do you define and use a primary key in a table?
A: To define a primary key in a database table, you need to specify a column that has unique values for each row and mark it as PRIMARY KEY
. This column will then be used to uniquely identify records in the table.
Q36: How do you create a relationship between two tables in a database?
A: A relationship between two tables can be created using a foreign key. This is a field in one table that references the primary key in another table, creating a relationship between them.
Q37: What data types are used for storing textual and numerical information in SQL?
A: In SQL, VARCHAR
and TEXT
data types are used for storing textual information, while INT
, FLOAT
, DOUBLE
, etc., are used for storing numerical information.
Q38: How do you add a new column to an existing table in a database?
A: To add a new column to an existing table in a database, you can use the ALTER TABLE
command, which allows you to add new columns or modify existing ones.
Q39: How do you delete a column from a table in a database?
A: To delete a column from a table in a database, you can use the ALTER TABLE
command with the DROP
keyword, which removes the specified column from the table.
Q40: What is an index in a database and how does it affect performance?
A: An index in a database is a data structure that speeds up the retrieval and sorting of records in a table based on a specific column. Using indexes improves query performance by reducing the time it takes to search and sort data.
Q41: How do you select a specific number of rows from a table using the LIMIT function?
A: To select a specific number of rows from a table in SQL, you can use the LIMIT
function, which limits the number of rows returned by a query. For example, SELECT * FROM table LIMIT 10
will return the first 10 rows from the table.
Q42: How do you select unique values from a column in a database table?
A: To select unique values from a column in a database table, you can use the DISTINCT
keyword in the SELECT
statement. For example, SELECT DISTINCT
column FROM
table will return only unique values from the specified column.
Q43: How do you select data from multiple tables using JOIN in SQL?
A: In SQL, to select data from multiple tables, you can use the JOIN
keyword, which allows you to combine data from different tables based on a specified condition. For example, SELECT * FROM table1 JOIN table2 ON table1.id = table2.id
will combine data from table1
and table2
based on the id column.
Q44: How do you change the data type of a column in a database table?
A: To change the data type of a column in a database table, you can use the ALTER TABLE
command with the MODIFY
keyword, which allows you to change the data type and other properties of the column.
Q45: How do you rename a table in a database?
A: To rename a table in a database, you can use the ALTER TABLE
command with the RENAME TO
keyword, which allows you to change the name of the table to a new one.
Q46: How do you select all rows where the value in a specific column meets a certain condition?
A: To select all rows where the value in a specific column meets a certain condition, you can use the WHERE keyword in the SELECT
statement. For example, SELECT * FROM table WHERE column = value
will return all rows from the table where the value in the specified column equals the specified value.
Q47: How do you group data by a specific column in a database table?
A: To group data by a specific column in a database table, you can use the GROUP BY
keyword in the SELECT
statement. For example, SELECT
column, COUNT(*) FROM
table GROUP BY
column will group the data by the values in the specified column and count the number of rows in each group.
Q48: How do you select the average value or sum of values in a specific column of a table?
A: To select the average value or sum of values in a specific column of a table in a database, you can use the aggregate functions AVG()
and SUM()
in the SELECT
statement. For example, SELECT AVG(column) FROM table
will return the average value of the column in the table.
Q49: How do you select data that does not meet a certain condition using the NOT IN keyword?
A: To select data that does not meet a certain condition, you can use the NOT IN
keyword in the WHERE
clause of the SELECT
statement. For example, SELECT * FROM table WHERE column NOT IN (value1, value2)
Will return all rows from the table where the value in the column is not equal to value1 or value2.
Q50: How do you establish a one-to-one relationship between two tables in a database?
A: In a one-to-one relationship between two tables, each record in one table corresponds to exactly one record in the other table, and vice versa. This relationship is typically established by creating a foreign key in one table that references the primary key of the other table. The foreign key column ensures that each record in the first table is associated with only one record in the second table. This type of relationship is commonly used to split a large table into smaller, more manageable parts while maintaining data integrity and minimizing redundancy.
Related courses
See All CoursesIntermediate
Intermediate SQL
This course is perfect for those who already have a basic understanding of SQL and want to delve into more advanced concepts to craft more powerful queries. Throughout the course, you will become familiar with data grouping and filtering grouped data. You will also learn how to work with multiple tables simultaneously, including how to combine them. Additionally, you will explore different types of table joins and how to apply them in practice.
Intermediate
Databases in Python
"Databases in Python" is a hands-on course designed to teach you the fundamentals of working with databases using Python, focusing on the sqlite3 library and SQLAlchemy. Youβll learn how to store, modify, and retrieve data, build efficient queries, and configure databases for your projects. The course covers both SQL basics and the ORM (Object-Relational Mapping) approach, which allows you to interact with databases through Python objects. This course is perfect for beginners looking to deepen their skills in data management, application development, and information handling.
Beginner
Introduction to SQL
This course is perfect for beginners ready to explore the world of SQL. Whether you're just starting out in database management or aiming to use SQL for your application development projects, this course covers the essentials. You'll quickly learn how to leverage the full potential of SQL, from querying and managing data to seamlessly integrating it into real-world applications. By the end of the course, you'll have the confidence and skills to solve practical problems with SQL and enhance your development process.
Content of this article