Related courses
See All CoursesBeginner
Python Data Structures
In this course, you'll get to know the fundamental data structures of the Python programming language. We'll explore utilizing Python's native data structures like lists, dictionaries, tuples, and sets to tackle different challenges.
Beginner
Introduction to Python
Python is a high-level, interpreted, general-purpose programming language. Distinguished from languages such as HTML, CSS, and JavaScript, which are mainly utilized in web development, Python boasts versatility across multiple domains, including software development, data science, and back-end development. This course will guide you through Python's fundamental concepts, equipping you with the skills to create your own functions by the conclusion of the program.
The Facade Pattern Explained
Simplify Complex Systems with a Unified Interface
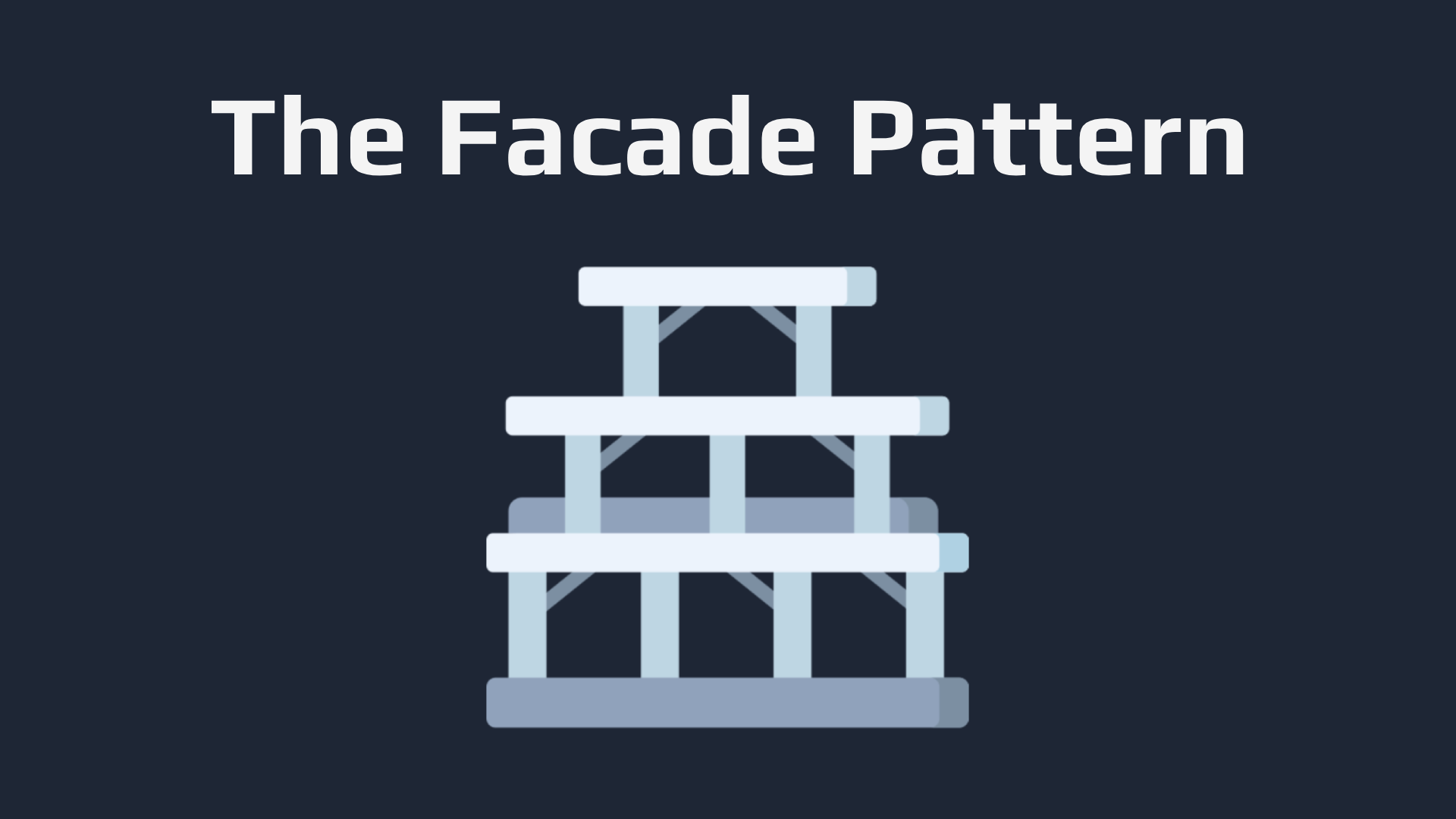
The Facade Pattern is a structural design pattern that provides a simplified interface to a complex subsystem. Instead of exposing a labyrinth of internal classes and methods, the pattern consolidates functionality into a single interface.
Think of it like a remote control for your home entertainment systemβyou donβt need to understand how the television, sound system, and streaming device work internally. You just press a button, and everything works together seamlessly.
What is the Facade Pattern?
The Facade Pattern hides the complexities of a system by providing a high-level interface. It doesn't restrict access to the underlying systems but simplifies usage by wrapping complex interactions.
Feature | Description |
---|---|
Type | Structural Design Pattern |
Primary Goal | Simplify interactions with complex subsystems |
Usage | When dealing with APIs, frameworks, or libraries |
Common Use Cases | GUIs, system initialization, or configuration tools |
Implementing the Facade Pattern
Let's walk through a practical example. Imagine youβre building a home theater system with multiple components DVD player, projector, amplifier, and lights.
Each of these classes would need to be managed individually if we didnβt implement a facade. That would mean a lot of repetitive setup and teardown code in the client.
Now we wrap all of the subsystems into a single, unified interface using the HomeTheaterFacade
class.
The watch_movie
method encapsulates all the setup steps needed to start a movie, while end_movie
handles the shutdown. This drastically simplifies client interaction.
Hereβs how the client code looks when we use the facade. Itβs clean, concise, and easy to understand.
Instead of initializing and controlling each device separately, the client uses two clear method calls. The complexity is hidden, but the power remains.
Start Learning Coding today and boost your Career Potential
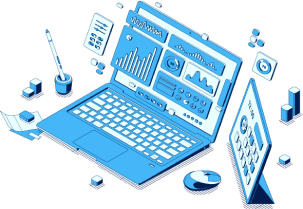
Advantages of Using the Facade Pattern
Benefit | Explanation |
---|---|
Simplification | Reduces the learning curve for using a system |
Loose Coupling | Minimizes dependencies between clients and subsystems |
Ease of Use | Provides a single point of control |
Improved Readability | Makes the code more maintainable and less error-prone |
Encapsulation | Hides the implementation details of subsystems |
The Facade Pattern is a powerful way to provide clean interfaces to complex systems. It's commonly used in frameworks, APIs, and layered architecture to create modular, maintainable codebases. By using a Facade, developers can shield the client code from low-level operations, resulting in cleaner and more focused logic.
FAQs
Q: What is the Facade Pattern?
A: The Facade Pattern is a structural design pattern that provides a simplified interface to a complex system of classes or modules.
Q: When should I use the Facade Pattern?
A: Use it when you want to hide complexity from clients or simplify interactions with complex subsystems.
Q: Can the Facade Pattern work with other patterns?
A: Yes, it can be combined with Singleton (to make the facade globally accessible), or Factory (to generate subsystem instances).
Q: Is the Facade Pattern the same as an API Gateway?
A: Not exactly. While both simplify access, an API Gateway handles HTTP requests and routing, while a Facade simplifies object-level or class-level interactions in code.
Q: Does the Facade Pattern replace the need to understand underlying systems?
A: Not necessarily. It simplifies use but doesnβt eliminate the need to understand the internals when debugging or extending features.
Related courses
See All CoursesBeginner
Python Data Structures
In this course, you'll get to know the fundamental data structures of the Python programming language. We'll explore utilizing Python's native data structures like lists, dictionaries, tuples, and sets to tackle different challenges.
Beginner
Introduction to Python
Python is a high-level, interpreted, general-purpose programming language. Distinguished from languages such as HTML, CSS, and JavaScript, which are mainly utilized in web development, Python boasts versatility across multiple domains, including software development, data science, and back-end development. This course will guide you through Python's fundamental concepts, equipping you with the skills to create your own functions by the conclusion of the program.
Top 25 C# Interview Questions and Answers
Master the Essentials and Ace Your C# Interview
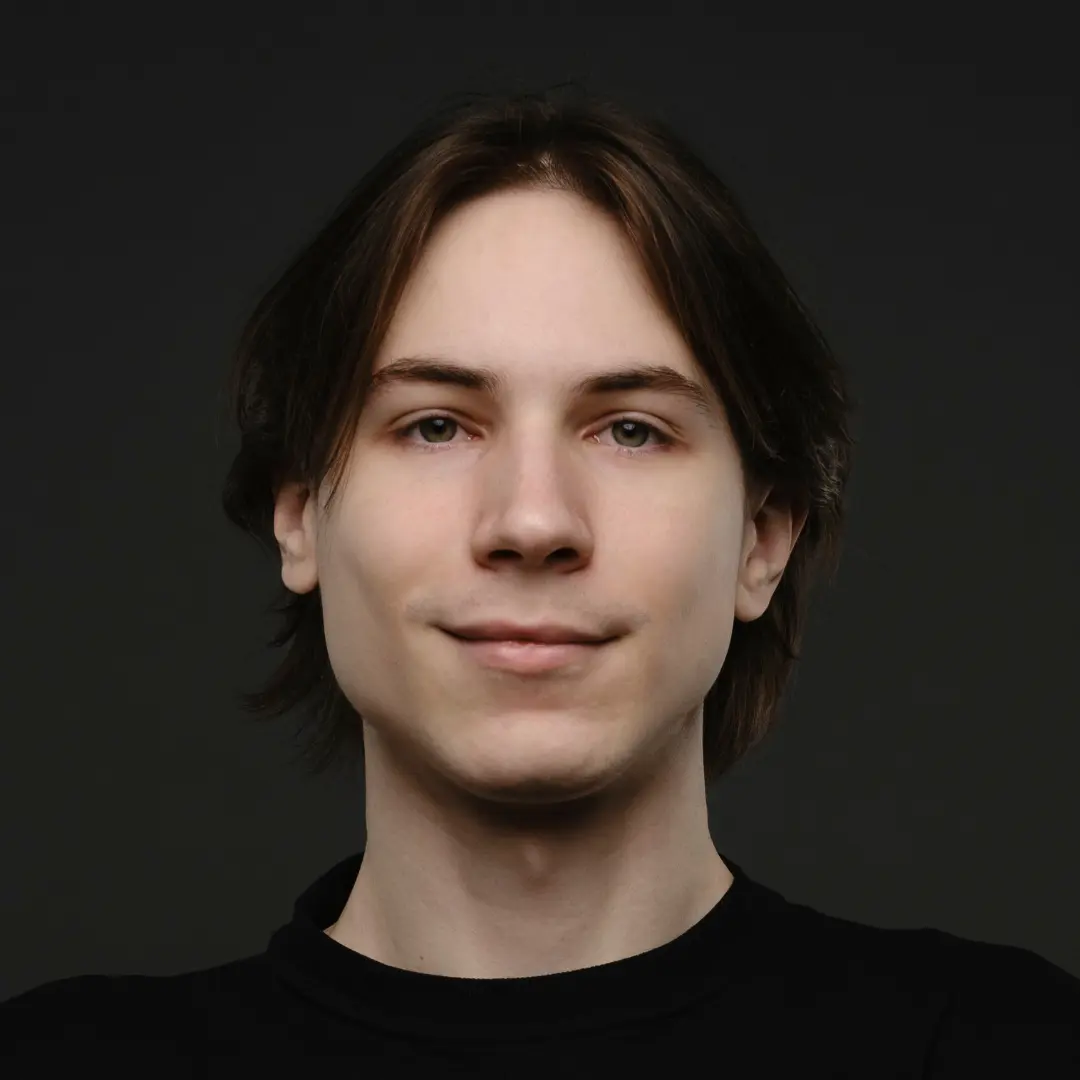
by Ihor Gudzyk
C++ Developer
Nov, 2024γ»17 min read
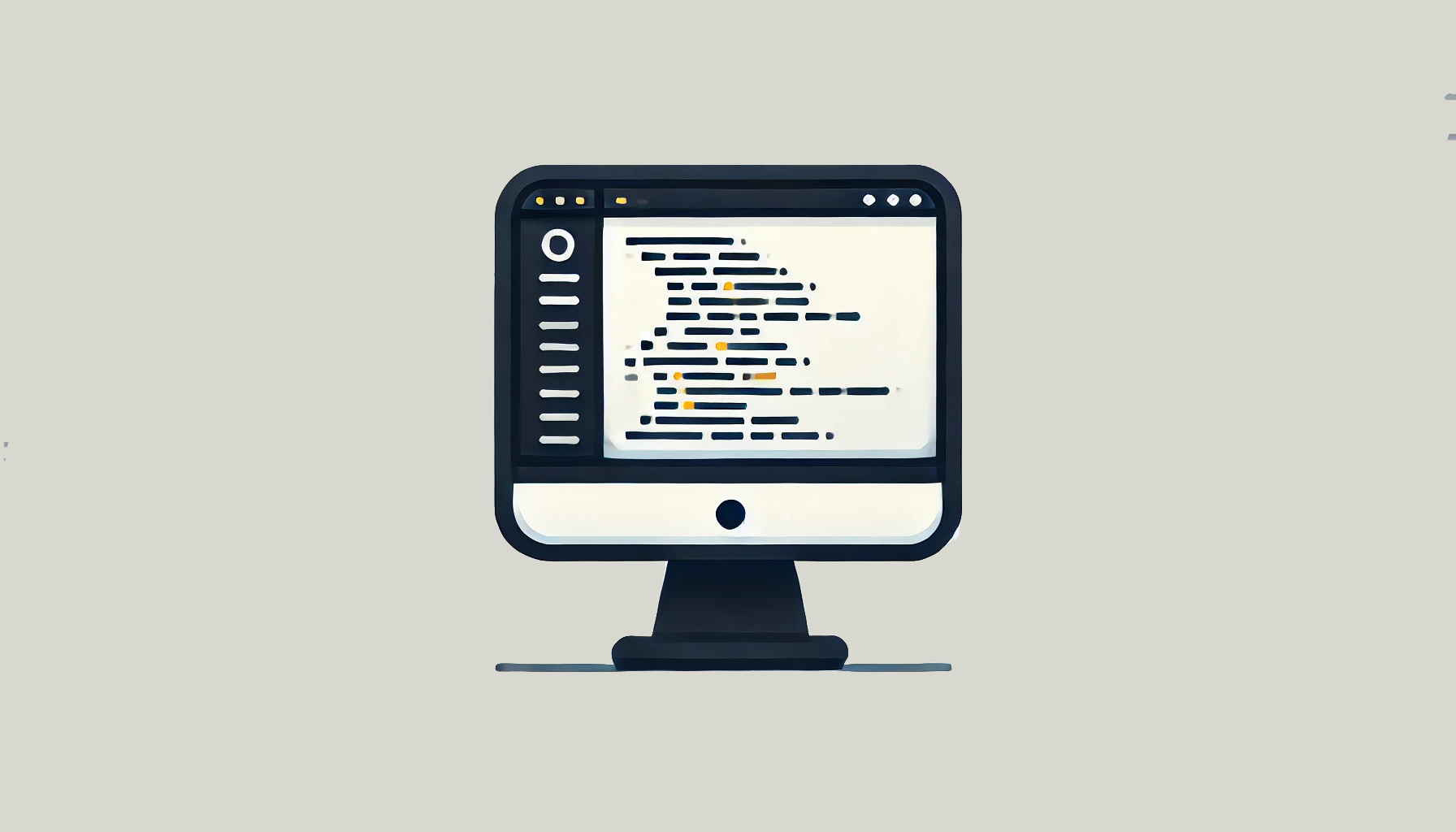
The Power of Collaboration or Contributing to Open Source Projects
Uniting in the World of Code
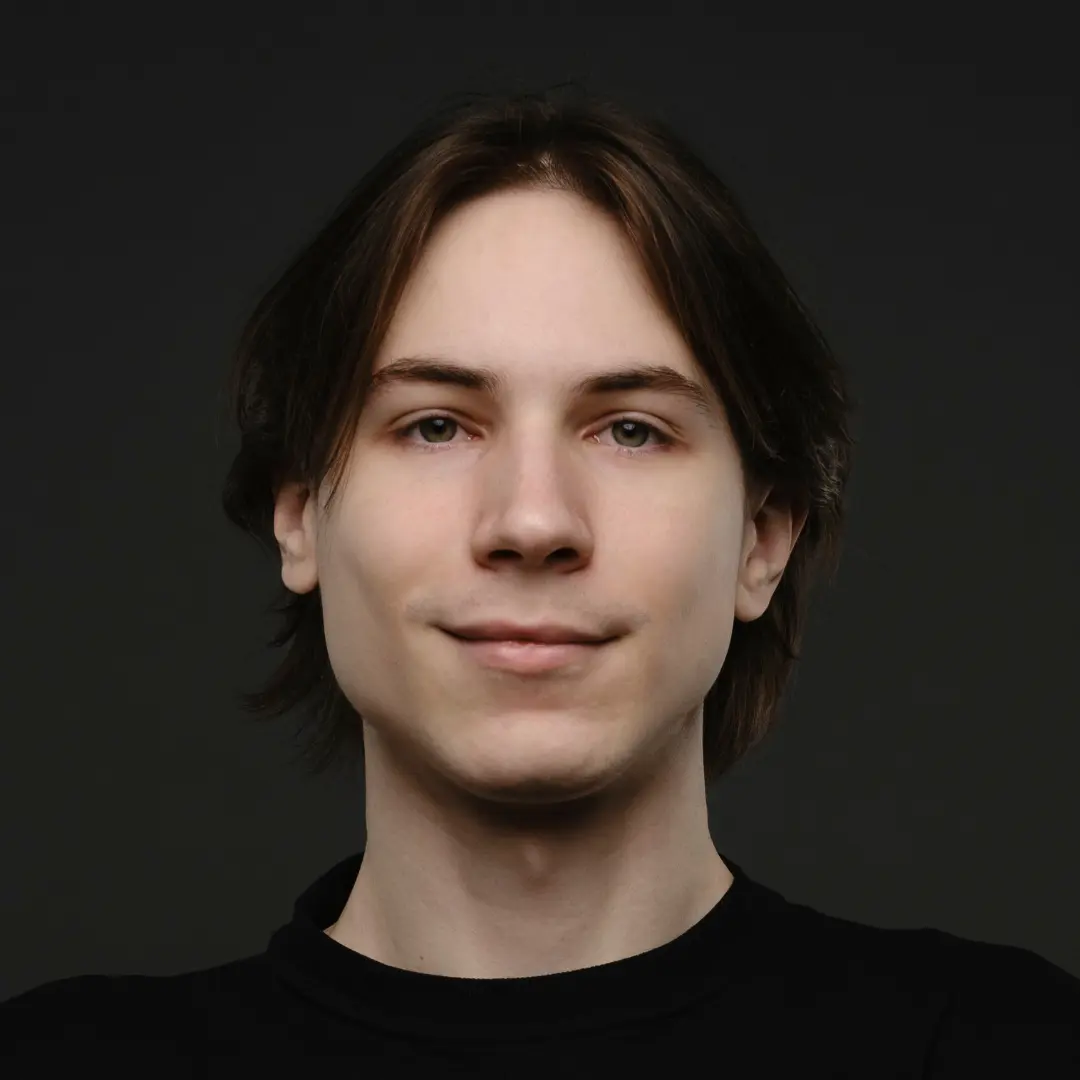
by Ihor Gudzyk
C++ Developer
Dec, 2023γ»9 min read
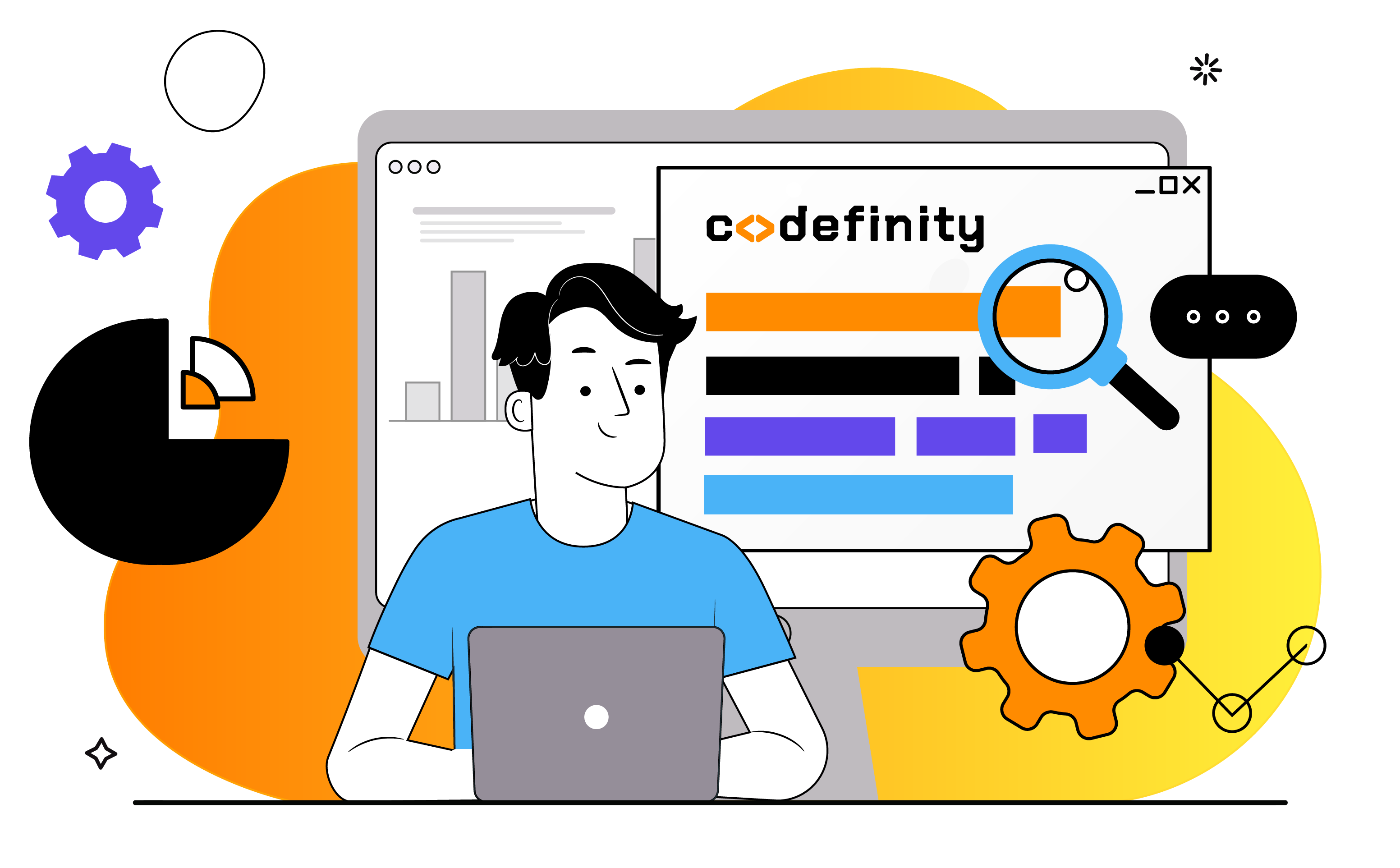
8 In-Demand Skills to Earn Big in 2025
Skills to stay ahead
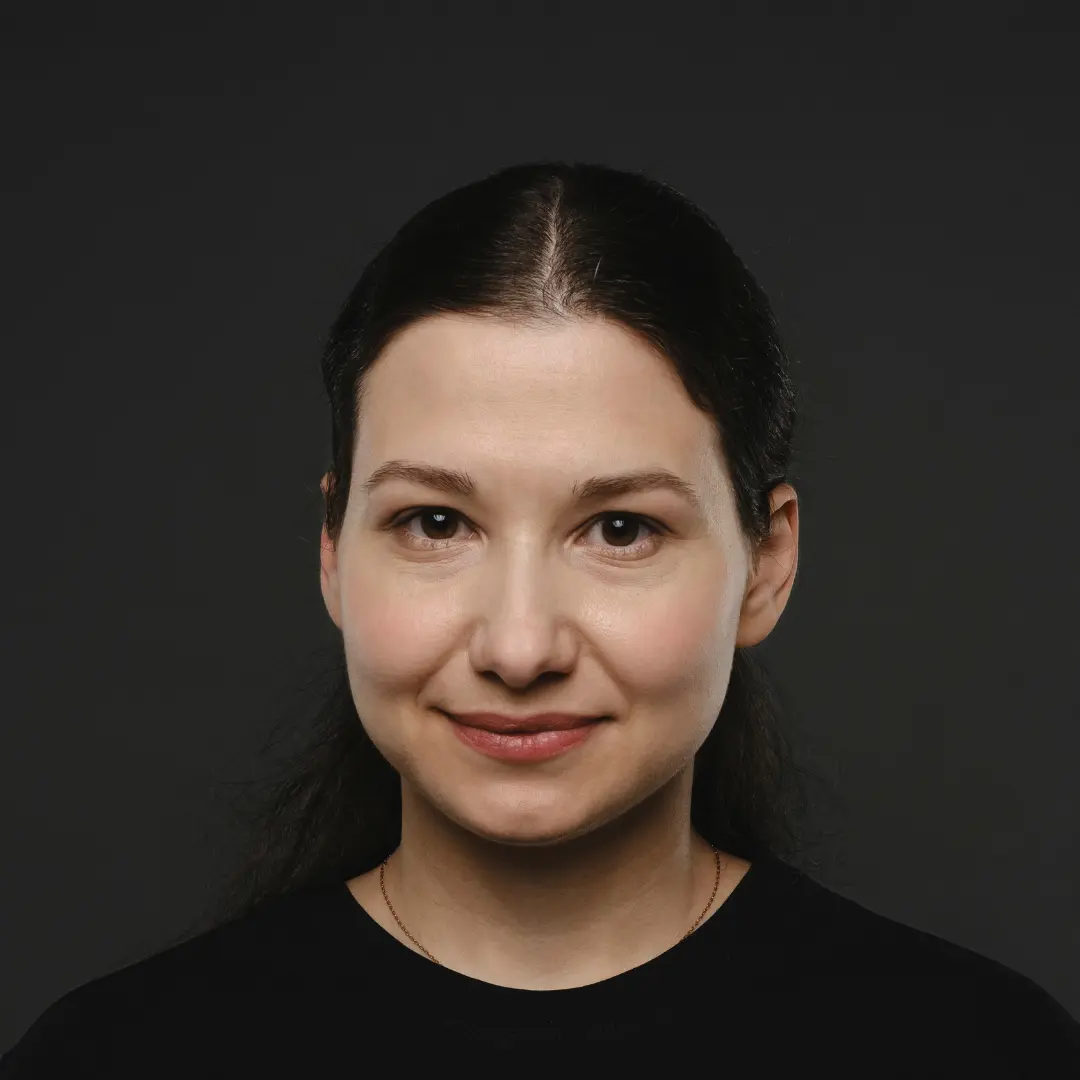
by Anastasiia Tsurkan
Backend Developer
Dec, 2024γ»23 min read
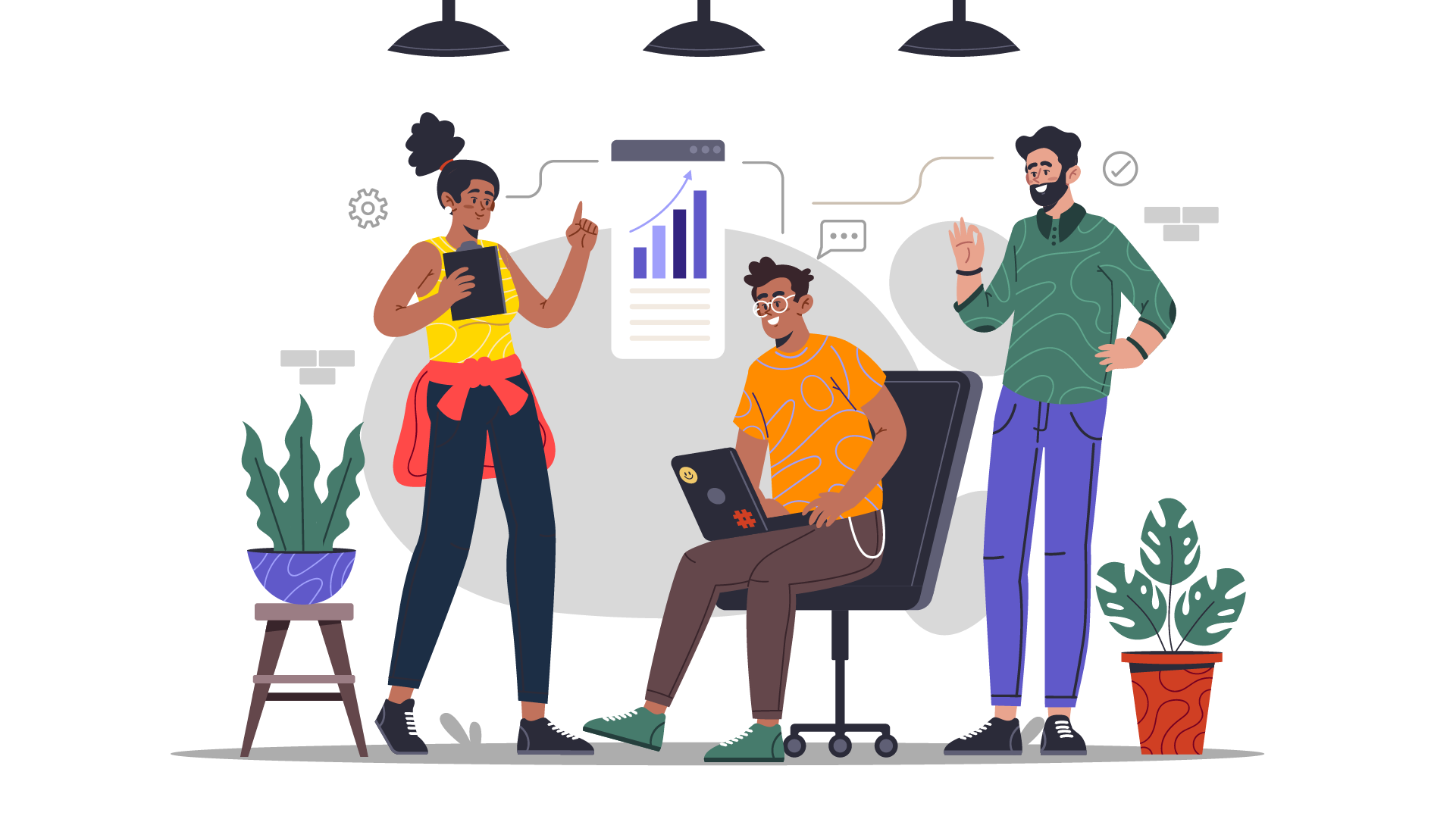
Content of this article