Related courses
See All CoursesUnderstanding Method Resolution Order in Python
Demystifying Class Inheritance and Method Resolution
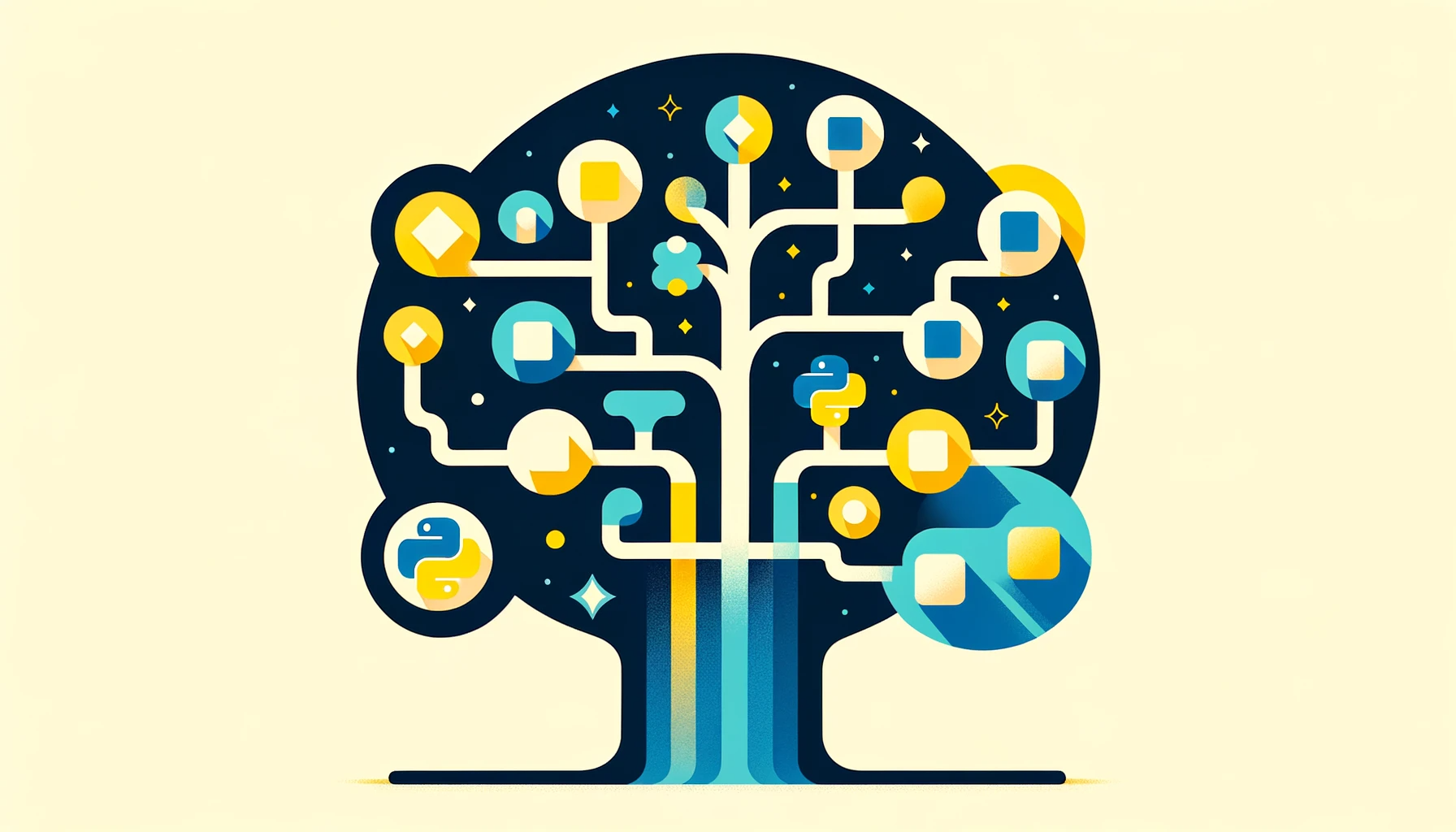
In the world of object-oriented programming (OOP), Python stands out for its simplicity and readability. One of the key concepts in Python's OOP is the Method Resolution Order (MRO). This article will guide beginners through the intricacies of MRO, explaining its importance and how it operates in Python.
What is Method Resolution Order?
Method Resolution Order is the sequence in which Python looks for a method in a hierarchy of classes. Especially in the context of multiple inheritances, where a class is derived from more than one base class, MRO becomes crucial to understand which method is actually being called.
Understanding MRO is critical in Python programming because it helps in debugging and reading code effectively. Knowing the order in which methods are resolved helps in predicting the behavior of your code, especially in complex class hierarchies.
Run Code from Your Browser - No Installation Required
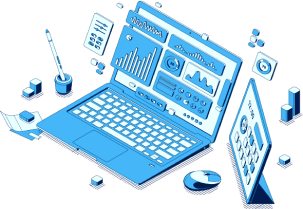
Understanding Classes and Inheritance in Python
Before diving into MRO, it's essential to understand classes and inheritance in Python.
A class in Python is like a blueprint for creating objects. It defines a set of attributes and methods that will characterize any object of that class.
python
Inheritance: Extending Classes
Inheritance allows a new class, known as a child class, to extend the properties and behaviors of an existing class, referred to as the parent class. This mechanism promotes code reuse.
python
Exploring Single Inheritance
Single inheritance occurs when a child class inherits from only one parent class. Let's explore this with an example:
python
In this case, the Dog
class inherits from the Animal
class. When you call the speak
method on a Dog
object, Python will use the method defined in the Dog
class, as it overrides the one in the Animal
class.
Start Learning Coding today and boost your Career Potential
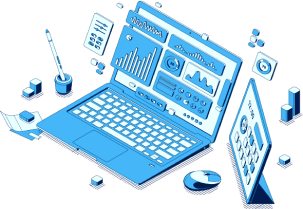
Delving into Multiple Inheritance
Multiple inheritances occur when a class inherits from more than one parent class. Python handles this through a specific MRO.
python
In this scenario, the Child
class inherits from both Father
and Mother
. The MRO determines which hobby
method gets called.
Python's MRO Algorithm: C3 Linearization
C3 Linearization is a deterministic algorithm used in Python to create a linear ordering of classes. This order respects two rules:
- Children precede their parents.
- If a class inherits from multiple classes, they are kept in the order specified in the tuple of the base class.
python
Let's delve into some practical examples to understand MRO better.
Example 1: Simple Multiple Inheritance Here, we define a scenario with straightforward multiple inheritances and examine the MRO.
python
Example 2: Complex Class Hierarchy
In this example, we create a more complex class hierarchy and use the __mro__
attribute to inspect the resolution order.
python
FAQs
Q: Can MRO result in errors or conflicts in Python?
A: Yes, especially in multiple inheritances, conflicting method names or improper use of super() can lead to issues. Understanding MRO helps to avoid these conflicts.
Q: How do I view the MRO of a class?
A: You can view the MRO by using the __mro__
attribute of the class, like ClassName.__mro__
.
Q: Is MRO unique to Python?
A: The concept of method resolution order exists in many object-oriented languages, but Python's C3 Linearization is a specific implementation.
Q: Does single inheritance have an MRO?
A: Yes, even in single inheritance, Python uses MRO, though it's straightforward as there's only one parent class.
Q: Can I override the MRO in Python?
A: You cannot override the MRO itself, but you can design your class hierarchy and use of methods to achieve the desired method resolution.
Q: How does super() work with MRO?
A: super() in Python works in conjunction with MRO to determine the next class in the hierarchy from which to call methods.
Related courses
See All CoursesThe SOLID Principles in Software Development
The SOLID Principles Overview
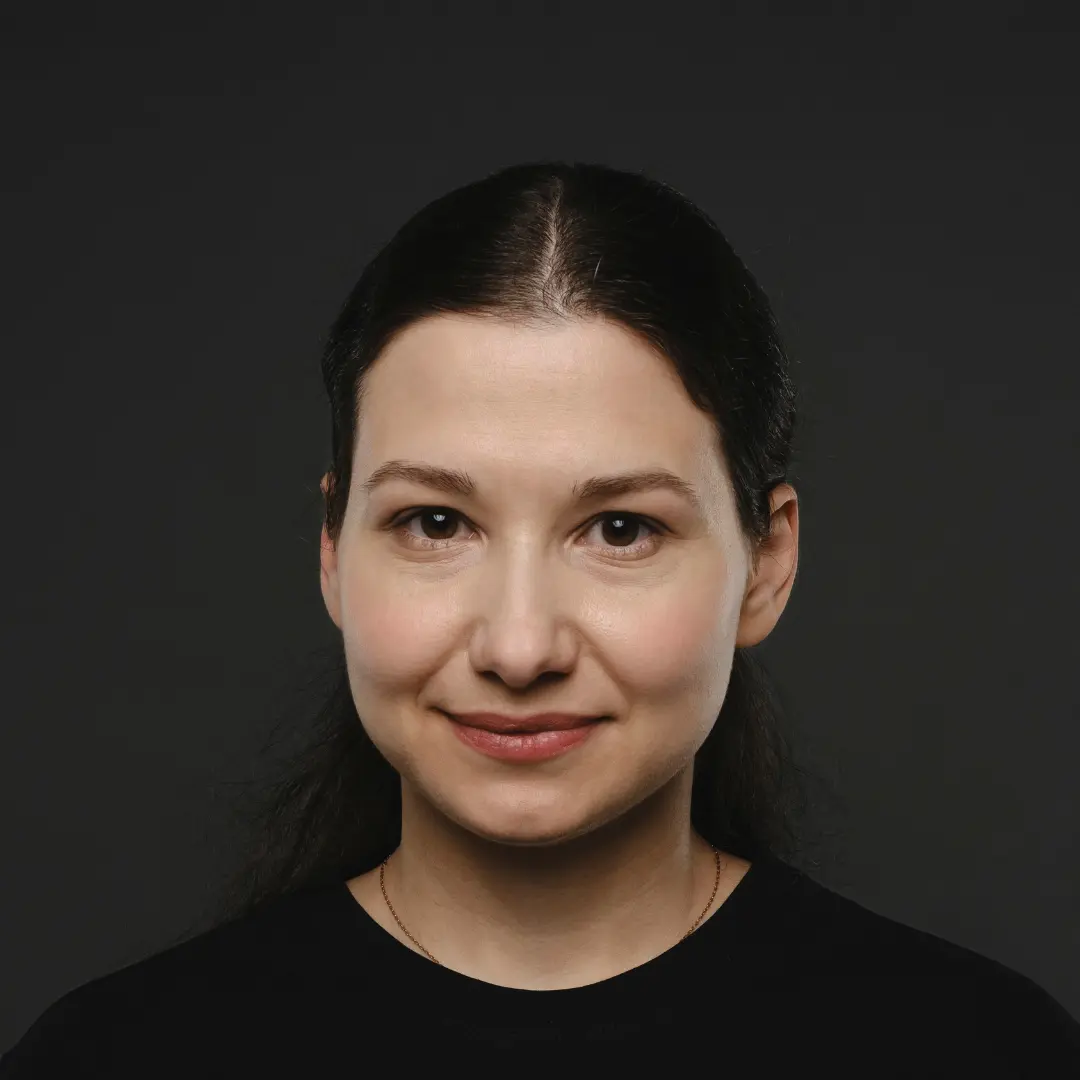
by Anastasiia Tsurkan
Backend Developer
Nov, 2023γ»8 min read
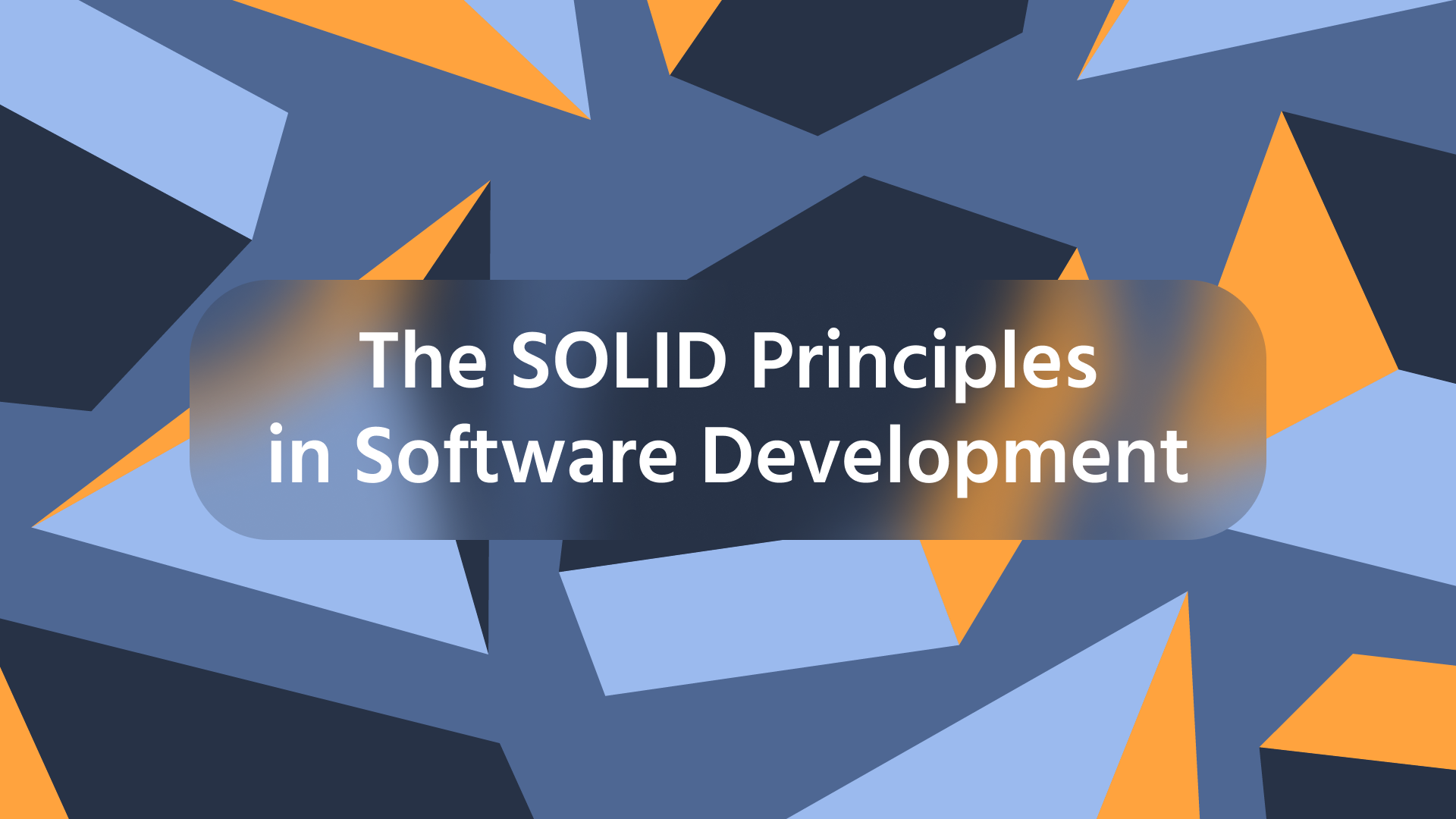
30 Python Project Ideas for Beginners
Python Project Ideas
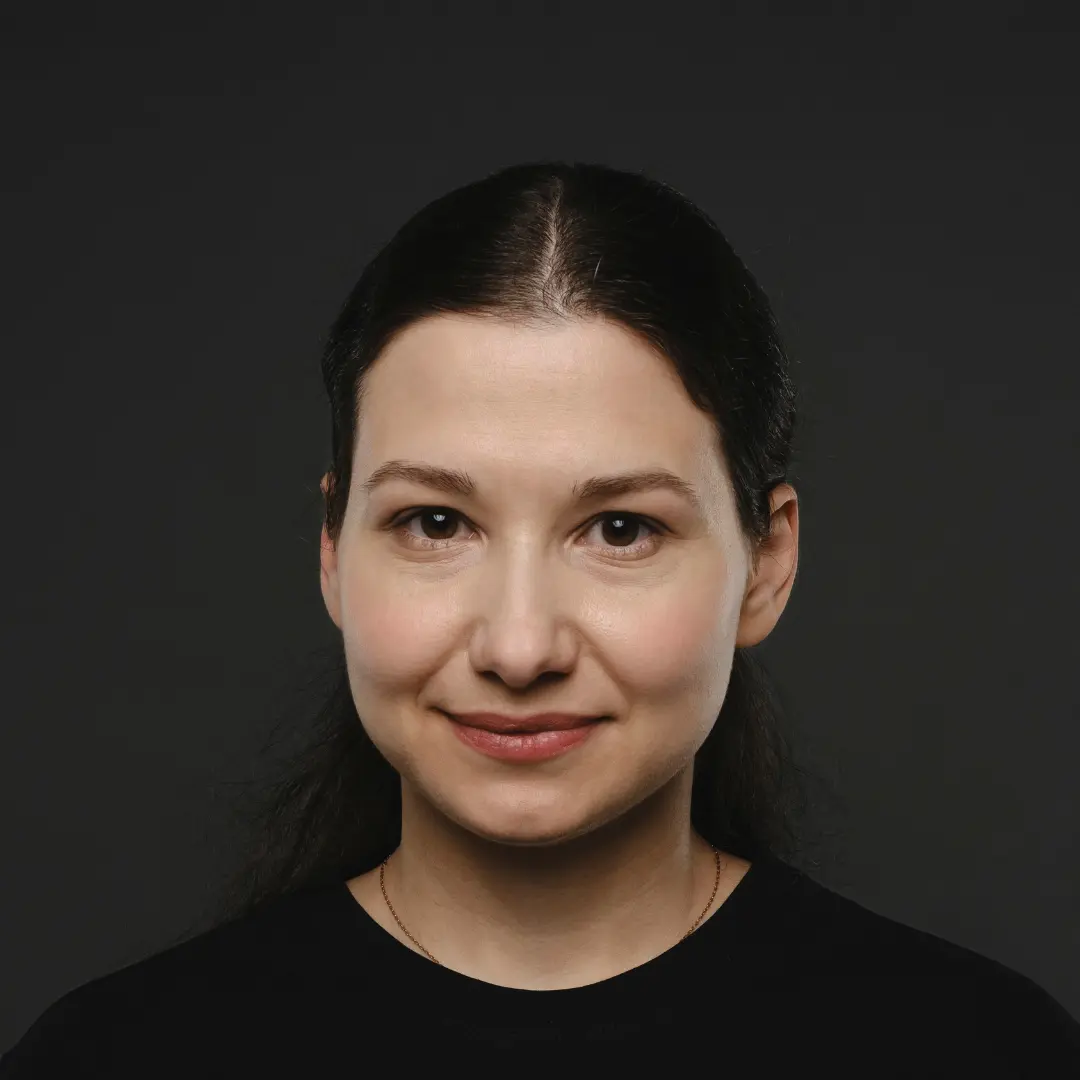
by Anastasiia Tsurkan
Backend Developer
Sep, 2024γ»14 min read
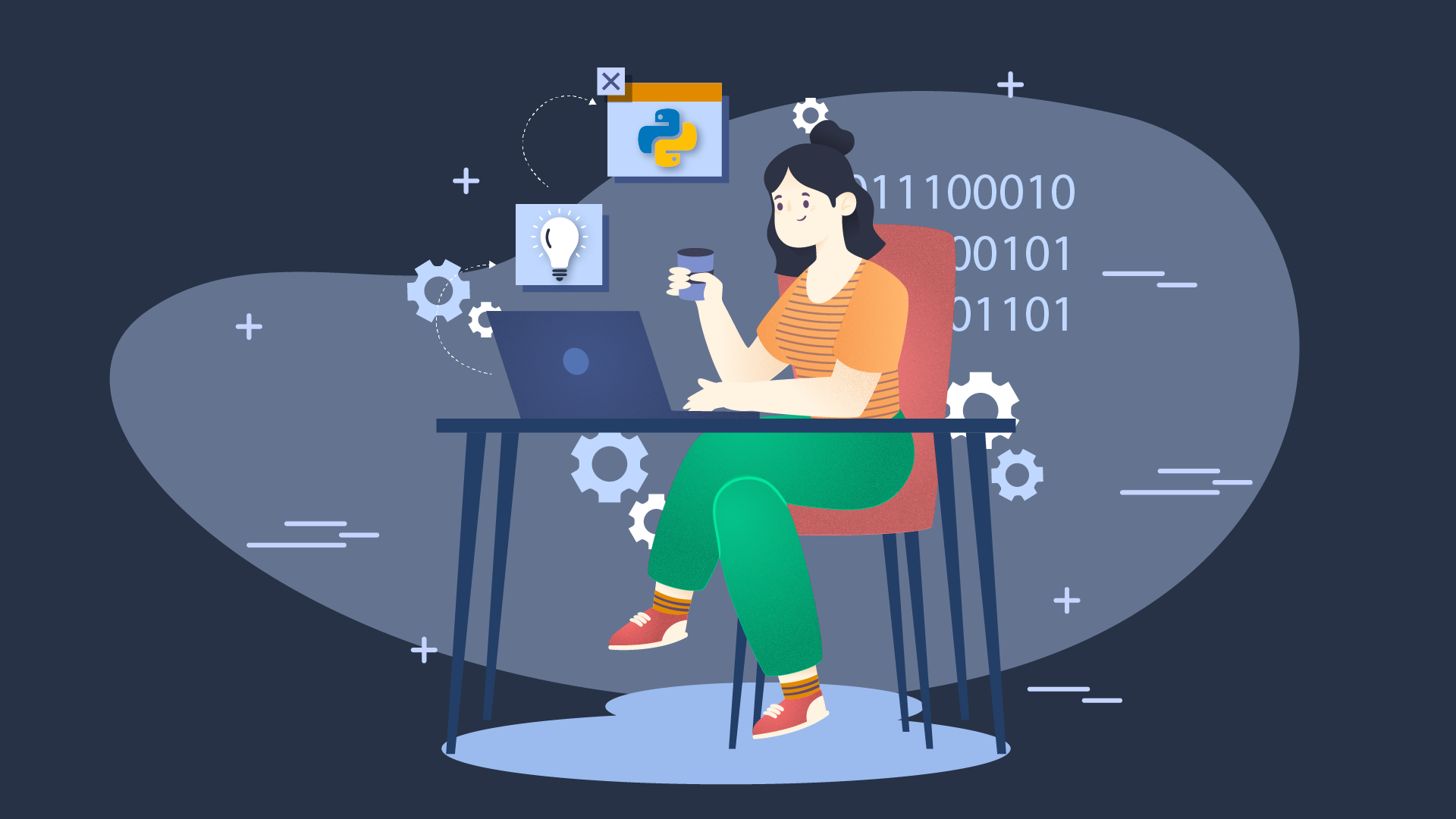
Asynchronous Programming in Python
Brief Intro to Asynchronous Programming
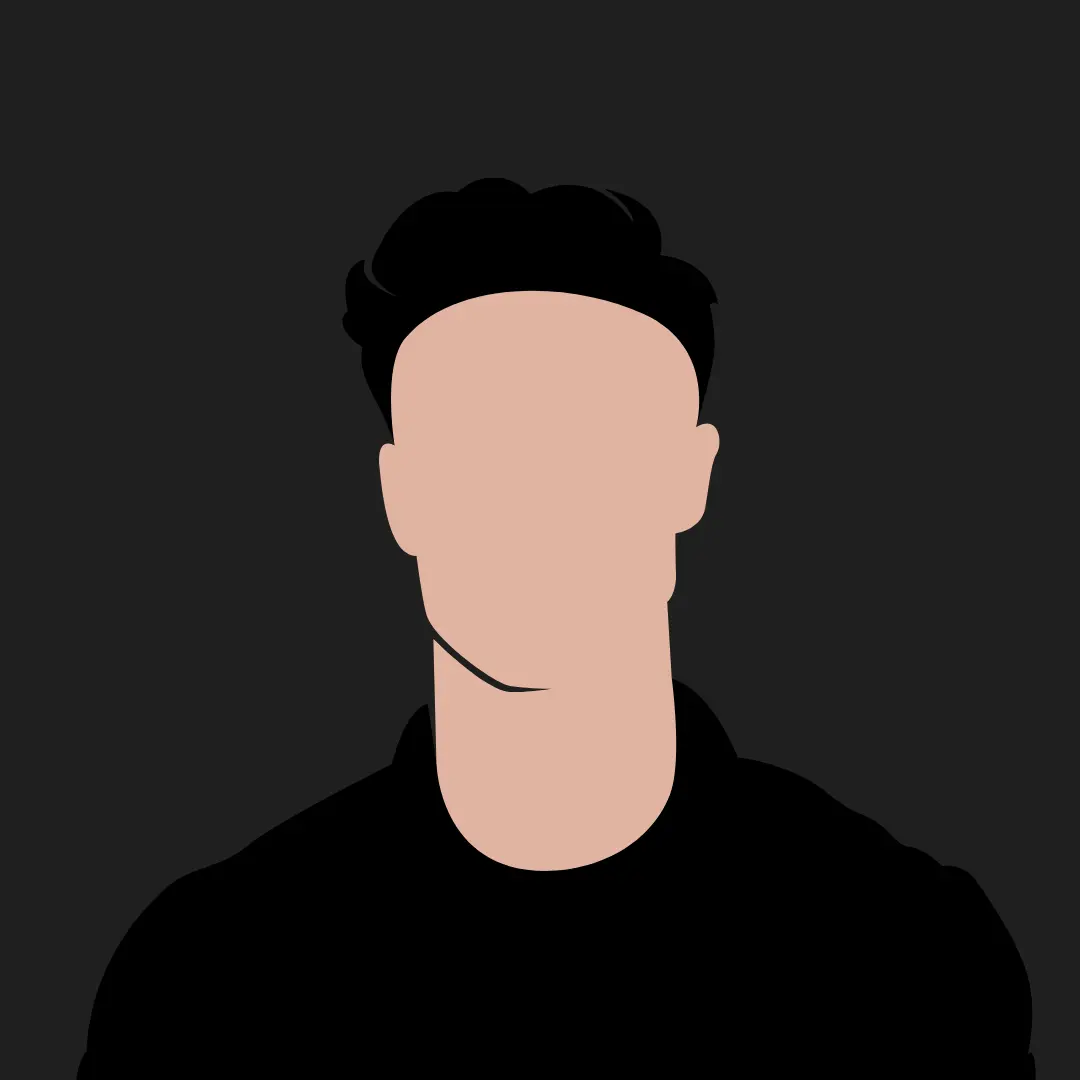
by Ruslan Shudra
Data Scientist
Dec, 2023γ»5 min read
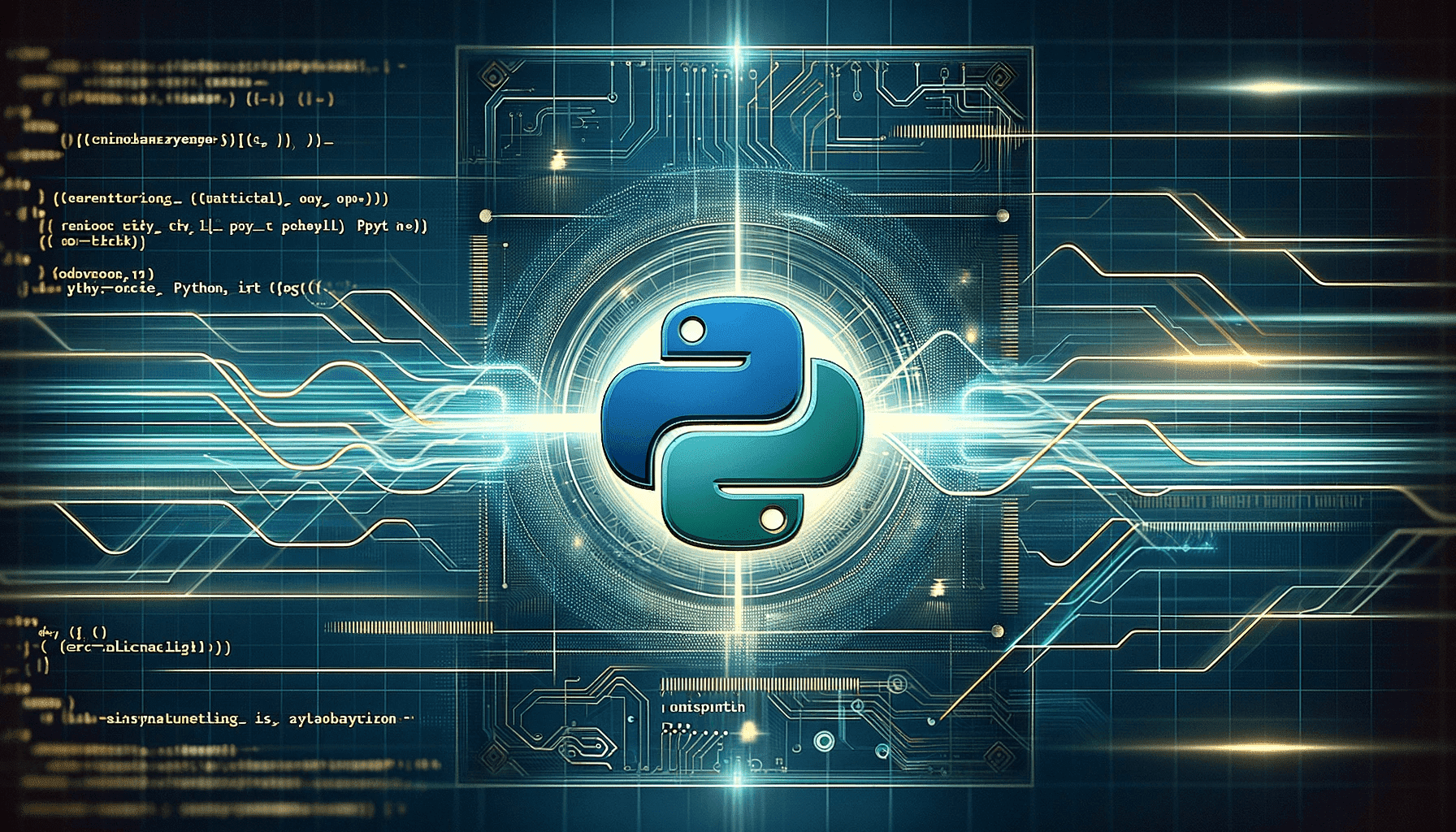
Content of this article