Related courses
See All CoursesUsing super in Python and Its Pitfalls
Navigating Inheritance with super
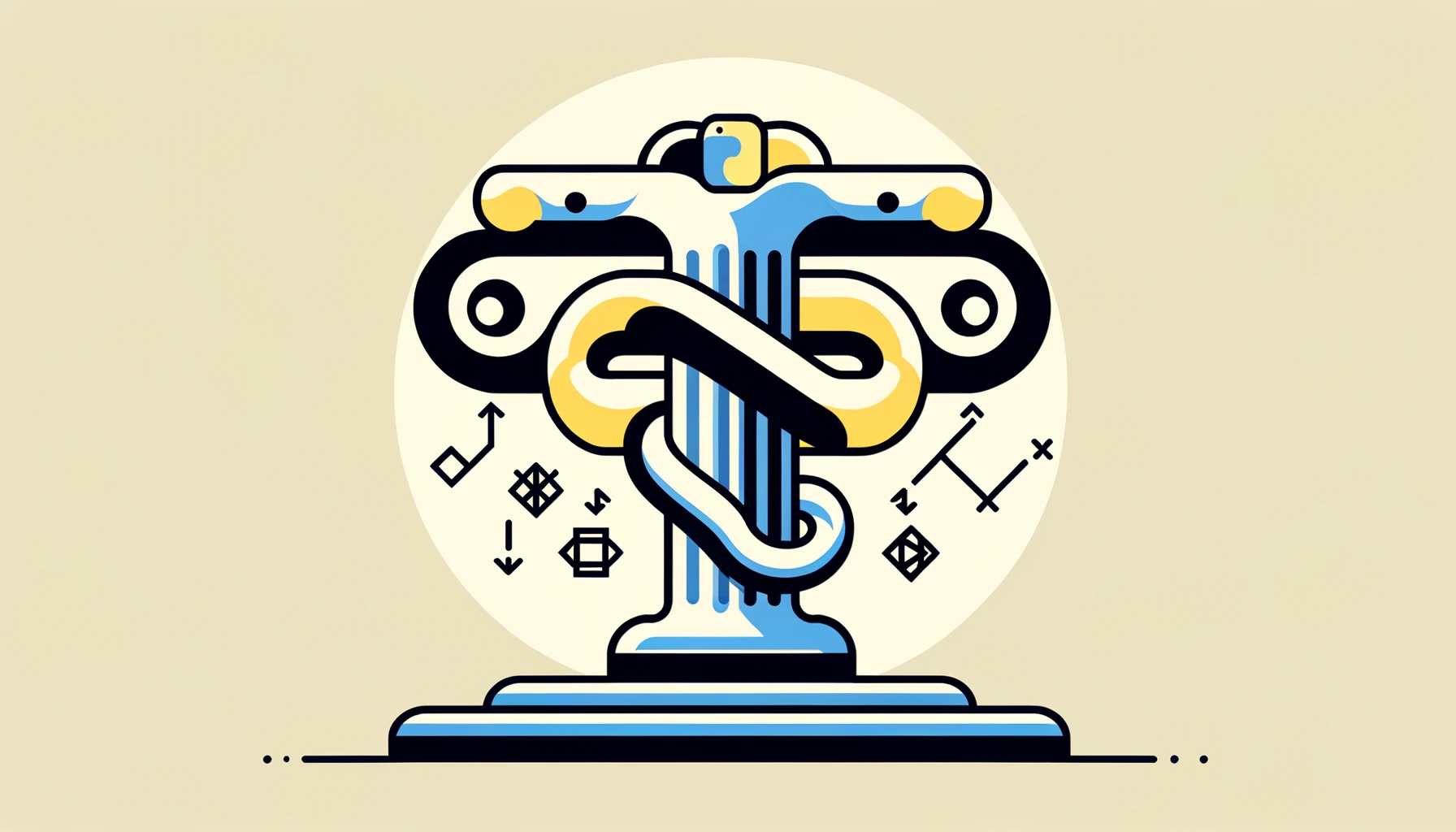
Python's super()
function is an integral part of object-oriented programming, facilitating a smoother inheritance mechanism by allowing subclasses to access methods from their superclass without directly naming them. This article delves into the nuances of super()
, discussing its benefits, common pitfalls, and contrasts it with the traditional approach of explicitly calling superclass methods by name.
What is `super()`?
At its core, super()
in Python returns a temporary object of the superclass that allows access to its methods. This feature is especially useful in inheritance and when overriding methods in the subclass. It streamlines method calls, making code more maintainable and less prone to errors.
Here is its general syntax:
python
super()
can be used within any method definition, where method_name
is the method in the superclass you wish to call, and args
are the arguments to that method.
Benefits of Using `super()`
1. Code Maintenance: By not hard-coding the parent class's name, super()
makes the code more adaptable and easier to update. Changes in the class hierarchy require less effort to manage.
2. Support for Multiple Inheritance: Python supports multiple inheritance, where a class can inherit features from more than one base class. super()
simplifies the call to methods when multiple classes are involved, respecting the method resolution order (MRO).
3. Consistency in Initializer Chains: Ensures that every parent class is properly initialized, which can be critical in deep inheritance hierarchies.
Run Code from Your Browser - No Installation Required
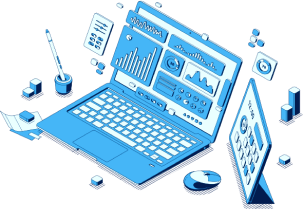
How to Use `super()`
The real power of super()
shines through in its ability to simplify and streamline the use of inherited methods. Here's a closer look at its application:
python
This example shows a basic inheritance scenario where Dog
inherits from Animal
. The Dog
class initializes itself and also ensures the Animal
class is properly initialized using super()
.
Common Pitfalls and Their Avoidance
While super()
is powerful, its misuse or misunderstanding can lead to several issues:
1. Method Resolution Order (MRO) Misunderstandings: super()
does not call the methods of the immediate parent class but follows the MRO, which can be counterintuitive in complex inheritance hierarchies. Understanding the MRO is crucial to predict how methods are called.
2. Inconsistent Initialization: Omitting a super().__init__()
call in a subclass can lead to uninitialized parent classes, causing errors or bugs that are hard to track down.
3. Misapplying in Single Inheritance: Using super()
in a straightforward single inheritance scenario might seem overkill, but it ensures consistency and future-proofs the code against changes in the class hierarchy.
Careful planning of class hierarchies and consistent use of super()
can mitigate most issues. Regularly reviewing the MRO and testing code for multiple inheritance scenarios are good practices.
`super()` vs. Explicit Class Name Calls
Traditionally, to call a method in a superclass, you would explicitly use the superclass's name. However, this approach has limitations:
- Hardcoding superclass names makes the code less flexible and more prone to errors if the inheritance hierarchy changes.
- Multiple inheritance becomes cumbersome as you must manually manage the order and logic of superclass method calls.
super()
, on the other hand, dynamically determines the next class in line according to the MRO, making method calls more predictable and manageable in complex inheritance scenarios.
Consider a scenario with multiple inheritance, highlighting how super()
manages complexity elegantly compared to explicit calls:
python
Using super()
ensures that each shout
method in the hierarchy is called in the correct order, respecting Python's MRO. This would be more complex and error-prone if done using explicit class names.
Start Learning Coding today and boost your Career Potential
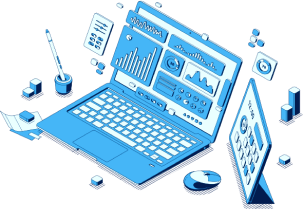
Conclusion
super()
is a powerful feature in Python that, when used correctly, can significantly enhance the flexibility and maintainability of code, especially in complex inheritance scenarios. Understanding its mechanics and common pitfalls is crucial for any Python developer looking to master object-oriented programming.
By comparing super()
with traditional explicit superclass calls, it's clear that super()
offers a more dynamic and robust approach to dealing with inheritance, particularly with its automatic handling of method resolution order and support for multiple inheritance.
FAQs
Q: Is super()
necessary in single inheritance scenarios?
A: While not strictly necessary, using super()
even in single inheritance scenarios is considered good practice for consistency and future-proofing code against changes.
Q: Can super()
handle static and class methods?
A: Yes, super()
can be used with both static and class methods, but it requires passing the class and instance (if applicable) explicitly to super()
.
Q: How does Python determine the method resolution order (MRO)?
A: Python uses the C3 linearization algorithm to determine the MRO. It ensures a consistent order by linearizing the class hierarchy.
Q: What are the implications of not using super()
correctly in multiple inheritance?
A: Incorrect use or omission of super()
in multiple inheritance can lead to skipped initializations, repeated method calls, or incorrect method resolutions, leading to bugs and unpredictable behavior.
Q: How can I view the MRO of a class?
A: You can view the MRO of a class by calling the __mro__
attribute or the mro()
method on the class. This can help in understanding how super()
resolves method calls.
Related courses
See All CoursesThe SOLID Principles in Software Development
The SOLID Principles Overview
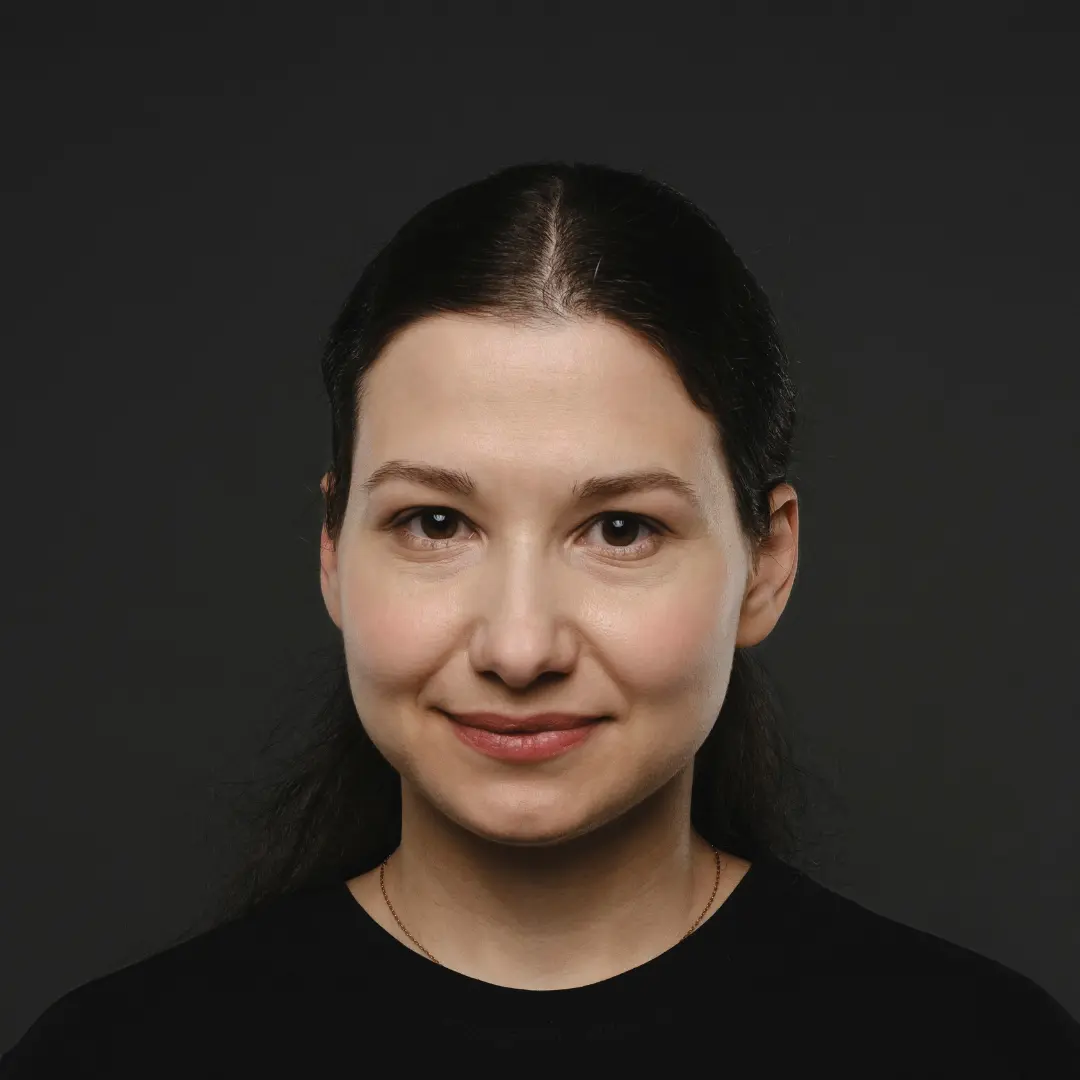
by Anastasiia Tsurkan
Backend Developer
Nov, 2023γ»8 min read
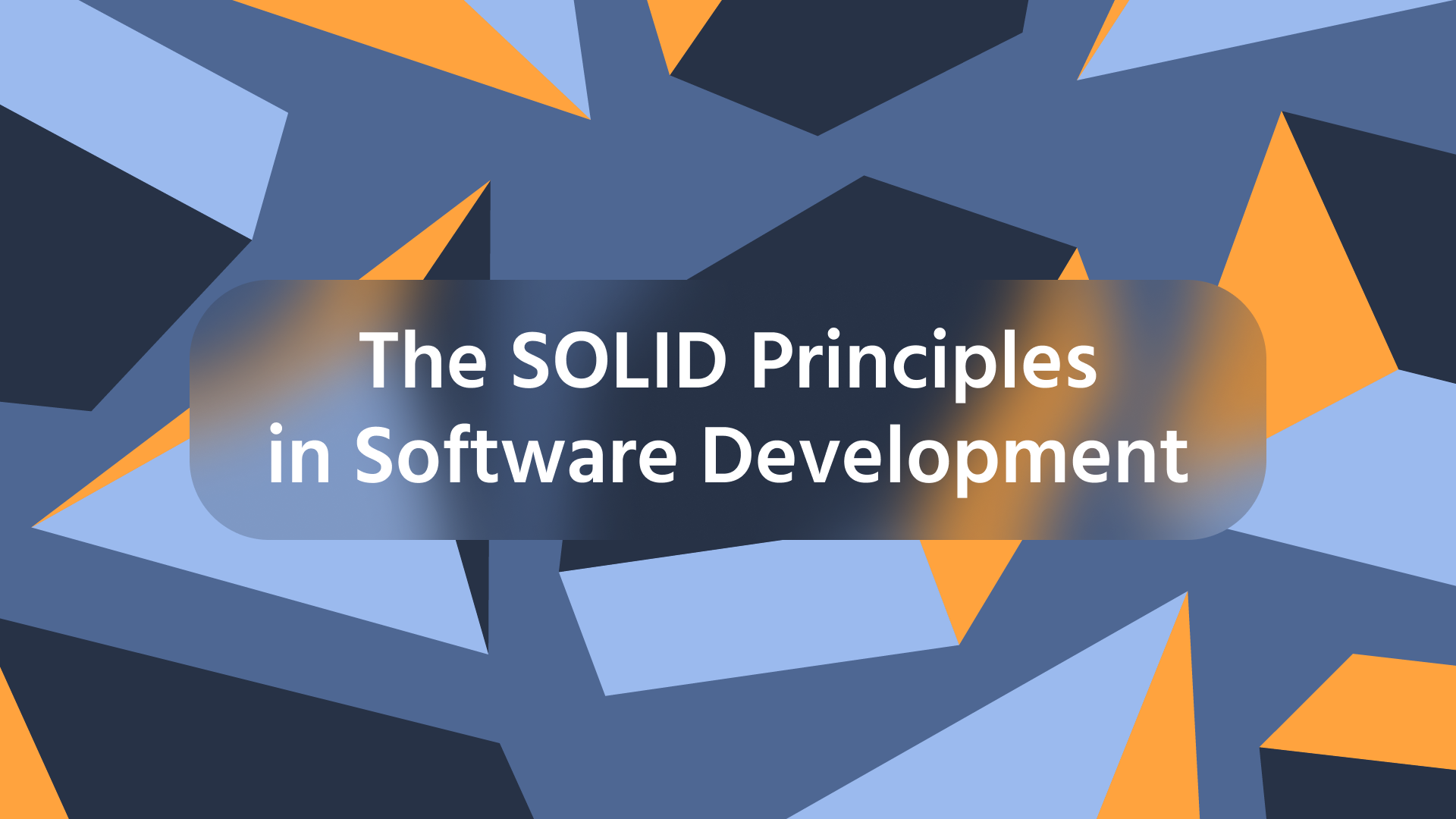
Match-case Operators in Python
Match-case Operators vs if-elif-else statements
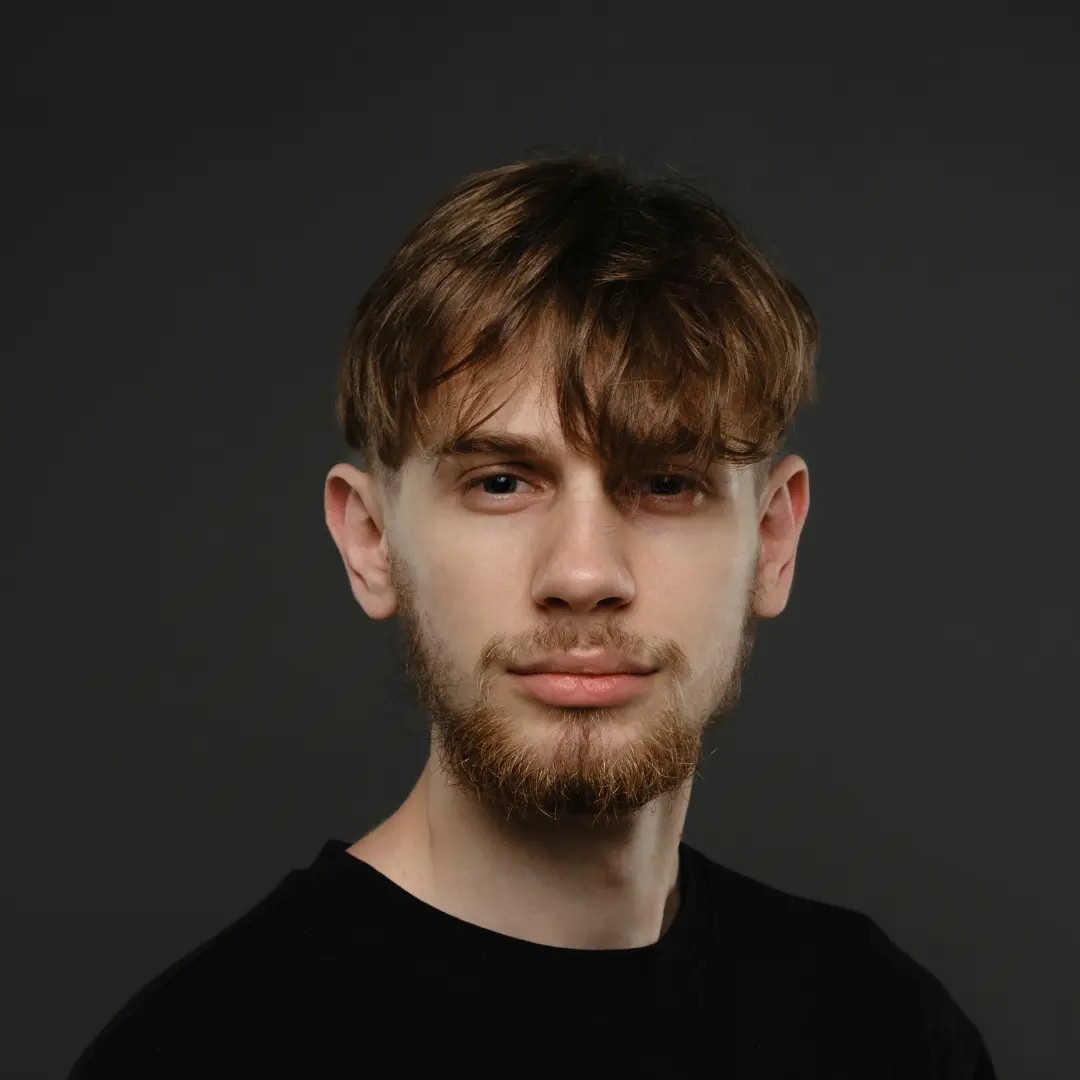
by Oleh Lohvyn
Backend Developer
Dec, 2023γ»6 min read
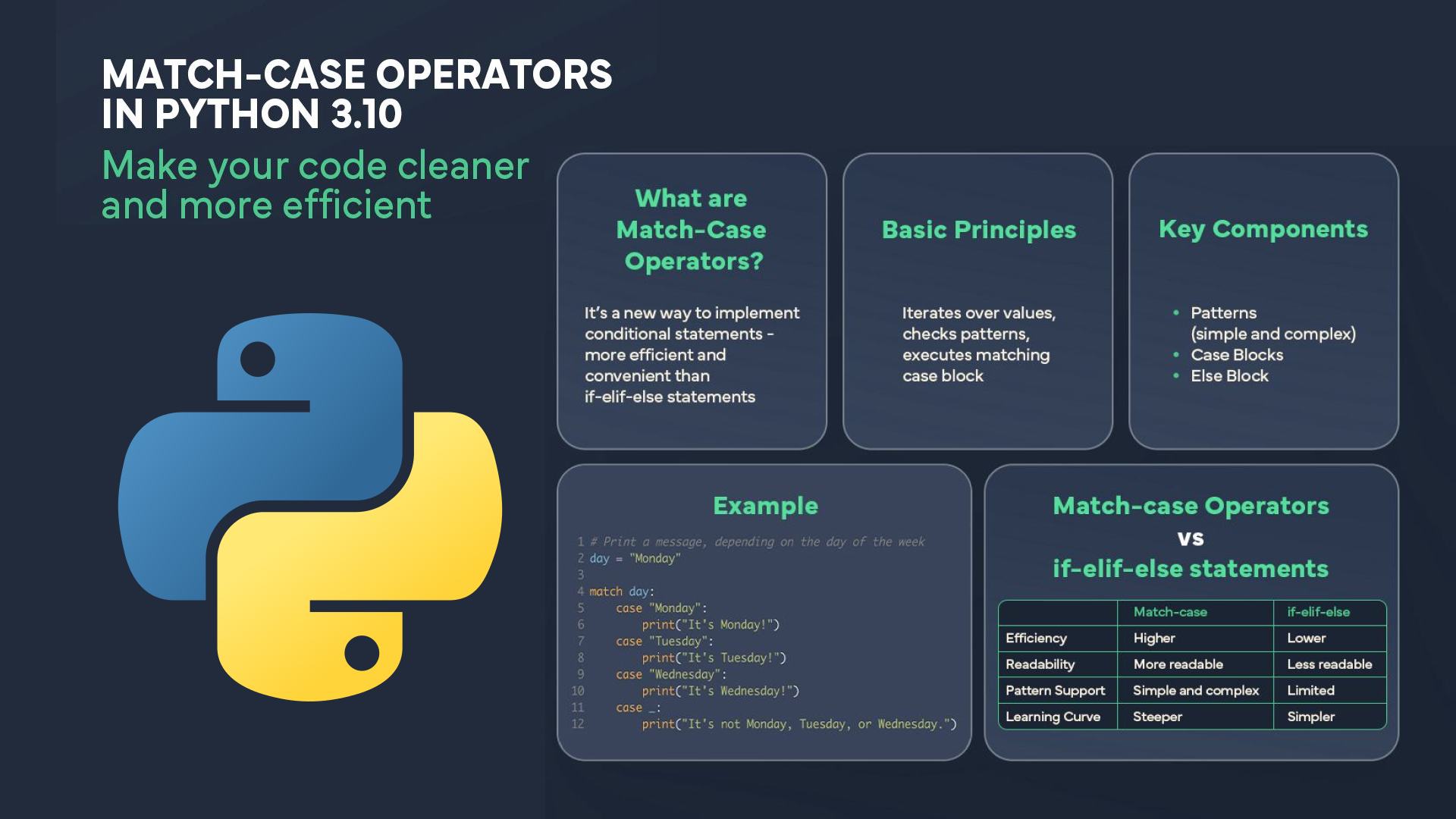
30 Python Project Ideas for Beginners
Python Project Ideas
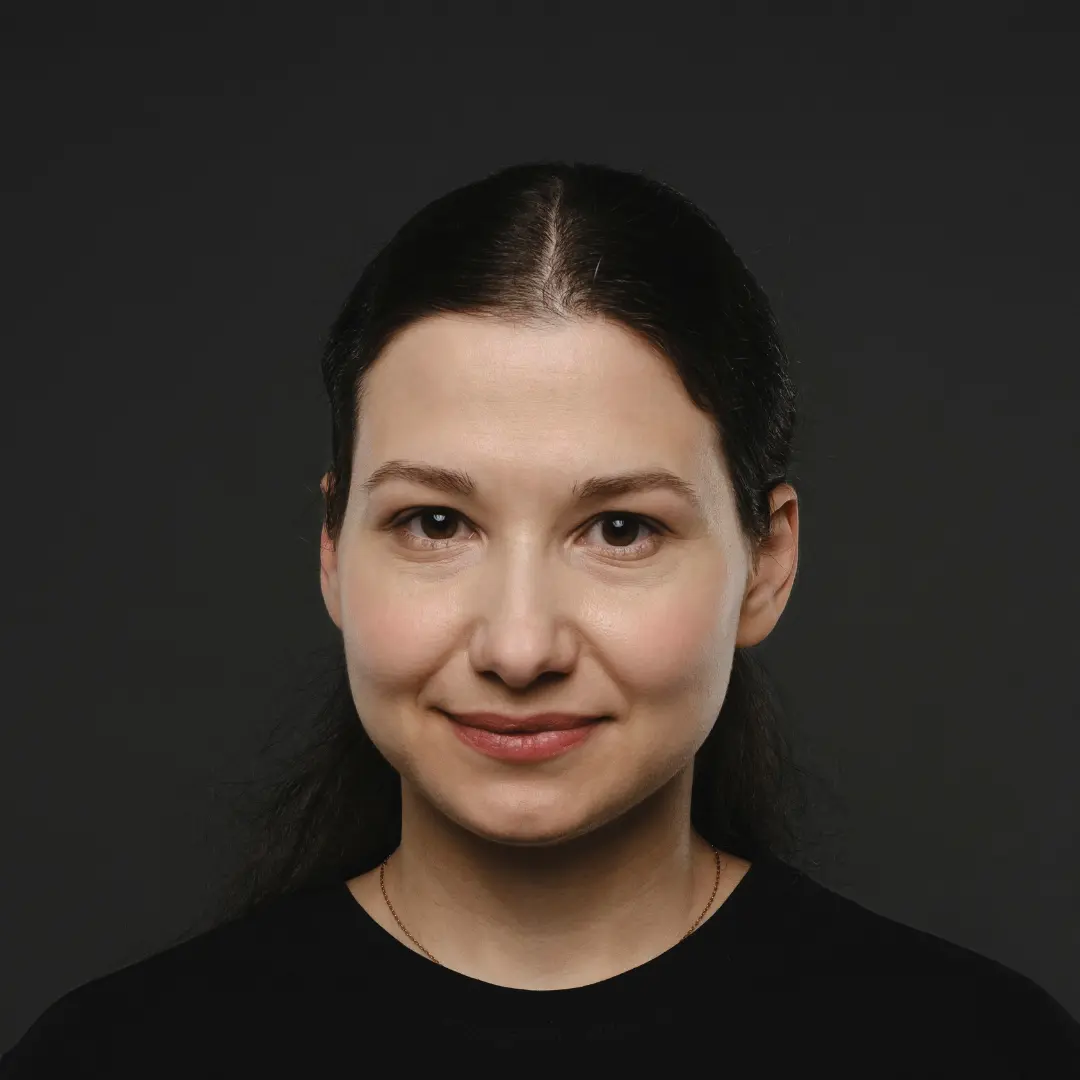
by Anastasiia Tsurkan
Backend Developer
Sep, 2024γ»14 min read
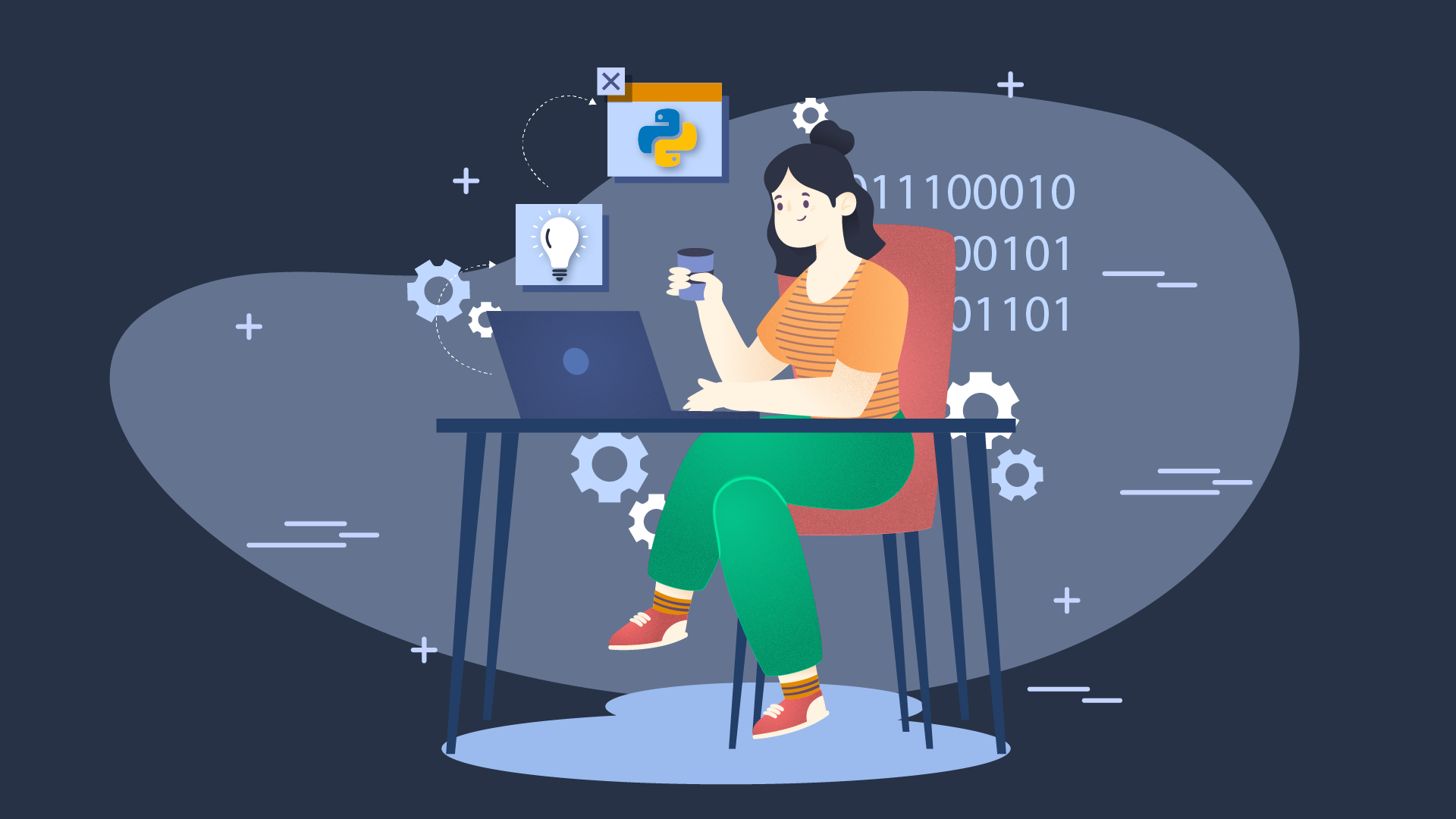
Content of this article