Related courses
See All CoursesIntermediate
React Mastery
Take your web development skills to the next level with the course on building modern web applications using React. Learn to create dynamic user interfaces, efficiently manage state and data, and leverage the latest technologies and best practices in web development. Join now and unlock the full potential of React to create highly interactive and engaging web apps that meet the demands of today's users.
Advanced
Next.js 14 Mastery for Building Modern Web Apps
Next.js is a React framework designed for creating full-stack web applications. With Next.js, you utilize React to construct user interfaces while benefiting from additional features and optimizations provided by the framework.
Beginner
Introduction to JavaScript
Learn the fundamentals of JavaScript, the backbone of dynamic web development. Discover essential concepts like syntax, variables, data types, and operators. Explore how to use conditional statements, loops, and functions to create interactive and efficient programs. Master the building blocks of JavaScript and lay the groundwork for more advanced programming skills.
Ways to Fetch Data in React Apps
Different Ways to Fetch Data in React: Fetch API, Axios, and Async/Await
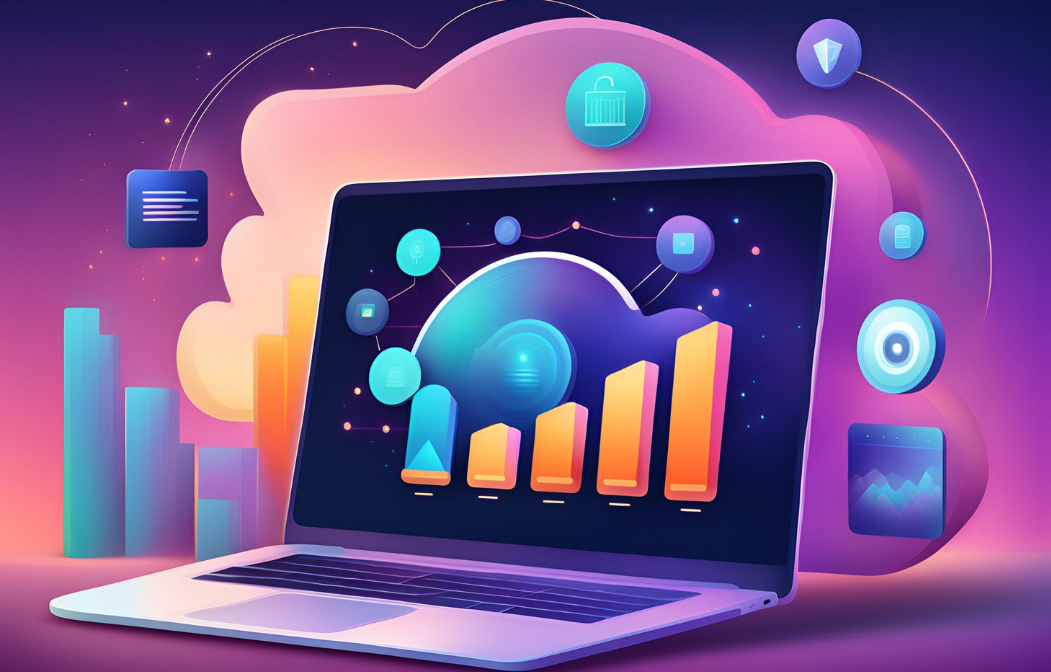
Fetching data from APIs is a common task in modern web development. In React, there are several methods to achieve this, each with its own benefits and use cases. This article will explore three primary methods: the Fetch API, Axios, and Async/Await.
Fetch API
The Fetch API is a built-in JavaScript interface that allows you to make network requests similar to XMLHttpRequest (XHR). However, the Fetch API is more powerful and flexible. It is built into modern web browsers and provides a more straightforward way to fetch resources asynchronously.
Features of Fetch API:
- Built-in: No need to install additional libraries;
- Promise-based: Uses JavaScript Promises to handle asynchronous operations;
- Streamlined Syntax: Easier to read and write compared to older XHR methods.
Example:
js
Run Code from Your Browser - No Installation Required
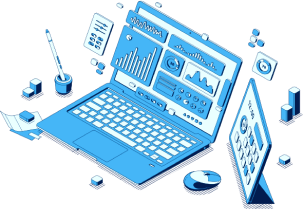
Axios
Axios is a popular JavaScript library for making HTTP requests from both the browser and Node.js. It simplifies the process of sending asynchronous HTTP requests to REST endpoints and performing CRUD operations. Axios is often preferred for its simplicity and additional features.
Features of Axios:
- Ease of Use: Simple and intuitive API;
- Interceptors: Allows you to intercept requests or responses before they are handled;
- Automatic JSON Transformation: Automatically transforms JSON data;
- Error Handling: Provides a more robust way to handle errors.
Example:
js
Async/Await
Async/Await is a modern JavaScript feature introduced in ES2017 (ES8). It provides a way to write asynchronous code in a synchronous manner, making it easier to read and maintain. Async functions return a Promise, and the await
keyword can be used to pause the execution until the Promise is resolved.
Features of Async/Await:
- Synchronous-like Code: Makes asynchronous code appear more like synchronous code;
- Error Handling: Easier to manage errors using try/catch blocks;
- Readability: Improves the readability of the code.
Example:
js
Conclusion
Choosing the right method for fetching data in React apps depends on your specific use case. The Fetch API is a robust, built-in solution ideal for simple requests. Axios offers additional features that simplify complex requests and error handling. Async/Await provides a cleaner syntax that improves code readability and maintainability. Understanding the strengths and limitations of each method will help you make an informed decision and write more efficient and maintainable React applications.
Start Learning Coding today and boost your Career Potential
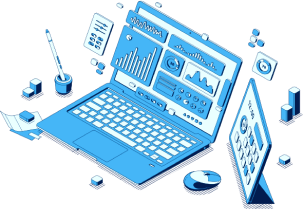
FAQs
Q: What is the Fetch API?
A: The Fetch API is a built-in JavaScript interface for making network requests. It uses Promises to handle asynchronous operations and is available in modern web browsers.
Q: Why should I use Axios instead of the Fetch API?
A: Axios offers a simpler and more intuitive API, automatic JSON transformation, and additional features like request/response interceptors and better error handling.
Q: What are the benefits of using Async/Await in JavaScript?
A: Async/Await makes asynchronous code look more like synchronous code, improving readability and maintainability. It also simplifies error handling with try/catch blocks.
Q: Do I need to install any libraries to use the Fetch API?
A: No, the Fetch API is built into modern web browsers, so no additional libraries are needed.
Q: Can I use Async/Await with the Fetch API?
A: Yes, Async/Await can be used with the Fetch API to write cleaner and more readable asynchronous code.
Q: Is Axios available for both browser and Node.js environments?
A: Yes, Axios can be used in both browser and Node.js environments, making it a versatile option for making HTTP requests.
Related courses
See All CoursesIntermediate
React Mastery
Take your web development skills to the next level with the course on building modern web applications using React. Learn to create dynamic user interfaces, efficiently manage state and data, and leverage the latest technologies and best practices in web development. Join now and unlock the full potential of React to create highly interactive and engaging web apps that meet the demands of today's users.
Advanced
Next.js 14 Mastery for Building Modern Web Apps
Next.js is a React framework designed for creating full-stack web applications. With Next.js, you utilize React to construct user interfaces while benefiting from additional features and optimizations provided by the framework.
Beginner
Introduction to JavaScript
Learn the fundamentals of JavaScript, the backbone of dynamic web development. Discover essential concepts like syntax, variables, data types, and operators. Explore how to use conditional statements, loops, and functions to create interactive and efficient programs. Master the building blocks of JavaScript and lay the groundwork for more advanced programming skills.
Accidental Innovation in Web Development
Product Development
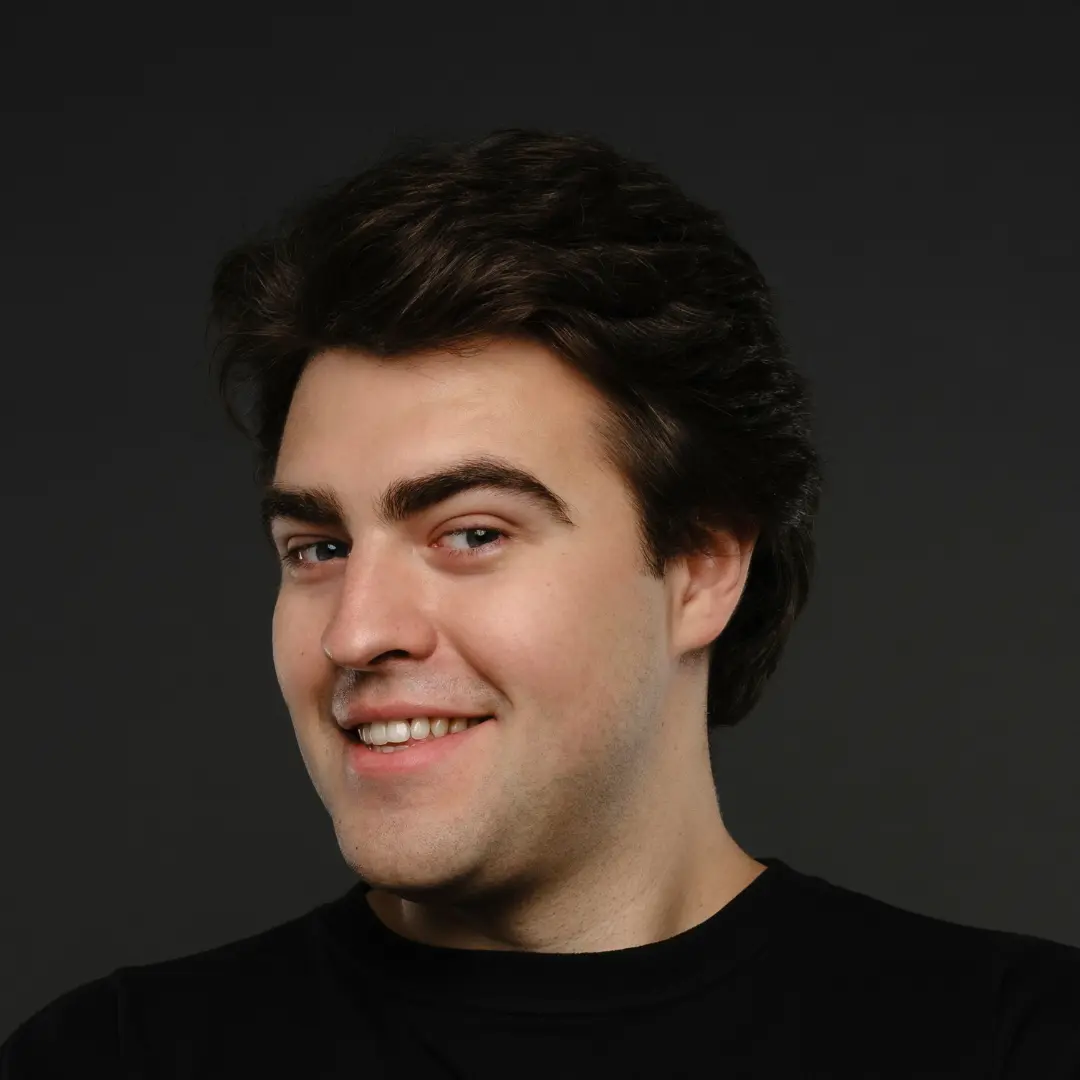
by Oleh Subotin
Full Stack Developer
May, 2024・5 min read
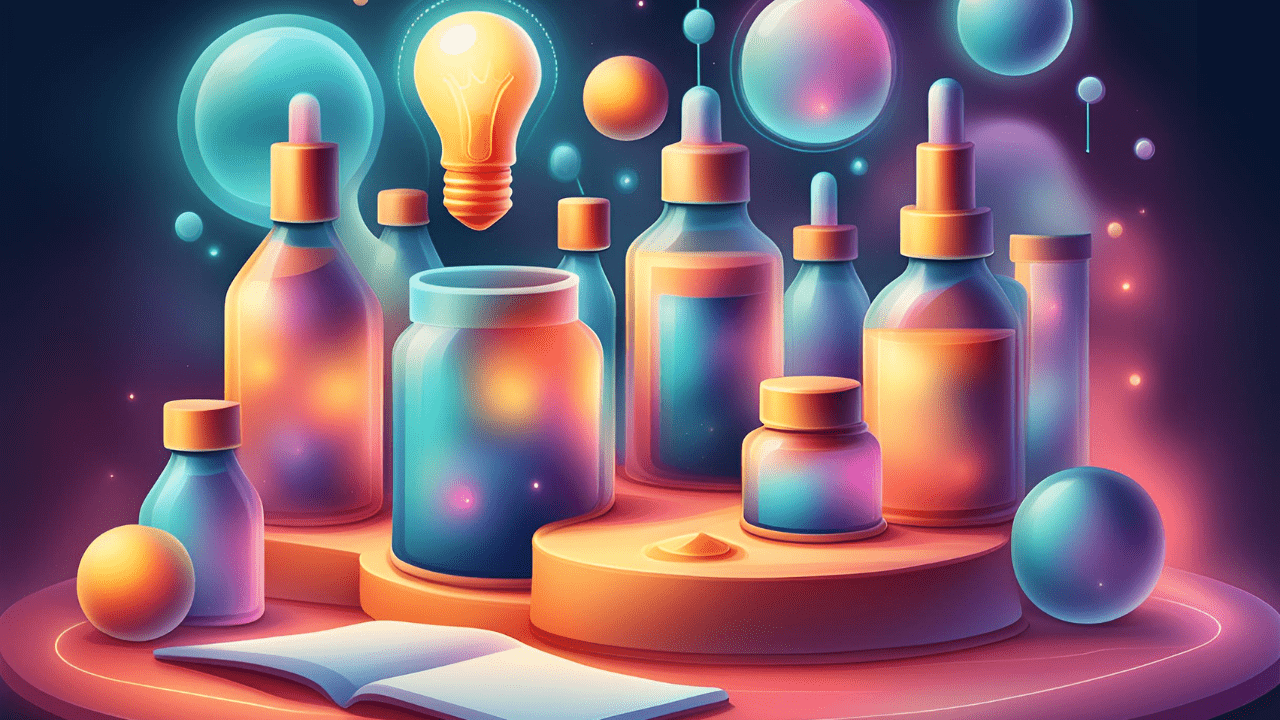
Navigating the YouTube Influencer Maze in Your Tech Career
Staying Focused and Disciplined Amidst Overwhelming Advice
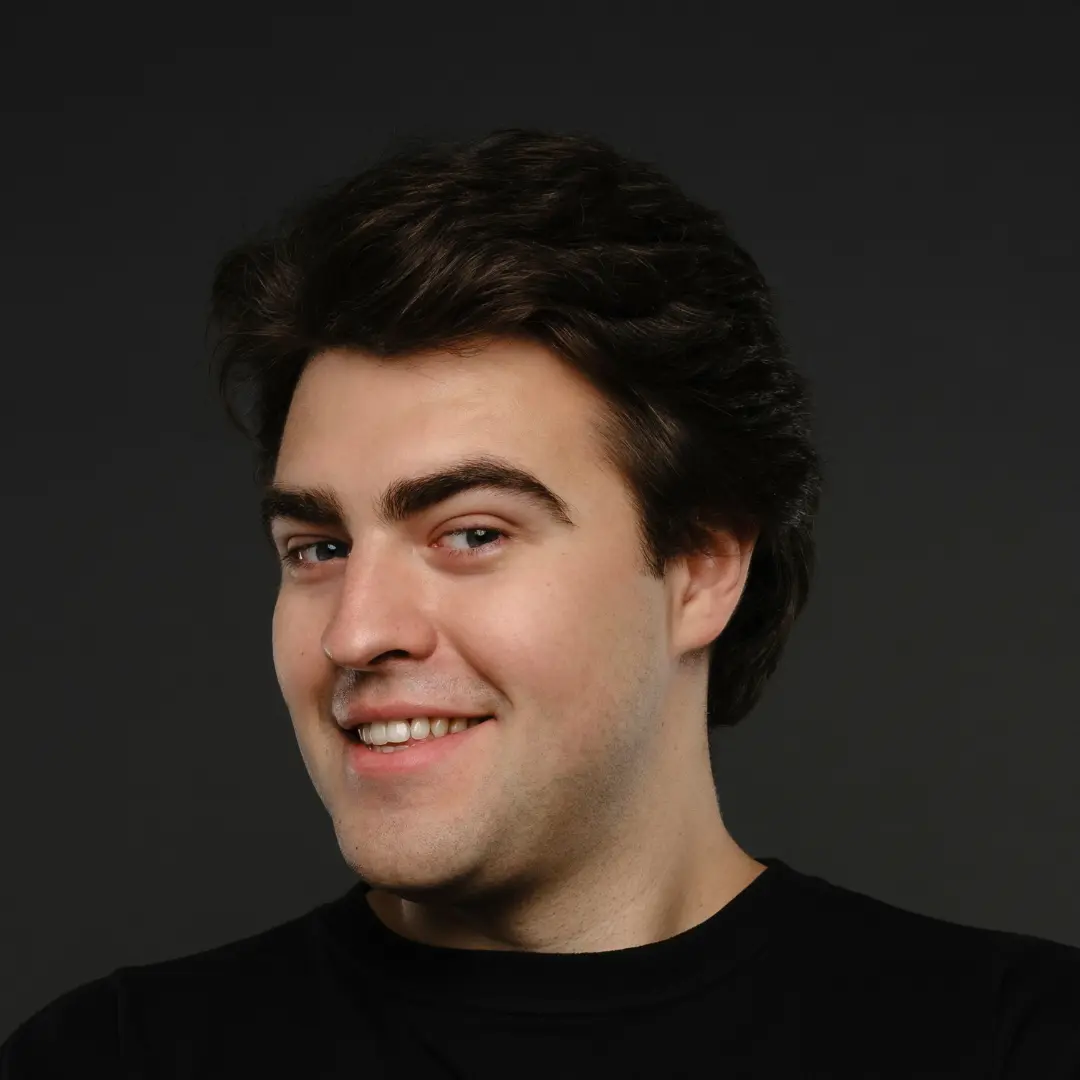
by Oleh Subotin
Full Stack Developer
Jul, 2024・5 min read
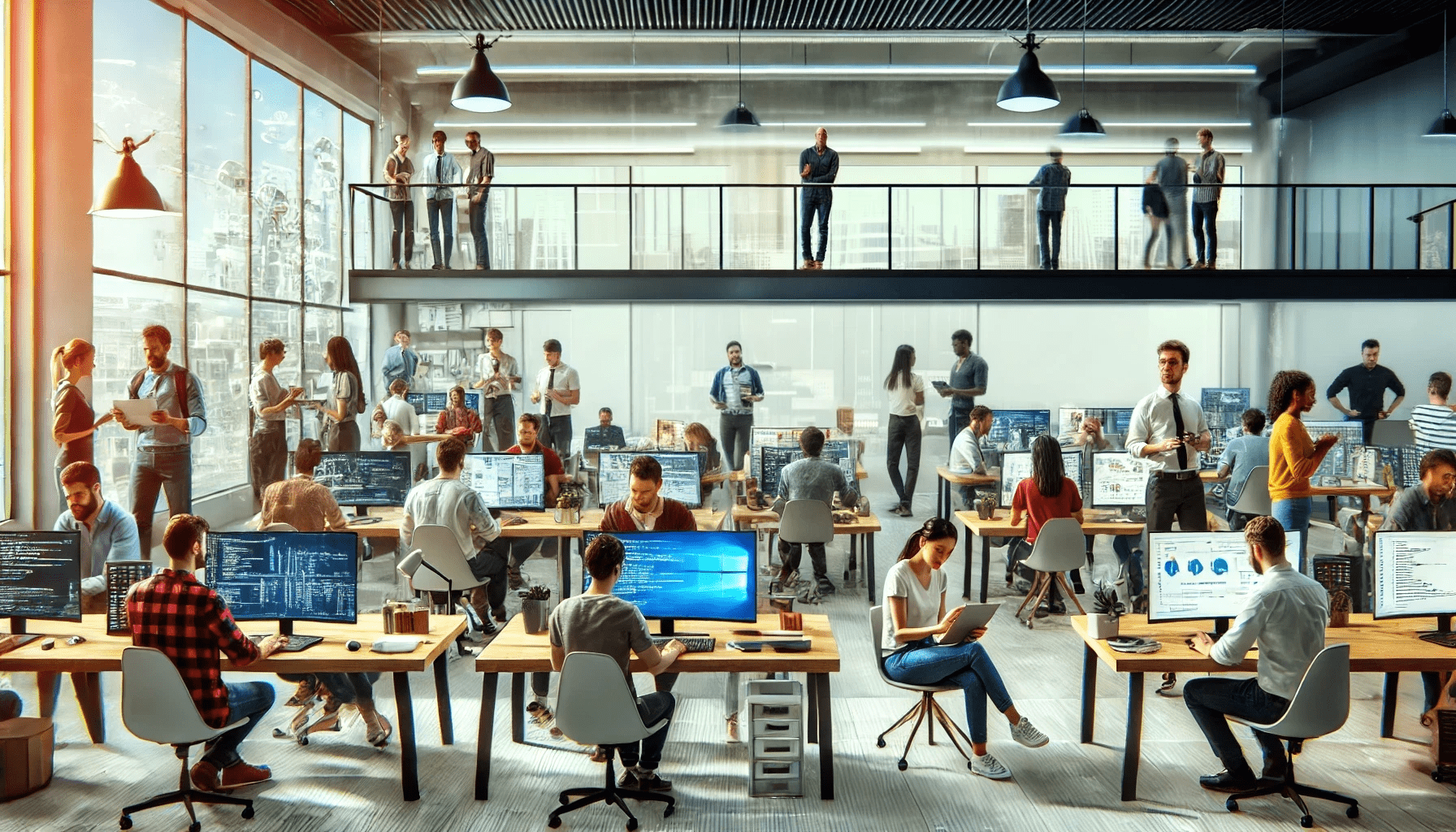
Is a College Degree Essential for a Career as a Developer
Evaluating the Role of Formal Education in Your Tech Career
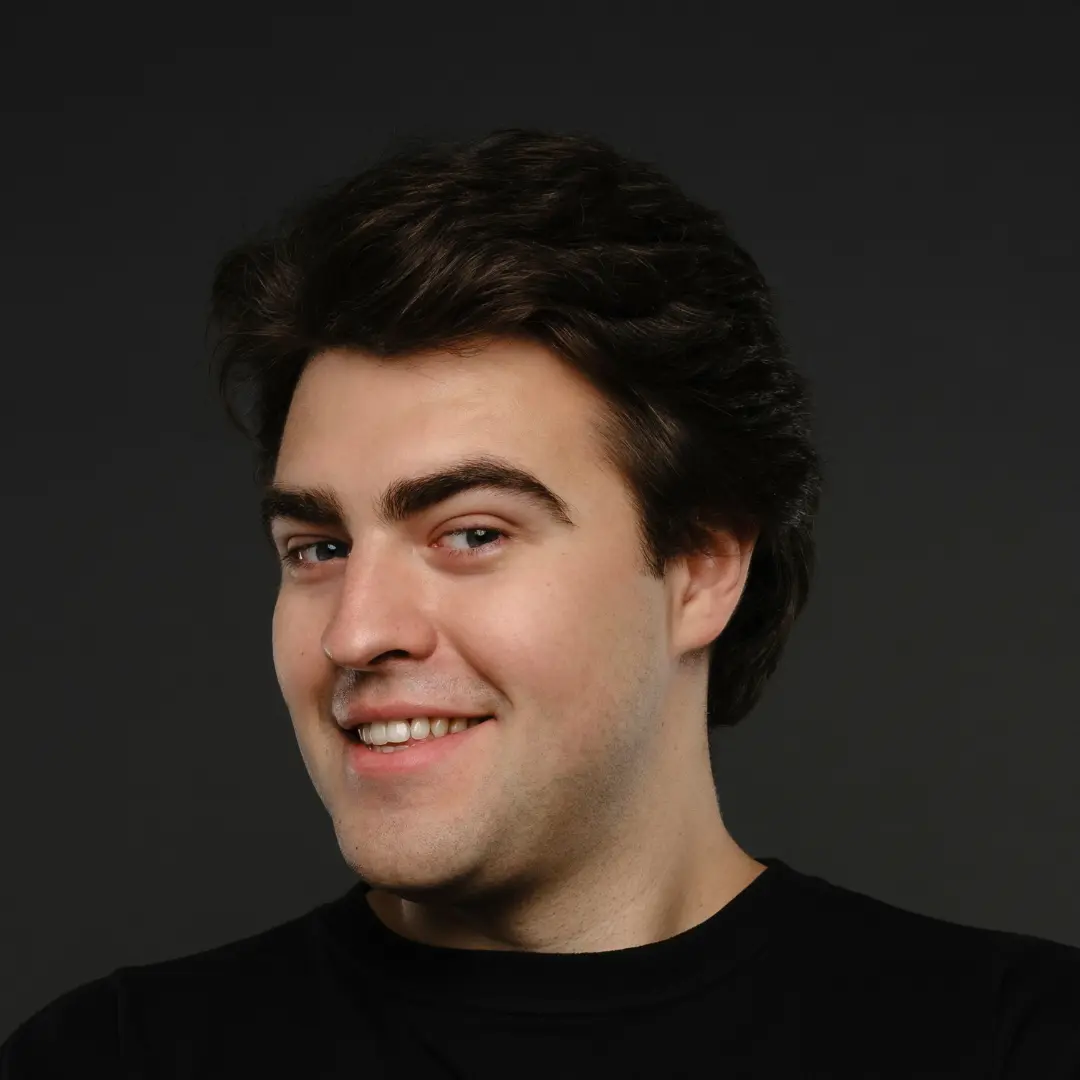
by Oleh Subotin
Full Stack Developer
Jul, 2024・6 min read
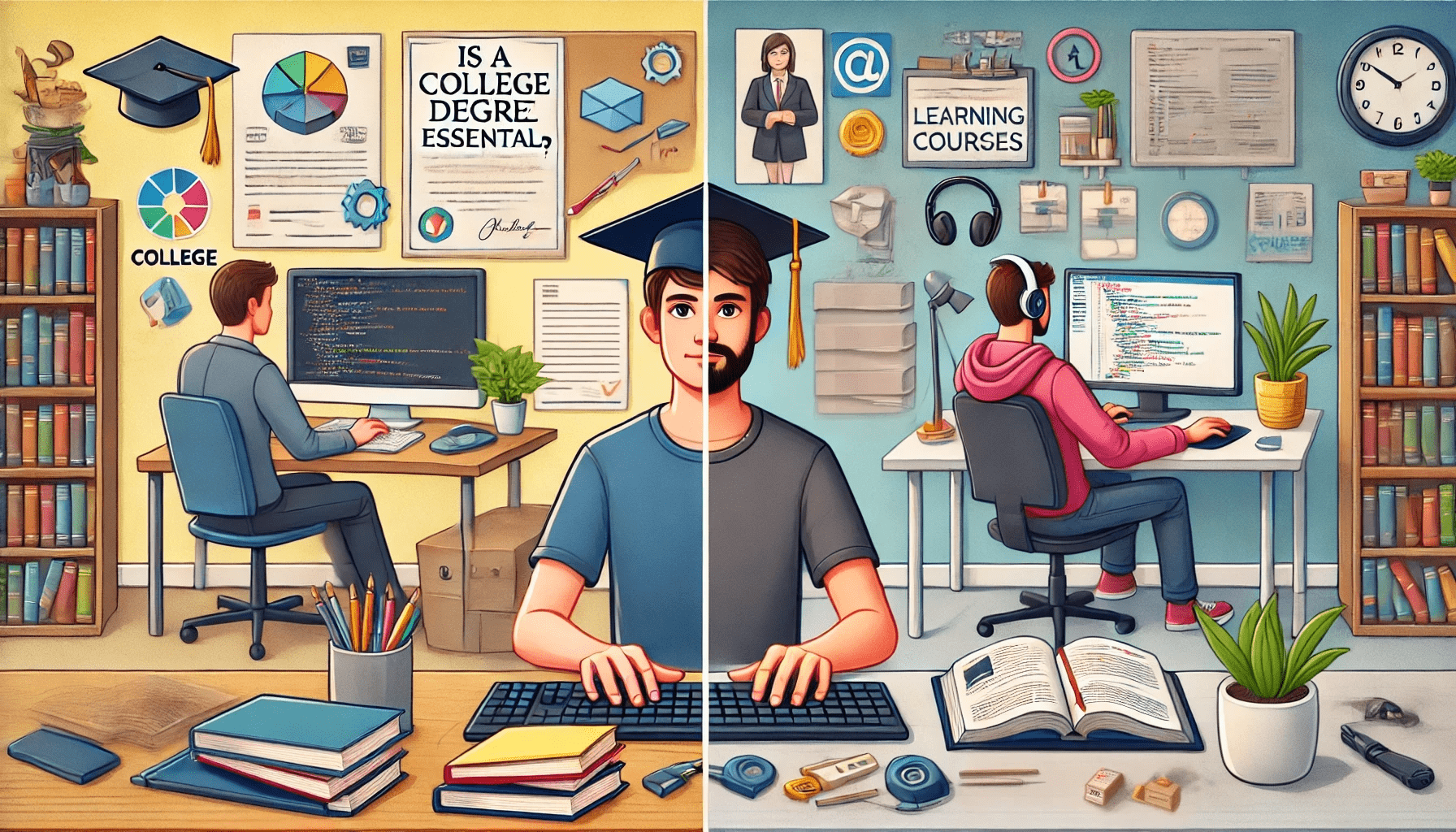
Content of this article