Introduction to JavaScript
Learn the fundamentals of JavaScript, the backbone of dynamic web development. Discover essential concepts like syntax, variables, data types, and operators. Explore how to use conditional statements, loops, and functions to create interactive and efficient programs. Master the building blocks of JavaScript and lay the groundwork for more advanced programming skills.Course
Gain insight into a topic and learn the fundamentals4.7
(318)
- Grasp the basic concepts fundamental to JavaScript including literals, keywords, and variables.
- Understand the structure and syntax of JavaScript code to effectively write and debug.
- Manipulate data using variables and learn the different data types JavaScript supports.
- Perform basic operations, use mathematical expressions, and manage data assignment efficiently.
Equip your business with cutting-edge Data and AI expertise.
Share it on social media and in your performance review
There are 6 modules in this course
Learn the fundamentals of JavaScript, the backbone of dynamic web development. Discover essential concepts like syntax, variables, data types, and operators. Explore how to use conditional statements, loops, and functions to create interactive and efficient programs. Master the building blocks of JavaScript and lay the groundwork for more advanced programming skills.Chosen by students of top schools
Why people choose Codefinity for their career
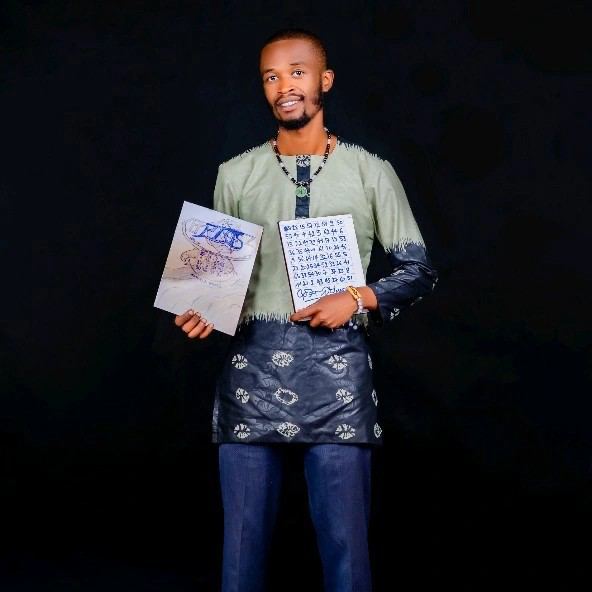
Kwizera Mugisha
The teaching methodology at Codefinity is excellent, and I particularly appreciate how it has prepared me to handle real-world coding problems. Currently, I am delving into Node.js and eagerly anticipate building full-stack projects that integrate all the knowledge I have gained.
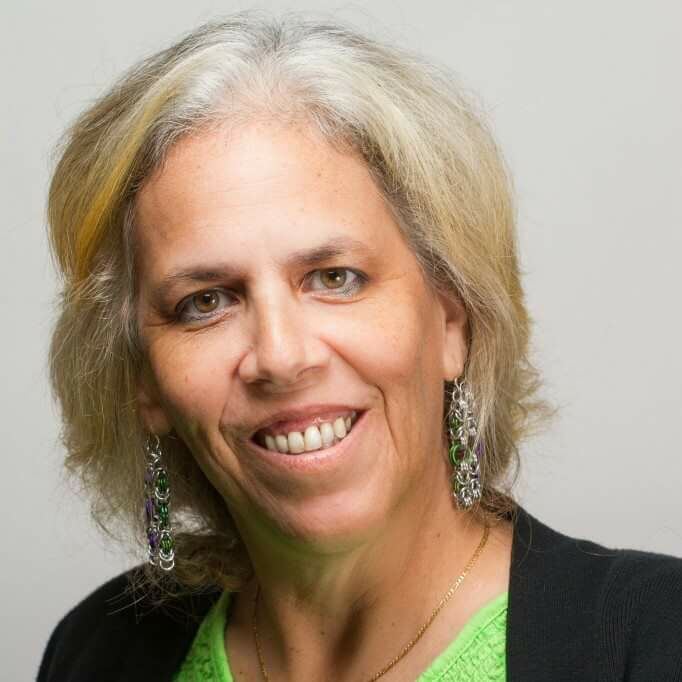
Sherry Barnes-Fox
My first course was 4 hours, I did it in a few days, "nugget-style. The instructions are very clear and easy to understand. There is even a hint to help you get the answer, and if you still cannot get the answer, then you can display the answer. I love the learning style that is used, it engages me.
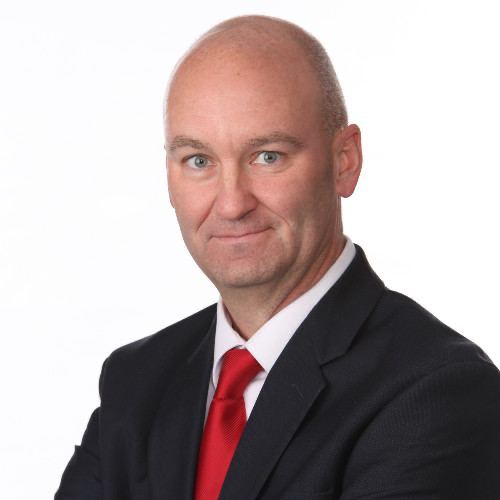
Bill Wagner
I have really liked the browser-based lessons that allow me to code within the lesson. The RUN button allows me to test the code I write before submitting for a grade.
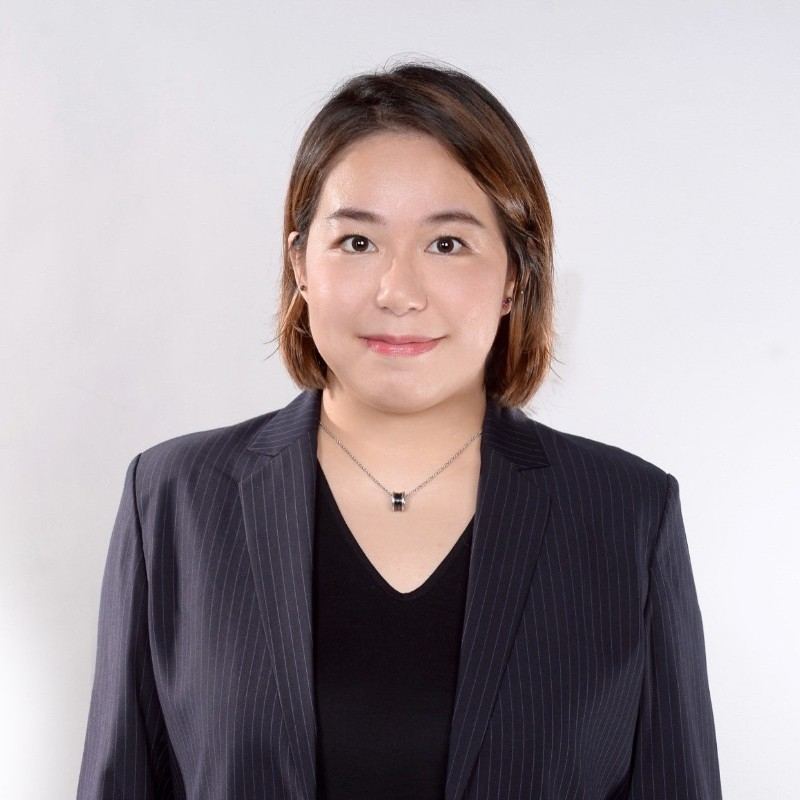
Stephanie Chan
As I went through the first course of the Python track, I liked the way the course was lay out (in easy and digestible modules) with little exercises at the end of each concept.
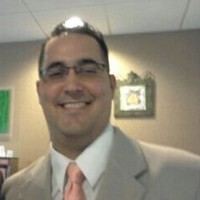
Daniel Chinea
I have gained a lot of practical and logical thinking skills, along with patience for myself and confidence in myself that I can learn programming.
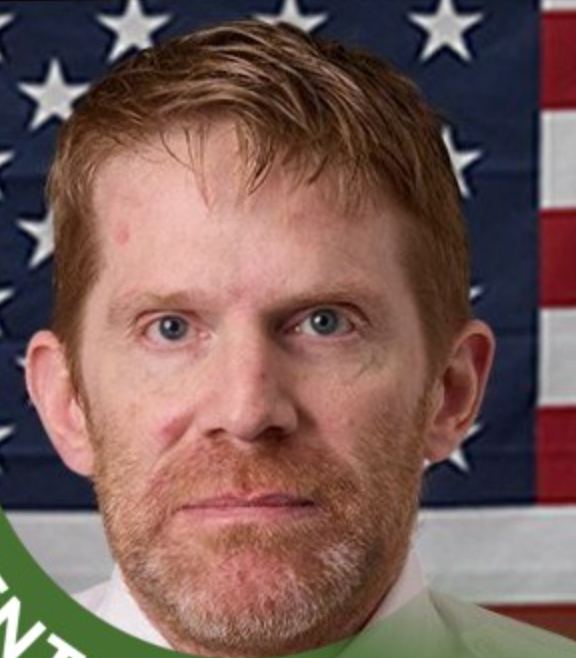
Steve Bruening
The learning was progressive and made it easy to follow along and make progress. I could feel my skills increasing and building on each other as the course went along.
Learner reviews
4.8
50 reviews
5
82%
4
18%
3
0%
2
0%
1
0%
Showing 3 of 50 reviews
Maria Oikonomidi
5
Reviewed on Feb 21, 2025
Artem Lazhenko
5
Reviewed on Feb 15, 2025
Erik Sodel
5
Reviewed on Feb 15, 2025
Recommended if you're interested in learning JavaScript
Embrace the fascination of Tech Skills! Our AI-assistant provides real-time feedback, personalized hints, and error explanations, empowering you to learn with confidence.
With Workspaces, you can create and share projects directly on our platform. We've prepared templates for your convenience
Take control of your career development and commence your path into mastering the latest technologies
Real-world projects elevate your portfolio, showcasing practical skills to impress potential employers
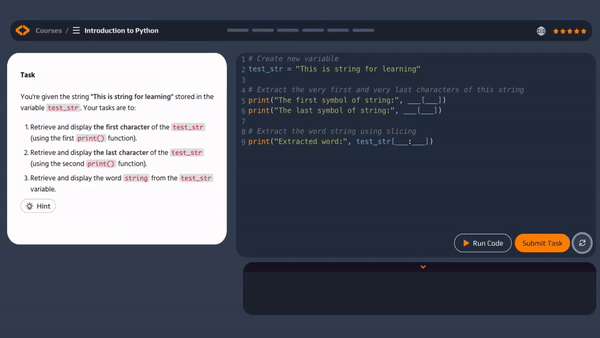
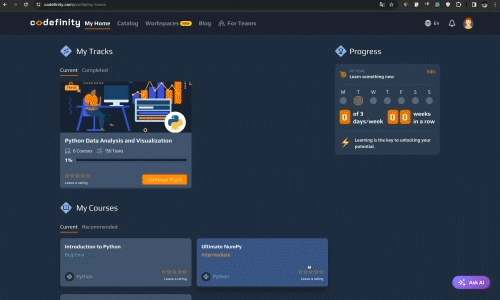
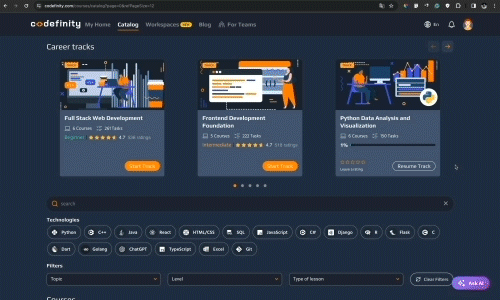
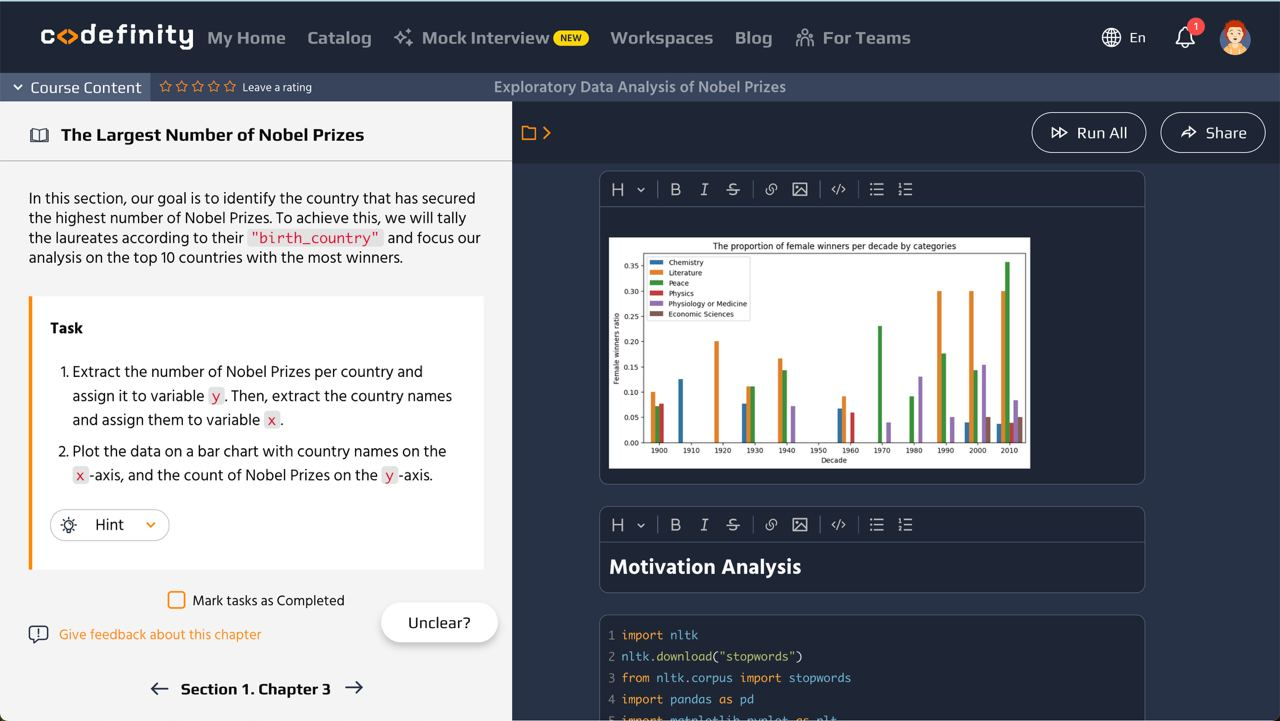
Full catalog access
One subscription opens up this course and our entire catalog of projects and skills.Your subscription also includes:
Frequently asked questions
Still have questions?
Write your question here