Related courses
See All CoursesAdvanced
Java OOP
Those who know OOP can program well. That's what many programmers say. Get ready for an important part of your Java learning journey, mastering which will greatly boost your programming skills in general. You will learn how to effectively use the Java development environment, the principles of Object-Oriented Programming (OOP), and best practices in OOP. You will learn to make your code flexible and deepen your knowledge of previously covered topics. Let's get started!
Intermediate
Java Extended
You will learn about best practices in coding, how to create your own methods and classes, and how to work with them and configure their interaction. You will also understand how Java works at the computer level and how code compilation generally works.
Beginner
Java Basics
Learn the fundamentals of Java and its key features in this course. By the end, you'll be able to solve simple algorithmic tasks and gain a clear understanding of how basic console Java applications operate.
What is Garbage Collector and How Does It Work in Java
Demystifying Java's Automated Memory Management
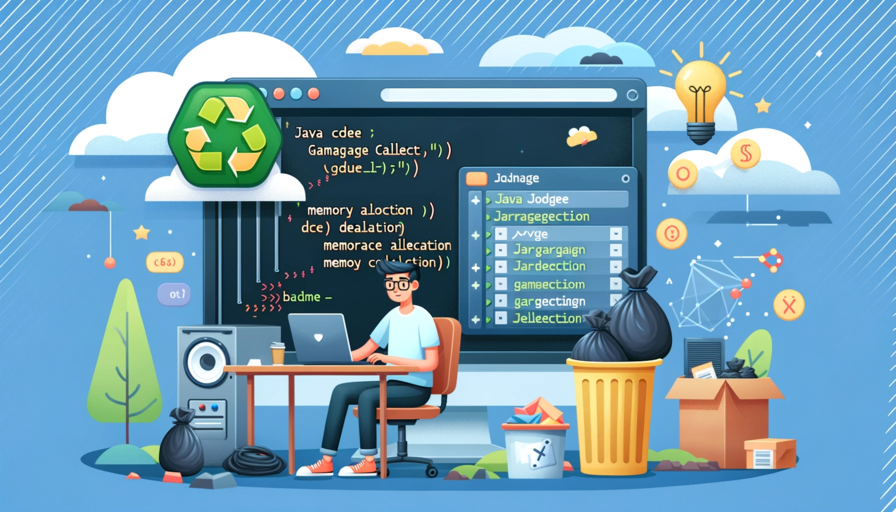
The concept of Garbage Collection in Java is fundamental yet often challenging for newcomers to grasp. This in-depth article aims to provide a thorough understanding of the Java Garbage Collector (GC), its mechanisms, its vital role in memory management, the object lifecycle in Java, and its function within the Spring framework. Additionally, we'll delve into practical examples to elucidate how garbage collection works in real-world Java applications.
The Essence of Garbage Collector in Java
The Garbage Collector in Java is an automatic memory management system that identifies and discards objects that are no longer in use, thereby freeing up memory resources and optimizing the application's performance.
Key Attributes:
- Automated Process: Java GC autonomously cleans up memory, relieving programmers from manual memory management.
- Memory Leak Prevention: It plays a critical role in preventing memory leaks, which can lead to application crashes and inefficiency.
How Garbage Collector Operates
The operation of the Garbage Collector in Java involves several steps to ensure efficient memory management.
Operational Steps:
- Marking: The first step is to identify the objects in memory that are no longer reachable from any active part of the application.
- Reachable Objects: Objects that can be accessed through active threads, static references, or other means.
- Unreachable Objects: Objects that are no longer accessible are marked for removal.
- Sweeping: This phase involves removing the marked objects from memory.
- Deletion: The actual removal of unreachable objects.
- Memory Reclamation: The memory previously occupied by deleted objects is reclaimed.
- Compaction: To prevent memory fragmentation, the Garbage Collector may compact the remaining objects, optimizing memory usage.
- Memory Optimization: This process ensures that used memory is contiguous, improving allocation and access speed.
Detailed Look at Java Object Lifecycle
Understanding the lifecycle of objects in Java is crucial to grasp how the Garbage Collector functions.
Lifecycle Stages:
- Object Creation: When an object is instantiated using new, it occupies a portion of the heap memory.
- Active Use: The object is used in the application, manipulated through references.
- Reference Removal: When all references to the object are removed, or the scope is exited, the object becomes inaccessible.
- Garbage Collection: Inaccessible objects are identified and removed by the Garbage Collector, freeing memory.
Run Code from Your Browser - No Installation Required
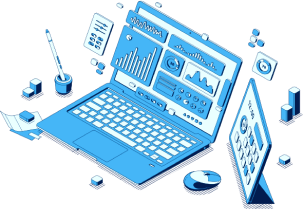
Garbage Collection Algorithms in Java
Java employs several algorithms to handle garbage collection, each tailored for different scenarios and memory conditions.
Common Algorithms:
- Mark-and-Sweep: This fundamental algorithm marks unreachable objects and sweeps them away.
- Generational Garbage Collection: It segregates objects into generations based on their lifespan for efficient collection.
- Parallel and Concurrent Collectors: These collectors utilize multiple threads for garbage collection, reducing pause times and increasing efficiency.
Practical Examples of Garbage Collection
To better understand the Garbage Collector in Java, let's explore some straightforward examples.
Simple Garbage Collection Example:
In this example, when the createGarbage
method finishes execution, the string "I am garbage" becomes unreachable and eligible for garbage collection.
Another Example Illustrating Object Inaccessibility:
Here, reassigning str1
to reference str2
renders the original string "Java" inaccessible, making it eligible for garbage collection.
Garbage Collector and the Spring Framework
In the context of the Spring framework, the Garbage Collector plays a significant role in managing the lifecycle of beans, which are the backbone of Spring's Dependency Injection mechanism.
Role in Spring:
- Bean Management: Spring creates and destroys beans as needed. Once a bean is no longer in use or the application context is closed, these objects become candidates for garbage collection.
- Optimizing Memory Usage: Proper management and timely disposal of beans ensure efficient memory use in Spring applications.
The Importance of Understanding Garbage Collector
Comprehending the Garbage Collector's functionality is vital for Java programmers for various reasons:
- Efficient Memory Management: Knowledge of GC mechanisms leads to writing memory-efficient code.
- Performance Optimization: Understanding how GC works helps in optimizing Java application performance.
- Best Coding Practices: It encourages the adoption of best coding practices that prevent memory leaks and other memory-related issues.
Start Learning Coding today and boost your Career Potential
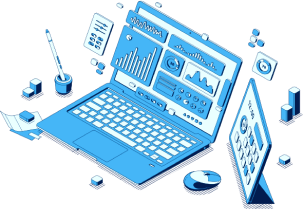
Learning Java and Garbage Collection with Codefinity.com
Mastering Java and its Garbage Collector is a critical step in becoming a proficient Java developer. Codefinity.com offers a wide range of courses and resources that thoroughly cover Java programming, including in-depth modules on memory management and Garbage Collection. Whether you're starting your programming journey or seeking to deepen your Java expertise, Codefinity.com provides an ideal learning platform.
Embark on your Java learning journey with Codefinity.com, and gain the knowledge and skills to excel in Java programming, understanding critical concepts like the Garbage Collector, the Spring framework, and more.
FAQs
Q: Can we force the Garbage Collector to run in Java?
A: While methods like System.gc() suggest running GC, it's generally not advisable as GC decisions are best left to the JVM for optimal performance.
Q: How does Garbage Collection impact Java application performance?
A: Garbage Collection can momentarily pause application execution, especially during major GC events, impacting performance. Understanding and optimizing GC can mitigate these effects.
Q: What are some best practices to optimize Garbage Collection in Java?
A: Best practices include minimizing object creation, reusing objects, and choosing appropriate GC algorithms based on application needs.
Q: How can I monitor the Garbage Collection process in Java?
A: Java provides tools like JVisualVM and GC logs for monitoring and analyzing GC activities.
Q: Is understanding Garbage Collection necessary for all Java developers?
A: Yes, a fundamental understanding of GC is crucial for all Java developers to write efficient and robust applications.
Related courses
See All CoursesAdvanced
Java OOP
Those who know OOP can program well. That's what many programmers say. Get ready for an important part of your Java learning journey, mastering which will greatly boost your programming skills in general. You will learn how to effectively use the Java development environment, the principles of Object-Oriented Programming (OOP), and best practices in OOP. You will learn to make your code flexible and deepen your knowledge of previously covered topics. Let's get started!
Intermediate
Java Extended
You will learn about best practices in coding, how to create your own methods and classes, and how to work with them and configure their interaction. You will also understand how Java works at the computer level and how code compilation generally works.
Beginner
Java Basics
Learn the fundamentals of Java and its key features in this course. By the end, you'll be able to solve simple algorithmic tasks and gain a clear understanding of how basic console Java applications operate.
The SOLID Principles in Software Development
The SOLID Principles Overview
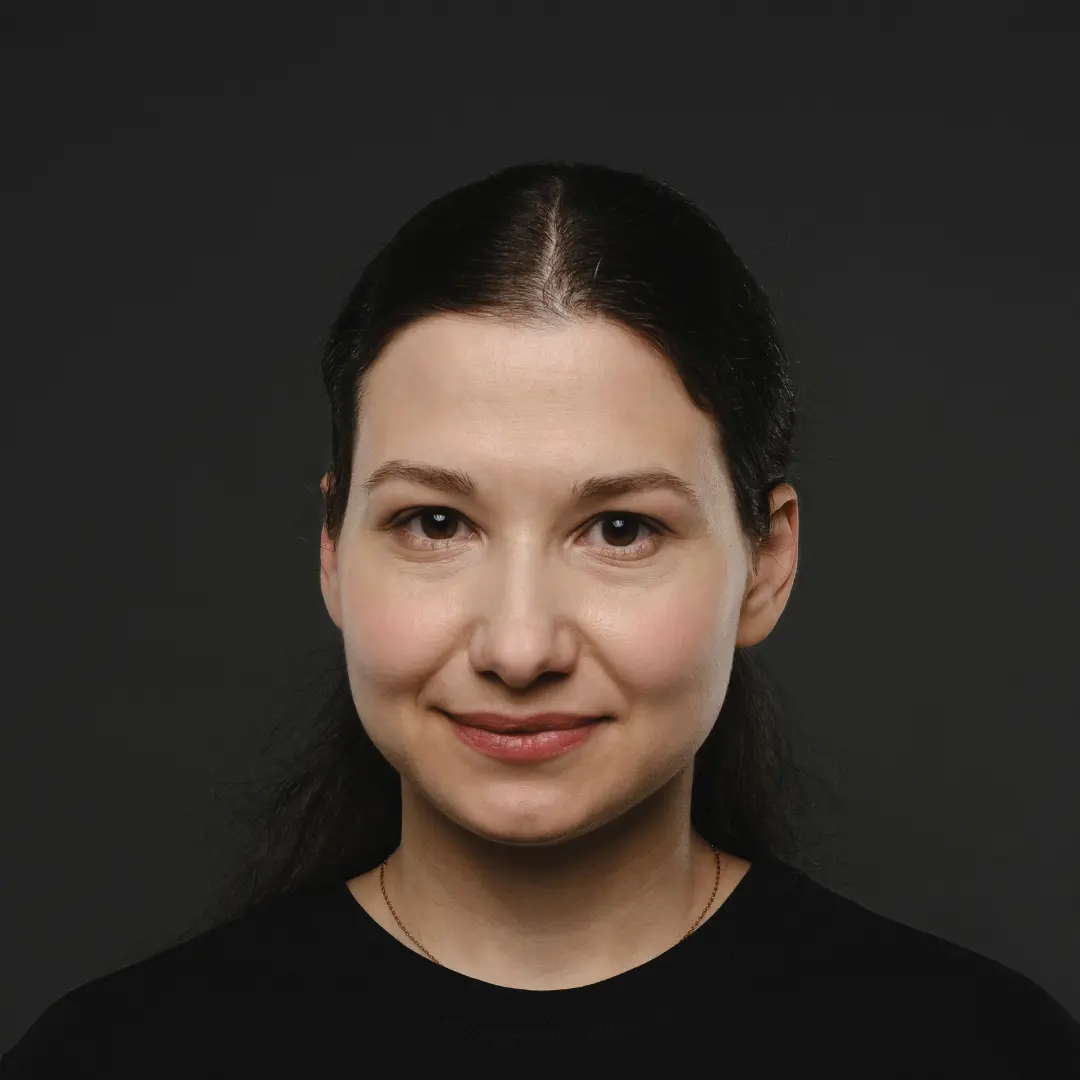
by Anastasiia Tsurkan
Backend Developer
Nov, 2023γ»8 min read
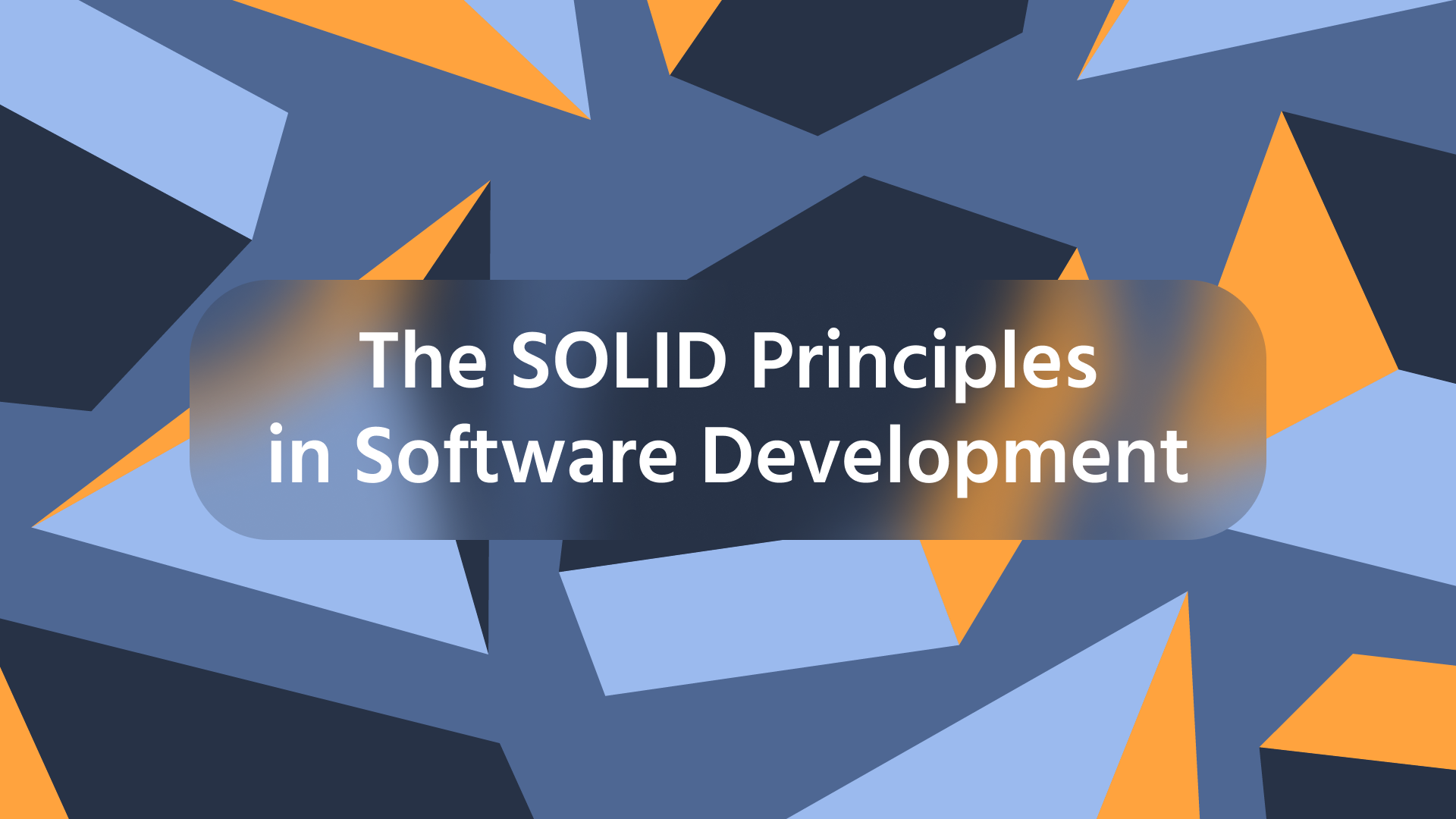
30 Python Project Ideas for Beginners
Python Project Ideas
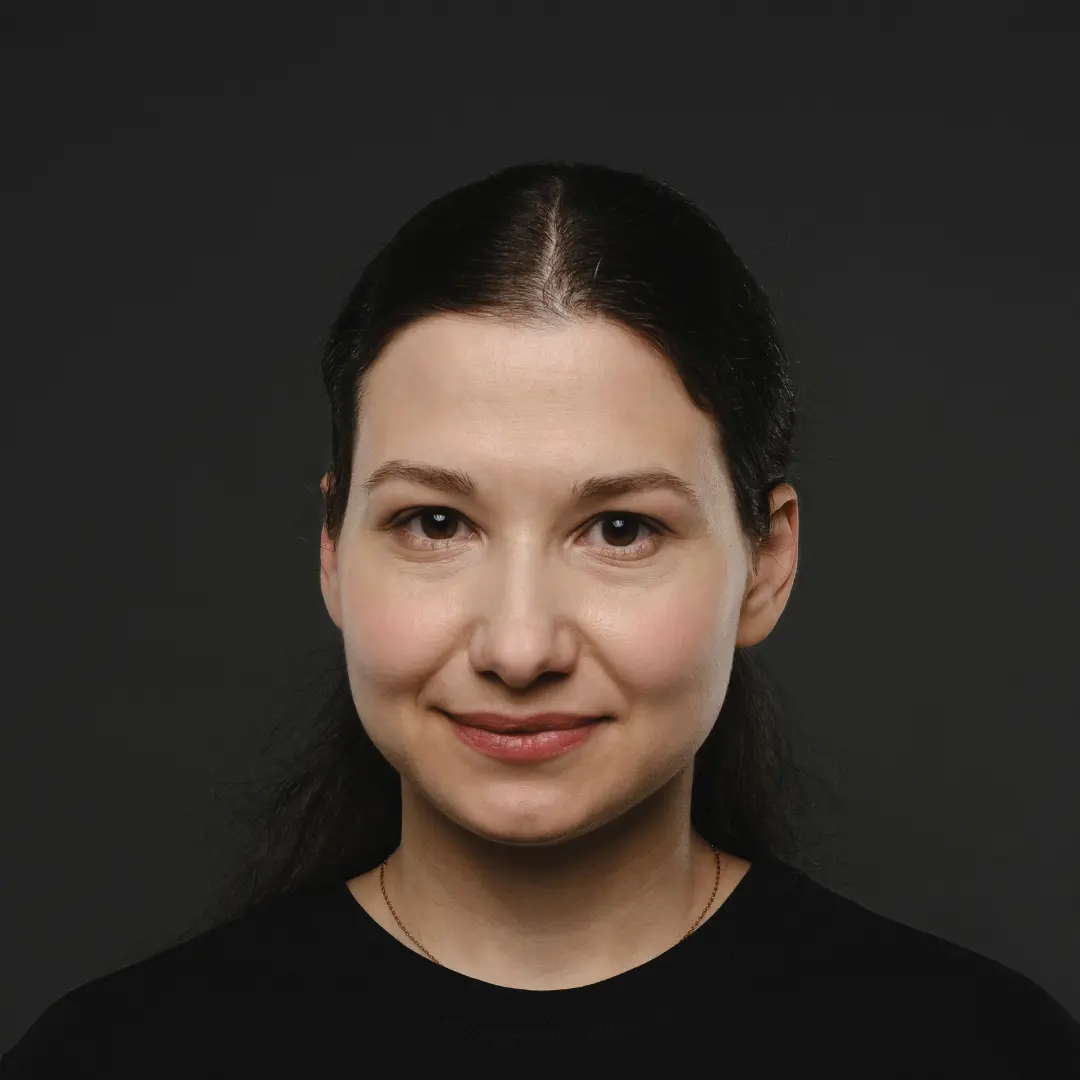
by Anastasiia Tsurkan
Backend Developer
Sep, 2024γ»14 min read
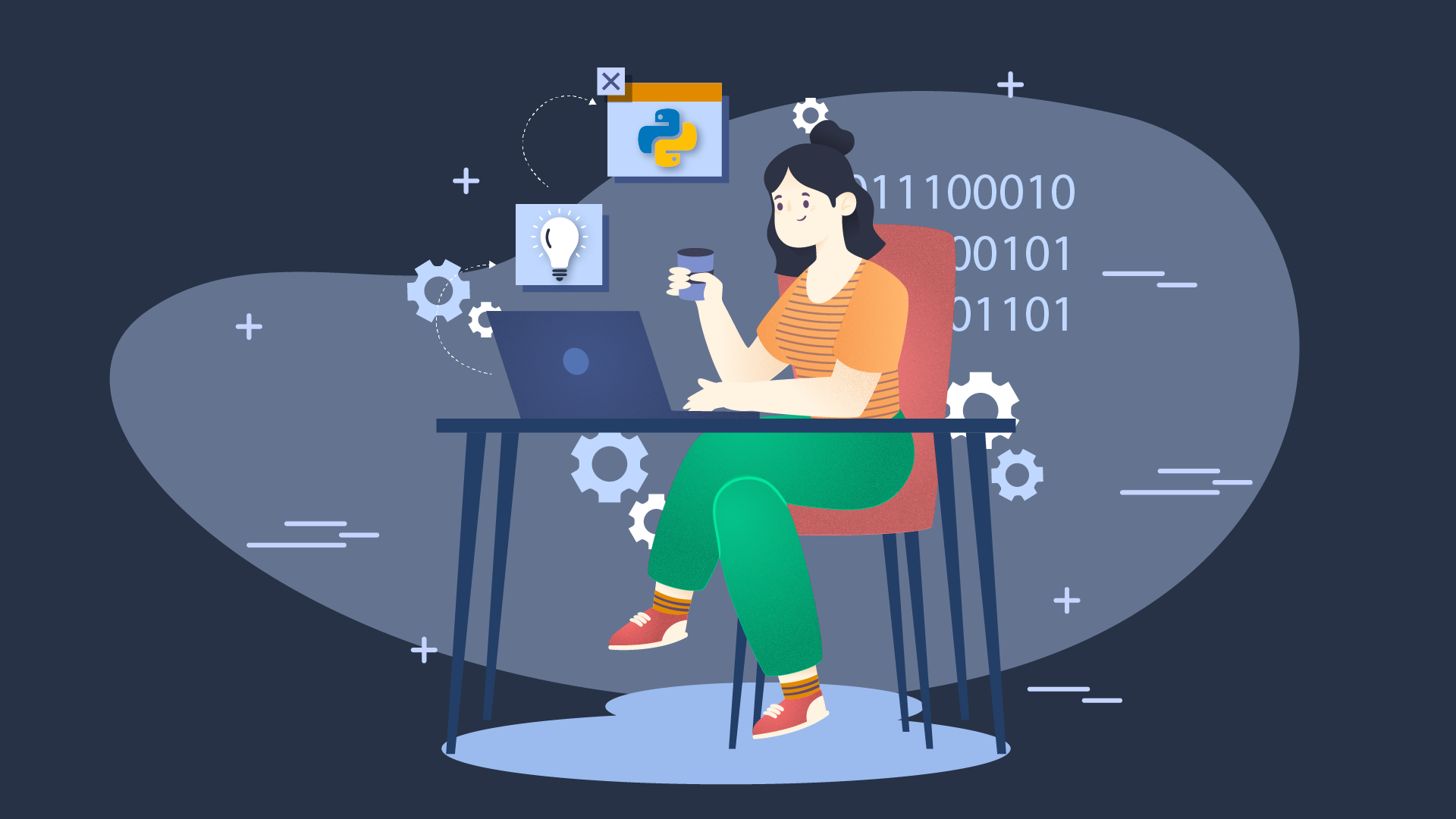
How Information Moves Across the Internet
Understanding Data Movement Through OSI and TCP/IP Models
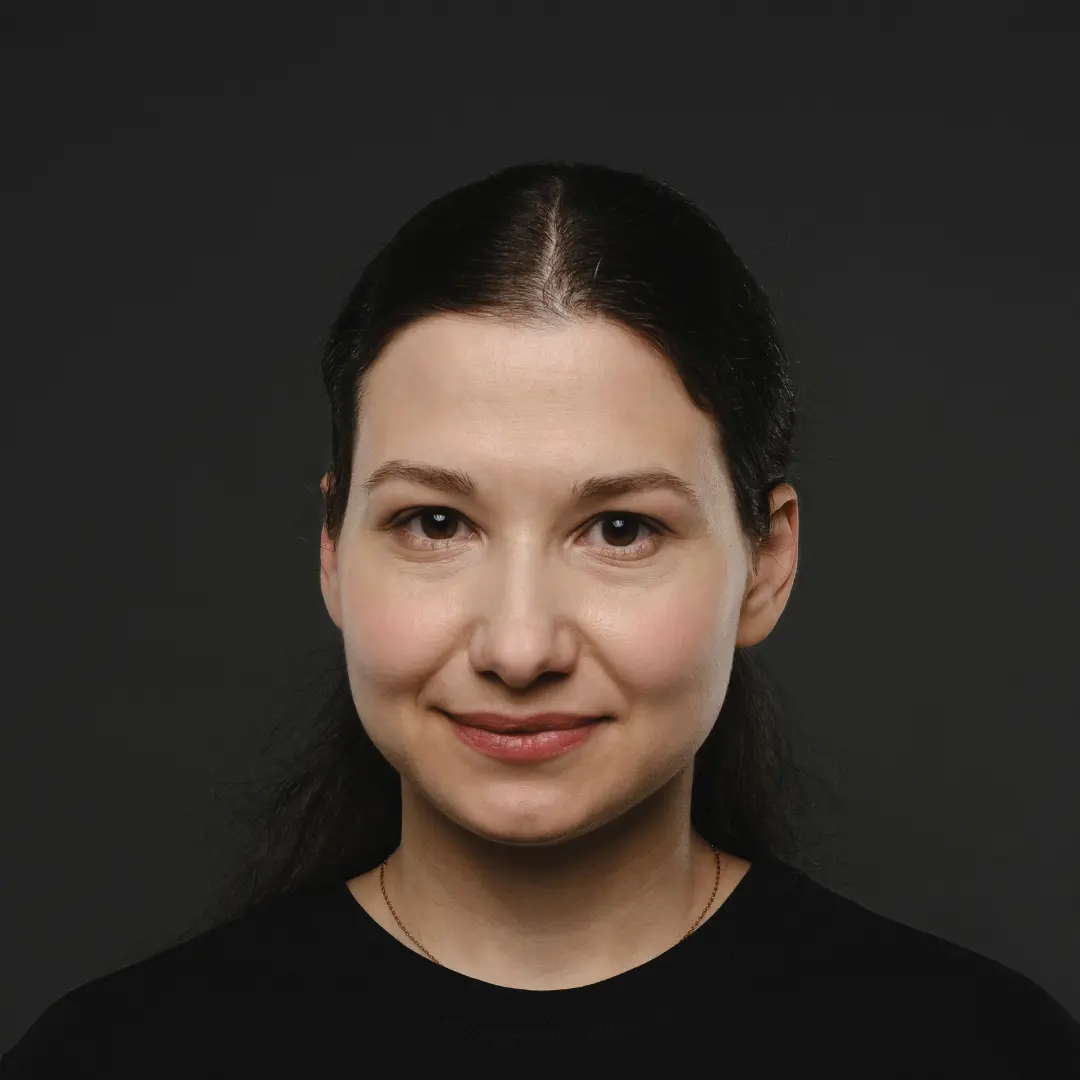
by Anastasiia Tsurkan
Backend Developer
Jan, 2024γ»6 min read
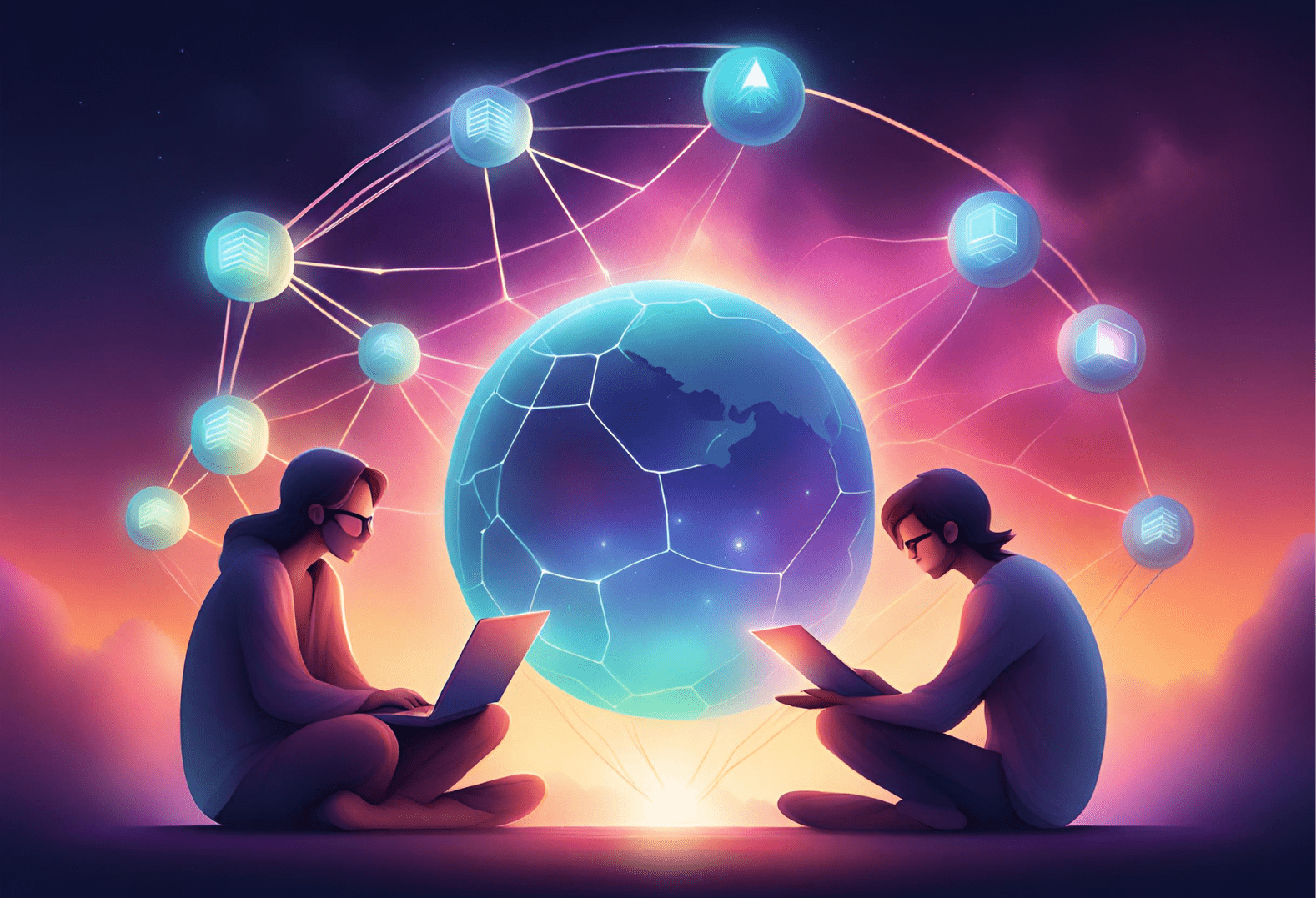
Content of this article