Related courses
See All CoursesAdvanced
Redux Toolkit & React
Discover the power of Redux Toolkit in conjunction with React, and supercharge your state management skills. This course comprehensively introduces Redux Toolkit, a powerful library for managing state. Learn how to streamline your Redux setup, simplify complex state logic, and create efficient, scalable React applications.
Intermediate
React Mastery
Take your web development skills to the next level with the course on building modern web applications using React. Learn to create dynamic user interfaces, efficiently manage state and data, and leverage the latest technologies and best practices in web development. Join now and unlock the full potential of React to create highly interactive and engaging web apps that meet the demands of today's users.
Advanced
React Router
Delve into the world of routing within React applications and learn how React Router streamlines the management of navigation, URL state, and dynamic component rendering, all in response to the current URL.
useState Hook in React with TypeScript
Guide to Using useState in React with TypeScript
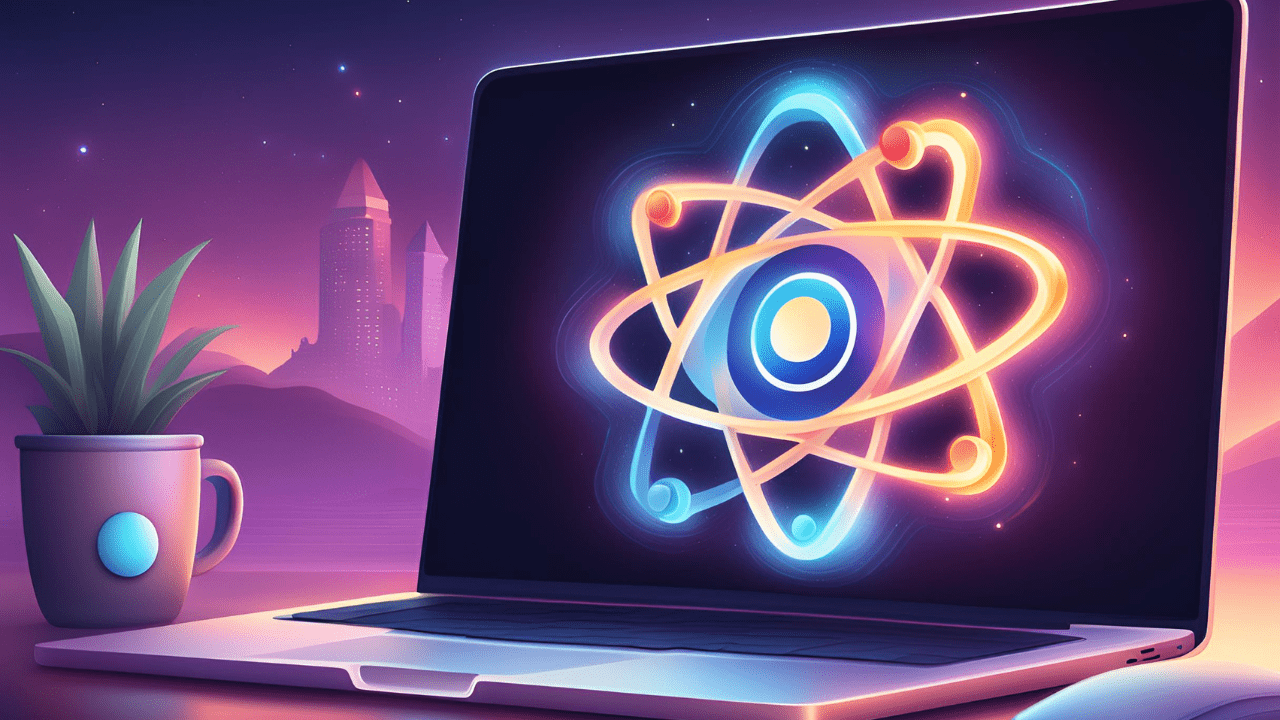
Introduction to useState and Its Uses
The useState
hook is one of the most fundamental hooks provided by React. It allows you to add state to functional components, making them more dynamic and interactive. Before hooks were introduced in React 16.8, state management was only possible in class components. With useState
, you can now manage state in a cleaner, more functional way.
Initializing State Variables with useState
When using TypeScript with useState
, it's important to define the type of the state. This ensures type safety and helps avoid runtime errors.
Syntax
The useState
hook is used as follows:
Here, state
is the current state value, and setState
is a function that updates the state.
Example
In this example, we initialize a state variable count
with the type number
and set its initial value to 0
.
Run Code from Your Browser - No Installation Required
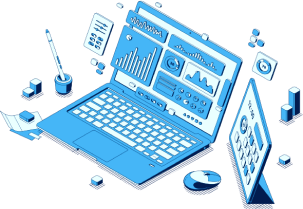
Updating State Variables with useState
Updating state with useState
is done by calling the setState
function returned by the hook. This function can take either a new state value or a function that receives the previous state and returns the new state.
Example
This approach ensures that the state update is based on the most recent state value, which is crucial when dealing with asynchronous updates.
Handling State Updates with useState
State updates in React are asynchronous and can be batched for performance improvements. This means that accessing the state immediately after calling setState
might not give you the updated value. Therefore, using a function to update the state, as shown above, is a recommended practice.
Example
In this example, we toggle the isOn
state between true
and false
using a function inside setState
.
Best Practices for Using useState with TypeScript
Type Inference
TypeScript can often infer the type of the state from the initial value. However, explicitly defining the type is a good practice for complex types or when the initial state is null
or undefined
.
Complex State Objects
For complex state objects, use TypeScript interfaces to define the shape of the state.
State Updaters
Use functional state updaters when the new state depends on the previous state to avoid potential bugs.
Avoid Overusing State
Not every piece of data needs to be in the state. Only use state for data that changes over time and affects what you render.
Start Learning Coding today and boost your Career Potential
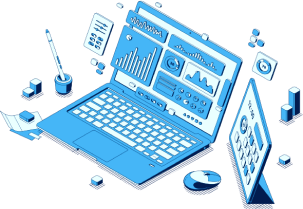
Examples of Common Use Cases for useState in React Applications
Form Handling
Managing form input state is a common use case for useState
.
Toggling UI Elements
Managing the visibility of UI elements, like a modal, is another common scenario.
Fetching Data
Using state to manage data fetched from an API.
Conclusion
The useState
hook is a powerful tool for managing state in functional components. When combined with TypeScript, it ensures type safety and enhances the developer experience by catching errors early during development. By following best practices and understanding common use cases, you can effectively use useState
to create dynamic and responsive React applications.
FAQs
Q: What is the useState
hook in React?
A: The useState
hook is a function that allows you to add state to functional components in React. It returns a state variable and a function to update that state.
Q: How do you initialize a state variable with useState
in TypeScript?
A: You initialize a state variable with useState
by calling it with an initial value. In TypeScript, you can also provide a type for the state variable:
Q: How do you update a state variable using useState
?
A: You update a state variable using the function returned by useState
. This function can take a new state value or a function that returns the new state based on the previous state:
Q: What are some best practices for using useState
with TypeScript?
A: 1. Use type inference or explicitly define state types. 2. Use functional state updaters when the new state depends on the previous state. 3. Keep state management simple and only use state for data that changes over time and affects rendering.
Q: Can you manage complex state objects with useState
in TypeScript?
A: Yes, you can manage complex state objects by using TypeScript interfaces to define the shape of the state:
Related courses
See All CoursesAdvanced
Redux Toolkit & React
Discover the power of Redux Toolkit in conjunction with React, and supercharge your state management skills. This course comprehensively introduces Redux Toolkit, a powerful library for managing state. Learn how to streamline your Redux setup, simplify complex state logic, and create efficient, scalable React applications.
Intermediate
React Mastery
Take your web development skills to the next level with the course on building modern web applications using React. Learn to create dynamic user interfaces, efficiently manage state and data, and leverage the latest technologies and best practices in web development. Join now and unlock the full potential of React to create highly interactive and engaging web apps that meet the demands of today's users.
Advanced
React Router
Delve into the world of routing within React applications and learn how React Router streamlines the management of navigation, URL state, and dynamic component rendering, all in response to the current URL.
Accidental Innovation in Web Development
Product Development
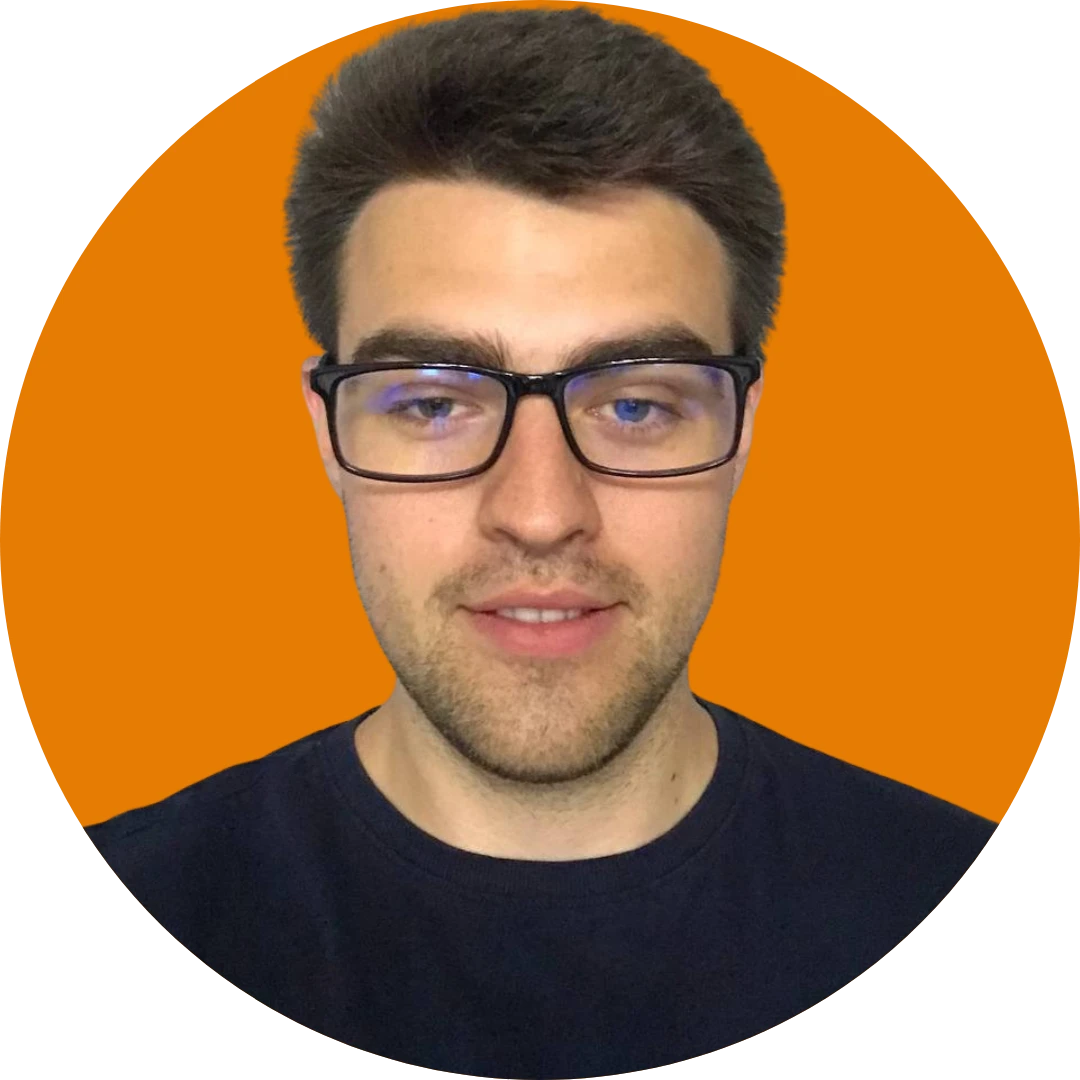
by Oleh Subotin
Full Stack Developer
May, 2024・5 min read
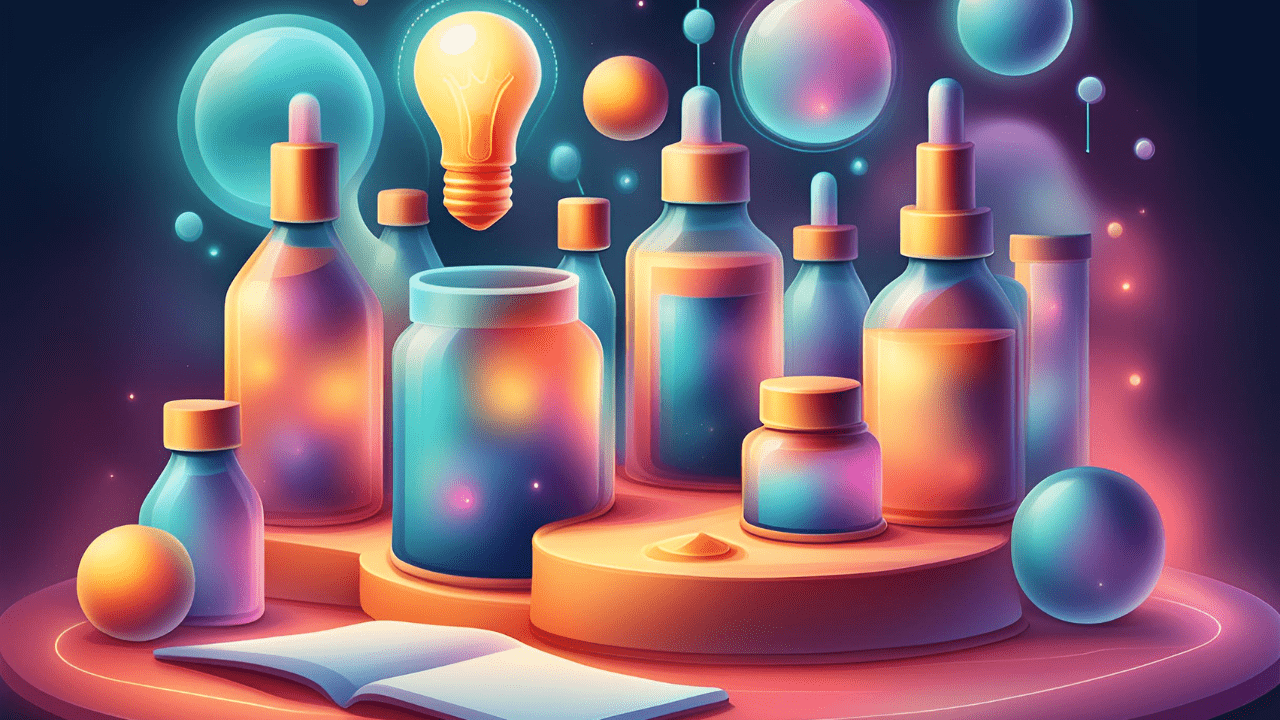
React useActionState Hook
The End of useState Hell in React Forms - How React 19 Revolutionizes Form Management
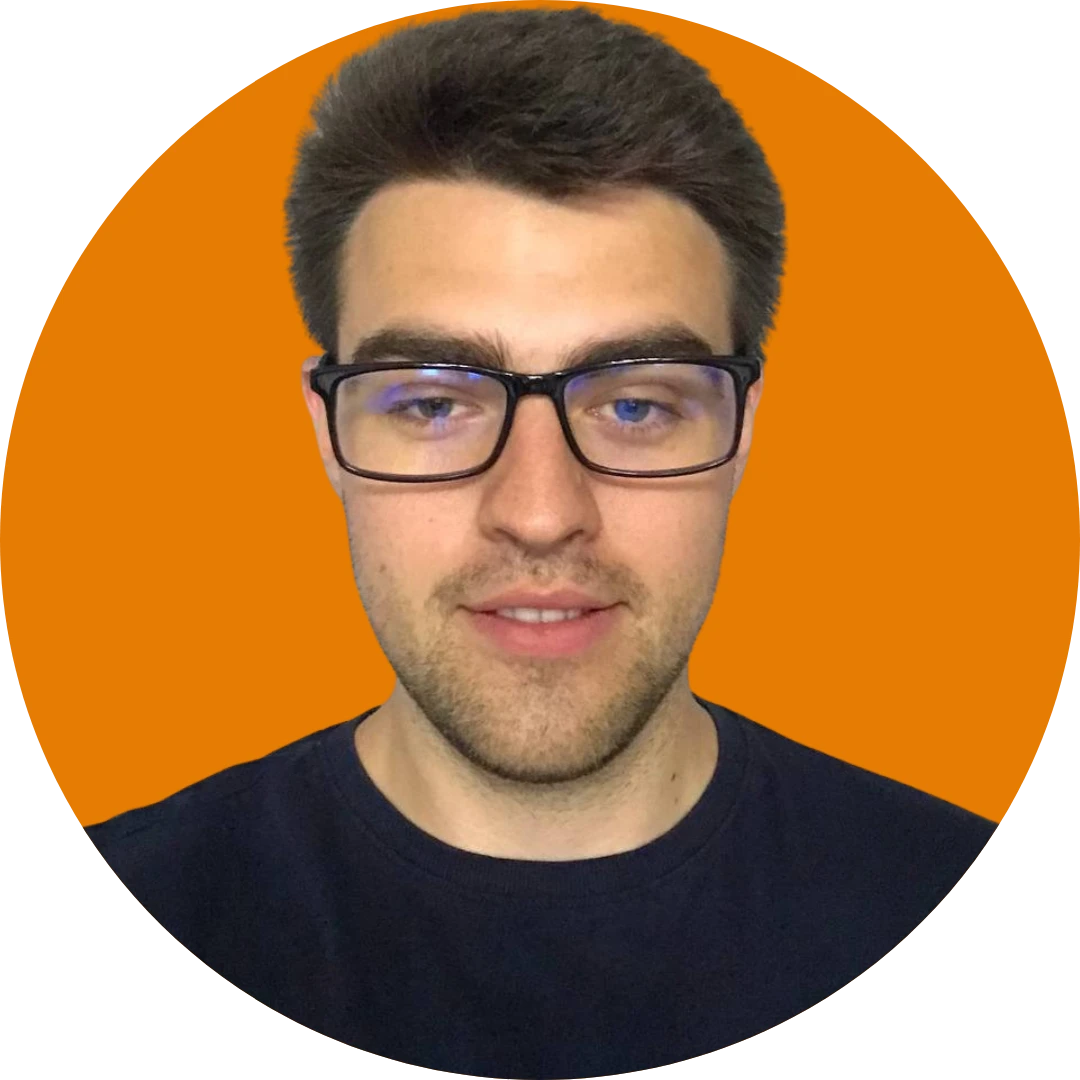
by Oleh Subotin
Full Stack Developer
Jan, 2025・9 min read
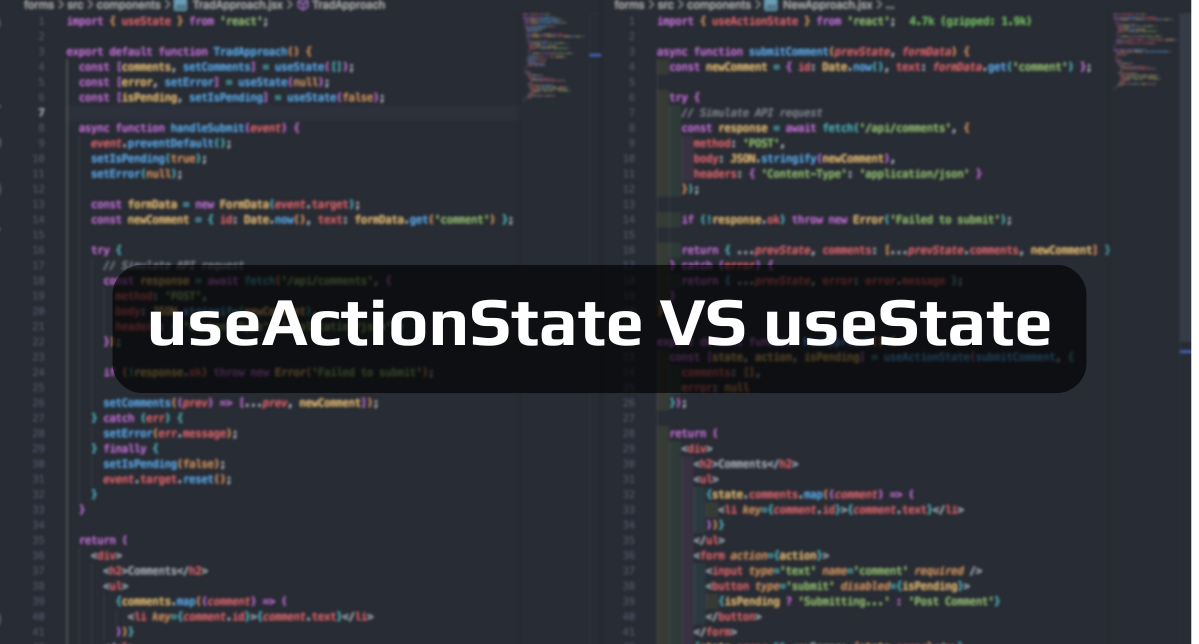
Navigating the YouTube Influencer Maze in Your Tech Career
Staying Focused and Disciplined Amidst Overwhelming Advice
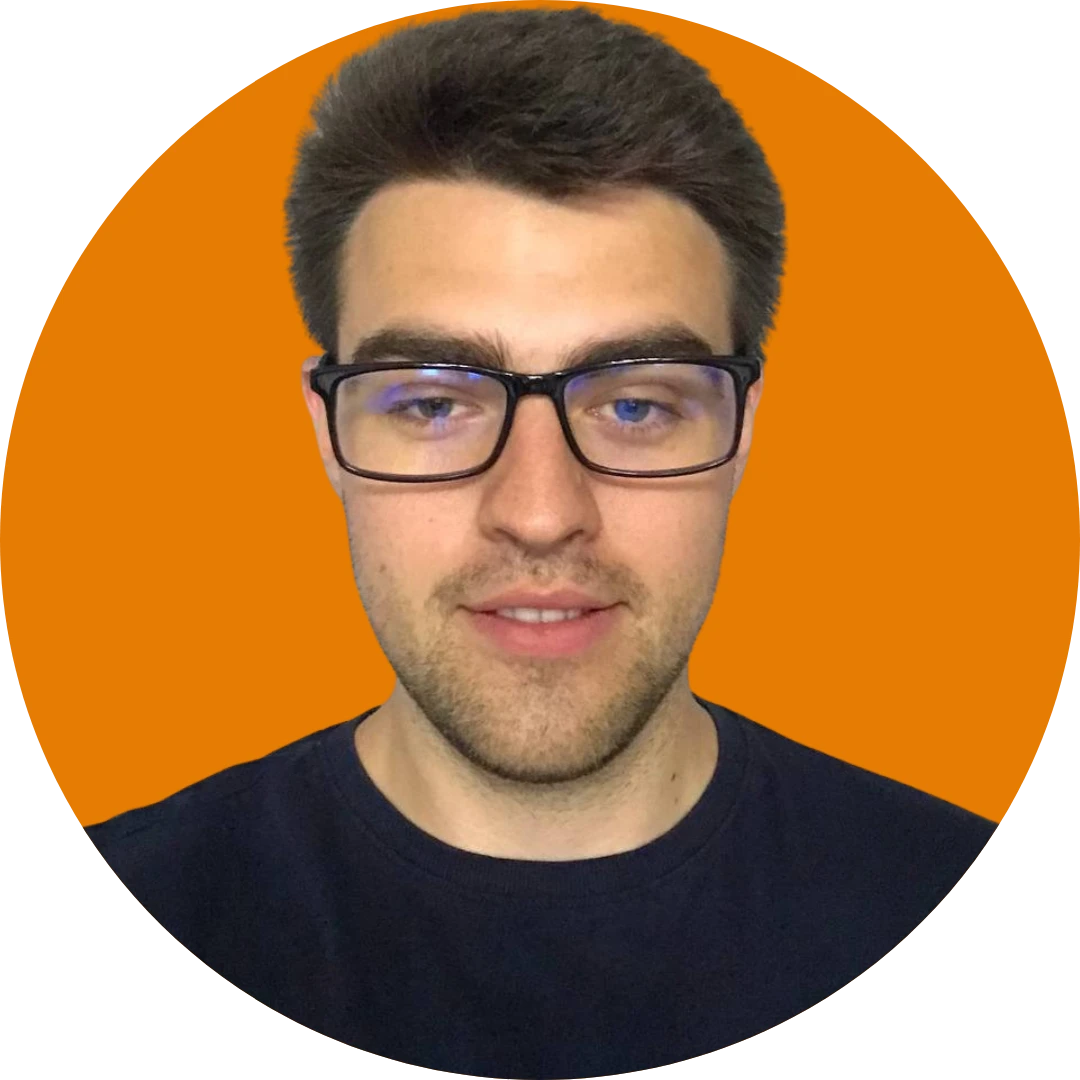
by Oleh Subotin
Full Stack Developer
Jul, 2024・5 min read
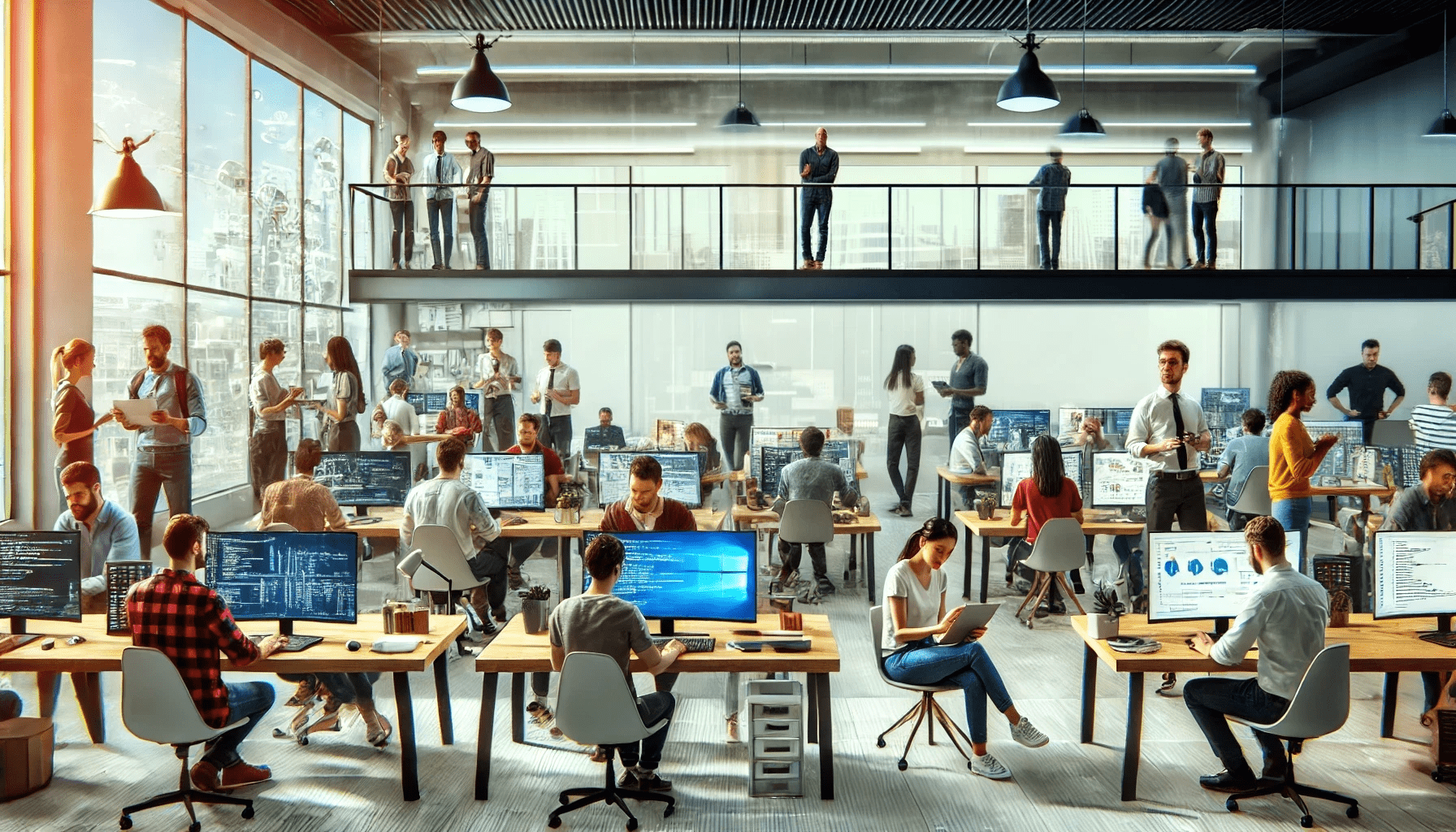
Content of this article