Cursos relacionados
Ver Todos los CursosPrincipiante
Introduction to JavaScript
Learn the fundamentals of JavaScript, the backbone of dynamic web development. Discover essential concepts like syntax, variables, data types, and operators. Explore how to use conditional statements, loops, and functions to create interactive and efficient programs. Master the building blocks of JavaScript and lay the groundwork for more advanced programming skills.
Avanzado
Backend Development with Node.js and Express.js
Boost your programming prowess with Node.js Mastery and take your skills to the next level. Dive into Node.js, Console Apps, Express.js, and API development to construct lightning-fast, scalable web apps utilizing top-notch industry standards.
Authentication in a Nodejs Application
A Comprehensive Guide to Implementing Authentication in a Nodejs Application
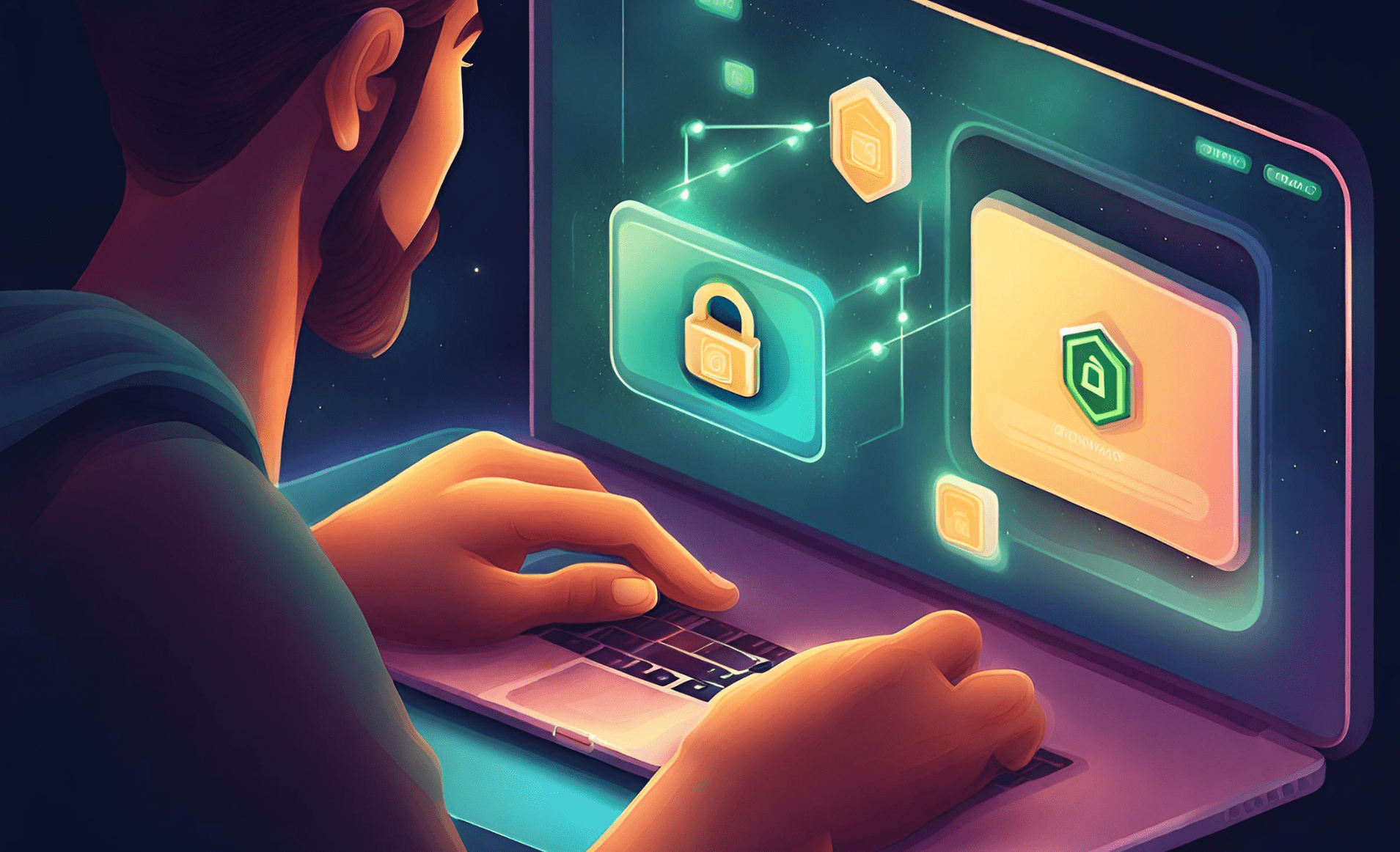
Introduction
Node.js has gained immense popularity for building scalable and efficient server-side applications. When developing applications that involve user interactions, implementing authentication becomes a crucial aspect to ensure security and user privacy. In this article, we will go through the process of adding user authentication to a Node.js application using popular frameworks or libraries.
Understanding Authentication
Authentication is the process of verifying the identity of a user, ensuring that they are who they claim to be. In the context of web applications, this typically involves the use of usernames and passwords. There are several authentication strategies, including session-based, token-based, and OAuth, each serving specific use cases.
Run Code from Your Browser - No Installation Required
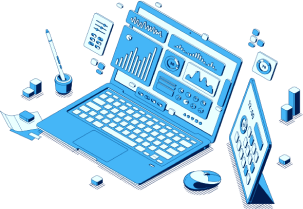
Popular Authentication Libraries/Frameworks in Node.js
Passport.js:
- Custom Strategies: In addition to the built-in strategies, Passport.js allows developers to implement custom authentication strategies. This flexibility enables integration with various identity providers and authentication mechanisms tailored to specific application requirements.
- Middleware Architecture: Passport.js operates as middleware in the Express.js application stack, making it easy to integrate into existing projects. This middleware-based approach allows developers to apply authentication to specific routes or groups of routes.
- Session Support: Passport.js provides session support, enabling the creation of persistent login sessions. This is beneficial for web applications that require users to stay logged in across multiple requests.
- Community and Ecosystem: Passport.js has a vibrant community and extensive ecosystem. There are numerous third-party strategies available for various authentication providers, making it easier to integrate with platforms like Google, Facebook, Twitter, etc.
jsonwebtoken (JWT):
- Stateless Authentication: One of the significant advantages of using JWT is its stateless nature. Since JWTs carry all necessary information within the token itself, there's no need for server-side storage of session data. This makes scaling and distributing applications more straightforward.
- Payload Customization: JWTs consist of a header, payload, and signature. Developers can customize the payload with user-specific information. This payload becomes part of the token, and the server can extract this information without querying a database, enhancing performance.
- Token Expiration and Refresh: The jsonwebtoken library allows developers to set token expiration times. Additionally, it supports the implementation of token refresh mechanisms, improving security by periodically updating tokens and reducing the risk associated with long-lived tokens.
- Middleware Integration: Developers can easily integrate JWT authentication into their Express.js middleware stack. This allows for the creation of protected routes that require a valid JWT to access.
It's worth mentioning that both Passport.js and jsonwebtoken can be used together. Developers often employ Passport.js for handling the authentication process and then use jsonwebtoken to generate and manage JWTs for subsequent secure communication.
Implementation Steps
1. Install Necessary Packages
- Use npm to install the required packages. In your terminal, run:
bash
- Brief explanation of each package:
- express: A web application framework for Node.js.
- passport: Authentication middleware for Node.js.
- passport-local: Passport strategy for authenticating with a username and password.
- express-session: Session middleware for Express.js.
2. Configure Passport.js
- Set up Passport.js in your application. This involves configuring Passport with the desired authentication strategy. For local authentication:
javascript
- Adjust the serialization and deserialization functions based on your user model and data storage.
3. Integrate Authentication Routes
- Create routes for user authentication, such as login and registration routes, using Express.js.
- Implement validation and authentication logic within these routes. For example:
js
4. Store User Data
- Decide on a method for storing user data. Passport.js supports various data store options, including in-memory, databases like MongoDB or MySQL, or custom stores.
- Connect Passport.js to your chosen data store. For example, if using MongoDB:
js
5. Generate and Verify JWT (Optional)
- If using token-based authentication, integrate JWT for generating and verifying tokens.
- Use the jsonwebtoken library. Example:
js
- Adjust the token payload and expiration based on your application requirements.
6. Secure Routes
- Implement middleware to secure routes that require authentication. Passport.js provides middleware functions for this purpose. For example:
js
- Use the
isAuthenticated
middleware to protect routes that should only be accessible to authenticated users.
Conclusion
Implementing authentication in a Node.js application is a critical step to enhance security and protect user data. By choosing the right authentication strategy and leveraging popular libraries like Passport.js or JSON Web Tokens, developers can ensure a robust and secure authentication process for their applications. Always stay updated on best practices and security considerations to provide a safe user experience.
Start Learning Coding today and boost your Career Potential
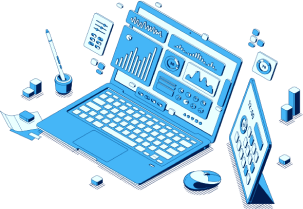
FAQs
Q: What is the significance of authentication in Node.js applications?
A: Authentication is crucial for verifying the identity of users, ensuring security, and protecting user privacy in server-side applications developed using Node.js.
Q: What are the common authentication strategies used in web applications?
A: Common authentication strategies include session-based, token-based, and OAuth, each serving specific use cases in the context of web applications.
Q: Can you recommend popular authentication libraries/frameworks for Node.js?
A: Yes, two popular options are Passport.js and jsonwebtoken (JWT). Passport.js offers flexibility with custom strategies and middleware architecture, while jsonwebtoken provides stateless authentication with token customization and expiration features.
Q: How does Passport.js enhance authentication in Node.js applications?
A: Passport.js operates as middleware in the Express.js stack, allowing easy integration, supports custom strategies, and provides session support for persistent login sessions.
Q: What advantages does jsonwebtoken (JWT) offer for authentication in Node.js?
A: JWT offers stateless authentication, payload customization, token expiration, and refresh features. It simplifies scaling and distribution by carrying necessary information within the token itself.
Q: Can Passport.js and jsonwebtoken be used together in a Node.js application?
A: Yes, developers often combine Passport.js for authentication and use jsonwebtoken to generate and manage JWTs for subsequent secure communication.
Cursos relacionados
Ver Todos los CursosPrincipiante
Introduction to JavaScript
Learn the fundamentals of JavaScript, the backbone of dynamic web development. Discover essential concepts like syntax, variables, data types, and operators. Explore how to use conditional statements, loops, and functions to create interactive and efficient programs. Master the building blocks of JavaScript and lay the groundwork for more advanced programming skills.
Avanzado
Backend Development with Node.js and Express.js
Boost your programming prowess with Node.js Mastery and take your skills to the next level. Dive into Node.js, Console Apps, Express.js, and API development to construct lightning-fast, scalable web apps utilizing top-notch industry standards.
The 80 Top Java Interview Questions and Answers
Key Points to Consider When Preparing for an Interview
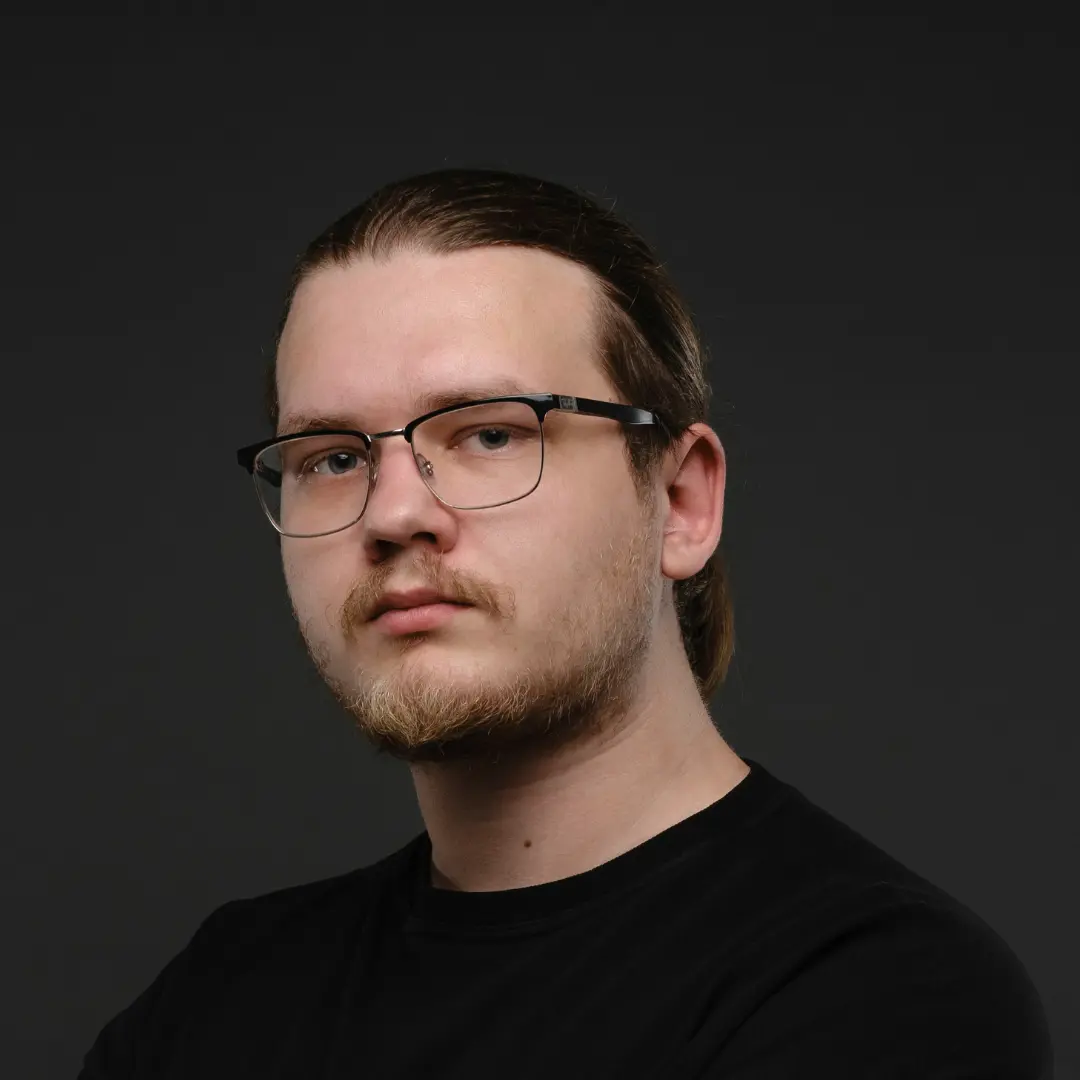
by Daniil Lypenets
Full Stack Developer
Apr, 2024・30 min read
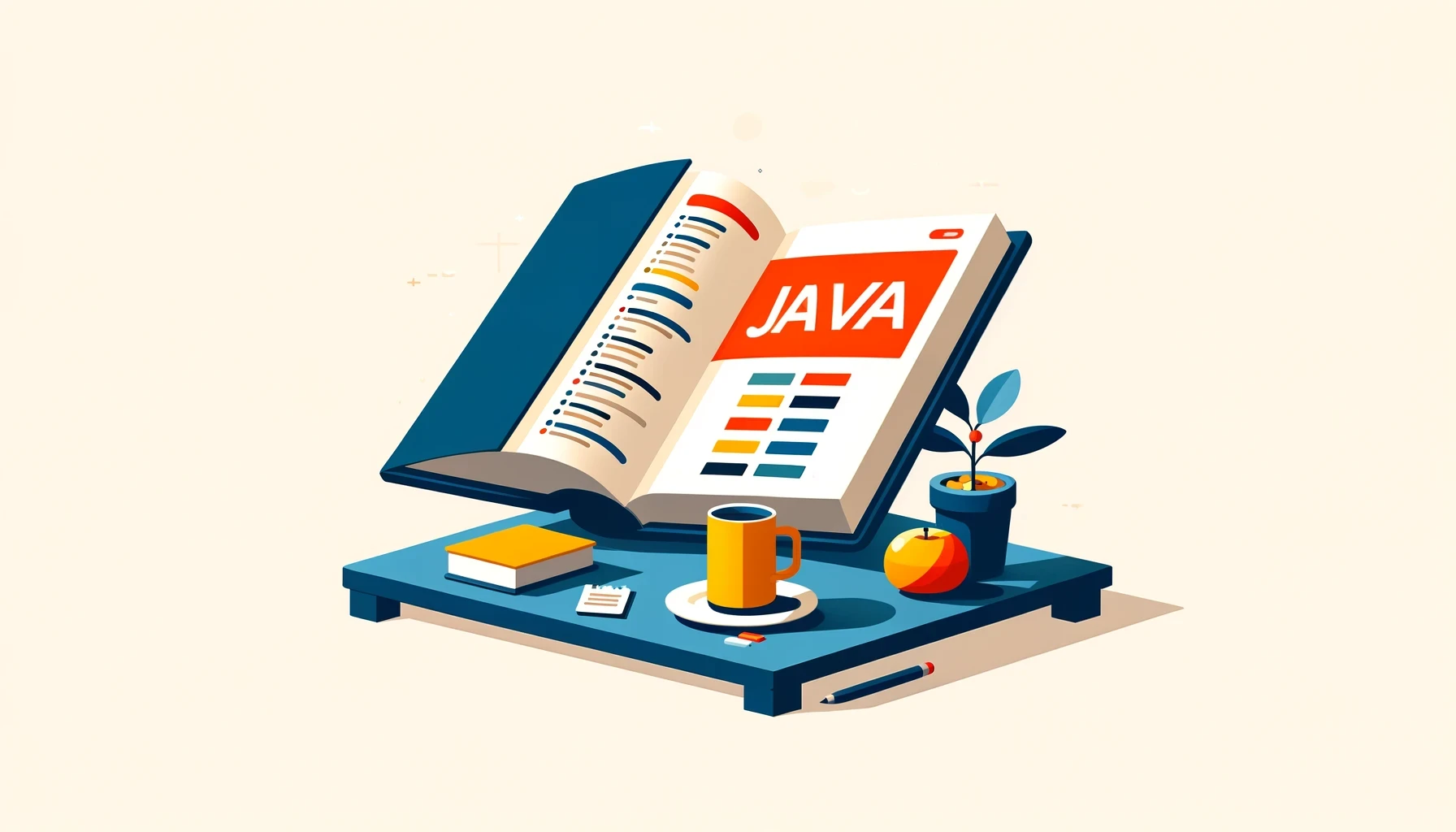
The 50 Top SQL Interview Questions and Answers
For Junior and Middle Developers
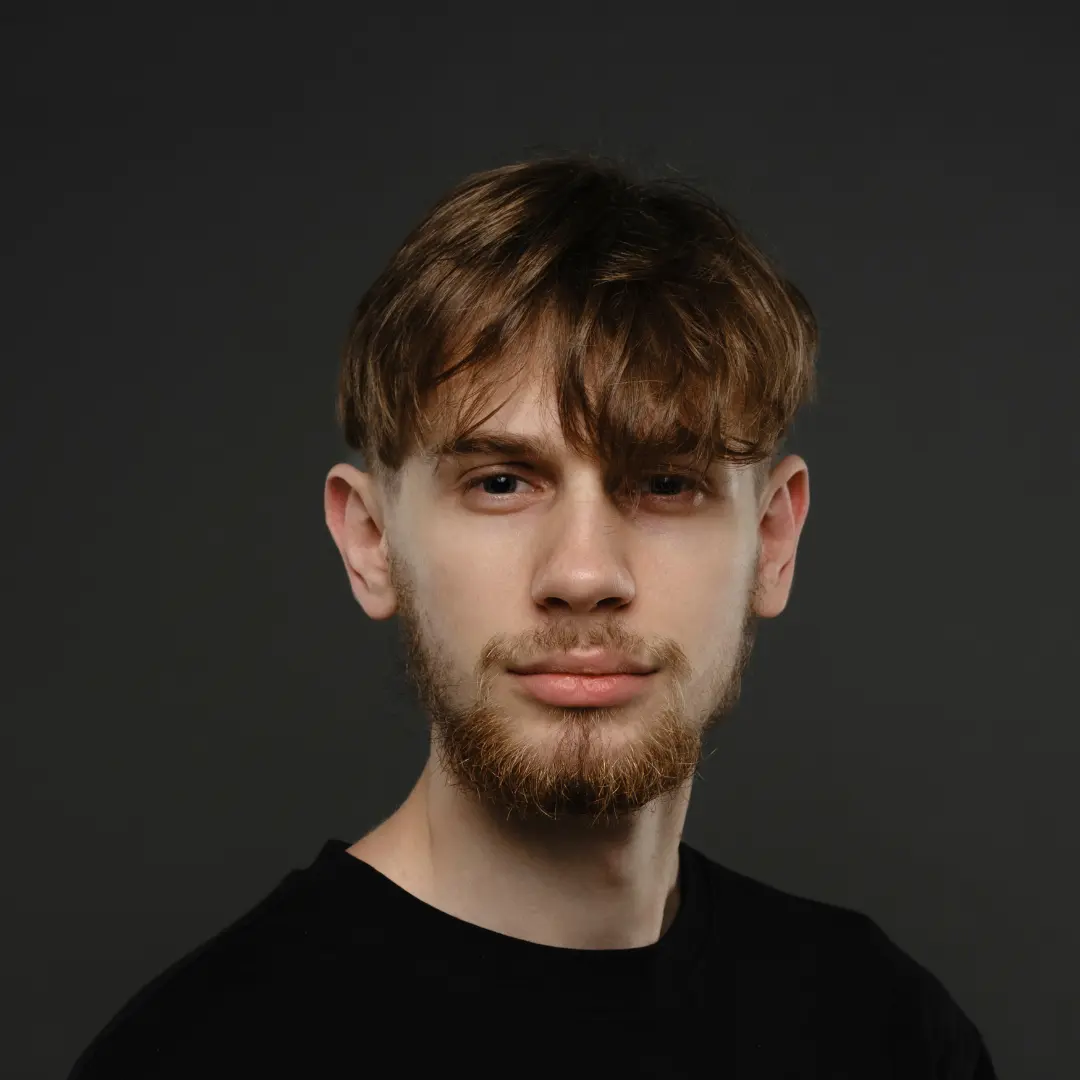
by Oleh Lohvyn
Backend Developer
Apr, 2024・31 min read
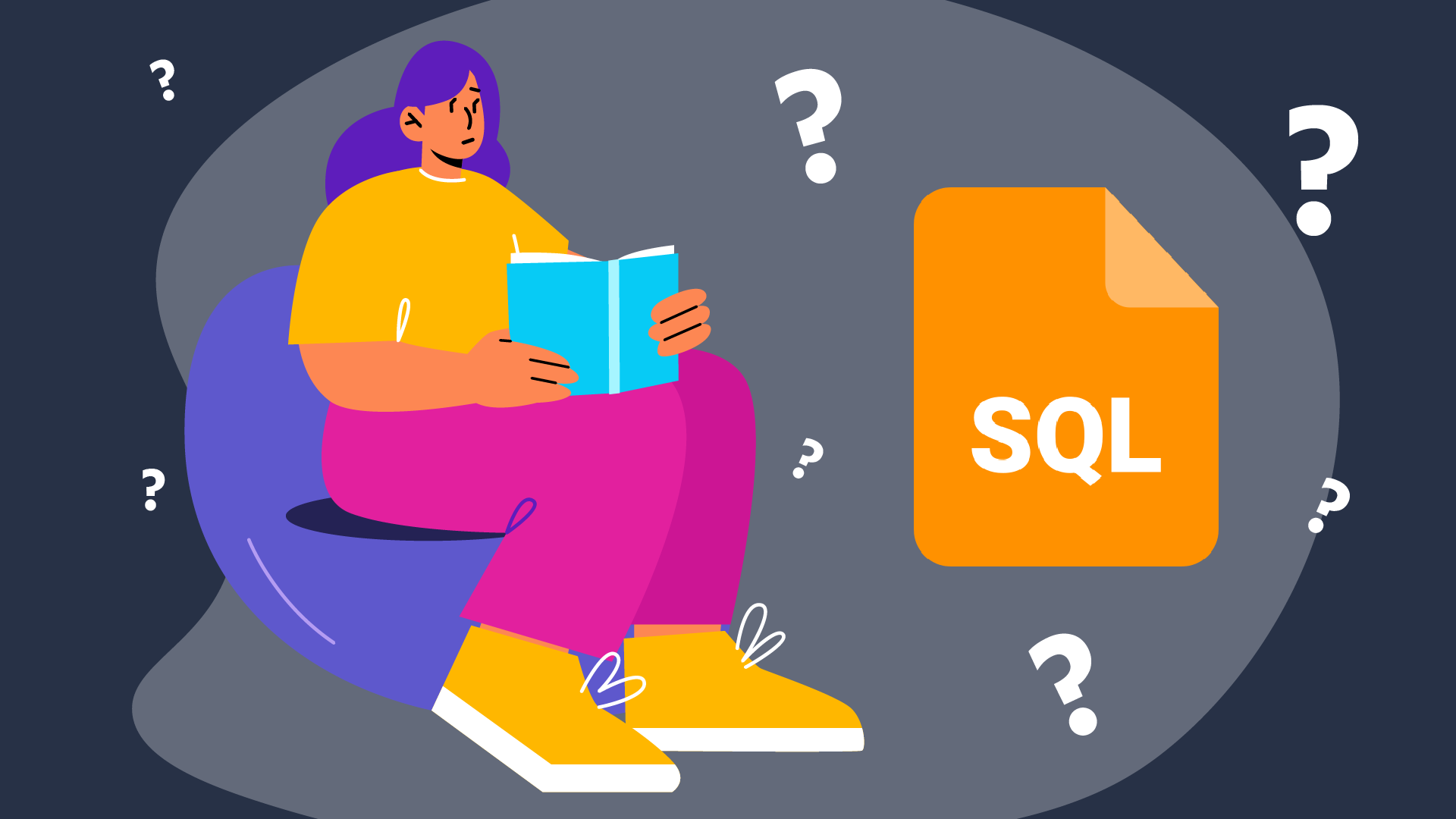
The SOLID Principles in Software Development
The SOLID Principles Overview
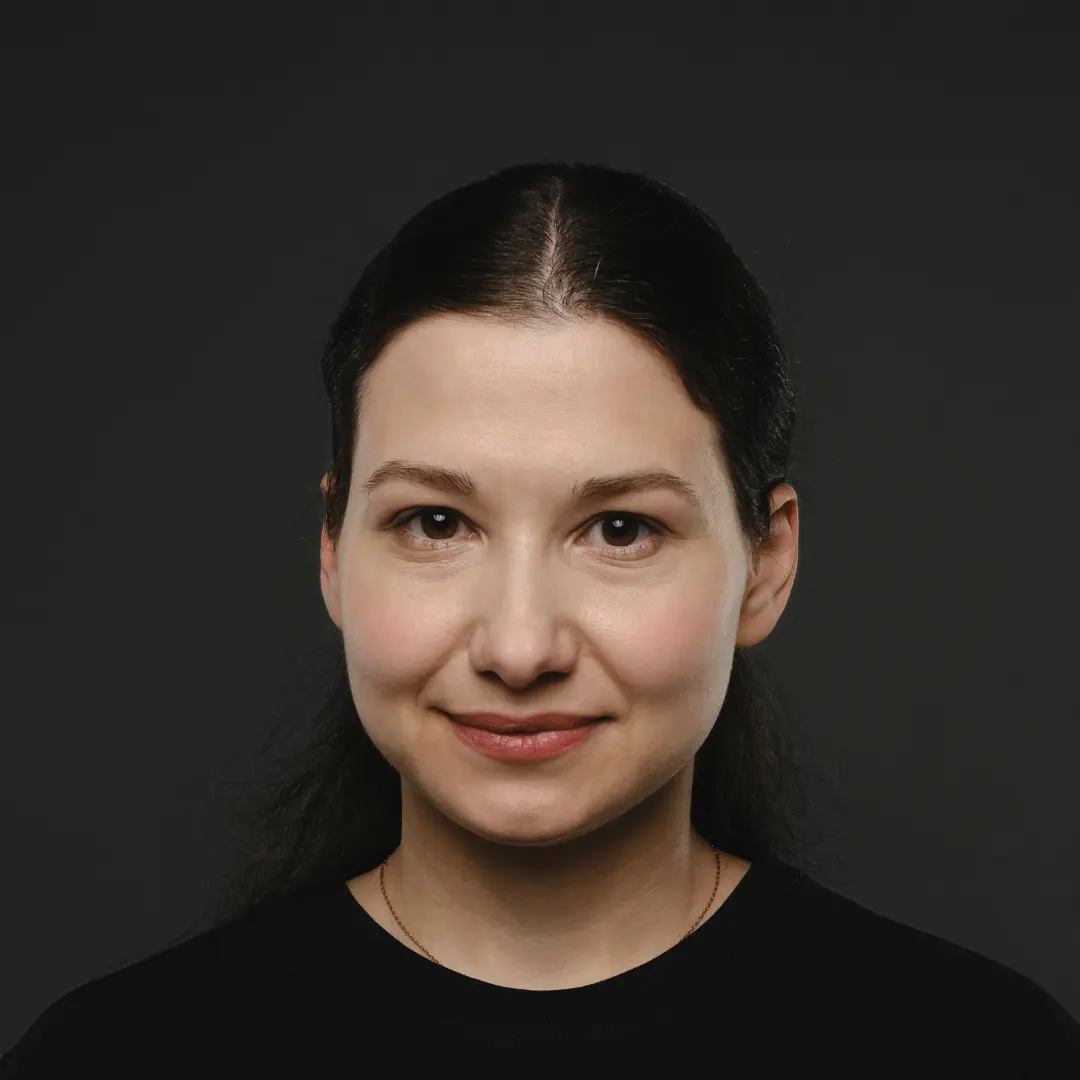
by Anastasiia Tsurkan
Backend Developer
Nov, 2023・8 min read
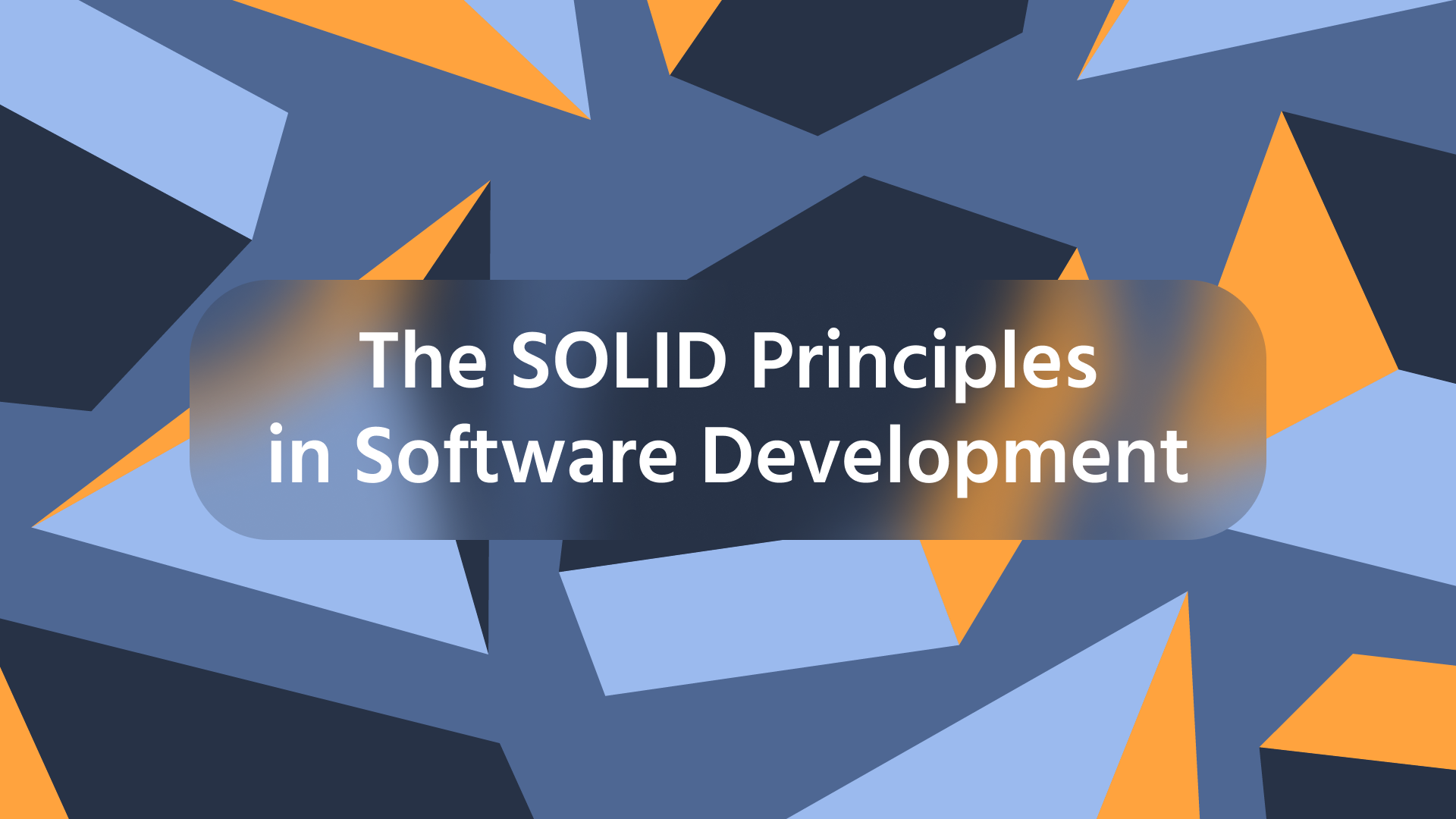
Contenido de este artículo