Cursos relacionados
Ver Todos los CursosPrincipiante
Introduction to JavaScript
Learn the fundamentals of JavaScript, the backbone of dynamic web development. Discover essential concepts like syntax, variables, data types, and operators. Explore how to use conditional statements, loops, and functions to create interactive and efficient programs. Master the building blocks of JavaScript and lay the groundwork for more advanced programming skills.
Intermedio
JavaScript Data Structures
Learn to work confidently with data in JavaScript by mastering objects and arrays. Understand how to create, access, and manage object properties and methods effectively. Dive into advanced object manipulation techniques, including iteration, cloning, and destructuring for cleaner code. Build a strong foundation in working with arrays and learn to manage, iterate, and modify array elements efficiently. Master advanced array methods like map, filter, find, and sort to transform and handle data effectively in your applications.
DOM Events in JavaScript
Understanding DOM Events and JavaScript Event Listeners
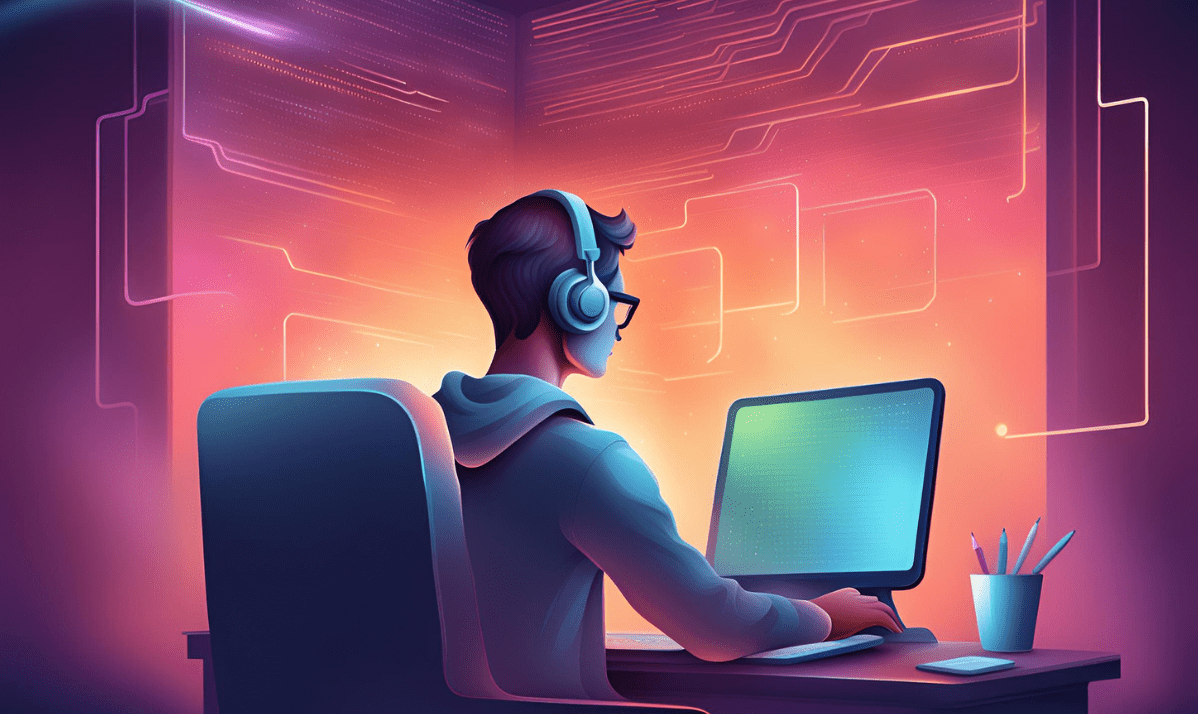
What are JavaScript Events?
JavaScript events are actions or occurrences in a web environment that can be detected and managed by scripts. They form the backbone of interactive features in web applications, such as clicks, key presses, mouse movements, and changes in the browser state.
Essential JavaScript Events and Examples
Click Event
Triggering actions with button clicks.
const button = document.getElementById("myButton");
button.addEventListener("click", function () {
alert("Button was clicked!");
});
Explanation:
getElementById("myButton")
: Selects the HTML element with the IDmyButton
;addEventListener("click", function() {...})
: Attaches a click event listener to the button. When the button is clicked, the anonymous function is executed, displaying an alert saying "Button was clicked!".
### Mouse Events Interactions for hover effects. ```javascript const hoverDiv = document.getElementById("hoverDiv"); hoverDiv.addEventListener("mouseover", function () { this.style.backgroundColor = "lightblue"; }); hoverDiv.addEventListener("mouseout", function () { this.style.backgroundColor = ""; }); ``` **Explanation:** - `getElementById("hoverDiv")`: Selects the HTML element with the ID `hoverDiv`; - `addEventListener("mouseover", function() {...})`: Attaches a mouseover event listener. When the mouse pointer enters the element, the background color changes to light blue; - `addEventListener("mouseout", function() {...})`: Attaches a mouseout event listener. When the mouse pointer leaves the element, the background color is reset.
### Keyboard Events Executing a function when a specific key is pressed. ```javascript document.addEventListener("keypress", function (event) { if (event.key === "Enter") { alert("Enter key was pressed"); } }); ``` **Explanation:** - `addEventListener("keypress", function(event) {...})`: Attaches a keypress event listener to the entire document. When any key is pressed, the function checks if the key pressed is "Enter"; - `if (event.key === "Enter")`: Checks if the key pressed is the "Enter" key; - `alert("Enter key was pressed")`: Displays an alert saying "Enter key was pressed" if the condition is met.
### Form Events Handling form submissions to validate or process data before sending it to a server. ```javascript const form = document.getElementById("myForm"); form.addEventListener("submit", function (event) { event.preventDefault(); alert("Form submitted!"); }); ``` **Explanation:** - `getElementById("myForm")`: Selects the HTML form element with the ID `myForm`; - `addEventListener("submit", function(event) {...})`: Attaches a submit event listener to the form; - `event.preventDefault()`: Prevents the default action of form submission, allowing you to handle the form data using JavaScript before it is sent to the server; - `alert("Form submitted!")`: Displays an alert saying "Form submitted!" when the form is submitted.
### Touch Events Responding to touch interactions, essential for mobile-friendly interfaces. ```javascript const touchArea = document.getElementById("touchArea"); touchArea.addEventListener("touchstart", function () { console.log("Touch started"); }); touchArea.addEventListener("touchend", function () { console.log("Touch ended"); }); ``` **Explanation:** - `getElementById("touchArea")`: Selects the HTML element with the ID `touchArea`; - `addEventListener("touchstart", function() {...})`: Attaches a touchstart event listener. When a touch starts on the element, it logs "Touch started" to the console; - `addEventListener("touchend", function() {...})`: Attaches a touchend event listener. When the touch ends, it logs "Touch ended" to the console.
### Visibility Change Events Handling browser tab visibility changes. ```javascript document.addEventListener("visibilitychange", function () { if (document.visibilityState === "hidden") { console.log("Tab is now hidden"); } else { console.log("Tab is now visible"); } }); ``` **Explanation:** - `addEventListener("visibilitychange", function() {...})`: Attaches a visibilitychange event listener to the document; - `if (document.visibilityState === "hidden")`: Checks if the document's visibility state is hidden; - `console.log("Tab is now hidden")`: Logs "Tab is now hidden" to the console if the document is hidden; - `console.log("Tab is now visible")`: Logs "Tab is now visible" to the console if the document is visible.
Run Code from Your Browser - No Installation Required
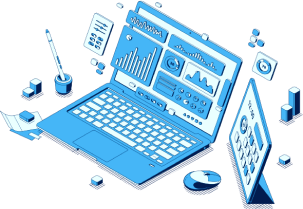
Conclusion
Understanding JavaScript events is fundamental to creating dynamic and interactive web applications. These events, ranging from clicks and key presses to touch interactions and visibility changes, form the core of user interaction on the web. By mastering essential event types and their handling, developers can build responsive interfaces that enhance user experience.
FAQs
Q: What are JavaScript events?
A: JavaScript events are actions or occurrences in a web environment that can be detected and managed by scripts. They enable interactive features in web applications, such as clicks, key presses, mouse movements, and changes in the browser state.
Q: Why are JavaScript events important in web development?
A: JavaScript events are crucial because they allow developers to create interactive and responsive web applications. They help in managing user interactions and dynamically updating the user interface, enhancing the overall user experience.
Q: What are some common types of JavaScript events?
A: Common types of JavaScript events include click events, mouse events (such as mouseover and mouseout), keyboard events (like keypress), form events (such as submit), touch events, and visibility change events.
Q: How do JavaScript events enhance user interactions on a website?
A: JavaScript events enhance user interactions by allowing the website to respond to user actions in real-time. For example, events can trigger animations, validate form inputs, display messages, and update content dynamically based on user behavior.
Q: What are best practices for handling JavaScript events?
A: Best practices for handling JavaScript events include:
- Using event delegation to manage events efficiently.
- Ensuring cross-browser compatibility.
- Avoiding memory leaks by properly removing event listeners when they are no longer needed.
- Keeping event handler functions lean and optimizing performance.
- Enhancing accessibility by considering keyboard and touch interactions.
Start Learning Coding today and boost your Career Potential
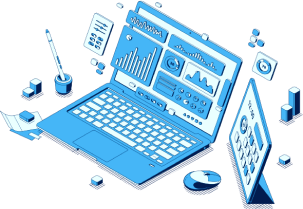
Cursos relacionados
Ver Todos los CursosPrincipiante
Introduction to JavaScript
Learn the fundamentals of JavaScript, the backbone of dynamic web development. Discover essential concepts like syntax, variables, data types, and operators. Explore how to use conditional statements, loops, and functions to create interactive and efficient programs. Master the building blocks of JavaScript and lay the groundwork for more advanced programming skills.
Intermedio
JavaScript Data Structures
Learn to work confidently with data in JavaScript by mastering objects and arrays. Understand how to create, access, and manage object properties and methods effectively. Dive into advanced object manipulation techniques, including iteration, cloning, and destructuring for cleaner code. Build a strong foundation in working with arrays and learn to manage, iterate, and modify array elements efficiently. Master advanced array methods like map, filter, find, and sort to transform and handle data effectively in your applications.
Navigating the YouTube Influencer Maze in Your Tech Career
Staying Focused and Disciplined Amidst Overwhelming Advice
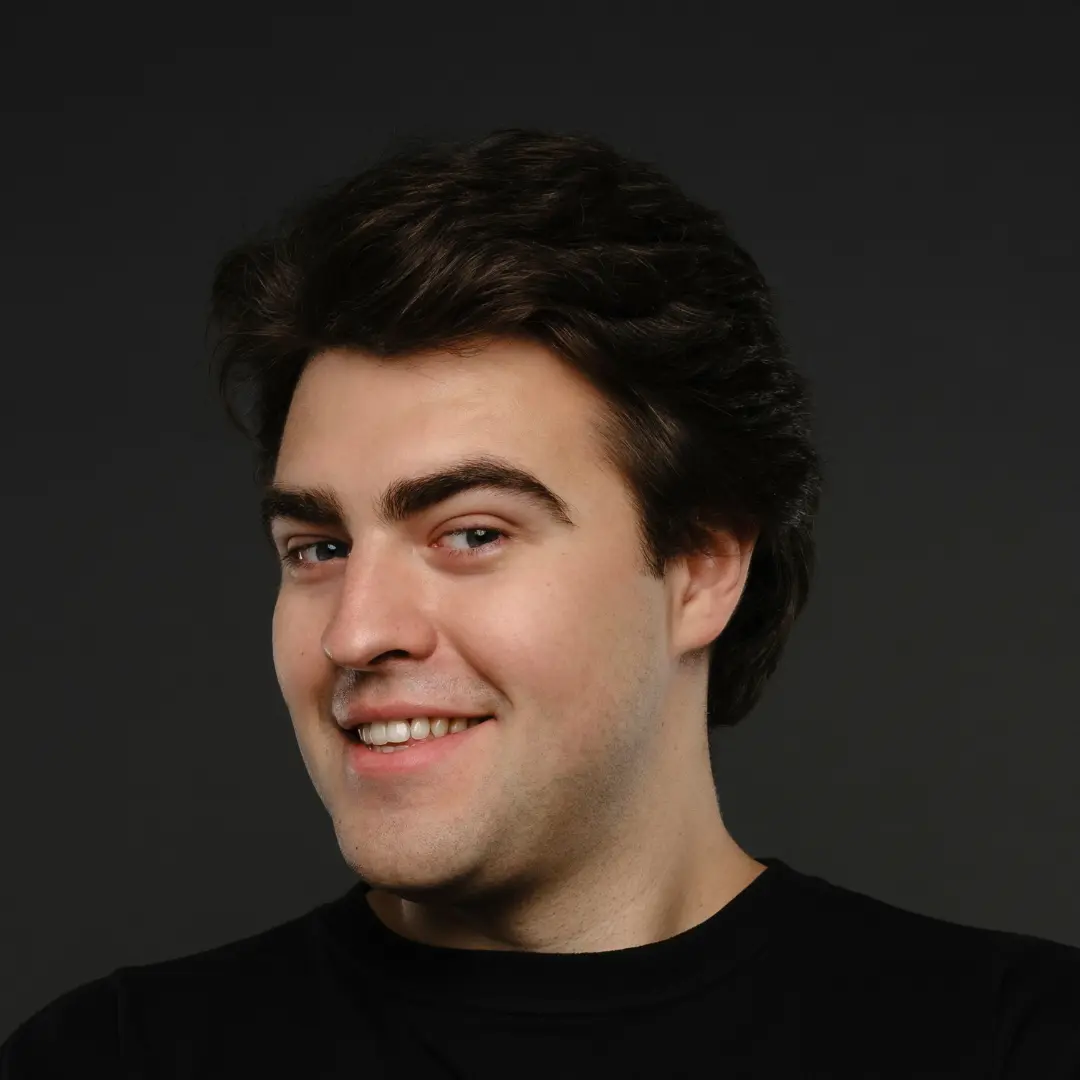
by Oleh Subotin
Full Stack Developer
Jul, 2024・5 min read
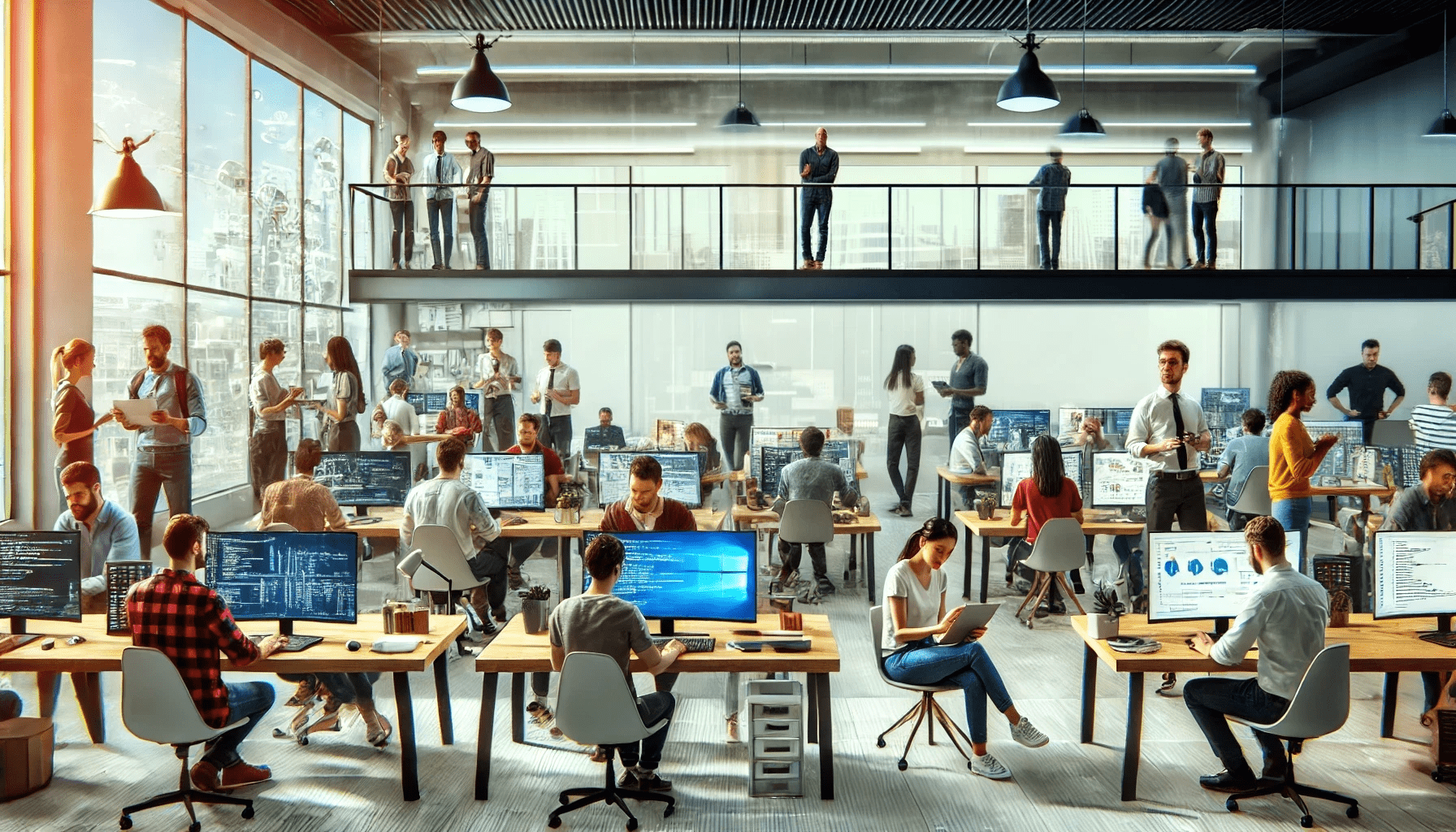
Accidental Innovation in Web Development
Product Development
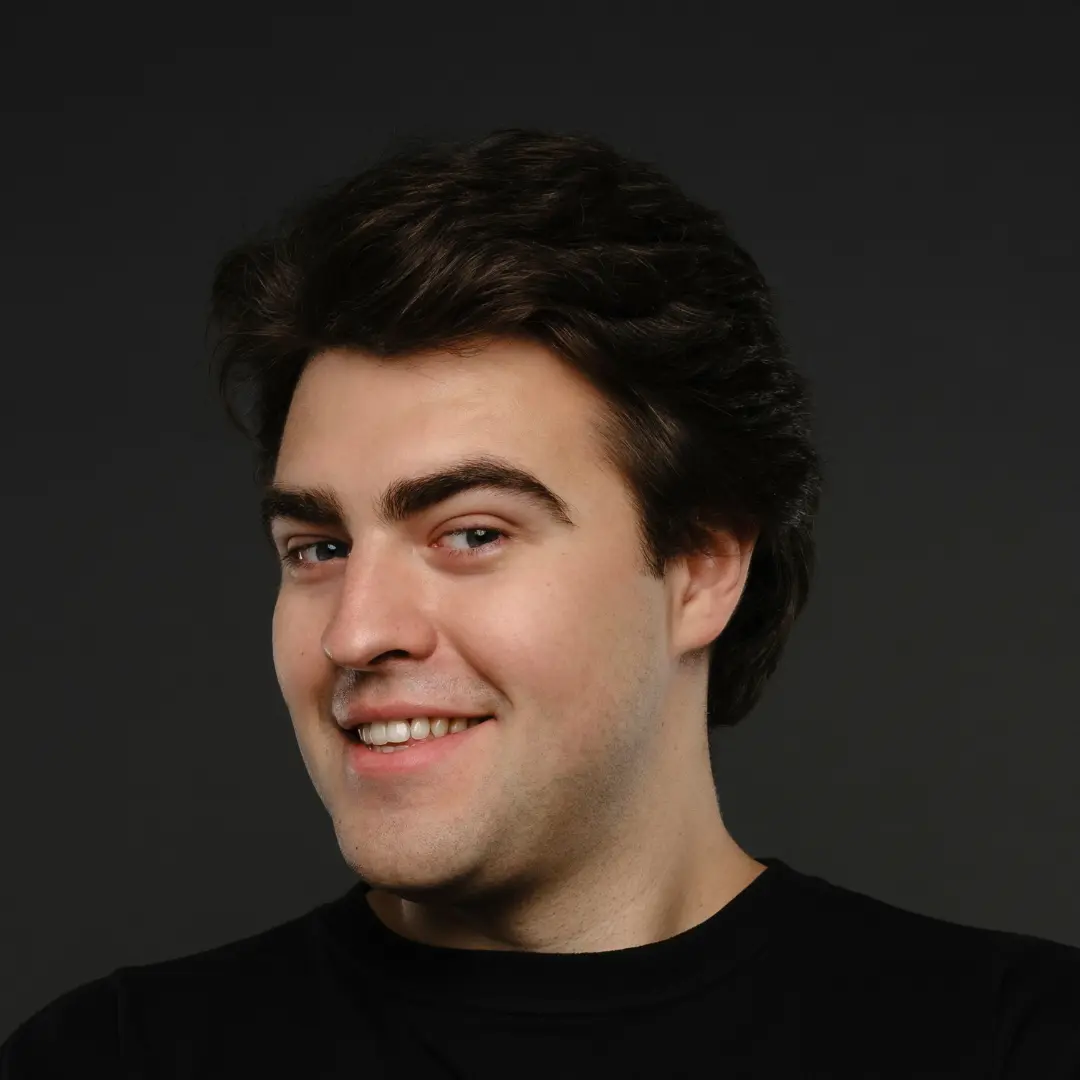
by Oleh Subotin
Full Stack Developer
May, 2024・5 min read
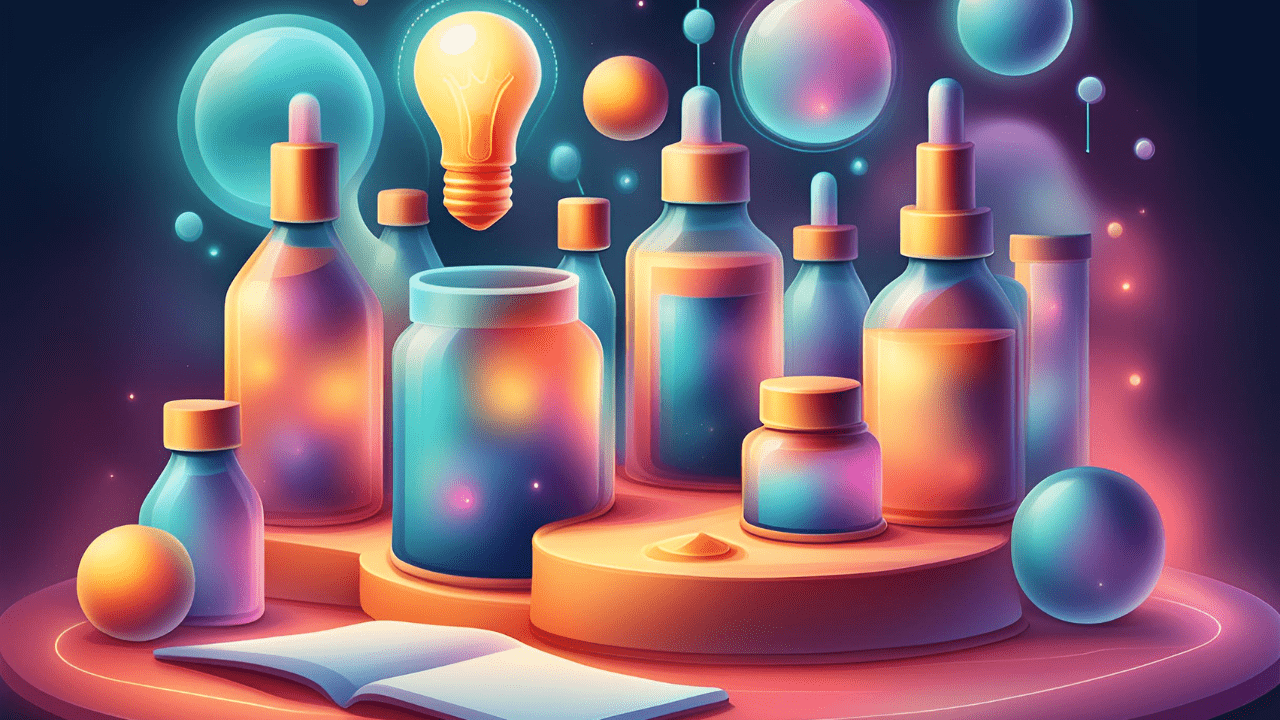
Is a College Degree Essential for a Career as a Developer
Evaluating the Role of Formal Education in Your Tech Career
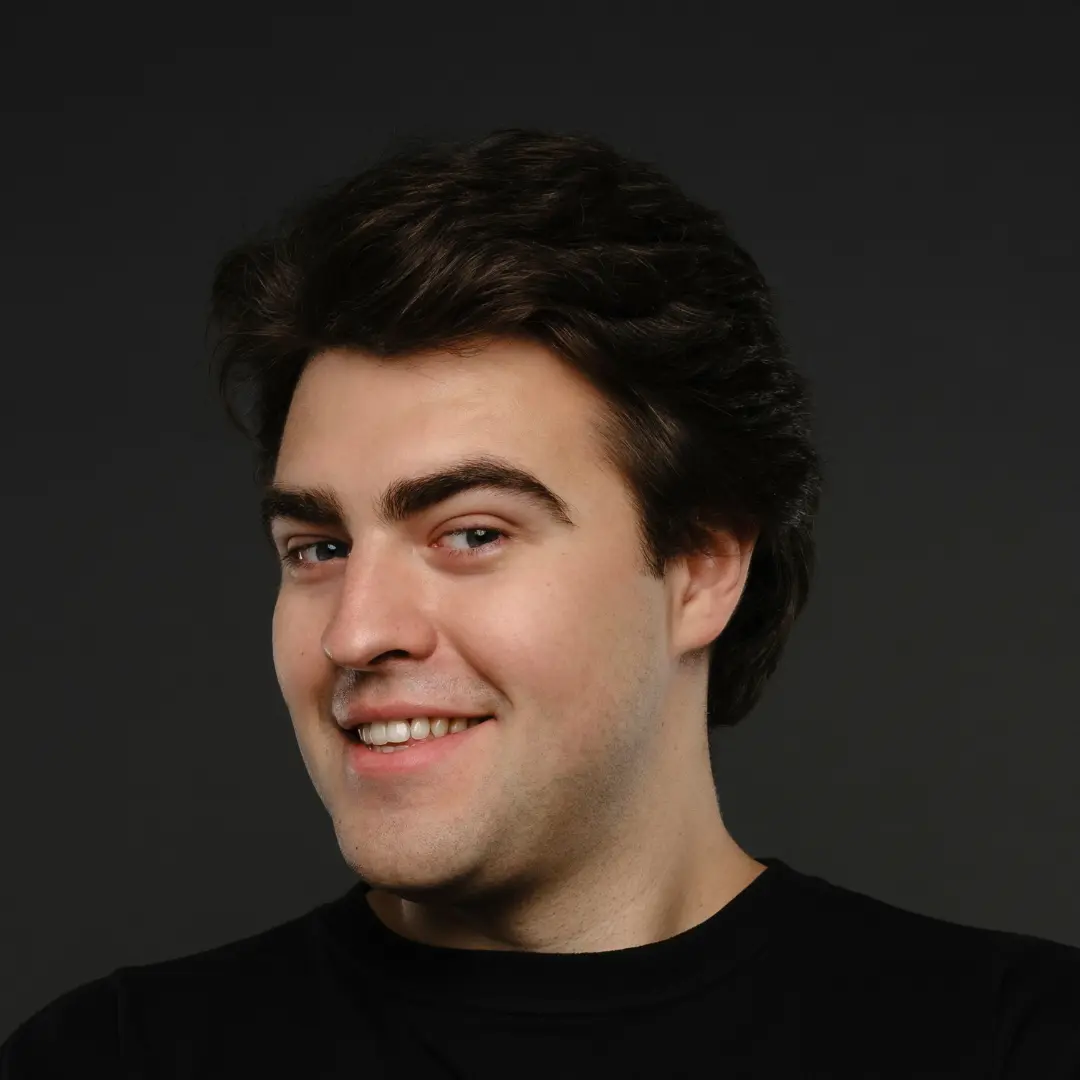
by Oleh Subotin
Full Stack Developer
Jul, 2024・6 min read
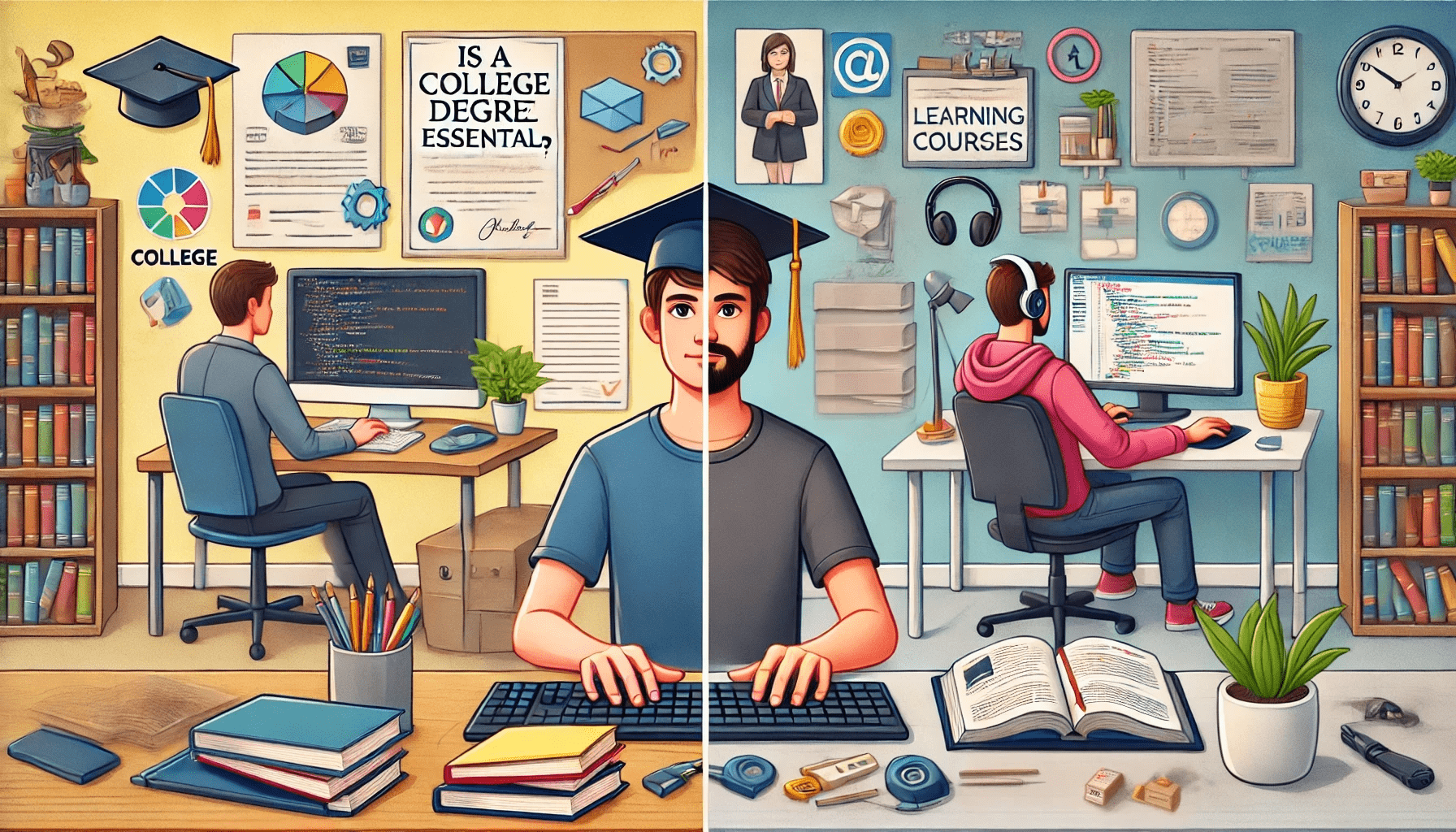
Contenido de este artículo