Cursos relacionados
Ver Todos los CursosPrincipiante
C Basics
This course offers a thorough introduction to the C programming language. Participants will delve into the core concepts, syntax, and structures of C, equipping them to craft basic programs. Key areas of focus encompass variables, data types, control structures, functions, arrays, and pointers. Engaging hands-on activities and projects will provide learners with tangible experience in problem-solving using C. Upon concluding this course, participants will possess a robust understanding of C programming and be primed to explore more intricate subjects.
Intermedio
C++ Pointers and References
Unlock the Power of Memory Manipulation. Dive deep into the fundamentals of programming with this comprehensive course designed for beginners and individuals seeking to strengthen their knowledge on pointers and references in C++. Mastering these essential concepts is crucial for unleashing the full potential of your programming skills.
Avanzado
Introduction to TensorFlow
Dive deep into the world of TensorFlow with our course, designed to give you a robust understanding of its core components. Begin with an exploration of tensors and the basics of TensorFlow framework. By the course's end, you'll have honed the skills to build tensor-driven systems, including crafting a basic neural network. Equip yourself with the knowledge to harness TensorFlow's full potential and set the foundation for advanced deep learning pursuits.
GPU Computing: NVIDIA CUDA Explained
Parallel Computing with CUDA
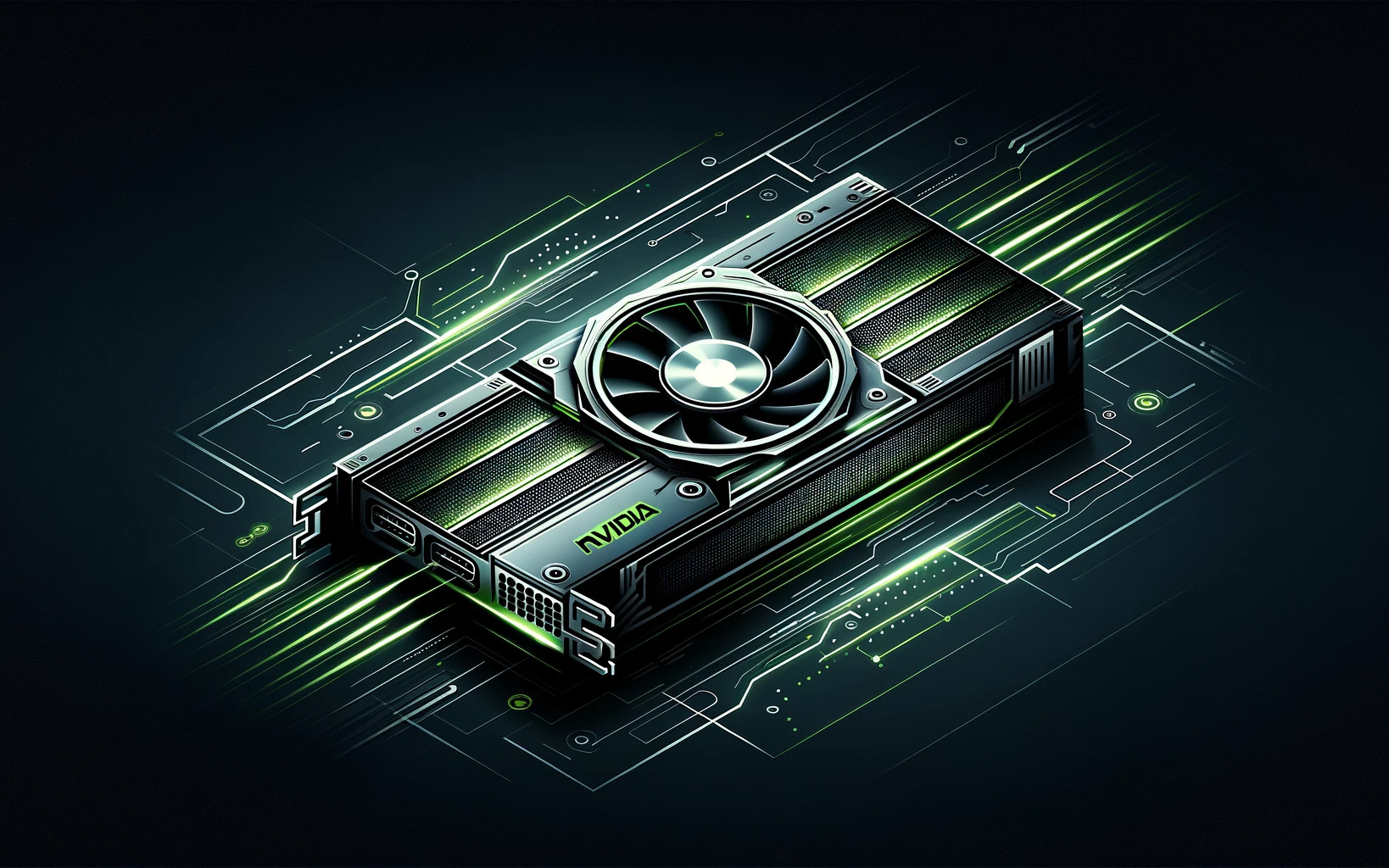
Introduction
In the modern computing landscape, the role of Graphics Processing Units (GPUs) has evolved significantly beyond just rendering graphics. Today, GPUs are at the forefront of accelerating various types of computations, especially in the realms of machine learning, scientific computing, and big data analysis.
NVIDIA's Compute Unified Device Architecture (CUDA) is a revolutionary parallel computing platform and programming model that has made it easier and more accessible for developers to use GPUs for general-purpose processing. This article will delve into the basics of GPU computing and NVIDIA CUDA, exploring its functionality and applications.
What is GPU Computing?
GPU computing, also known as GPGPU (General-Purpose computing on Graphics Processing Units), involves the use of a GPU to perform computation in applications traditionally handled by the Central Processing Unit (CPU). GPUs are particularly well-suited to handle tasks that can be parallelized, i.e., tasks that can be divided into many smaller operations to be carried out simultaneously.
How GPUs Work
Unlike CPUs, which have a few cores optimized for sequential serial processing, GPUs have thousands of smaller, more efficient cores designed for handling multiple tasks simultaneously. This makes GPUs highly effective for algorithms where processing of large blocks of data can be done in parallel.
Run Code from Your Browser - No Installation Required
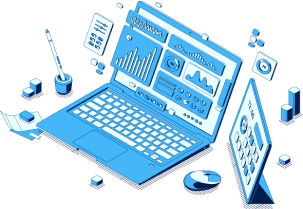
What is NVIDIA CUDA
NVIDIA CUDA is a software layer that gives direct access to the GPU's virtual instruction set and parallel computational elements, for the execution of compute kernels.
Key Components of CUDA
- CUDA Kernels: These are C/C++ functions that CUDA executes on the GPU. Each kernel is executed by an array of CUDA threads.
- CUDA Thread Hierarchy: CUDA organizes threads into blocks and grids. A kernel is executed as a grid of thread blocks.
- CUDA Memory Management: CUDA provides various types of memory (global, shared, constant, and texture), each with unique scopes, lifetimes, and caching behaviors.
Getting Started with CUDA
For beginners, starting with CUDA can seem daunting. Here’s a simplified view of how to begin GPU programming with CUDA:
- Hardware Requirement: You need a compatible NVIDIA GPU that supports CUDA.
- Software Setup: Install the latest NVIDIA driver and the CUDA Toolkit from NVIDIA’s website. The toolkit includes necessary libraries, debugging and optimization tools, a compiler, and a runtime library.
- Programming Model: Understand the basics of parallel computing, the CUDA programming model, and memory management. CUDA extends C/C++ so knowledge of these languages is required.
Simple CUDA Example
The provided code example is a basic CUDA program written in C/C++ that demonstrates how to add two arrays using GPU acceleration. In a real-world, more details and checks might be necessary to ensure robustness and clarity.
c
Including Standard Libraries and CUDA Headers
The example begins by including the standard I/O library, stdio.h
, for basic input and output operations, and the CUDA header files cuda_runtime.h
that define the CUDA runtime API.
Defining the CUDA Kernel
The vectorAdd
function is defined with the __global__
specifier, which indicates that it is a CUDA kernel that should be executed on the GPU:
__global__
: Specifies thatvectorAdd
is a kernel function.int i = threadIdx.x;
: Determines the index of the thread within its block. CUDA organizes threads into blocks, and blocks into a grid.threadIdx.x
provides the x-index of the thread within its block.- Conditional Execution: Ensures that the code inside the conditional block executes only if the thread index
i
is less than the size of the arrays, preventing out-of-bounds memory access.
Main Function
The main()
function serves as the entry point for the program, where the host code is executed.
Memory Allocation and Initialization
Memory for the input and output arrays is allocated on the device using cudaMalloc
, and the arrays are initialized on the host.
Kernel Execution
The kernel is launched with 1 block of n
threads:
- Execution Configuration:
<<<1, n>>>
specifies that the kernel is launched with 1 block containingn
threads.
Retrieving the Result
After the kernel execution, the results are copied back from the device to the host:
c
Cleanup
Finally, the allocated memory is freed:
c
Error Handling
To make the code more robust, especially for educational purposes or real-world applications, you should add error checking after each CUDA API call. This can be done using something like:
c
Repeat this for each CUDA call (e.g., cudaMalloc
, cudaMemcpy
, and kernel launch) to ensure all operations complete successfully.
Applications of CUDA
CUDA has been instrumental in advancing computation in various fields:
- Machine Learning: Speeds up training of neural networks.
- Scientific Computing: Used for simulations and calculations in physics, chemistry, and biology.
- Video and Image Processing: Powers real-time video processing and editing tools.
- Finance: Used in high-frequency trading algorithms.
Start Learning Coding today and boost your Career Potential
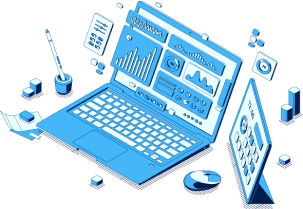
Advanced CUDA Concepts
For more experienced users, CUDA offers depth and complexity that can significantly optimize performance:
- Streams and Concurrency: Execute multiple kernels or memory transfers concurrently.
- Dynamic Parallelism: Allows kernels to launch other kernels.
- Unified Memory: Simplifies memory management by allowing the CPU and GPU to share a single view of memory.
Conclusion
NVIDIA CUDA has transformed the landscape of parallel computing, making it more accessible and robust for a wide range of applications. Whether you are a novice interested in accelerating simple tasks, or an advanced developer optimizing complex algorithms, CUDA offers tools and capabilities to significantly enhance performance and efficiency.
FAQs
Q: How do I check if my NVIDIA GPU supports CUDA?
A: You can check your GPU model on the NVIDIA website to see if it supports CUDA. Most modern NVIDIA GPUs do.
Q: What programming languages can be used with CUDA?
A: CUDA primarily supports programming in C/C++. However, there are extensions available for Python, Java, and other languages, typically through third-party libraries like PyCUDA for Python.
Q: Is CUDA only available on NVIDIA GPUs?
A: Yes, CUDA is a proprietary technology developed by NVIDIA for their GPUs. AMD and other vendors have their own parallel computing platforms like ROCm.
Q: Can CUDA be used for deep learning?
A: Absolutely. CUDA accelerates deep learning frameworks like TensorFlow and PyTorch, which can run up to 50x faster on a GPU than on a CPU alone.
Q: Where can I learn more about CUDA programming?
A: NVIDIA provides extensive documentation and tutorials on their official website. Additionally, online courses and tutorials are available to help beginners and advanced users alike.
Cursos relacionados
Ver Todos los CursosPrincipiante
C Basics
This course offers a thorough introduction to the C programming language. Participants will delve into the core concepts, syntax, and structures of C, equipping them to craft basic programs. Key areas of focus encompass variables, data types, control structures, functions, arrays, and pointers. Engaging hands-on activities and projects will provide learners with tangible experience in problem-solving using C. Upon concluding this course, participants will possess a robust understanding of C programming and be primed to explore more intricate subjects.
Intermedio
C++ Pointers and References
Unlock the Power of Memory Manipulation. Dive deep into the fundamentals of programming with this comprehensive course designed for beginners and individuals seeking to strengthen their knowledge on pointers and references in C++. Mastering these essential concepts is crucial for unleashing the full potential of your programming skills.
Avanzado
Introduction to TensorFlow
Dive deep into the world of TensorFlow with our course, designed to give you a robust understanding of its core components. Begin with an exploration of tensors and the basics of TensorFlow framework. By the course's end, you'll have honed the skills to build tensor-driven systems, including crafting a basic neural network. Equip yourself with the knowledge to harness TensorFlow's full potential and set the foundation for advanced deep learning pursuits.
The SOLID Principles in Software Development
The SOLID Principles Overview
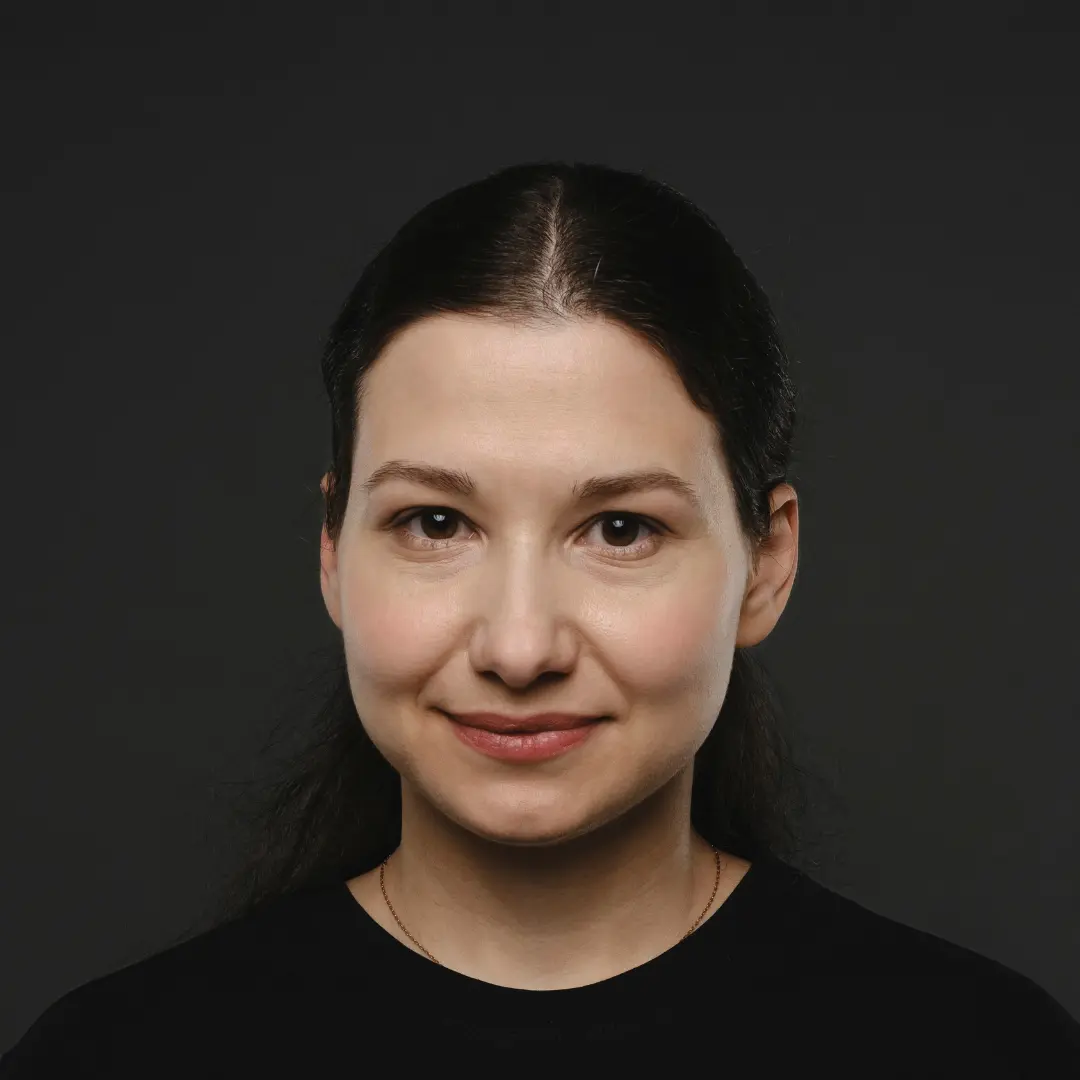
by Anastasiia Tsurkan
Backend Developer
Nov, 2023・8 min read
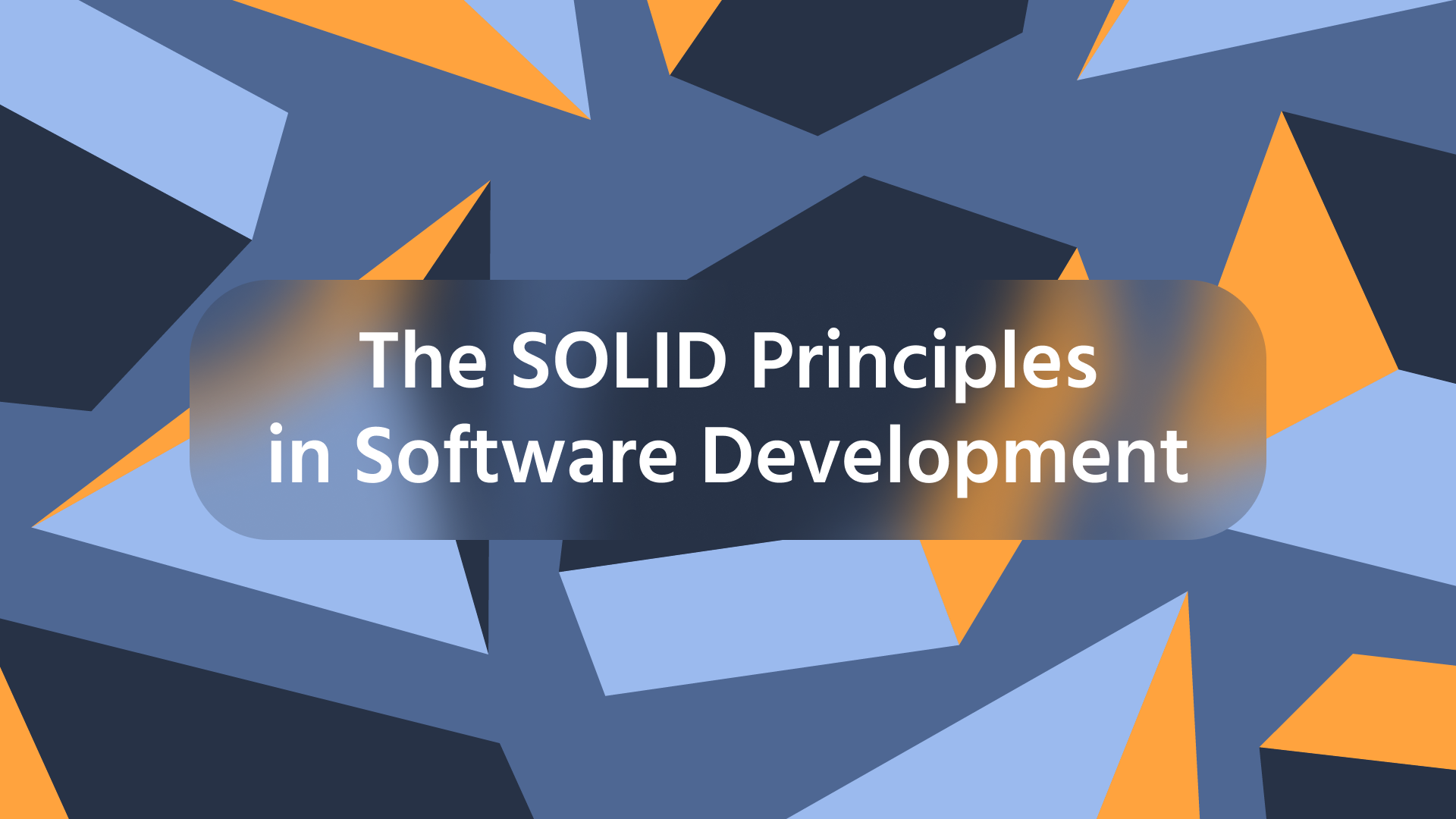
The Facade Pattern Explained
Simplify Complex Systems with a Unified Interface
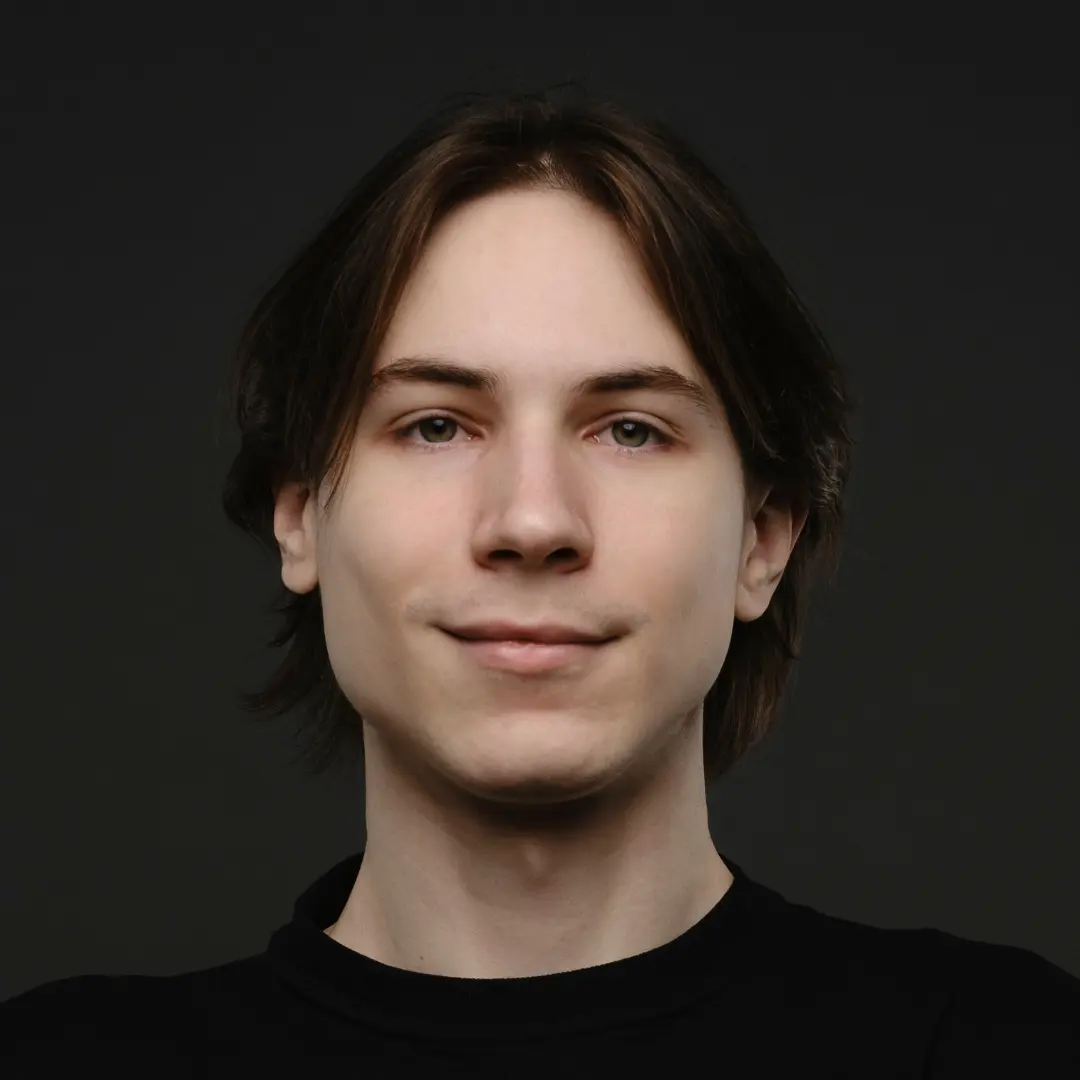
by Ihor Gudzyk
C++ Developer
May, 2025・6 min read
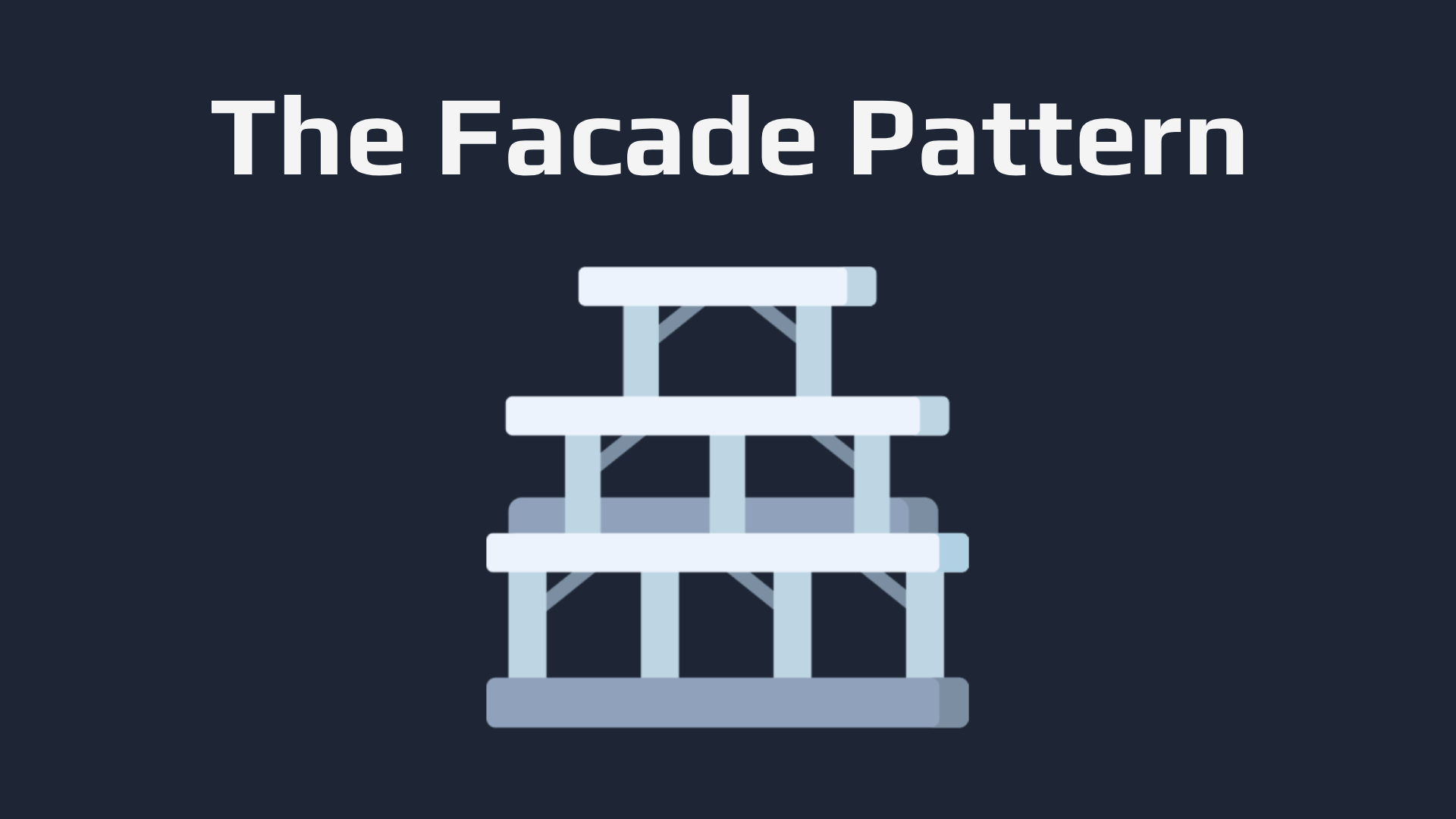
30 Python Project Ideas for Beginners
Python Project Ideas
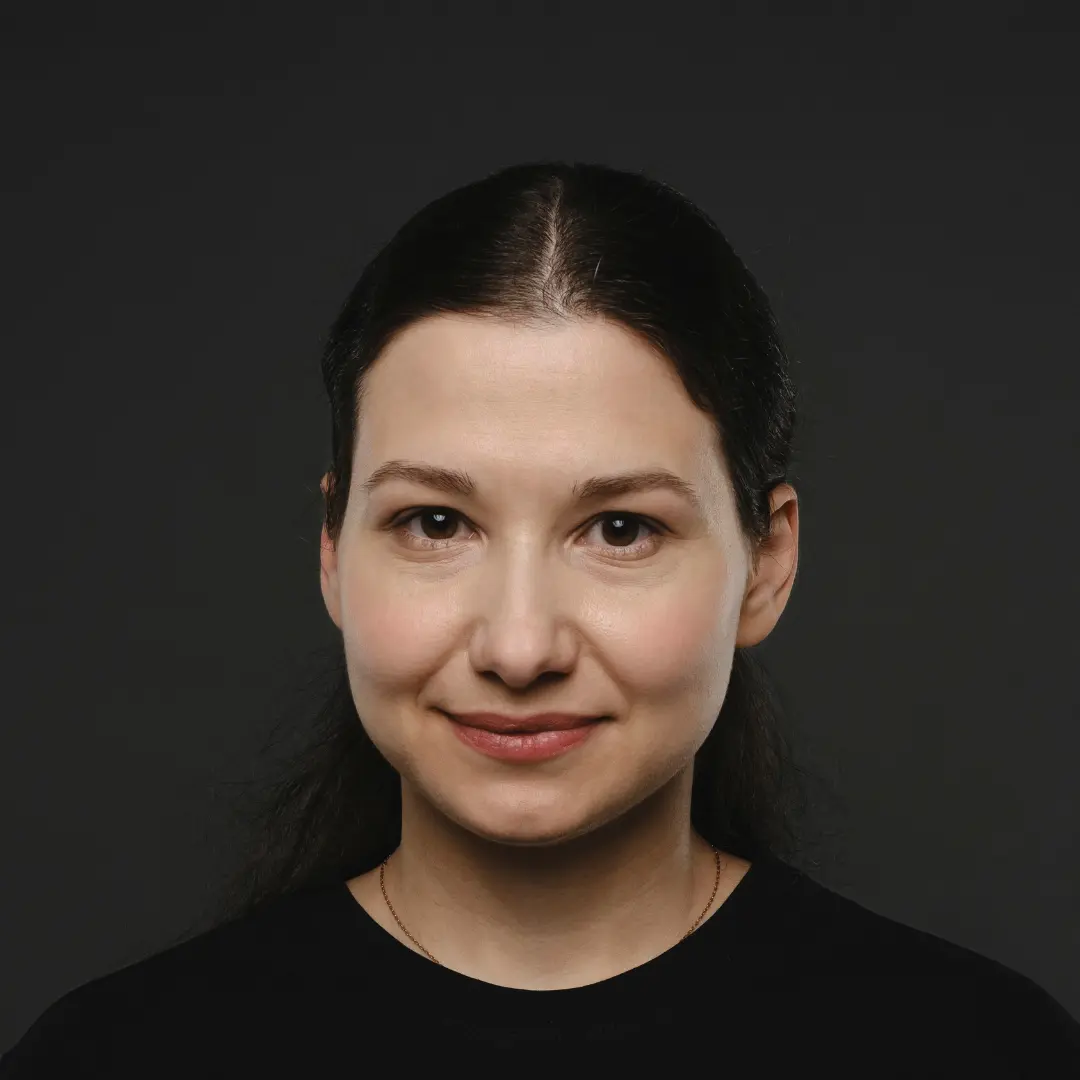
by Anastasiia Tsurkan
Backend Developer
Sep, 2024・14 min read
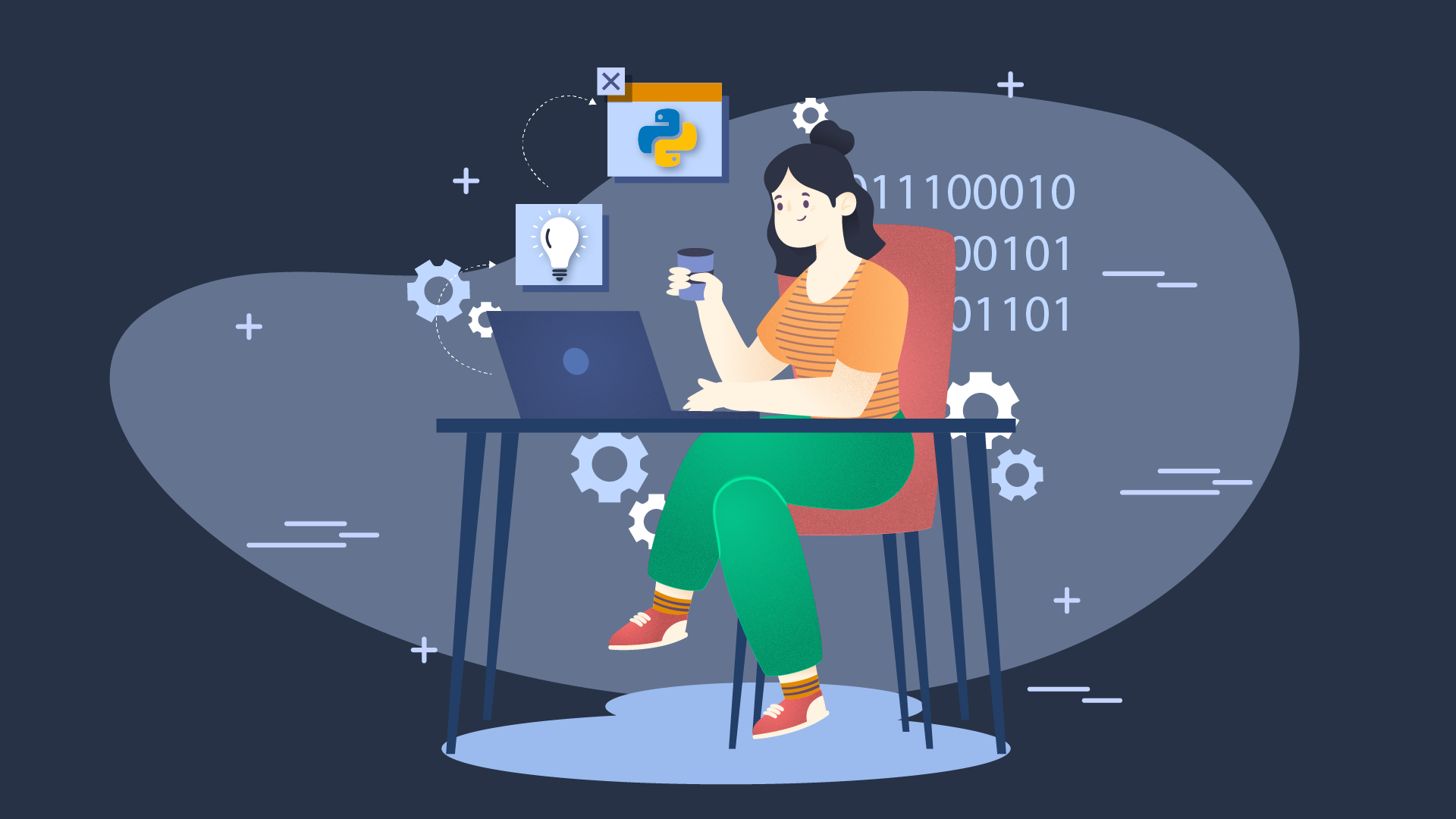
Contenido de este artículo