Cursos relacionados
Ver Todos los CursosPrincipiante
Introduction to JavaScript
Learn the fundamentals of JavaScript, the backbone of dynamic web development. Discover essential concepts like syntax, variables, data types, and operators. Explore how to use conditional statements, loops, and functions to create interactive and efficient programs. Master the building blocks of JavaScript and lay the groundwork for more advanced programming skills.
Intermedio
JavaScript Data Structures
Discover the foundational building blocks of JavaScript. Immerse yourself in objects, arrays, and crucial programming principles, becoming proficient in the fundamental structures that form the basis of contemporary web development.
Principiante
Introduction to TypeScript
This course is designed for absolute beginners who want to master the basics of the TypeScript programming language. TypeScript is a modern and powerful language that extends the capabilities of JavaScript, making your code more reliable and readable. We will start from the very basics, covering variables, data types, comparison operators, and conditional statements. Then, we will delve into working with arrays and loops. Upon completing this course, you will be ready to create simple programs in TypeScript and continue your learning journey into more advanced topics.
Mastering Asynchronous JavaScript
Efficient Asynchronous Programming
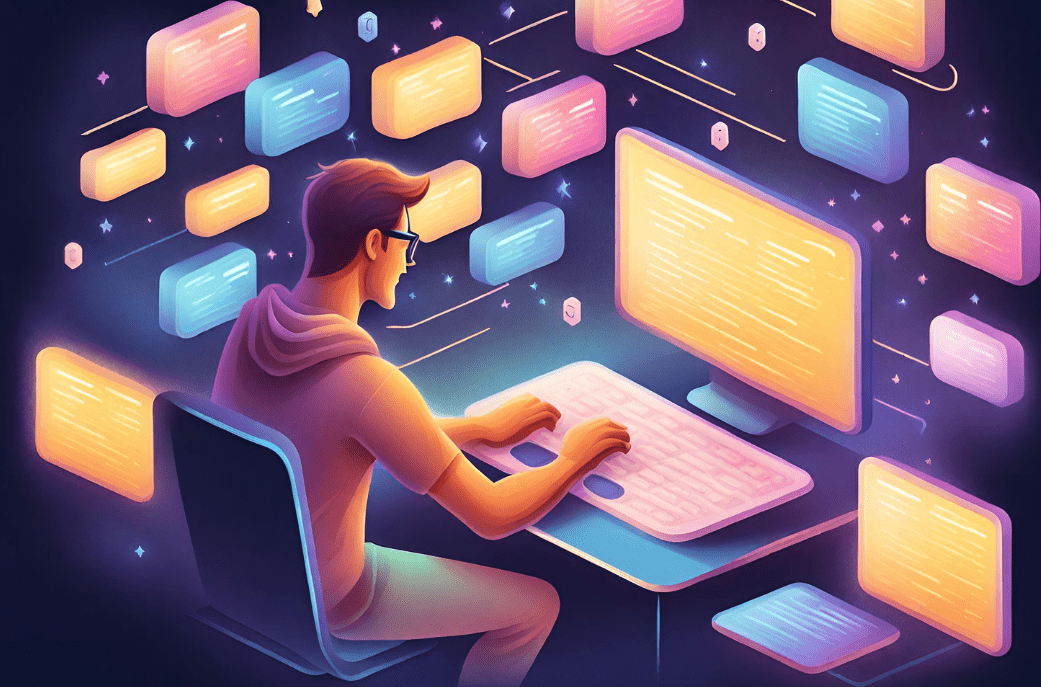
Introduction
In the realm of modern web development, asynchronous programming plays a pivotal role in ensuring smooth and responsive user experiences. JavaScript and its superset TypeScript are no strangers to this concept, with asynchronous operations being fundamental for handling various tasks such as fetching data from servers, processing user input, and interacting with APIs. However, navigating through asynchronous code can sometimes be challenging, leading to issues like callback hell and decreased code readability. In this article, we'll delve into the world of asynchronous JavaScript, exploring different patterns and techniques to effectively manage asynchronous operations.
Run Code from Your Browser - No Installation Required
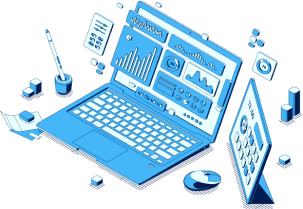
Understanding Asynchronous JavaScript
At its core, asynchronous JavaScript enables code execution to continue while waiting for certain operations to complete, rather than halting the entire program. This is particularly crucial in scenarios where tasks may take an unpredictable amount of time, such as network requests or file I/O operations.
Callbacks
Callbacks are one of the earliest forms of handling asynchronous operations in JavaScript. They involve passing a function as an argument to another function, to be executed once the asynchronous task is complete. While callbacks are functional, they can lead to deeply nested code structures, commonly referred to as "callback hell," making code maintenance and readability cumbersome.
Example:
js
Promises
Promises provide a more structured approach to handling asynchronous code compared to callbacks. They represent a proxy for a value that will eventually become available, either resolving with a value or rejecting with an error. Promises offer a cleaner syntax and help mitigate callback hell by enabling chaining of asynchronous operations.
Example:
js
Async/Await
Introduced in ES2017, async/await provides a syntactic sugar on top of promises, offering a more intuitive and synchronous-like way of writing asynchronous code. Async functions return a promise implicitly, allowing developers to write asynchronous code in a more linear fashion, enhancing readability and maintainability.
Example:
js
Best Practices for Managing Asynchronous Code
While asynchronous programming offers immense flexibility, following best practices to ensure code remains manageable and maintainable is essential.
- Use Promises Wisely: Favor promises over callbacks for cleaner code. Embrace promise chaining to sequence asynchronous operations effectively.
- Leverage Async/Await: Embrace async/await for writing asynchronous code that resembles synchronous logic, making it easier to understand and maintain.
- Error Handling: Always handle errors appropriately using try/catch blocks or .catch() with promises to prevent unhandled rejections.
- Avoid Callback Hell: Refactor deeply nested callback structures by modularizing code or migrating to promises or async/await.
- Keep Functions Small and Focused: Break down complex asynchronous operations into smaller, manageable functions, promoting code reusability and readability.
Start Learning Coding today and boost your Career Potential
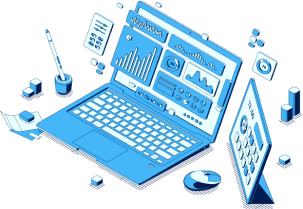
Conclusion
Asynchronous programming is a cornerstone of modern JavaScript and TypeScript development. By understanding and implementing various asynchronous patterns like callbacks, promises, and async/await, developers can write efficient, maintainable code while avoiding common pitfalls such as callback hell. Embracing best practices ensures that asynchronous code remains manageable and enhances overall code quality, leading to better user experiences in web applications.
FAQs
Q: What is asynchronous JavaScript, and why is it important?
A: Asynchronous JavaScript allows code execution to continue while waiting for certain operations to complete, rather than halting the entire program. This is crucial for tasks that may take an unpredictable amount of time, such as network requests or file I/O operations.
Q: What are callbacks, and how are they used in handling asynchronous operations?
A: Callbacks involve passing a function as an argument to another function, to be executed once the asynchronous task is complete. While functional, callbacks can lead to nested code structures, known as "callback hell," making code maintenance and readability cumbersome.
Q: What are promises, and how do they improve asynchronous code handling compared to callbacks?
A: Promises provide a structured approach to handling asynchronous code by representing a proxy for a value that will eventually become available, either resolving with a value or rejecting with an error. They offer cleaner syntax and enable chaining of asynchronous operations, helping mitigate "callback hell."
Q: What is async/await, and how does it simplify asynchronous code writing?
A: Async/await, introduced in ES2017, offers a syntactic sugar on top of promises, providing a more intuitive and synchronous-like way of writing asynchronous code. Async functions implicitly return a promise, allowing developers to write asynchronous code in a linear fashion, enhancing readability and maintainability.
Cursos relacionados
Ver Todos los CursosPrincipiante
Introduction to JavaScript
Learn the fundamentals of JavaScript, the backbone of dynamic web development. Discover essential concepts like syntax, variables, data types, and operators. Explore how to use conditional statements, loops, and functions to create interactive and efficient programs. Master the building blocks of JavaScript and lay the groundwork for more advanced programming skills.
Intermedio
JavaScript Data Structures
Discover the foundational building blocks of JavaScript. Immerse yourself in objects, arrays, and crucial programming principles, becoming proficient in the fundamental structures that form the basis of contemporary web development.
Principiante
Introduction to TypeScript
This course is designed for absolute beginners who want to master the basics of the TypeScript programming language. TypeScript is a modern and powerful language that extends the capabilities of JavaScript, making your code more reliable and readable. We will start from the very basics, covering variables, data types, comparison operators, and conditional statements. Then, we will delve into working with arrays and loops. Upon completing this course, you will be ready to create simple programs in TypeScript and continue your learning journey into more advanced topics.
The SOLID Principles in Software Development
The SOLID Principles Overview
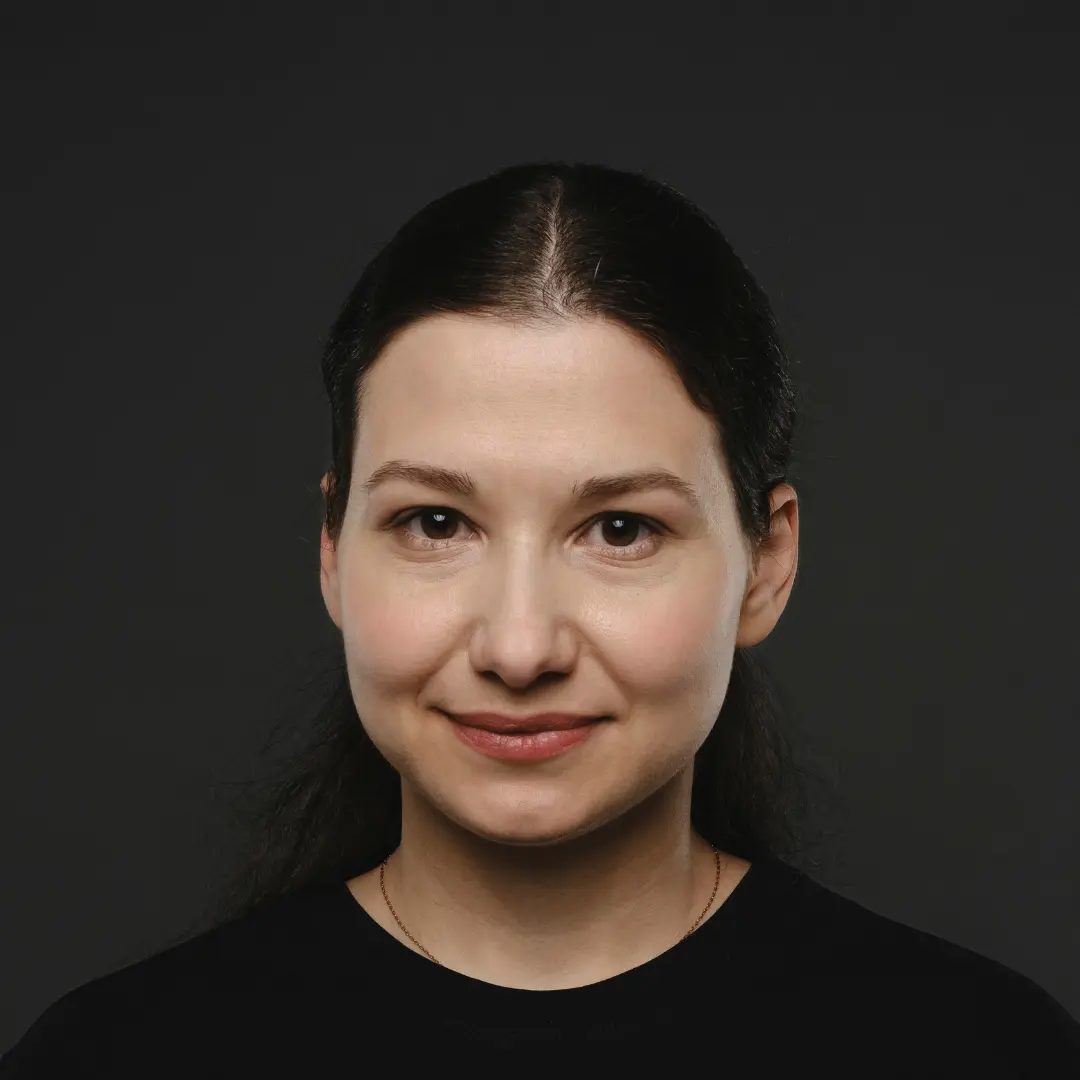
by Anastasiia Tsurkan
Backend Developer
Nov, 2023・8 min read
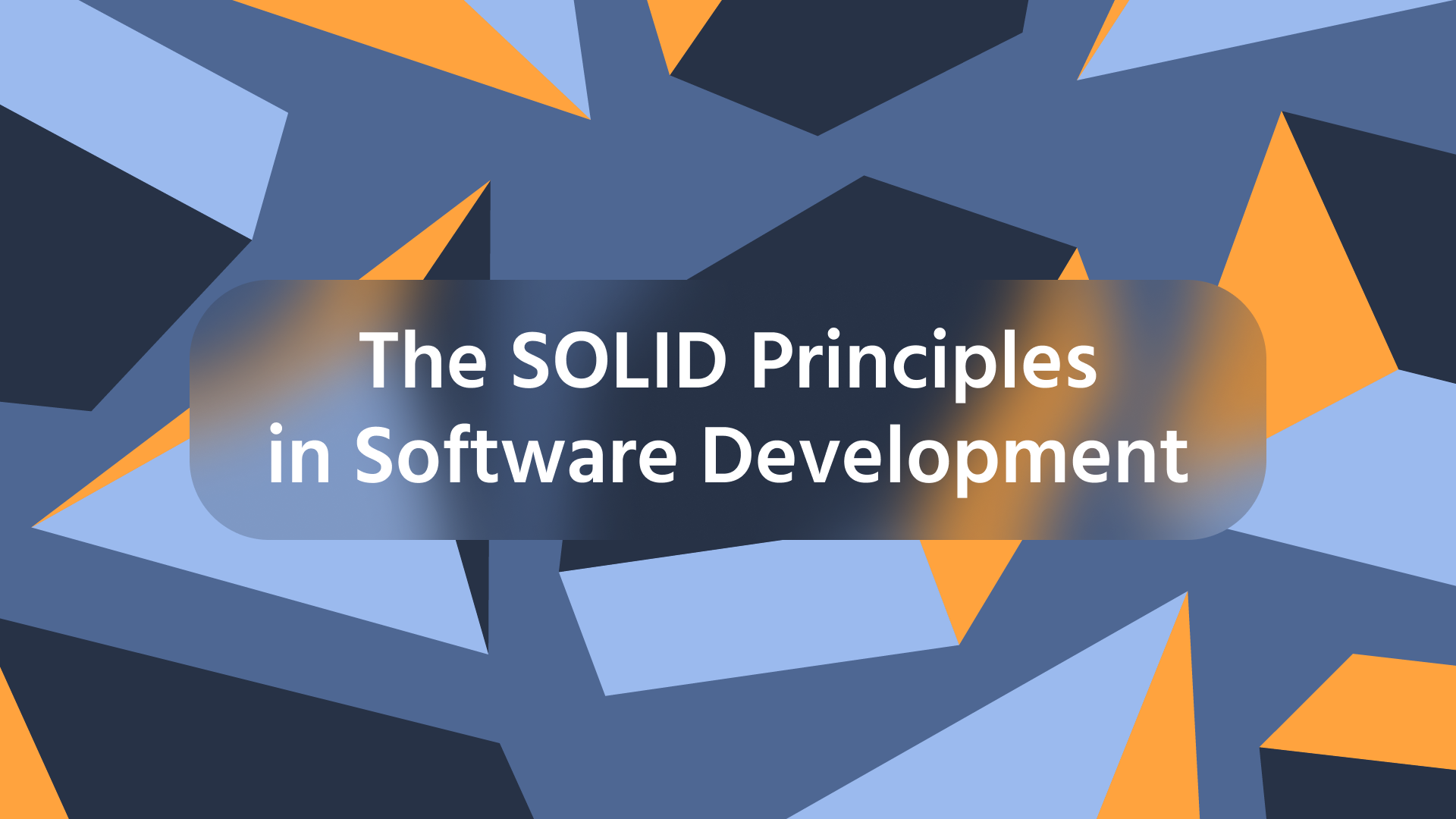
Match-case Operators in Python
Match-case Operators vs if-elif-else statements
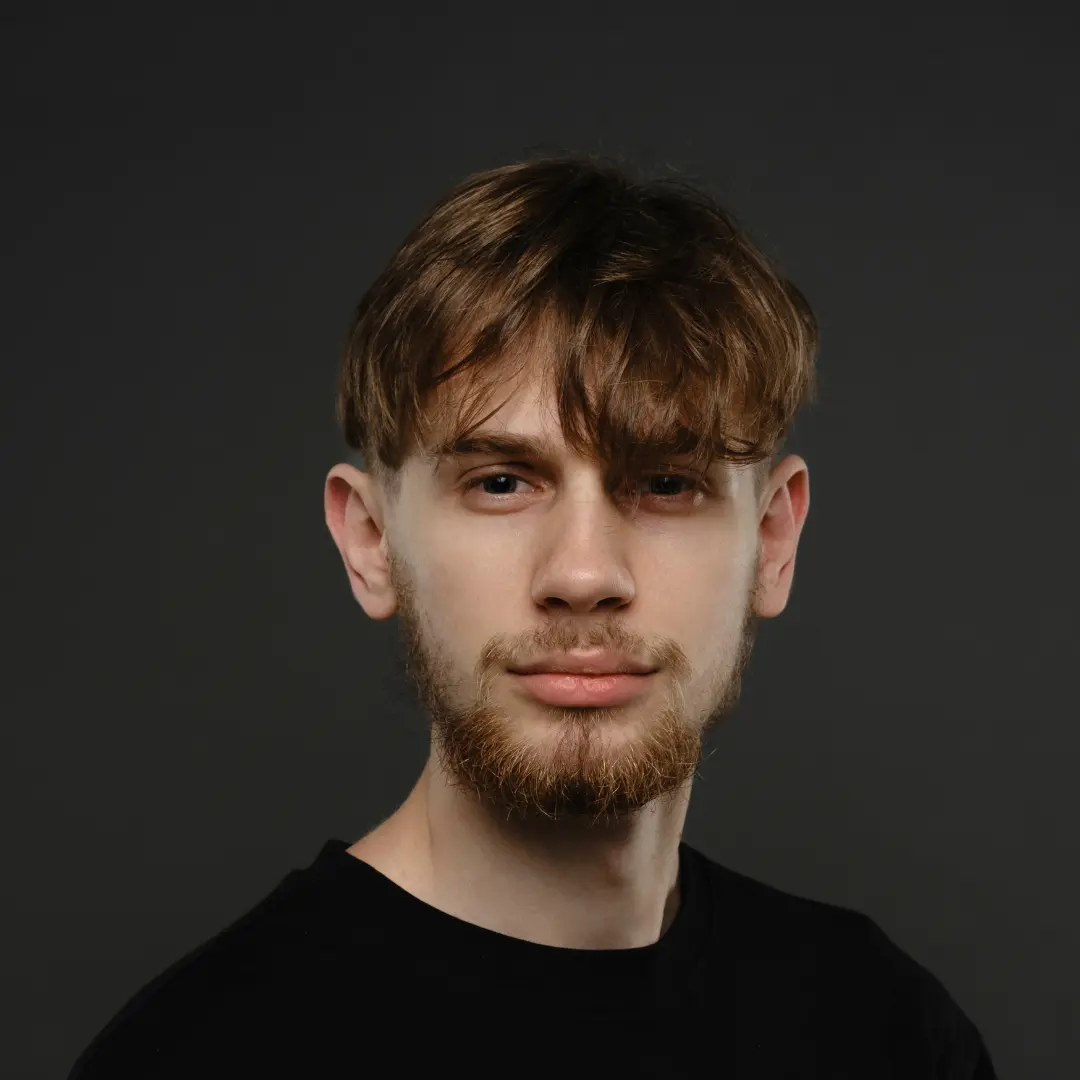
by Oleh Lohvyn
Backend Developer
Dec, 2023・6 min read
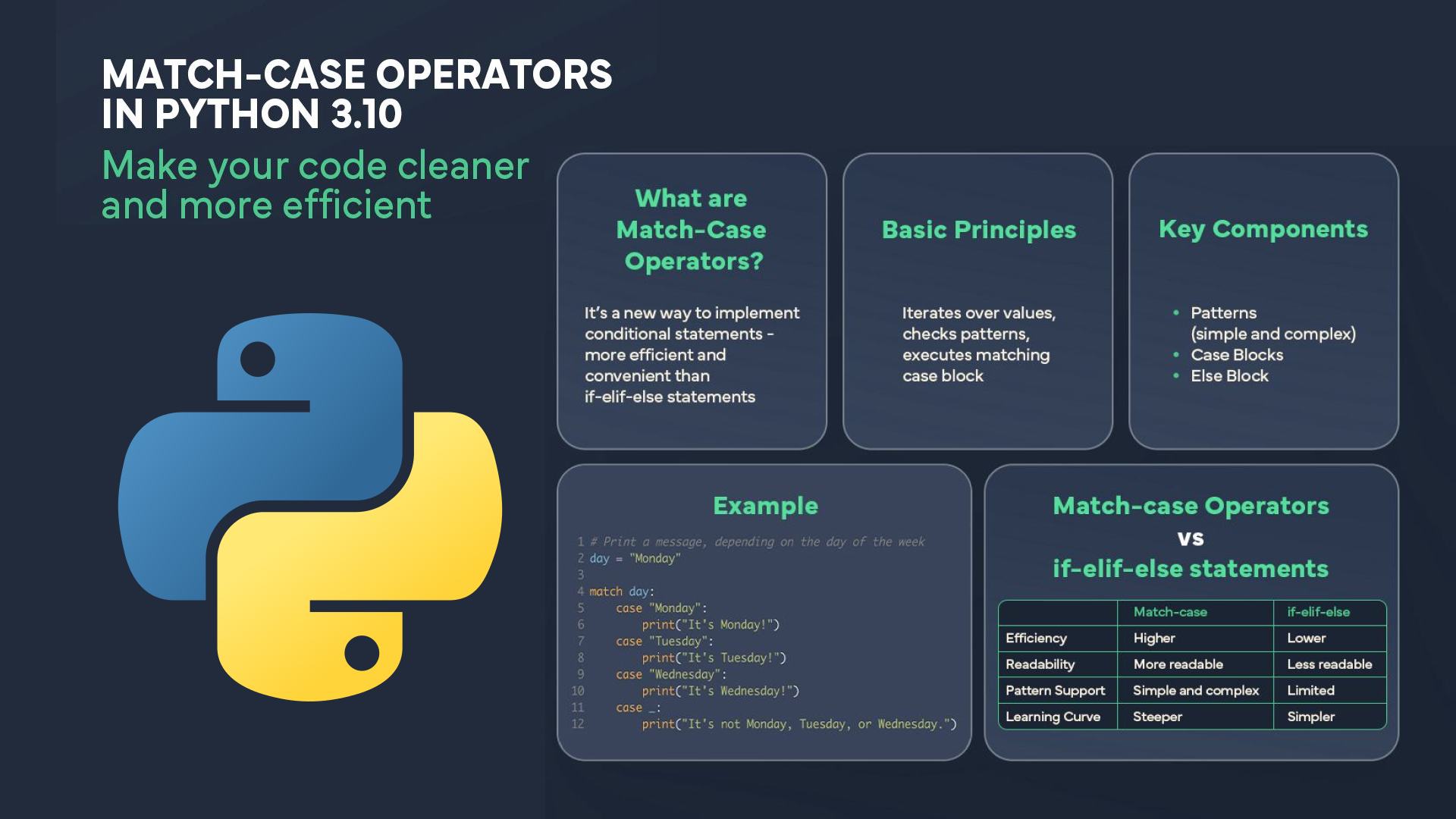
30 Python Project Ideas for Beginners
Python Project Ideas
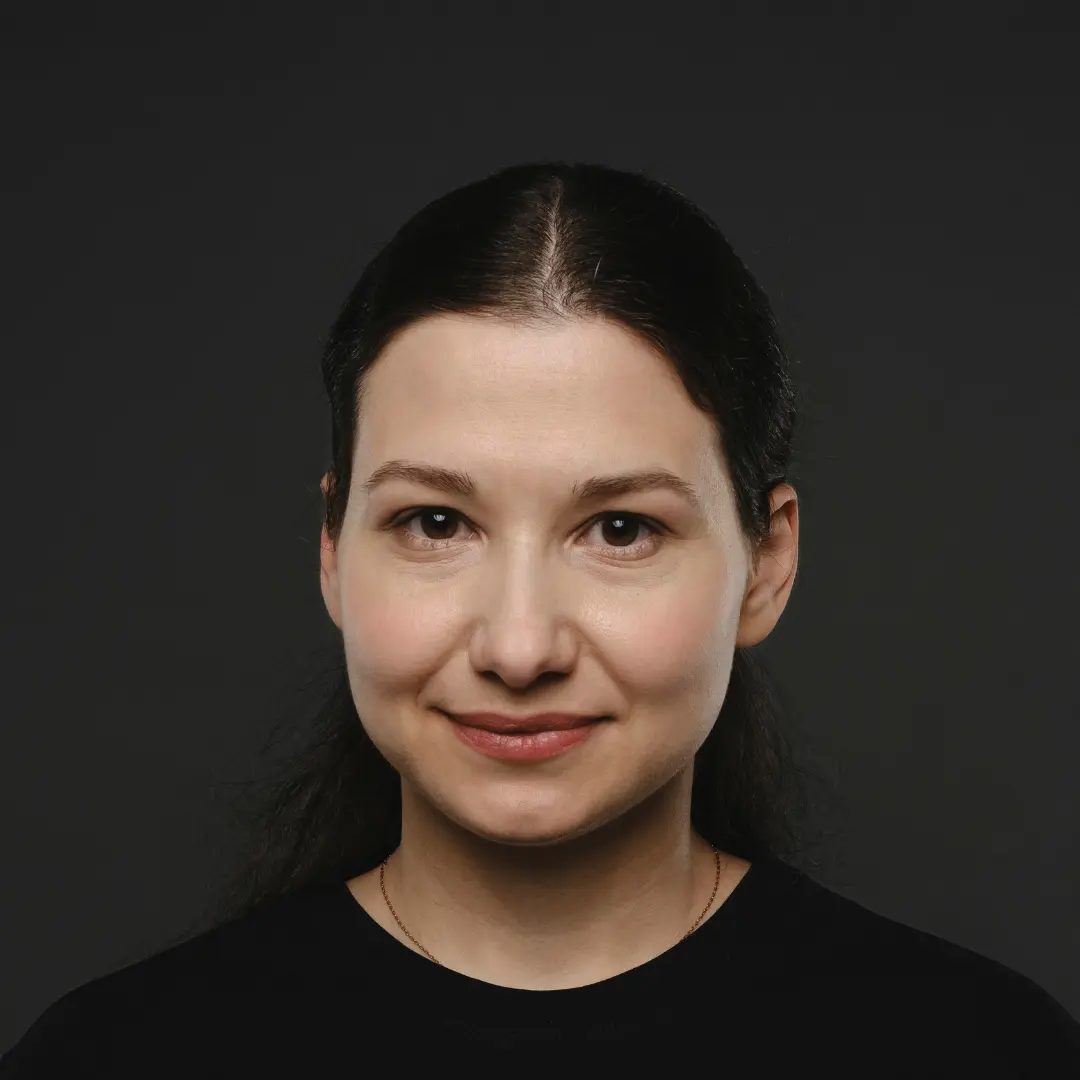
by Anastasiia Tsurkan
Backend Developer
Sep, 2024・14 min read
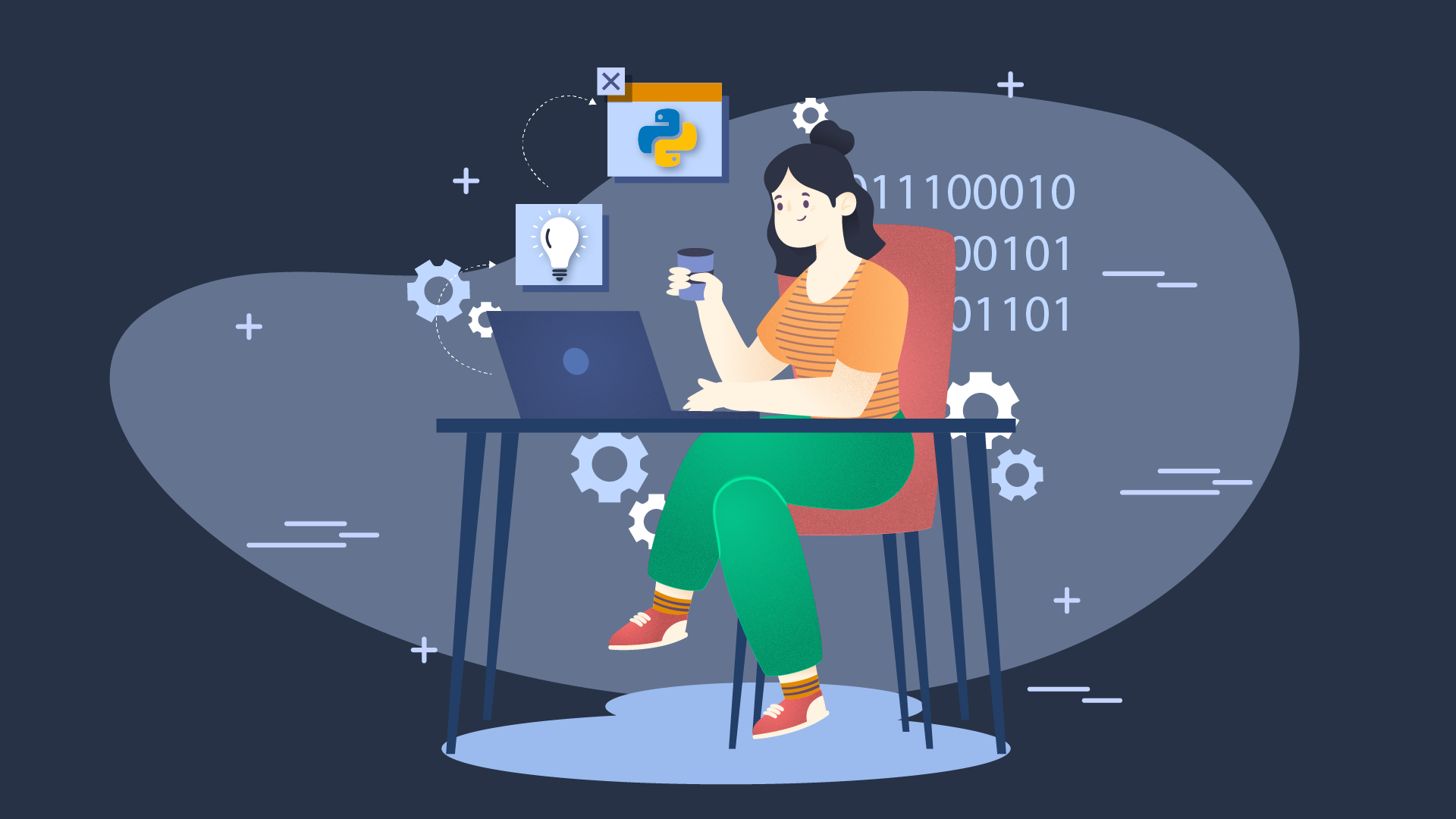
Contenido de este artículo