Cursos relacionados
Ver Todos los CursosAvanzado
Django REST Framework
The Django REST Framework course opens the gateway to the world of web interface development, where you'll learn to create connections between servers and clients through APIs. As you delve into the technical details, you'll master how to transform complex data structures into network-friendly formats and vice versa. You'll understand how to efficiently handle user requests and return responses in a format that seamlessly integrates into web applications.
Avanzado
Django ORM Ninja: Advanced Techniques for Developers
Learn how to build applications using the Django ORM framework. This is the next step in expanding your knowledge of Django development.
Intermedio
Django: Build Your First Website
This exciting course is designed for those who aspire to learn web development and create their own website using the powerful Django framework with the Python programming language. From the basics to advanced functionalities, the course offers everything you need to successfully launch your first web project.
Mastering REST APIs in Django A Comprehensive Guide
REST API
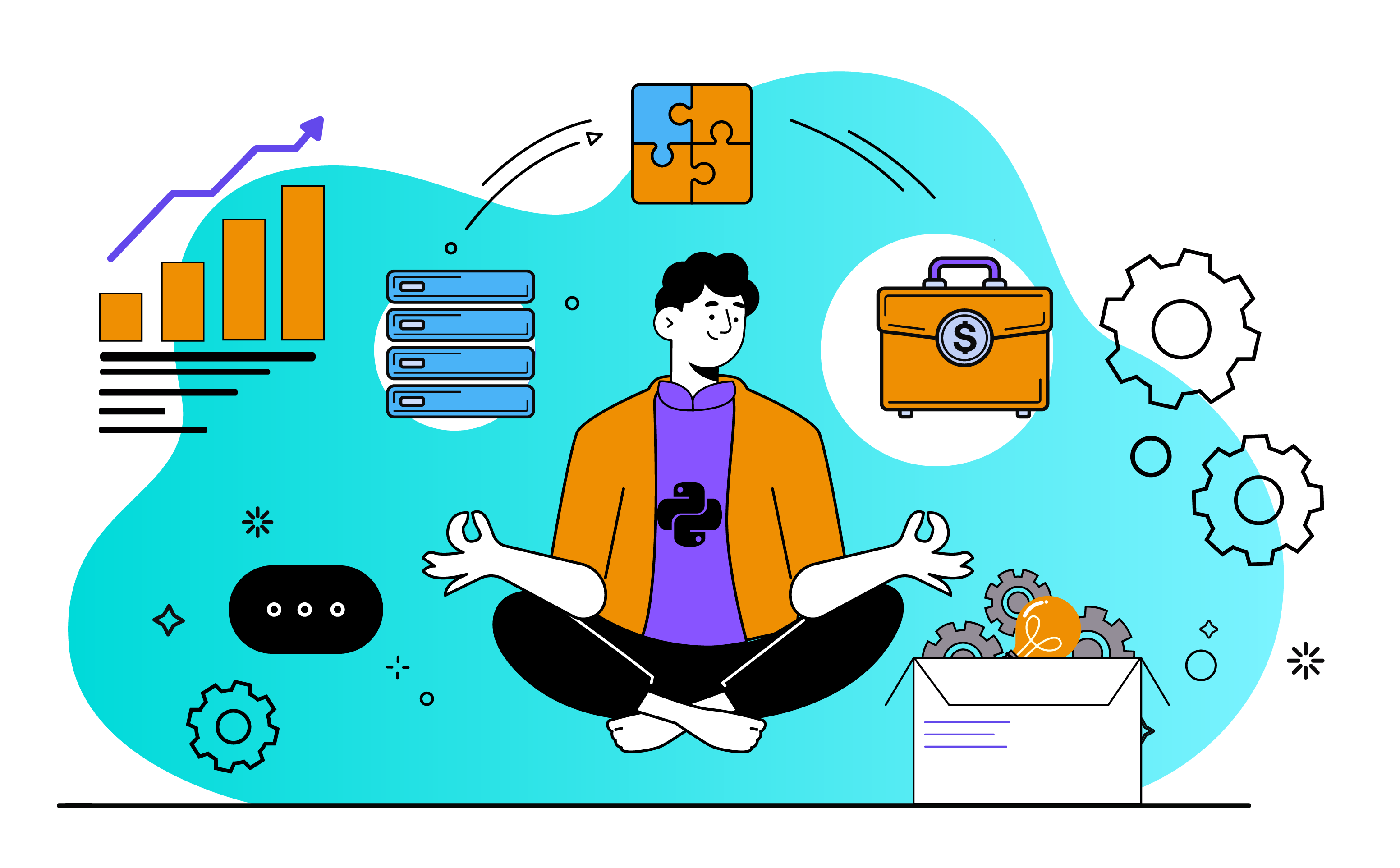
Django, a high-level Python web framework, is renowned for facilitating rapid development and clean, pragmatic design. It simplifies the construction of complex, database-driven websites, enabling developers to focus on crafting robust applications efficiently.
Central to modern web development is the REST API (Representational State Transfer Application Programming Interface), a standard that allows different software systems to communicate via HTTP methods. REST APIs are integral in creating responsive, scalable web applications, making them indispensable in today's interconnected digital landscape.
In the Django context, REST APIs are particularly significant. Django's comprehensive features, coupled with the Django REST Framework (DRF), provide a powerful toolkit for building and managing RESTful web services. This synergy allows developers to efficiently create versatile, high-performance web applications, leveraging Django's robustness and REST API's flexibility.
Section 1: Understanding REST APIs
RESTful services are an architectural style for designing networked applications. They rely on stateless, client-server communication, where each request from any client contains all the information needed to service the request. At its core, REST uses standard HTTP methods, making it easily adoptable. These methods include:
- GET: Retrieve data from a server.
- POST: Send data to a server to create a new resource.
- PUT: Update existing data on a server.
- DELETE: Remove data from a server.
By using these methods, REST APIs facilitate straightforward and efficient data manipulation over the web, adhering to principles of simplicity and scalability.
Run Code from Your Browser - No Installation Required
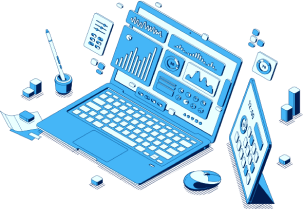
Section 2: Django REST Framework (DRF)
The Django REST Framework (DRF) is a powerful and versatile toolkit for building Web APIs in Django. It enhances Django's capabilities, making it more efficient and effective for developing complex API architectures. DRF stands out for its user-friendly, modular, and customizable nature, allowing developers to build APIs tailored to their specific needs.
Key features of DRF include:
- Serialization: It transforms complex data types like querysets and model instances into JSON, XML, or other content types. This feature is vital for sending data over HTTP and ensuring compatibility with different systems.
- Authentication: DRF supports various authentication schemes, such as token-based authentication, session authentication, and third-party packages (e.g., OAuth). This flexibility ensures secure access to APIs, essential in protecting sensitive data and resources.
- View Sets: These are abstractions over the traditional Django views, providing a simple interface to define the logic for different HTTP methods. View sets reduce the amount of boilerplate code required, making it easier to develop and maintain APIs.
DRF's integration with Django's ORM and its powerful, customizable tools for handling web requests make it an ideal choice for developers looking to create professional-grade Web APIs.
Section 3: Setting Up a Django Project with REST API
Creating a REST API in Django involves initial project setup and integrating the Django REST Framework (DRF). Here's a step-by-step guide to getting started:
Project Setup:
- Install Django: Begin by installing Django using the command
pip install django
. - Create a New Project: Use
django-admin startproject your_project_name
to create a new Django project. - Start an App: Inside your project directory, start a new app with
python manage.py startapp your_app_name
.
Integrating Django REST Framework:
- Install DRF: Add DRF to your project by running
pip install djangorestframework
. - Update Settings: In your project's
settings.py
, addrest_framework
to theINSTALLED_APPS
list.
Model Creation and Migration:
- Define a Model: In your app's
models.py
, define a simple model. For example:
python
- Migrate the Model: Run
python manage.py makemigrations
to create migration files for your model, followed bypython manage.py migrate
to apply the migration to the database.
Create a Serializer:
- In a new file
serializers.py
in your app, create a serializer for your model:
python
Build a View:
- In
views.py
, create a view using DRF's viewsets:
python
URL Routing:
- In your project's
urls.py
, set up the URL routing for your API:
python
Following these steps, you'll have a basic Django project with a REST API, capable of handling CRUD operations for your defined model. This setup serves as a foundation for further expansion and customization of your API.
Section 4: Building Your First API
Once your Django project and the Django REST Framework (DRF) are set up, the next step is to create your first API that performs CRUD (Create, Read, Update, Delete) operations. Here’s a guide to building a basic API:
CRUD Operations:
- Create: POST requests allow clients to create a new record in the database.
- Read: GET requests enable clients to read or retrieve data.
- Update: PUT requests are used to update existing data.
- Delete: DELETE requests remove data from the database.
Serializers in DRF:
- Serializers play a crucial role in DRF. They convert complex data types like querysets and model instances into JSON format that can be easily rendered into content types like XML for use in HTTP requests.
- They also provide deserialization, allowing parsed data to be converted back into complex types after validating the incoming data.
Implementing CRUD in Views:
- Using DRF's
viewsets
, you can define the behavior of your CRUD operations. AModelViewSet
automatically provides implementations for basic CRUD actions. - For example, in your
views.py
:
python
URL Routing with Routers:
- DRF routers handle URL routing for your API. The
DefaultRouter
class automatically creates the routes for your API's CRUD operations. - In your
urls.py
:
python
Testing Your API:
- Once you’ve set up the views and routed the URLs, you can test your API using tools like
curl
or Postman. This involves sending different HTTP requests (GET, POST, PUT, DELETE) to your API’s endpoints and observing the responses.
By following these steps, you’ll have a functioning API in Django capable of performing CRUD operations. The serializers handle data conversion and validation, while viewsets and routers streamline the process of defining behavior and routing for your API.
Start Learning Coding today and boost your Career Potential
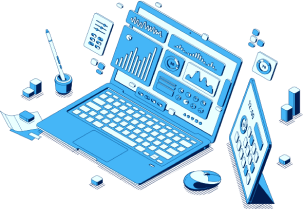
Section 5: Authentication and Permissions
Authentication and permissions are crucial components in the security of REST APIs. They ensure that only authorized users can access certain data and perform specific actions. Django REST Framework (DRF) provides a flexible authentication and permissions system to manage access control efficiently.
Importance of Authentication and Permissions:
- Authentication verifies the identity of a user, while permissions determine the actions that authenticated users are allowed to perform.
- Proper implementation of these components is vital to protect sensitive data and to comply with data privacy regulations.
Authentication Methods in DRF:
- Token Authentication: This method provides a token on user login, which must be included in subsequent API requests. It's simple and effective for single-user scenarios.
- Session Authentication: Utilizes Django's session framework, suitable for web applications where the same system handles both frontend and backend.
- OAuth: A more complex but robust method, often used for third-party integrations, providing tokens with limited access for external applications.
Implementing Authentication:
- To use token authentication, first add
rest_framework.authtoken
in yourINSTALLED_APPS
, and runpython manage.py migrate
to create the token model. - In
settings.py
, define the default authentication classes:
python
- For OAuth, you would typically integrate a third-party package like
django-oauth-toolkit
.
Setting Up Permissions:
- Permissions in DRF are used to grant or deny access to different parts of your API.
- You can set global permissions in
settings.py
or apply them to individual views or viewsets.
Implementing Permission Classes in Views:
- In your viewsets, you can define permission classes to restrict access. For example:
python
By implementing authentication and permissions, you enhance the security of your Django REST API, ensuring that only authorized users can access and manipulate data.
Section 6: Testing and Documentation
Testing and documentation are key aspects of developing robust and maintainable REST APIs. They ensure your API behaves as expected and provide clear guidance for those who use it.
Testing REST APIs
Importance of Testing:
- Testing verifies that your API endpoints perform correctly under various conditions and helps to identify any bugs or security vulnerabilities.
- It's crucial for maintaining code quality, especially when adding new features or refactoring existing code.
Writing Test Cases:
- Django’s
TestCase
class provides a framework for writing tests for your API endpoints. - Create a test file in your application directory, for example,
test_views.py
. - Write test functions to send HTTP requests to your API and assert responses. For example:
python
- Run tests using
python manage.py test
.
Documenting APIs
Importance of Documentation:
- Documentation is essential for API usability and adoption. It provides users with guidelines on how to effectively interact with your API.
Tools for Documentation:
- Swagger: A widely-used tool for documenting APIs. It offers a user-friendly interface and interactive documentation. You can integrate Swagger with Django REST Framework using packages like
drf-yasg
. - DRF’s Built-in Documentation: DRF includes a built-in API documentation feature that automatically generates documentation for your API endpoints. Enable it by adding the following to your
urls.py
:
python
By focusing on thorough testing and comprehensive documentation, you not only ensure the reliability and security of your API but also enhance its accessibility and ease of use for other developers.
Conclusion
Throughout this exploration of REST APIs in the Django framework, we've seen how they are not just a feature but a cornerstone of modern web development. Django, with its robust and efficient design, combined with the versatility of Django REST Framework (DRF), provides a powerful platform for building high-quality web APIs.
The importance of REST APIs in Django cannot be overstated. They enable developers to create scalable, maintainable, and secure web applications that can interact seamlessly with various clients, including web browsers and mobile devices. By leveraging Django's capabilities and DRF's extended toolkit, developers can build APIs that are not only functional but also adhere to best practices.
As you embark on your journey with Django and DRF, remember that the learning process is continuous and experimental. The flexibility of Django and the extensibility of DRF mean that there are always new techniques to discover and best practices to implement. The community around these technologies is vibrant and supportive, offering a wealth of resources for further learning and exploration.
Whether you're a beginner just starting out or an experienced developer looking to enhance your skills, the world of Django and DRF offers endless possibilities. Embrace the challenge, experiment with new ideas, and continue to expand your knowledge. By doing so, you'll be well-equipped to contribute to the ever-evolving landscape of web development with Django and REST APIs.
Cursos relacionados
Ver Todos los CursosAvanzado
Django REST Framework
The Django REST Framework course opens the gateway to the world of web interface development, where you'll learn to create connections between servers and clients through APIs. As you delve into the technical details, you'll master how to transform complex data structures into network-friendly formats and vice versa. You'll understand how to efficiently handle user requests and return responses in a format that seamlessly integrates into web applications.
Avanzado
Django ORM Ninja: Advanced Techniques for Developers
Learn how to build applications using the Django ORM framework. This is the next step in expanding your knowledge of Django development.
Intermedio
Django: Build Your First Website
This exciting course is designed for those who aspire to learn web development and create their own website using the powerful Django framework with the Python programming language. From the basics to advanced functionalities, the course offers everything you need to successfully launch your first web project.
The 80 Top Java Interview Questions and Answers
Key Points to Consider When Preparing for an Interview
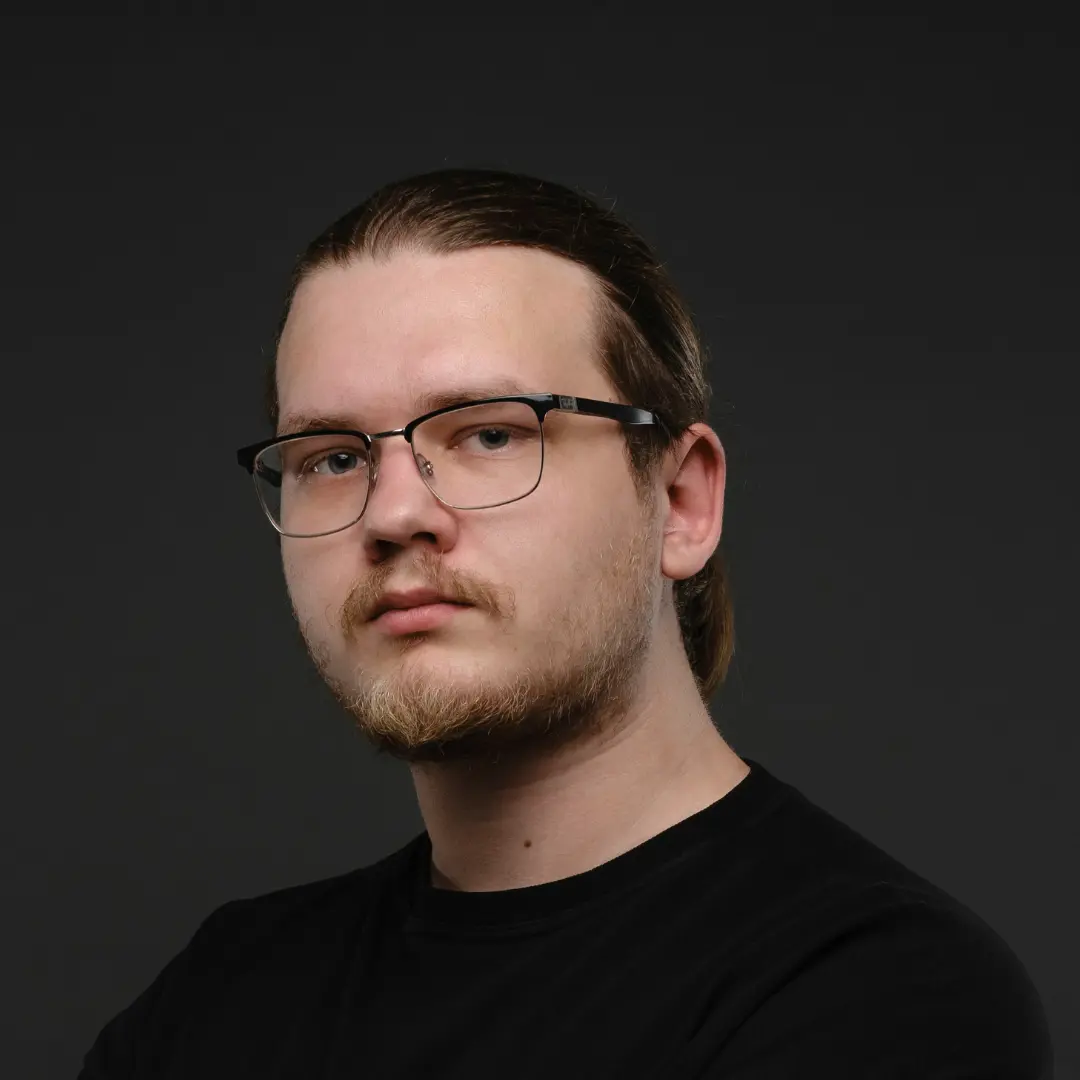
by Daniil Lypenets
Full Stack Developer
Apr, 2024・30 min read
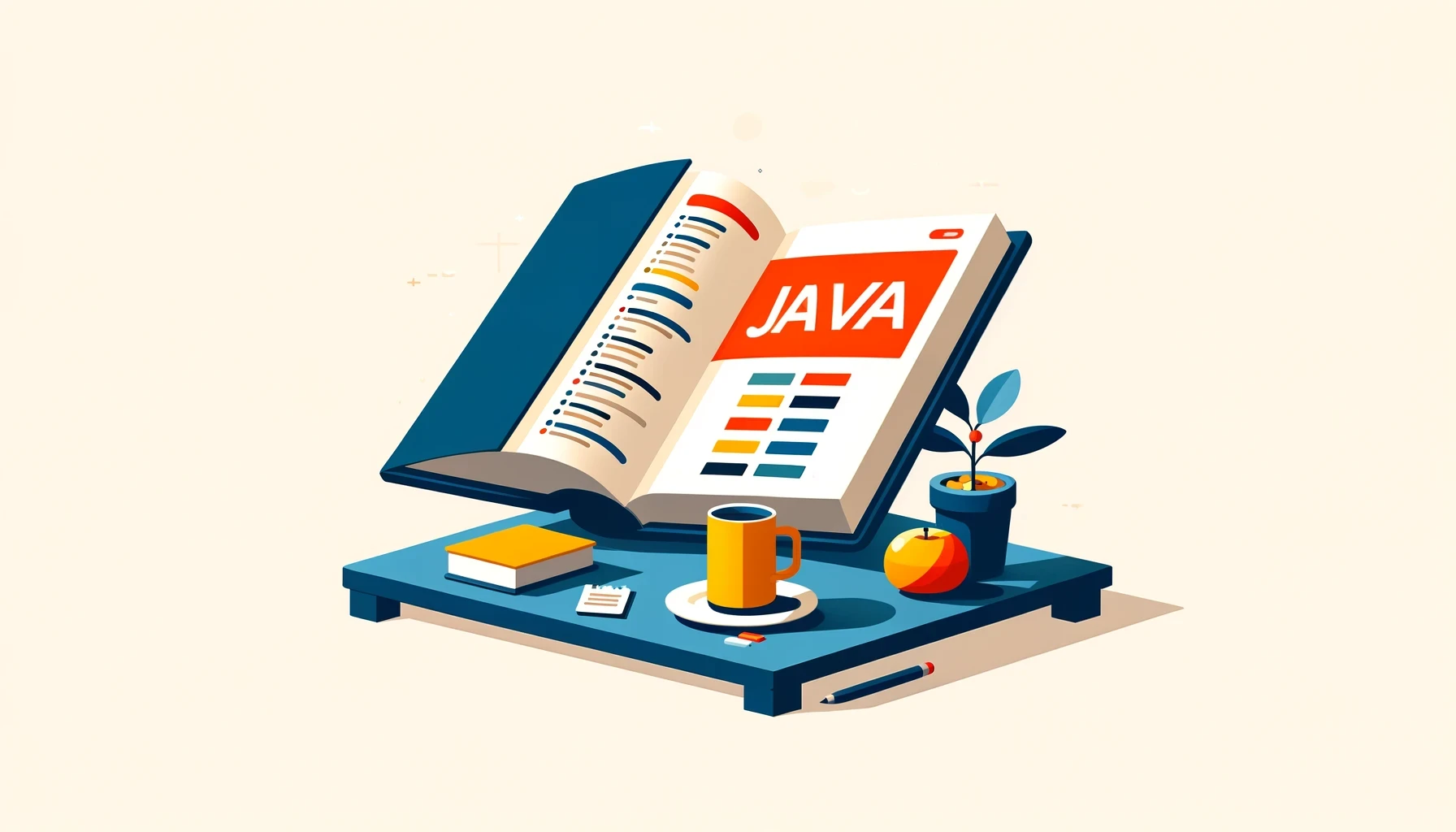
The 50 Top SQL Interview Questions and Answers
For Junior and Middle Developers
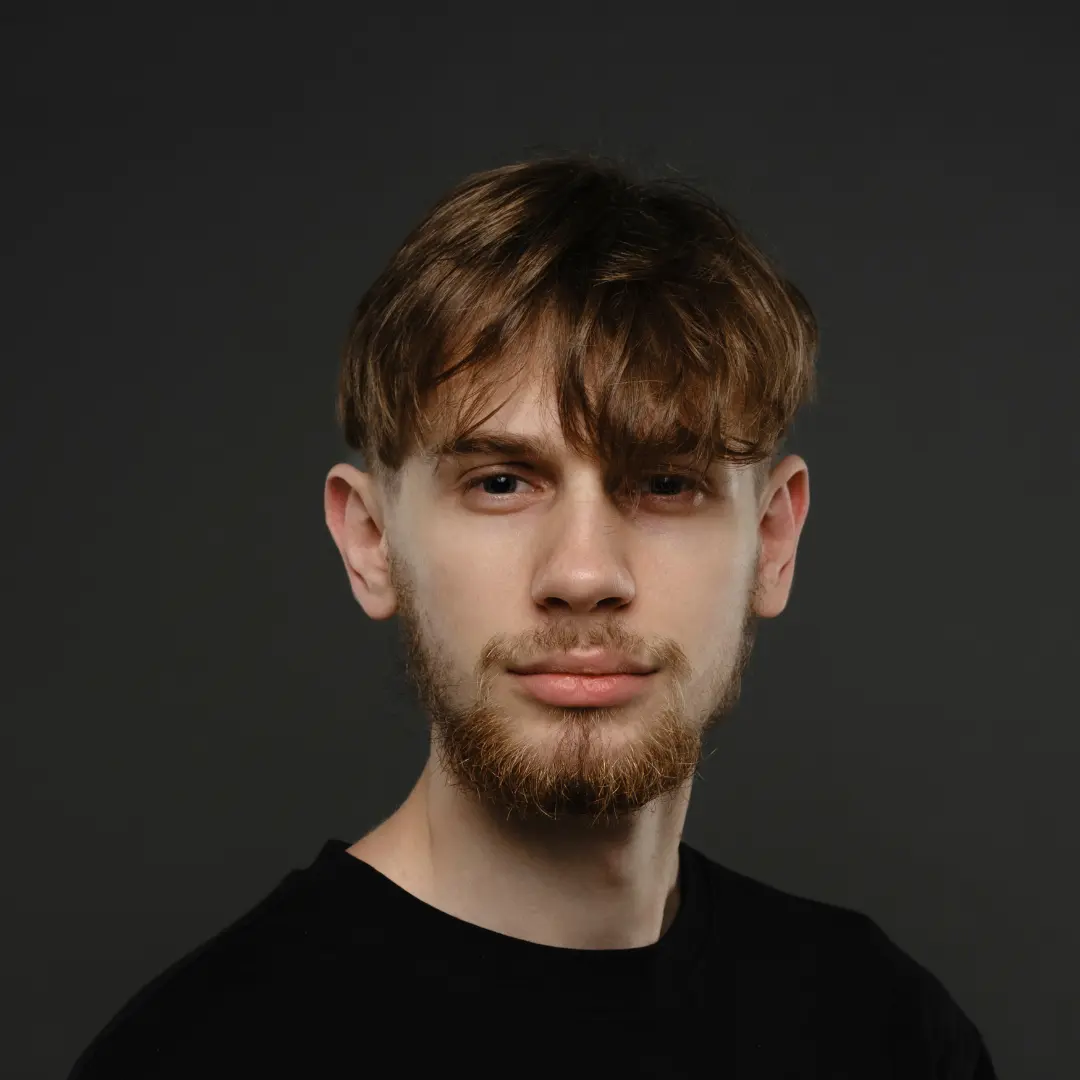
by Oleh Lohvyn
Backend Developer
Apr, 2024・31 min read
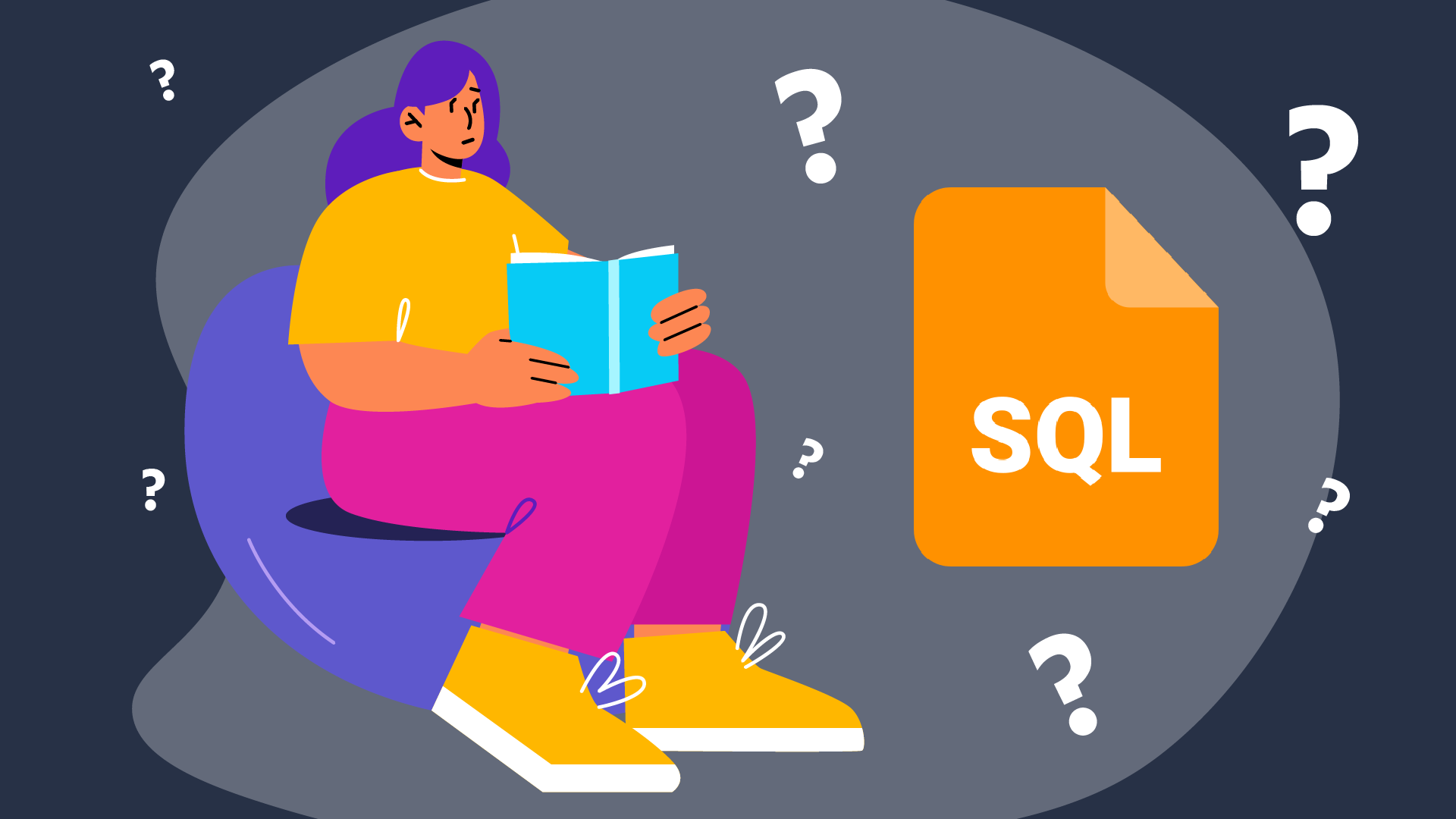
The SOLID Principles in Software Development
The SOLID Principles Overview
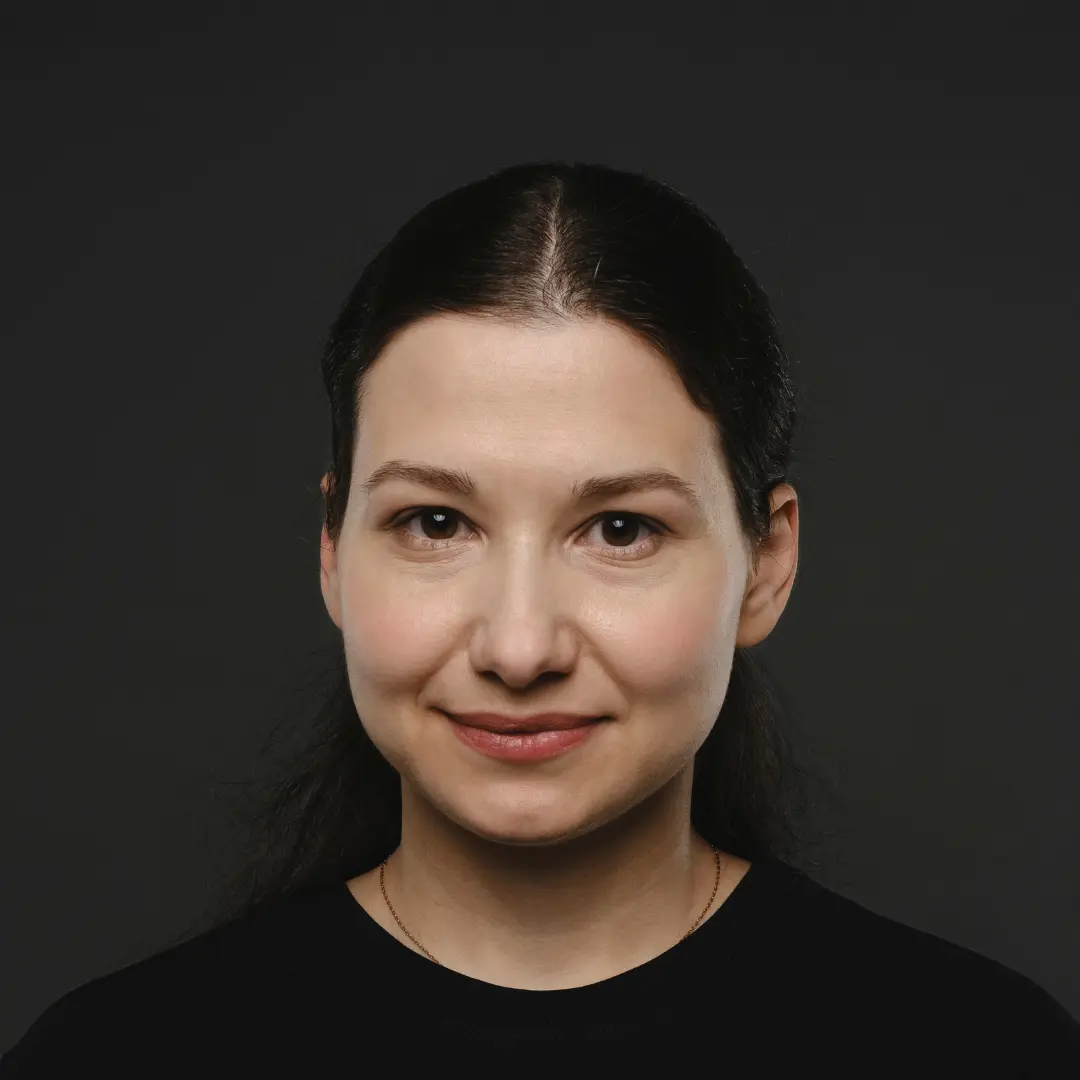
by Anastasiia Tsurkan
Backend Developer
Nov, 2023・8 min read
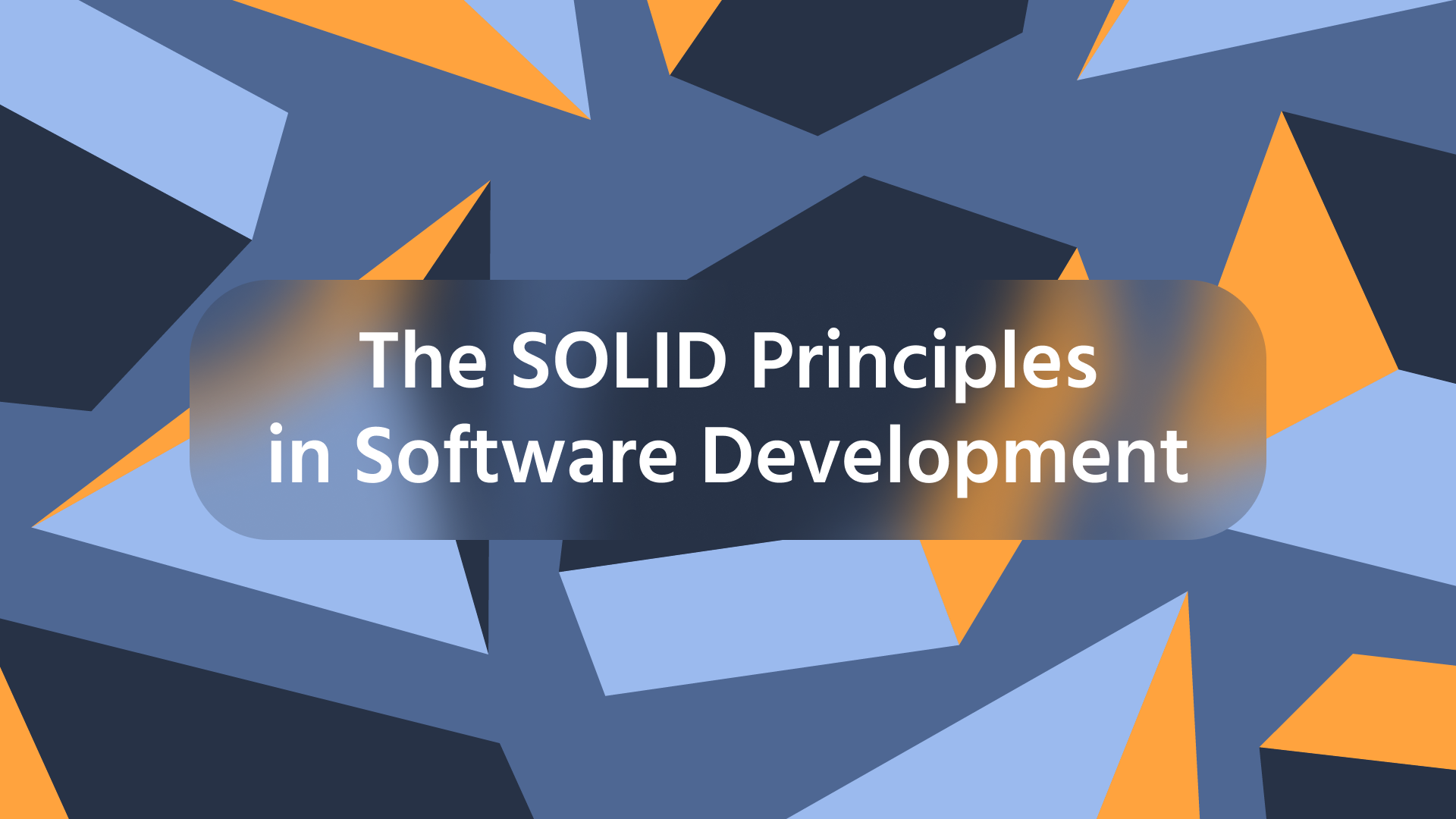
Contenido de este artículo