Cursos relacionados
Ver Todos los CursosAvanzado
Java OOP
Those who know OOP can program well. That's what many programmers say. Get ready for an important part of your Java learning journey, mastering which will greatly boost your programming skills in general. You will learn how to effectively use the Java development environment, the principles of Object-Oriented Programming (OOP), and best practices in OOP. You will learn to make your code flexible and deepen your knowledge of previously covered topics. Let's get started!
Intermedio
C++ OOP
Welcome to the exciting world of Object-Oriented Programming (OOP) with C++! This course will provide a comprehensive understanding the fundamentals of OOP and how they are implemented. Our focus will be on key concepts such as classes, objects, inheritance, polymorphism and encapsulation. Let's Start the Journey!
Intermedio
Structuring Applications Using OOP Principles
This is a hands-on course that will help you understand the basics of object-oriented programming in Python. You will learn how to create classes, work with objects, use inheritance, and extend the capabilities of your programs.
Object-Oriented Programming (OOP)
Why Do We Need OOP?
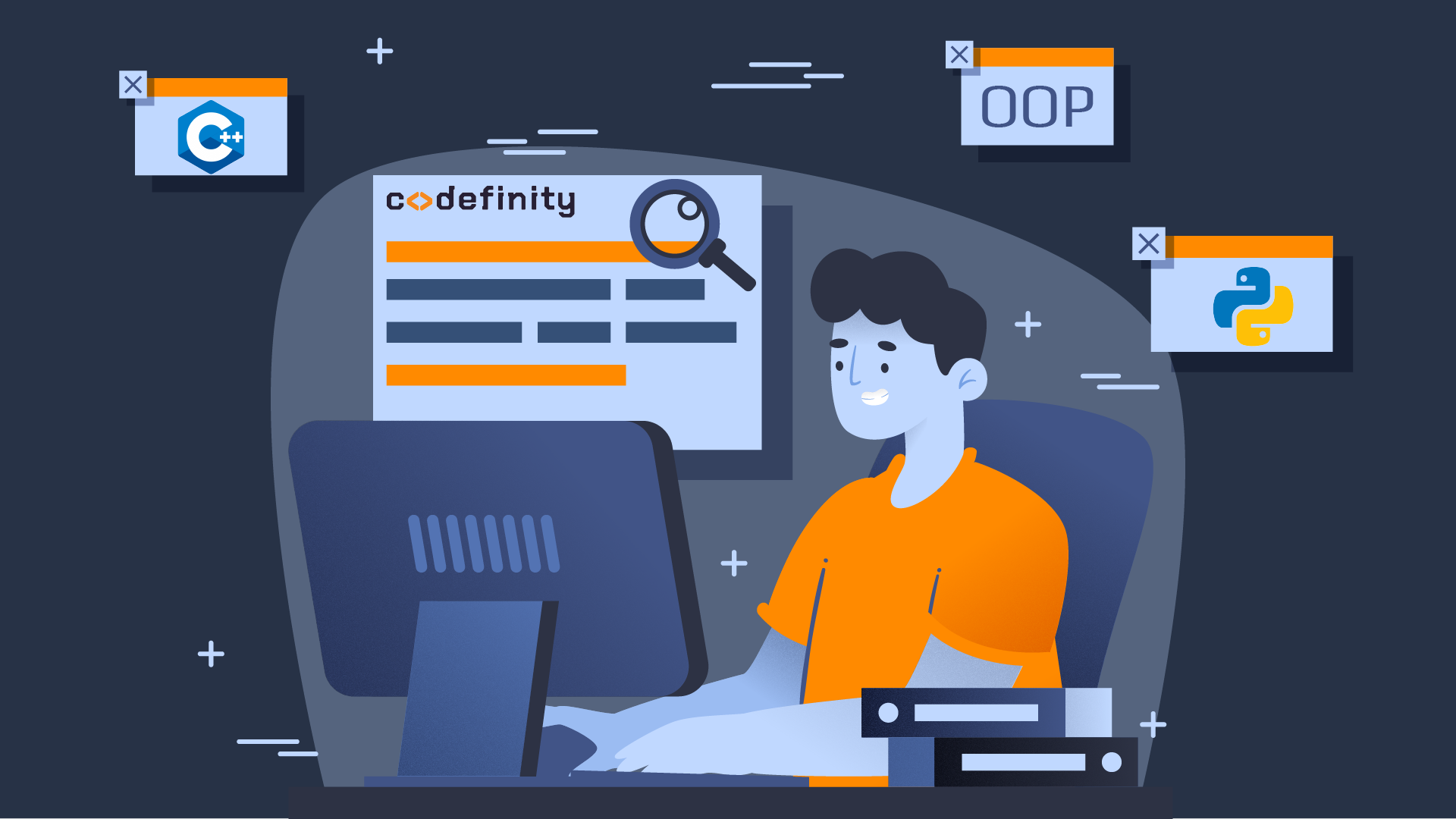
Why Do We Need OOP?
Imagine you're building a house without a plan—just stacking bricks chaotically without paying attention to how they fit together. At first, things might seem fine, but the more bricks you add, the harder it gets to understand what's going on and how it's all holding up. This is what programming looks like without any clear structure or approach. Every piece of code becomes independent and uncontrollable, and small changes start causing big problems.
This is where Object-Oriented Programming (OOP) comes in. OOP is like having a well-organized construction plan where every element (object) has its place, purpose, and clear interaction with others. It allows developers to build organized, maintainable structures where each piece of code does a specific job and can easily adapt to changes.
Compared to procedural programming, which often looks like a long stream of commands, OOP breaks the code into separate objects, each with its own properties and actions. This not only simplifies development but also makes it easier to maintain and extend projects over time.
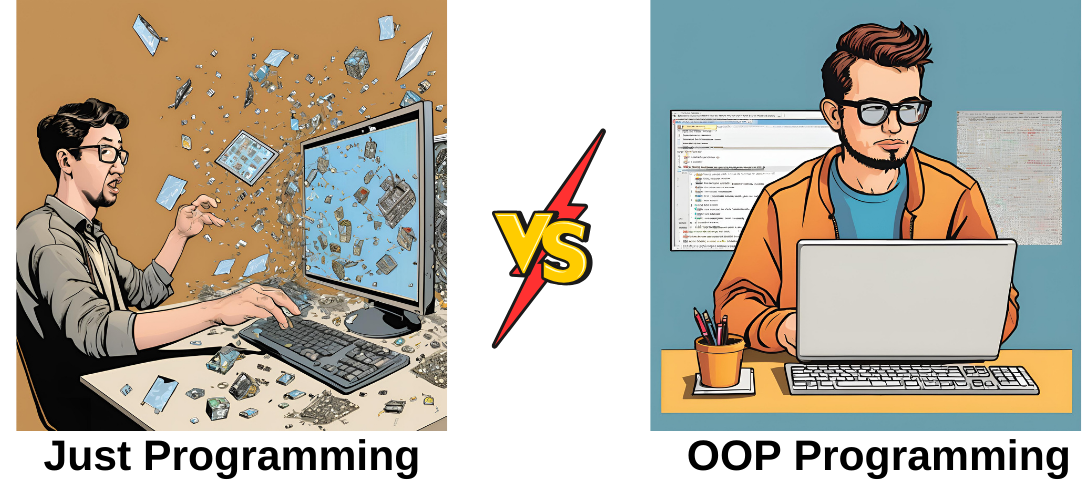
OOP helps prevent the situation where code becomes a mess—hard to manage and even harder to understand. Every object in OOP has a clear role and is responsible for its own actions. It’s important to realize that a chaotic approach to programming may work at the start, but as your project grows, it turns into a nightmare. OOP solves this problem by bringing order and structure to your code, making it more readable and manageable.
Core Principles of OOP
Object-Oriented Programming (OOP) is built on several fundamental principles that help create clean, modular, and maintainable code. In this section, we will explore these core principles and explain why they are crucial for effective programming.
Encapsulation
Encapsulation is the process of hiding the internal state of an object and only allowing access to it through well-defined methods. Think of an object as a box with a button that controls access to its contents. You can press the button to interact with the contents, but you can't directly manipulate what's inside the box. This ensures that data is protected and can only be modified in controlled ways.
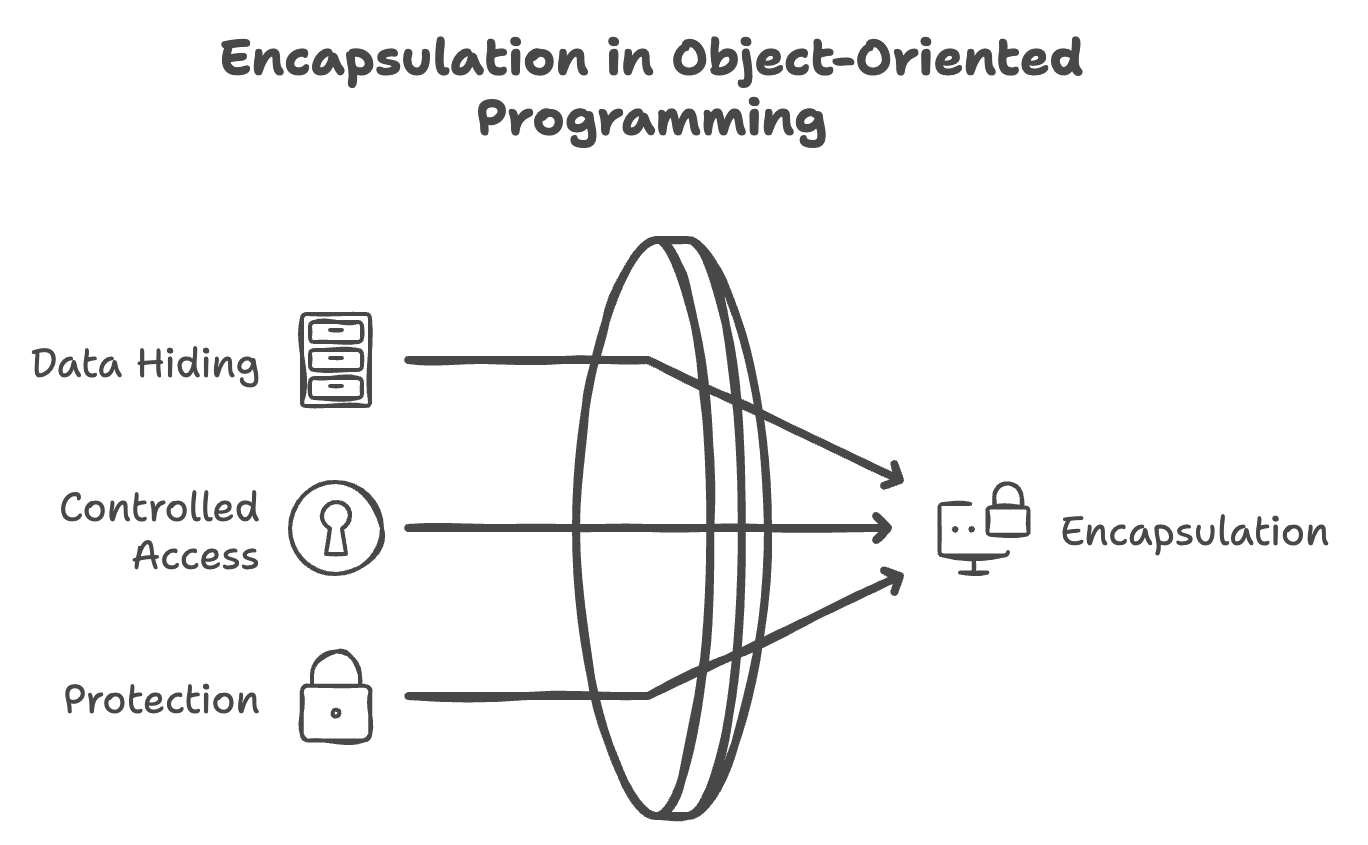
Run Code from Your Browser - No Installation Required
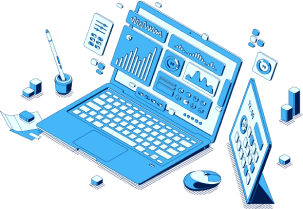
Inheritance
Inheritance allows you to create new classes based on existing ones. The new class (child) inherits all properties and methods from the parent class but can also add its own. This helps reduce code duplication and makes extending the code easier.
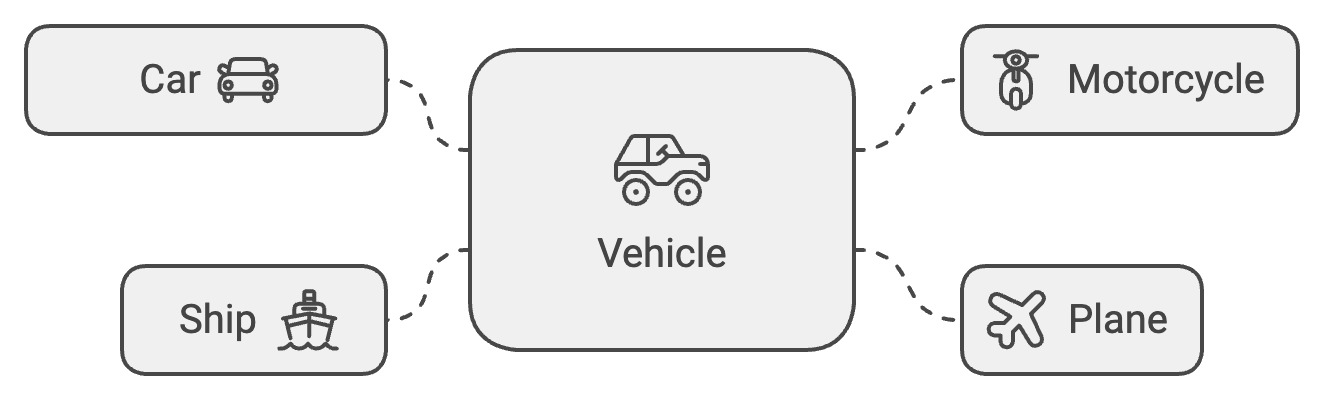
Polymorphism
Polymorphism allows objects of different classes to be treated as objects of a common superclass. It enables methods to do different things based on the object they are acting upon. It’s like a universal remote control where one button can perform different functions depending on which device you're using.
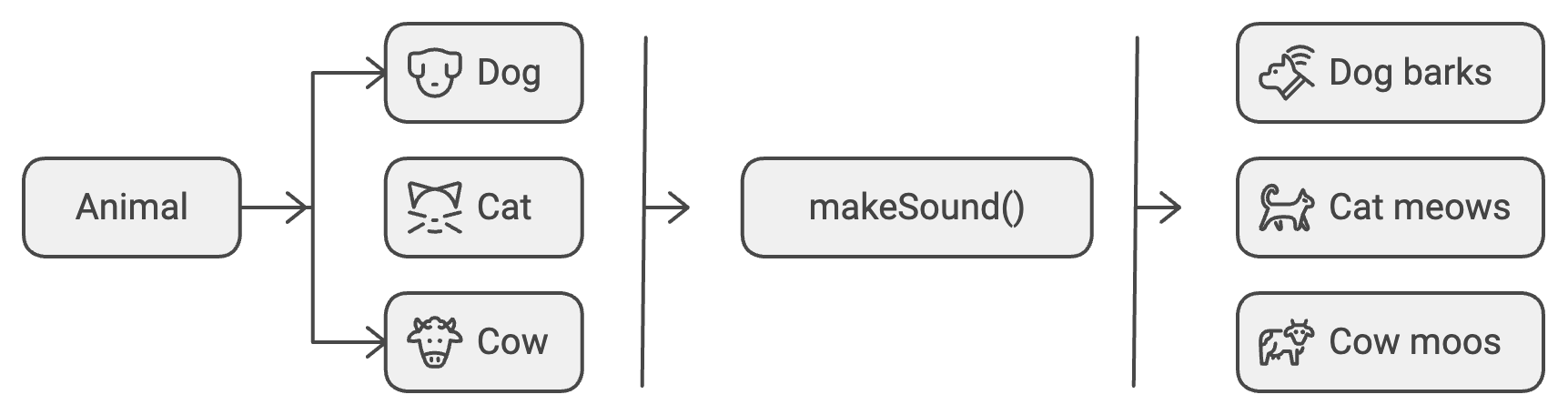
Abstraction
Abstraction hides the complex implementation details and provides a simple interface. It’s like driving a car, where you only need to interact with the steering wheel and pedals, without needing to understand how the engine works.
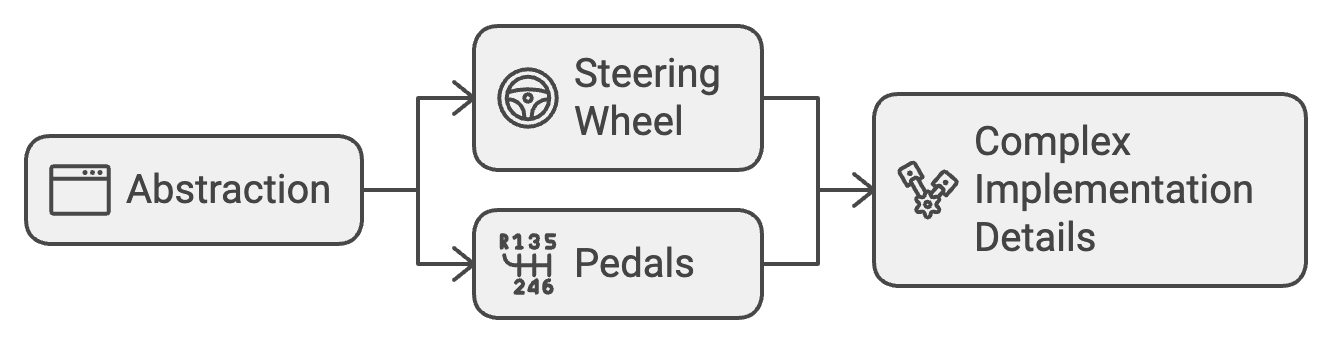
Each of these principles helps make code more organized, understandable, and easier to maintain. Encapsulation controls data access, inheritance avoids duplication, polymorphism provides flexibility, and abstraction simplifies complexity. Understanding and applying these principles are key to successfully using OOP in your projects.
Classes and Objects
In object-oriented programming (OOP), classes and objects are the fundamental building blocks. They help in organizing and structuring code to make it more modular, reusable, and easier to understand. Let’s delve into the definition of classes and objects, explore examples in different programming languages, and understand the core concepts associated with them.
Definition of Classes and Objects
A class is a blueprint or template for creating objects. It defines a type of object according to the data and methods it should have. An object is an instance of a class. It encapsulates data (attributes) and methods that operate on the data. Think of a class as a blueprint for a house, and an object as an actual house built from that blueprint.
Start Learning Coding today and boost your Career Potential
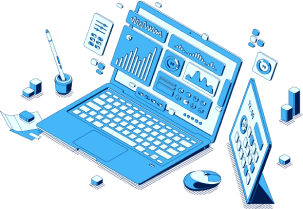
Classes in Different Programming Languages
Understanding how classes are defined and used in various languages helps in grasping their versatility and applying them effectively in different development environments. By comparing examples from languages like Python, Java, and C++, we can see how each language approaches the creation and utilization of classes, showcasing both the commonalities and unique features of their class systems. This comparison provides a deeper insight into the practical applications of classes and their role in designing robust and maintainable software.
Python
In Python, classes are defined using the class
keyword. Here’s a simple example:
In this example:
__init__
is the constructor that initializes the object's attributes;bark
is a method that defines behavior;name
andage
are attributes of theDog
class.
Java
In Java, classes are defined with the class
keyword, and constructors are defined similarly:
In this example:
Dog
is a class with a constructor to initializename
andage
;bark
is a method;name
andage
are attributes.
C++
In C++, classes are also defined with the class
keyword:
In this example:
Dog
is a class with a constructor and a method;name
andage
are attributes.
Syntax and Concepts
Here are the definitions of key terms:
Constructor
A special method that is automatically called when an object is created. It initializes the object’s attributes.
Method
A function defined within a class that operates on the class’s attributes. Methods define the behavior of the objects created from the class.
Attributes
Variables that hold data for the objects created from a class. They represent the state of the object.
Advantages of OOP in Software Development
Object-oriented programming (OOP) offers several significant advantages that enhance the development and maintenance of software. These benefits stem from OOP’s core principles, such as encapsulation, inheritance, and polymorphism, which contribute to more modular, reusable, and manageable code.
One key advantage of OOP is reducing code duplication. By leveraging inheritance and polymorphism, developers can create reusable components and extend existing classes rather than writing new code from scratch. This not only saves time but also helps maintain consistency and reduces the likelihood of errors.
Another important benefit is easier maintenance and scalability. OOP’s modular structure allows for easier updates and modifications. Since classes encapsulate data and behaviors, changes to one part of the system are less likely to affect other parts, making it simpler to adapt and expand the software as requirements evolve.
To visually summarize these benefits, consider a mind map illustration. This map would highlight key advantages of OOP, such as reduced code duplication, easier maintenance, and improved scalability. By mapping out these benefits, the mind map provides a clear and memorable overview of how OOP enhances software development.
In practical terms, OOP is widely used in various real-world projects. For instance, frameworks like Django and Spring leverage OOP principles to structure complex systems efficiently. A detailed infographic or schematic can illustrate how OOP is applied in these frameworks, showing their architectural design and how they utilize OOP concepts to manage and organize code effectively. This visual representation not only demonstrates the practical application of OOP but also highlights its role in building robust, scalable systems.
By understanding these advantages and seeing how OOP is used in real-world scenarios, developers can better appreciate the value of OOP principles and apply them effectively in their own projects.
FAQs
Q: What is object-oriented programming (OOP)?
A: Object-oriented programming (OOP) is a programming paradigm based on the concept of "objects," which combine data and methods. It promotes organizing code into reusable classes, enhancing modularity, maintainability, and scalability.
Q: What are the main principles of OOP?
A: The main principles of OOP are encapsulation, inheritance, polymorphism, and abstraction. Encapsulation involves bundling data and methods within a class. Inheritance allows a class to inherit attributes and methods from another class. Polymorphism enables objects to be treated as instances of their parent class, and abstraction hides complex implementation details, showing only essential features.
Q: How does OOP reduce code duplication?
A: OOP reduces code duplication through inheritance and polymorphism. Inheritance allows classes to reuse code from existing classes, while polymorphism enables the use of methods in a generalized way, reducing the need to duplicate code for similar operations.
Q: Why is OOP considered easier to maintain?
A: OOP is easier to maintain because of its modular structure. Changes to a class are less likely to affect other parts of the system, thanks to encapsulation. This modular approach makes it simpler to update and extend code without introducing errors elsewhere in the program.
Q: Can you provide examples of real-world applications of OOP?
A: Yes, real-world applications of OOP include frameworks like Django and Spring. These frameworks use OOP principles to organize and manage complex systems efficiently. For example, Django uses OOP to structure its components and manage data models, while Spring leverages OOP for creating scalable enterprise applications.
Q: How can I visualize the advantages of OOP?
A: The advantages of OOP can be visualized using a mind map that highlights key benefits such as reduced code duplication, easier maintenance, and improved scalability. Additionally, infographics or schematics showing the application of OOP in real-world frameworks can help illustrate its practical benefits.
Cursos relacionados
Ver Todos los CursosAvanzado
Java OOP
Those who know OOP can program well. That's what many programmers say. Get ready for an important part of your Java learning journey, mastering which will greatly boost your programming skills in general. You will learn how to effectively use the Java development environment, the principles of Object-Oriented Programming (OOP), and best practices in OOP. You will learn to make your code flexible and deepen your knowledge of previously covered topics. Let's get started!
Intermedio
C++ OOP
Welcome to the exciting world of Object-Oriented Programming (OOP) with C++! This course will provide a comprehensive understanding the fundamentals of OOP and how they are implemented. Our focus will be on key concepts such as classes, objects, inheritance, polymorphism and encapsulation. Let's Start the Journey!
Intermedio
Structuring Applications Using OOP Principles
This is a hands-on course that will help you understand the basics of object-oriented programming in Python. You will learn how to create classes, work with objects, use inheritance, and extend the capabilities of your programs.
The SOLID Principles in Software Development
The SOLID Principles Overview
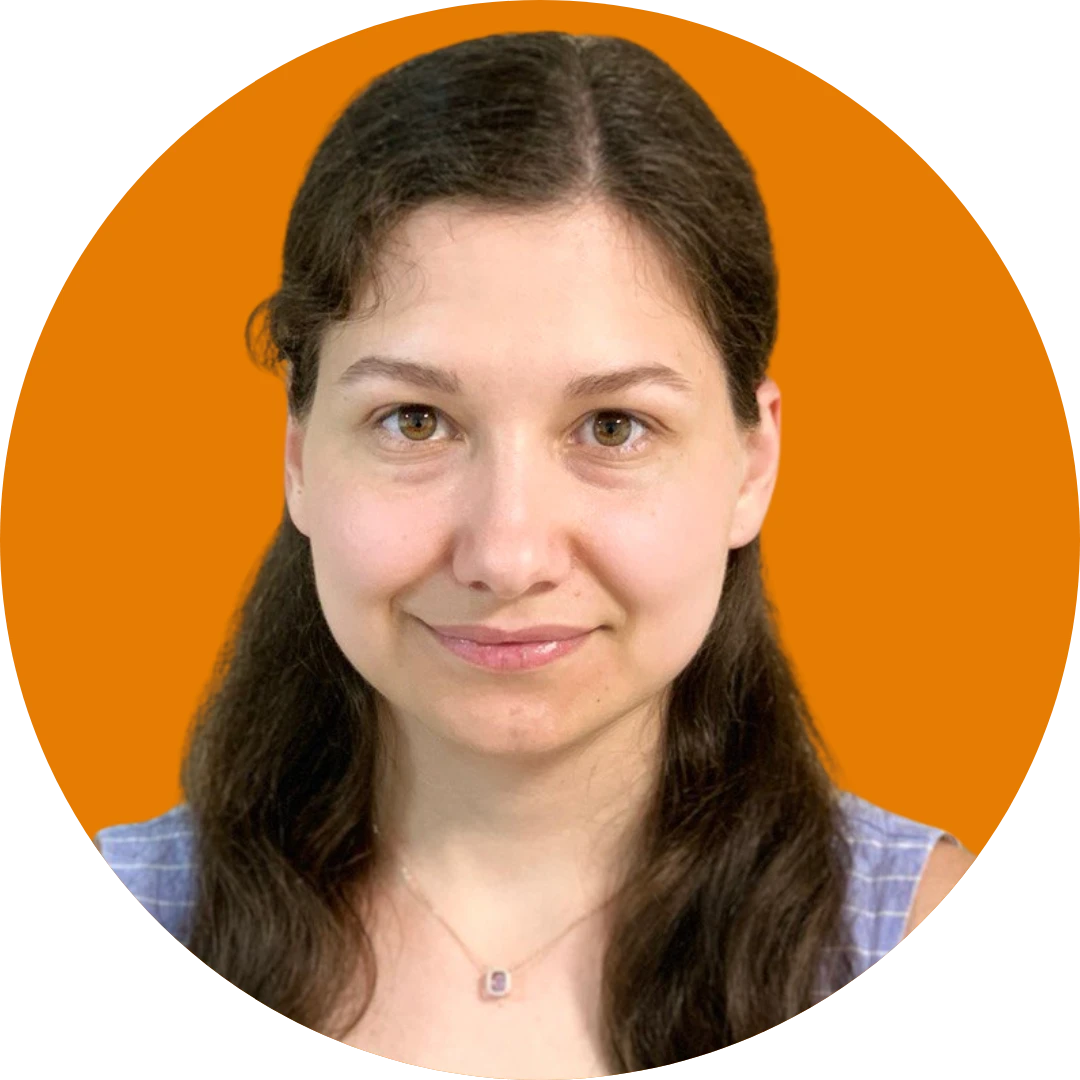
by Anastasiia Tsurkan
Backend Developer
Nov, 2023・8 min read
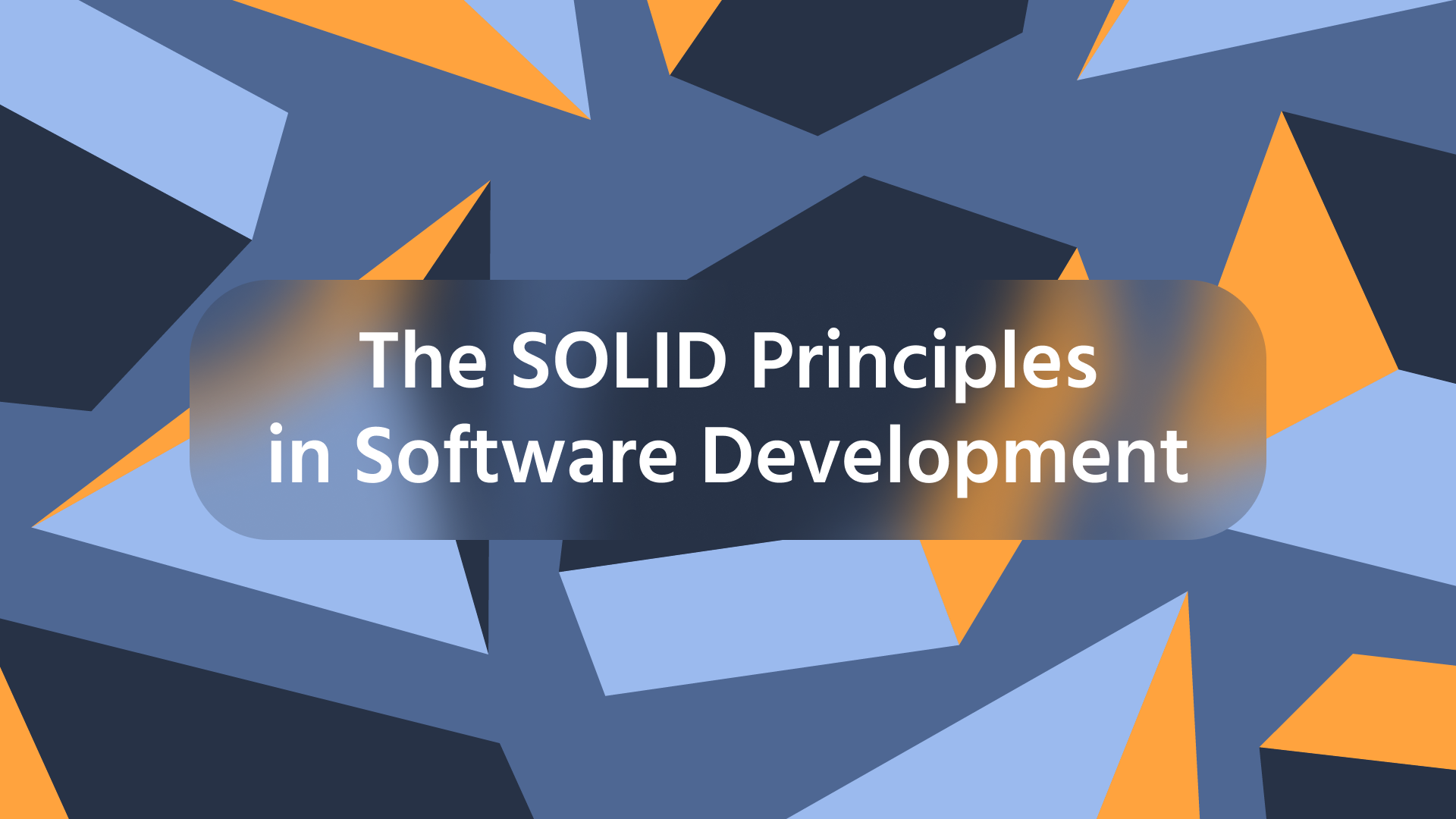
Match-case Operators in Python
Match-case Operators vs if-elif-else statements
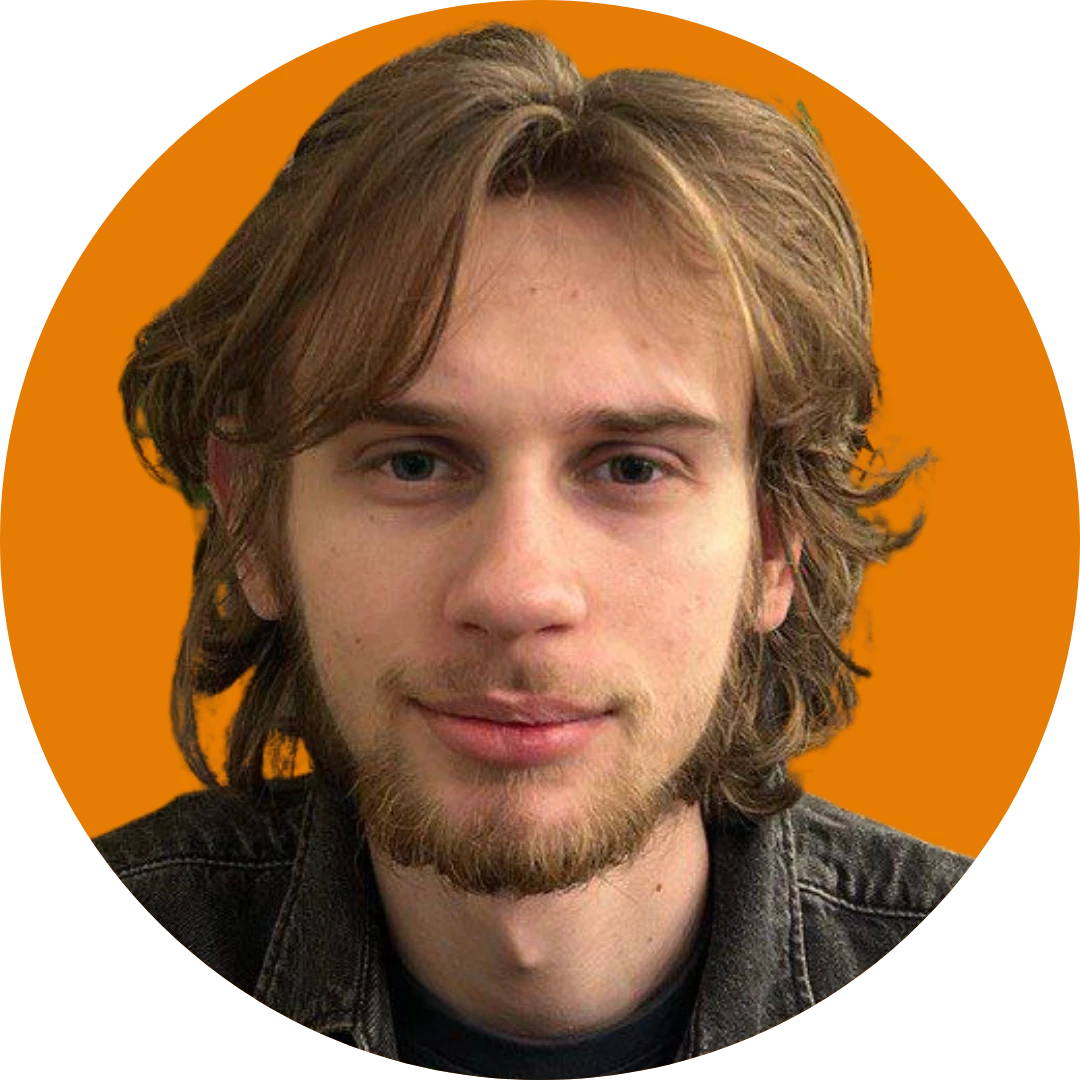
by Oleh Lohvyn
Backend Developer
Dec, 2023・6 min read
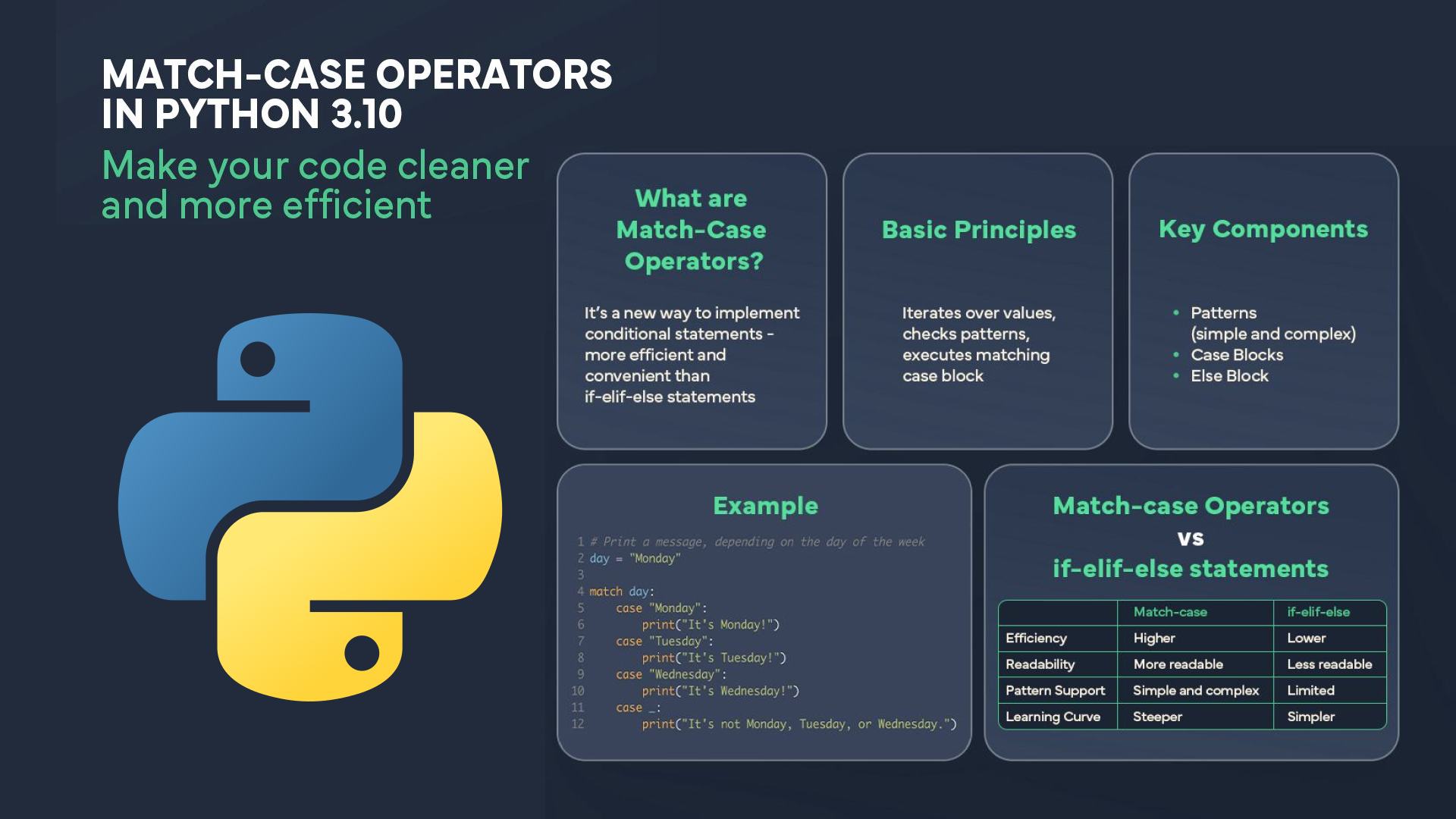
30 Python Project Ideas for Beginners
Python Project Ideas
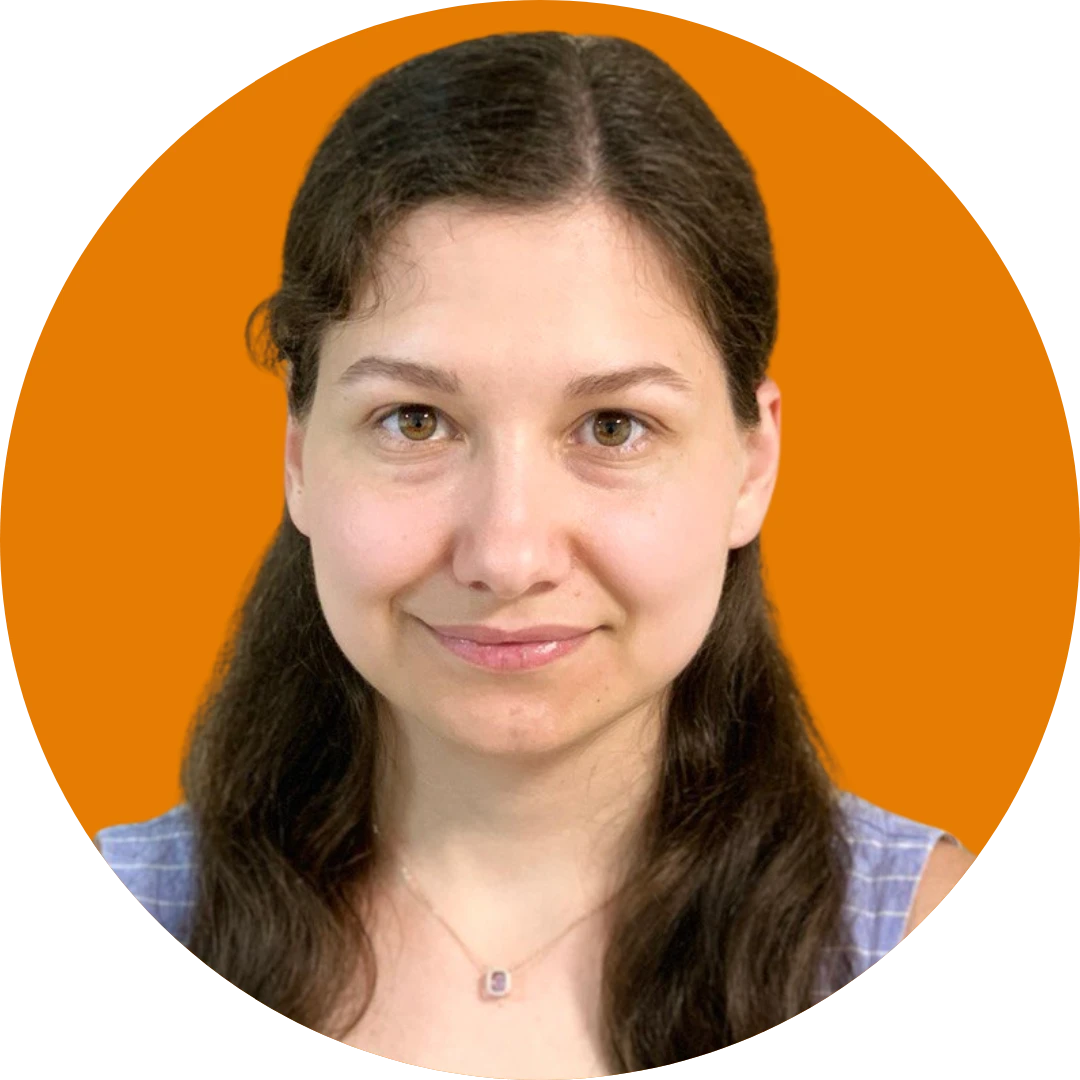
by Anastasiia Tsurkan
Backend Developer
Sep, 2024・14 min read
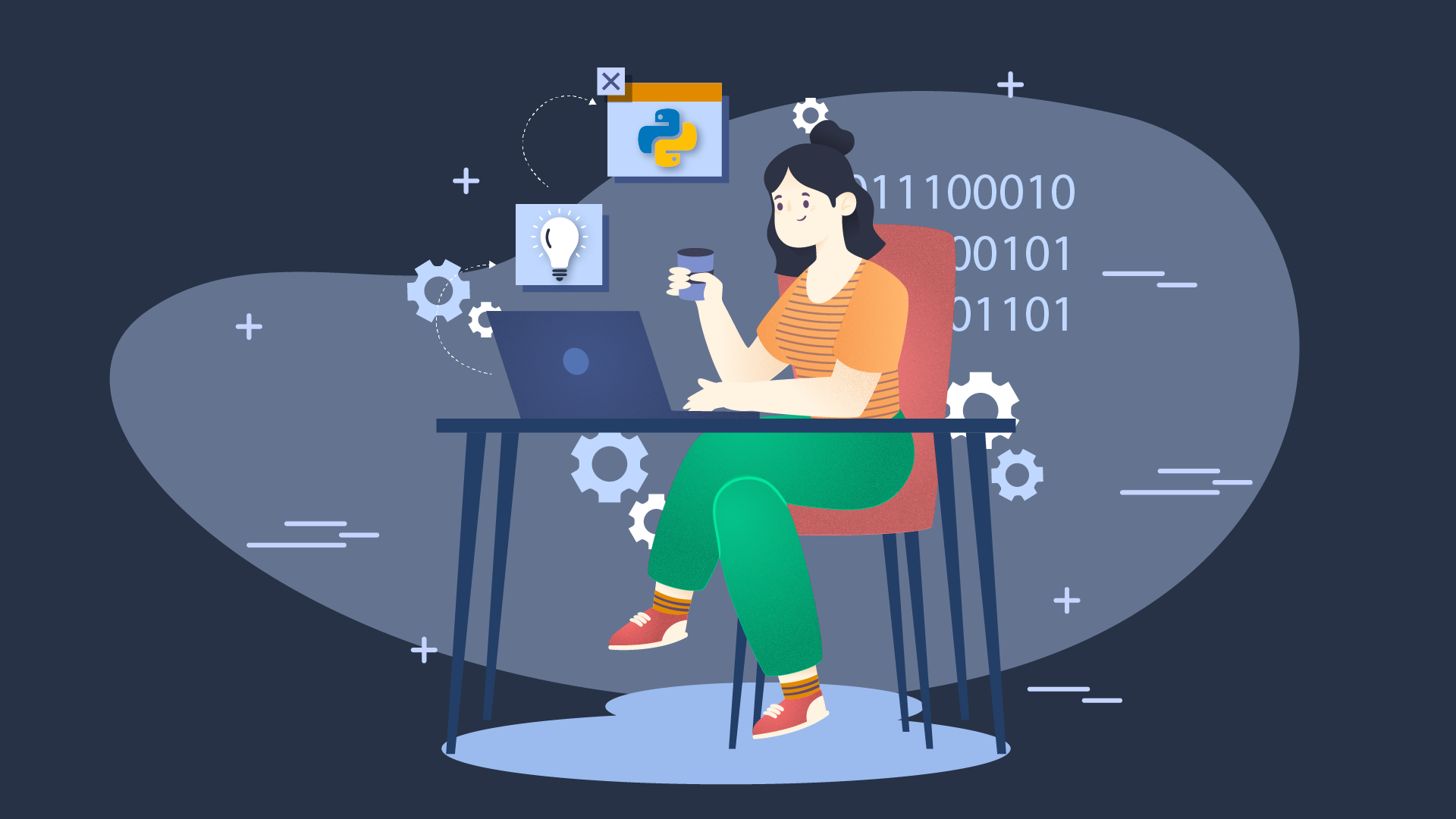
Contenido de este artículo