Cursos relacionados
Ver Todos los CursosUnderstanding Preprocessor Directives in C++
The Cornerstone of Efficient C++ Coding
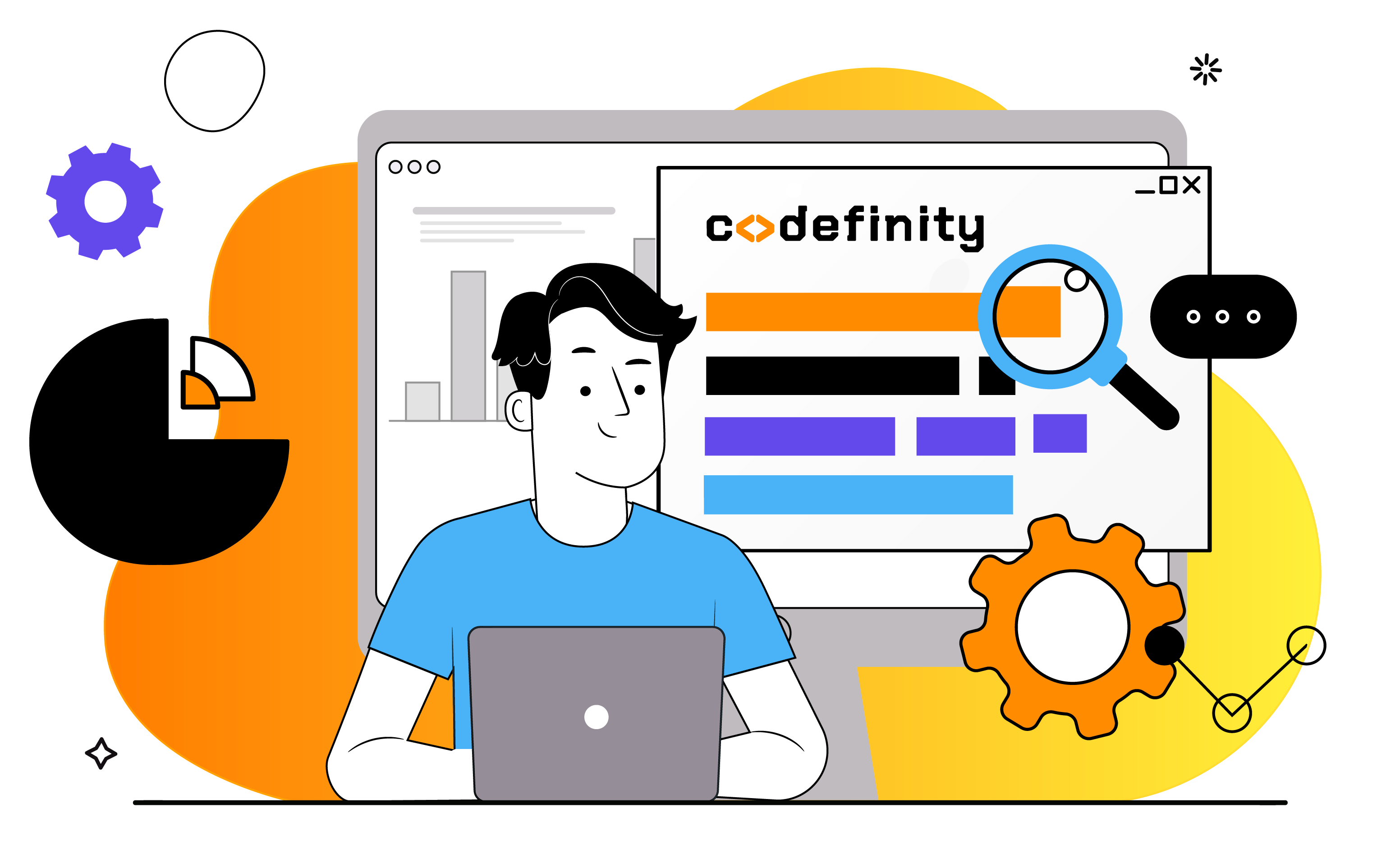
Preprocessor directives are a fundamental aspect of C++ programming, providing a powerful toolset for code optimization and management. These directives, processed before the actual compilation of code, play a critical role in controlling the compilation process, managing dependencies, and optimizing code for different environments.
What are Preprocessor Directives?
Preprocessor directives are commands that are processed by the preprocessor before the actual compilation of the source code. They are used to provide instructions to the preprocessor, which is a tool or component of the compiler responsible for preparing the source code for compilation.
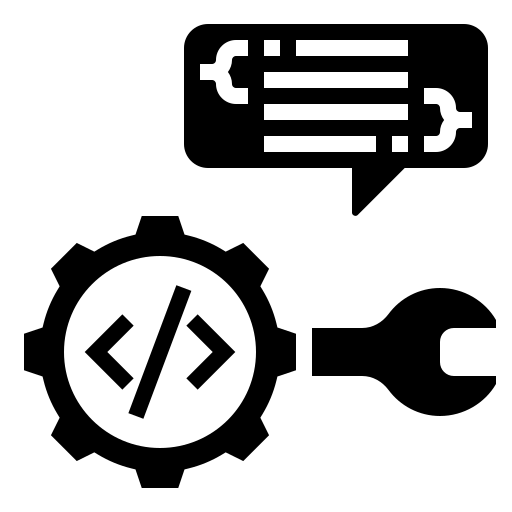
Common Types of Preprocessor Directives
- #include: For including other files.
- #define: For defining macros.
- #if, #ifdef, #ifndef, #else, #elif, #endif: Conditional compilation.
- #pragma: For issuing special commands to the compiler, like disabling warnings.
Run Code from Your Browser - No Installation Required
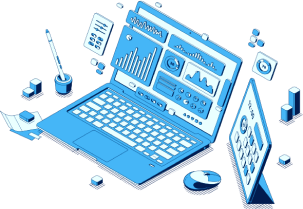
Understanding #include Directive
The #include directive is used to include the contents of a file in the program. It's commonly used to include library headers.
- #include
: For standard library headers. - #include "filename": For user-defined headers.
Header Guards
Header files often contain declarations and definitions that can be included in multiple files. To prevent issues related to multiple inclusion, header guards are commonly used. A header guard typically looks like this:
There is an alternative to traditional header guards. Instead of using the #ifndef, #define, and #endif directives, you can use #pragma once at the beginning of your header file to achieve the same effect.
This directive tells the compiler to include the contents of the file only once during the compilation of a translation unit.
Preprocessor Directives Use Cases
Creating macros
Macros are a way to define constants or functions that are expanded by the preprocessor.
Getting rid of magic numbers
You can use the #define directive to get rid of magic numbers and create constants and make your code easier to understand and cleaner
Conditional Compilation
C++ preprocessor offers conditional directives like #if, #ifdef, and #ifndef to compile code selectively.
- Compile code for specific platforms | - Exclude debug code from release builds |
Start Learning Coding today and boost your Career Potential
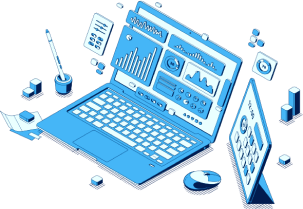
Conclusion
Preprocessor directives are an integral part of C++ programming, offering a range of functionalities from file inclusion to conditional compilation.
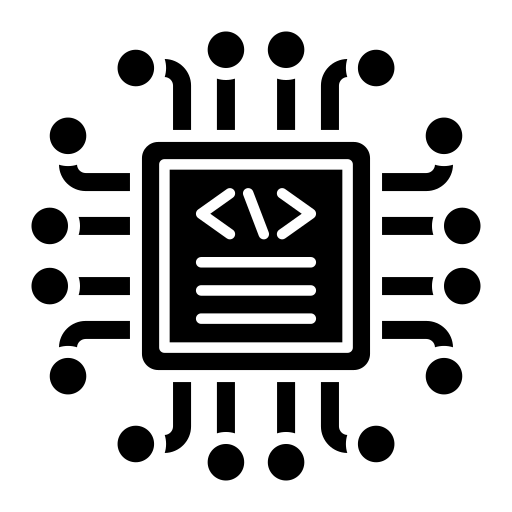
Their proper use can greatly enhance the efficiency, portability, and readability of C++ code. As a C++ programmer, mastering these directives is essential for writing optimized and maintainable code.
FAQs
Q: Are preprocessor directives part of the C++ standard?
A: Yes, they are a standard part of C++ but are handled before the actual compilation.
Q: Can preprocessor directives be nested?
A: Yes, directives like #if can be nested within each other.
Q: Is there a limit to the length of macro definitions?
A: Practically, no. However, excessively long macros can reduce readability and maintainability of code.
Q: How do preprocessor directives affect compilation time?
A: They can both reduce and increase compilation time depending on their usage.
Q: Are there alternatives to macros for constants in modern C++?
A: Yes, constexpr and const are often better alternatives for defining constants in modern C++.
Cursos relacionados
Ver Todos los CursosThe DRY Principle
Maximizing Efficiency in Code and Development
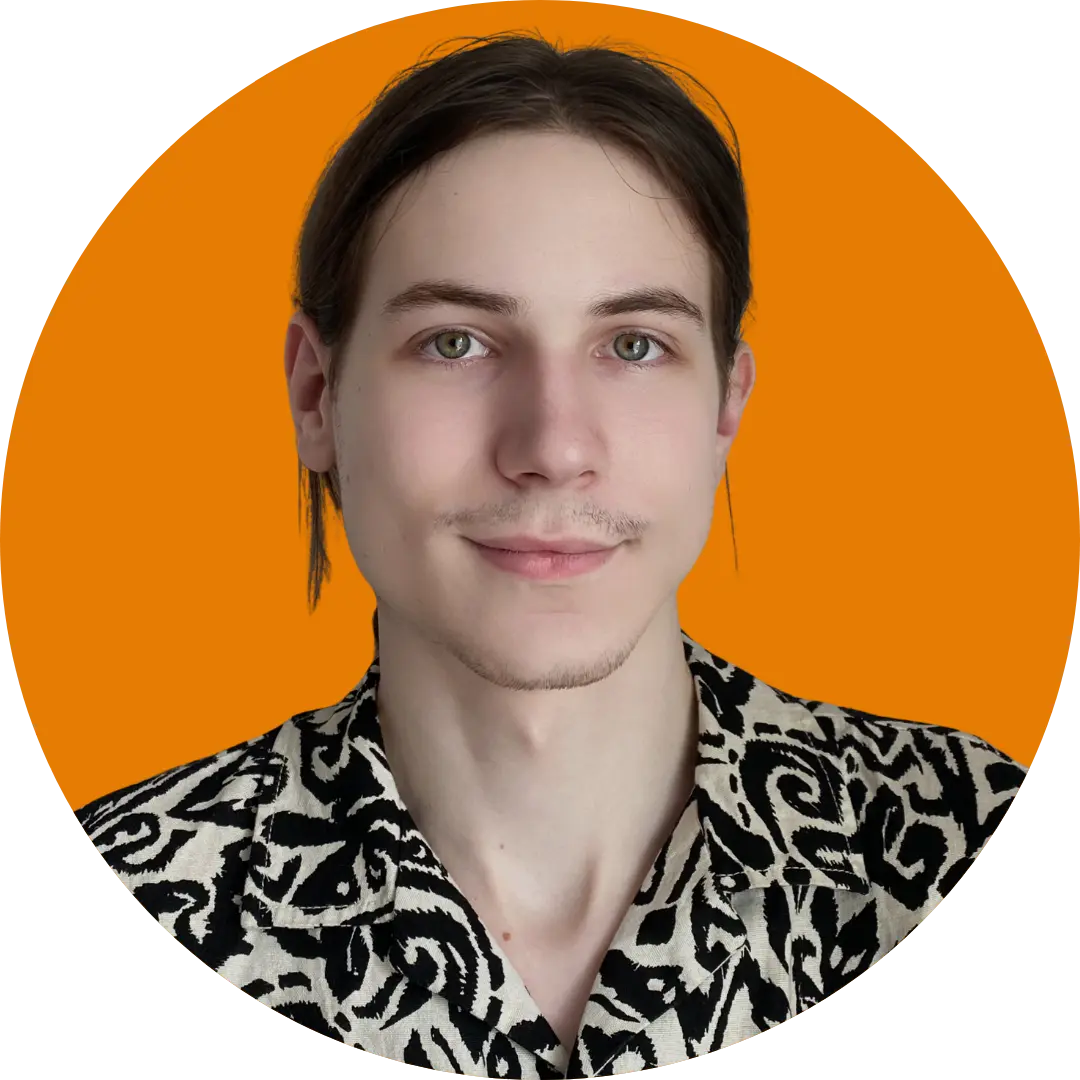
by Ihor Gudzyk
C++ Developer
Aug, 2024・9 min read
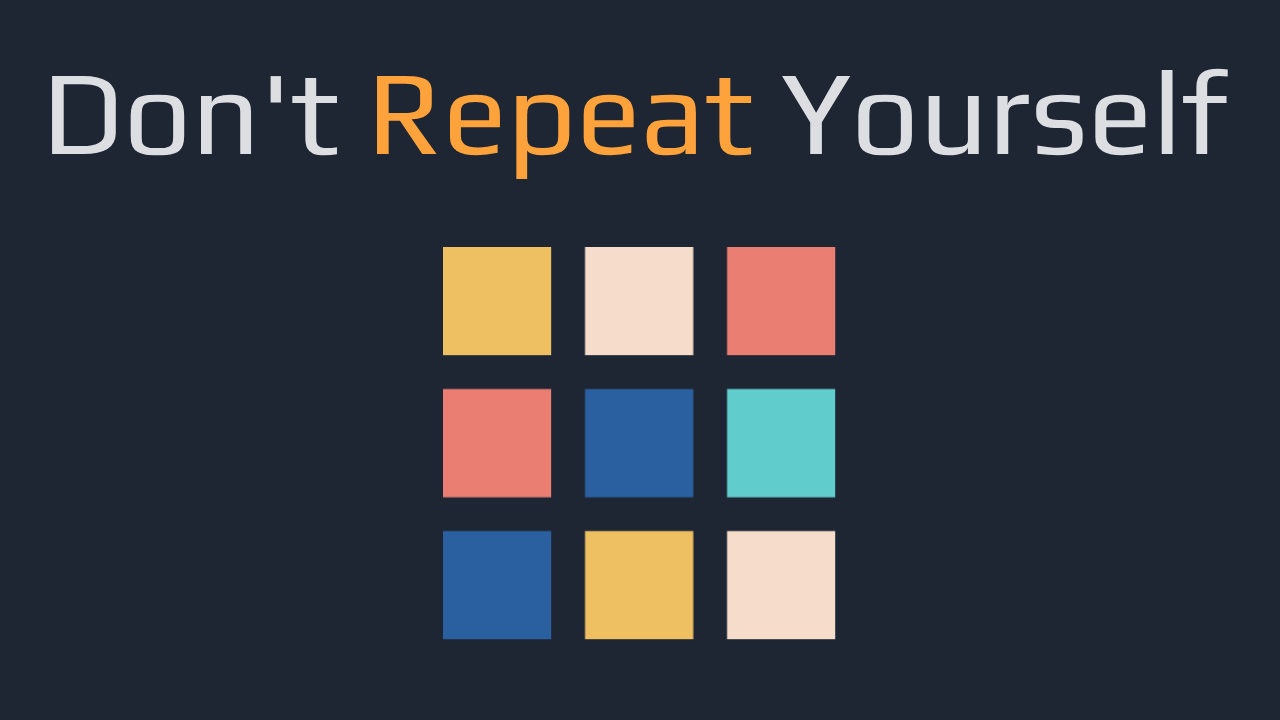
Introduction to Regular Expressions for Beginners
A Guide to Understanding and Using Regex in Programming
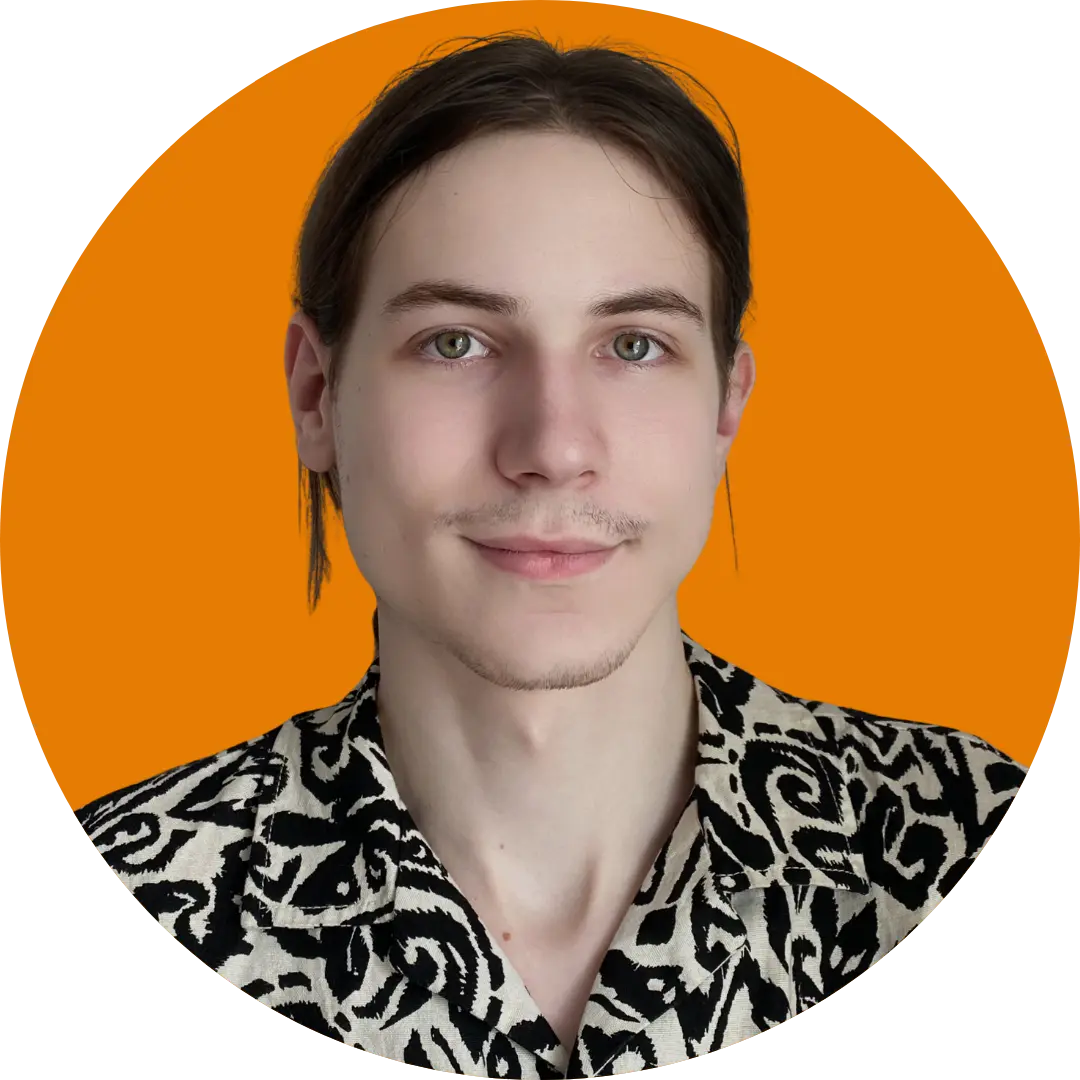
by Ihor Gudzyk
C++ Developer
Sep, 2024・13 min read
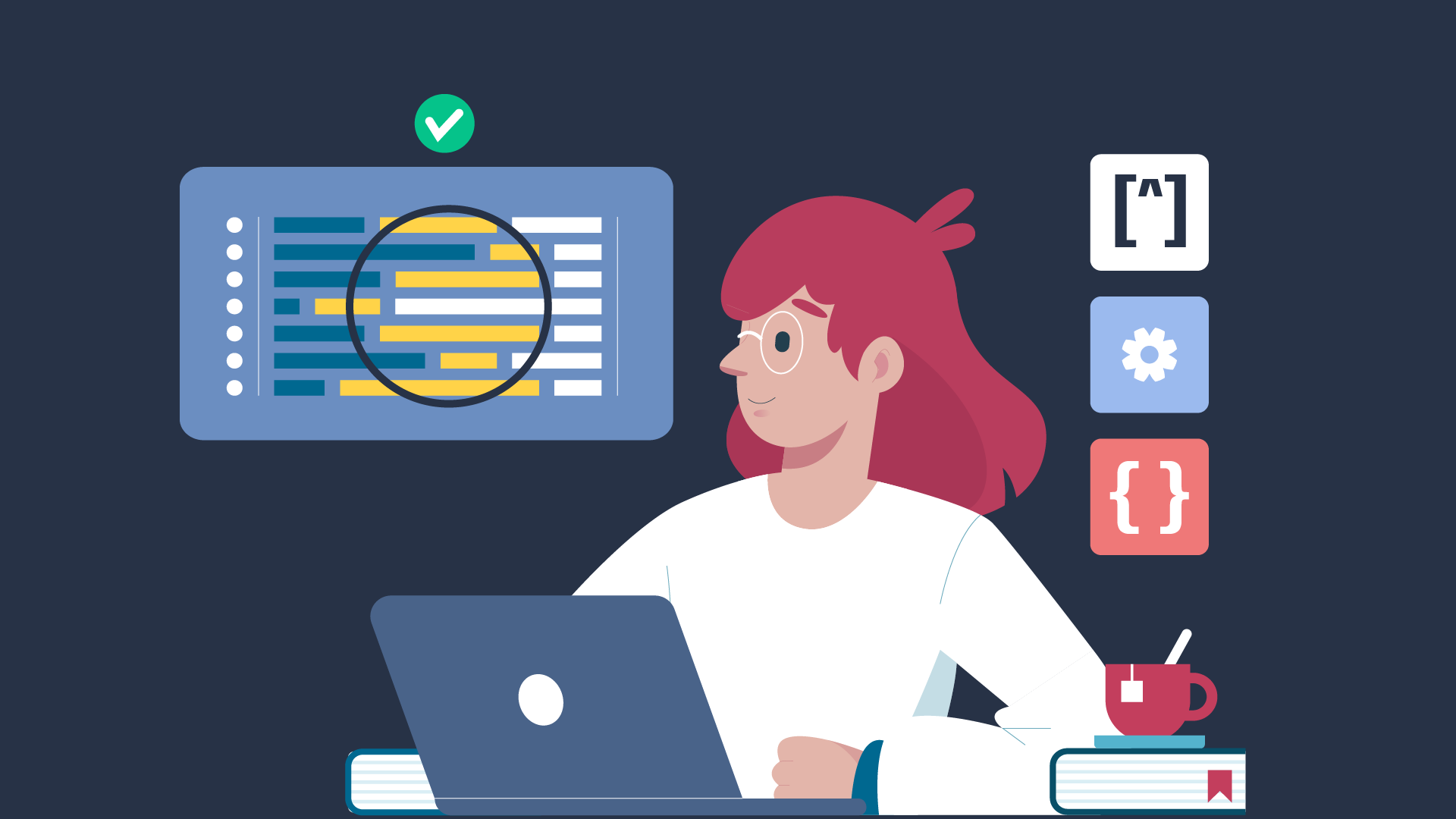
The Role of C++ in Embedded Systems Programming
Unraveling the Power of C++ in the Realm of Microcontrollers and Beyond
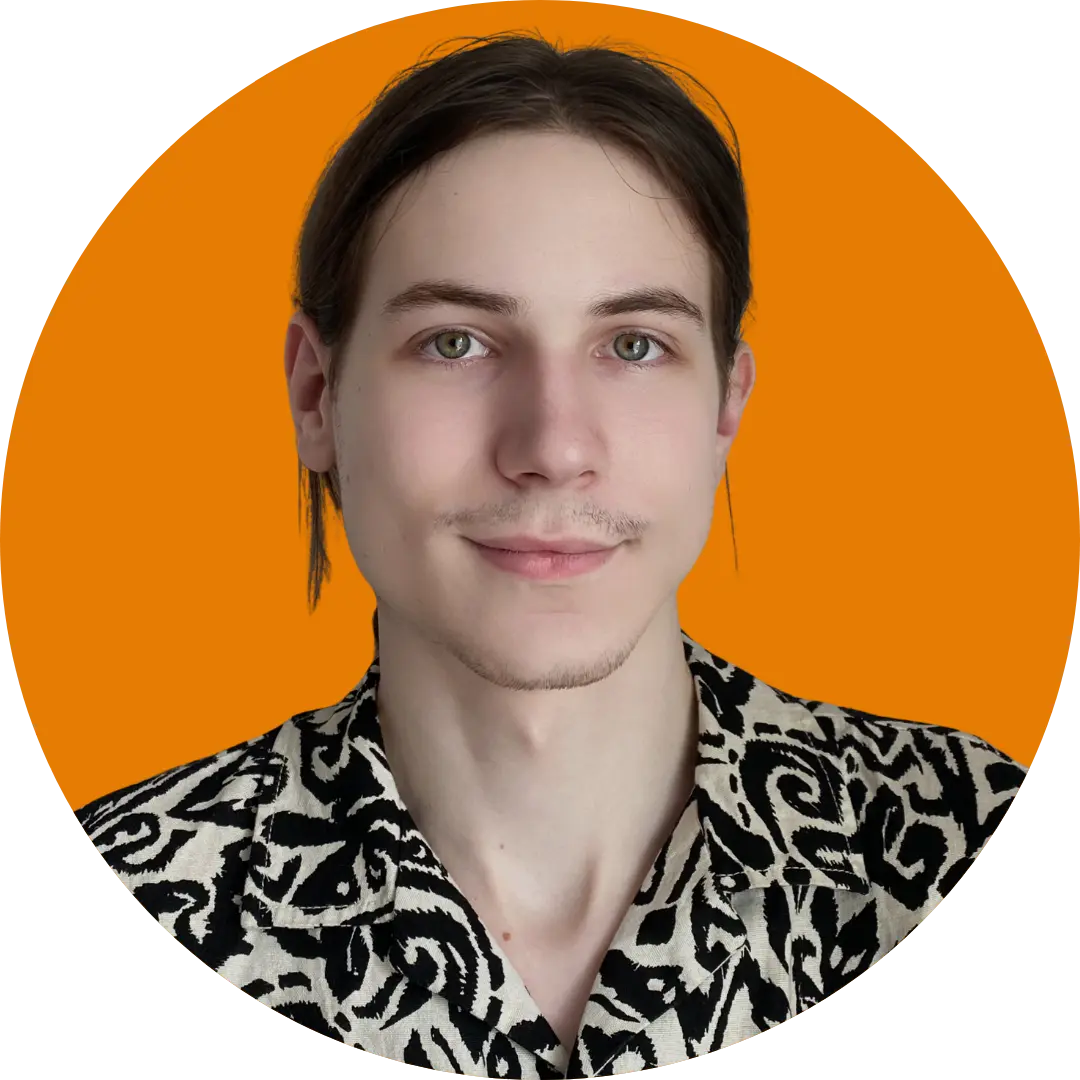
by Ihor Gudzyk
C++ Developer
Dec, 2023・6 min read
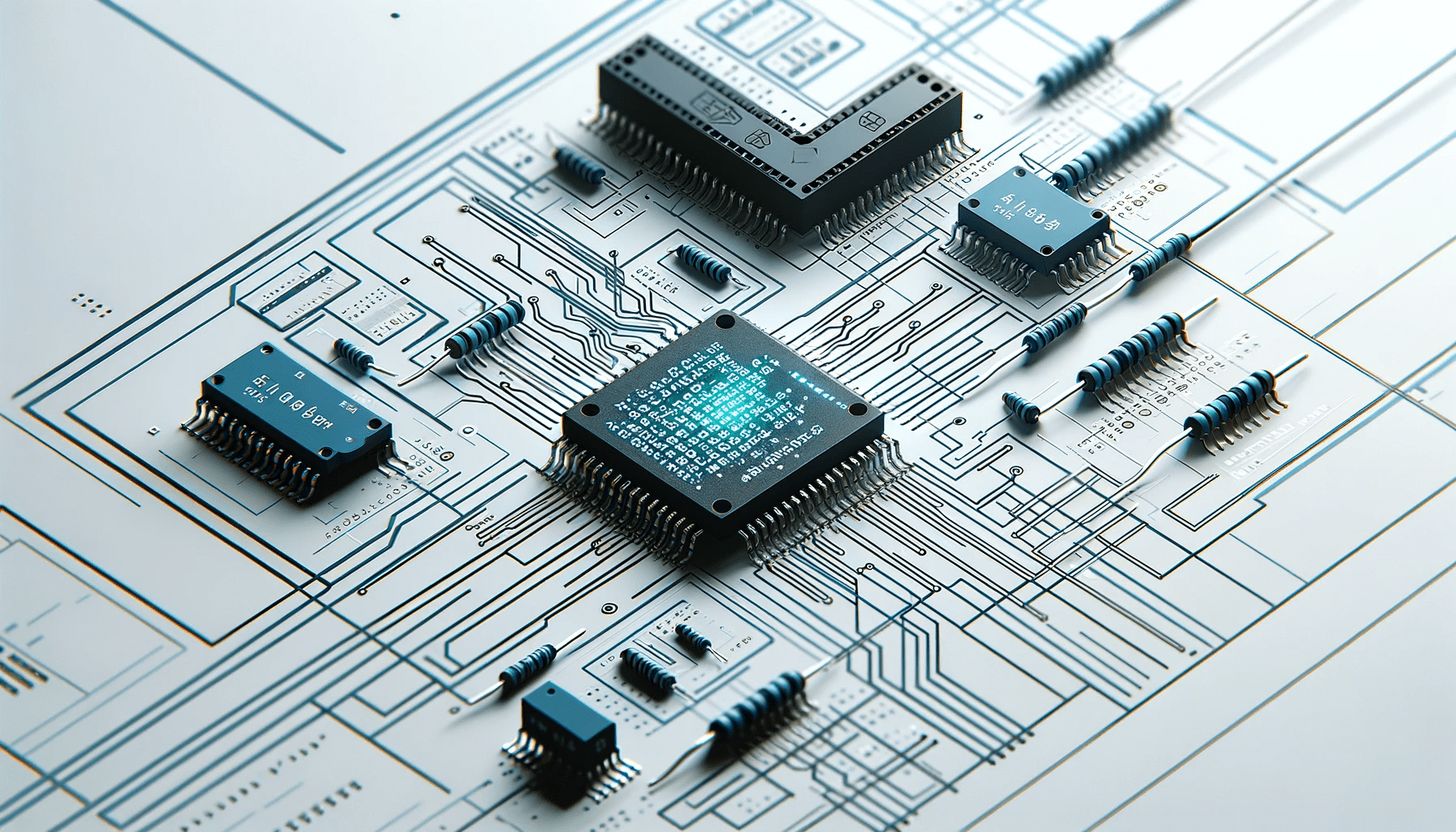
Contenido de este artículo