Cursos relacionados
Ver Todos os CursosConditional Types and Mapped Types in TypeScript
Exploring Conditional Types and Mapped Types
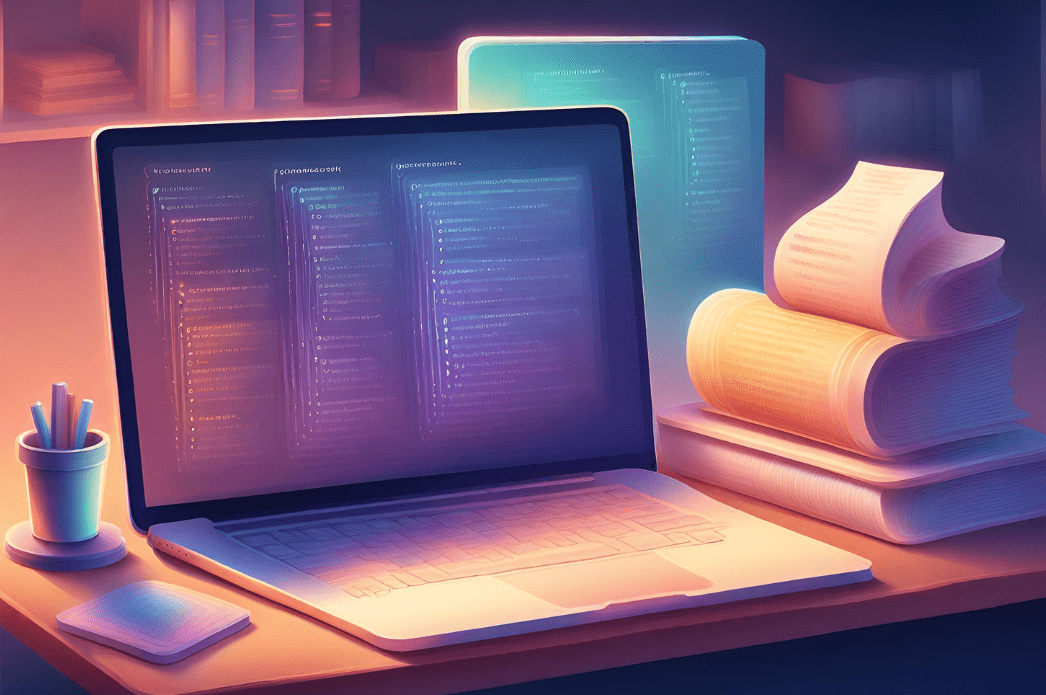
Introduction
TypeScript, the statically-typed superset of JavaScript, continues to revolutionize the way developers write robust and maintainable code. With its advanced type system, TypeScript offers a plethora of features that enhance code quality and developer productivity. Among these features, Conditional Types and Mapped Types stand out as powerful tools for creating flexible and reusable codebases.
Conditional Types
Conditional Types in TypeScript allow developers to create type transformations based on conditional logic. They enable the definition of types that depend on the structure of other types. This is particularly useful for creating flexible and adaptable type definitions that adjust to runtime conditions.
Syntax
T
: The type to be evaluated.U
: The condition against whichT
is checked.X
: The type returned if the condition istrue
.Y
: The type returned if the condition isfalse
.
Explanation
- Type Evaluation:
T
extendsU
checks if typeT
extends (or is assignable to) typeU
. - Conditional Logic: If the condition is
true
, the type resolves toX
; otherwise, it resolves toY
.
Use Cases
- Filtering Types: Create types based on certain conditions, such as extracting properties from objects that meet specific criteria.
- Dynamic Type Mapping: Enable dynamic type mapping based on runtime conditions.
- Type Guards: Facilitate type guards to narrow down types based on runtime checks.
Run Code from Your Browser - No Installation Required
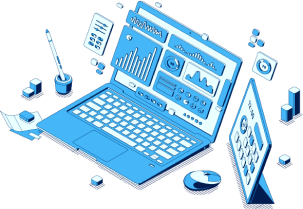
Conditional Types Examples
Example 1: Type Unions with Conditional Types
In this example, the FirstArgument
conditional type extracts the type of the first argument of a function type. It utilizes TypeScript's infer
keyword to infer the type of the first argument of the provided function type T
.
Example 2: Mapping Over Union Types
Here, the StringOrNumber
conditional type maps over a union type and converts each constituent type to either string
or number
. This demonstrates how conditional types can be used to handle union types dynamically.
Mapped Types
Mapped Types provide a concise way to transform the properties of existing types in TypeScript. They allow for iterating over the properties of a type and creating new types with modified or computed properties.
Syntax
T
: The original type whose properties are being mapped.K
: The keys (properties) of the original type.keyof T
: A TypeScript utility type that represents the union of all property names of typeT
.
Explanation
- Iteration: The mapped type iterates over each property of the original type.
- Transformation Logic: The logic applied to transform each property of the original type.
- New Type Construction: A new type is constructed with transformed properties.
Use Cases
- Adding Modifiers: Modify properties of a type, such as making them
readonly
oroptional
. - Transforming Properties: Apply transformations to properties, such as changing their types or values.
- Creating Subsets: Generate subsets of a type by selectively including or excluding certain properties.
Mapped Types Examples
Example 1: Partial and Required Mapped Types
In this example, we define mapped types PartialProperties
and RequiredProperties
to make properties either optional or required. This showcases how mapped types can manipulate the modifier of each property dynamically.
Example 2: Immutable Mapped Types
Here, the Immutable
mapped type creates an immutable version of the provided type by making all properties readonly
. This illustrates how mapped types can be used to enforce immutability in TypeScript.
Start Learning Coding today and boost your Career Potential
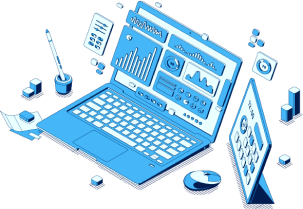
Conclusion
Conditional Types and Mapped Types in TypeScript provide developers with powerful tools for creating dynamic and flexible type definitions. By leveraging these features, developers can write more expressive and reusable code, leading to enhanced code quality and maintainability.
FAQs
Q: What are Conditional Types in TypeScript?
A: Conditional Types in TypeScript allow developers to create type transformations based on conditional logic. They enable the definition of types that depend on the structure of other types, providing flexibility and adaptability in type definitions.
Q: How do Conditional Types work in TypeScript?
A: Conditional Types use a syntax similar to ternary expressions (condition ? X : Y
) to evaluate a type based on a condition. If the condition is true, the type resolves to X
; otherwise, it resolves to Y
.
Q: What are some use cases of Conditional Types?
A: Conditional Types can be used for filtering types based on conditions, dynamic type mapping, and implementing type guards to narrow down types based on runtime checks.
Q: What are Mapped Types in TypeScript?
A: Mapped Types in TypeScript provide a concise way to transform the properties of existing types. They allow for iterating over the properties of a type and creating new types with modified or computed properties.
Q: How do Mapped Types work in TypeScript?
A: Mapped Types iterate over each property of the original type, apply transformation logic to each property, and construct a new type with transformed properties.
Q: What are some common use cases of Mapped Types?
A: Mapped Types can be used for adding modifiers to properties, transforming properties, and creating subsets of types by selectively including or excluding certain properties.
Cursos relacionados
Ver Todos os CursosThe SOLID Principles in Software Development
The SOLID Principles Overview
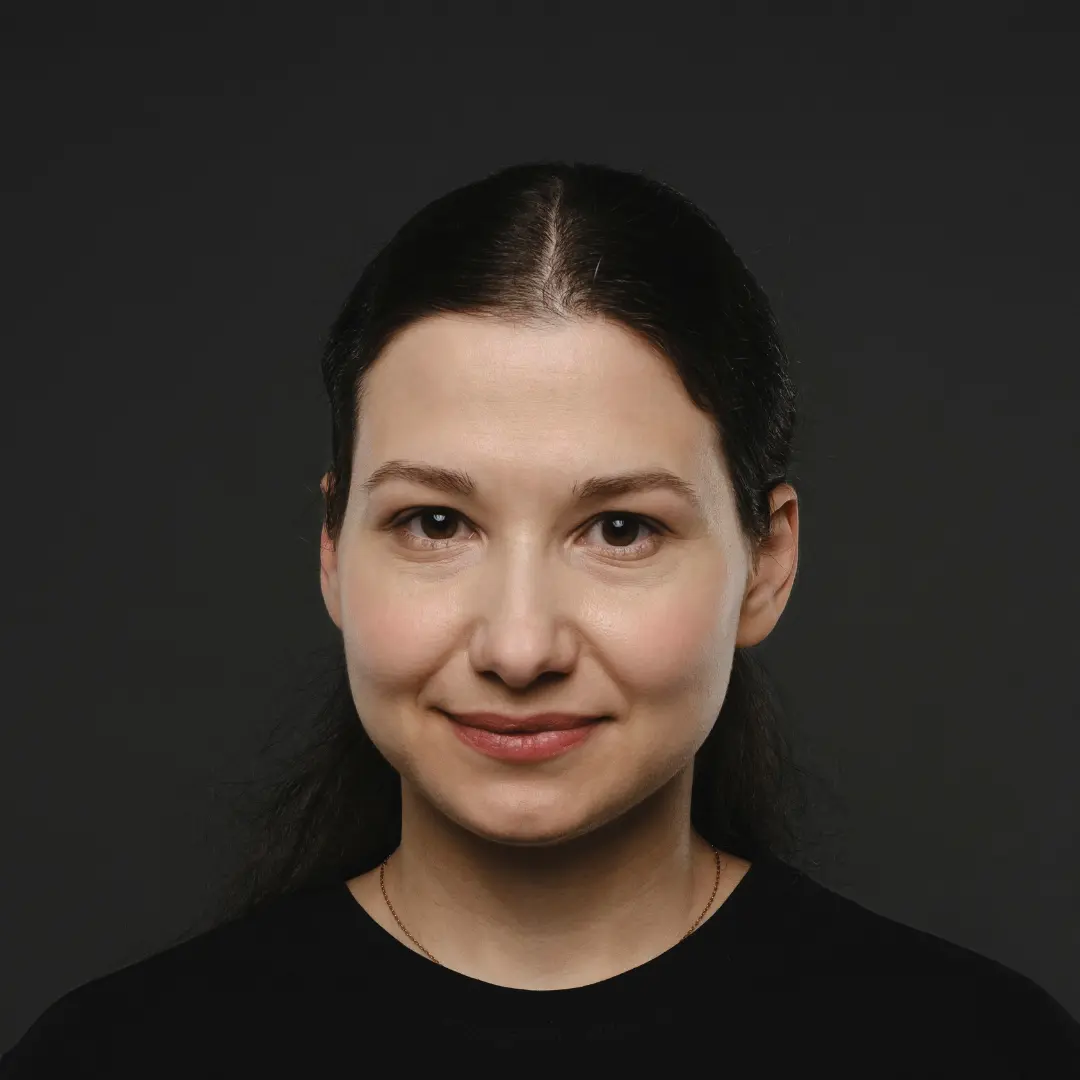
by Anastasiia Tsurkan
Backend Developer
Nov, 2023・8 min read
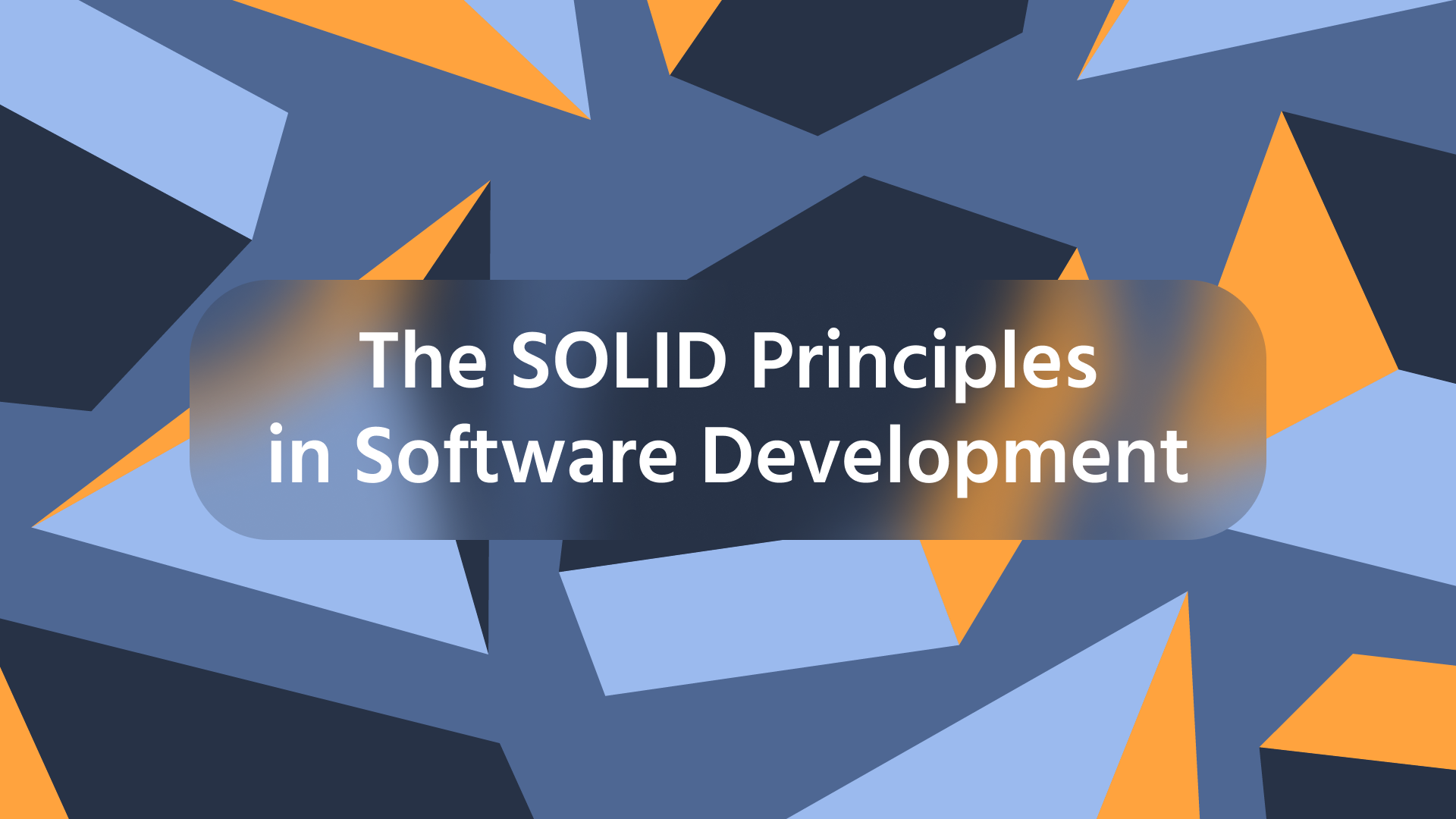
Match-case Operators in Python
Match-case Operators vs if-elif-else statements
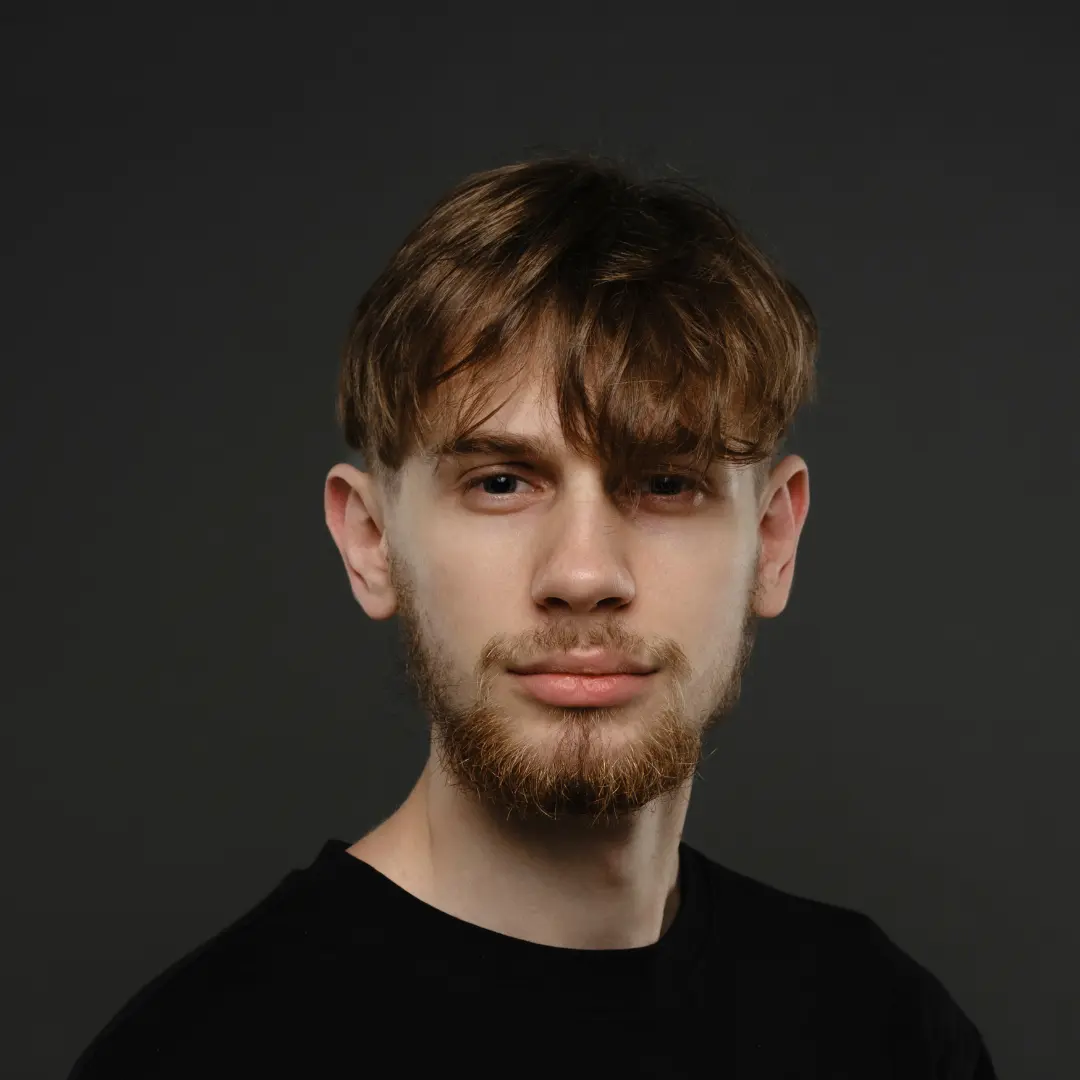
by Oleh Lohvyn
Backend Developer
Dec, 2023・6 min read
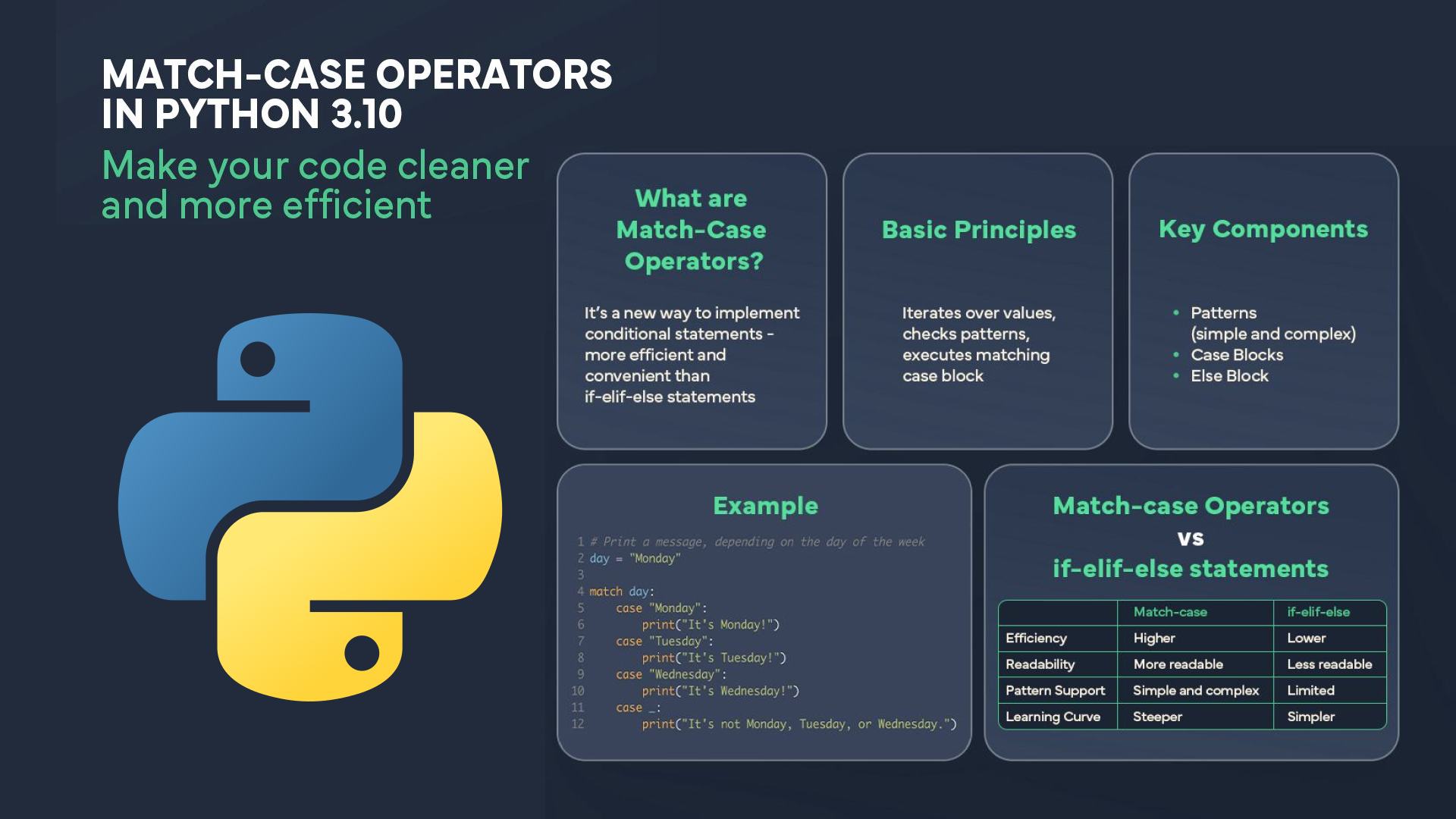
30 Python Project Ideas for Beginners
Python Project Ideas
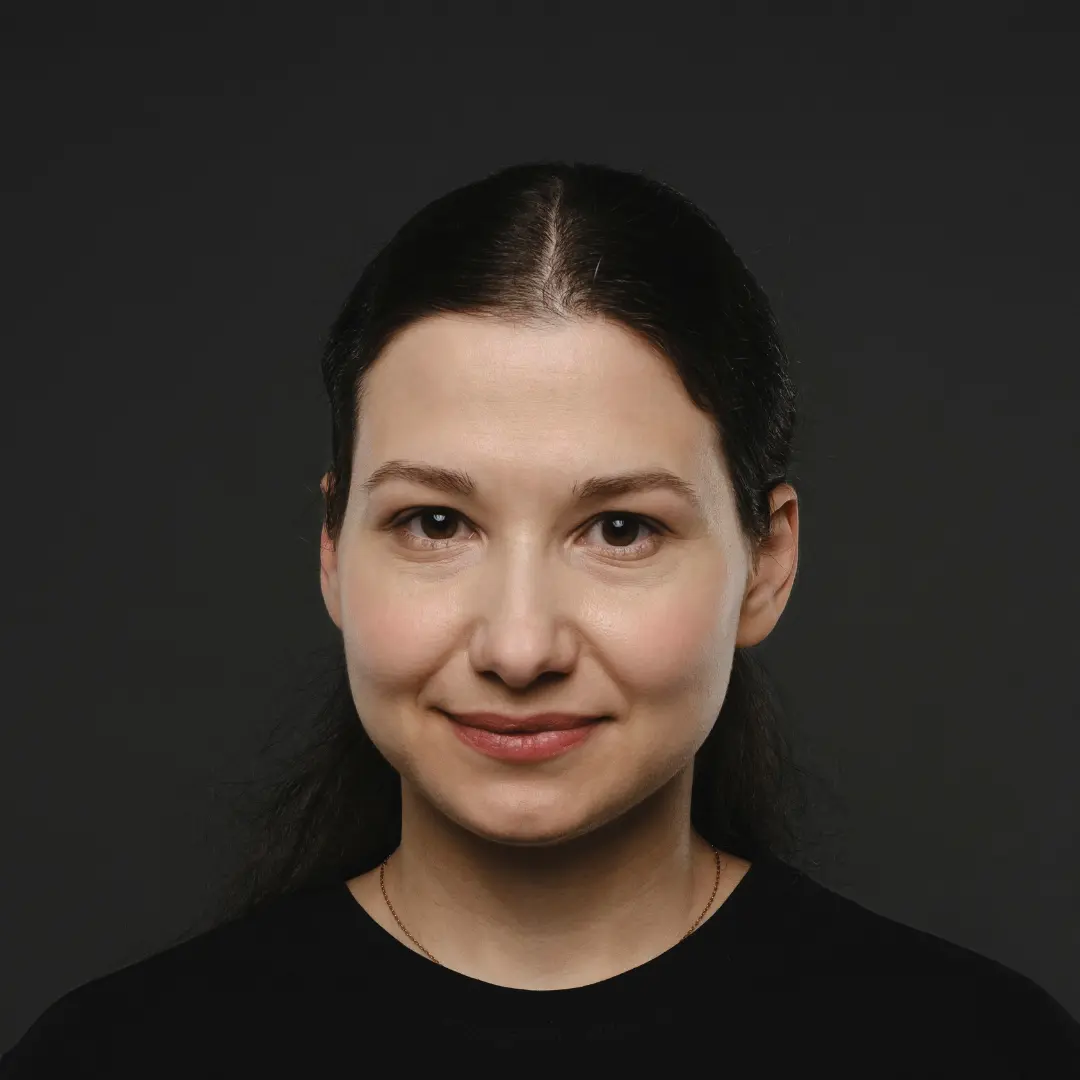
by Anastasiia Tsurkan
Backend Developer
Sep, 2024・14 min read
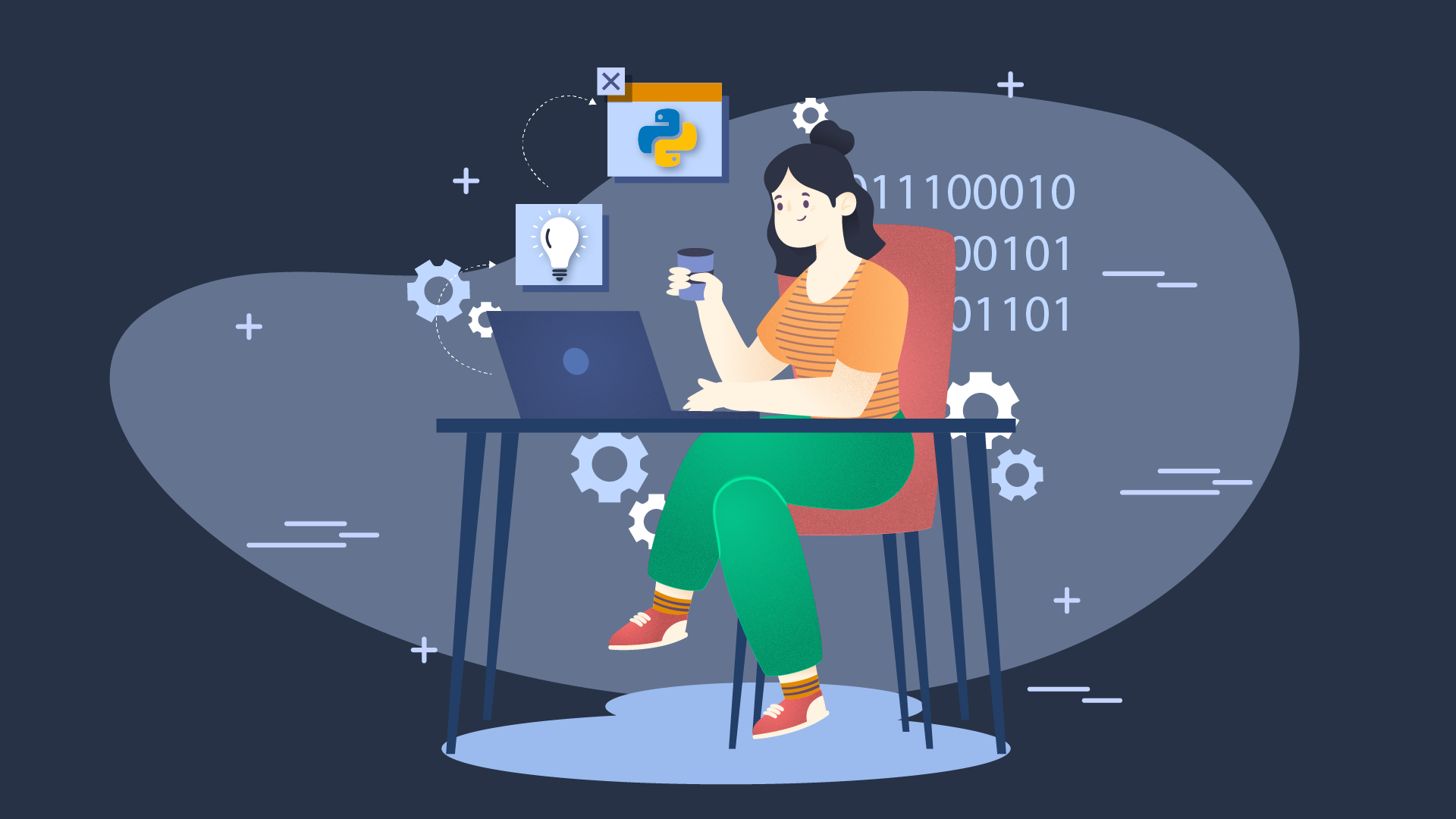
Conteúdo deste artigo