Cursos relacionados
Ver Todos os CursosIniciante
C++ Data Types
Developing a comprehensive understanding of data types is crucial when learning a programming language. This course offers an opportunity to delve deeper into data types in C++, gaining insights into how they are stored in memory. Additionally, the course covers the topic of type conversion.
Intermediário
C++ Pointers and References
Unlock the Power of Memory Manipulation. Dive deep into the fundamentals of programming with this comprehensive course designed for beginners and individuals seeking to strengthen their knowledge on pointers and references in C++. Mastering these essential concepts is crucial for unleashing the full potential of your programming skills. Let's start!
Iniciante
C++ Introduction
Start your path to becoming a skilled developer by mastering the foundational principles of programming through C++. Whether you're starting from scratch or already have some coding experience, this course will provide you with the solid foundation needed to become a proficient developer and open the doors to a wide range of career opportunities in software development and engineering. Let's study C++!
Float vs Double
A Comprehensive Guide
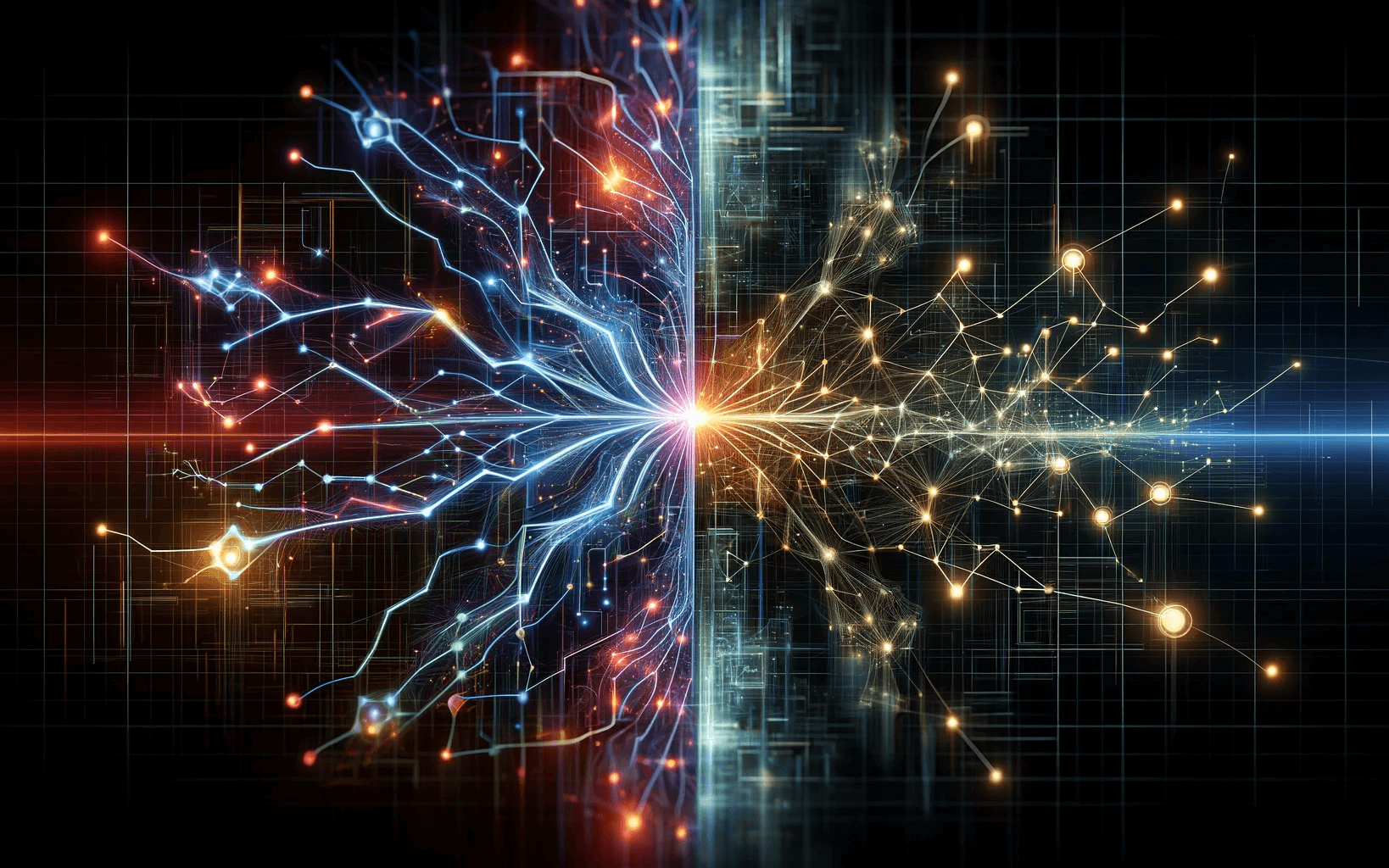
Introduction to Float and Double
What are Float and Double?
Float
and double
are data types used in programming to store numerical values with decimal points. Both are used to represent floating point numbers, but they do so with different precisions and storage requirements. The float
type, typically a single-precision 32-bit IEEE 754 floating point, is used for decimal values that do not require extreme precision. The double
type, usually a double-precision 64-bit IEEE 754 floating point, provides a greater precision and is used when more accurate calculations are necessary.
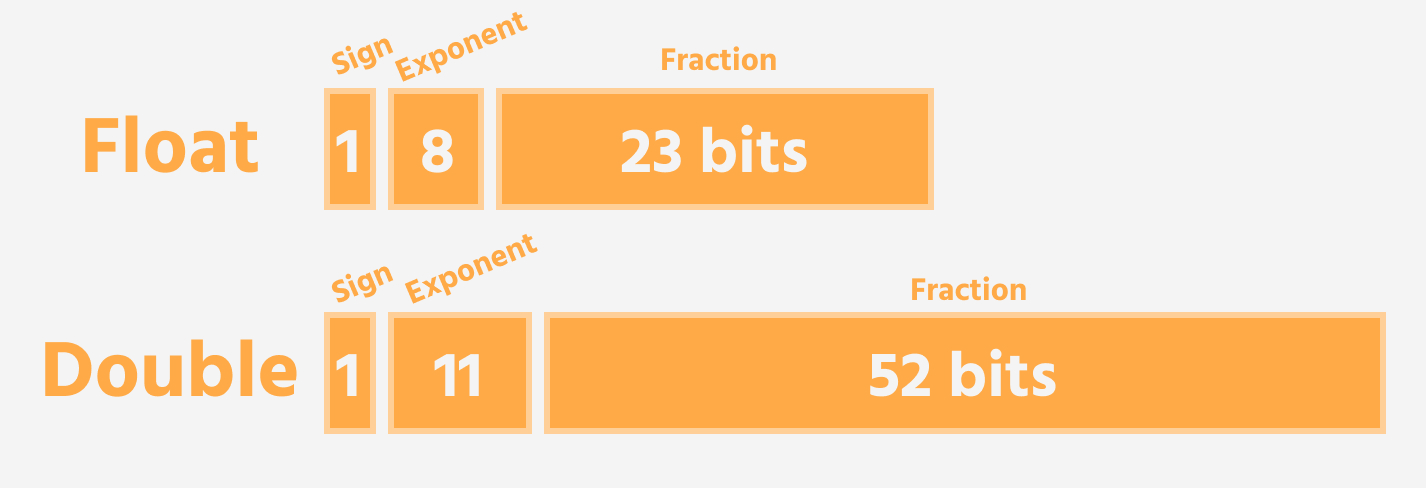
You can read the full IEEE 754 standard in this scientific article.
Importance of Float and Double in Programming
In programming, choosing between float
and double
can significantly affect the precision of the calculations and the overall performance of the application. They are crucial in fields such as scientific computing, graphics programming, and any other domain that requires handling of large numbers with fractional parts.
Key Differences Between Float and Double
Precision and Range
The primary difference between float
and double
is the precision. Float
is a single-precision float with about 7 decimal digits of precision and a range from approximately 1.4E-45
to 3.4E+38
. On the other hand, double
offers double the precision with about 15 decimal digits and a range from approximately 4.9E-324
to 1.8E+308
, making it more suitable for accurate calculations.
For the double
type, you can consider up to 15 decimal digits to be precise and reliable. For the float
type, up to 7 decimal digits are considered accurate. Beyond these precisions, any additional digits may not be accurately represented due to the limitations in how these data types store numbers .
Memory Usage
Float
consumes 32 bits of memory, while double
consumes 64 bits. The choice between them can affect the application's memory usage, especially when dealing with large data arrays or matrices.
Performance Considerations
The performance impact of using float
versus double
can vary depending on the processor architecture. Some systems handle double-precision calculations as efficiently as single-precision, while others, especially those with less processing power like mobile devices, might process single-precision floats faster.
Run Code from Your Browser - No Installation Required
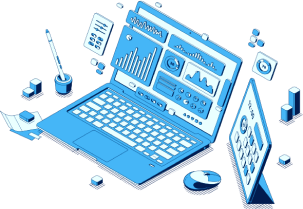
When to Use Float vs Double
Choosing Float for Specific Applications
Float
is typically used when the data does not require extreme precision and memory bandwidth is more critical. This might be the case in applications like graphics processing where high performance is crucial but exact precision is less critical.
Choosing Double for High Precision Needs
For applications that require high precision and accuracy, such as complex scientific calculations or financial algorithms where small errors can significantly affect outcomes, double
is generally preferred despite its higher resource consumption.
Attribute | Float | Double |
---|---|---|
Precision | 7 decimal digits | 15 decimal digits |
Memory Usage | 32 bits (4 bytes) | 64 bits (8 bytes) |
Typical Use Cases | Graphics, sound processing, simple calculations | Scientific computing, precise measurements, financial calculations |
Performance Metrics | Faster on some hardware, less memory intensive | Slower on some hardware, more memory intensive but provides higher precision |
Examples of Float and Double in Programming
Float Example in C/C++
In C and C++, a float is declared with the keyword float
. It typically provides up to 7 decimal digits of precision. Here's how you can define a float variable:
Double Example in C/C++
A double in C and C++ offers more precision, typically up to 15 decimal digits. It is declared with the keyword double
. Here’s an example:
You can read more about technical detail in this documentation.
Float and Double in Java
Java also uses float
and double
types, similar to C/C++. The float
type in Java is a single-precision 32-bit IEEE 754 floating point and double
is a double-precision 64-bit.
Float vs Double in Python
Python does not have a separate float and double type distinction. It uses double
precision floating-point numbers for all decimal values. Here's how you define a floating point number in Python:
Float and Double in JavaScript
JavaScript does not differentiate between different types of floating point numbers; it only has one data type for decimals — number
, which is implemented as double-precision floating point according to IEEE 754.
Common Pitfalls and Best Practices
Rounding Errors and Precision Loss
One of the common pitfalls when using floating point numbers (float
and double
) is rounding errors and precision loss. These issues arise because these data types cannot precisely represent all real numbers, and operations on them can accumulate small errors that can affect the outcome of computations. This is particularly problematic in scientific computations, financial calculations, and other fields requiring high precision.
Best Practices for Using Float and Double
To mitigate issues related to precision and rounding errors, it is crucial to follow best practices:
- Understand the precision needs: Always choose the data type based on the precision requirements of your application. Use
double
when more precision is needed. - Beware of equality checks: When comparing floating point numbers, consider using a tolerance for equality to account for possible small differences.
- Use appropriate functions and libraries: Utilize functions and libraries that are designed to handle floating point numbers accurately, especially for complex mathematical operations.
- Educate yourself on floating point arithmetic: Understanding how floating point arithmetic works can help you avoid common mistakes and make better decisions in your code design.
Start Learning Coding today and boost your Career Potential
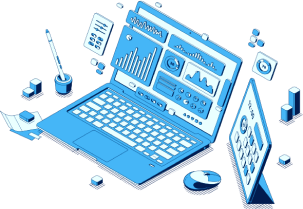
Conclusion
Summary of Key Points
- Precision and Range:
Float
provides up to 7 decimal digits of precision and a smaller range, whiledouble
offers up to 15 decimal digits and a much broader range. - Memory Usage:
Float
uses 32 bits of storage, whereasdouble
uses 64 bits, impacting the application's memory usage. - Performance Considerations: The choice between
float
anddouble
can affect computational performance, particularly on platforms with limited processing capabilities.
Final Thoughts on Float vs Double
Choosing between float
and double
depends on the requirements of precision, performance, and memory in your application. While float
might be sufficient for many applications, double
provides greater precision and is essential in high-stake calculations where the slight inaccuracies could lead to significant consequences.
FAQs
Q: What is the maximum value for Float and Double types?
A: The maximum value for a float
is approximately 3.4E+38
, and for a double
, it is about 1.8E+308
, reflecting the larger range and precision capabilities of the double
type.
Q: Can Float and Double be used interchangeably?
A: They can be used interchangeably in some contexts, but careful consideration of the application's precision needs is crucial, as double
offers higher precision and should be used in scenarios requiring detailed accuracy.
Q: How do Float and Double handle very large or very small numbers?
A: Both types manage large and small numbers using scientific notation. However, double
has a significantly larger range and precision, making it capable of handling much larger or smaller values compared to float
.
Q: What are the implications of using Float vs Double in graphics programming?
A: In graphics programming, float
is often sufficient and preferred due to less memory consumption and faster processing, as high precision is typically not required for graphical calculations.
Q: Are there any performance differences when using Float vs Double in different programming languages?
A: Performance can vary by programming language and hardware. Some environments process double
values as efficiently as float
values, but in resource-constrained environments like mobile devices, float
may offer performance benefits.
Cursos relacionados
Ver Todos os CursosIniciante
C++ Data Types
Developing a comprehensive understanding of data types is crucial when learning a programming language. This course offers an opportunity to delve deeper into data types in C++, gaining insights into how they are stored in memory. Additionally, the course covers the topic of type conversion.
Intermediário
C++ Pointers and References
Unlock the Power of Memory Manipulation. Dive deep into the fundamentals of programming with this comprehensive course designed for beginners and individuals seeking to strengthen their knowledge on pointers and references in C++. Mastering these essential concepts is crucial for unleashing the full potential of your programming skills. Let's start!
Iniciante
C++ Introduction
Start your path to becoming a skilled developer by mastering the foundational principles of programming through C++. Whether you're starting from scratch or already have some coding experience, this course will provide you with the solid foundation needed to become a proficient developer and open the doors to a wide range of career opportunities in software development and engineering. Let's study C++!
The SOLID Principles in Software Development
The SOLID Principles Overview
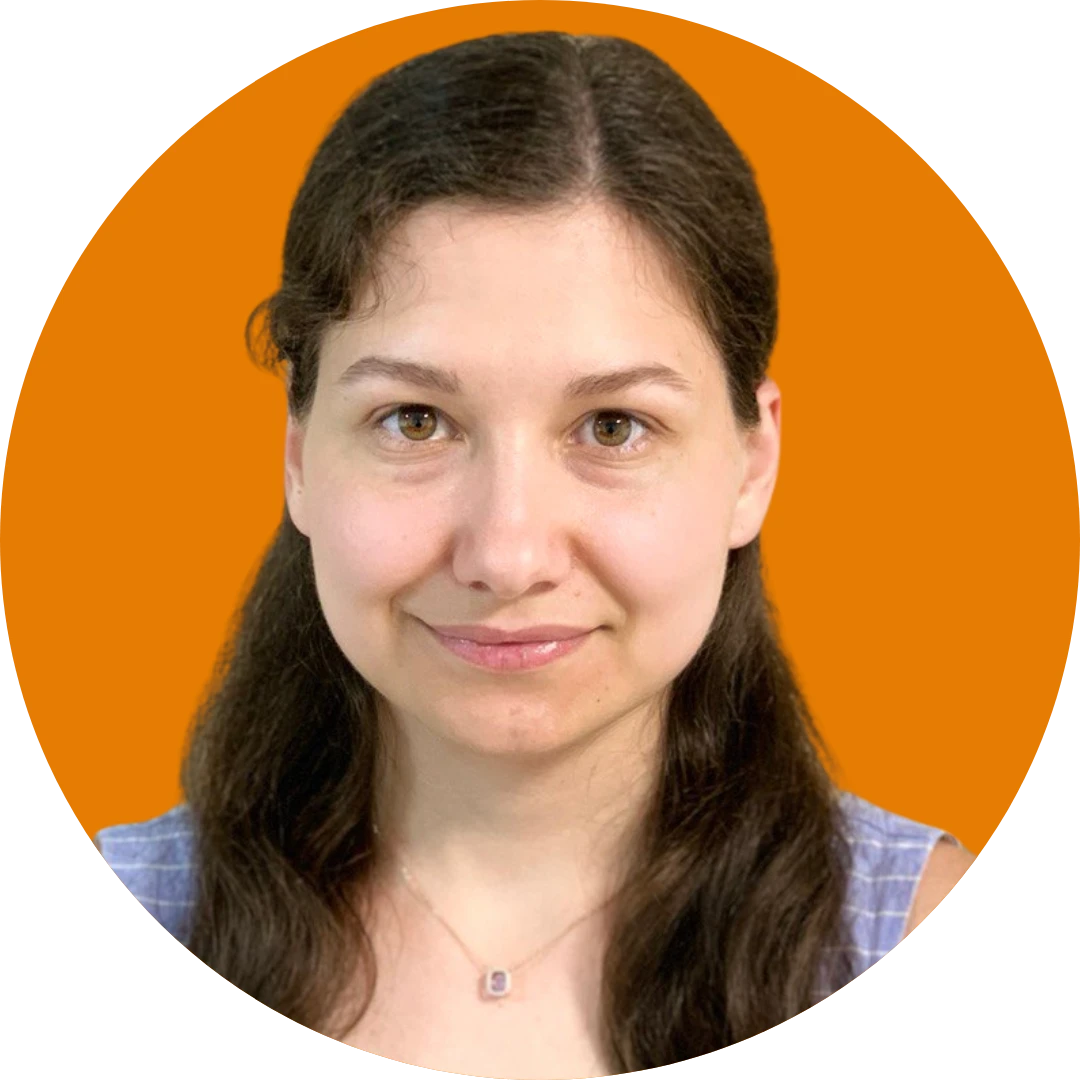
by Anastasiia Tsurkan
Backend Developer
Nov, 2023・8 min read
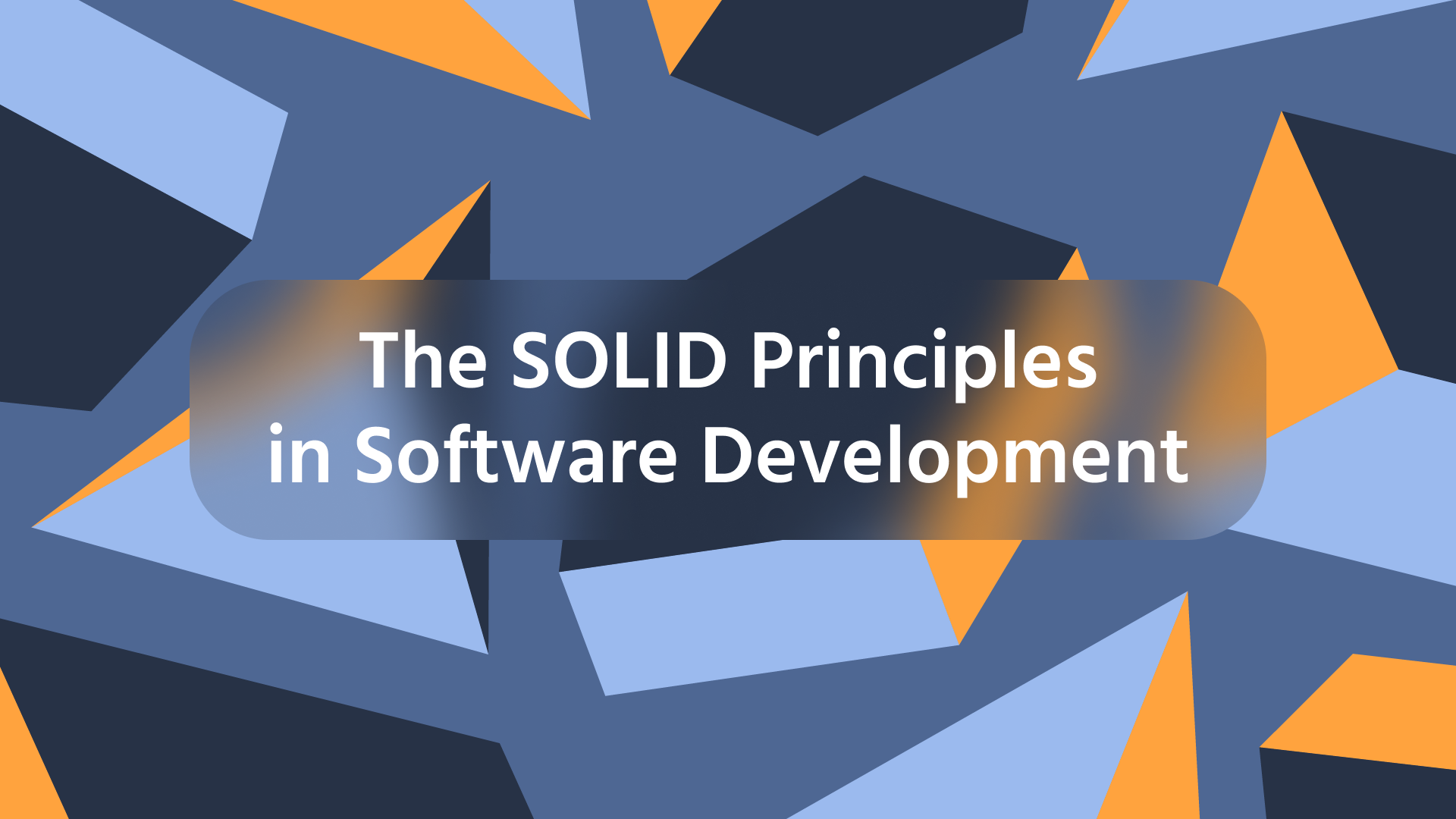
30 Python Project Ideas for Beginners
Python Project Ideas
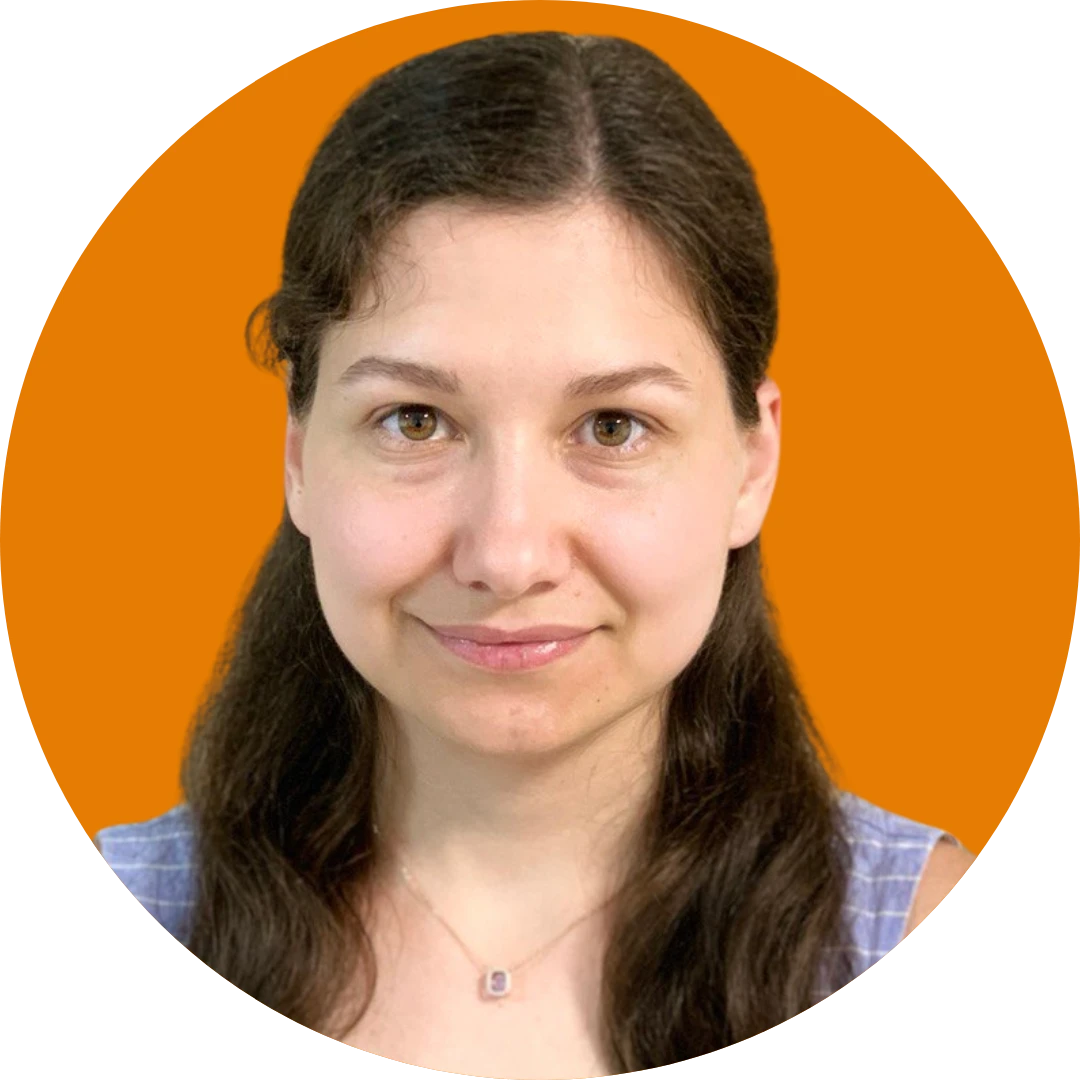
by Anastasiia Tsurkan
Backend Developer
Sep, 2024・14 min read
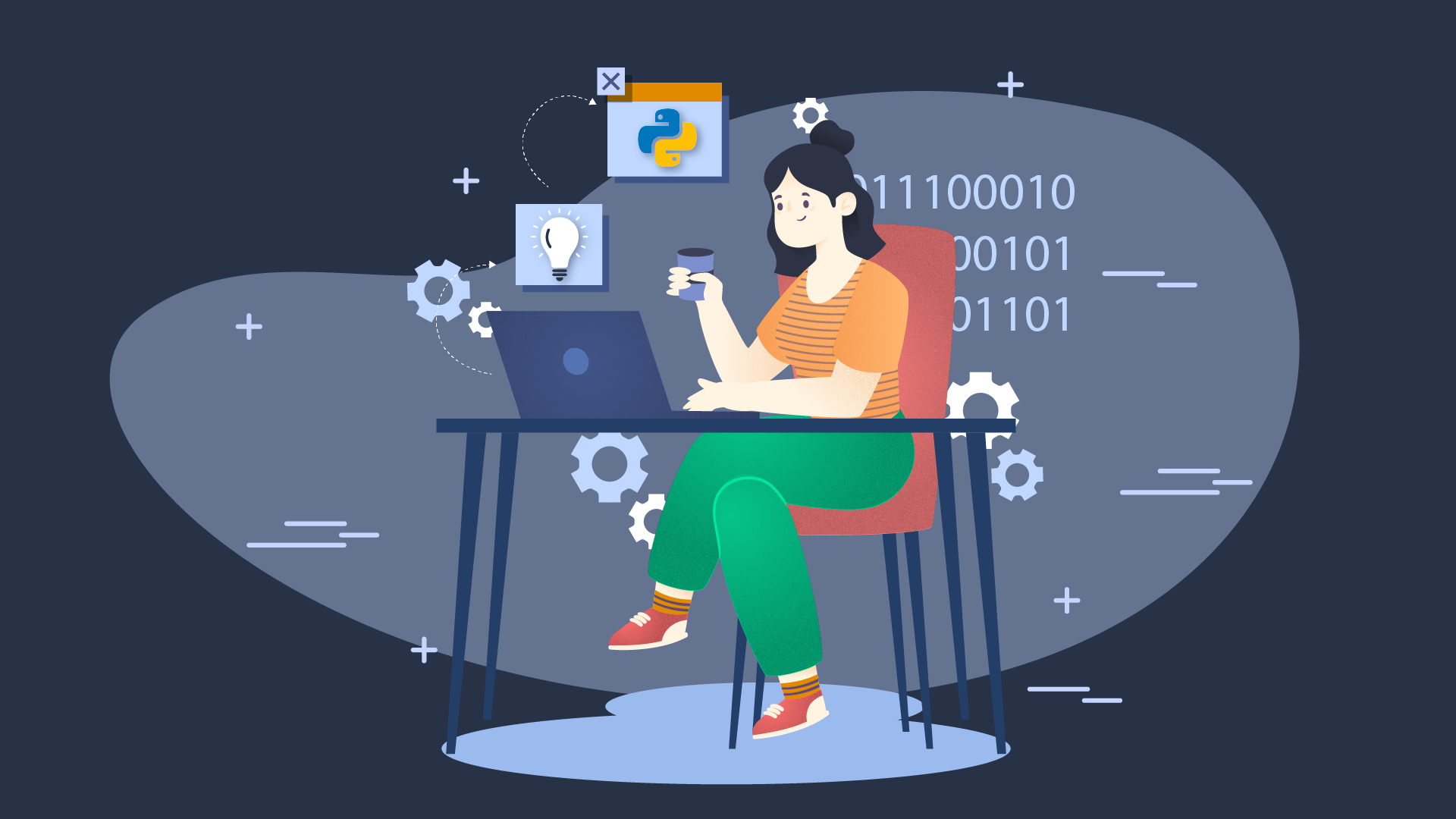
Asynchronous Programming in Python
Brief Intro to Asynchronous Programming
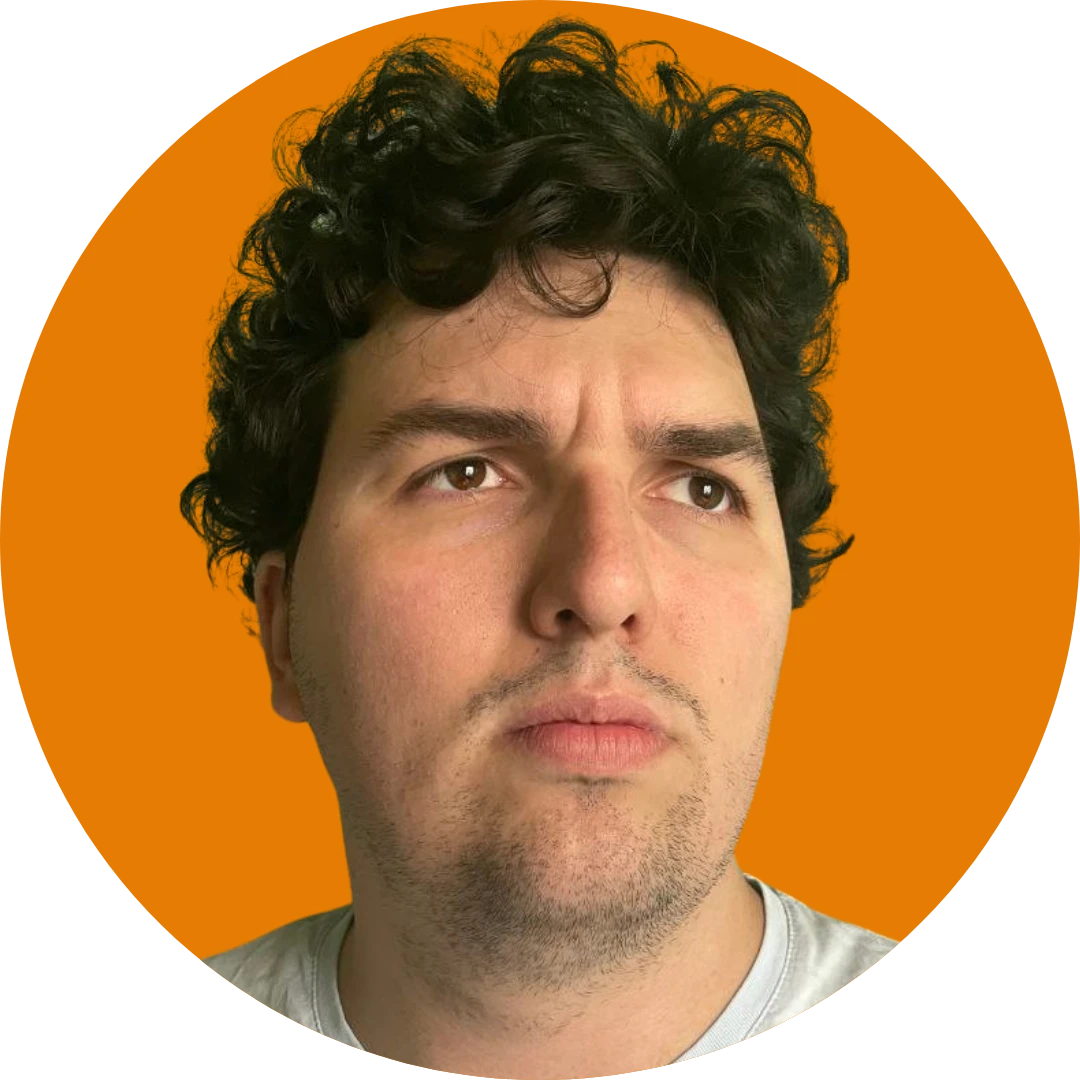
by Ruslan Shudra
Data Scientist
Dec, 2023・5 min read
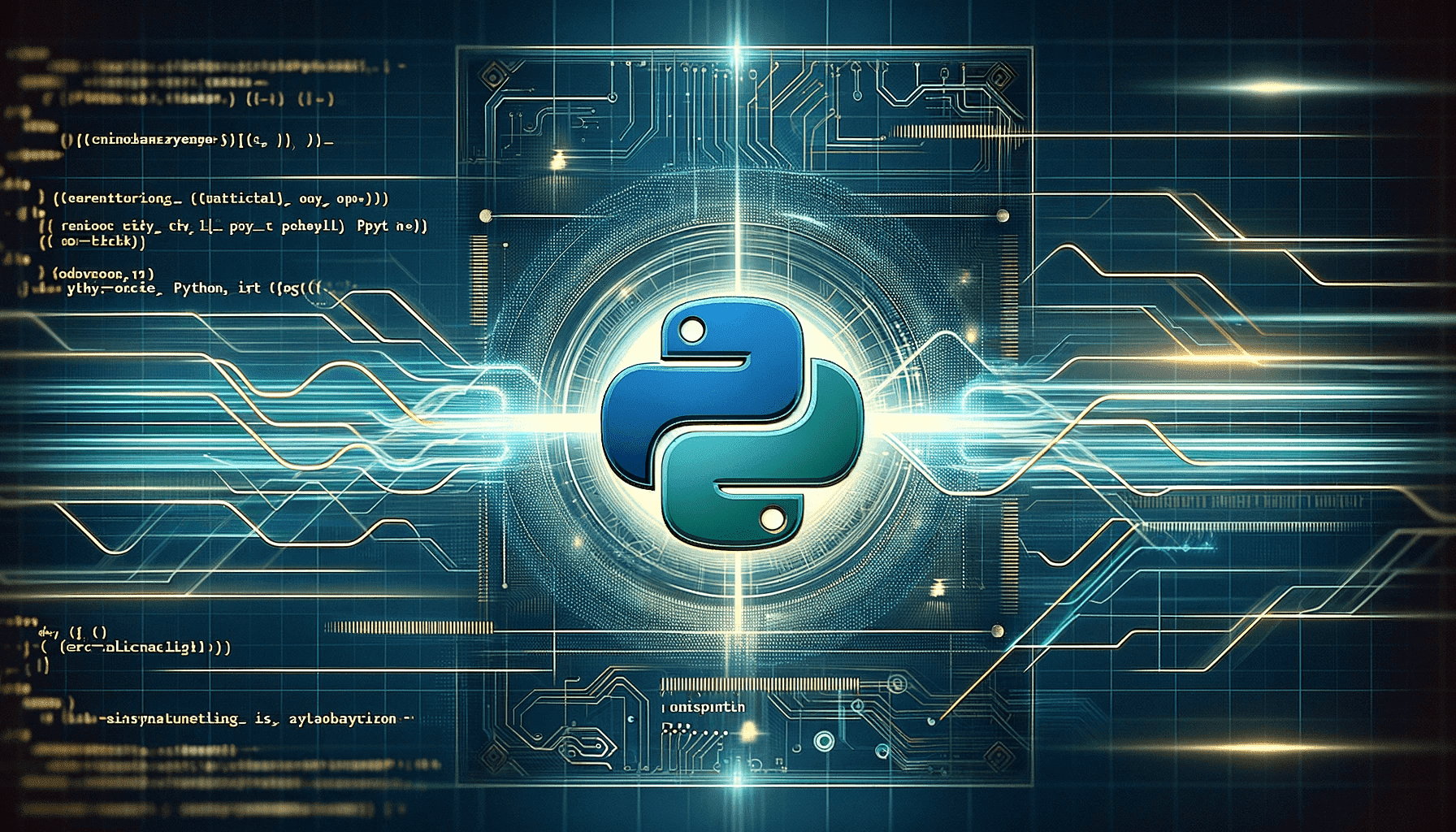
Conteúdo deste artigo