Using var in Java
Understanding the var Keyword in Java
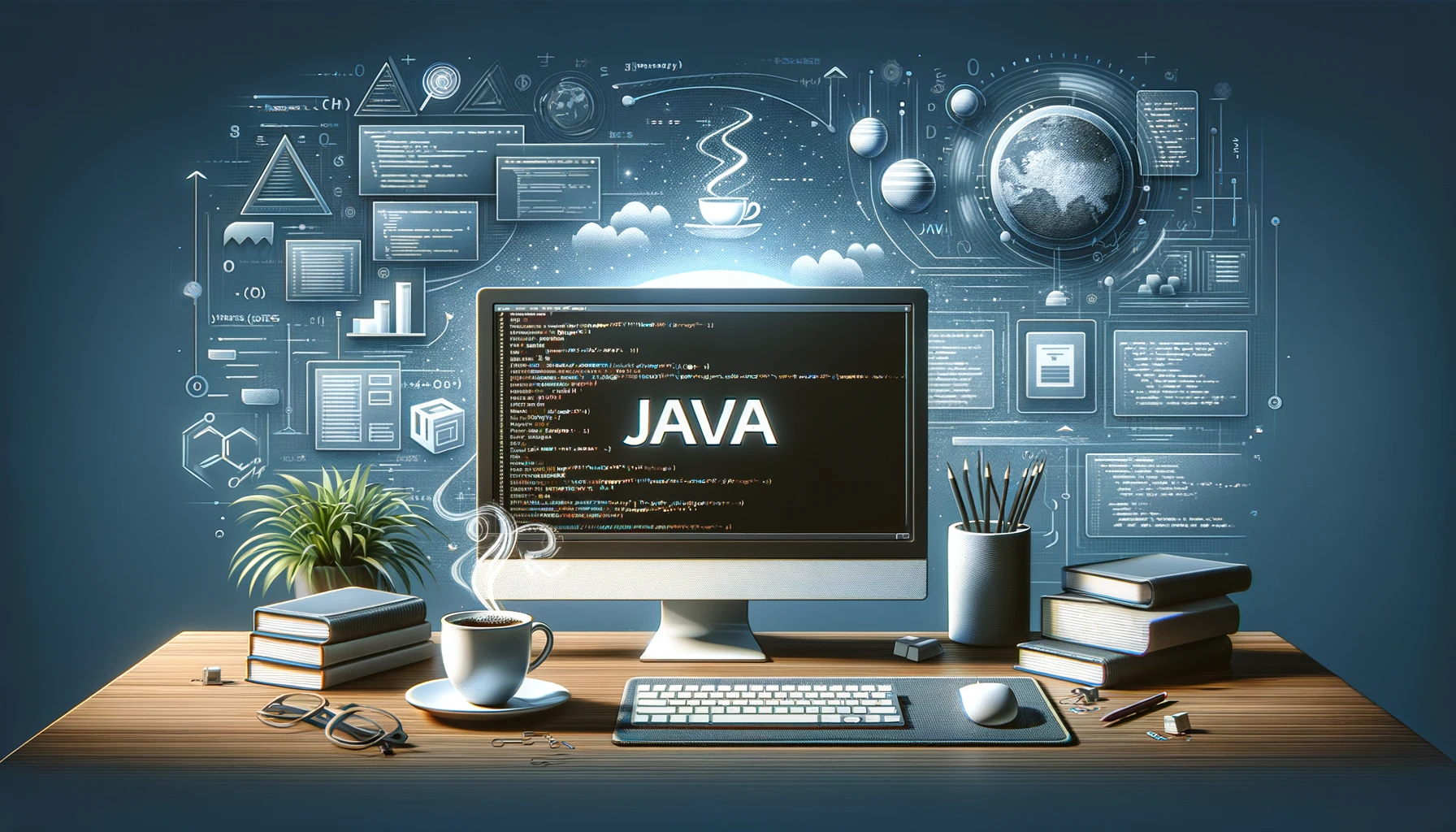
Java 10 introduced a new feature that was long-awaited by many developers: the var
keyword. This small addition can make your code cleaner and more readable by allowing you to infer variable types instead of explicitly declaring them. In this article, we'll dive deep into how to use var
, its benefits, and some best practices to keep in mind.
What is var?
The var
keyword in Java is a feature known as "local variable type inference." This means that the compiler can infer the type of a local variable at compile time, based on the context in which it is used. For example, instead of declaring a variable with a specific type, you can use var
, and the compiler will determine the type for you.
Consider this simple example:
java
In these examples, var
replaces the explicit type declaration (String
, int
, ArrayList<String>
), making the code shorter and, in many cases, easier to read.
Run Code from Your Browser - No Installation Required
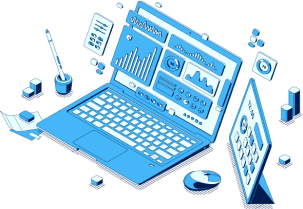
Benefits of Using var
- Readability: By removing the need for explicit type declarations,
var
can make your code more concise and readable. - Maintenance: Refactoring becomes easier since changing the type of a variable only requires changing the right-hand side of the assignment.
- Reduced Boilerplate:
var
can help reduce the amount of boilerplate code, especially when working with complex generic types.
To illustrate, consider the declaration of a map. Without var
, it looks like this:
java
With var
, it simplifies to:
java
The second example is shorter and easier to read, especially when dealing with long generic type declarations.
Limitations of var
While var
offers many benefits, it's important to understand its limitations to avoid potential pitfalls.
- Local Variables Only:
var
can only be used for local variables. It cannot be used for class fields, method parameters, or return types. - Inference Ambiguity: In some cases,
var
can make the code less readable if the inferred type is not obvious from the context. - Not for All Cases: Using
var
indiscriminately can lead to code that is hard to understand. It’s important to usevar
judiciously.
For example, the following code may be confusing:
java
In this case, it’s not clear what type x
is, which can make the code harder to understand.
Best Practices for Using var
- Use
var
When the Type is Clear from Context: If the type is clear from the context,var
can make the code cleaner. - Avoid
var
for Primitive Types: Usingvar
for primitives can reduce readability since the inferred type might not be obvious. - Use Descriptive Variable Names: Descriptive variable names can help mitigate the loss of explicit type information.
- Avoid Complex Expressions: Avoid using
var
with complex expressions where the inferred type might not be obvious.
A good practice example would be:
java
Conversely, a bad practice example might be:
java
Start Learning Coding today and boost your Career Potential
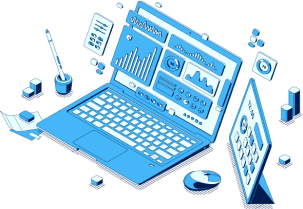
Common Use Cases for var
- Collections: Simplifying the declaration of collections.
- Streams: Enhancing readability in stream operations.
- Loop Variables: Cleaner loop variable declarations.
For instance, when working with collections:
java
When using streams, var
can improve readability:
java
In loops, var
makes variable declarations more concise:
java
FAQs
Q: Can var
be used for method return types?
A: No, var
can only be used for local variable declarations.
Q: Is var
available in all versions of Java?
A: No, var
was introduced in Java 10. It is not available in earlier versions.
Q: Does using var
affect performance?
A: No, var
does not affect performance. It is purely a compile-time feature.
Q: Can I use var
with array declarations?
A: Yes, you can use var
with array declarations. For example: var numbers = new int[]{1, 2, 3};
Q: What happens if the type cannot be inferred when using var
?
A: If the compiler cannot infer the type, it will produce a compilation error.
The SOLID Principles in Software Development
The SOLID Principles Overview
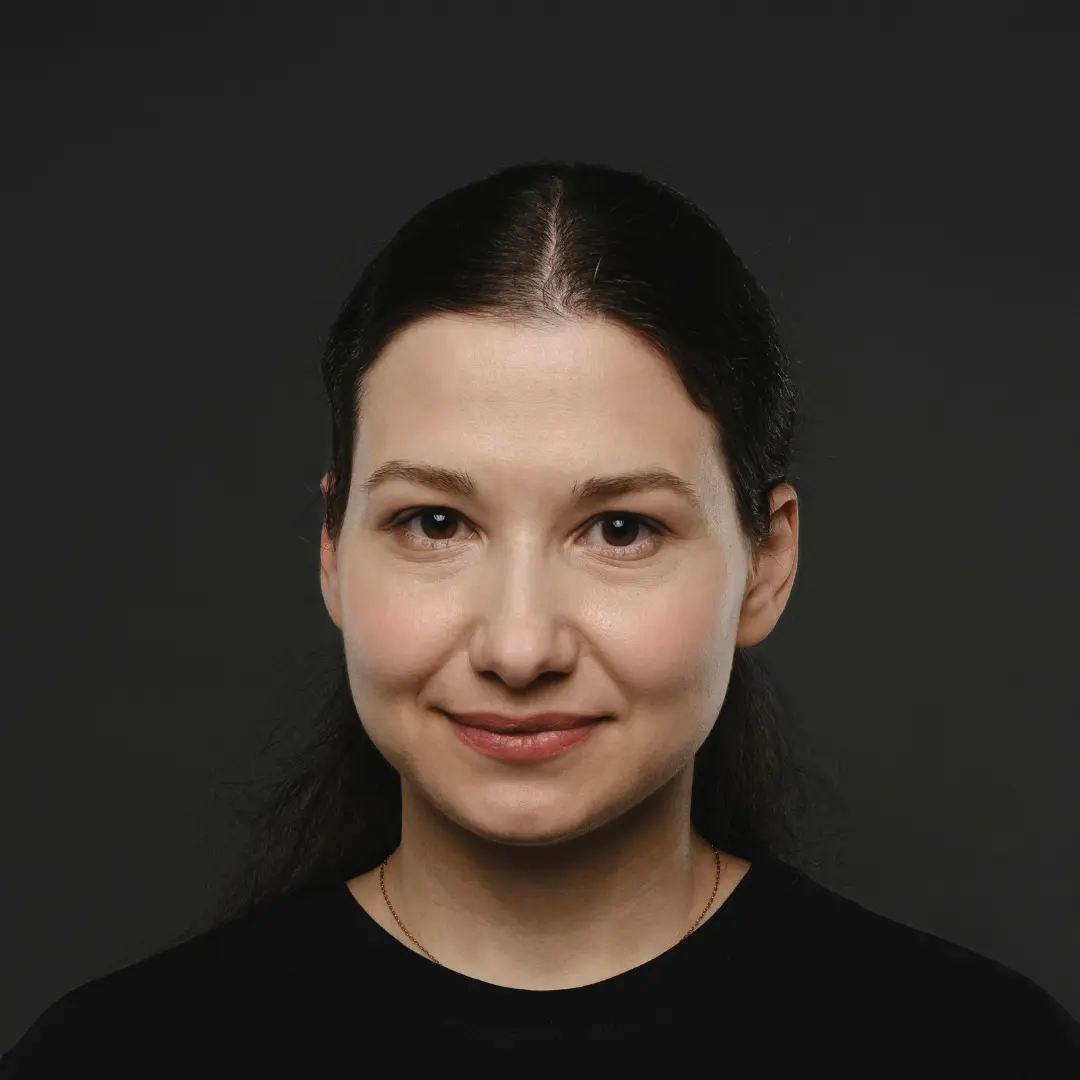
by Anastasiia Tsurkan
Backend Developer
Nov, 2023・8 min read
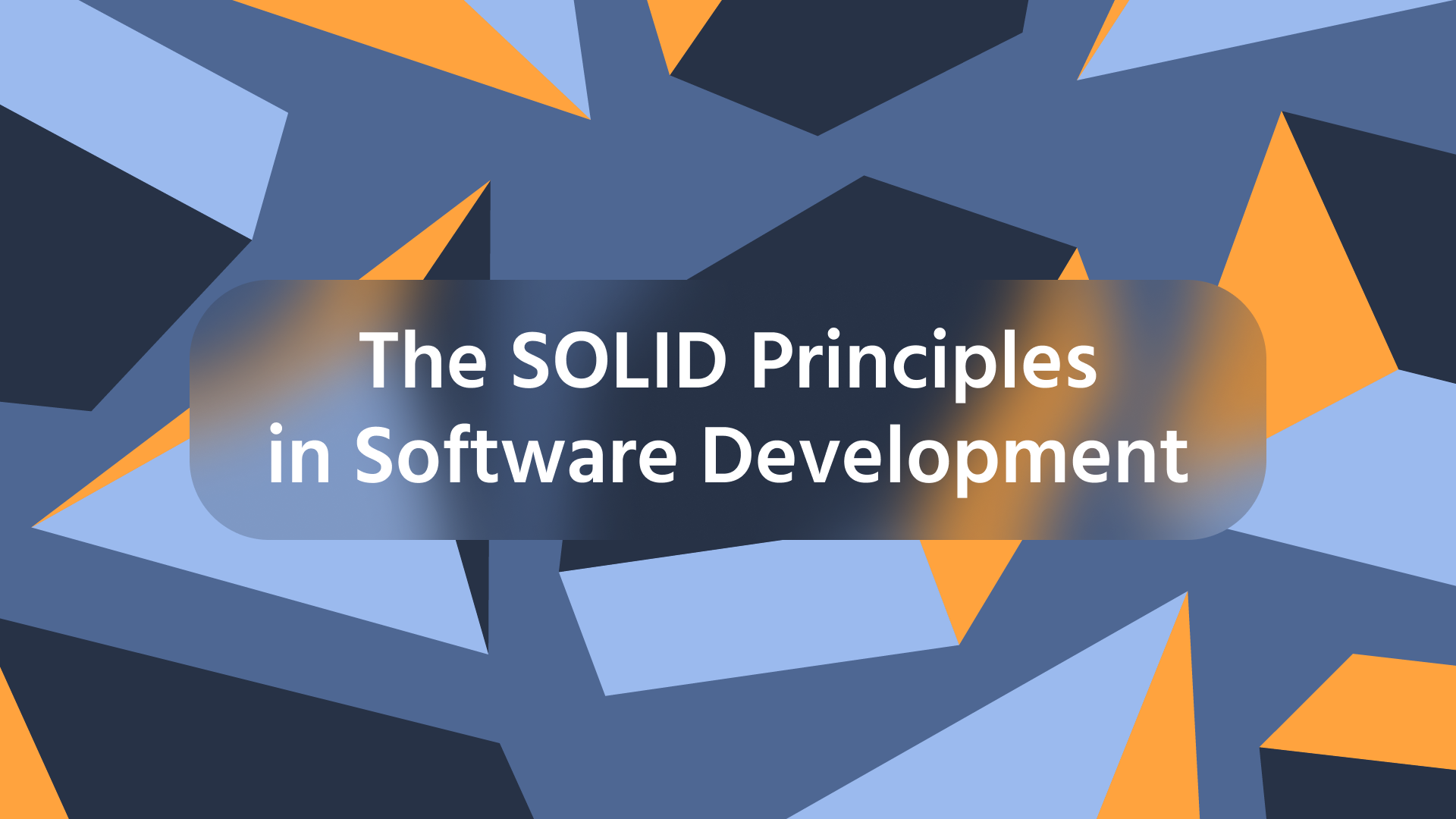
Match-case Operators in Python
Match-case Operators vs if-elif-else statements
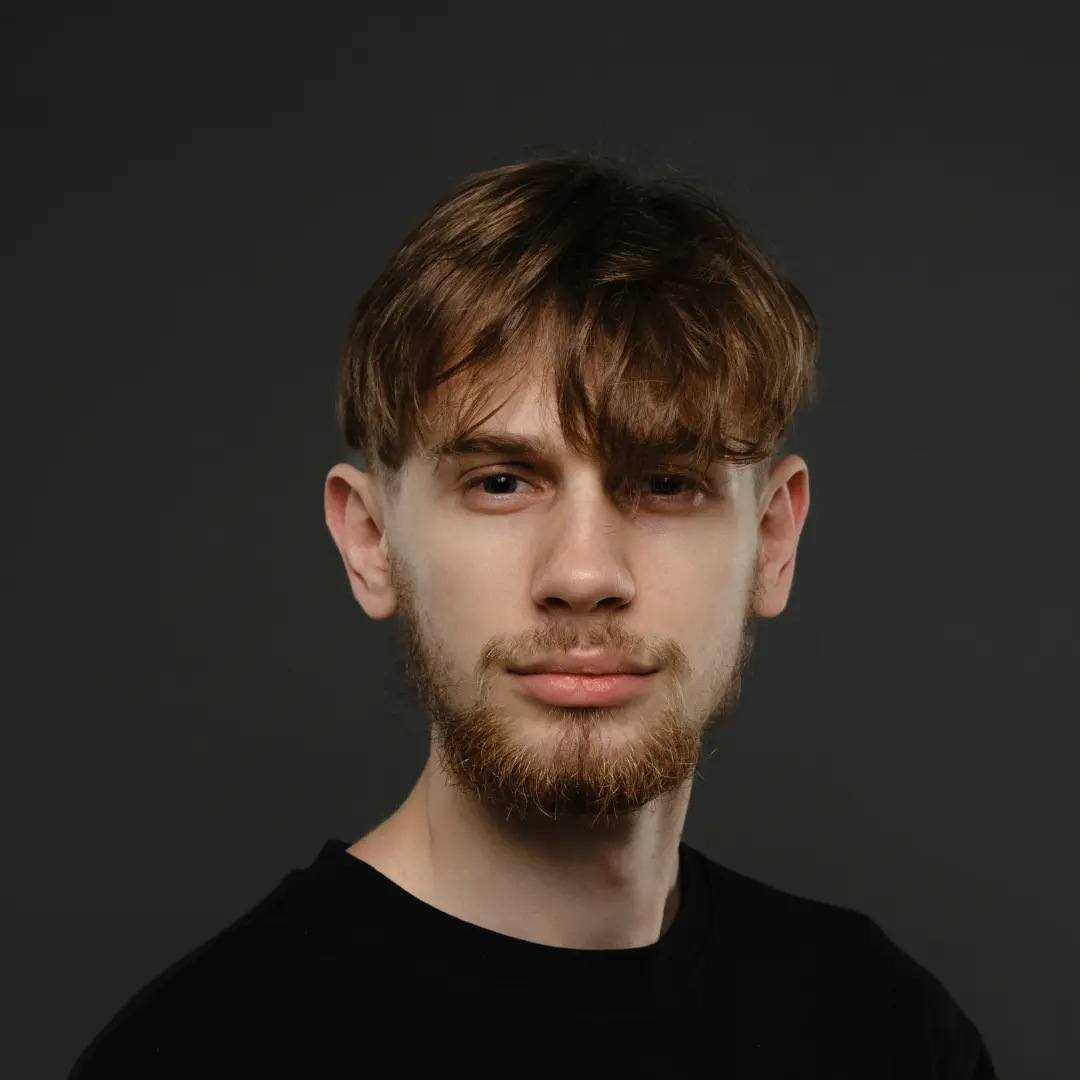
by Oleh Lohvyn
Backend Developer
Dec, 2023・6 min read
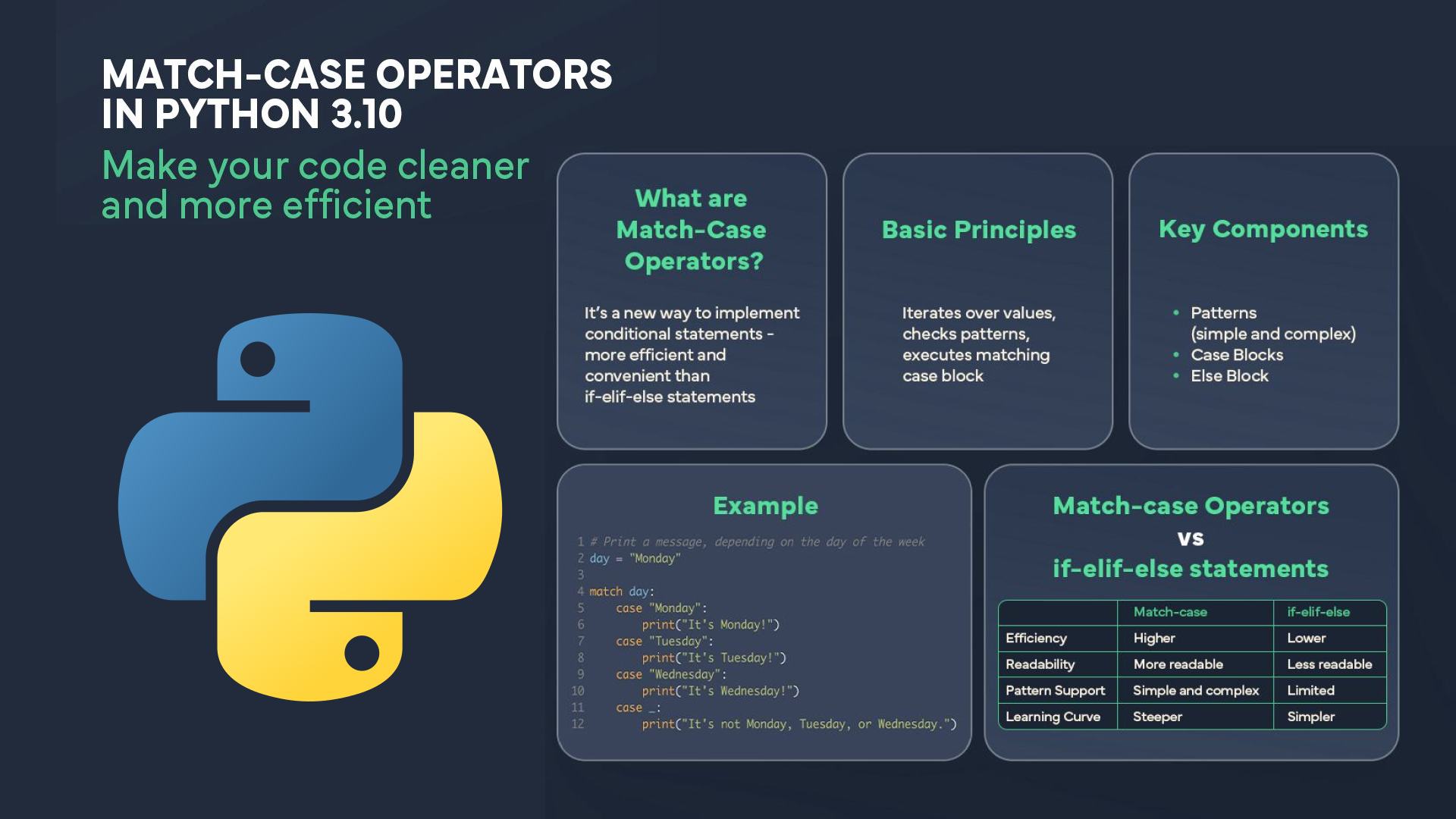
30 Python Project Ideas for Beginners
Python Project Ideas
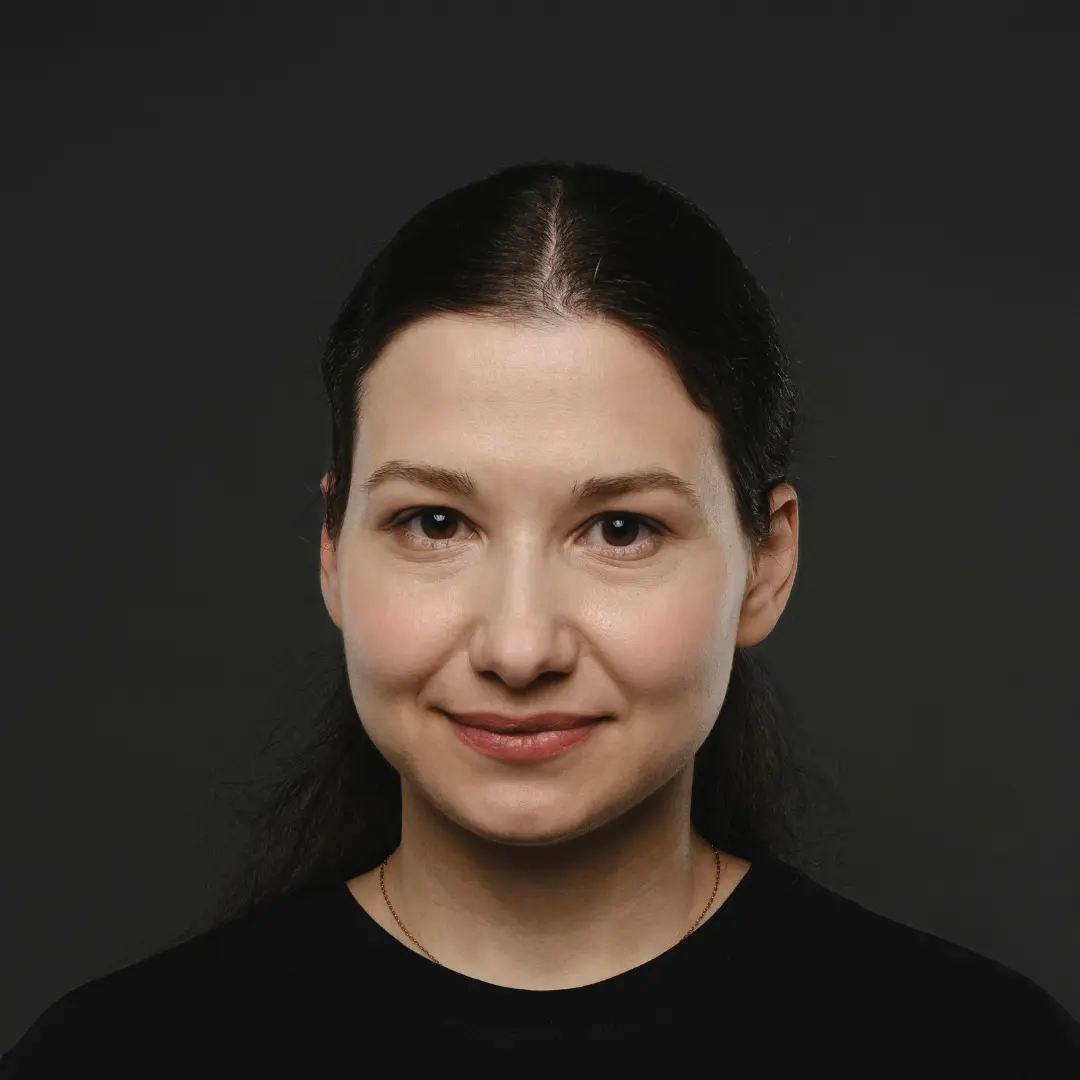
by Anastasiia Tsurkan
Backend Developer
Sep, 2024・14 min read
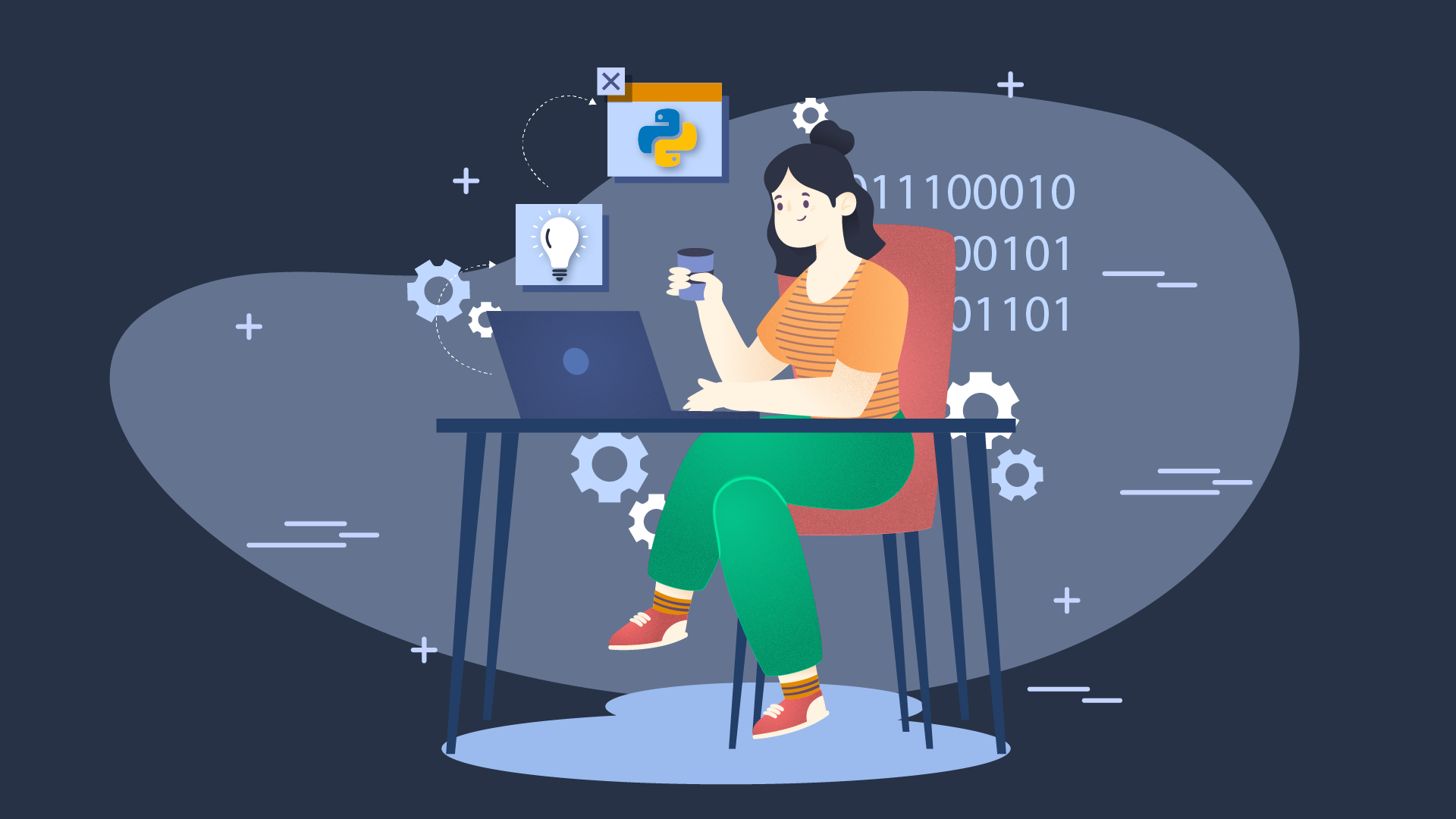
Conteúdo deste artigo