Курси по темі
Всі курсиБазовий
Python Data Structures
In this course, you'll get to know the fundamental data structures of the Python programming language. We'll explore utilizing Python's native data structures like lists, dictionaries, tuples, and sets to tackle different challenges.
Базовий
Introduction to Python
Python is an interpreted high-level general-purpose programming language. Unlike HTML, CSS, and JavaScript, which are primarily used for web development, Python is versatile and can be used in various fields, including software development, data science, and back-end development. In this course, you'll explore the core aspects of Python, and by the end, you'll be crafting your own functions!
Базовий
Python Functions Tutorial
You'll have a solid grasp of how important functions are in Python, no matter your field in computer science. We'll cover creating functions, defining arguments, handling return values, and exploring their use in Python.
Choosing the Best Python Compiler: A Comprehensive Guide
Python Compilers Overview
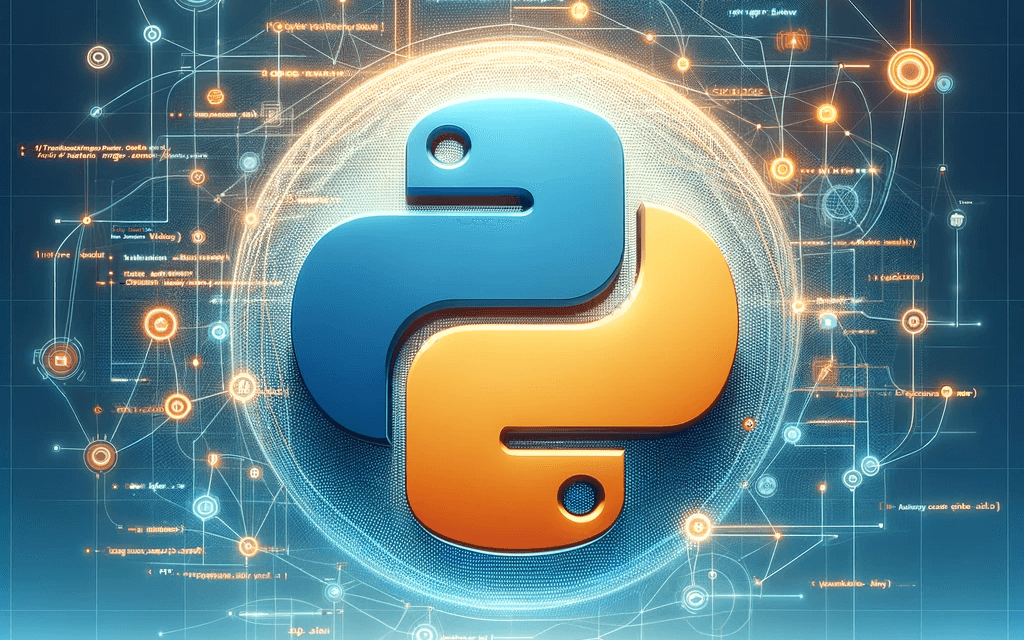
Introduction to Python Compilers
What is a Python Compiler?
Despite the common reference to Python compilers, it's important to note that Python primarily uses an interpreter, not a compiler in the traditional sense. This interpreter reads and executes the Python code directly, line by line, which differs from traditional compilers that convert code into machine language before execution. This process makes Python very flexible and easy to debug, but it can also affect execution speed.
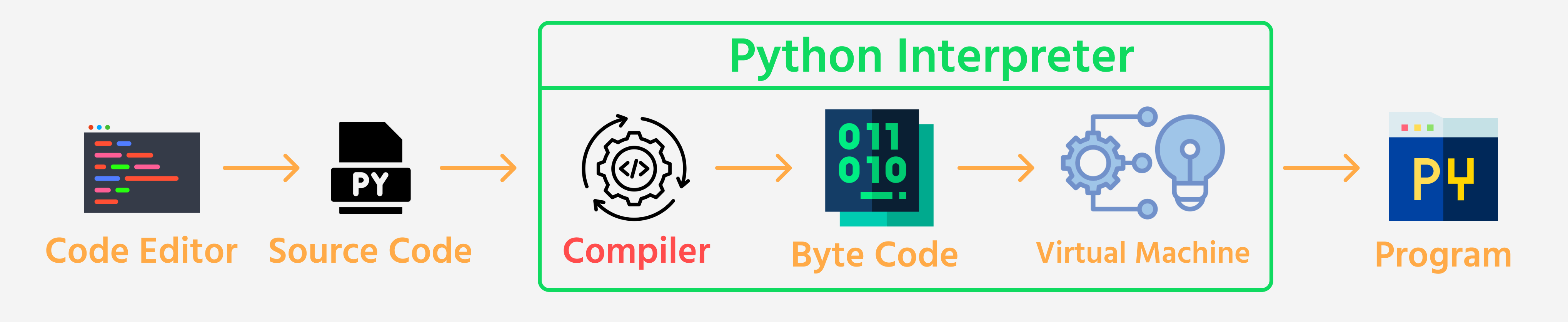
However, Python does include a compilation step where the interpreter converts the source code into bytecode, which is a lower-level, platform-independent representation. This bytecode is then executed by Python's virtual machine. Thus, when we refer to "Python compilers," we are often discussing implementations that enhance or replace this standard interpretative process to optimize performance or target different runtime environments.
Importance of Choosing the Right Compiler
Selecting the right Python compiler is crucial as it can significantly affect the performance, compatibility, and productivity of your development projects. It determines how efficiently your Python programs run and can influence your workflow and support for various Python features.
Top Python Compilers in 2024
When exploring Python development environments, several prominent Python compilers (or implementations) stand out. Each offers unique features and optimizations tailored to different types of projects and performance needs. Here’s a quick overview of the most widely-used Python compilers in the industry:
Overview of Python Compilers
1. CPython
CPython is the standard and most widely used implementation of the Python programming language. It is both an interpreter and a compiler, providing a solid balance between performance and ease of use. CPython translates Python code into bytecode before executing it, which allows for excellent integration with C extensions and libraries.
2. PyPy
PyPy is renowned for its performance improvements over CPython, thanks to its Just-In-Time (JIT) compiler. It aims to execute Python code faster by dynamically compiling Python bytecodes to machine code at runtime. PyPy is particularly effective for long-running processes due to its optimization capabilities.
3. Jython
Jython compiles Python code to Java bytecode, allowing Python programs to run on the Java Virtual Machine (JVM). This makes it a great choice for integrating Python with Java, accessing Java frameworks, and using Java libraries in Python programs.
4. IronPython
IronPython is tailored for compatibility with the .NET Framework, compiling Python code to .NET Common Intermediate Language (CIL). It enables developers to use Python scripts and libraries within the .NET framework and access .NET functionalities directly.
5. MicroPython
MicroPython is designed for use in microcontrollers and in constrained environments. It implements a subset of Python standards and includes specific libraries to optimize Python code to run on hardware with limited resources like RAM and processing power.
6. Stackless Python
Stackless Python enhances Python with support for microthreads, allowing for concurrent programming without traditional thread-related overhead. It's particularly useful for applications requiring a large number of simultaneously active tasks, like game development or network servers.
7. Brython
Brython (Browser Python) is an implementation of Python 3 for client-side web programming via a JavaScript framework. It allows Python code to run in browsers, utilizing web APIs as seamlessly as JavaScript.
8. Nuitka
Nuitka is a Python-to-C++ compiler that translates Python code into optimized C++ executables. It can significantly improve the performance of Python applications by generating faster code while maintaining compatibility with the vast majority of Python libraries.
9. Pyston
Pyston is a fork of CPython, with additional optimizations primarily aimed at improving the performance of large applications. It uses JIT techniques similar to PyPy but focuses on maintaining maximal compatibility with CPython.
Run Code from Your Browser - No Installation Required
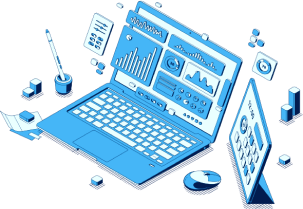
Factors to Consider When Choosing a Python Compiler
Performance and Speed
Evaluate the execution speed of compilers to ensure they meet the performance requirements of your project.
Compatibility with Python Versions
Ensure the compiler supports the Python version you are using, especially if your codebase uses newer language features.
Ease of Use and Learning Curve
Consider how easy it is to set up and use the compiler, especially if you are new to Python.
Community Support and Documentation
Robust community support and comprehensive documentation are essential for troubleshooting and learning how to use the compiler effectively.
Integration with Development Tools
Check compatibility with the tools and environments you’re already using for a smoother development experience.
Compiler | Performance and Speed | Compatibility with Python Versions | Ease of Use and Learning Curve | Integration with Development Tools |
---|---|---|---|---|
CPython | Standard performance, suitable for most applications | Full support for all Python versions | Very easy to set up and use | Excellent integration with all major Python tools |
PyPy | High performance with JIT compilation, best for long-running applications | High compatibility with CPython, some issues with C-extension modules | Moderate learning curve due to JIT setup | Good, but some issues with C-extension compatibility |
Jython | Good performance on Java platform, integrates with JVM optimizations | Supports Python 2.x and some 3.x features | Moderate, requires JVM knowledge | Seamless integration with Java tools and libraries |
IronPython | Good performance within .NET framework, leverages CLR optimizations | Supports Python 2.7 syntax and features | Easy for developers familiar with .NET | Integrates well with .NET ecosystem |
MicroPython | Optimized for microcontrollers, may not match desktop performance | Supports a subset of Python 3.x | Relatively easy for embedded systems developers | Specific to embedded systems and IoT devices |
How to Install and Set Up a Python Compiler
Here are a few examples of how to install different Python compilers:
Step-by-Step Installation Guide for CPython
To install CPython:
- Download the latest version of CPython from the official Python website.
- Run the installer. Make sure to check the box that says "Add Python to PATH" before clicking "Install Now."
- Verify the installation by opening your command line or terminal and typing
python --version
. This should display the Python version if it was installed correctly.
Setting Up PyPy for Performance Optimization
To set up PyPy for enhanced performance:
- Download the appropriate PyPy version for your operating system from PyPy’s official website.
- Extract the downloaded file to a directory of your choice.
- Add the PyPy directory to your system’s PATH environment variable to run PyPy from anywhere in your command line.
- Replace Python with PyPy in your project’s environment, or use a virtual environment specifically for PyPy with
pypy -m venv my-pypy-env
.
Using Jython for Java Integration
To integrate Jython with Java:
- Download the latest Jython installer from Jython's official website.
- Run the installer and follow the instructions, making sure to install it where you have Java installed.
- Set the
JYTHON_HOME
environment variable to point to your Jython installation directory. - Update your Java project settings to include Jython’s
jython.jar
in your project's classpath.
Common Issues and Troubleshooting
Compilation Errors and How to Fix Them
Compilation errors can occur due to syntax errors, incompatible types, or bugs in the compiler itself. To fix them:
- Check the error message for details about the line number and type of error.
- Review your code for any syntax issues or incorrect uses of variables.
- Ensure all modules and dependencies are installed correctly.
Performance Bottlenecks in Different Compilers
Performance bottlenecks can vary between compilers:
- CPython may experience bottlenecks with heavy I/O operations.
- PyPy might have issues with JIT compilation overhead initially, which may slow down the start-up performance but generally speeds up execution as more code is run.
- MicroPython could be constrained by the hardware’s limitations, affecting both speed and capability on microcontrollers.
- Jython might encounter bottlenecks when interfacing between Python code and Java libraries, especially with complex object conversions and Java Virtual Machine (JVM) overhead.
- IronPython often faces performance issues when integrating with .NET libraries due to the dynamic nature of Python and the static nature of the .NET framework, which can complicate interactions.
- Brython might experience slower performance in the web browser environment compared to more traditional JavaScript as it involves parsing Python code to JavaScript.
- Stackless Python could have bottlenecks related to tasklet switching, especially in programs with a high number of microthreads, affecting the overall concurrency model.
Each compiler has its unique strengths and weaknesses in terms of performance, and understanding these can help developers choose the right tool for their specific needs.
Compatibility Issues with Libraries
Not all Python libraries are compatible with every Python compiler:
- Check the library documentation for compatibility.
- Test libraries in your development environment before deployment.
- Use alternative libraries that support your chosen compiler, if necessary.
Start Learning Coding today and boost your Career Potential
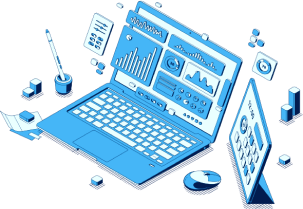
Conclusion: Making the Right Choice
Summary of Key Points
We have explored various Python compilers, each with unique features suitable for different types of projects. Understanding your project needs and the strengths of each compiler is crucial for selecting the right one.
Final Recommendations for Different Use Cases
For beginners and general programming: CPython.
For performance-sensitive applications: PyPy.
For integration with Java or .NET environments: Jython and IronPython respectively.
For embedded systems: MicroPython.
FAQs
Q: What is the best Python compiler for beginners?
A: Beginners may find CPython the best fit as it is the standard Python interpreter and is the easiest to set up and use. It provides clear and concise documentation which is crucial for learners.
Q: Which Python compiler is best overall?
A: The best compiler depends on specific needs, but PyPy stands out for performance due to its Just-In-Time compilation approach, making it ideal for speed-intensive applications.
Q: Are Python compilers free to use?
A: Most Python compilers, including CPython, PyPy, Jython, and IronPython, are open-source and free to use. Some specialized versions like MicroPython for embedded systems might have commercial versions.
Q: Where can I run Python code online?
A: Python code can be run online on platforms like Repl.it, Google Colab, and PythonAnywhere. These platforms support various Python compilers and offer integrated development environments.
Q: What are the differences between CPython and PyPy?
A: CPython is the standard Python implementation and runs Python scripts as they are. PyPy, however, incorporates a Just-In-Time compiler which makes it much faster for most Python code after some initial overhead.
Q: Can I use multiple Python compilers on the same machine?
A: Yes, you can install and use multiple Python compilers on the same machine, but it's essential to manage the environment paths correctly to avoid conflicts.
Q: How do I choose a compiler for data science projects?
A: For data science projects, compilers that can integrate seamlessly with data-oriented libraries (like NumPy, pandas) are crucial. CPython, with its extensive ecosystem, remains a preferred choice.
Q: What should I do if my code runs differently on different compilers?
A: Differences in code behavior across compilers are usually due to how each handles Python's dynamic features. It's recommended to test code across those compilers and adhere closely to standard Python practices to minimize discrepancies.
Курси по темі
Всі курсиБазовий
Python Data Structures
In this course, you'll get to know the fundamental data structures of the Python programming language. We'll explore utilizing Python's native data structures like lists, dictionaries, tuples, and sets to tackle different challenges.
Базовий
Introduction to Python
Python is an interpreted high-level general-purpose programming language. Unlike HTML, CSS, and JavaScript, which are primarily used for web development, Python is versatile and can be used in various fields, including software development, data science, and back-end development. In this course, you'll explore the core aspects of Python, and by the end, you'll be crafting your own functions!
Базовий
Python Functions Tutorial
You'll have a solid grasp of how important functions are in Python, no matter your field in computer science. We'll cover creating functions, defining arguments, handling return values, and exploring their use in Python.
Best Laptops for Coding in 2024
Top Picks and Key Features for the Best Coding Laptops
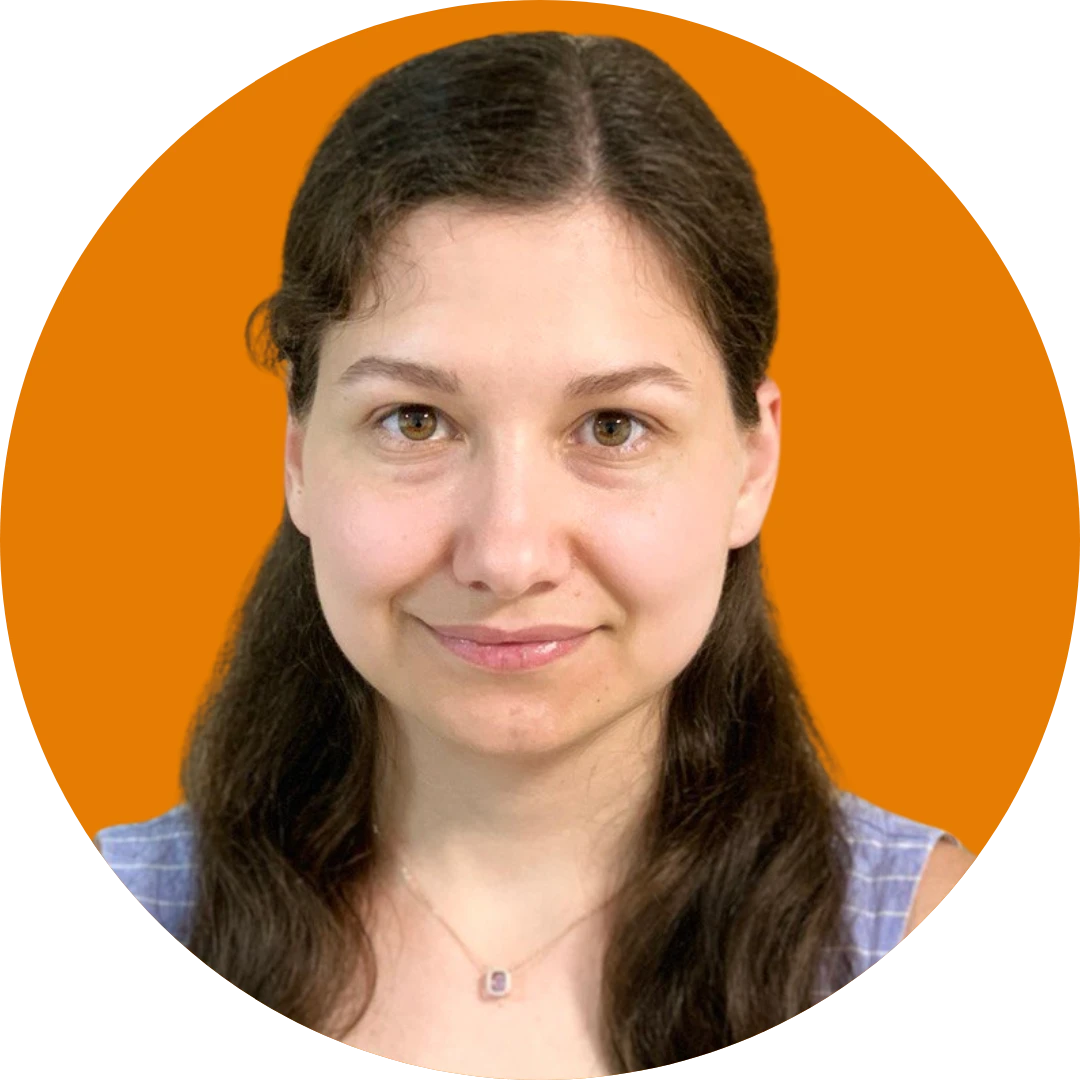
by Anastasiia Tsurkan
Backend Developer
Aug, 2024・12 min read
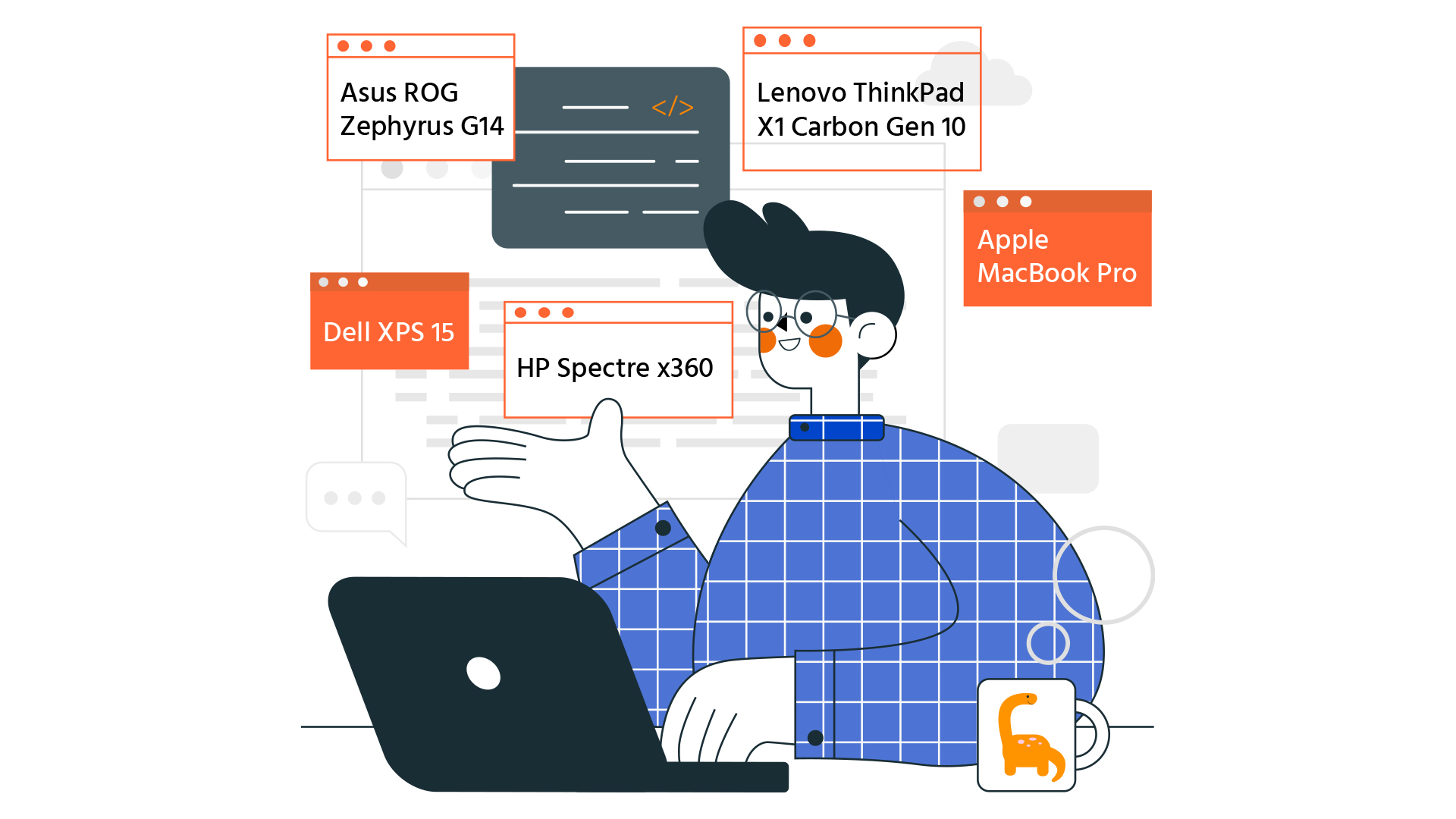
Top 10 Popular Applications Built with Python
Exploring the Versatility and Impact of Python in Modern Applications
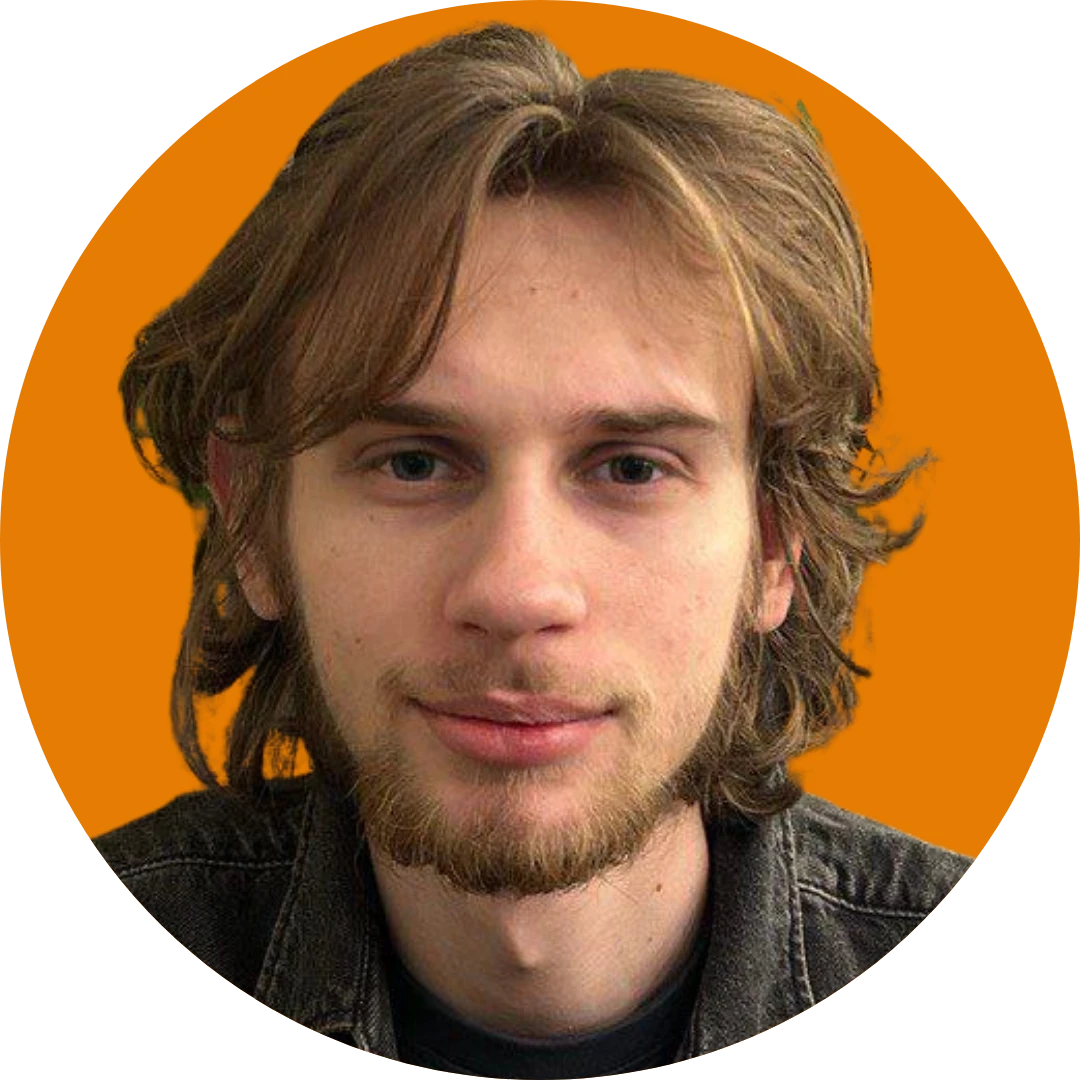
by Oleh Lohvyn
Backend Developer
Oct, 2024・3 min read
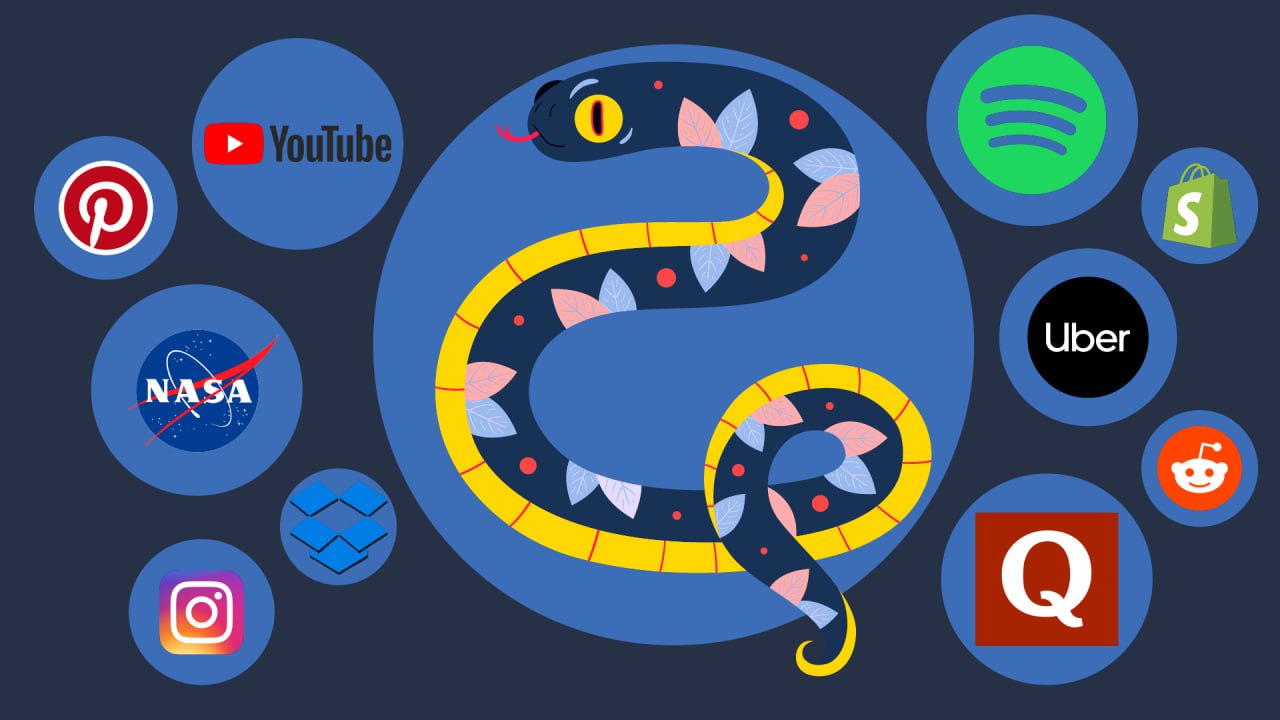
Top 5 IT Profession Challenges and How to Overcome Them
Navigating Though the Complexities of The Job
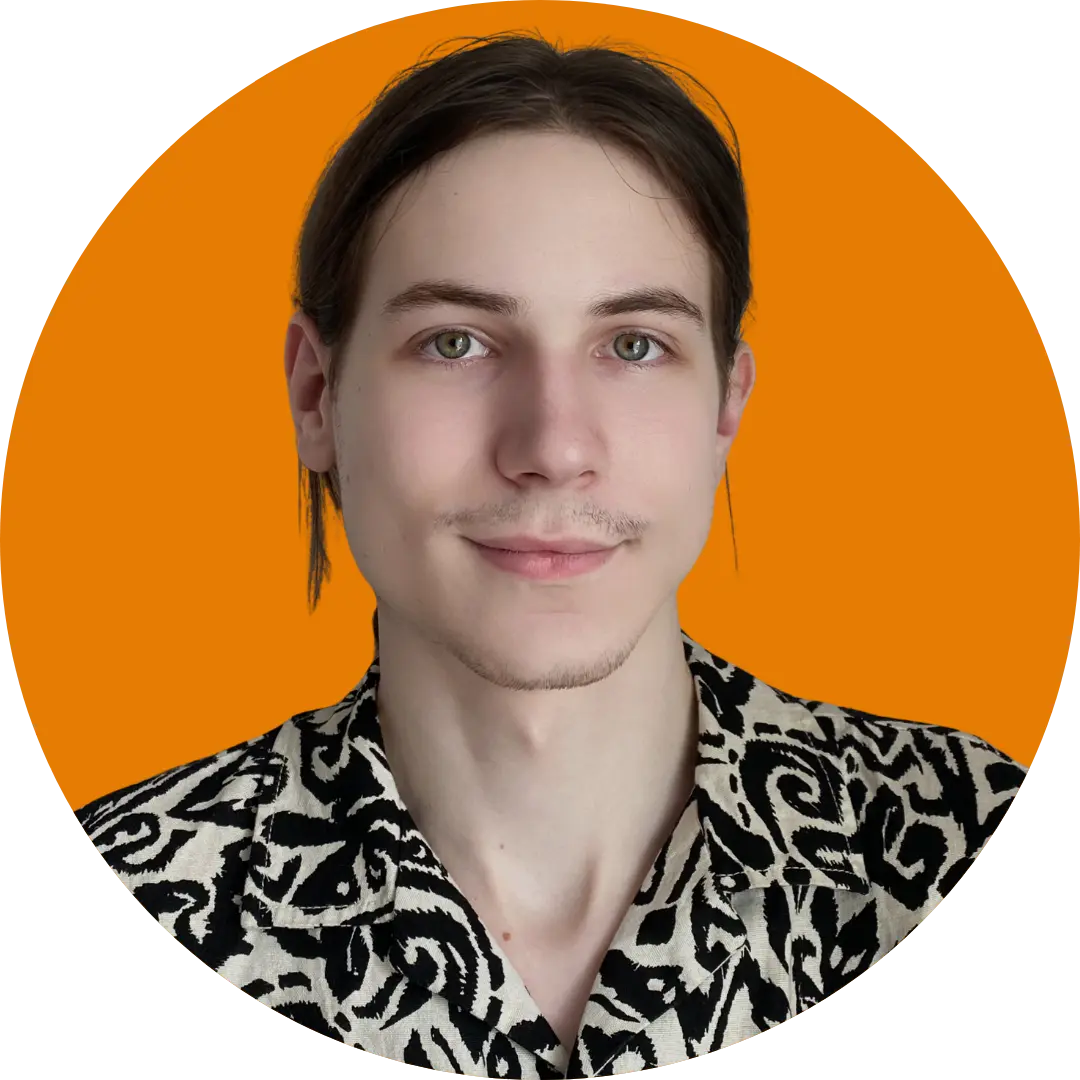
by Ihor Gudzyk
C++ Developer
Dec, 2023・5 min read
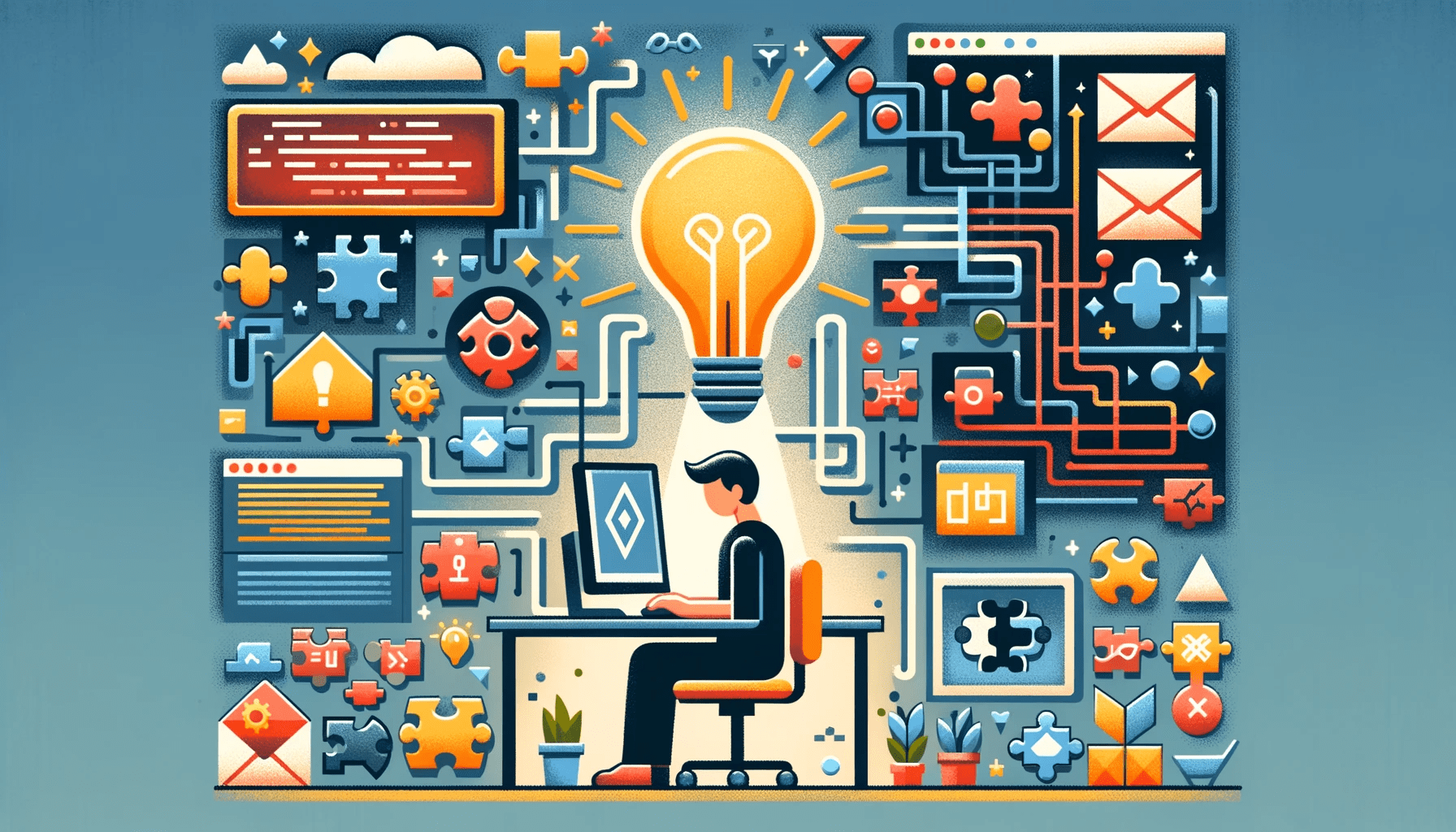
Зміст